/*
* Summary: internal data structures, constants and functions
* Description: Internal data structures, constants and functions used
* by the XSLT engine.
* They are not part of the API or ABI, i.e. they can change
* without prior notice, use carefully.
*
* Copy: See Copyright for the status of this software.
*
* Author: Daniel Veillard
*/
#ifndef __XML_XSLT_INTERNALS_H__
#define __XML_XSLT_INTERNALS_H__
#include <libxml/tree.h>
#include <libxml/hash.h>
#include <libxml/xpath.h>
#include <libxml/xmlerror.h>
#include <libxml/dict.h>
#include <libxml/xmlstring.h>
#include <libxslt/xslt.h>
#include "xsltexports.h"
#include "xsltlocale.h"
#include "numbersInternals.h"
#ifdef __cplusplus
extern "C" {
#endif
/* #define XSLT_DEBUG_PROFILE_CACHE */
/**
* XSLT_IS_TEXT_NODE:
*
* check if the argument is a text node
*/
#define XSLT_IS_TEXT_NODE(n) ((n != NULL) && \
(((n)->type == XML_TEXT_NODE) || \
((n)->type == XML_CDATA_SECTION_NODE)))
/**
* XSLT_MARK_RES_TREE_FRAG:
*
* internal macro to set up tree fragments
*/
#define XSLT_MARK_RES_TREE_FRAG(n) \
(n)->name = (char *) xmlStrdup(BAD_CAST " fake node libxslt");
/**
* XSLT_IS_RES_TREE_FRAG:
*
* internal macro to test tree fragments
*/
#define XSLT_IS_RES_TREE_FRAG(n) \
((n != NULL) && ((n)->type == XML_DOCUMENT_NODE) && \
((n)->name != NULL) && ((n)->name[0] == ' '))
/**
* XSLT_REFACTORED_KEYCOMP:
*
* Internal define to enable on-demand xsl:key computation.
* That's the only mode now but the define is kept for compatibility
*/
#define XSLT_REFACTORED_KEYCOMP
/**
* XSLT_FAST_IF:
*
* Internal define to enable usage of xmlXPathCompiledEvalToBoolean()
* for XSLT "tests"; e.g. in <xsl:if test="/foo/bar">
*/
#define XSLT_FAST_IF
/**
* XSLT_REFACTORED:
*
* Internal define to enable the refactored parts of Libxslt.
*/
/* #define XSLT_REFACTORED */
/* ==================================================================== */
/**
* XSLT_REFACTORED_VARS:
*
* Internal define to enable the refactored variable part of libxslt
*/
#define XSLT_REFACTORED_VARS
#ifdef XSLT_REFACTORED
extern const xmlChar *xsltXSLTAttrMarker;
/* TODO: REMOVE: #define XSLT_REFACTORED_EXCLRESNS */
/* TODO: REMOVE: #define XSLT_REFACTORED_NSALIAS */
/**
* XSLT_REFACTORED_XSLT_NSCOMP
*
* Internal define to enable the pointer-comparison of
* namespaces of XSLT elements.
*/
/* #define XSLT_REFACTORED_XSLT_NSCOMP */
/**
* XSLT_REFACTORED_XPATHCOMP:
*
* Internal define to enable the optimization of the
* compilation of XPath expressions.
