/*
* smbusmodule.c - Python bindings for Linux SMBus access through i2c-dev
* Copyright (C) 2005-2007 Mark M. Hoffman <mhoffman@lightlink.com>
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; version 2 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston,
* MA 02110-1301, USA.
*/
#include <Python.h>
#include "structmember.h"
#include <sys/ioctl.h>
#include <stdlib.h>
#include <stdio.h>
#include <fcntl.h>
#include <linux/i2c.h>
#include <linux/i2c-dev.h>
#include <i2c/smbus.h>
/*
** These are required to build this module against Linux older than 2.6.23.
*/
#ifndef I2C_SMBUS_I2C_BLOCK_BROKEN
#undef I2C_SMBUS_I2C_BLOCK_DATA
#define I2C_SMBUS_I2C_BLOCK_BROKEN 6
#define I2C_SMBUS_I2C_BLOCK_DATA 8
#endif
PyDoc_STRVAR(SMBus_module_doc,
"This module defines an object type that allows SMBus transactions\n"
"on hosts running the Linux kernel. The host kernel must have I2C\n"
"support, I2C device interface support, and a bus adapter driver.\n"
"All of these can be either built-in to the kernel, or loaded from\n"
"modules.\n"
"\n"
"Because the I2C device interface is opened R/W, users of this\n"
"module usually must have root permissions.\n");
typedef struct {
PyObject_HEAD
int fd; /* open file descriptor: /dev/i2c-?, or -1 */
int addr; /* current client SMBus address */
int pec; /* !0 => Packet Error Codes enabled */
} SMBus;
static PyObject *
SMBus_new(PyTypeObject *type, PyObject *args, PyObject *kwds)
{
SMBus *self;
if ((self = (SMBus *)type->tp_alloc(type, 0)) == NULL)
return NULL;
self->fd = -1;
self->addr = -1;
self->pec = 0;
return (PyObject *)self;
}
PyDoc_STRVAR(SMBus_close_doc,
"close()\n\n"
"Disconnects the object from the bus.\n");
static PyObject *
SMBus_close(SMBus *self)
{
if ((self->fd != -1) && (close(self->fd) == -1)) {
PyErr_SetFromErrno(PyExc_IOError);
return NULL;
}
self->fd = -1;
self->addr = -1;
self->pec = 0;
Py_INCREF(Py_None);
return Py_None;
}
static void
SMBus_dealloc(SMBus *self)
{
PyObject *ref = SMBus_close(self);
Py_XDECREF(ref);
#if PY_MAJOR_VERSION >= 3
Py_TYPE(self)->tp_free((PyObject *)self);
#else
self->ob_type->tp_free((PyObject *)self);
#endif
}
#define MAXPATH 16
PyDoc_STRVAR(SMBus_open_doc,
"open(bus)\n\n"
"Connects the object to the specified SMBus.\n");
static PyObject *
SMBus_open(SMBus *self, PyObject *args, PyObject *kwds)
{
int bus;
char path[MAXPATH];
static char *kwlist[] = {"bus", NULL};
if (!PyArg_ParseTupleAndKeywords(args, kwds, "i:open", kwlist, &bus))
return NULL;
if (snprintf(path, MAXPATH, "/dev/i2c-%d", bus) >= MAXPATH) {
PyErr_SetString(PyExc_OverflowError,
"Bus number is invalid.");
return NULL;
}
if ((self->fd = open(path, O_RDWR, 0)) == -1) {
PyErr_SetFromErrno(PyExc_IOError);
return NULL;
}
Py_INCREF(Py_None);
return Py_None;
}
static int
SMBus_init(SMBus *self, PyObject *args, PyObject *kwds)
{
int bus = -1;
static char *kwlist[] = {"bus", NULL};
if (!PyArg_ParseTupleAndKeywords(args, kwds, "|i:__init__",
kwlist, &bus))
return -1;
if (bus >= 0) {
SMBus_open(self, args, kwds);
if (PyErr_Occurred())
return -1;
}
return 0;
}
/*
* private helper function, 0 => success, !0 => error
*/
static int
SMBus_set_addr(SMBus *self, int addr)
{
int ret = 0;
if (self->addr != addr) {
ret = ioctl(self->fd, I2C_SLAVE, addr);
self->addr = addr;
}
return ret;
}
#define SMBus_SET_ADDR(self, addr) do { \
if (SMBus_set_addr(self, addr)) { \
PyErr_SetFromErrno(PyExc_IOError); \
return NULL; \
} \
} while(0)
PyDoc_STRVAR(SMBus_write_quick_doc,
"write_quick(addr)\n\n"
"Perform SMBus Quick transaction.\n");
static PyObject *
SMBus_write_quick(SMBus *self, PyObject *args)
{
int addr;
__s32 result;
if (!PyArg_ParseTuple(args, "i:write_quick", &addr))
return NULL;
SMBus_SET_ADDR(self, addr);
if ((result = i2c_smbus_write_quick(self->fd, I2C_SMBUS_WRITE))) {
PyErr_SetFromErrno(PyExc_IOError);
return NULL;
}
Py_INCREF(Py_None);
return Py_None;
}
PyDoc_STRVAR(SMBus_read_byte_doc,
"read_byte(addr) -> result\n\n"
"Perform SMBus Read Byte transaction.\n");
static PyObject *
SMBus_read_byte(SMBus *self, PyObject *args)
{
int addr;
__s32 result;
if (!PyArg_ParseTuple(args, "i:read_byte", &addr))
return NULL;
SMBus_SET_ADDR(self, addr);
if ((result = i2c_smbus_read_byte(self->fd)) < 0) {
PyErr_SetFromErrno(PyExc_IOError);
return NULL;
}
return Py_BuildValue("l", (long)result);
}
