/* Arm MVE intrinsics include file.
Copyright (C) 2019-2023 Free Software Foundation, Inc.
Contributed by Arm.
This file is part of GCC.
GCC is free software; you can redistribute it and/or modify it
under the terms of the GNU General Public License as published
by the Free Software Foundation; either version 3, or (at your
option) any later version.
GCC is distributed in the hope that it will be useful, but WITHOUT
ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public
License for more details.
You should have received a copy of the GNU General Public License
along with GCC; see the file COPYING3. If not see
<http://www.gnu.org/licenses/>. */
#ifndef _GCC_ARM_MVE_H
#define _GCC_ARM_MVE_H
#if __ARM_BIG_ENDIAN
#error "MVE intrinsics are not supported in Big-Endian mode."
#elif !__ARM_FEATURE_MVE
#error "MVE feature not supported"
#else
#include <stdint.h>
#ifndef __cplusplus
#include <stdbool.h>
#endif
#include "arm_mve_types.h"
#ifndef __ARM_MVE_PRESERVE_USER_NAMESPACE
#define vst4q(__addr, __value) __arm_vst4q(__addr, __value)
#define vdupq_n(__a) __arm_vdupq_n(__a)
#define vabsq(__a) __arm_vabsq(__a)
#define vclsq(__a) __arm_vclsq(__a)
#define vclzq(__a) __arm_vclzq(__a)
#define vnegq(__a) __arm_vnegq(__a)
#define vaddlvq(__a) __arm_vaddlvq(__a)
#define vaddvq(__a) __arm_vaddvq(__a)
#define vmovlbq(__a) __arm_vmovlbq(__a)
#define vmovltq(__a) __arm_vmovltq(__a)
#define vmvnq(__a) __arm_vmvnq(__a)
#define vrev16q(__a) __arm_vrev16q(__a)
#define vrev32q(__a) __arm_vrev32q(__a)
#define vrev64q(__a) __arm_vrev64q(__a)
#define vqabsq(__a) __arm_vqabsq(__a)
#define vqnegq(__a) __arm_vqnegq(__a)
#define vshrq(__a, __imm) __arm_vshrq(__a, __imm)
