#include "DSP280x_Device.h"
#include "DSP280x_Examples.h"
#include "stdio.h"
#include "IQmathLib.h"
#include "string.h"
#include "adf.h"
#include "monitor.h"
#include "rs485.h"
#include "math.h"
/***************************************************************/
/********************Loop 2 parameters*************************/
#include "Hilbert.h" //hilbert transform
#include "LPF.h" //low pass filter
#define B_L 25 //hilbert filter order
#define Sample_len 128 //watch length of sample data
#define Sample_len1 1 // sample length in fact
#define filter_delay ((B_L-1)/2+1) //another path of hilbert transform delay
#define LPF_L 21 //lpf filter order
//#define level 0x800
#define ref_dc_rem 0x00 //DC_remove of ref
#define err_dc_rem 0x01 //DC_remove of err
#define I_lpf 0x00 //LPF of I
#define Q_lpf 0x01 //LPF of Q
#define DC_mask 128 // average length for DC remove
#define level 0x10000
#define count_mask 128
/*************************************************************/
#define SGB_INIT 192
/************************Loop1 parameter*************************/
#define N_LOOP1 10
#define POUT_OFF 510
#define POUT_OFF_SGB 400
/*********************************************/
//DAC configuration
#define CHIP1 0x00
#define CHIP2 0x01
#define CH_DPA_BIAS 0x01
#define CH_HPA_BIAS 0x01
#define CH_EPA_BIAS 0x05
#define CH_SGB_ATT 0x03
#define CH_MATT 0x04
#define CH_MPHS1 0x02
#define CH_MPHS2 0x06
#define CH_EATT 0x00
#define CH_EPHS1 0x00
#define CH_EPHS2 0x02
#define ADC_MODCLK 0x3
#define PIN_ALC_OFFSET 1
//board1
//#define PIN_ALC_OFFSET_DAC -40
//board2