PyDoc_STRVAR(SMBus_write_byte_doc,
"write_byte(addr, val)\n\n"
"Perform SMBus Write Byte transaction.\n");
static PyObject *
SMBus_write_byte(SMBus *self, PyObject *args)
{
int addr, val;
__s32 result;
if (!PyArg_ParseTuple(args, "ii:write_byte", &addr, &val))
return NULL;
SMBus_SET_ADDR(self, addr);
if ((result = i2c_smbus_write_byte(self->fd, (__u8)val)) < 0) {
PyErr_SetFromErrno(PyExc_IOError);
return NULL;
}
Py_INCREF(Py_None);
return Py_None;
}
PyDoc_STRVAR(SMBus_read_byte_data_doc,
"read_byte_data(addr, cmd) -> result\n\n"
"Perform SMBus Read Byte Data transaction.\n");
static PyObject *
SMBus_read_byte_data(SMBus *self, PyObject *args)
{
int addr, cmd;
__s32 result;
if (!PyArg_ParseTuple(args, "ii:read_byte_data", &addr, &cmd))
return NULL;
SMBus_SET_ADDR(self, addr);
if ((result = i2c_smbus_read_byte_data(self->fd, (__u8)cmd)) < 0) {
PyErr_SetFromErrno(PyExc_IOError);
return NULL;
}
return Py_BuildValue("l", (long)result);
}
PyDoc_STRVAR(SMBus_write_byte_data_doc,
"write_byte_data(addr, cmd, val)\n\n"
"Perform SMBus Write Byte Data transaction.\n");
static PyObject *
SMBus_write_byte_data(SMBus *self, PyObject *args)
{
int addr, cmd, val;
__s32 result;
if (!PyArg_ParseTuple(args, "iii:write_byte_data", &addr, &cmd, &val))
return NULL;
SMBus_SET_ADDR(self, addr);
if ((result = i2c_smbus_write_byte_data(self->fd,
(__u8)cmd, (__u8)val)) < 0) {
PyErr_SetFromErrno(PyExc_IOError);
return NULL;
}
Py_INCREF(Py_None);
return Py_None;
}
PyDoc_STRVAR(SMBus_read_word_data_doc,
"read_word_data(addr, cmd) -> result\n\n"
"Perform SMBus Read Word Data transaction.\n");
static PyObject *
SMBus_read_word_data(SMBus *self, PyObject *args)
{
int addr, cmd;
__s32 result;
if (!PyArg_ParseTuple(args, "ii:read_word_data", &addr, &cmd))
return NULL;
SMBus_SET_ADDR(self, addr);
if ((result = i2c_smbus_read_word_data(self->fd, (__u8)cmd)) < 0) {
PyErr_SetFromErrno(PyExc_IOError);
return NULL;
}
return Py_BuildValue("l", (long)result);
}
PyDoc_STRVAR(SMBus_write_word_data_doc,
"write_word_data(addr, cmd, val)\n\n"
"Perform SMBus Write Word Data transaction.\n");
static PyObject *
SMBus_write_word_data(SMBus *self, PyObject *args)
{
int addr, cmd, val;
__s32 result;
if (!PyArg_ParseTuple(args, "iii:write_word_data", &addr, &cmd, &val))
return NULL;
SMBus_SET_ADDR(self, addr);
if ((result = i2c_smbus_write_word_data(self->fd,
(__u8)cmd, (__u16)val)) < 0) {
PyErr_SetFromErrno(PyExc_IOError);
return NULL;
}
Py_INCREF(Py_None);
return Py_None;
}
PyDoc_STRVAR(SMBus_process_call_doc,
"process_call(addr, cmd, val)\n\n"
"Perform SMBus Process Call transaction.\n");
static PyObject *
SMBus_process_call(SMBus *self, PyObject *args)
{
int addr, cmd, val;
__s32 result;
if (!PyArg_ParseTuple(args, "iii:process_call", &addr, &cmd, &val))
return NULL;
SMBus_SET_ADDR(self, addr);
if ((result = i2c_smbus_process_call(self->fd,
(__u8)cmd, (__u16)val)) < 0) {
Py
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
1.存放路径:rk3399源码目录下的external目录 2.解压:tar -zxvf i2c-tools-4.1.tar.gz 3.编辑文件:在 解压后的i2c-tools-4.1 目录添加 Android.mk 编译文件 4.编译: 先退回到源码目录:/home/ych/Work/rk3399/fw/proj/firefly-rk3399-Industry source build/envsetup.sh mmm external/i2c-tools-4.1/ 5.生成工具目录:/home/ych/Work/rk3399/fw/proj/firefly-rk3399-Industry/out/target/product/rk3399_firefly/system/bin目录下i2cdetect,i2cdump,i2cget,i2cset,i2ctransfer这些工具可以push到Android开发板中使用
资源推荐
资源详情
资源评论
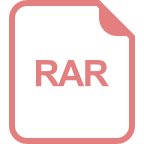
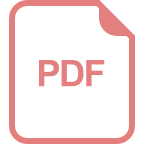
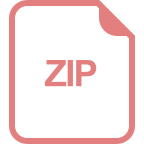
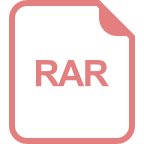
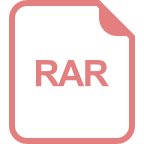
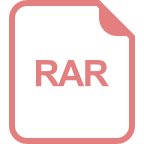
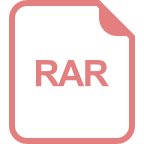
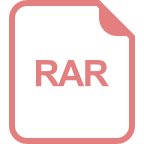
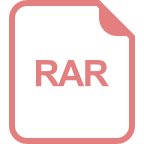
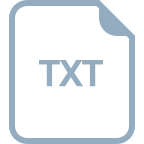
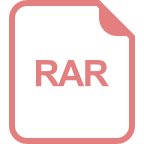
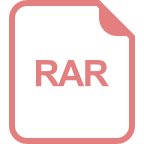
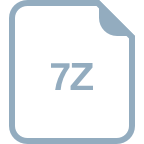
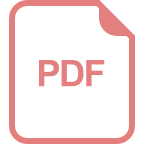
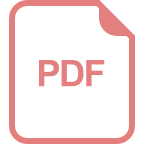
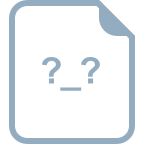
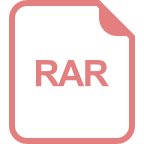
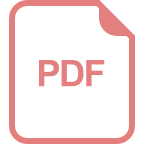
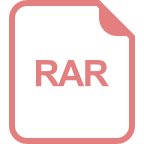
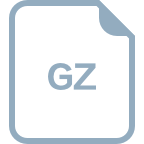
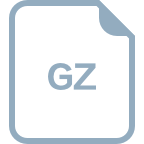
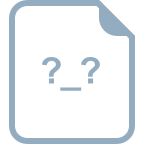
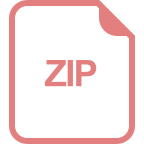
收起资源包目录