#define vaddlvq_p(__a, __p) __arm_vaddlvq_p(__a, __p)
#define vcmpneq(__a, __b) __arm_vcmpneq(__a, __b)
#define vshlq(__a, __b) __arm_vshlq(__a, __b)
#define vsubq(__a, __b) __arm_vsubq(__a, __b)
#define vrmulhq(__a, __b) __arm_vrmulhq(__a, __b)
#define vrhaddq(__a, __b) __arm_vrhaddq(__a, __b)
#define vqsubq(__a, __b) __arm_vqsubq(__a, __b)
#define vqaddq(__a, __b) __arm_vqaddq(__a, __b)
#define vorrq(__a, __b) __arm_vorrq(__a, __b)
#define vornq(__a, __b) __arm_vornq(__a, __b)
#define vmulq(__a, __b) __arm_vmulq(__a, __b)
#define vmulltq_int(__a, __b) __arm_vmulltq_int(__a, __b)
#define vmullbq_int(__a, __b) __arm_vmullbq_int(__a, __b)
#define vmulhq(__a, __b) __arm_vmulhq(__a, __b)
#define vmladavq(__a, __b) __arm_vmladavq(__a, __b)
#define vminvq(__a, __b) __arm_vminvq(__a, __b)
#define vminq(__a, __b) __arm_vminq(__a, __b)
#define vmaxvq(__a, __b) __arm_vmaxvq(__a, __b)
#define vmaxq(__a, __b) __arm_vmaxq(__a, __b)
#define vhsubq(__a, __b) __arm_vhsubq(__a, __b)
#define vhaddq(__a, __b) __arm_vhaddq(__a, __b)
#define veorq(__a, __b) __arm_veorq(__a, __b)
#define vcmphiq(__a, __b) __arm_vcmphiq(__a, __b)
#define vcmpeqq(__a, __b) __arm_vcmpeqq(__a, __b)
#define vcmpcsq(__a, __b) __arm_vcmpcsq(__a, __b)
#define vcaddq_rot90(__a, __b) __arm_vcaddq_rot90(__a, __b)
#define vcaddq_rot270(__a, __b) __arm_vcaddq_rot270(__a, __b)
#define vbicq(__a, __b) __arm_vbicq(__a, __b)
#define vandq(__a, __b) __arm_vandq(__a, __b)
#define vaddvq_p(__a, __p) __arm_vaddvq_p(__a, __p)
#define vaddvaq(__a, __b) __arm_vaddvaq(__a, __b)
#define vaddq(__a, __b) __arm_vaddq(__a, __b)
#define vabdq(__a, __b) __arm_vabdq(__a, __b)
#define vshlq_r(__a, __b) __arm_vshlq_r(__a, __b)
