# Vive Grip Demo Scene
Load up the scene and press play. It'll give you an idea of what Vive Grip can do with little effort and almost no extra code.
Some things you might want to try:
- Trying to push the heavy box with the light box
- Flipping the bubble gun 360 and catching it on the way down
- Move the light box onto the heavy box without grabbing it
See EXTENSIONS.md for more examples on how to use code hooks to modify the behaviour of Vive Grip.
## How to create the...
### Stack of boxes (easy)
- `ViveGrip_Grabbable`
- `Anchor.enabled`
- `Rotation.mode`
- `Rigidbody`
The boxes are cubes with `ViveGrip_Grabbable` attached. This automatically adds a `Rigidbody` and makes it so I can grip them with my controller's grip point. I changed the mass of each so that Unity's physics engine would handle the details of the Iight difference.
By playing with `Anchor.enabled` and `Rotation.mode` I can get different effects with the boxes. I enabled the anchor for the small box to make it feel more like a small object I am gripping in my palm. I also set the rotation mode to `Disabled` on the big box to give it a feeling of being unweildy or difficult to hold on to.
### Composite Toy (easy)
- `ViveGrip_Grabbable`
- `ViveGrip_Highlighter`
- `ViveGrip_TintChildrenEffect`
Sometimes objects need to be made of multiple renderers or colliders as children. Grabbable will handle "Compound Colliders" by looking in a Collider's parent for a grabbable or interactable if it can't find one in its own object. However, I need to tell Vive Grip to highlight the children of the object as well. I do this by selecting the `ViveGrip_TintChildrenEffect` highlight effect on the grabbable from the Inspector.
### Slider (easy)
- `ViveGrip_Grabbable`
- `Rotation.mode`
- `Rigidbody`
- `Vibrate`
The slider is a cube with `ViveGrip_Grabbable` attached. It's `Rigidbody` is constrained to only move on one axis. To prevent it from going too far, I added two invisible objects with colliders at either end of its movement. The track is just for show and has no collider.
I also set the rotation mode to `Disabled` so that it doesn't bother trying to rotate it with the controller. This isn't strictly necessary due to the constraints but is trivial enough that I might as well.
To give some sense of weight to the slider as it moves, I leverage the `Vibrate` method on the grip point's controller. By providing the duration in milliseconds at the strength of the vibration (from 0 to 1), I provide some feedback based on how far the slider was moved.
### Switch (easy)
- `ViveGrip_Interactable`
- `BoxCollider`
- `ViveGrip_EventBridge`
The switch leverages the power of Vive Grip events from the comfort of Unity's Inspector. To keep things simple, I'm simply going to trigger the `Flip()` method on the switch to toggle its rotation.
By adding the `ViveGrip_Interactable` script, I allow the object to be highlighted and interacted with. It doesn't need to be picked up so a `Rigidbody` won't be automatically added. Instead, I use the default collider.
Adding the `ViveGrip_EventBridge` script let's me bridge Vive Grip events to custom methods through the Inspector. In this case I select the objects and methods to flip the switch and attach it to an "Interaction Start". You can read EXTENSIONS.md for more detailed information on the events available.
The Button and Bubble Gun examples show when you may want to create an interaction in code instead of through Unity's Inspector.
### Manager (intermediate)
- `ViveGrip_JointFactory.LINEAR_DRIVE_MULTIPLIER`
- `ViveGrip_JointFactory.ANGULAR_DRIVE_MULTIPLIER`
- `ViveGrip_Object.highlightEffect`
- `ViveGrip_Highlighter.enabled`
- `ViveGrip_Highlighter.UpdateEffect`
Vive Grip exposes a few variables that you may want to take advantage of for the whole scene instead of an object-to-object basis. For example, here I change the grip strength and object highlighting.
The grip strength is divided into the "linear" and "angular" drive. In simpler terms, these are the positional and rotational strength of all your grips. By changing these variables, I change the strength of each subsequent grab. The defaults are what have been found to be useful in most situations but there can be many benefits to changing this for your unique needs.
