# google-translate-api
[](https://github.com/vitalets/google-translate-api/actions)
[](https://www.npmjs.com/package/@vitalets/google-translate-api)
[](https://github.com/sindresorhus/xo)
[](https://coveralls.io/github/vitalets/google-translate-api?branch=master)
A **free** and **unlimited** API for Google Translate :dollar: :no_entry_sign: for Node.js.
## Features
- Auto language detection
- Spelling correction
- Language correction
- Fast and reliable – it uses the same servers that [translate.google.com](https://translate.google.com) uses
## Why this fork?
This fork of original [matheuss/google-translate-api](https://github.com/matheuss/google-translate-api) contains several improvements:
- New option `client="t|gtx"`. Setting `client="gtx"` seems to work even with outdated token, see [this discussion](https://github.com/matheuss/google-translate-api/issues/79#issuecomment-425679193) for details
- Fixed extraction of TKK ceed from current `https://translate.google.com` sources (via [@vitalets/google-translate-token](https://github.com/vitalets/google-translate-token))
- Removed unsecure `unsafe-eval` dependency (See [#2](https://github.com/vitalets/google-translate-api/pull/2))
- Added [daily CI tests](https://travis-ci.org/vitalets/google-translate-api/builds) to get notified if Google API changes
- Added support for custom `tld` (especially to support `translate.google.cn`, see [#7](https://github.com/vitalets/google-translate-api/pull/7))
- Added support for outputting pronunciation (see [#17](https://github.com/vitalets/google-translate-api/pull/17))
- Added support for custom [got](https://github.com/sindresorhus/got) options. It allows to use proxy and bypass request limits (see [#25](https://github.com/vitalets/google-translate-api/pull/25))
- Added support for language extensions from outside of the API (see [#18](https://github.com/vitalets/google-translate-api/pull/18))
- Added TypeScript definitions (see [#50](https://github.com/vitalets/google-translate-api/pull/50), thanks to [@olavoparno](https://github.com/olavoparno))
- Migrated to Google's latest batch-style RPC API (see [#60](https://github.com/vitalets/google-translate-api/pull/60), thanks to [@vkedwardli](https://github.com/vkedwardli))
## Install
```
npm install @vitalets/google-translate-api
```
## Usage
From automatic language detection to English:
```js
const translate = require('@vitalets/google-translate-api');
translate('Ik spreek Engels', {to: 'en'}).then(res => {
console.log(res.text);
//=> I speak English
console.log(res.from.language.iso);
//=> nl
}).catch(err => {
console.error(err);
});
```
From English to Dutch with a typo:
```js
translate('I spea Dutch!', {from: 'en', to: 'nl'}).then(res => {
console.log(res.text);
//=> Ik spreek Nederlands!
console.log(res.from.text.autoCorrected);
//=> true
console.log(res.from.text.value);
//=> I [speak] Dutch!
console.log(res.from.text.didYouMean);
//=> false
}).catch(err => {
console.error(err);
});
```
Sometimes, the API will not use the auto corrected text in the translation:
```js
translate('I spea Dutch!', {from: 'en', to: 'nl'}).then(res => {
console.log(res);
console.log(res.text);
//=> Ik spea Nederlands!
console.log(res.from.text.autoCorrected);
//=> false
console.log(res.from.text.value);
//=> I [speak] Dutch!
console.log(res.from.text.didYouMean);
//=> true
}).catch(err => {
console.error(err);
});
```
You can also add languages in the code and use them in the translation:
``` js
translate = require('google-translate-api');
translate.languages['sr-Latn'] = 'Serbian Latin';
translate('translator', {to: 'sr-Latn'}).then(res => ...);
```
## Proxy
Google Translate has request limits. If too many requests are made, you can either end up with a 429 or a 503 error.
You can use **proxy** to bypass them:
```js
const tunnel = require('tunnel');
translate('Ik spreek Engels', {to: 'en'}, {
agent: tunnel.httpsOverHttp({
proxy: {
host: 'whateverhost',
proxyAuth: 'user:pass',
port: '8080',
headers: {
'User-Agent': 'Node'
}
}
}
)}).then(res => {
// do something
}).catch(err => {
console.error(err);
});
```
## Does it work from web page context?
No. `https://translate.google.com` does not provide [CORS](https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS) http headers allowing access from other domains.
