package com.android.ide.eclipse.adt.internal.sdk;
import com.android.ddmlib.IDevice;
import com.android.ide.eclipse.adt.AdtPlugin;
import com.android.ide.eclipse.adt.internal.project.AndroidClasspathContainerInitializer;
import com.android.ide.eclipse.adt.internal.project.BaseProjectHelper;
import com.android.ide.eclipse.adt.internal.project.ProjectHelper;
import com.android.ide.eclipse.adt.internal.resources.manager.GlobalProjectMonitor;
import com.android.ide.eclipse.adt.internal.resources.manager.GlobalProjectMonitor.IFileListener;
import com.android.ide.eclipse.adt.internal.resources.manager.GlobalProjectMonitor.IProjectListener;
import com.android.ide.eclipse.adt.internal.resources.manager.GlobalProjectMonitor.IResourceEventListener;
import com.android.ide.eclipse.adt.internal.sdk.AndroidTargetData.LayoutBridge;
import com.android.ide.eclipse.adt.internal.sdk.ProjectState.LibraryDifference;
import com.android.ide.eclipse.adt.internal.sdk.ProjectState.LibraryState;
import com.android.prefs.AndroidLocation.AndroidLocationException;
import com.android.sdklib.AndroidVersion;
import com.android.sdklib.IAndroidTarget;
import com.android.sdklib.ISdkLog;
import com.android.sdklib.SdkConstants;
import com.android.sdklib.SdkManager;
import com.android.sdklib.internal.avd.AvdManager;
import com.android.sdklib.internal.project.ProjectProperties;
import com.android.sdklib.internal.project.ProjectPropertiesWorkingCopy;
import com.android.sdklib.internal.project.ProjectProperties.PropertyType;
import com.android.sdklib.io.StreamException;
import org.eclipse.core.resources.IFile;
import org.eclipse.core.resources.IFolder;
import org.eclipse.core.resources.IMarkerDelta;
import org.eclipse.core.resources.IPathVariableManager;
import org.eclipse.core.resources.IProject;
import org.eclipse.core.resources.IProjectDescription;
import org.eclipse.core.resources.IResource;
import org.eclipse.core.resources.IResourceDelta;
import org.eclipse.core.resources.IWorkspaceRoot;
import org.eclipse.core.resources.IncrementalProjectBuilder;
import org.eclipse.core.resources.ResourcesPlugin;
import org.eclipse.core.runtime.CoreException;
import org.eclipse.core.runtime.IPath;
import org.eclipse.core.runtime.IProgressMonitor;
import org.eclipse.core.runtime.IStatus;
import org.eclipse.core.runtime.Path;
import org.eclipse.core.runtime.Status;
import org.eclipse.core.runtime.jobs.Job;
import org.eclipse.jdt.core.IClasspathEntry;
import org.eclipse.jdt.core.IJavaProject;
import org.eclipse.jdt.core.JavaCore;
import java.io.File;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.Map.Entry;
/**
* Central point to load, manipulate and deal with the Android SDK. Only one SDK can be used
* at the same time.
*
* To start using an SDK, call {@link #loadSdk(String)} which returns the instance of
* the Sdk object.
*
* To get the list of platforms or add-ons present in the SDK, call {@link #getTargets()}.
*/
public final class Sdk {
private static final String PROP_LIBRARY = "_library"; //$NON-NLS-1$
private static final String PROP_LIBRARY_NAME = "_library_name"; //$NON-NLS-1$
public static final String CREATOR_ADT = "ADT"; //$NON-NLS-1$
public static final String PROP_CREATOR = "_creator"; //$NON-NLS-1$
private final static Object sLock = new Object();
private static Sdk sCurrentSdk = null;
/**
* Map associating {@link IProject} and their state {@link ProjectState}.
* <p/>This <b>MUST NOT</b> be accessed directly. Instead use {@link #getProjectState(IProject)}.
*/
private final static HashMap<IProject, ProjectState> sProjectStateMap =
new HashMap<IProject, ProjectState>();
/**
* Data bundled using during the load of Target data.
* <p/>This contains the {@link LoadStatus} and a list of projects that attempted
* to compile before the loading was finished. Those projects will be recompiled
* at the end of the loading.
*/
private final static class TargetLoadBundle {
LoadStatus status;
final HashSet<IJavaProject> projecsToReload = new HashSet<IJavaProject>();
}
private final SdkManager mManager;
private final AvdManager mAvdManager;
/** Map associating an {@link IAndroidTarget} to an {@link AndroidTargetData} */
private final HashMap<IAndroidTarget, AndroidTargetData> mTargetDataMap =
new HashMap<IAndroidTarget, AndroidTargetData>();
/** Map associating an {@link IAndroidTarget} and its {@link TargetLoadBundle}. */
private final HashMap<IAndroidTarget, TargetLoadBundle> mTargetDataStatusMap =
new HashMap<IAndroidTarget, TargetLoadBundle>();
private final String mDocBaseUrl;
private final LayoutDeviceManager mLayoutDeviceManager = new LayoutDeviceManager();
/**
* Classes implementing this interface will receive notification when targets are changed.
