/*
** $Id: helloworld.c,v 1.1.1.1 2003/06/03 06:57:15 weiym Exp $
**
** Listing 2.1
**
** helloworld.c: Sample program for MiniGUI Programming Guide
** The first MiniGUI Application
**
** Copyright (C) 2003 Feynman Software.
**
** License: GPL
*/
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <minigui/common.h>
#include <minigui/minigui.h>
#include <minigui/gdi.h>
#include <minigui/window.h>
#include <minigui/control.h>
int map[32][24]={0};
static int TestMainWinProc(HWND hWnd, int message, WPARAM wParam, LPARAM lParam)
{
HDC hdc;
static int x=0,y=0;
static int x_pos,x_p;
static int y_pos,y_p;
static int s1=0,s2=0,s3=0,s4=0,p=1,t=0,i;
static int s5=0,s6=0,s7=0,s8=0;
static BITMAP bitmap;
int m,n;
switch (message) {
case MSG_PAINT:
hdc = BeginPaint (hWnd);
LoadBitmap(hdc,&bitmap, "logo256.bmp");
FillBoxWithBitmap (hdc, 0, 0, 640, 480, &bitmap);
for(;x<24;x++)
{
MoveTo(hdc,0,20*x+20);
LineTo(hdc,640,20*x+20);
for(;y<32;y++)
{
MoveTo(hdc,20*y+20,0);
LineTo(hdc,20*y+20,480);
}
}
EndPaint (hWnd, hdc);
return 0;
// break;
case MSG_LBUTTONDOWN:
hdc = GetClientDC (hWnd);
//key_flags = (DWORD)wParam;
x_pos = LOSWORD (lParam);
y_pos = HISWORD (lParam);
x_p=(int)((x_pos/20.0)+0.5)*20;
y_p=(int)((y_pos/20.0)+0.5)*20;
if(p%2==0)
{
hdc = BeginPaint (hWnd);
SetBrushColor (hdc, PIXEL_black);
FillCircle (hdc, x_p, y_p, 8);
EndPaint (hWnd, hdc);
//if(map[x_p][y_p]==2)
// break;
map[x_p/20][y_p/20]=1;
for(i=0;i<5;i++)
{
if (map[x_p/20+i][y_p/20]==1)
s1++;
}
for(i=0;i<5;i++)
{
if (map[x_p/20-i-1][y_p/20]==1)
s1++;
if(s1>4)
{
s1=0;
t=1;
}
}
s1=0;
for(i=0;i<5;i++)
{
if (map[x_p/20][y_p/20+i]==1)
s2++;
}
for(i=0;i<5;i++)
{
if (map[x_p/20][y_p/20-i-1]==1)
s2++;
if(s2>4)
{
s2=0;
t=1;
}
}
s2=0;
for(i=0;i<5;i++)
{
if (map[x_p/20+i][y_p/20+i]==1)
s3++;
}
for(i=0;i<5;i++)
{
if (map[x_p/20-i-1][y_p/20-i-1]==1)
s3++;
if(s3>4)
{
s3=0;
t=1;
}
}
s3=0;
for(i=0;i<5;i++)
{
if (map[x_p/20+i][y_p/20-i]==1)
s4++;
}
for(i=0;i<5;i++)
{
if (map[x_p/20-i-1][y_p/20+i+1]==1)
s4++;
if(s4>4)
{
s4=0;
t=1;
}
}
s4=0;
if(t==1)
{
t=0;
printf("black win\n");
MessageBox (hWnd, "黑棋获胜", "result", MB_OK | MB_ICONINFORMATION);
return 0;
//InvalidateRect(hWnd,NULL,TRUE);
// MessageBox(hWnd,"black win","result",MB_OK | MB_ICONINFORMATION);
}
}
else if((p%2)!=0)
{
hdc = BeginPaint (hWnd);
SetBrushColor (hdc, PIXEL_lightwhite);
FillCircle (hdc, x_p, y_p, 8);
EndPaint (hWnd, hdc);
/*if(map[x_p][y_p]==1)
break;*/
map[x_p/20][y_p/20]=2;
/*for(m=0;m<24;m++)
{
for(n=0;n<32;n++)
{
printf("%d ",map[n][m]);
if(n==31)
printf("\n");
}
}*/
for(i=0;i<5;i++)
{
if (map[x_p/20+i][y_p/20]==2)
s5++;
}
