#include "IVNET_H264Decoder.h"
#include <ippcore.h>
#include <ippi.h>
#include <ippcc.h>
int ivnetlib_open(void)
{
//ippStaticInit();
return 0;
}
int ivnetlib_close(void)
{
return 0;
}
/*
USING IPP
*/
int ivnetlib_convertYV12toRGB24(unsigned char *pYV12,unsigned char *pRGB,int w,int h)
{
unsigned char* yuv[3];
yuv[0] = pYV12;
yuv[1] = pYV12 + w * h;
yuv[2] = yuv[1] + (w * h >> 2);
int srcStep[3];
srcStep[0] = w;
srcStep[1] = w/2;
srcStep[2] = w/2;
IppiSize roi;
roi.width = w;
roi.height = h;
::ippiYUV420ToRGB_8u_P3C3R(yuv,srcStep,pRGB,w*3,roi);
return 0;
}
int ivnetlib_convertYV12toRGB32(unsigned char *pYV12,unsigned char *pRGB,int w,int h)
{
unsigned char* yuv[3];
yuv[0] = pYV12;
yuv[1] = pYV12 + w * h;
yuv[2] = yuv[1] + (w * h >> 2);
int srcStep[3];
srcStep[0] = w;
srcStep[1] = w/2;
srcStep[2] = w/2;
IppiSize roi;
roi.width = w;
roi.height = h;
::ippiYUV420ToRGB_8u_P3AC4R(yuv,srcStep,pRGB,w*4,roi);
return 0;
}
int ivnetlib_convertI420toRGB24(unsigned char *pYV12,unsigned char *pRGB,int w,int h)
{
unsigned char* yuv[3];
yuv[0] = pYV12;
yuv[2] = pYV12 + w * h;
yuv[1] = yuv[2] + (w * h >> 2);
int srcStep[3];
srcStep[0] = w;
srcStep[1] = w/2;
srcStep[2] = w/2;
IppiSize roi;
roi.width = w;
roi.height = h;
::ippiYUV420ToRGB_8u_P3C3R(yuv,srcStep,pRGB,w*3,roi);
return 0;
}
int ivnetlib_convertI420toRGB32(unsigned char *pYV12,unsigned char *pRGB,int w,int h)
{
unsigned char* yuv[3];
yuv[0] = pYV12;
yuv[2] = pYV12 + w * h;
yuv[1] = yuv[2] + (w * h >> 2);
int srcStep[3];
srcStep[0] = w;
srcStep[1] = w/2;
srcStep[2] = w/2;
IppiSize roi;
roi.width = w;
roi.height = h;
::ippiYUV420ToRGB_8u_P3AC4R(yuv,srcStep,pRGB,w*4,roi);
return 0;
}
int ivnetlib_convertYV12toYUY2(unsigned char *pYV12,unsigned char *pRGB24,int width,int height)