#define PIN_ALC_OFFSET_DAC 0
#pragma CODE_SECTION(adc_isr, "ramfuncs");
#pragma CODE_SECTION(sample_loop1, "ramfuncs");
long ipcb[N_LOOP1];
Uint16 hw_DisCurrent;
const Uint16 ahw_FCurrent[11] = {2400,2160,1701,1259,973,817,698,596,551,528,490};
const Uint16 loop1_opt[8] = {900, 940, 1000, 1020, 1092, 1152, 1180, 1340};
// -20, -19, -18, -17, -16, -15, -14, -13
//board 1
/*const unsigned char aby_ALC[27] = {100,153,198,240,282,322,362,400,438,472,506,538,568,597,623,648,671,693,713,733,
// -3 -2 -1 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
750,766,782,796,810,824,838};*/
// 17 18 19 20 21 22 23
//board2
const unsigned char aby_ALC[23] = {60,115,155,200,250,298,345,390,433,475,513,551,587,620,651,680,707,733,755,777,
// -3 -2 -1 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
797,816,833};
/*********************************************/
//Loop2 global parameter
int16 AD_ref=0;
int16 AD_err=0;
int16 DC_r=0;
int32 DC_r_sum=0;
int16 DC_count_ref=0;
int16 DC_e=0;
int32 DC_e_sum=0;
int16 DC_count_err=0;
int16 ref_signal=0;
int16 err_signal=0;
int32 level_1=0;
int16 switch_1=0;
int32 amp_I=328;
int32 phase_Q=384;
int32 amp_I1=0;
int32 phase_Q1=0;
int32 op_I=0;
int32 op_Q=0;
int32 op_I_pre=0;
int32 op_Q_pre=0;
int32 op_I_pre1=0;
int32 op_Q_pre1=0;
int32 op_I1=0;
int32 op_Q1=0;
Uint16 sample_err_signal[Sample_len1]={0};
Uint16 sample_ref_signal[Sample_len1]={0};
int16 Hilbert_delay[filter_delay]={0};
int16 Hilbert_buffer[B_L]={0};
int16 LPF_buffer_I[LPF_L]={0};
int16 LPF_buffer_Q[LPF_L]={0};
int16 LPF_ref[LPF_L]={0};
int16 LPF_err[LPF_L]={0};