*/
#define XSLT_REFACTORED_XPATHCOMP
#ifdef XSLT_REFACTORED_XSLT_NSCOMP
extern const xmlChar *xsltConstNamespaceNameXSLT;
/**
* IS_XSLT_ELEM_FAST:
*
* quick test to detect XSLT elements
*/
#define IS_XSLT_ELEM_FAST(n) \
(((n) != NULL) && ((n)->ns != NULL) && \
((n)->ns->href == xsltConstNamespaceNameXSLT))
/**
* IS_XSLT_ATTR_FAST:
*
* quick test to detect XSLT attributes
*/
#define IS_XSLT_ATTR_FAST(a) \
(((a) != NULL) && ((a)->ns != NULL) && \
((a)->ns->href == xsltConstNamespaceNameXSLT))
/**
* XSLT_HAS_INTERNAL_NSMAP:
*
* check for namespace mapping
*/
#define XSLT_HAS_INTERNAL_NSMAP(s) \
(((s) != NULL) && ((s)->principal) && \
((s)->principal->principalData) && \
((s)->principal->principalData->nsMap))
/**
* XSLT_GET_INTERNAL_NSMAP:
*
* get pointer to namespace map
*/
#define XSLT_GET_INTERNAL_NSMAP(s) ((s)->principal->principalData->nsMap)
#else /* XSLT_REFACTORED_XSLT_NSCOMP */
/**
* IS_XSLT_ELEM_FAST:
*
* quick check whether this is an xslt element
*/
#define IS_XSLT_ELEM_FAST(n) \
(((n) != NULL) && ((n)->ns != NULL) && \
(xmlStrEqual((n)->ns->href, XSLT_NAMESPACE)))
/**
* IS_XSLT_ATTR_FAST:
*
* quick check for xslt namespace attribute
*/
#define IS_XSLT_ATTR_FAST(a) \
(((a) != NULL) && ((a)->ns != NULL) && \
(xmlStrEqual((a)->ns->href, XSLT_NAMESPACE)))
#endif /* XSLT_REFACTORED_XSLT_NSCOMP */
/**
* XSLT_REFACTORED_MANDATORY_VERSION:
*
* TODO: Currently disabled to surpress regression test failures, since
* the old behaviour was that a missing version attribute
* produced a only a warning and not an error, which was incerrect.
* So the regression tests need to be fixed if this is enabled.
*/
/* #define XSLT_REFACTORED_MANDATORY_VERSION */
/**
* xsltPointerList:
*
* Pointer-list for various purposes.
*/
typedef struct _xsltPointerList xsltPointerList;
typedef xsltPointerList *xsltPointerListPtr;
struct _xsltPointerList {
void **items;
int number;
int size;
};
#endif
/**
* XSLT_REFACTORED_PARSING:
*
* Internal define to enable the refactored parts of Libxslt
* related to parsing.
*/
/* #define XSLT_REFACTORED_PARSING */
/**
* XSLT_MAX_SORT:
*
* Max number of specified xsl:sort on an element.
*/
#define XSLT_MAX_SORT 15
/**
* XSLT_PAT_NO_PRIORITY:
*
* Specific value for pattern without priority expressed.
*/
#define XSLT_PAT_NO_PRIORITY -12345789
/**
* xsltRuntimeExtra:
*
* Extra information added to the transformation context.
*/
typedef struct _xsltRuntimeExtra xsltRuntimeExtra;
typedef xsltRuntimeExtra *xsltRuntimeExtraPtr;
struct _xsltRuntimeExtra {
void *info; /* pointer to the extra data */
xmlFreeFunc deallocate; /* pointer to the deallocation routine */
union { /* dual-purpose field */
void *ptr; /* data not needing deallocation */
int ival; /* integer value storage */
} val;
};
/**
* XSLT_RUNTIME_EXTRA_LST:
* @ctxt: the transformation context
* @nr: the index
*
* Macro used to access extra information stored in the context
*/
#define XSLT_RUNTIME_EXTRA_LST(ctxt, nr) (ctxt)->extras[(nr)].info
/**
* XSLT_RUNTIME_EXTRA_FREE:
* @ctxt: the transformation context
* @nr: the index
*
* Macro used to free extra information stored in the context
*/
#define XSLT_RUNTIME_EXTRA_FREE(ctxt, nr) (ctxt)->extras[(nr)].deallocate
/**
* XSLT_RUNTIME_EXTRA:
* @ctxt: the transformation context
* @nr: the index
*
* Macro used to define extra information stored in the context
*/
#define XSLT_RUNTIME_EXTRA(ctxt, nr, typ) (ctxt)->extras[(nr)].val.typ
/**
* xsltTemplate:
*
* The in-memory structure corresponding to an XSLT Template.
