TensorFlow-Android:示例项目如何使用自定义模型在Android中实现TensorFlow
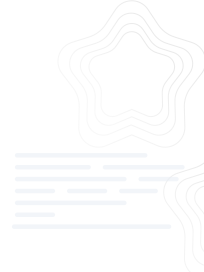

在Android平台上实现TensorFlow,通常是为了利用其强大的机器学习能力为移动应用增添智能特性。本教程将深入探讨如何在Android项目中集成TensorFlow,并使用自定义的机器学习模型进行预测。我们将主要关注Java语言的实现,因为这是Android开发的主要语言。 我们需要理解TensorFlow Lite是TensorFlow用于移动和嵌入式设备的轻量级解决方案。它允许在本地运行经过训练的模型,无需云端支持,从而提供快速响应和低功耗性能。 **1. 集成TensorFlow Lite** 要将TensorFlow Lite引入Android项目,首先要在`build.gradle`文件中添加依赖项: ```groovy dependencies { implementation 'org.tensorflow:tensorflow-lite:版本号' } ``` 请确保使用最新或兼容的版本号。 **2. 准备自定义模型** 在使用TensorFlow Lite之前,你需要一个预先训练好的模型。这可以通过TensorFlow API创建或从已有的开源资源获取。模型应转换为`.tflite`格式,适合在Android上运行。使用`tflite_convert`命令行工具或者Python API可以完成转换。 **3. 在Android中加载模型** 在Android应用中,通过`TFLiteModelInterpreter`加载模型。以下代码展示了如何加载`.tflite`文件: ```java File modelFile = new File(getAssets(), "model.tflite"); try { tfliteModel = TFLiteInterpreter.loadModel(context.getAssets(), modelFile.getAbsolutePath()); } catch (IOException e) { e.printStackTrace(); } ``` 这里的`context`是Android应用程序上下文,`model.tflite`是模型文件名。 **4. 创建输入和输出对象** TensorFlow Lite模型需要输入数据进行计算,并返回输出结果。使用`ByteBuffer`对象来处理这些数据。确定模型的输入和输出张量索引: ```java int[] inputTensorIndex = {0}; // 假设输入张量的索引是0 int[] outputTensorIndex = {0}; // 假设输出张量的索引是0 ``` 然后创建`ByteBuffer`实例并分配内存: ```java int inputShape[] = tfliteModel.getInputTensor(inputTensorIndex[0]).shape(); // 获取输入张量的形状 ByteBuffer inputBuffer = ByteBuffer.allocateDirect(inputShape[1] * inputShape[2] * inputShape[3] * 4); // 分配足够的内存 ``` **5. 执行模型** 现在可以将数据传递给模型并获取结果了: ```java Map<Integer, Object> inputs = new HashMap<>(); inputs.put(inputTensorIndex[0], inputBuffer); Map<Integer, Object> outputs = new HashMap<>(); tfliteModel.run(inputs, outputs); ByteBuffer outputBuffer = (ByteBuffer) outputs.get(outputTensorIndex[0]); ``` `run()`方法执行模型,输入和输出都是通过哈希映射传递的。 **6. 处理输出** 根据模型的输出类型,解析`outputBuffer`以获取结果。例如,如果输出是一个分类任务,可能需要找到具有最高概率的类别: ```java float[] outputArray = new float[outputBuffer.limit()]; outputBuffer.rewind(); outputBuffer.asFloatBuffer().get(outputArray); int maxIndex = 0; float maxValue = outputArray[0]; for (int i = 1; i < outputArray.length; i++) { if (outputArray[i] > maxValue) { maxIndex = i; maxValue = outputArray[i]; } } String predictedClass = classes[maxIndex]; // classes数组存储了每个类别的标签 ``` **7. 错误处理和资源释放** 在应用不再需要模型时,记得释放资源: ```java tfliteModel.close(); ``` 以上就是如何在Android项目中使用TensorFlow Lite运行自定义模型的基本步骤。根据实际需求,你可能还需要处理数据预处理、异步预测、多线程等问题。记住,调试和测试对于确保模型在Android设备上正常工作至关重要。通过不断优化和调整,你的应用将能够充分利用TensorFlow的强大功能,为用户提供智能且高效的体验。
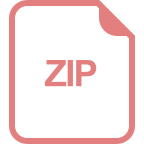
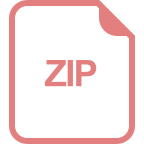
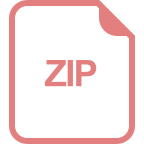
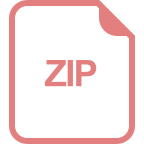
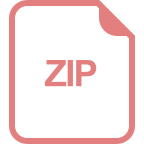
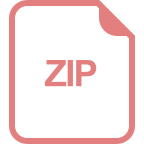
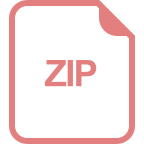
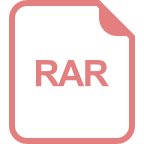
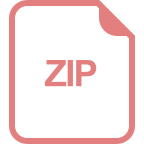
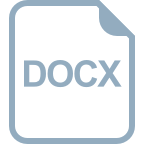
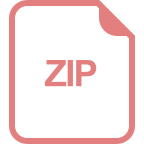
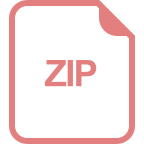
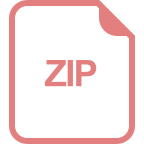
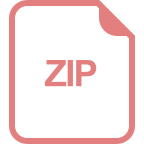
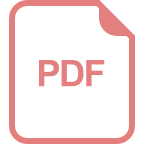
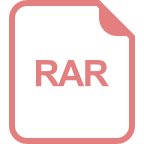
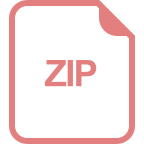
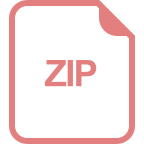
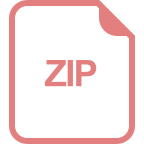
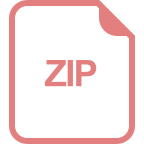