Disabling a highlight on an per-object basis can be done easily by changing the `ViveGrip_Object.highlightEffect`. However, because I need to re-enable it to what it was I simply disable the highlighter script. To be able to select a new highlighter for objects in realtime, I call `ViveGrip_Highlighter.UpdateEffect` when Unity validates the dropdown. I may or may not want to do this when the scene starts so I add a boolean to decide.
### Button (intermediate)
- `ViveGrip_Interactable`
- `BoxCollider`
- `ViveGripInteractionStart(ViveGrip_GripPoint gripPoint)`
- `Vibrate`
The button is a cube with `ViveGrip_Interactable` attached. As with the Switch, I use the default collider instead of a `Rigidbody`.
The attached script will move the button in and out when triggered. Since it's done in code, I can use the `ViveGrip_GripPoint` parameter to give immediate haptic feedback to the correct controller with `gripPoint.Vibrate(duration, strength)`.
### Dial and light (intermediate)
- `ViveGrip_Grabbable`
- `Rigidbody`
The dial is a cylinder with `ViveGrip_Grabbable` attached. The `Rigidbody` position is frozen but it rotates freely with a hinge joint. I also set some limits to prevent it from rotating all the way around.
The attached script will read the rotation of the dial and use that to set the light's color.
### Hands (intermediate)
- `ViveGrip_GripPoint`
- `ViveGripTouchStart(ViveGrip_GripPoint gripPoint)`
- `ViveGripTouchStop(ViveGrip_GripPoint gripPoint)`
- `ViveGripGrabStart(ViveGrip_GripPoint gripPoint)`
- `ViveGripGrabStop(ViveGrip_GripPoint gripPoint)`
The hands are a grip point and model attached to the controller tracked objects. To start things off I add a hand model sibling and adjust the grip point anchor to roughly match the palm of the hand.
In order to give visual cues to the player, I change the hand mesh when an object is touched and the hand fades when something is grabbed. The logic is hooked into the methods that get called on the controller and all its children when starting or stopping a touch or grab. There are also a few edge cases that get handled around the hand-at-rest cue.
### Lever (intermediate)
- `ViveGrip_Grabbable`
- `Anchor.localPosition`
- `Rigidbody`
- `HingeJoint`
- `Vibrate`
- `BoxCollider`
The lever is a model with `ViveGrip_Grabbable` attached. To make sure that it gets gripped by the handle, I set the anchor's local position appropriately. When I enable the anchor, a Gizmo in the Scene view gives me a visual cue as to where the anchor will be positioned.
The lever needs to rotate when its pulled, which I achieve with a hinge joint. It behaves as you would expect by configuring the joint's anchor and axis appropriately and setting some `Rigidbody` constraints.
A script also provides a sense of weight using vibrations, similar to the use in the Slider.
Unlike the other examples with a mesh, this uses Compound Colliders (see: https://docs.unity3d.com/Manual/class-Rigidbody.html) to create the collisions from primitive meshes. Everything is handled for you when the Vive Grip scripts are in the parent of the collider game objects.
### Tar ball (intermediate)
- `ViveGrip_Grabbable`
- `ViveGripTouchStart(ViveGrip_GripPoint gripPoint)`
- `ViveGrip_GripPoint.ToggleGrab()`
- `ViveGrip_GripPoint.HoldingSomething()`
- `ViveGrip_GripPoint.enabled`
Sometimes you want an object that gets grabbed or dropped forcefully. The tar ball shows this off by sticking to any unoccupied grip point on touch and disabling it until it gets shaken off.