#define vrshlq(__a, __b) __arm_vrshlq(__a, __b)
#define vqshlq(__a, __b) __arm_vqshlq(__a, __b)
#define vqshlq_r(__a, __b) __arm_vqshlq_r(__a, __b)
#define vqrshlq(__a, __b) __arm_vqrshlq(__a, __b)
#define vminavq(__a, __b) __arm_vminavq(__a, __b)
#define vminaq(__a, __b) __arm_vminaq(__a, __b)
#define vmaxavq(__a, __b) __arm_vmaxavq(__a, __b)
#define vmaxaq(__a, __b) __arm_vmaxaq(__a, __b)
#define vbrsrq(__a, __b) __arm_vbrsrq(__a, __b)
#define vshlq_n(__a, __imm) __arm_vshlq_n(__a, __imm)
#define vrshrq(__a, __imm) __arm_vrshrq(__a, __imm)
#define vqshlq_n(__a, __imm) __arm_vqshlq_n(__a, __imm)
#define vcmpltq(__a, __b) __arm_vcmpltq(__a, __b)
#define vcmpleq(__a, __b) __arm_vcmpleq(__a, __b)
#define vcmpgtq(__a, __b) __arm_vcmpgtq(__a, __b)
#define vcmpgeq(__a, __b) __arm_vcmpgeq(__a, __b)
#define vqshluq(__a, __imm) __arm_vqshluq(__a, __imm)
#define vqrdmulhq(__a, __b) __arm_vqrdmulhq(__a, __b)
#define vqdmulhq(__a, __b) __arm_vqdmulhq(__a, __b)
#define vmlsdavxq(__a, __b) __arm_vmlsdavxq(__a, __b)
#define vmlsdavq(__a, __b) __arm_vmlsdavq(__a, __b)
#define vmladavxq(__a, __b) __arm_vmladavxq(__a, __b)
#define vhcaddq_rot90(__a, __b) __arm_vhcaddq_rot90(__a, __b)
#define vhcaddq_rot270(__a, __b) __arm_vhcaddq_rot270(__a, __b)
#define vqmovntq(__a, __b) __arm_vqmovntq(__a, __b)
#define vqmovnbq(__a, __b) __arm_vqmovnbq(__a, __b)
#define vmulltq_poly(__a, __b) __arm_vmulltq_poly(__a, __b)
#define vmullbq_poly(__a, __b) __arm_vmullbq_poly(__a, __b)
#define vmovntq(__a, __b) __arm_vmovntq(__a, __b)
#define vmovnbq(__a, __b) __arm_vmovnbq(__a, __b)
#define vmlaldavq(__a, __b) __arm_vmlaldavq(__a, __b)