{
return 0;
}
int ivnetlib_convertYV12toYVYU(unsigned char *pYV12,unsigned char *pRGB24,int width,int height)
{
return 0;
}
int ivnetlib_convertYUY2toYV12(unsigned char *pYV12,unsigned char *pRGB24,int width,int height)
{
return 0;
}
int ivnetlib_convertYUY2toI420(unsigned char *pYV12,unsigned char *pRGB24,int width,int height)
{
return 0;
}
int ivnetlib_convertYUY2toRGB24(unsigned char *pYV12,unsigned char *pRGB24,int width,int height)
{
IppiSize roi;
roi.width = width;
roi.height = height;
::ippiCbYCr422ToRGB_8u_C2C3R(pYV12,width*2,pRGB24,width*3,roi);
return 0;
}
int ivnetlib_convertYUY2toRGB32(unsigned char *pYV12,unsigned char *pRGB24,int width,int height)
{
return 0;
}
int ivnetlib_convertYVYUtoRGB24(unsigned char *pYV12,unsigned char *pRGB24,int width,int height)
{
return 0;
}
int ivnetlib_convertYVYUtoRGB32(unsigned char *pYV12,unsigned char *pRGB24,int width,int height)
{
return 0;
}
extern int ivnetlib_convertRGB24toYV12(unsigned char *pRGB,unsigned char *pYUV,int w,int h)
{
unsigned char* yuv[3];
yuv[0] = pYUV;
yuv[2] = pYUV + w * h;
yuv[1] = yuv[2] + (w * h >> 2);
int dstStep[3];
dstStep[0] = w;
dstStep[1] = w/2;
dstStep[2] = w/2;
IppiSize roi;
roi.width = w;
roi.height = h;
// convert and flip
// ::ippiRGBToYUV420_8u_C3P3R(pRGB+w*h*3,-w*3,yuv,dstStep,roi);
::ippiRGBToYUV420_8u_C3P3R(pRGB,w*3,yuv,dstStep,roi);
return 0;
}
extern int ivnetlib_convertRGB24toYUY2(unsigned char *pRGB,unsigned char *pYUV,int width,int height)
{
unsigned char* yuv[3];
yuv[0] = pYUV;
yuv[2] = pYUV + width * height;
yuv[1] = yuv[2] + (width * height >> 2);
IppiSize roi;
roi.width = width;
roi.height = height;
int dstStep[3];
dstStep[0] = width;
dstStep[1] = width/2;
dstStep[2] = width/2;