When the touch method is triggered, I trigger `ToggleGrab()` which I know will grab the tar ball. To prevent the player from dropping
没有合适的资源?快使用搜索试试~ 我知道了~
ViveGrip:用于Unity中SteamVR开发的基于物理的抓取
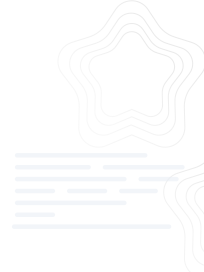
共1062个文件
meta:539个
cs:138个
wav:114个


温馨提示
Vive握把 Vive Grip可帮助您使用SteamVR突出显示,抓取游戏对象并与之互动。 它利用Unity的物理引擎和一个简单的界面来抽象强大的ConfigurableJoint组件。 包括创建加权对象,杠杆,刻度盘,枪支等的示例。 有关软件包的详细信息,快速入门说明和教程,请参见。 有关示例用法和场景,请参见。 有关汇总的代码工具,请参见。 请参阅以获取unitypackage文件。 为什么要使用Vive Grip? 完全支持Unity的物理系统进行逼真的交互 支持HTC Vive,Oculus Rift,Windows Mixed Reality等 广泛的插件系统,可在交互和
资源推荐
资源详情
资源评论
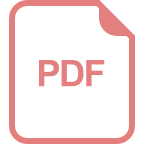
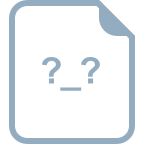
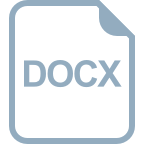
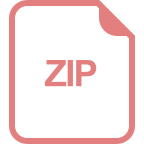
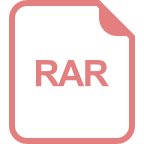
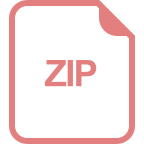
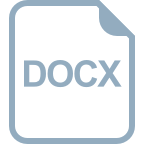
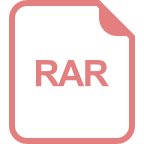
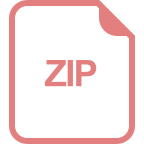
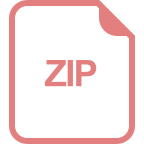
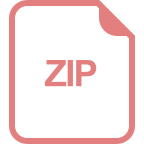