//int16 LPF_buffer_D[LPF_L]={0};
int16 non_hilbert=0;
int16 finished_hilbert=0;
int32 yn=0;
int32 temp;
//int16 err_delay[filter_delay]={0};
//int16 I_pre;
//int16 Q_pre;
int16 I_module=0;
int16 Q_module=0;
int16 I_lpf_later=0;
int16 Q_lpf_later=0;
int32 loop2_level=0;
int16 Gain_I_low=1;
int16 Gain_I_high=1;
int16 Gain_Q_low=1;
int16 Gain_Q_high=1;
int16 calculate_count=0;
int16 ref_index=0;
int16 sample_switch=0x00;
//int16 clear_buffer_count=0;
int16 calculate_count_1=0;
//int16 calculate_start=0;
//int16 select=0;
int16 sum_count=0;
int16 s_count=0;
/************************************************/
// see the Conversion results
int16 ref[Sample_len]={0};
int16 err[Sample_len]={0};
int16 DC_ref[Sample_len]={0};
int16 DC_err[Sample_len]={0};
int16 ref_hilberted[Sample_len]={0};
int16 ref_non_hilberted[Sample_len]={0};
//int16 MIX_I[Sample_len]={0};
//int16 MIX_Q[Sample_len]={0};
int16 LPF_I[Sample_len]={0};
int16 LPF_Q[Sample_len]={0};
int32 Level_1[Sample_len]={0};
int32 level_2[Sample_len]={0};
int16 level_3[Sample_len]={0};
/************************************************/
//Loop1 global parameter
Uint16 com_flag;
int16 hw_PIN_Offset;
Uint16 tune;
int32 Pout1_l, Pout1, Pout1_opt;
int32 Pout2_l, Pout2, Pout2_opt;
int32 m_att_opt, m_phs2_opt, e_att_opt, e_phs2_opt;
Uint16 step_matt, step_mphs2;
Uint16 dir;
Uint16 error;
Uint16 sum_pin, sum_bpout, sum_rf_onoff;
float Pin, Fpout, Bpout,Pin_ALC,Swave,Swave_Out;
float Pin_l;
Uint16 sum_hpa_temp, sum_pd_err;
Uint16 sum_ld1, sum_ld2, sum_ld3, sum_fpout;
Uint16 opposite_flag;
int16 sgb;