::ippiRGBToYUV420_8u_C3P3R(pRGB,width*3,yuv,dstStep,roi);
return 0;
}
int ivnetlib_scaleRGB32(
unsigned char* dst,int dw, int dh, int dzx, int dzy, int dzw, int dzh,
unsigned char* src,int sw, int sh, int szx, int szy, int szw, int szh,int interpolation)
{
IppiSize ssz;
IppiSize dsz;
IppiRect sroi;
double zoomFactorX;
double zoomFactorY;
ssz.width = sw;
ssz.height = sh;
dsz.width = dzw;
dsz.height = dzh;
sroi.x = szx;
sroi.y = szy;
sroi.width = szw;
sroi.height = szh;
zoomFactorX = static_cast<double>(dzw) / static_cast<double>(szw);
zoomFactorY = static_cast<double>(dzh) / static_cast<double>(szh);
::ippiResize_8u_AC4R(
src,
ssz,
sw * 4,
sroi,
dst + (dzy * dw + dzx) * 4,
dw * 4,
dsz,
zoomFactorX,
zoomFactorY,
interpolation);
return 0;
}
int ivnetlib_scaleRGB24(
unsigned char* dst,int dw, int dh, int dzx, int dzy, int dzw, int dzh,
unsigned char* src,int sw, int sh, int szx, int szy, int szw, int szh,int interpolation)
{
IppiSize ssz;
IppiSize dsz;
IppiRect sroi;
double zoomFactorX;
double zoomFactorY;
ssz.width = sw;
ssz.height = sh;
dsz.width = dzw;
dsz.height = dzh;
sroi.x = szx;
sroi.y = szy;
sroi.width = szw;
sroi.height = szh;
zoomFactorX = static_cast<double>(dzw) / static_cast<double>(szw);
zoomFactorY = static_cast<double>(dzh) / static_cast<double>(szh);
::ippiResize_8u_C3R(
src,
ssz,
sw * 3,
sroi,
dst + (dzy * dw + dzx) * 3,
dw * 3,
dsz,
zoomFactorX,
zoomFactorY,
interpolation);
return 0;
}
int ivnetlib_scaleYV12(
unsigned char* dst,int dw, int dh, int dzx, int dzy, int dzw, int dzh,
unsigned char* src,int sw, int sh, int szx, int szy, int szw, int szh,int interpolation )
{
IppiSize ssz;
IppiSize dsz;
IppiRect sroi;
unsigned char* syuv[3];
unsigned char* dyuv[3];
double zoomFactorX;
double zoomFactorY;