for(i=0;i<5;i++)
{
if (map[x_p/20-i-1][y_p/20]==2)
s5++;
if(s5>4)
{
s5=0;
t=2;
}
}
s5=0;
for(i=0;i<5;i++)
{
if (map[x_p/20][y_p/20+i]==2)
s6++;
}
for(i=0;i<5;i++)
{
if (map[x_p/20][y_p/20-i-1]==2)
s6++;
if(s6>4)
{
s6=0;
t=2;
}
}
s6=0;
for(i=0;i<5;i++)
{
if (map[x_p/20+i][y_p/20+i]==2)
s7++;
}
for(i=0;i<5;i++)
{
if (map[x_p/20-i-1][y_p/20-i-1]==2)
s7++;
if(s7>4)
{
s7=0;
t=2;
}
}
s7=0;
for(i=0;i<5;i++)
{
if (map[x_p/20+i][y_p/20-i]==2)
s8++;
}
for(i=0;i<5;i++)
{
if (map[x_p/20-i-1][y_p/20+i+1]==2)
s8++;
if(s8>4)
{
s8=0;
t=2;
}
}
s8=0;
if(t==2)
{
t=0;
printf("lightwhite win\n");
MessageBox (hWnd, "白棋获胜", "result", MB_OK | MB_ICONINFORMATION);
// InvalidateRect(hWnd,NULL,TRUE);
// MessageBox(hWnd,"lightwhite win","result",MB_OK | MB_ICONINFORMATION);
}
}
p++;
//break;
return 0;
case MSG_CLOSE:
UnloadBitmap (&bitmap);
DestroyMainWindow (hWnd);
PostQuitMessage (hWnd);
return 0;
}
return DefaultMainWinProc(hWnd, message, wParam, lParam);
}
void InitCreateInfo(PMAINWINCREATE pCreateInfo)
{
//pCreateInfo->dwStyle = WS_THICKFRAME;
pCreateInfo->dwStyle =WS_VISIBLE | WS_BORDER | WS_CAPTION;
pCreateInfo->spCaption = "The first main window" ;
pCreateInfo->hMenu = 0;
pCreateInfo->hCursor = GetSystemCursor(0);
pCreateInfo->hIcon = 0;
pCreateInfo->MainWindowProc = TestMainWinProc;
pCreateInfo->lx = 0;
pCreateInfo->ty = 0;
pCreateInfo->rx = 640;
pCreateInfo->by = 480;
pCreateInfo->iBkColor = COLOR_lightwhite;
pCreateInfo->dwAddData = 0;
pCreateInfo->hHosting = HWND_DESKTOP;
}
//int GUIAPI DialogBoxIndirectParam
void* TestWindowMain(void* data)
{
MSG Msg;
MAINWINCREATE CreateInfo;
HWND hMainWnd;
InitCreateInfo(&CreateInfo);
if( !(hMainWnd = CreateMainWindow(&CreateInfo)) )
return NULL;
ShowWindow(hMainWnd, SW_SHOWNORMAL);
while( GetMessage(&Msg, hMainWnd) ) {
DispatchMessage(&Msg);
}
MainWindowThreadCleanup(hMainWnd);
return NULL;
}
int MiniGUIMain(int argc, const char* argv[])
{
pthread_t thread;
CreateThreadForMainWindow(&thread, NULL, TestWindowMain, 0);
return 0;
}
#ifndef _LITE_VERSION
#include <minigui/dti.c>
#endif
wuziqi.zip_makingynd_minigui vc
版权申诉
9 浏览量
2022-09-23
03:25:09
上传
评论
收藏 2.36MB ZIP 举报

我虽横行却不霸道
- 粉丝: 72
- 资源: 1万+
最新资源
- ZEND解密dezender12
- sony 索尼IMX334摄像头模组电路板AD版硬件PCB图(6层板).zip
- 基于flask和echarts融合交易策略的bitfinex可视化微服务.zip
- 包含了wvp-assist.tar wvp-talk.tar zlmediakit.tar .
- 3r4efgh53wgrf43tw
- 2024新版Java基础从入门到精通全套视频+资料下载
- Spring AI大模型视频教程+ChatGPT视频教程+OpenAI大模型视频教程(资料+视频教程)
- ABB工业机器人教程PDF版本
- 123321123323211
- 三相桥式全桥整流电路MATALB Simulink仿真文件
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


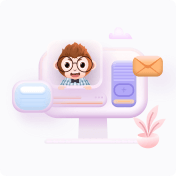