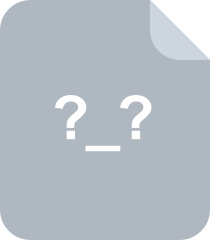
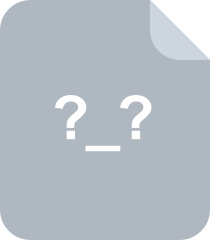
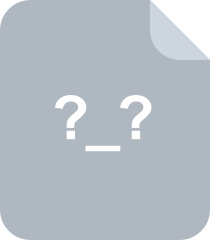
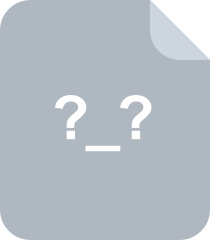
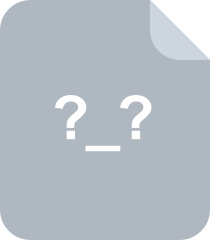
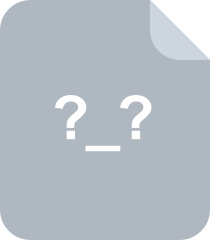
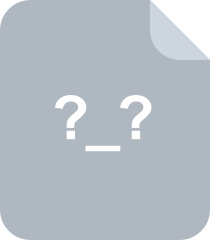