ssz.width = sw;
ssz.height = sh;
dsz.width = dw;
dsz.height = dh;
sroi.x = szx;
sroi.y = szy;
sroi.width = szw;
sroi.height = szh;
zoomFactorX = static_cast<double>(dzw) / static_cast<double>(szw);
zoomFactorY = static_cast<double>(dzh) / static_cast<double>(szh);
// YUV 각각의 저장 위치 계산
syuv[0] = src;
syuv[1] = src + sw * sh;
syuv[2] = syuv[1] + (sw * sh >> 2);
dyuv[0] = dst;
dyuv[1] = dst + dw * dh;
dyuv[2] = dyuv[1] + (dw * dh >> 2);
IppStatus result =::ippiResize_8u_C1R(
syuv[0],
ssz,
sw,
sroi,
dyuv[0] + (dzy * dw + dzx),
dw,
dsz,
zoomFactorX,
zoomFactorY,
interpolation); // for Y factor
ssz.width /= 2;
ssz.height /= 2;
dsz.width /= 2;
dsz.height /= 2;
sroi.x /= 2;
sroi.y /= 2;
sroi.width /= 2;
sroi.height /= 2;
result =::ippiResize_8u_C1R(
syuv[1],
ssz,
sw / 2,
sroi,
dyuv[1] + (((dzy/2) * (dw/2)) + dzx/2 ),
dw / 2,
dsz,
zoomFactorX,
zoomFactorY,
interpolation);
result =::ippiResize_8u_C1R(
syuv[2],
ssz,
sw / 2,
sroi,
dyuv[2] + (((dzy/2) * (dw/2)) + dzx/2 ),
dw / 2,
dsz,
zoomFactorX,
zoomFactorY,
interpolation);
return 0;
}
int ivnetlib_scaleYUY2(
unsigned char* dst,int dw, int dh, int dzx, int dzy, int dzw, int dzh,
unsigned char* src,int sw, int sh, int szx, int szy, int szw, int szh,int interpolation)
{
IppiSize ssz;
IppiSize dsz;
IppiRect sroi;
double zoomFactorX;
double zoomFactorY;
ssz.width = sw;
ssz.height = sh;
dsz.width = dzw;
dsz.height = dzh;
sroi.x = szx;
sroi.y = szy;
sroi.width = szw;
sroi.height = szh;
zoomFactorX = static_cast<d
没有合适的资源?快使用搜索试试~ 我知道了~
IVNET_H264Decoder_free.rar_Free!_H264Decoder_h.264 player _play
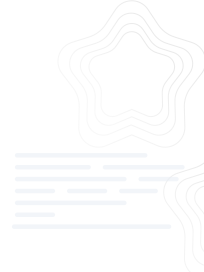
共64个文件
h:31个
a:8个
pc:5个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 149 浏览量
2022-09-19
20:02:34
上传
评论
收藏 1.81MB RAR 举报
温馨提示
