List of CE specific functions and variables:
TrainerOrigin : A variable that contains the path of the trainer that launched cheat engine (Only set when launched as a trainer)
process : A variable that contains the main modulename of the currently opened process
MainForm: The main ce gui
AddressList: The address list of the main ce gui
getCEVersion(): Returns a floating point value specifying the version of cheat engine
getCheatEngineFileVersion(): Returns the full version data of the cheat engine version. A raw integer, and a table containing major, minor, release and build
activateProtection(): Prevents basic memory scanners from opening the cheat engine process
enableDRM(altitude OPTIONAL, secondaryprocessid OPTIONAL ) : Prevents normal memory scanners from reading the Cheat Engine process (kernelmode) The secondaryprocessid lets you protect another process. E.g the game itself, so they can't easily see what you change
fullAccess(address,size): Changes the protection of a block of memory to writable and executable
loadTable(filename, merge OPTIONAL): Loads a .ct or .cetrainer. If merge is provided and set to true it will not clear the old table
loadTable(stream ,merge OPTIONAL, ignoreluascriptdialog BOOLEAN): Loads a table from a stream object
saveTable(filename, protect OPTIONAL): Saves the current table. If protect is provided and set to true and the filename has the .CETRAINER extension, it will protect it from reading normally
note: addresses can be strings, they will get interpreted by ce's symbolhandler
copyMemory(sourceAddress: integer, size: integer, destinationAddress:integer SEMIOPTIONAL, Method:integer OPTIONAL):
Copies memory from the given address to the destination address
If no destinationAddress is given(or nil), CE will allocate a random address for you
Method can be:
nil/0: Copy from target process to target process
1: Copy from target process to CE Memory
2: Copy from CE Memory to target process
3: Copy from CE Memory to CE Memory
Returns the address of the copy on success, nil on failure
readBytes(address,bytecount, ReturnAsTable ) : returns the bytes at the given address. If ReturnAsTable is true it will return a table instead of multiple bytes
Reads the bytes at the given address and returns a table containing the read out bytes
writeBytes(address, x,x,x,x,...) : Write the given bytes to the given address from a table
writeBytes(address, table) : Write the given bytes to the given address from a table
readSmallInteger(address) : Reads a 16-bit integer from the specified address
readInteger(address) : Reads a 32-bit integer from the specified address
readQword(address): Reads a 64-bit integer from the specified address
readPointer(address): In a 64-bit target this equals readQword, in a 32-bit target readInteger()
readFloat(address) : Reads a single precision floating point value from the specified address
readDouble(address) : Reads a double precision floating point value from the specified address
readString(address, maxlength, widechar OPTIONAL) : Reads a string till it encounters a 0-terminator. Maxlength is just so you won't freeze for too long, set to 6000 if you don't care too much. Set WideChar to true if it is encoded using a widechar formatting
writeSmallInteger(address,value) : Writes a 16-bit integer to the specified address. Returns true on success
writeInteger(address,value) : Writes a 32-bit integer to the specified address. Returns true on success
writeQword(address, value): Write a 64-bit integer to the specified address. Returns true on success
writePointer(address,value)
writeFloat(address,value) : Writes a single precision floating point to the specified address. Returns true on success
writeDouble(address,value) : Writes a double precision floating point to the specified address. Returns true on success
writeString(address,text, widechar OPTIONAL) : Write a string to the specified address. Returns true on success
readBytesLocal(address,bytecount, ReturnAsTable) : See readBytes but then it's for Cheat engine's memory
readSmallIntegerLocal(address) : Reads a 16-bit integer from the specified address in CE's memory
readIntegerLocal(address) : Reads a 32-bit integer from the specified address in CE's memory
readQwordLocal(address) : Reads a 64-bit integer from the specified address in CE's memory
readPointerLocal(address) : ReadQwordLocal/ReadIntegerLocal depending on the cheat engine build
readFloatLocal(address) : Reads a single precision floating point value from the specified address in CE's memory
readDoubleLocal(address) : Reads a double precision floating point value from the specified address in CE's memory
readStringLocal(address, maxlength, widechar OPTIONAL)
writeSmallIntegerLocal(address,value) : Writes a 16-bit integer to the specified address in CE's memory. Returns true on success
writeIntegerLocal(address,value) : Writes a 32-bit integer to the specified address in CE's memory. Returns true on success
writeQwordLocal(address,value) : Writes a 64-bit integer to the specified address in CE's memory. Returns true on success
writePointerLocal(address,value)
writeFloatLocal(address,value) : Writes a single precision floating point to the specified address in CE's memory. Returns true on success
writeDoubleLocal(address,value) : Writes a double precision floating point to the specified address in CE's memory. Returns true on success
writeStringLocal(address,string, widechar OPTIONAL)
writeBytesLocal(address, x,x,x,x,...) : See writeBytes but then it's for Cheat Engine's memory
writeBytesLocal(address, table, , count) : See writeBytes but then it's for Cheat Engine's memory
readSmallInteger, readInteger, readSmallIntegerLocal, readIntegerLocal
can also have second boolean parameter. If true, value will be signed.
