# IRremote Arduino Library
Available as Arduino library "IRremote"
### [Version 2.8.0](https://github.com/z3t0/Arduino-IRremote/releases) - work in progress
[](https://opensource.org/licenses/MIT)
[](https://github.com/z3t0/Arduino-IRremote/commits/master)
[](https://www.ardu-badge.com/IRremote)
[](https://gitter.im/z3t0/Arduino-IRremote?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
[](https://github.com/z3t0/Arduino-IRremote/actions)
This library enables you to send and receive using infra-red signals on an Arduino.
Tutorials and more information will be made available on [the official homepage](http://z3t0.github.io/Arduino-IRremote/).
# Installation
Click on the LibraryManager badge above to see the instructions.
# FAQ
- IR does not work right when I use Neopixels (aka WS2811/WS2812/WS2812B)
Whether you use the Adafruit Neopixel lib, or FastLED, interrupts get disabled on many lower end CPUs like the basic arduinos for longer than 50 us. In turn, this stops the IR interrupt handler from running when it needs to. There are some solutions to this on some processors, [see this page from Marc MERLIN](http://marc.merlins.org/perso/arduino/post_2017-04-03_Arduino-328P-Uno-Teensy3_1-ESP8266-ESP32-IR-and-Neopixels.html)
- The default IR timer on AVR's is timer 2. Since the Arduino Tone library as well as analogWrite() for pin 3 and pin 11 requires timer 2, this functionality cannot be used simultaneously.
- You can use **multiple IR receiver** by just connecting the output pins of several IR receivers together. The IR receivers use an NPN transistor as output device with just a 30k resistor to VCC. This is almost "open collector" and allows connecting of several output pins to one Arduino input pin.
# Supported IR Protocols
Aiwa, BoseWave, Denon, Dish, JVC, Lego, LG, MagiQuest, NEC, Panasonic, RC5, RC6, Samsung, Sanyo, Sharp, Sony, Whynter, (Pronto).<br/>
Protocols can be switched off and on by changing the lines in *IRremote.h*:
```
#define DECODE_<PROTOCOL_NAME> 1
#define SEND_<PROTOCOL_NAME> 1
```
# [Wiki](https://github.com/z3t0/Arduino-IRremote/wiki)
This is a quite old but maybe useful wiki for this library.
# Compile options / macros for this library
To customize the library to different requirements, there are some compile options / makros available.<br/>
Modify it by commenting them out or in, or change the values if applicable. Or define the macro with the -D compiler option for gobal compile (the latter is not possible with the Arduino IDE, so consider to use [Sloeber](https://eclipse.baeyens.it).
| Name | File | Default value | Description |
|-|-|-|-|
| `DEBUG` | IRremote.h | disabled | Enables lots of lovely debug output. |
| `USE_NEC_STANDARD` | IRremote.h | disabled | Use LSB first, address/code schema for encoding. |
| `USE_NO_SEND_PWM` | IRremote.h | disabled | Use no carrier PWM, just simulate an active low receiver signal. |
| `USE_SOFT_SEND_PWM` | IRremote.h | disabled | Use carrier PWM generation in software, instead of hardware PWM. |
| `PULSE_CORRECTION_MICROS` | IRremote.h | 3 | If USE_SOFT_SEND_PWM, this amount is subtracted from the on-time of the pulses. |
| `USE_SPIN_WAIT` | IRremote.h | disabled | If USE_SOFT_SEND_PWM, use spin wait instead of delayMicros(). |
| `RAW_BUFFER_LENGTH` | IRremoteint.h | 101 | Buffer size of raw input buffer. Must be odd! |
| `IR_SEND_DUTY_CYCLE` | IRremoteBoardDefs.h | 30 | Duty cycle of IR send signal. |
| `MICROS_PER_TICK` | IRremoteBoardDefs.h | 50 | Resolution of the raw input buffer data. |
### Modifying library properties with Arduino IDE
First use *Sketch/Show Sketch Folder (Ctrl+K)*.<br/>
If you did not yet stored the example as your own sketch, then you are instantly in the right library folder.<br/>
Otherwise you have to navigate to the parallel `libraries` folder and select the library you want to access.<br/>
In both cases the library files itself are located in the `src` directory.<br/>
### Modifying library properties with Sloeber IDE
If you are using Sloeber as your IDE, you can easily define global symbols with *Properties/Arduino/CompileOptions*.<br/>

