ProducerConsumer:用Java中的代码解决生产者和消费者问题
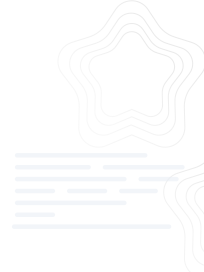

生产者消费者问题是一个经典的多线程同步问题,它模拟了两个不同类型的进程——生产者和消费者,它们共享一个有限大小的缓冲区。生产者将数据放入缓冲区,而消费者则从中取出数据。在这个过程中,必须确保当缓冲区满时,生产者不会继续添加数据;当缓冲区空时,消费者也不会尝试取数据。Java提供了多种机制来解决这个问题,包括synchronized关键字、wait()和notify()方法,以及高级的java.util.concurrent并发工具。 在Java中,我们可以使用阻塞队列(BlockingQueue)来简化生产者消费者问题的实现。 BlockingQueue是一种线程安全的数据结构,它内置了对多线程操作的同步控制。当队列满时,生产者尝试插入元素会阻塞;当队列空时,消费者尝试取出元素也会阻塞。这样可以避免资源的浪费,保证程序的高效运行。 下面是一个简单的基于BlockingQueue实现的生产者消费者模型: ```java import java.util.concurrent.*; public class ProducerConsumerExample { private final BlockingQueue<Integer> queue = new LinkedBlockingQueue<>(10); public static void main(String[] args) { ProducerConsumerExample example = new ProducerConsumerExample(); Thread producer = new Thread(example::produce); Thread consumer = new Thread(example::consume); producer.start(); consumer.start(); } private void produce() { while (true) { try { int data = generateData(); queue.put(data); System.out.println("Produced: " + data); Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } } private void consume() { while (true) { try { int data = queue.take(); System.out.println("Consumed: " + data); Thread.sleep(1500); } catch (InterruptedException e) { e.printStackTrace(); } } } private int generateData() { return (int) (Math.random() * 100); } } ``` 在这个例子中,我们创建了一个容量为10的LinkedBlockingQueue,然后启动生产者线程和消费者线程。生产者线程不断生成随机数据并将其放入队列,消费者线程则从队列中取出并消费数据。`put()`方法在队列满时会阻塞生产者,而`take()`方法在队列空时会阻塞消费者。 除了BlockingQueue,还可以使用Semaphore(信号量)或ReentrantLock(可重入锁)等并发工具来实现生产者消费者问题。Semaphore可以限制同时访问某个资源的线程数量,而ReentrantLock则提供了更细粒度的锁控制。 例如,使用ReentrantLock和Condition,生产者和消费者可以通过条件变量来通信: ```java import java.util.LinkedList; import java.util.Queue; import java.util.concurrent.locks.Condition; import java.util.concurrent.locks.Lock; import java.util.concurrent.locks.ReentrantLock; public class ProducerConsumerExampleWithLock { private final Queue<Integer> queue = new LinkedList<>(); private final Lock lock = new ReentrantLock(); private final Condition notEmpty = lock.newCondition(); private final Condition notFull = lock.newCondition(); public static void main(String[] args) { ProducerConsumerExampleWithLock example = new ProducerConsumerExampleWithLock(); Thread producer = new Thread(example::produce); Thread consumer = new Thread(example::consume); producer.start(); consumer.start(); } private void produce() { while (true) { try { lock.lock(); while (queue.size() == 10) { notFull.await(); } int data = generateData(); queue.add(data); System.out.println("Produced: " + data); notEmpty.signal(); lock.unlock(); Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } } private void consume() { while (true) { try { lock.lock(); while (queue.isEmpty()) { notEmpty.await(); } int data = queue.remove(); System.out.println("Consumed: " + data); notFull.signal(); lock.unlock(); Thread.sleep(1500); } catch (InterruptedException e) { e.printStackTrace(); } } } private int generateData() { return (int) (Math.random() * 100); } } ``` 在这个版本中,我们使用ReentrantLock来控制对共享资源(queue)的访问,并通过notEmpty和notFull两个条件变量来协调生产者和消费者的执行。 Java提供了一系列强大的并发工具,使得解决生产者消费者问题变得简单且高效。在实际开发中,我们需要根据具体需求选择合适的方法来实现同步和通信,确保多线程程序的正确性和性能。
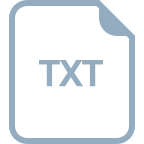
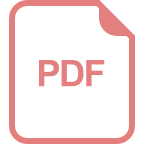
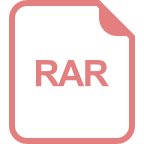
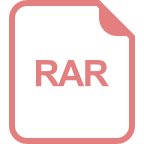
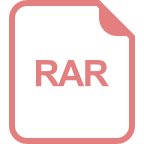
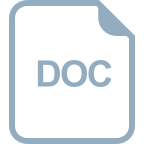
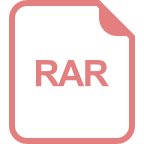
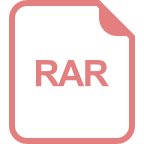
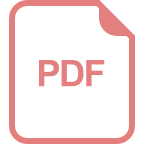
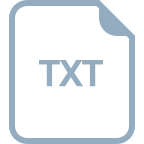
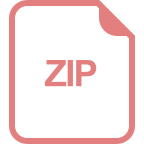
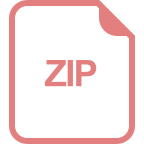
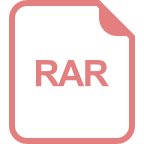
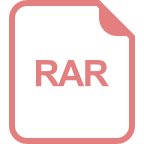
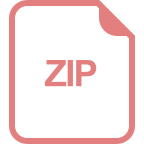
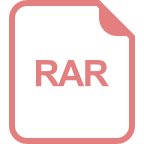
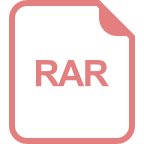
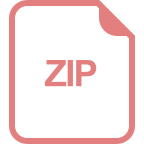
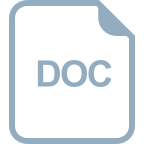
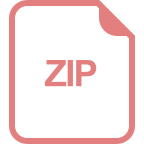
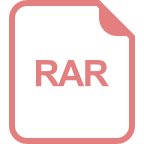
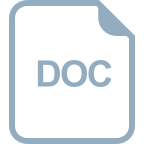
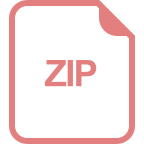
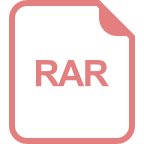


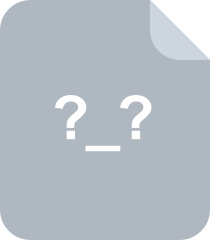
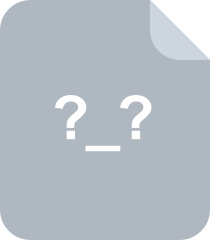
- 1
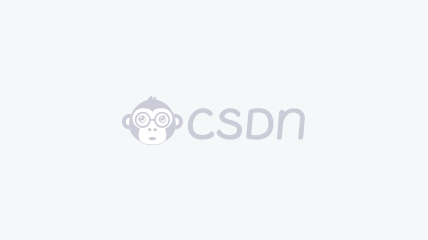

- 粉丝: 26
- 资源: 4577
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

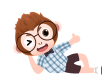
最新资源

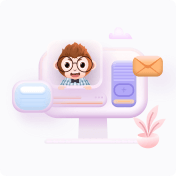