float Pin_threshold,Pin_max;
float temperature,temperature_max;
Uint16 PinOverFlag,TempOverFlag,SwaveOverFlag;
Uint16 sgb_adjust, sgb_adjust_l;
int32 e_phs2_delta, e_att_delta;
Uint16 temp_change, temp_change_l;
unsigned int e_att_Buff = 0,m_att_Buff = 0,sgb_att_Buff = 0;
/* PA parameter define*/
int32 sgb_att = 0x000;
int32 m_att = 0x278;
int32 m_phs2 = 0x128;
int32 e_att = 304;
int32 e_phs2 = 237;
int32 bias_dpa = 0x000;
int32 bias_epa = 0x000;
int32 bias_hpa = 0x9e4;
int32 m_phs1 = 0x3df;
int32 e_phs1 = 0x000;
struct{
unsigned SWITCH_FLAG:1;
unsigned SWICON_PC:1;
}work = {0,0};
//********************************************************fan.xu*********************
//******************************sample data num*************************
Uint16 sum_AD0,sum_AD1,sum_AD2,sum_AD3,sum_AD4,sum_AD5,sum_AD6,sum_AD7,sum_AD8,sum_AD9,sum_AD10,sum_AD11,sum_AD12,sum_AD13,sum_AD14,sum_AD15;
//**********************************************************************
//******************************sample conn1****************************
Uint16 CONN1_AA0,CONN1_AA5,CONN1_AA6,CONN1_AA7,CONN1_AB0,CONN1_AB1,CONN1_AB2,CONN1_AB3,CONN1_AB4,CONN1_AB5,CONN1_AB6,CONN1_AB7;
//******************************sample conn2,3,4************************
Uint16 CONN2_Vin1,CONN2_Vin2,CONN2_Vin3,CONN2_Vin4,CONN2_Vin5,CONN2_Vin6,CONN2_Vin7,CONN2_Vin8,CONN2_Vin9,CONN2_Vin10,CONN2_Vin11,CONN2_Vin12,CONN3_Vin13,CONN3_Vin14,CONN3_Vin15,CONN3_Vin16,CONN3_Vin17,CONN3_Vin18,CONN3_Vin19,CONN3_Vin20,CONN3_Vin21,CONN3_Vin22,CONN3_Vin23,CONN3_Vin24,CONN4_Vin25,CONN4_Vin26,CONN4_Vin27,CONN4_Vin28,CONN4_Vin29,CONN4_Vin30,CONN4_Vin31,CONN4_Vin32;