# Handling unknown Protocols
## Disclaimer
This library was never designed to handle long codes like the ones used by air conditioners. See [Recording long Infrared Remote control signals with Arduino](https://www.analysir.com/blog/2014/03/19/air-conditioners-problems-recording-long-infrared-remote-control-signals-arduino).<br/>
The main reason is, that it was designed to fit inside MCUs with relatively low levels of resources and was intended to work as a library together with other applications which also require some resources of the MCU to operate.
## Hints
If you do not know which protocol your IR transmitter uses, you have several choices.
- Use the [IRreceiveDumpV2 example](examples/IRreceiveDumpV2) to dump out the IR timing. You can then reproduce/send this timing with the [IRsendRawDemo example](examples/IRsendRawDemo). For **long codes** like from air conditioners, you can **change the length of the input buffer** in [IRremoteInt.h](src/private/IRremoteInt.h#L30).
- If you have a bigger Arduino board at hand (> 100 kByte program space) you can try the [IRremoteDecode example](https://github.com/bengtmartensson/Arduino-DecodeIR/blob/master/examples/IRremoteDecode/IRremoteDecode.ino) of the Arduino library [DecodeIR](https://github.com/bengtmartensson/Arduino-DecodeIR).
- Use [IrScrutinizer](http://www.harctoolbox.org/IrScrutinizer.html). It can automatically generate a send sketch for your protocol by exporting as "Arduino Raw". It supports IRremote, the old [IRLib](https://github.com/cyborg5/IRLib) and [Infrared4Arduino](https://github.com/bengtmartensson/Infrared4Arduino).
- Use the [IRMP AllProtocol example](https://github.com/ukw100/IRMP#allprotocol-example) and you will see some serial output if the protocol is one of the 40 supported protocols.
- To increase strength of sent output signal you can increase the current through the send diode, or use 2 diodes in series, since one IR diode requires only 1.5 volt. Changing `IR_SEND_DUTY_CYCLE` to 50 increases the signal current by 40%.
## Other IR libraries
[Here](https://github.com/ukw100/IRMP#quick-comparison-of-4-arduino-ir-receiving-libraries) you find a **short comparison matrix** of 4 popular Arduino IR libraries.<br/>
[Here](https://github.com/crankyoldgit/IRremoteESP8266) you find an **ESP8266/ESP32** version of IRremote with an **[impressive list of supported protocols](https://github.com/crankyoldgit/IRremoteESP8266/blob/master/SupportedProtocols.md)**.
# Supported Boards
- Arduino Uno / Mega / Leonardo / Duemilanove / Diecimila / LilyPad / Mini / Fio / Nano etc.
- Teensy 1.0 / 1.0++ / 2.0 / 2++ / 3.0 / 3.1 / Teensy-LC; Credits: @PaulStoffregen (Teensy Team)
- Sanguino
- ATmega8, 48, 88, 168, 328
- ATmega8535, 16, 32, 164, 324, 644, 1284,
- ATmega64, 128
- ATmega4809 (Nano every)
- ATtiny84, 85
- SAMD21 (receive only)
- ESP32
- ESP8266 is supported in a fork based on an old codebase that isn't as recent, but it works reasonably well given that perfectly timed sub millisecond interrupts are different on that chip. See https://github.com/markszabo/IRremoteESP8266
- Sparkfun Pro Micro
We are open to suggestions for adding support to new boards, however we highly recommend you contact your supplier first and ask them to provide support from their side.
## Hardware specifications
The timer
没有合适的资源?快使用搜索试试~ 我知道了~
收起资源包目录




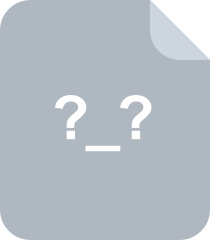
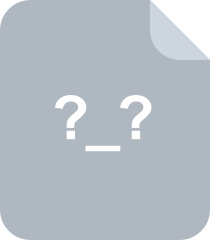
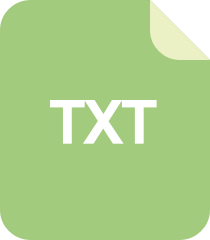