*/
typedef struct _xsltTemplate xsltTemplate;
typedef xsltTemplate *xsltTemplatePtr;
struct _xsltTemplate {
struct _xsltTemplate *next;/* chained list sorted by priority */
struct _xsltStylesheet *style;/* the containing stylesheet */
xmlChar *match; /* the matching string */
float priority; /* as given from the stylesheet, not computed */
const xmlChar *name; /* the local part of the name QName */
const xmlChar *nameURI; /* the URI part of the name QName */
const xmlChar *mode;/* the local part of the mode QName */
const xmlChar *modeURI;/* the URI part of the mode QName */
xmlNodePtr content; /* the template replacement value */
xmlNodePtr elem; /* the source element */
/*
* TODO: @inheritedNsNr and @inheritedNs won't be used in the
* refactored code.
*/
int inheritedNsNr; /* number of inherited namespaces */
xmlNsPtr *inheritedNs;/* inherited non-excluded namespaces */
/* Profiling informations */
int nbCalls; /* the number of time the template was called */
unsigned long time; /* the time spent in this template */
void *params; /* xsl:param instructions */
int templNr; /* Nb of templates in the stack */
int templMax; /* Size of the templtes stack */
xsltTemplatePtr *templCalledTab; /* templates called */
int *templCountTab; /* .. and how often */
};
/**
* xsltDecimalFormat:
*
* Data structure of decimal-format.
*/
typedef struct _xsltDecimalFormat xsltDecimalFormat;
typedef xsltDecimalFormat *xsltDecimalFormatPtr;
struct _xsltDecimalFormat {
struct _xsltDecimalFormat *next; /* chained list */
xmlChar *name;
/*
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
一、摘要 主要内容:python数据可视化大屏; 适用人群:Python初学者,数据分析师,或有志从事数据分析工作的人员; 准备软件:Anaconda(Spyder:代码编译)或Pycharm、Navicat Premium 12(数据库)。 二、内容 1、Pyecharts图表; 2、连接数据库(bartest.py含数据库连接代码); 3、大屏看板-监控中心。 三、使用库 1、pyecharts 2、pymysql 3、BeautifulSoup 4、operator
资源推荐
资源详情
资源评论
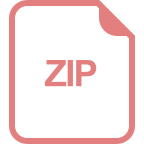
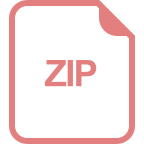
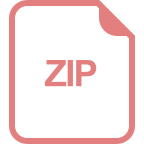
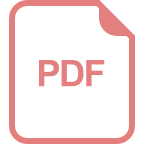
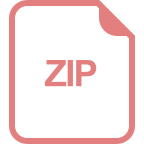
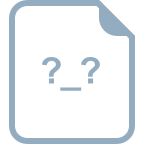
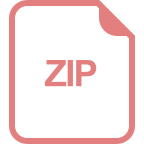
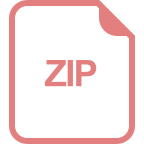
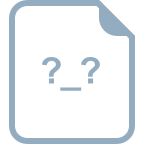
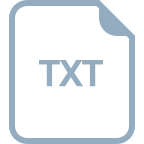
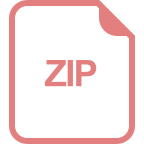
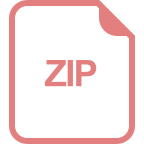
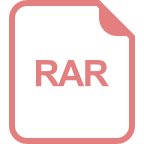
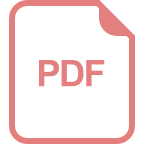
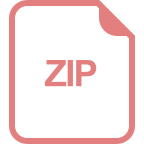
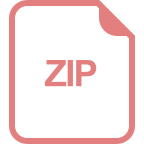