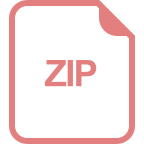
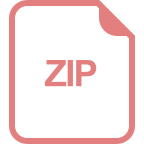
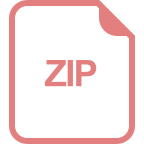
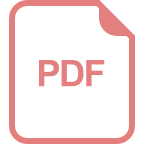
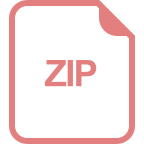
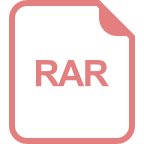
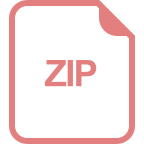
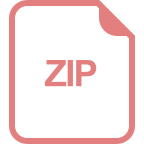
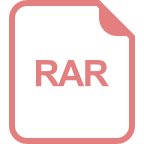
收起资源包目录

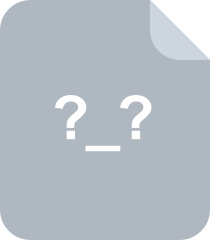
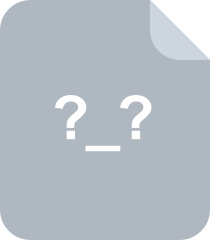
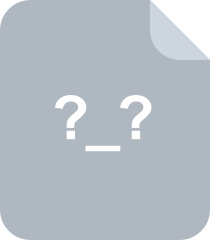
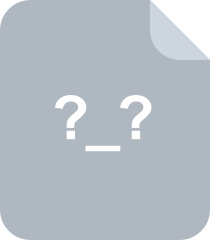
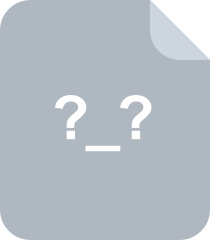
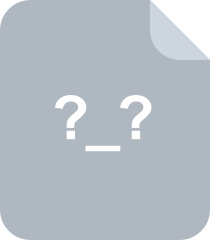
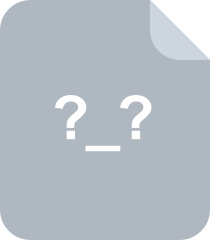
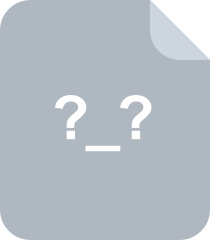
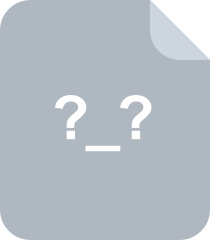
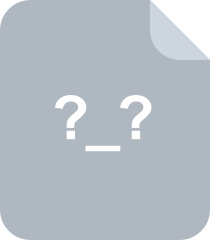
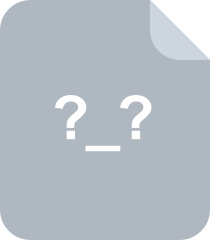
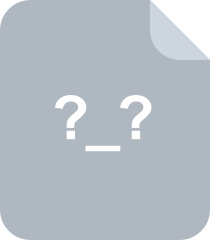
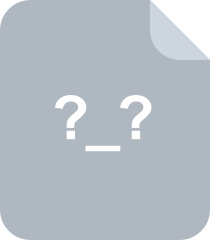
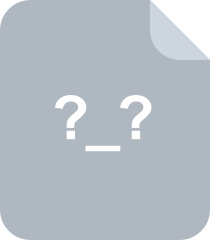
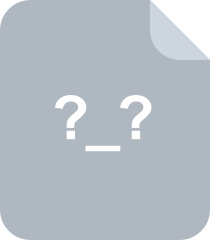
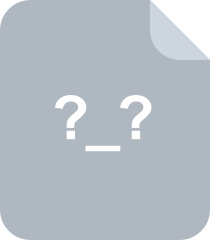