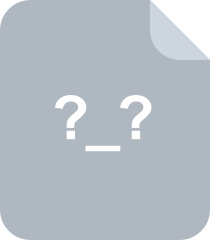
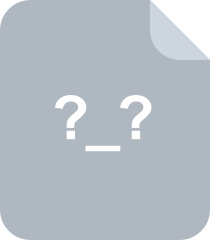
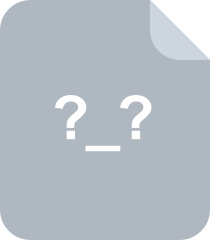
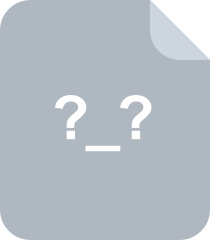
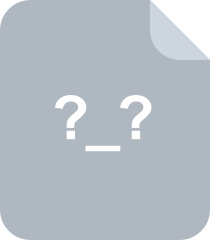
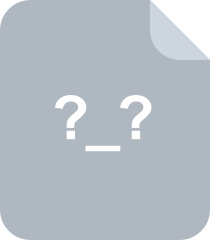
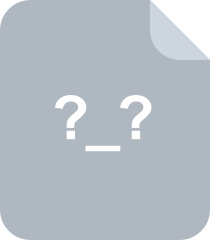
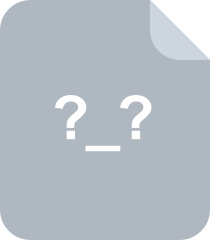
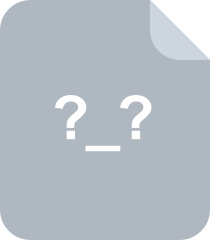
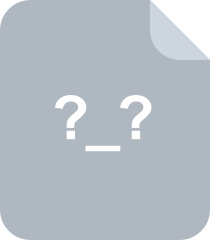
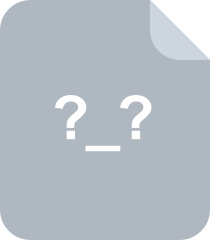
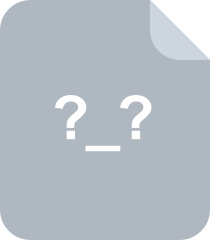
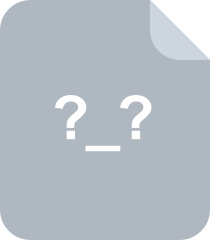
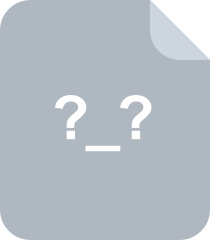
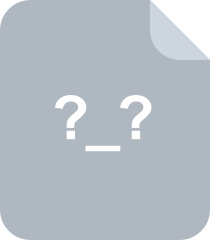
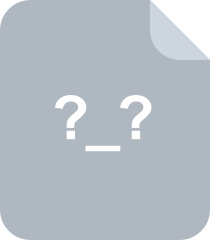
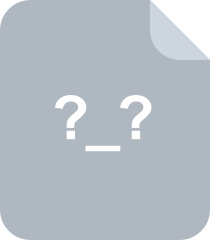
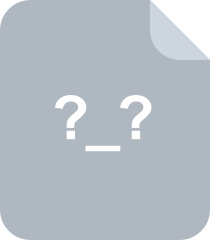
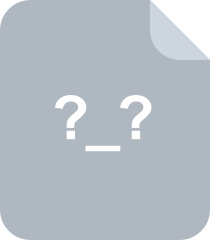
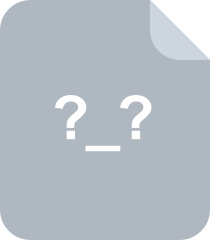
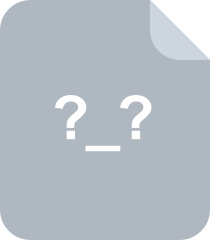
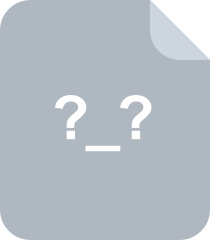
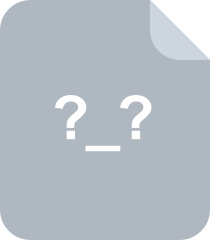
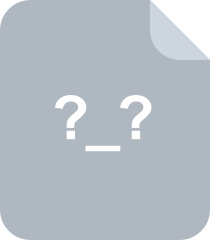
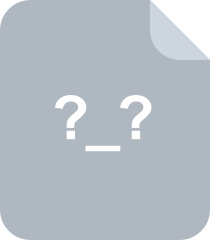