wordToByteTable(number): {} - Converts a word to a bytetable
dwordToByteTable(number): {} - Converts a dword to a bytetable
qwordToByteTable(number): {} - Converts a qword to a bytetable
floatToByteTable(number): {} - Converts a float to a bytetable
doubleToByteTable(number): {} - Converts a double to a bytetable
stringToByteTable(string): {} - Converts a string to a bytetable
wideStringToByteTable(string): {} - Converts a string to a widestring and converts that to a bytetable
byteTableToWord(table): number - Converts a bytetable to a word
byteTableToDword(table): number - Converts a bytetable to a dword
byteTableToQword(table): number - Converts a bytetable to a qword
byteTableToFloat(table): number - Converts a bytetable to a float
byteTableToDouble(table): number - Converts a bytetable to a double
byteTableToString(table): string - Converts a bytetable to a string
byteTableToWideString(table): string - Converts a bytetable to a widestring and convets that to a string
bOr(int1, int2) : Binary Or
bXor(int1, int2) : Binary Xor
bAnd(int1, int2) : Binary And
bShl(int, int2) : Binary shift left
bShr(int, int2) : Binary shift right
bNot(int) : Binary not
writeRegionToFile(filename, sourceaddress,size) : Writes the given region to a file. Returns the number of bytes written
readRegionFromFile(filename, destinationaddress)
resetLuaState(): This will create a new lua state that will be used. (Does not destroy the old one, so memory leak)
createRef(...): integer - Returns an integer reference that you can use with getRef. Useful for objects that can only store integers and need to reference lua objects. (Component.Tag...)
getRef(integer): ... - Returns whatever the reference points out
destroyRef(integer) - Removes the reference
encodeFunction(function): string - Converts a given function into an encoded string that you can pass on to decodeFunction
decodeFunction(string): function - Converts an encoded string back into a function. Note th

weixin_42632254
- 粉丝: 0
- 资源: 1
最新资源
- “海油杯”焊工技能竞赛中不锈钢管道焊接操作技巧 - .pdf
- “链蓖机托辊轴”异种金属焊接技术的探索与应用 - .pdf
- “十-五”期间石化工程建设中焊接技术的发展.pdf
- “水煤浆”气化特殊材质工艺管道现场焊接技术.pdf
- 基于java+springboot+mysql+微信小程序的戏曲文化苑小程序 源码+数据库+论文(高分毕业设计).zip
- 00Cr17Ni14Mo2不锈钢高压管道焊接工艺.pdf
- 00Cr19Ni10厚板焊接工艺的优化 - .pdf
- 00Cr18Ni14M02Cu2不锈钢焊接工艺对耐海水腐蚀的影响.pdf
- 0Cr18Ni9Ti奥氏体不锈钢焊接接头应力腐蚀行为的研究.pdf
- 0.3mm厚镀镍钢片微电阻点焊接头组织性能研究 - .pdf
- 0Cr25Ni20与20-号材料焊接热裂纹的研究 - .pdf
- 0Gr17Ni13M02Ti+Q235不锈复合钢板的焊接工艺研究 - .pdf
- 1C_r13不锈钢与Q235碳钢的异种钢焊接技术.pdf
- 01国家体育场焊接方管桁架单K节点设计研究.pdf
- 基于java+springboot+mysql+微信小程序的乡村研学旅行平台 源码+数据库+论文(高分毕业设计).zip
- 1Cr5Mo钢与20钢管异种钢接头的焊接.pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


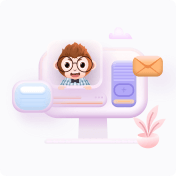