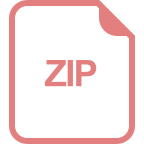
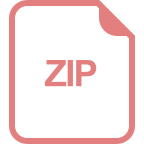
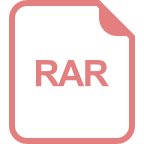
收起资源包目录

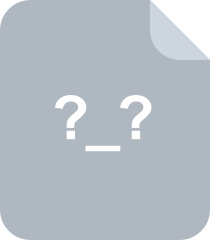
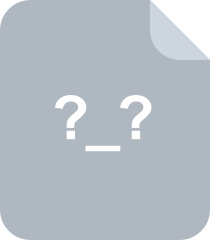
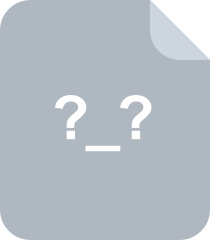
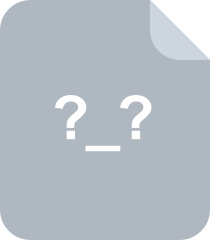
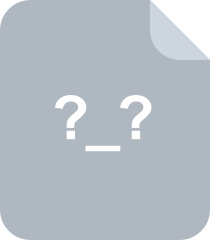
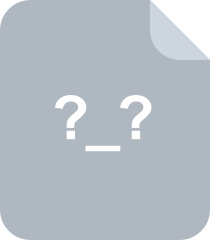
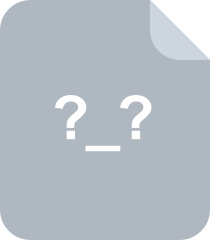
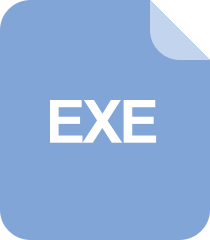
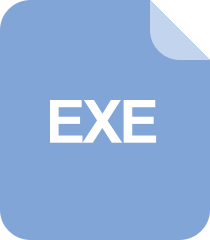
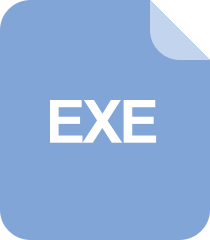
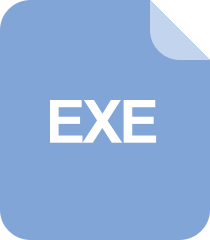
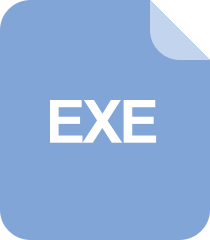
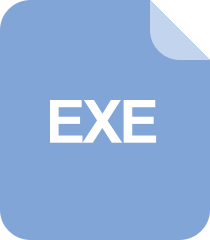
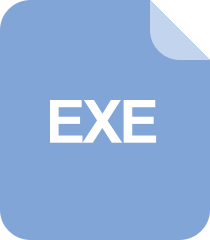
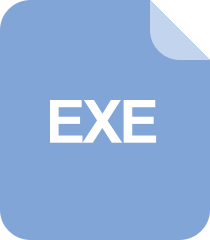
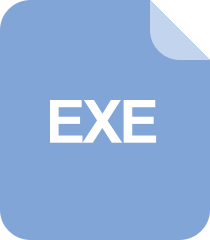
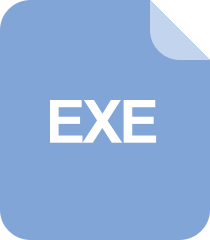
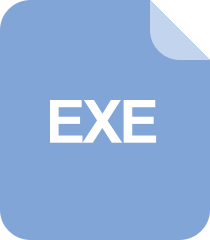
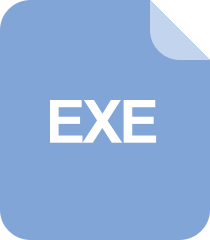
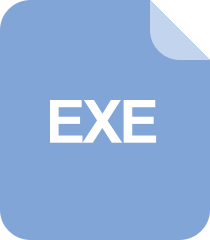
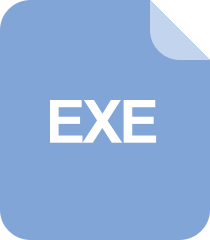
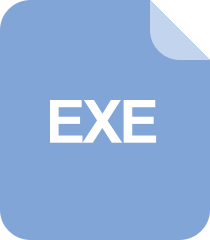
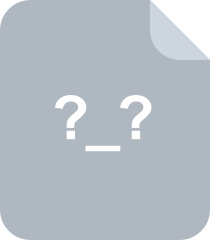
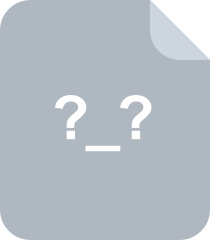
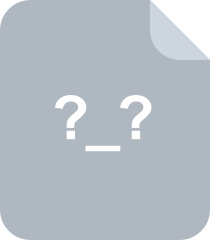
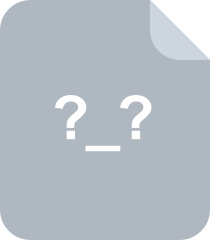
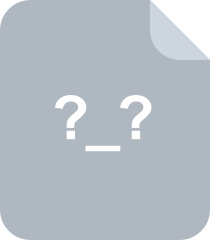
