package main
import (
"crypto/md5"
"fmt"
"io"
"net/http"
"os"
"path/filepath"
"github.com/gin-gonic/gin"
)
type Config struct {
MaxFileSize int64 `json:"max_file_size"`
AllowedExts []string `json:"allowed_exts"`
UploadPath string `json:"upload_path"`
HttpUlr string `json:"http_url"`
}
func loadConfig() (*Config, error) {
// 加载配置文件,定义各项参数
return &Config{
MaxFileSize: 2 * 1024 * 1024, // 文件最大2MB
AllowedExts: []string{".jpg", ".jpeg", ".png", ".gif"}, // 允许的文件类型
UploadPath: "/www/wwwroot/website/upload/", // 图片上传存放目录
HttpUlr: "http://127.0.0.1:11259/upload/", // 图片访问URL
}, nil
}
func isFileAllowed(fileName string, allowedExts []string) bool {
ext := filepath.Ext(fileName)
for _, allowedExt := range allowedExts {
if ext == allowedExt {
return true
}
}
return false
}
func handleUpload(c *gin.Context) {
// 添加header token验证
token := c.Request.Header.Get("Authorization")
if token != "e583005c4b99107633bbe93a9fe59f83" {
c.JSON(http.StatusBadRequest, gin.H{"success": false, "message": "Invalid token"})
return
}
// 获取上传文件和其他表单数据
file, header, err := c.Request.FormFile("upload_file")
if err != nil {
c.JSON(http.StatusBadRequest, gin.H{"success": false, "message": "Failed to fetch file"})
return
}
defer file.Close()
// 检查文件大小是否超过限制
config, err := loadConfig()
if err != nil {
c.JSON(http.StatusInternalServerError, gin.H{"success": false, "message": "Failed to load config"})
return
}
if header.Size > config.MaxFileSize {
c.JSON(http.StatusBadRequest, gin.H{"success": false, "message": "File too large"})
return
}
// 检查文件类型是否符合要求
if !isFileAllowed(header.Filename, config.AllowedExts) {
c.JSON(http.StatusBadRequest, gin.H{"success": false, "message": "Invalid file type"})
return
}
// 根据上传表单的名称生成存储路径和文件名
fileName := header.Filename
// 保存文件时将文件名进行哈希,避免文件名重复
fileName = fmt.Sprintf("%x", md5.Sum([]byte(fileName))) + filepath.Ext(fileName)
filePath := filepath.Join(config.UploadPath, fileName)
// 创建一个新文件并将上传数据写入该文件中
newFile, err := os.Create(filePath)
if err != nil {
c.JSON(http.StatusInternalServerError, gin.H{"success": false, "message": "Failed to create file"})
return
}
defer newFile.Close()
_, err = io.Copy(newFile, file)
if err != nil {
c.JSON(http.StatusInternalServerError, gin.H{"success": false, "message": "Failed to save file"})
return
}
// 响应 JSON 数据给客户端
c.JSON(http.StatusOK, gin.H{"success": true, "message": "", "url": config.HttpUlr + fileName})
}
func main() {
r := gin.New()
r.Use(gin.Logger())
r.Use(gin.Recovery()) // 添加 Recovery 中间件
r.POST("/upload", handleUpload)
r.Run(":11258")
}
没有合适的资源?快使用搜索试试~ 我知道了~
Typora 图片上传插件 golang
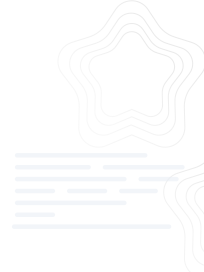
共10个文件
sum:2个
bat:2个
go:2个