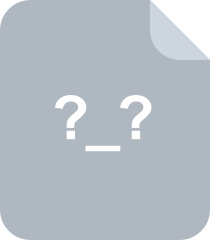

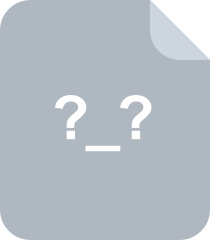

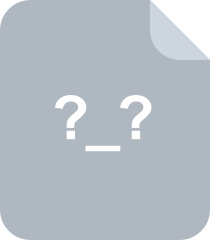
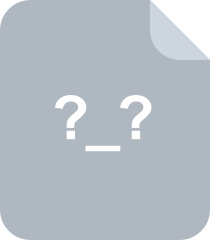
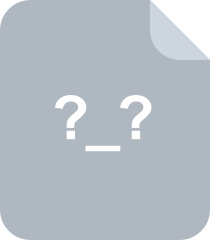
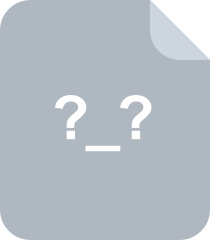
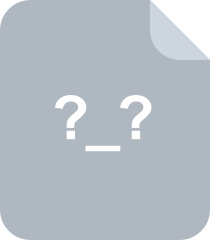

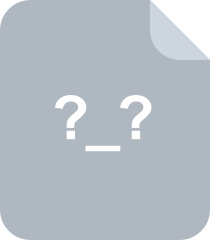
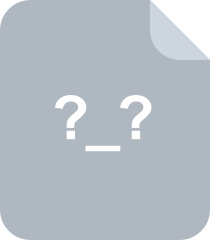
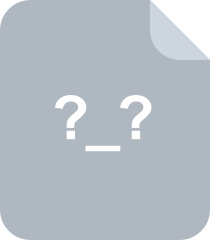