#define vqmovuntq(__a, __b) __arm_vqmovuntq(__a, __b)
#define vqmovunbq(__a, __b) __arm_vqmovunbq(__a, __b)
#define vshlltq(__a, __imm) __arm_vshlltq(__a, __imm)
#define vshllbq(__a, __imm) __arm_vshllbq(__a, __imm)
#define vqdmulltq(__a, __b) __arm_vqdmulltq(__a, __b)
#define vqdmullbq(__a, __b) __arm_vqdmullbq(__a, __b)
#define vmlsldavxq(__a, __b) __arm_vmlsldavxq(__a, __b)
#define vmlsldavq(__a, __b) __arm_vmlsldavq(__a, __b)
#define vmlaldavxq(__a, __b) __arm_vmlaldavxq(__a, __b)
#define vrmlaldavhq(__a, __b) __arm_vrmlaldavhq(__a, __b)
#define vaddlvaq(__a, __b) __arm_vaddlvaq(__a, __b)
#define vrmlsldavhxq(__a, __b) __arm_vrmlsldavhxq(__a, __b)
#define vrmlsldavhq(__a, __b) __arm_vrmlsldavhq(__a, __b)
#define vrmlaldavhxq(__a, __b) __arm_vrmlaldavhxq(__a, __b)
#define vabavq(__a, __b, __c) __arm_vabavq(__a, __b, __c)
#define vbicq_m_n(__a, __imm, __p) __arm_vbicq_m_n(__a, __imm, __p)
#define vqrshrnbq(__a, __b, __imm) __arm_vqrshrnbq(__a, __b, __imm)
#define vqrshrunbq(__a, __b, __imm) __arm_vqrshrunbq(__a, __b, __imm)
#define vrmlaldavhaq(__a, __b, __c) __arm_vrmlaldavhaq(__a, __b, __c)
#define vshlcq(__a, __b, __imm) __arm_vshlcq(__a, __b, __imm)
#define vpselq(__a, __b, __p) __arm_vpselq(__a, __b, __p)
#define vrev64q_m(__inactive, __a, __p) __arm_vrev64q_m(__inactive, __a, __p)
#define vqrdmlashq(__a, __b, __c) __arm_vqrdmlashq(__a, __b, __c)
#define vqrdmlahq(__a, __b, __c) __arm_vqrdmlahq(__a, __b, __c)
#define vqdmlashq(__a, __b, __c) __arm_vqdmlashq(__a, __b, __c)
#define vqdmlahq(__a, __b, __c) __arm_vqdmlahq(__a, __b, __c)
#define vmvnq_m(__inactive, __a, __p) __arm_vmvnq_m(__inactive, __a, __p)
#define vmlasq(__a, __b, __c) __arm_vmlasq(__a, __b, __c)