需积分: 5 0 下载量 154 浏览量
2023-04-26
15:07:09
上传
评论 1
收藏 24KB ZIP 举报
温馨提示
golang 1.20 除了服务端有个gin没有用别的库,理论上跨平台没问题的,自行测试吧 只支持自建服务器,包含了Typora编辑器插件以及服务端,整个代码以及逻辑都是CHATGPT生成的,我仅仅做了打包处理。 上传接口有header的Authorization验证,勉强可以防止被滥用了。 修改好代码里的相关变量后build,server丢服务器上运行,client.exe放到你想放的地方然后把程序路径完整的复制到Typora 的上传配置那里。 不好的地方就是golang build的文件挺大的,对比了下我自己C++写的大太多了! 纯属娱乐,不喜勿骂哈!
资源推荐
资源详情
资源评论
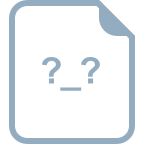
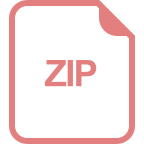
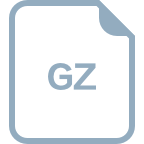
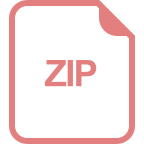
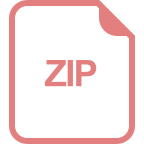
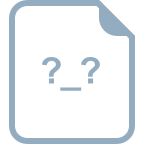
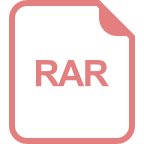
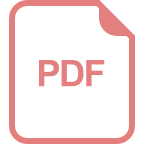
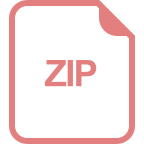
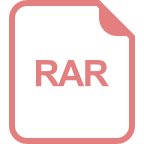
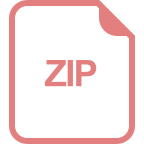
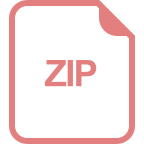
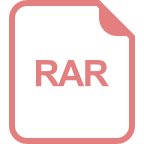
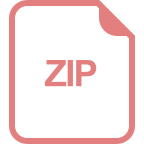
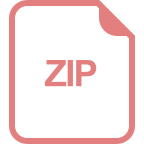
收起资源包目录



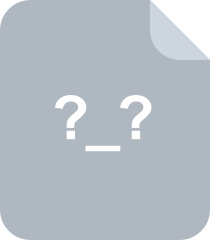
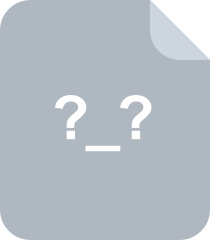
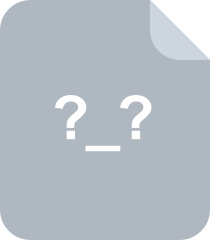
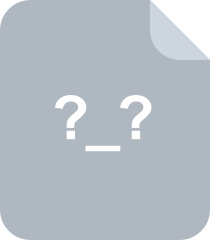
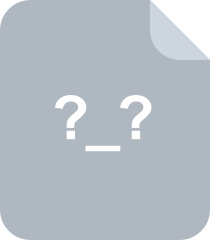

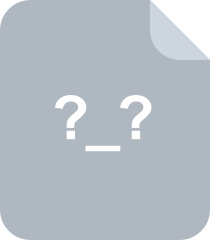
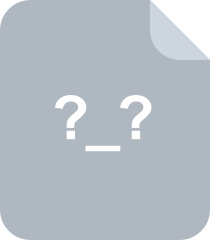
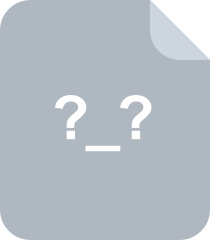
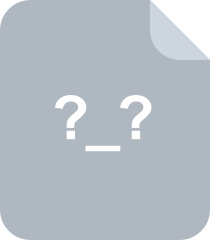
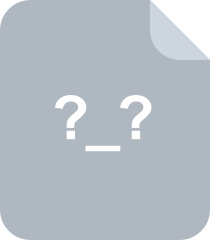
共 10 条
- 1
资源评论
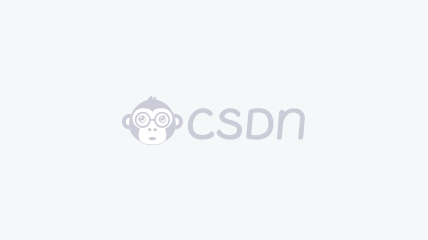

TBOAI
- 粉丝: 7
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

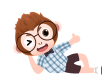
安全验证
文档复制为VIP权益,开通VIP直接复制