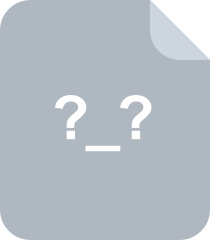


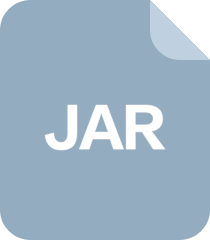
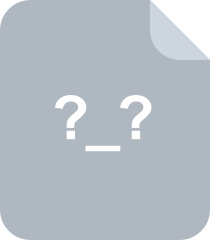
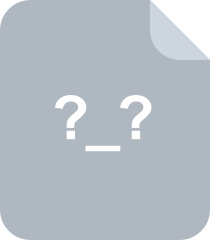
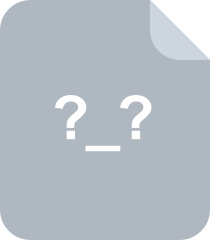
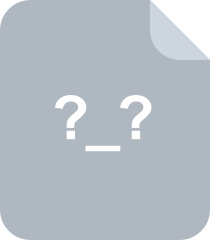
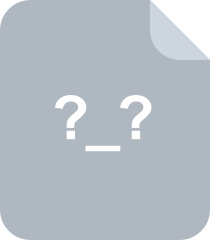

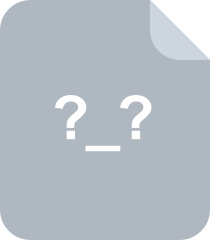
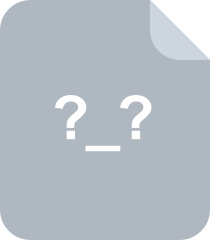
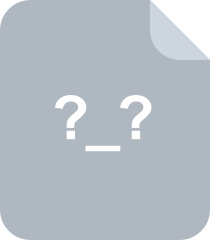


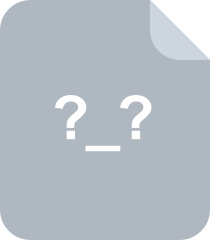

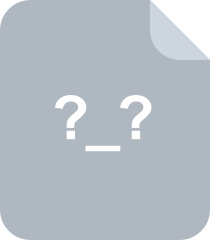

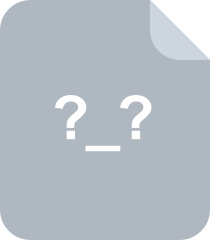

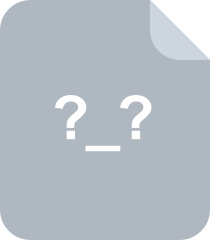
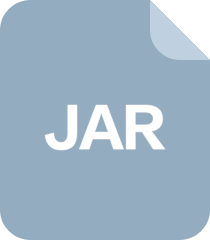







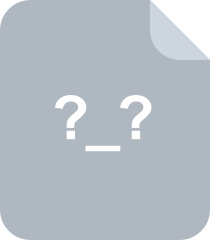

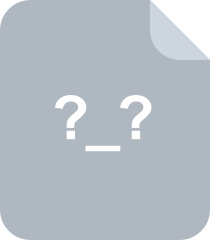





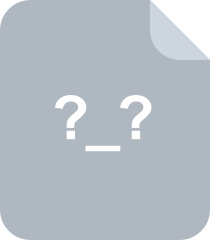


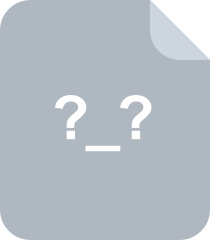
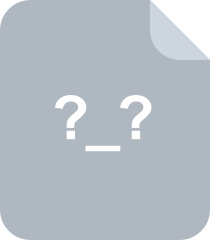
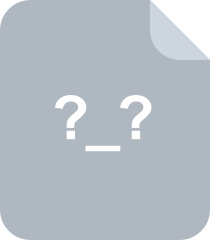