Video decode player for H.264 full source code
资源推荐
资源详情
资源评论
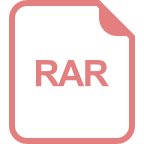
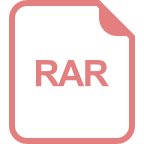
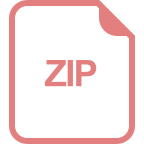
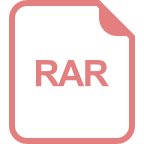
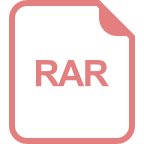
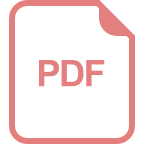
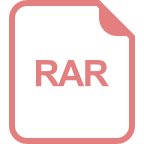
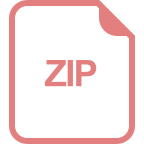
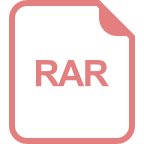
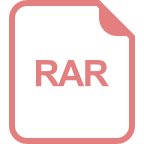
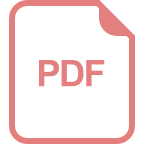
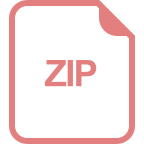
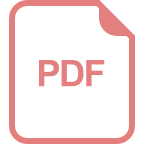
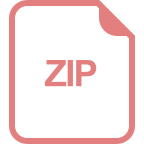
收起资源包目录


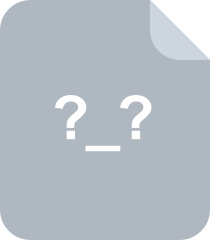

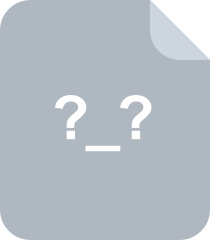
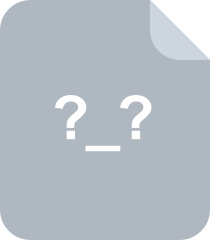
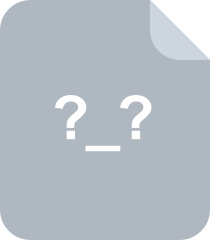
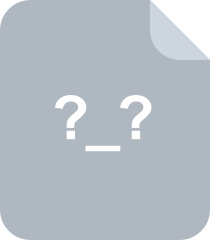
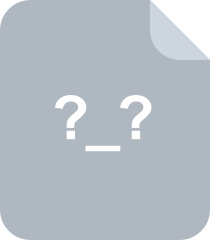
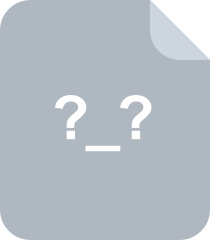
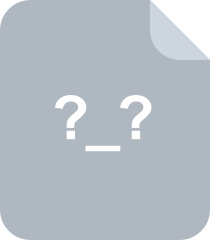
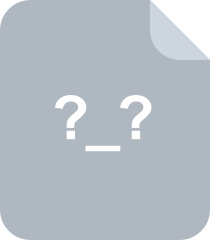
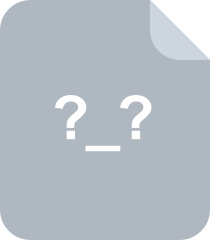
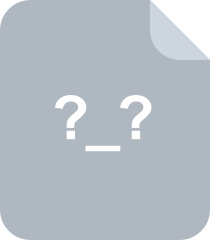
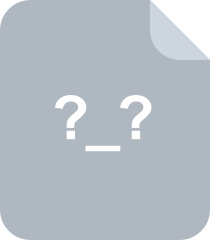
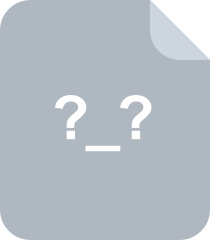
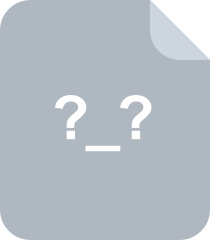
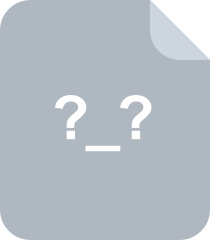
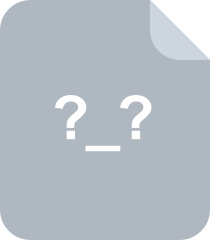
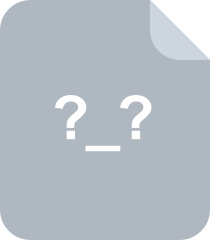
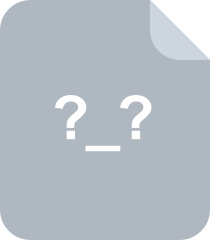
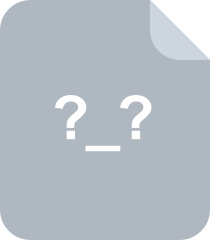
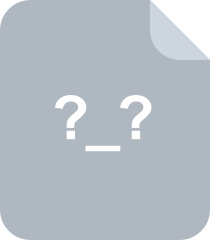
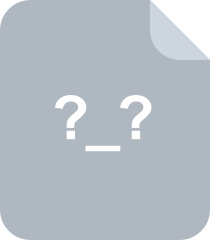



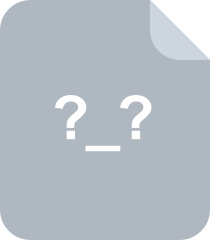
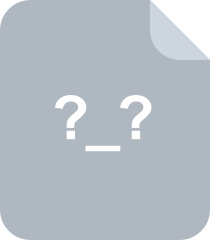
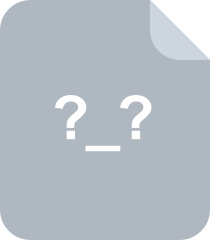
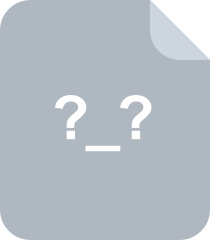
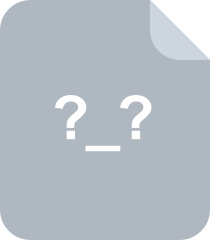
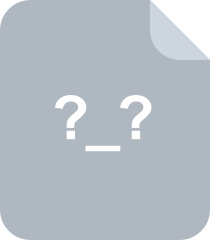