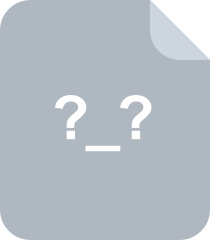
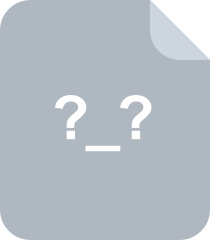
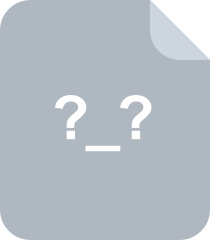
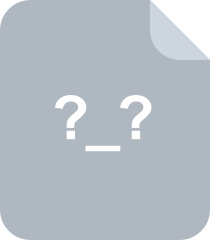
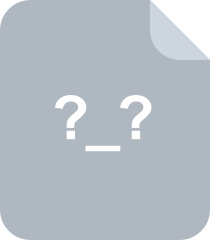
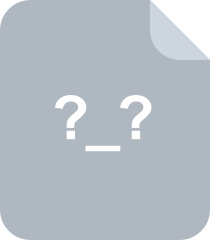
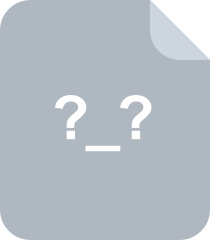
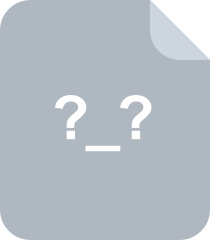
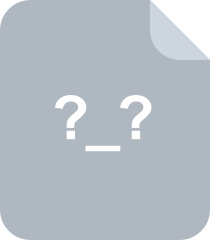
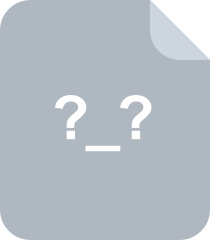
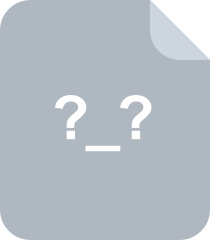
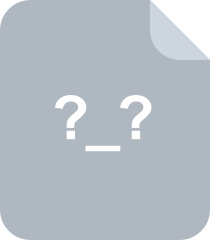
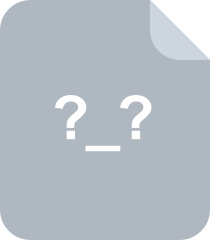
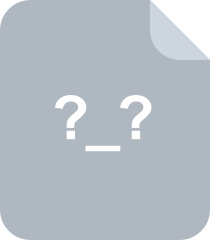
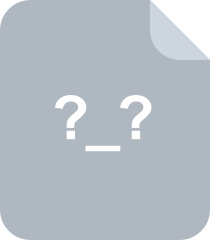
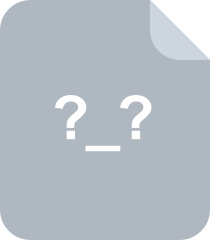
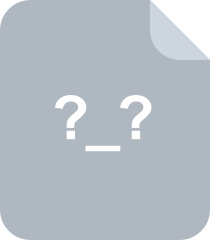
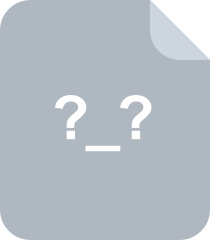
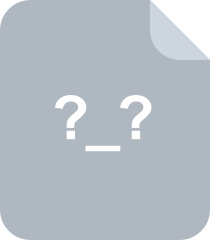
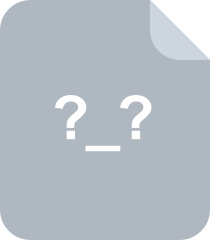
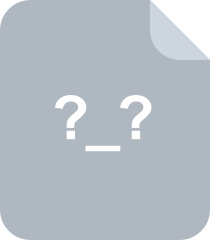
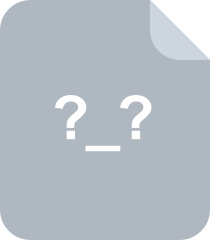
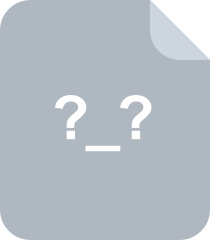
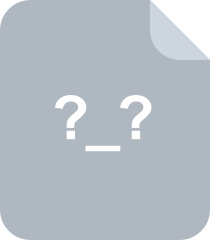
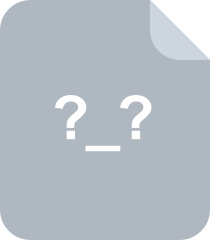
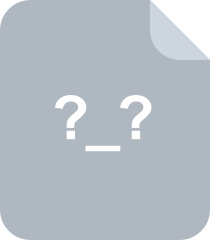
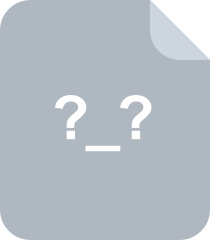
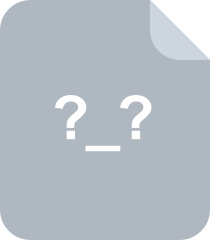
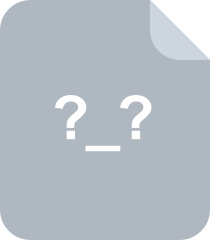
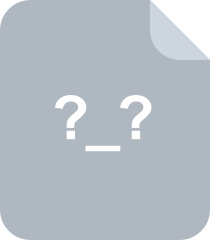
