/* Copyright (c) 2006, Spruha Electronic Systems
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in
the documentation and/or other materials provided with the
distribution.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
POSSIBILITY OF SUCH DAMAGE. */
/* This Program is to be used with AVRTRN Microcontroller Kit from Spruha Electronic Systems
Program Tests the ADC Channel e.g. Channel 1. PA0 PIN 40 of ATMega16.
Connect the Shorting Link near PORTA Header. This Connects Pin 40 PA0 to the Multiturn Potentiometer.
Connect PORTB to LCDPORT by 10 core Cable. This connects the LCD Display to PORTB.
Connect the PC COM Port to the RS232 PORT of the Kit by the Cable provided.
The include files LCD.C, UART.C, DIGITAL_DISPLAY.c are in the directory \include.
The Program measures the Voltage by converting the Analog Voltage to Digital. The ADC here is 10 Bit.
The ADC Count is converted to give Display in Volts. The Internal Reference of 5v is used.
The Output is Displayed on the LCD. If the Digital Display ( Optional Peripheral ) is present the
voltage is Displayed on the Digital Display Card. The Voltage value is also sent to PC through RS232 PORT.
The Hex Count is Displayed on the Top Line and the corrosponding calculated Voltage is displayed on
second line.
*/
#include <avr/io.h>
#include <avr/signal.h>
#include <avr/interrupt.h>
#include "include\uart.c"
#include "include\lcd.c"
#include "include\digital_display.c"
#define TOLERANCE 4//0//2//10
int mod(int m);
void ADC_Display(unsigned char adc_pin);
void dis_num(int p,char* dis);
void dis_num_lcd(int p,char* lcddis);
void dis_hex(int p,char* lcddis);
void delay1(int );
void delay(uint32_t);
int main(void)
{
char lcddis[7];
char dis[5]="0000";
unsigned short full=55;
unsigned short old=44,lastlast=99;
unsigned char adc_pin=0;
int k;
long int temp;
union _adc_data {
unsigned char dt[2];
unsigned short data;
} adc_data;
dis_string(dis);//Display Data 0000 on the Digital Display
lcd_init();//initialize the LCD Display Unit
uart_init();//Initialize the UART. Set Baud Rate
uart_puts("SPRUHA ADC TEST");//Send String to RS232 Port
uart_putc(13);
uart_putc(10);
ADMUX = (0x40 + adc_pin);//Set Channel 1 of ADC i.e. PA0
while(1)
{
ADCSR = 0xC0; //Control Word And SOC (using divisor of 2)
loop_until_bit_is_set ( ADCSR, ADIF ); //Wait For EOC or while(ADIF==0)
adc_data.dt[0] = ADCL;//full 8bit Read Lower Byte
adc_data.dt[1] = ADCH; //lsb 2 Read Higher Byte
full=adc_data.data & 0x03ff;
if(mod(full-old)>TOLERANCE)//Two samples are compared and displayed only if the Difference is
old=full; //more than TOLERANCE. Here TOLERANCE set as 4. This is to avoid Flickering on the Display
else if(mod(lastlast-full)>TOLERANCE)
{
lastlast=full;
dis_hex(full,lcddis);
lcd_curpos(0);
lcd_string("ADC HEX = ");
lcd_curpos(10);
lcd_string(lcddis);//Displays Hex Count on the 1st Line of LCD
uart_puts(lcddis);//sends the Hex Count on RS232 UART
temp=(long)full*500;//Count Conversion to volt value. 1023=5v
temp/=1023;
k=(int)temp;
dis_num(k,dis);
dis_num_lcd(k,lcddis);
lcd_curpos2L(0);
lcd_string("ADC VOLT: ");
lcd_curpos2L(10);
lcd_string(lcddis);//Displays Voltage on the 2nd Line of LCD
uart_puts(lcddis);//sends the Voltage value on RS232 UART
uart_putc(13);
uart_putc(10);
}
}
}
/**************************************************************************************************
* ADMUX - Multiplexer Selection Register
* 7 6 5 4 3 2 1 0
* REFS1 REFS0 ADLAR MUX4 MUX3 MUX2 MUX1 MUX0
*
* REFS1 REFS0
* 0 0 - AREF, Internal Vref Turned off
* 0 1 - AVCC with external capacitor at AREF pin
* 1 0 - Reserved
* 1 1 - Internal 2.56V voltage reference with external capacitor at AREF pin
*
* ADLAR - Affects the presentation of the ADC conversion result 0=Right Adjusted, 1=Left Adjusted
* MUX4 to MUX0 - Analog channel and Gain selection bits
* Our value is 0100XXXX i.e. 0x4X
**************************************************************************************************
* ADCSR - ADC Control & Status Register
* 7 6 5 4 3 2 1 0
* ADEN ADSC ADATE ADIF ADIE ADSP2 ADSP1 ADSP0
*
* ADEN - ADC Enable
* ADSC - ADC Start Of Conversion
* ADATE - ADC Auto Trigger Enable (on +ive edge)
* ADIF - ADC End Of Conversion (set to High on EOC)
* ADIE - ADC Interrupt Enable
* ADSP2 to ADSP0 - Division Factor between XTAL and internal clock of ADC
* :. our contorl word is 11000000 i.e. 0xC0
**************************************************************************************************/
void delay(uint32_t us)
{
while ( us ) us--;
}
void delay1(int num1)
{
int i;
for(i=0;i<num1;i++)
delay(28000);
}
/* in dis_num you send the integer and the pointer to the string i.e. dis
the dis_string(dis); will then send the number to the digital display.