//***********************************************************************************
Uint16 sample_AD0[SAMP_REP];
Uint16 sample_AD1[SAMP_REP];
Uint16 sample_AD2[SAMP_REP];
Uint16 sample_AD3[SAMP_REP];
Uint16 sample_AD4[SAMP_REP];
Uint16 sample_AD5[SAMP_REP];
Uint16 sample_AD6[SAMP_REP];
Uint16 sample_AD7[SAMP_REP];
Uint16 sample_AD8[SAMP_REP];
Uint16 sample_AD9[SAMP_REP];
Uint16 sample_AD10[SAMP_REP];
Uint16 sample_AD11[SAMP_REP];
Uint16 sample_AD12[SAMP_REP];
Uint16 sample_AD13[SAMP_REP];
Uint16 sample_AD14[SAMP_REP];
Uint16 sample_AD15[SAMP_REP];
//******************************************************
interrupt void adc_isr(void);
interrupt void scia_isr(void);
interrupt void scia_tsr(void);
int16 DC_remove(int16 ch,int16 dc_in);
void Hilbert_transform(int16 hilbert_in);
void IQ_module(void);
int16 LPF_transform(int16 ch,int16 lpf_in);
void sample_ref(Uint16 samp_len);
void calculate_level(void);
void calculate_out(void);
void pre_process_loop2(void);
void process_loop2(void);
void Board_Init(void);
void Sample(Uint16 samp_rep);
void DACL_Init();
void DAC_write(int32 value, int32 H, int32 L, Uint16 chip, Uint16 ch);
void Bias_Init(void);
void ad_config();
没有合适的资源?快使用搜索试试~ 我知道了~
board1_3_bak.rar_TMSF2802_dsp采样
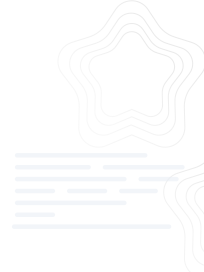
共149个文件
h:37个
c:24个
gel:20个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 134 浏览量
2022-09-21
18:14:48
上传
评论
收藏 1.68MB RAR 举报
温馨提示
TI TMSF2802 DSP实现AD采样与串口通信
资源推荐
资源详情
资源评论
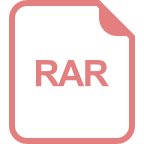
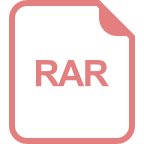