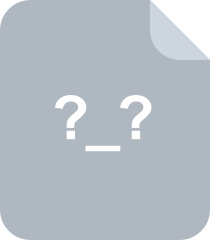
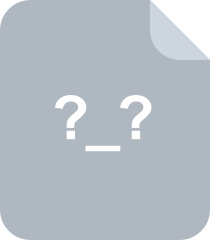
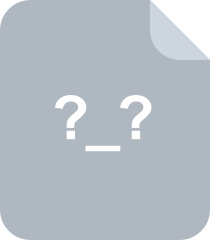
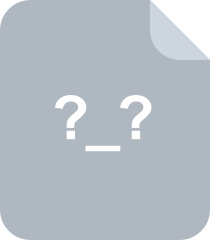
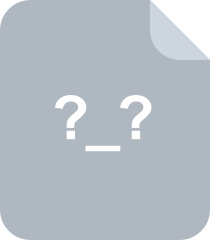
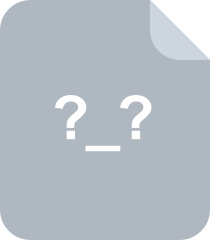
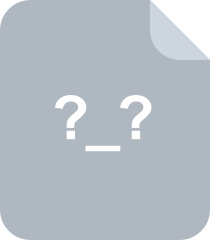
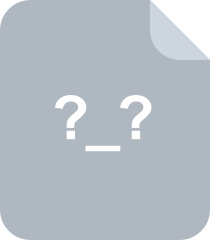
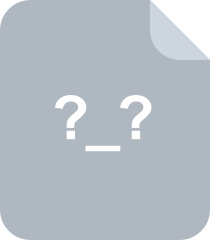
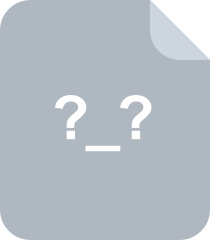
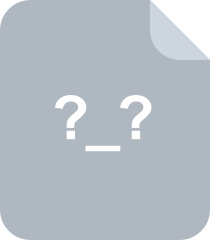
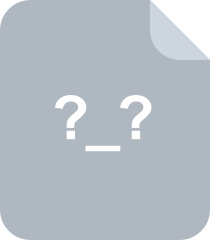
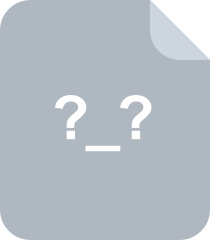
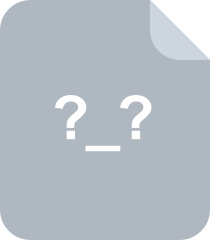
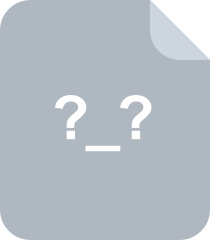
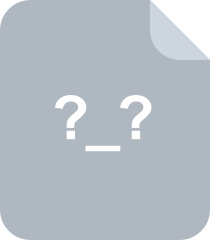
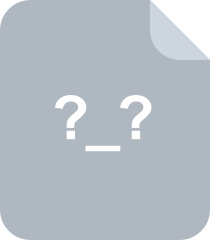
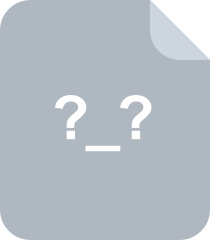
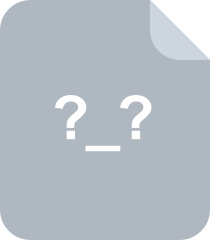
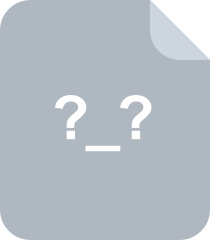
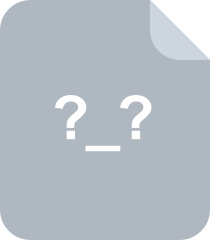
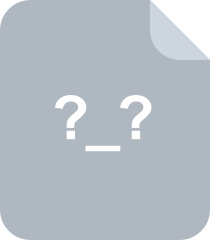
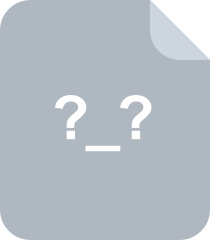

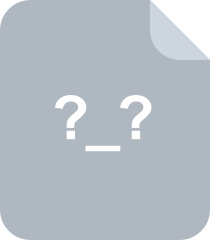
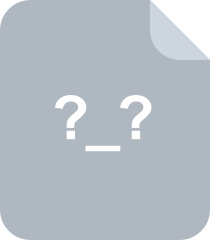
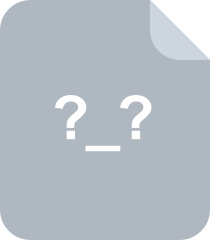
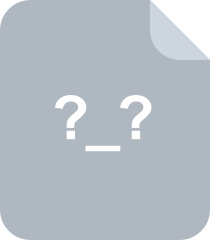
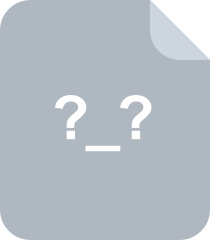
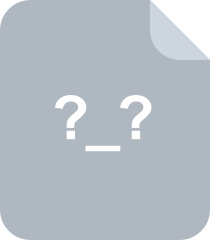

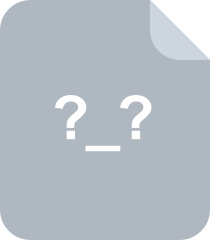
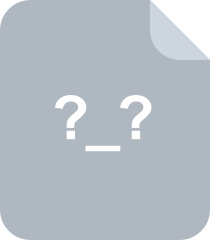
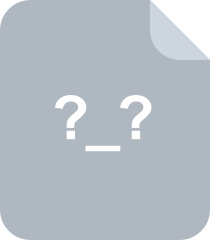
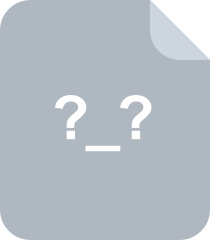
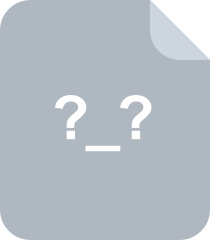
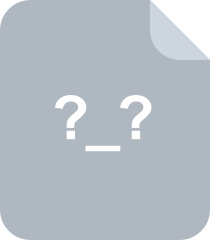
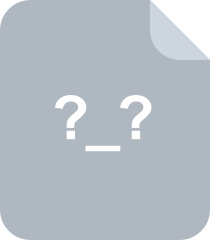
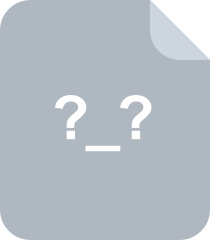
共 64 条
- 1
资源评论
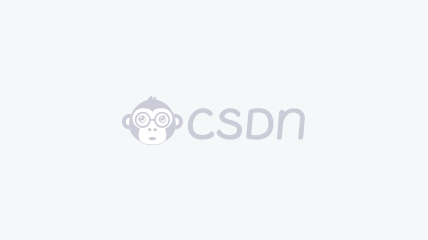

我虽横行却不霸道
- 粉丝: 72
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

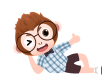
安全验证
文档复制为VIP权益,开通VIP直接复制