#define vmlaq(__a, __b, __c) __arm_vmlaq(__a, __b, __c)
#define vmladavq_p(__a, __b, __p) __arm_vmladavq_p(__a, __b, __p)
#define vmladavaq(__a, __b, __c) __arm_vmladavaq(__a, __b, __c)
#define vminvq_p(__a, __b, __p) __arm_vminvq_p(__a, __b, __p)
#define vmaxvq_p(__a, __b, __p) __arm_vmaxvq_p(__a, __b, __p)
#define vdupq_m(__inactive, __a, __p) __arm_vdupq_m(__inactive, __a, __p)
#define vcmpneq_m(__a, __b, __p) __arm_vcmpneq_m(__a, __b, __p)
#define vcmphiq_m(__a, __b, __p) __arm_vcmphiq_m(__a, __b, __p)
#define vcmpeqq_m(__a, __b, __p) __arm_vcmpeqq_m(__a, __b, __p)
#define vcmpcsq_m(__a, __b, __p) __arm_vcmpcsq_m(__a, __b, __p)
#define vcmpcsq_m_n(__a, __b, __p) __arm_vcmpcsq_m_n(__a, __b, __p)
#define vclzq_m(__inactive, __a, __p) __arm_vclzq_m(__inactive, __a, __p)
#define vaddvaq_p(__a, __b, __p) __arm_vaddvaq_p(__a, __b, __p)
#define vsriq(__a, __b, __imm) __arm_vsriq(__a, __b, __imm)
#define vsliq(__a, __b, __imm) __arm_vsliq(__a, __b, __imm)
#define vshlq_m_r(__a, __b, __p) __arm_vshlq_m_r(__a, __b, __p)
#define vrshlq_m_n(__a, __b, __p) __arm_vrshlq_m_n(__a, __b, __p)
#define vqshlq_m_r(__a, __b, __p) __arm_vqshlq_m_r(__a, __b, __p)
#define vqrshlq_m_n(__a, __b,
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
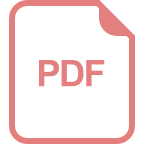
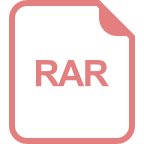
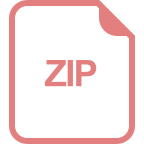
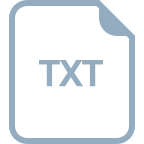
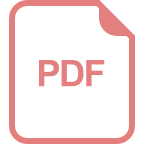
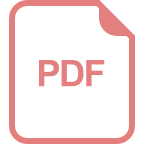
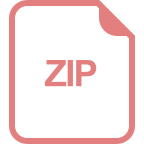
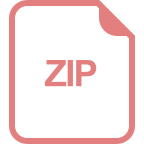
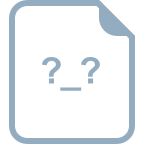
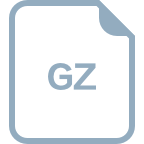