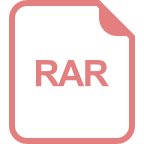
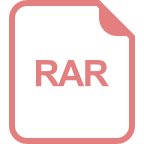
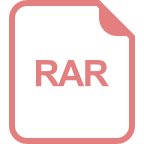
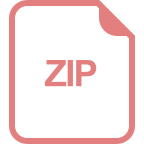
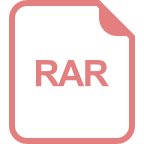
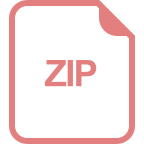
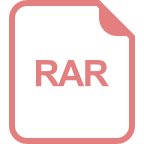
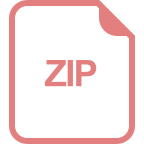
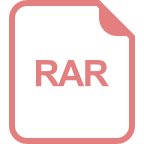
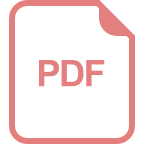
收起资源包目录

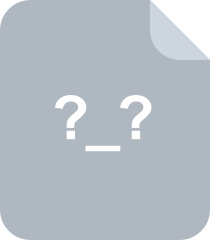
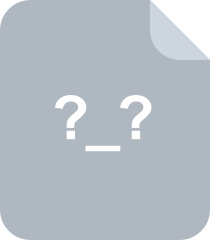
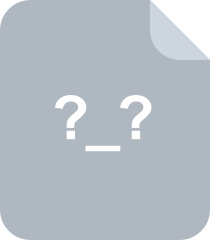
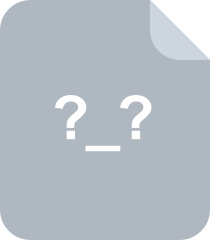
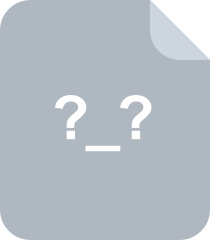
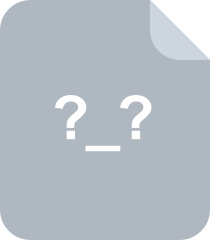
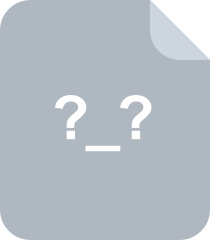
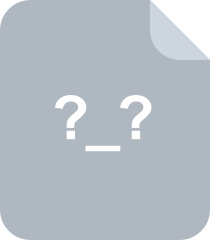
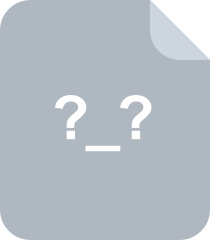
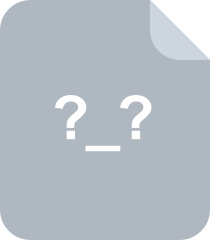
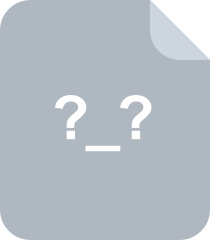
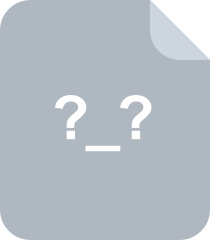
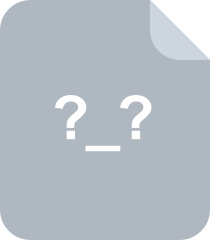
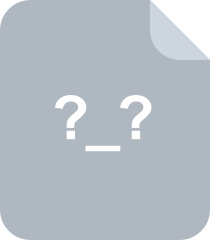
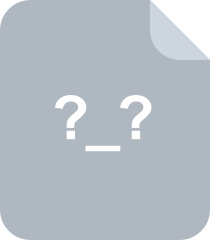
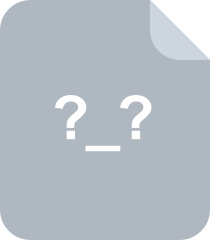
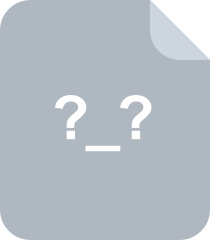
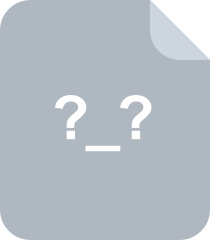
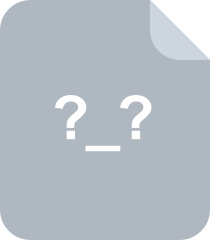
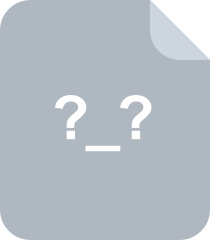
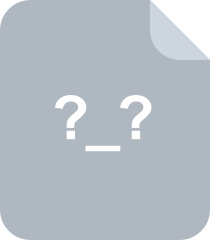
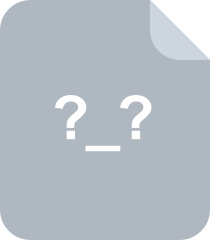
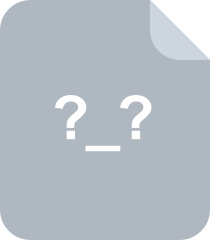
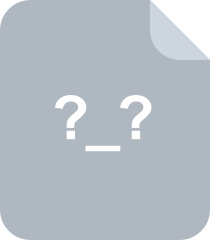
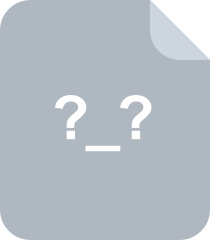
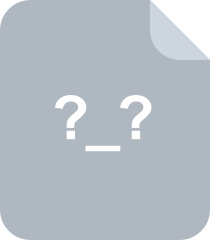
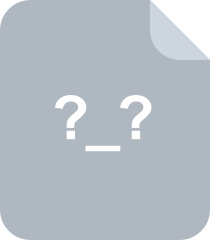
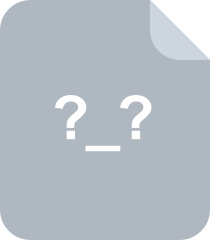