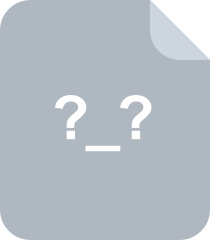
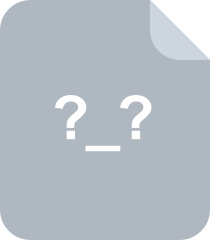
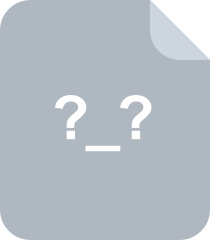
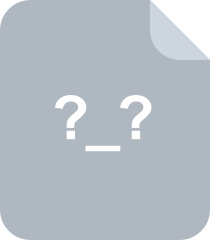
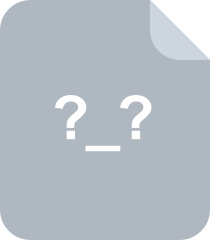
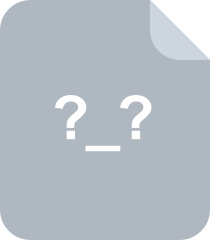
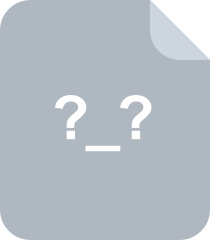
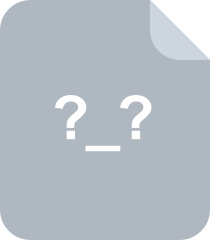
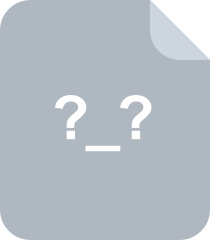
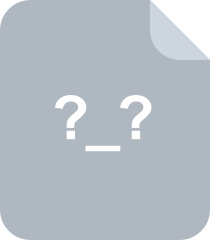
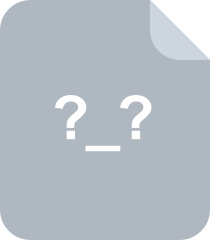
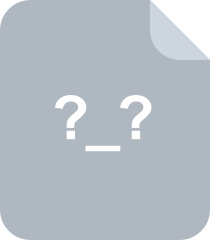
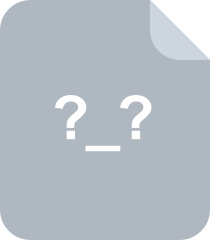
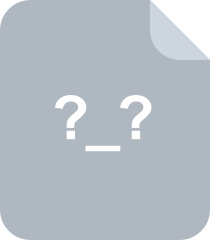
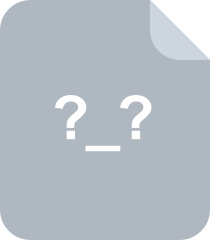
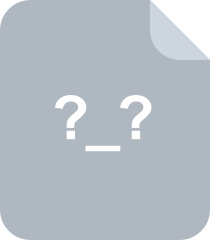
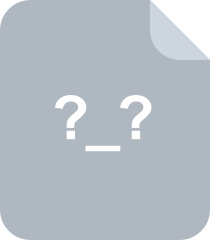
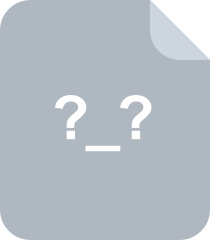
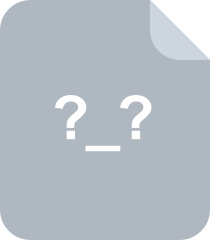

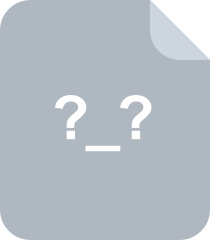
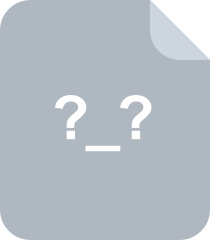
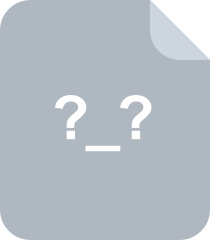
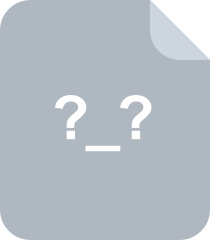
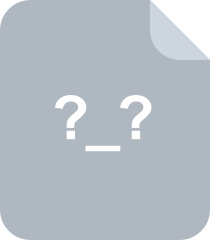
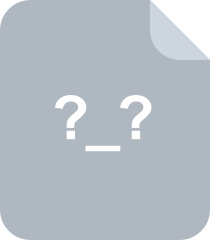
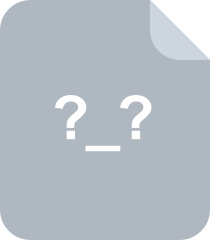
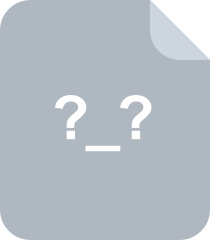
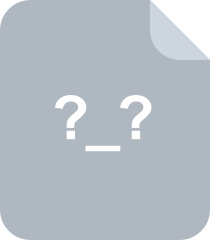
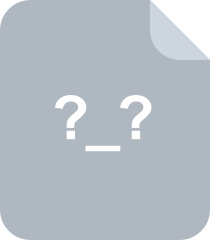