*/
public interface ITargetChangeListener {
/**
* Sent when project has its target changed.
*/
void onProjectTargetChange(IProject changedProject);
/**
* Called when the targets are loaded (either the SDK finished loading when Eclipse starts,
* or the SDK is changed).
*/
void onTargetLoaded(IAndroidTarget target);
/**
* Called when the base content of the SDK is parsed.
*/
void onSdkLoaded();
}
/**
* Basic abstract implementation of the ITargetChangeListener for the case where both
* {@link #onProjectTargetChange(IProject)} and {@link #onTargetLoaded(IAndroidTarget)}
* use the same code based on a simple test requiring to know the current IProject.
*/
public static abstract class TargetChangeListener implements ITargetChangeListener {
/**
* Returns the {@link IProject} associated with the listener.
*/
public abstract IProject getProject();
/**
* Called when the listener needs to take action on the event. This is only called
* if {@link #getProject()} and the {@link IAndroidTarget} associated with the project
* match the values received in {@link #onProjectTargetChange(IProject)} and
* {@link #onTargetLoaded(IAndroidTarget)}.
*/
public abstract void reload();
public void onProjectTargetChange(IProject changedProject) {
if (changedProject != null && changedProject.equals(getProject())) {
reload();
}
}
public void onTargetLoaded(IAndroidTarget target) {
IProject project = getProject();
if (target != null && target.equals(Sdk.getCurrent().getTarget(project))) {
reload();
}
}
public void onSdkLoaded() {
// do nothing;
}
}
/**
* Returns the lock object used to synchronize all operations dealing with SDK, targets and
* projects.
*/
public static final Object getLock() {
return sLock;
}
/**
* Loads an SDK and returns an {@link Sdk} object if success.
* <p/>If the SDK failed to load, it displays an error to the user.
* @param sdkLocation the OS path to the SDK.
*/
public static Sdk loadSdk(String sdkLocation) {
synchronized (sLock) {
if (sCurrentSdk != null) {
sCurrentSdk.dispose();
sCurrentSdk = null;
}
final ArrayList<String> logMessages = new ArrayList<String>();
ISdkLog log = new ISdkLog() {
public void error(Throwable throwable, String errorFormat, Object... arg) {
if (errorFormat != null) {
logMessages.add(String.format("

我虽横行却不霸道
- 粉丝: 98
- 资源: 1万+
最新资源
- 新瑞能源(储能系统解决方案提供商,东莞市新瑞能源技术有限公司)创投信息
- 《Cocos 游戏开发从入门到精通全攻略》,为你开启游戏开发的大门
- 调制信号的连续小波变换CWT时频谱图分析:二次线性Chirp调频信号、蝙蝠回声定位信号及神户地震数据的时频定位能力展示(MATLAB r2021b),调制信号的连续小波变 CWT时频谱图 程序运行环境
- 行者AI(游戏全产业链AI赋能平台,成都潜在人工智能科技有限公司)创投信息
- Java毕业设计-springboot-vue-大学生创新创业项目管理系统(源码+sql脚本+29页零基础部署图文详解+30页论文+环境工具+教程+视频+模板).zip
- Java毕业设计-springboot-vue-船运物流管理系统(源码+sql脚本+29页零基础部署图文详解+23页论文+环境工具+教程+视频+模板).zip
- Java毕业设计-springboot-vue-大学生计算机基础网络教学系统(源码+sql脚本+29页零基础部署图文详解+27页论文+环境工具+教程+视频+模板).zip
- 云骥智行(L4自动驾驶解决方案提供商,上海云骥智行智能科技有限公司)创投信息
- Java毕业设计-springboot-vue-大学生平时成绩量化管理系统(源码+sql脚本+29页零基础部署图文详解+33页论文+环境工具+教程+视频+模板).zip
- Java毕业设计-springboot-vue-大学生在线租房平台(源码+sql脚本+29页零基础部署图文详解+33页论文+环境工具+教程+视频+模板).zip
- Java毕业设计-springboot-vue-大学生就业服务平台(源码+sql脚本+29页零基础部署图文详解+40页论文+环境工具+教程+视频+模板).zip
- STM32 F103系列芯片OTA远程升级:WiFi连接下的可靠固件更新流程 升级过程包括HTTP GET指令获取服务器固件信息、版本对比、下载地址写入flash及重启更新等步骤 升级文件需进行CRC
- Java毕业设计-springboot-vue-当代中国获奖知名作家信息管理系统(源码+sql脚本+29页零基础部署图文详解+30页论文+环境工具+教程+视频+模板).zip
- Java毕业设计-springboot-vue-房屋租赁管理系统(源码+sql脚本+29页零基础部署图文详解+32页论文+环境工具+教程+视频+模板).zip
- Java毕业设计-springboot-vue-扶贫助农系统(源码+sql脚本+29页零基础部署图文详解+32页论文+环境工具+教程+视频+模板).zip
- H桥驱动circuitjs1软件仿真
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


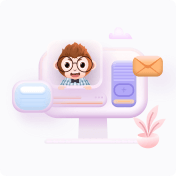