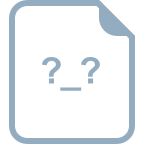
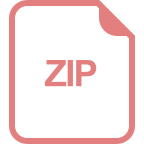
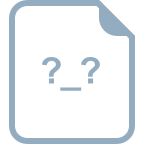
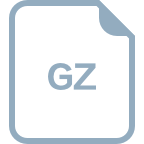
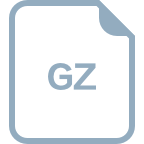
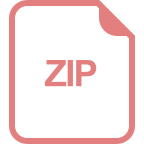
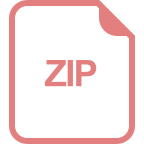
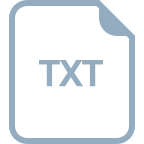
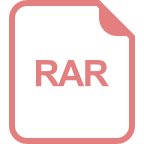
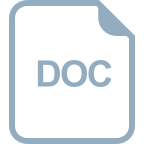
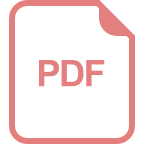
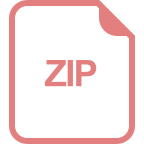
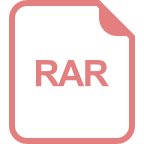
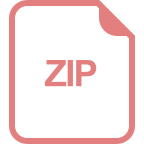
收起资源包目录

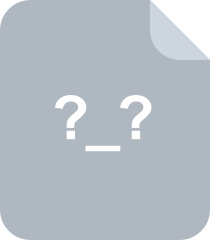
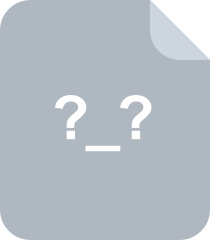
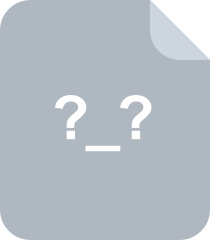
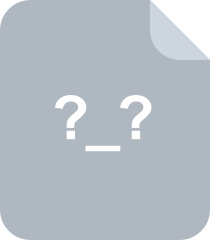
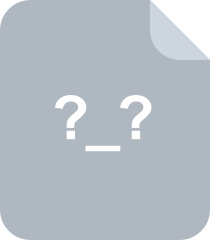
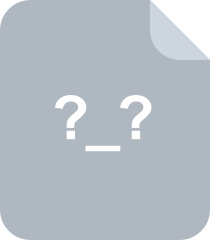
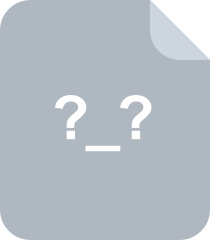
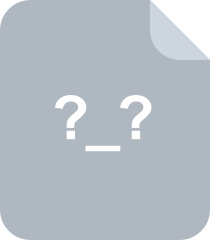
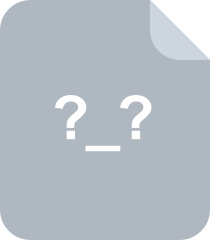
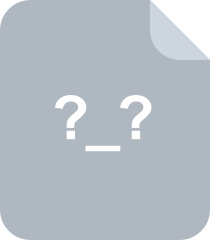
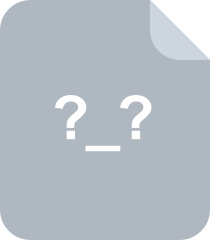
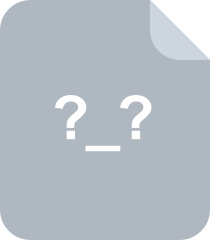
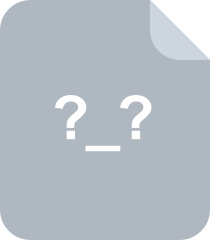
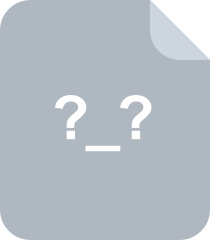
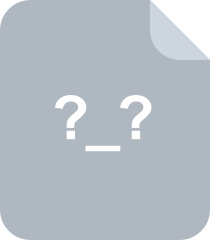
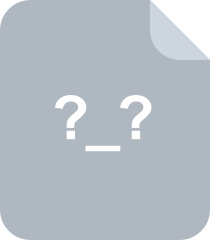
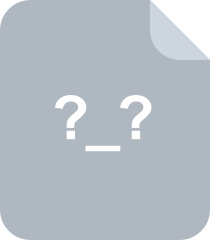
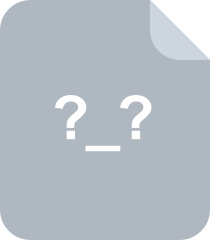
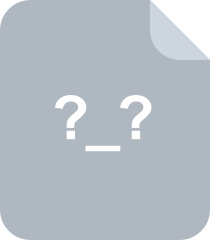
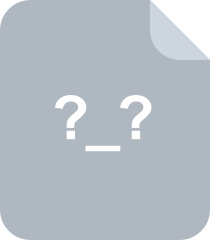
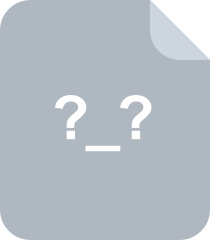
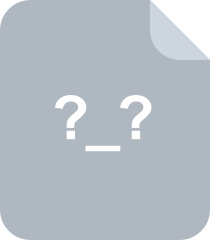
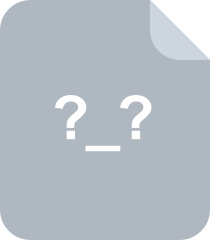
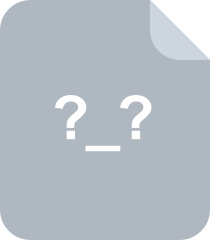
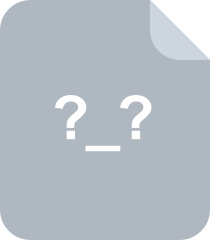
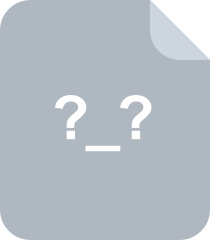
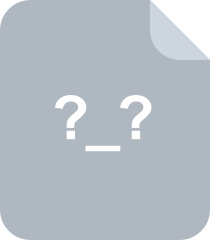