This subroutine converts the Number into string with ASCCI value
*/
void dis_num(int p,char* dis)
{
int i,j=1000;
for(i=0;i<4;i++)
{
dis[i]=(p/j)%10+'0';
j/=10;
}
dis[4]=0;
dis_string(dis);
}
/* in dis_num_lcd you send the integer and the pointer to the string
the lcd_string(lcddis); will then print the Number with decimal.
In lcddis[0], lcddis[1] msb two digits in lcddis[2] '.' and lcddis[3] and lcddis[4]
lsb two digits and lcddis[5]=' ' lcddis[6]=NULL are put.
This subroutine converts the Number into string with decimal after two digits
in ASCCI.
*/
void dis_num_lcd(int p,char* lcddis)
{
int i,j=1000;
for(i=0;i<2;i++)
{
lcddis[i]=(p/j)%10+'0';
j/=10;
}
lcddis[2]='.';
for(i=3;i<5;i++)
{
lcddis[i]=(p/j)%10+'0';
j/=10;
}
lcddis[5]=' ';
lcddis[6]=0;
}
/* in dis_hex you send the Hex Value and the pointer to the string
the lcd_string(lcddis); will then print the Number.
This subroutine converts the Hexadecimal Number into string with ASCCI value
*/
void dis_hex(int p,char* lcddis)
{
int i;
lcddis[0]=p/256;
lcddis[1]=(p/16)%16;
lcddis[2]=p%16;
for(i=0;i<3;i++)
if(lcddis[i]<=9)
lcddis[i]+='0';
else
lcddis[i]+=0x37;
lcddis[3]=' ';
lcddis[4]=' ';
lcddis[5]=' ';
lcddis[6]=0;
}
int mod(int m)
{
if(m>0)
return m;
else
return (-m);
}

我虽横行却不霸道
- 粉丝: 95
- 资源: 1万+
最新资源
- 各章随堂测试题面.zip
- 基于模糊PID桥式起重机防摇控制设计 请认真阅读以下内容: 1.基本内容:文中以桥式起重机小车-吊重系统为研究对象,研究起重机的防摇摆控制方法,基于拉格朗日方程建立了小车-吊重的动力学模型并求解出传递
- 毕业论文,基于Django实现学生信息管理系统
- seasar官方文档翻译版
- 电机控制算法无差电流预测控制顶刊复现 Model-Free Predictive Current Control of PMSM Drives Based on Extended State Obs
- 傅里叶变换在图像相关性与模板匹配中的应用及MATLAB实现
- YOLO姿态识别绘制关键点
- Peaks函数FFT变换.m
- 4b044体育商品推荐_springboot+vue.zip
- 碱性水溶液(AWE AlK)电解槽双极板多物理场分析及拓扑优化流道设计(针对热效率最大化及扩散功耗最小化设计) 以工业碱性水电解槽的紧凑组装结构为基础,建立了耦合电场、欧拉-欧拉k-ε湍流场及固体传热
- echarts-5.6.0.zip
- 4b043网络海鲜市场_springboot+vue.zip
- 4b042旅游网站_springboot+vue.zip
- 百度热力图定量数据csv,shp,tif 重庆市20240805日20点
- 4b047北部湾地区助农平台_springboot+vue.zip
- 4b046基于SpringBoot的茶叶商城系统的设计与实现_vue.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


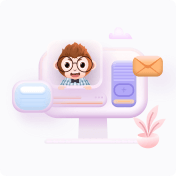