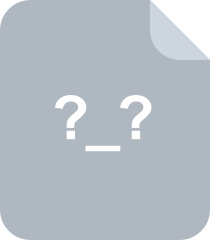
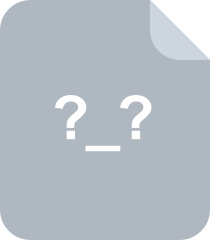
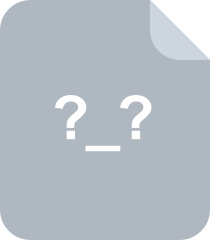
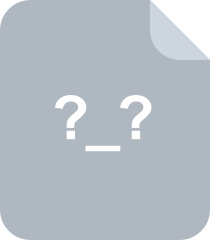
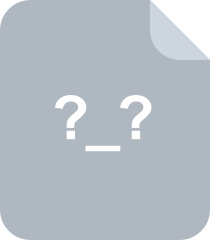


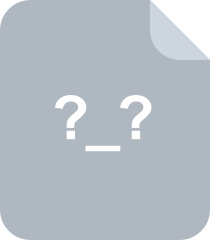

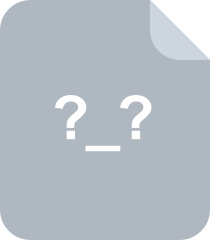

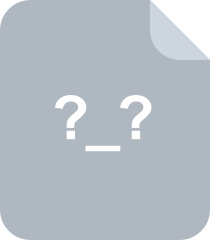

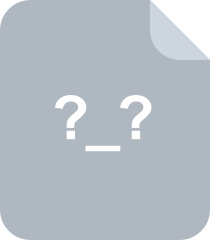

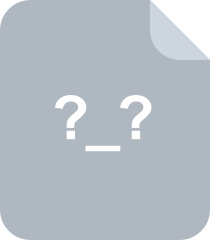

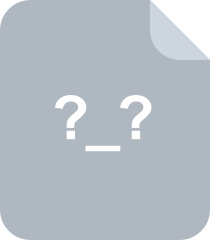

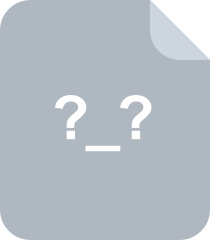

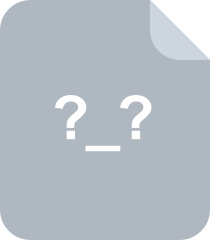

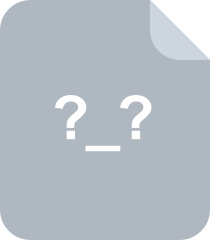

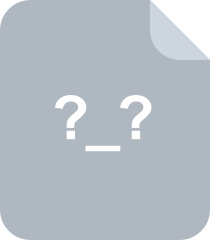

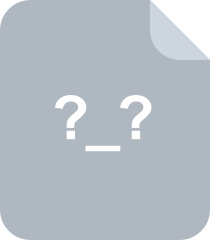
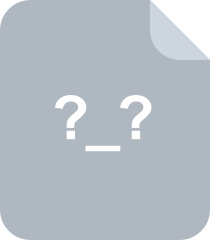