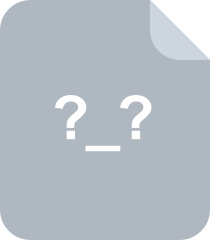
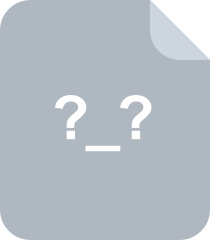
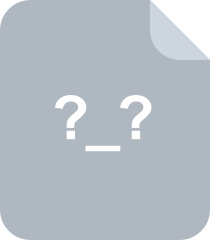
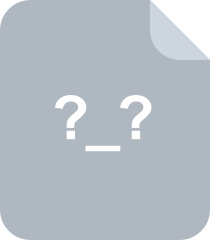
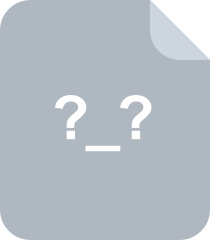
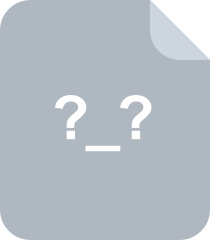
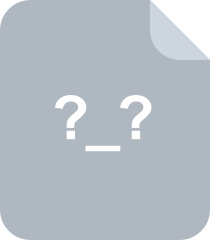
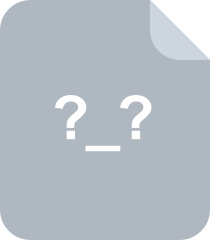
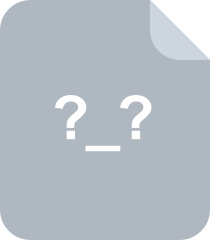
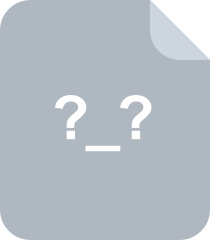
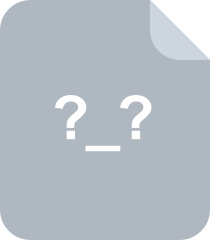
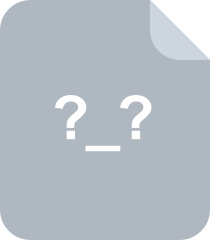
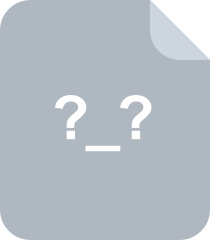
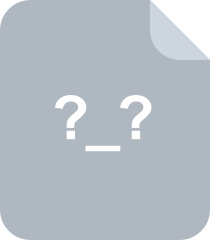
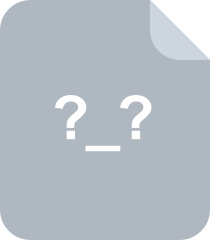
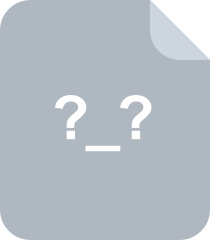
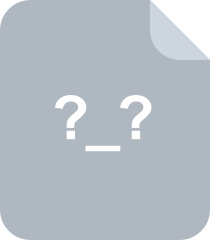
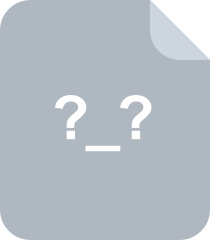
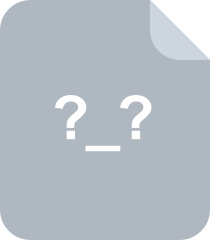
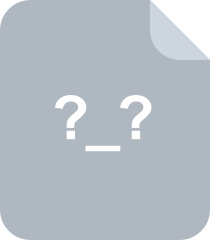
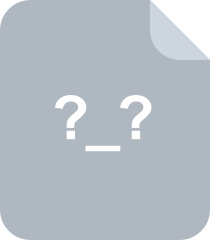
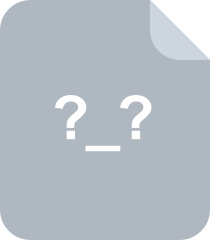
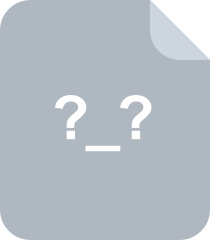
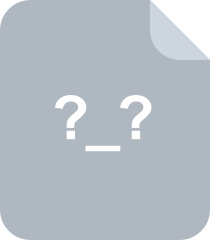
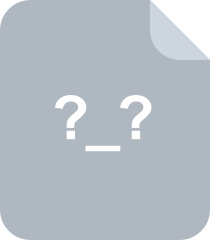
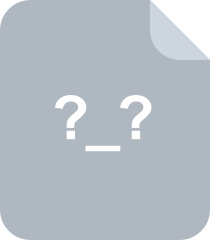
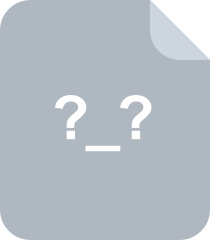
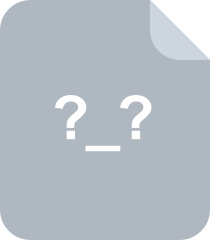
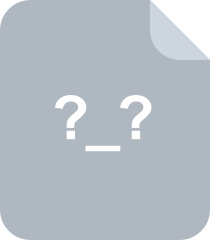
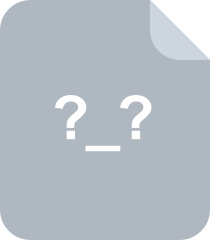
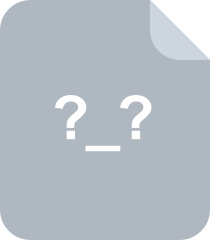
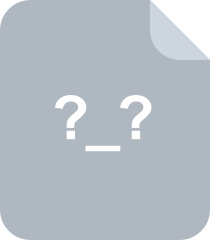
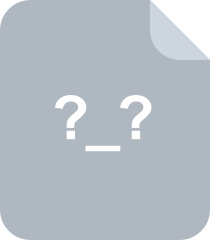
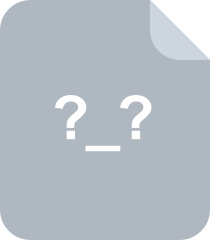
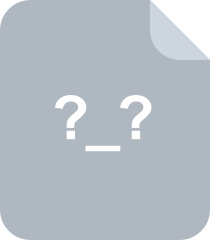
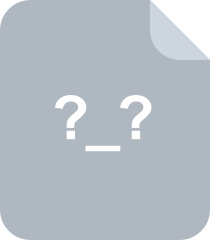
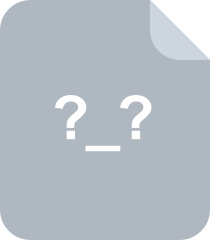
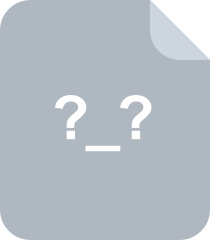
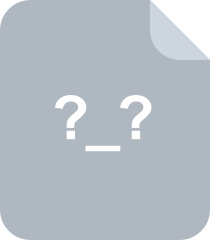
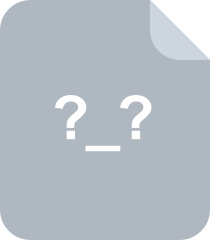