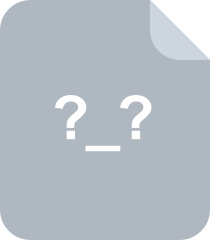
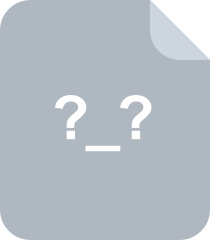
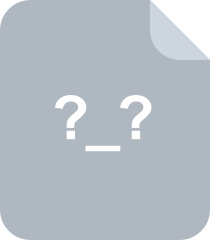
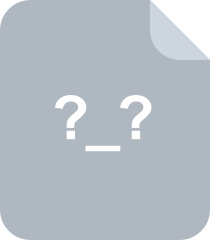
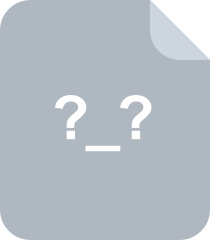
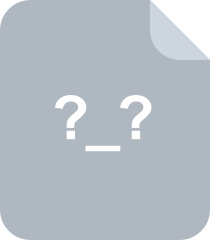
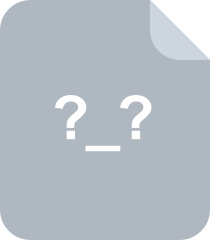
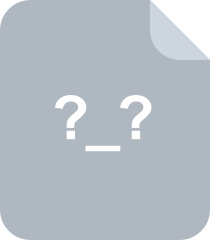
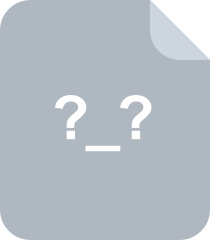
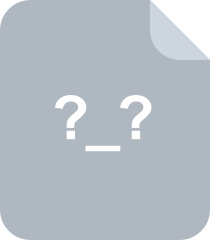
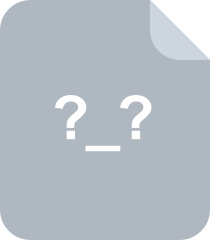
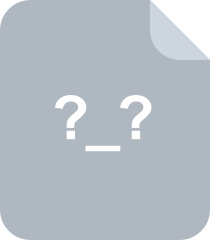
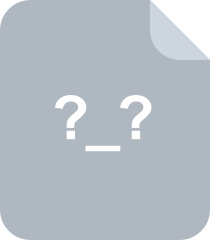
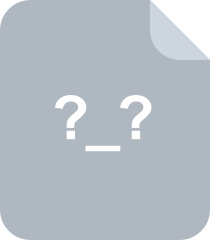
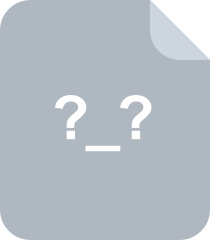
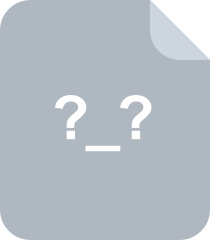
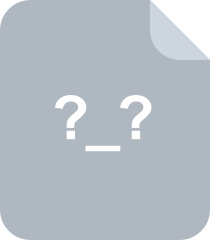
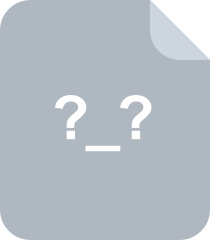
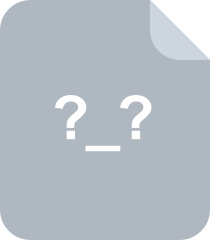
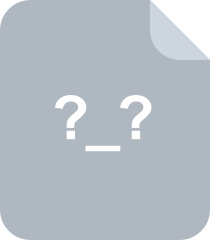
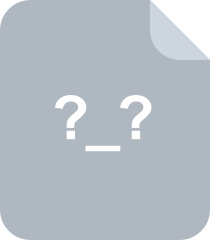
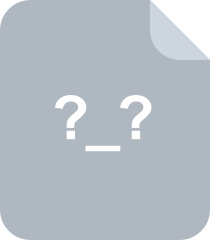
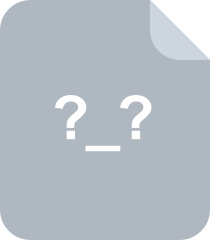
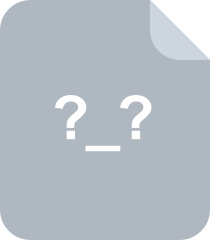
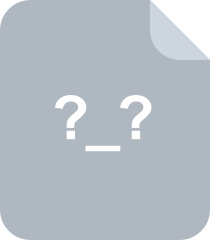
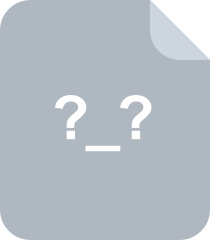
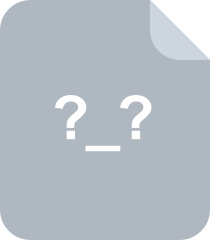
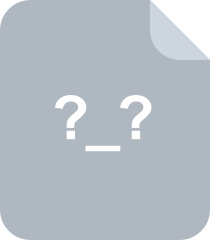
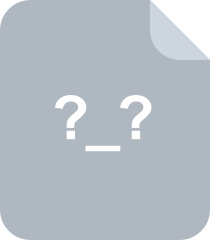
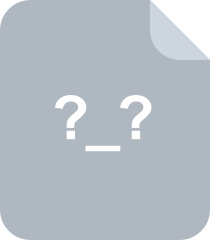
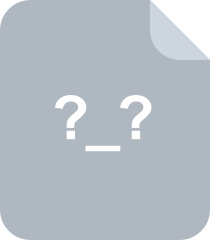
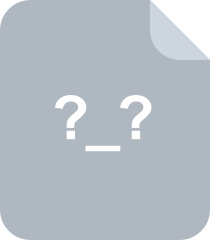
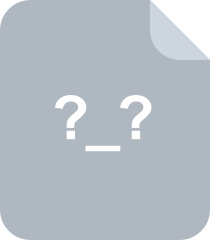
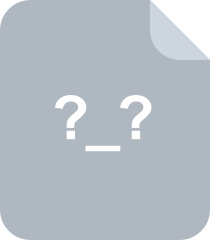
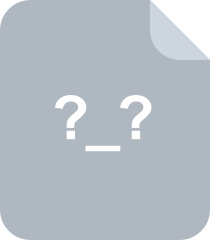
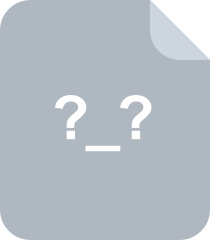
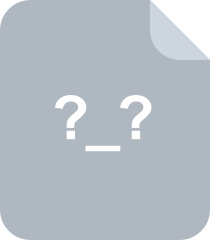
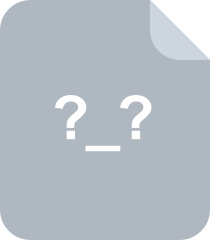
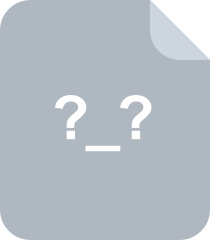