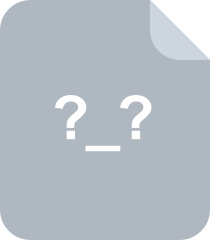
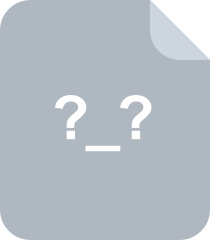
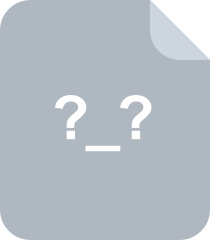
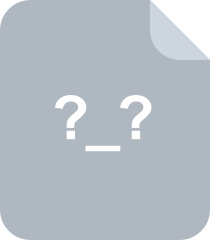
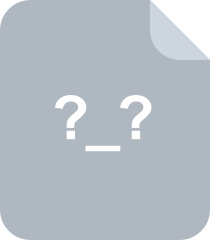
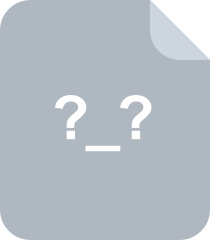
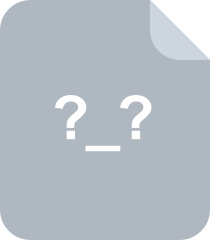
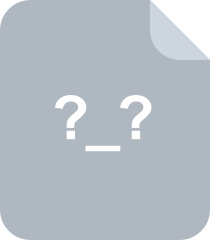
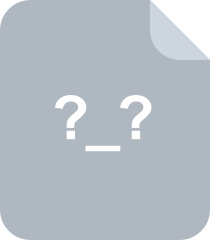
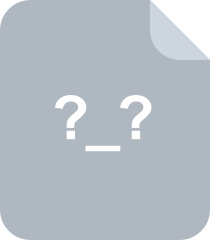
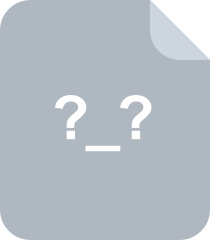
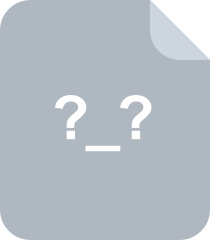
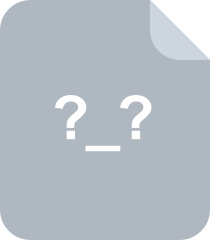
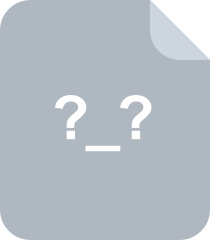
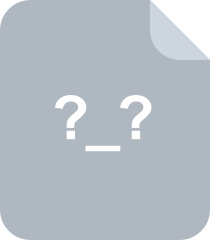
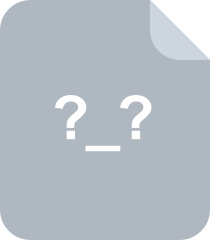
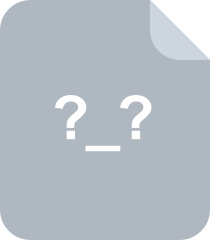
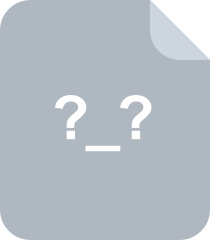
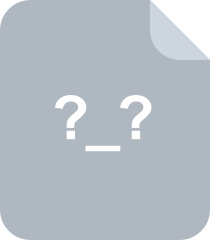
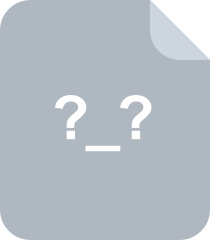
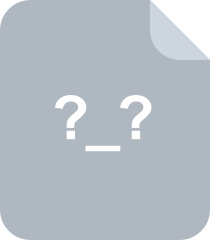
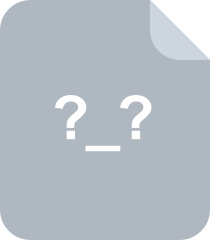
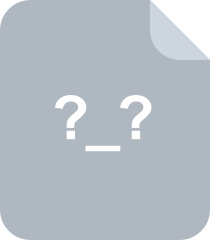
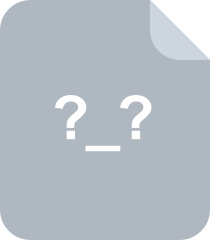
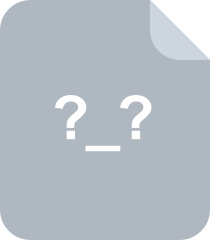
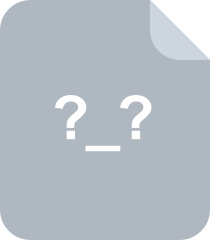
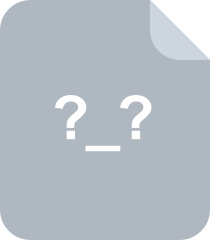
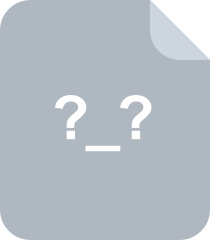
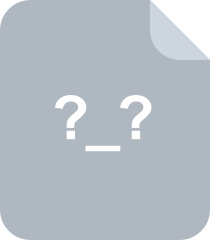
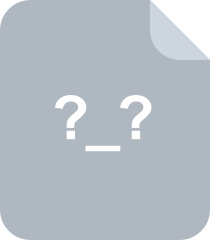