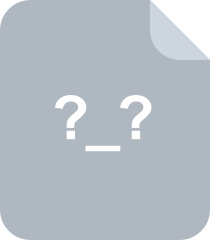
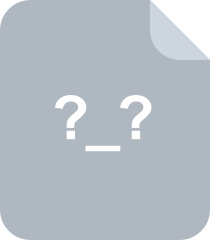
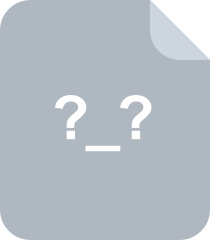
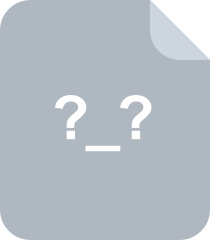
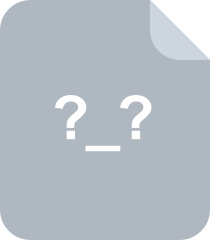
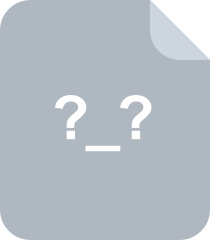
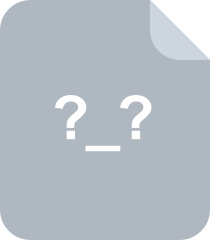
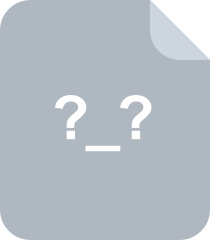
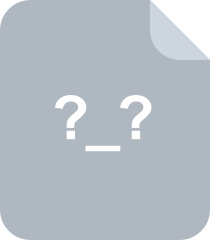
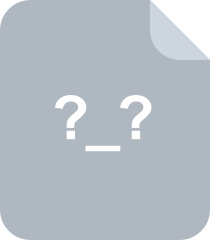
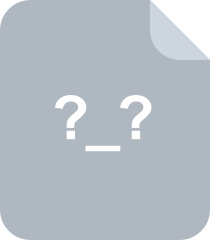
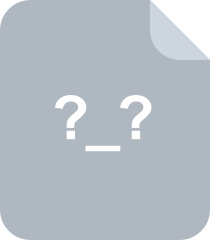
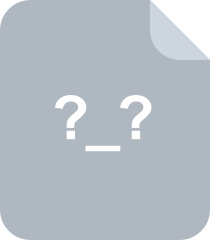
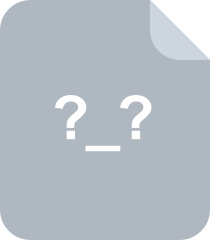
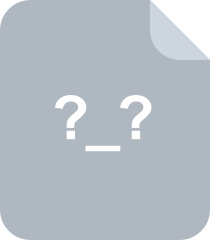
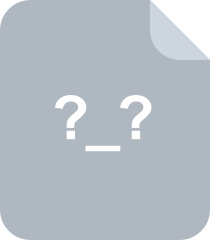
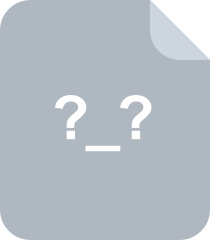
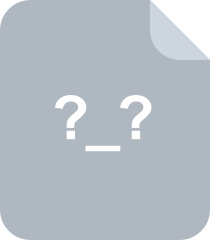
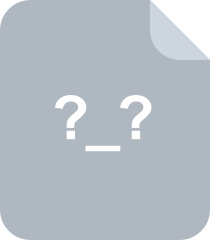
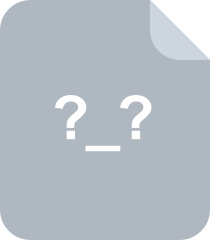
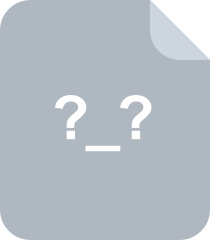
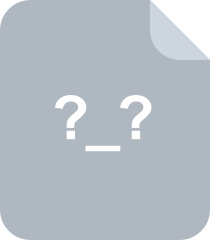
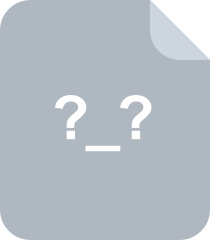
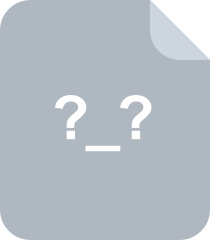
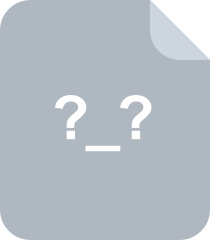
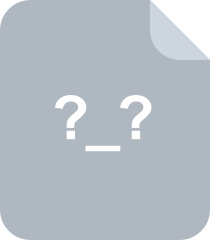
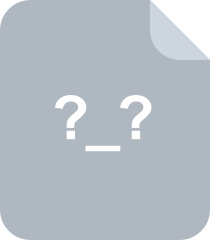
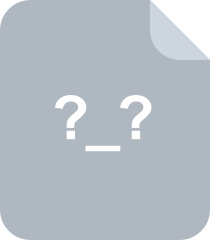
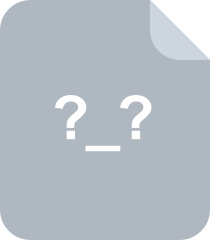
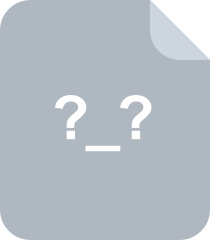
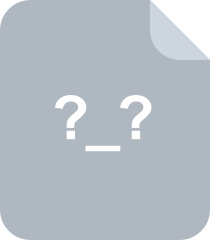
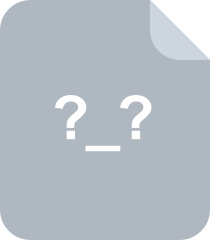
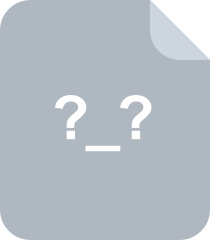
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
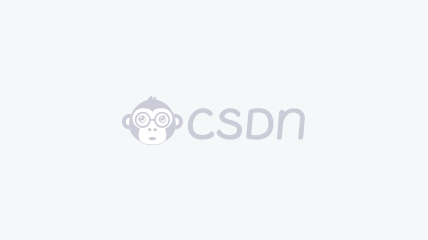


酥稣苏
- 粉丝: 23
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

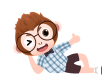
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


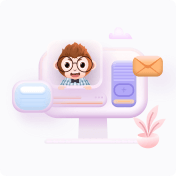
安全验证
文档复制为VIP权益,开通VIP直接复制