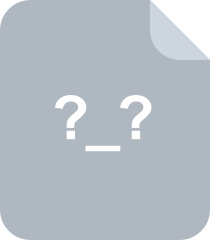
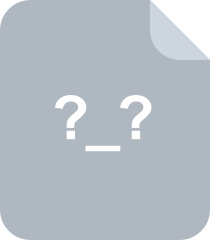
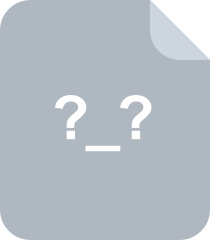
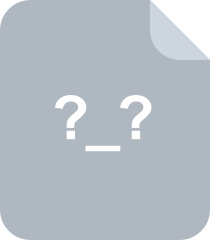
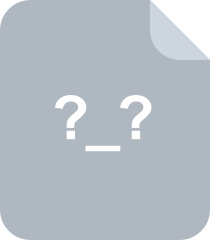
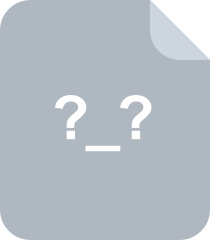
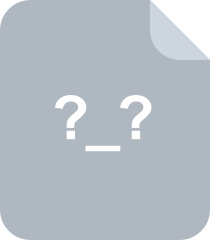
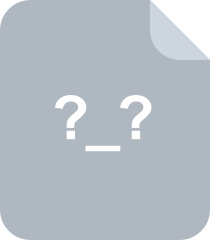
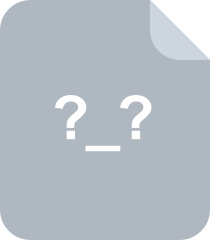
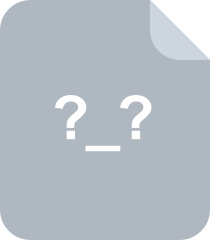
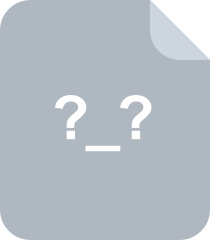
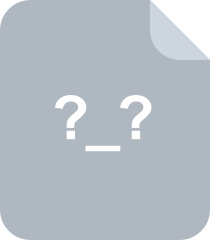
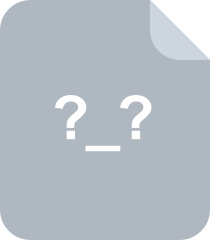
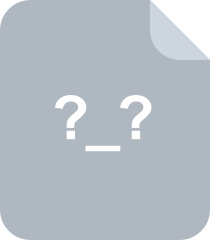
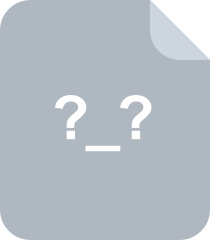
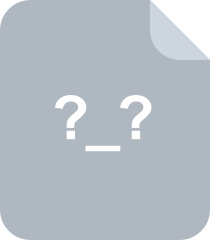
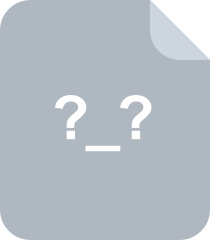
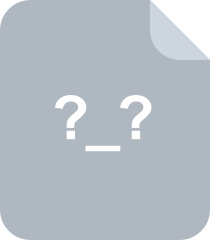
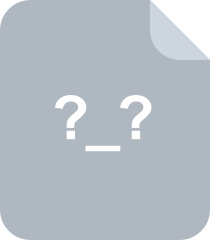
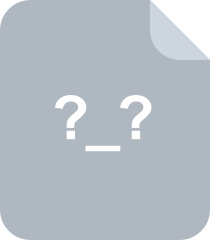
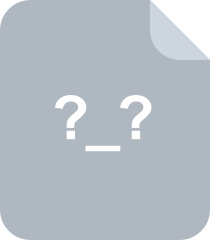
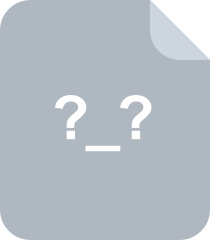
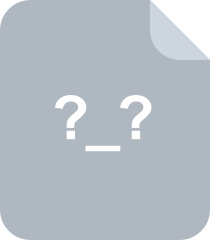
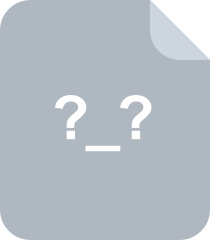
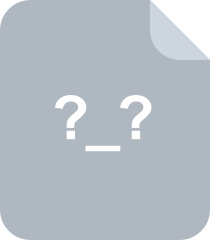