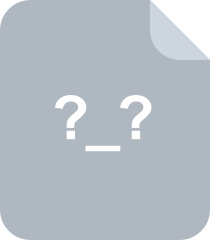
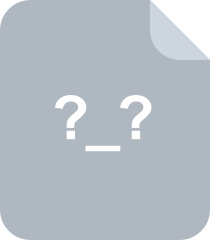
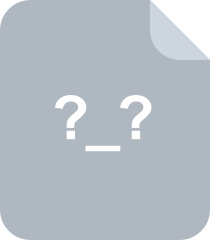
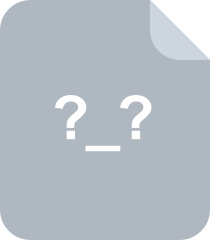
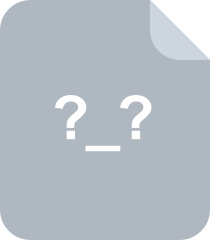
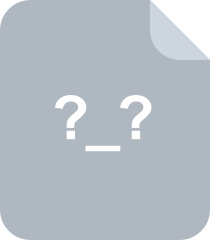
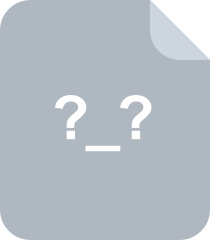
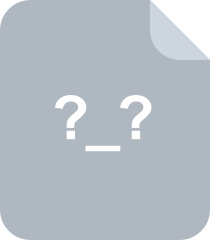
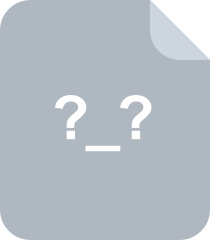
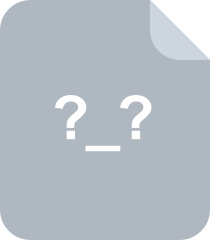
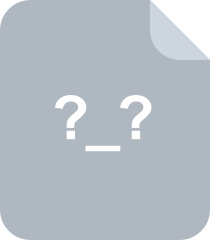
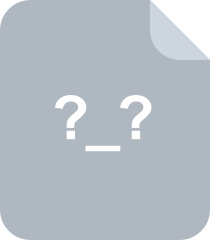
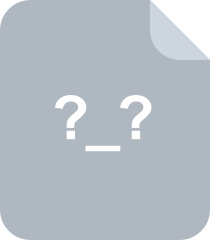
共 1653 条
- 1
- 2
- 3
- 4
- 5
- 6
- 17

Officetouch数据科学
- 粉丝: 1071
- 资源: 3

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

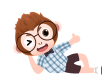
最新资源
- 适用于 Python 的 LINE 消息 API SDK.zip
- 适用于 Python 的 AWS 开发工具包.zip
- 适用于 Python 3 的 Django LDAP 用户身份验证后端 .zip
- 基于PBL-CDIO的材料成型及控制工程课程设计实践与改革
- JQuerymobilea4中文手册CHM版最新版本
- 适用于 Python 2 和 3 以及 PyPy (ws4py 0.5.1) 的 WebSocket 客户端和服务器库.zip
- 适用于 AWS 的 Python 无服务器微框架.zip
- 适用于 Apache Cassandra 的 DataStax Python 驱动程序.zip
- WebAPI-案例-年会抽奖.html
- 这里有一些基础问题和一些棘手问题的解答 还有hackerrank,hackerearth,codechef问题的解答 .zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


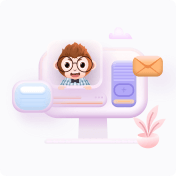
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页