## API
### translate(text, [options], [gotOptions])
#### text
Type: `string`
The text to be translated
#### options
Type: `object`
##### from
Type: `string` Default: `auto`
The `text` language. Must be `auto` or one of the codes/names (not case sensitive) contained in [languages.js](https://github.com/vitalets/google-translate-api/blob/master/languages.js)
##### to
Type: `string` Default: `en`
The language in which the text should be translated. Must be one of the codes/names (case sensitive!) contained in [languages.js](https://github.com/vitalets/google-translate-api/blob/master/languages.js).
##### raw
Type: `boolean` Default: `false`
If `true`, the returned object will have a `raw` property with the raw response (`string`) from Google Translate.
##### client
Type: `string` Default: `"t"`
Query parameter `client` used in API calls. Can be `t|gtx`.
##### tld
Type: `string` Default: `"com"`
TLD for Google translate host to be used in API calls: `https://translate.google.{tld}`.
#### gotOptions
Type: `object`
The got options: https://github.com/sindresorhus/got#options
### Returns an `object`:
- `text` *(string)* – The translated text.
- `from` *(object)*
- `language` *(object)*
- `didYouMean` *(boolean)* - `true` if the API suggest a correction in the source language
- `iso` *(string)* - The [code of the language](https://github.com/vitalets/google-translate-api/blob/master/languages.js) that the API has recognized in the `text`
- `text` *(object)*
- `autoCorrected` *(boolean)* – `true` if the API has auto corrected the `text`
- `value` *(string)* – The auto corrected `text` or the `text` with suggested corrections
- `didYouMean` *(boolean)* – `true` if the API has suggested corrections to the `text`
- `raw` *(string)* - If `options.raw` is true, the raw response from Google Translate servers. Otherwise, `''`.
Note that `res.from.text` will only be returned if `from.text.autoCorrected` or `from.text.didYouMean` equals to `true`. In this case, it will have the corrections delimited with brackets (`[ ]`):
```js
translate('I spea Dutch').then(res => {
console.log(res.from.text.value);
//=> I [speak] Dutch
}).catch(err => {
console.error(err);
});
```
Otherwise, it will be an empty `string` (`''`).
## License
MIT © [Matheus Fernandes](http://matheus.top), forked and maintained by [Vitaliy Potapov](https://github.com/vitalets).

仰光的瑞哥
- 粉丝: 20
- 资源: 4623
最新资源
- 不同颜色机器护垫检测27-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 西门子S7-1500博图程序 例程,大型生产线案例,程序涵盖有机器人块,汽缸块,电机块,伺服块,可调用,扫码块,可学习参考,快速提升技能 ,编程使用的语言有SCL,LD,STL,GRAPH 非常全
- java gis 开发中,点线面shp样例文件
- C++图书管理系统源代码(高分期末大作业项目)
- 安卓同步助手-1.apk
- GEATC 电脑 G5 型号官网驱动附件(win7/64位)
- LLC开关电源,60V5A半桥LLC串联谐振开关电源设计方案,提供原理图和PCB,BOM表,变压器制作说明书,配套半桥LLC电源软件 备注:原理图和PCB用AD软件打开
- Screenshot_20241229_173539_com.xunmeng.pinduoduo.jpg
- 2基于改进粒子群算法的微电网多目标优化调度 以微电网的运行成本、环境保护成本之和最小为目标,建立微电网环保与经济调度模型,并采用改进的PSO 算法对优化模型进行求解
- 基于ssm的超市进销存管理系统源码(java毕业设计完整源码+LW).zip
- 三菱PLC程序三菱Q系列案例三菱plc大型自动化程序生产线程序 规格如下: Q系列大型程序伺服12轴Q01U RS232通讯CCD 应用 实际使用中程序,详细中文注释 2个模块QD70P8,QD7
- 基于ssm的有机蔬菜商城源码(java毕业设计完整源码+LW).zip
- 不同颜色机器护垫检测47-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 基于ssm的游戏售卖网站源码(java毕业设计完整源码+LW).zip
- 基于ssm的企业仓储管理系统源码(java毕业设计完整源码+LW).zip
- 芝麻录屏 电脑高清录屏 会议录屏 直播录屏
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


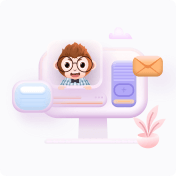
评论1