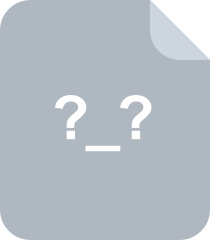
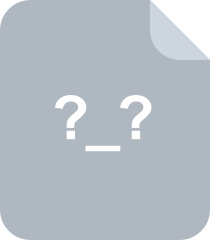
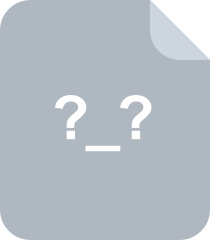
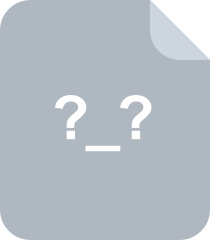
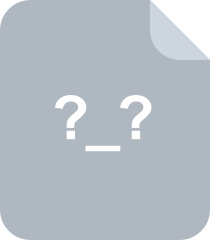
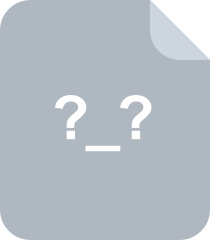
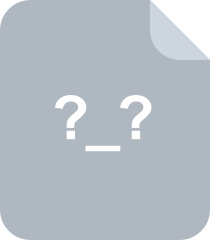
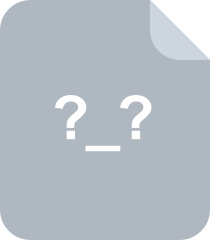
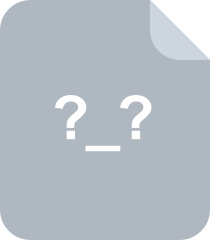
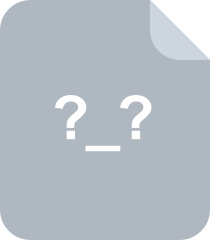
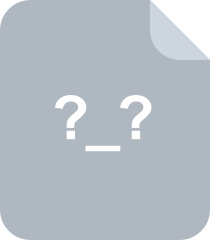
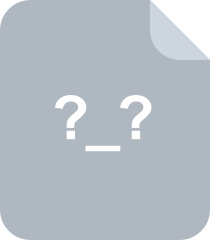
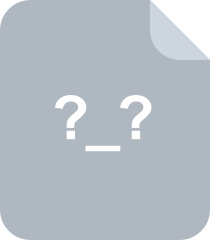
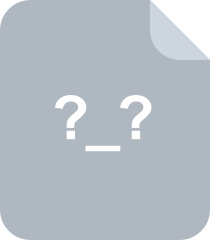
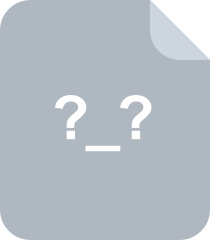
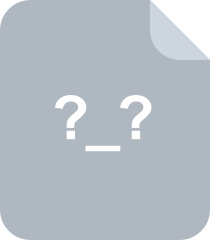
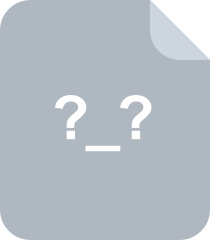
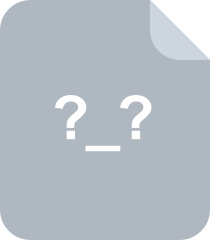
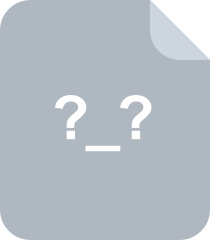
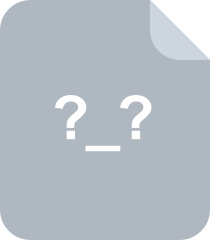
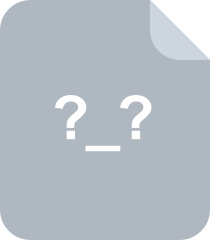
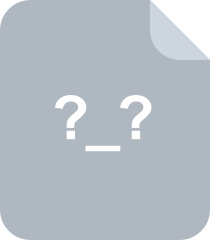
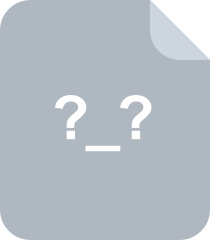
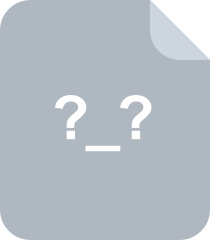
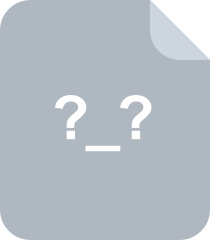
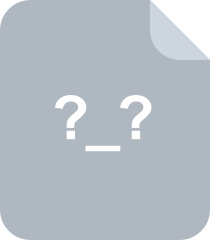