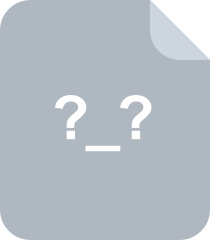

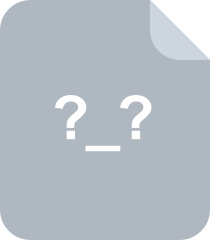

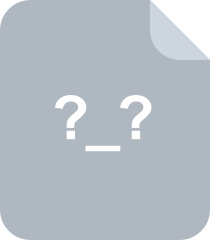

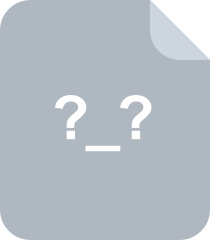

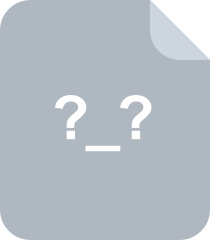

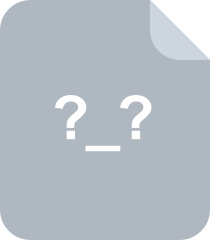

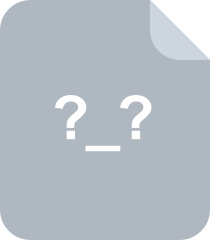

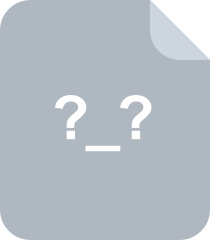
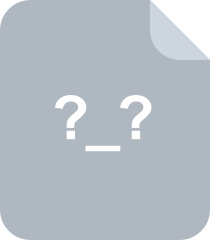
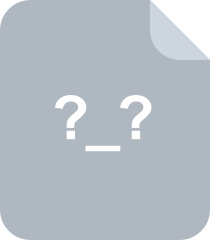
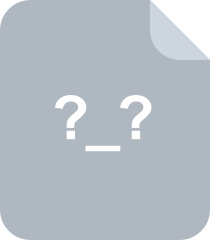
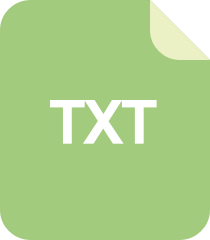
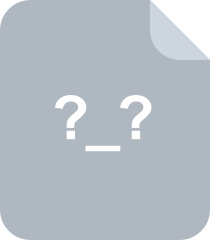
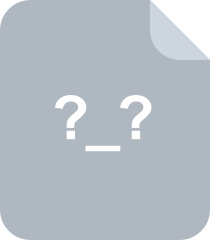
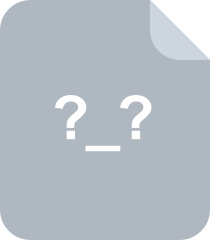
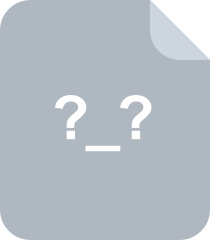
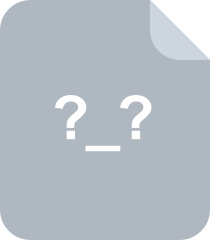
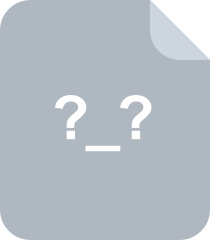
共 63 条
- 1
资源推荐
资源预览
资源评论

128 浏览量
199 浏览量
141 浏览量
2022-09-23 上传

174 浏览量

116 浏览量
2021-05-11 上传

107 浏览量

195 浏览量


181 浏览量
188 浏览量
2021-02-12 上传
114 浏览量
166 浏览量
139 浏览量
126 浏览量

139 浏览量

106 浏览量

140 浏览量

121 浏览量
129 浏览量
2024-09-24 上传
资源评论
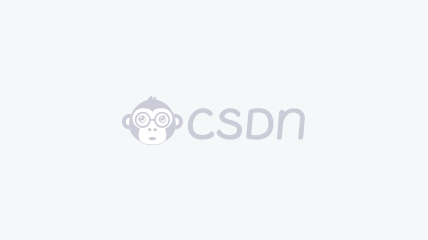


IT永勇
- 粉丝: 999
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

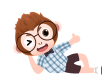
最新资源
- lsb-release,安装磐维数据库,安装oracle数据库等常用的依赖包
- glibc-devel,安装磐维数据库,安装oracle数据库等常用的依赖包
- redhat-lsb-submit-security,安装磐维数据库,安装oracle数据库等常用的依赖包
- 可以在mac下开发的微雪esp32触摸屏开发板的支持包
- redhat-lsb-core,安装磐维数据库,安装oracle数据库等常用的依赖包
- redhat-lsb-core,安装磐维数据库,安装oracle数据库等常用的依赖包
- 非常好的在线聊天系统源代码100%好用.zip
- libpng,安装磐维数据库,安装oracle数据库等常用的依赖包
- 飞机检测12-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord数据集合集.rar
- redhad-lsb,安装磐维数据库,安装oracle数据库等常用的依赖包
安全验证
文档复制为VIP权益,开通VIP直接复制