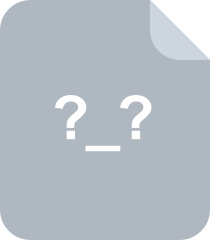
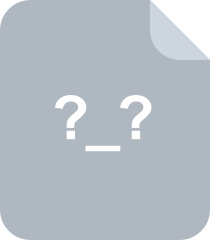
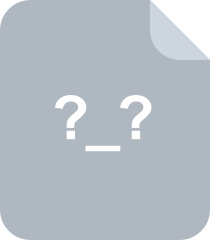
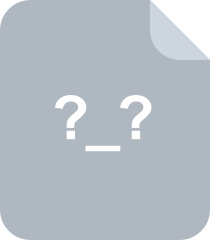

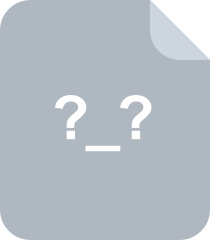
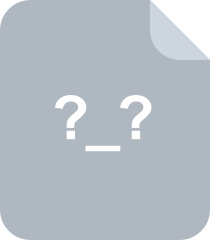
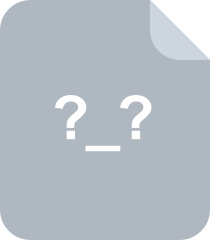
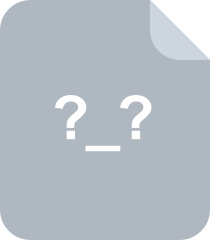
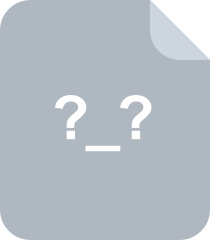
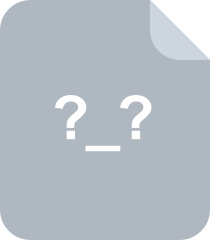
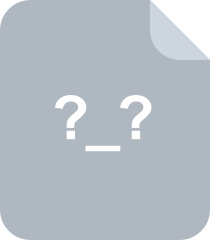
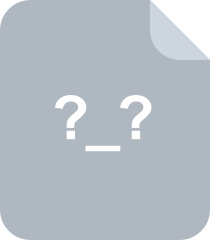
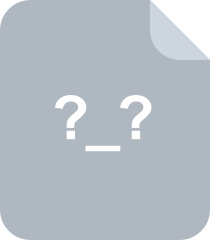
共 59 条
- 1
资源评论
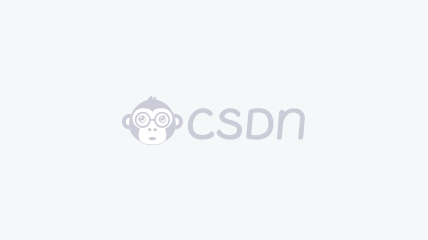

艾特5号
- 粉丝: 18
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

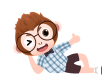
最新资源
- 从XML生成可与Ajax共同使用的JSON中文WORD版最新版本
- silverlight通过WebService连接数据库中文WORD版最新版本
- 使用NetBeans连接SQLserver2008数据库教程中文WORD版最新版本
- XPath实例中文WORD版最新版本
- XPath语法规则中文WORD版最新版本
- XPath入门教程中文WORD版最新版本
- ORACLE数据库管理系统体系结构中文WORD版最新版本
- Sybase数据库安装以及新建数据库中文WORD版最新版本
- tomcat6.0配置oracle数据库连接池中文WORD版最新版本
- hibernate连接oracle数据库中文WORD版最新版本
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


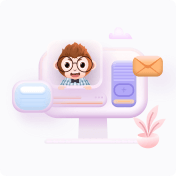
安全验证
文档复制为VIP权益,开通VIP直接复制