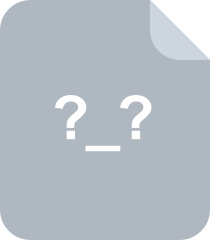
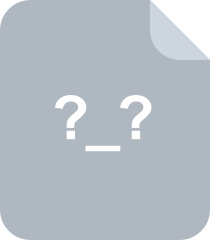
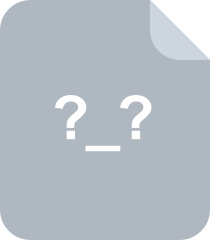
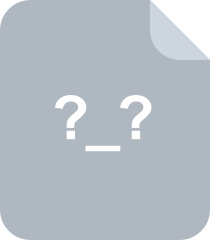
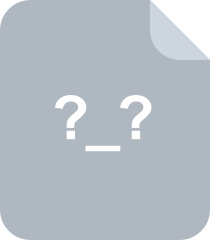
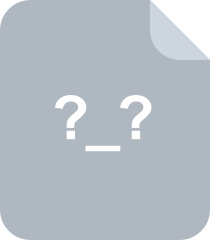
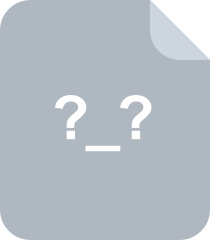
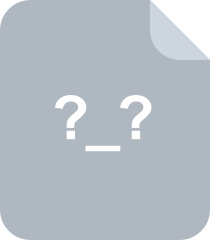
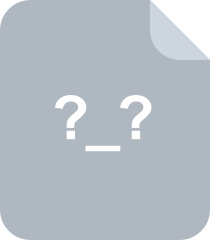
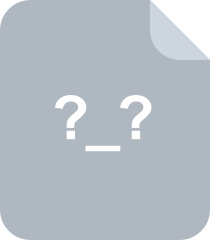
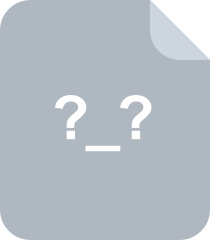
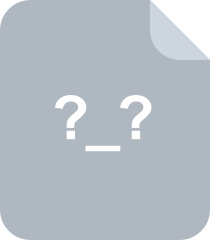
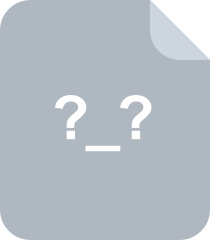
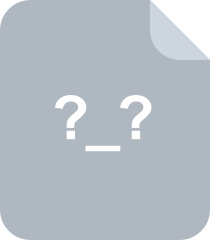
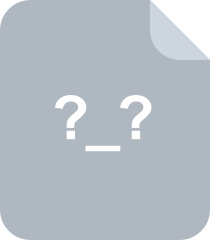
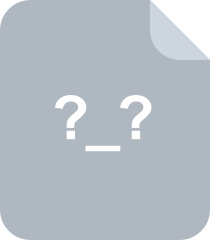
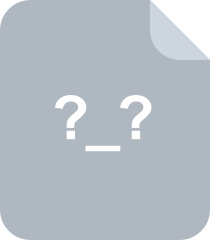
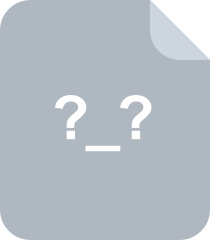
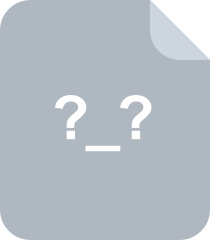
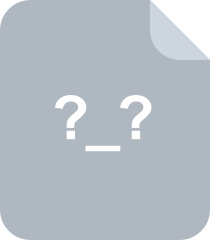
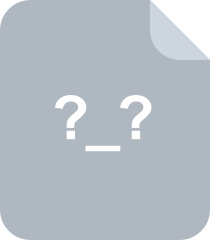
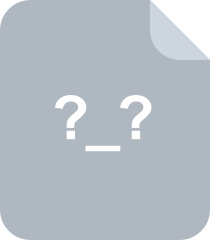
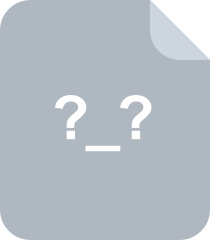
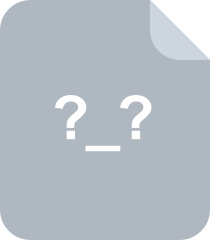
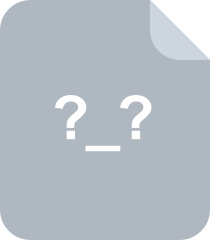
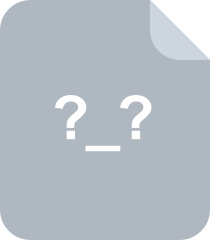
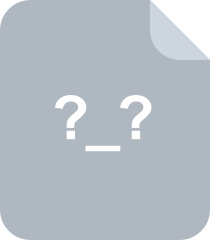
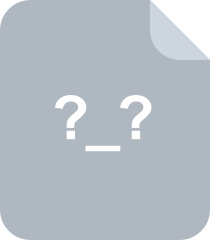
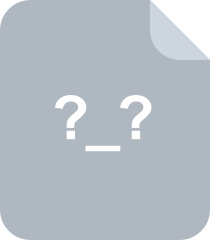
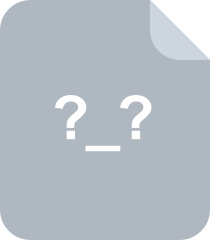
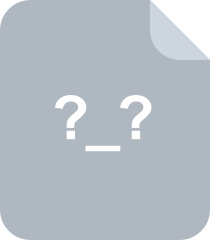
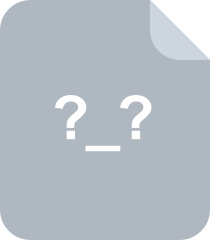
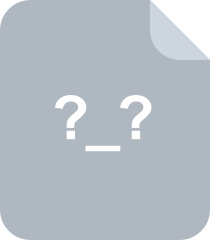
共 149 条
- 1
- 2
资源评论
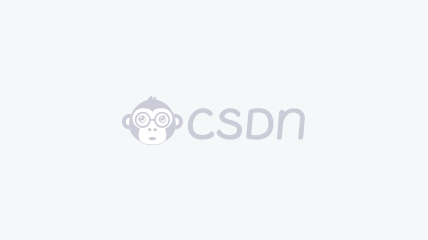

我虽横行却不霸道
- 粉丝: 75
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

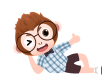
安全验证
文档复制为VIP权益,开通VIP直接复制