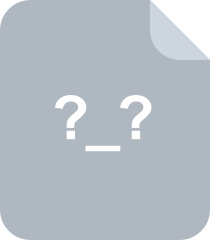
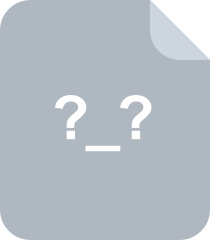
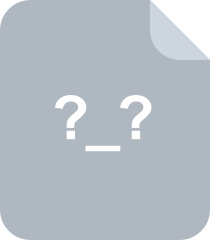
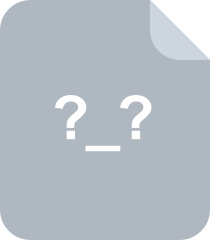
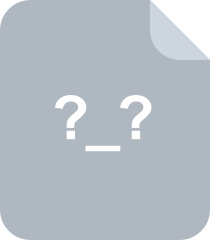
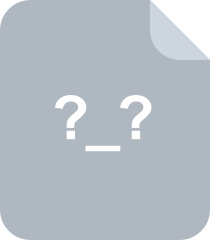
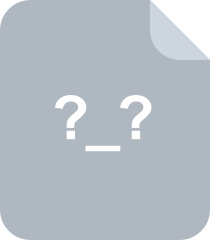
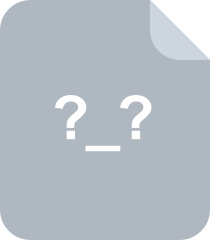
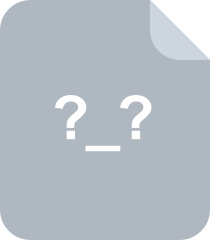
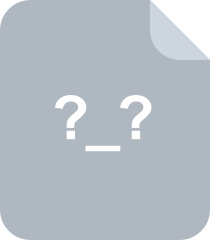
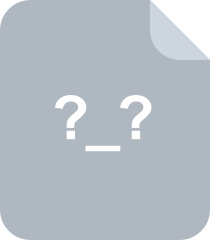
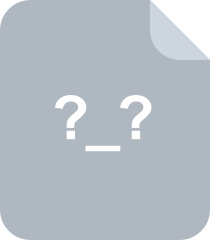
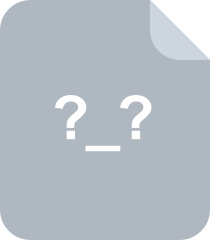
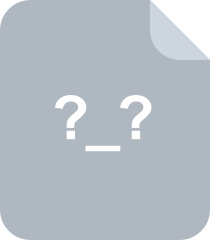
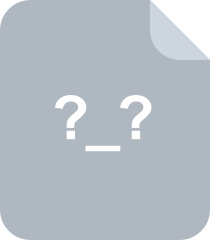
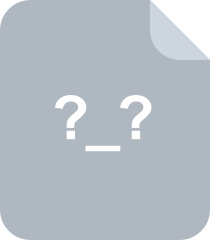
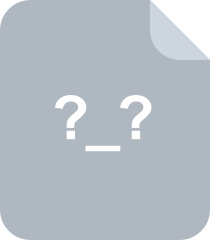
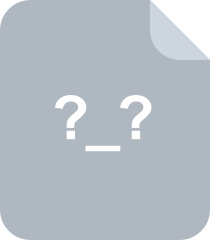
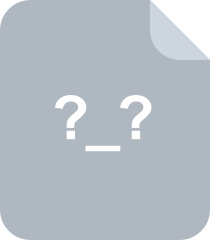
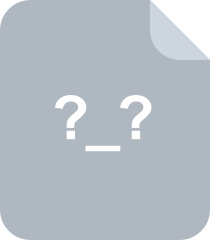
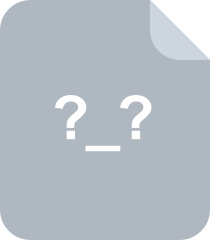
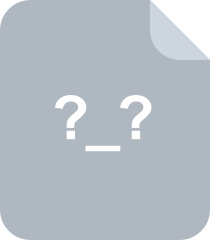
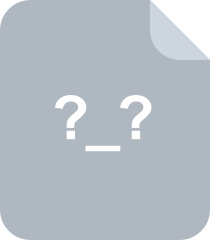
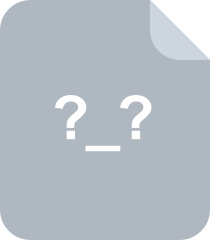
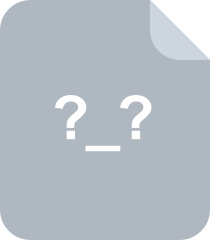
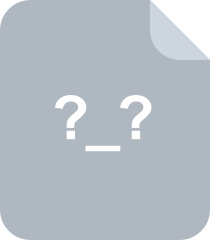
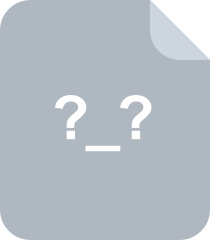
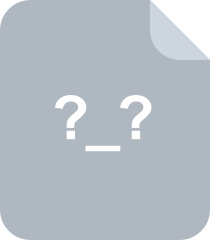
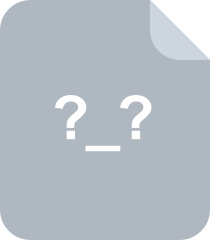
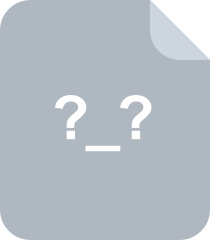
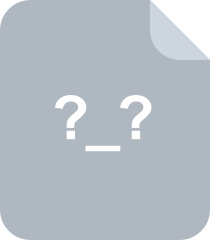
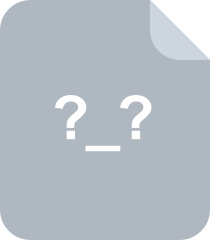
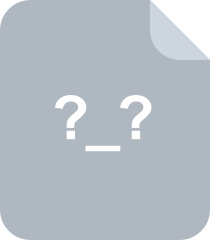
共 1062 条
- 1
- 2
- 3
- 4
- 5
- 6
- 11
资源评论
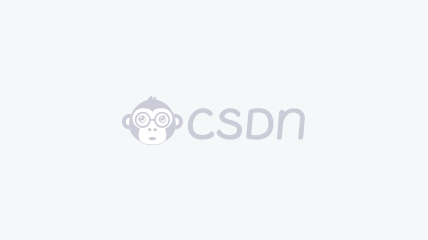
- 美味开发2021-08-03用户下载后在一定时间内未进行评价,系统默认好评。

唐荣轩
- 粉丝: 38
- 资源: 4626
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

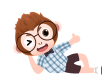
最新资源
- xdxdxdxdxdxdxdxdaaaaaaaa
- score.sql 数据库表格
- 技术资料分享信利4.3单芯片TFT1N4633-Ev1.0非常好的技术资料.zip
- 技术资料分享手机-SMS-PDU-格式参考手册非常好的技术资料.zip
- 技术资料分享Z-Stackapi函数非常好的技术资料.zip
- 技术资料分享Z-Stack-API-Chinese非常好的技术资料.zip
- 技术资料分享Z-Stack 开发指南非常好的技术资料.zip
- 技术资料分享Zigbee协议栈中文说明免费非常好的技术资料.zip
- 技术资料分享Zigbee协议栈及应用实现非常好的技术资料.zip
- 技术资料分享ZigBee协议栈的研究与实现非常好的技术资料.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


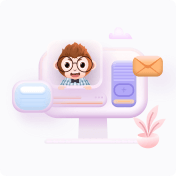
安全验证
文档复制为VIP权益,开通VIP直接复制