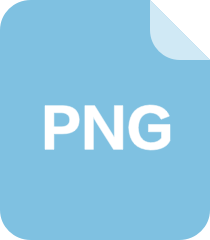
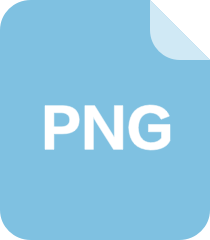

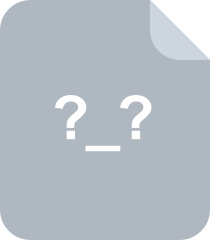

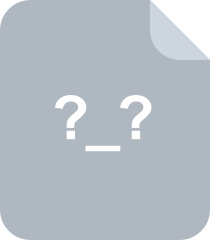

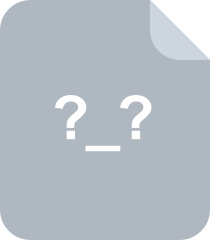
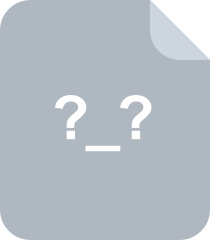

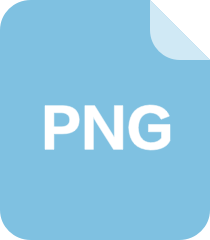
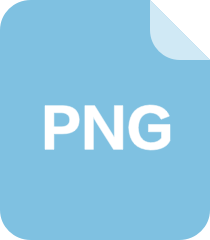

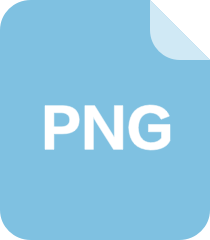
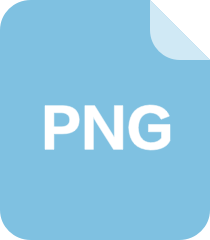

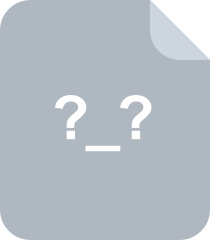

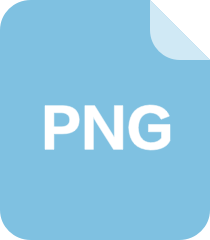
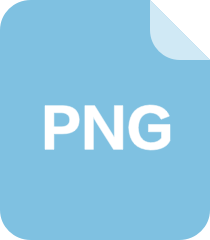

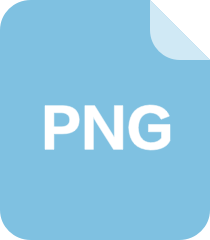
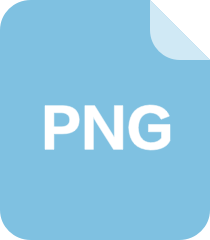

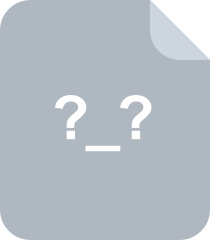
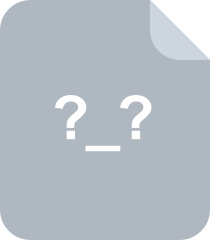
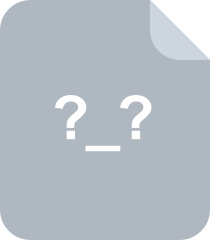
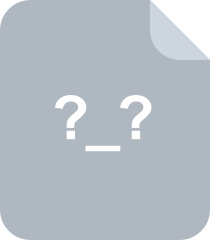
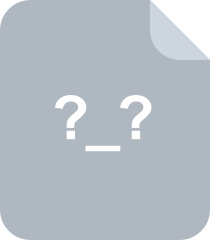






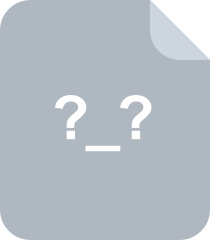
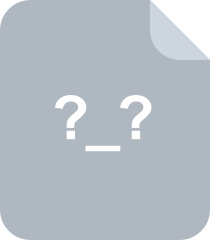

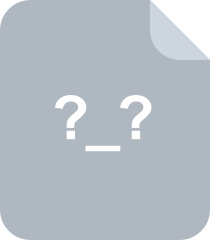
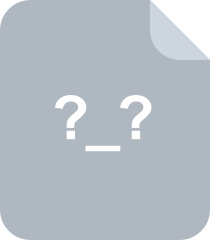
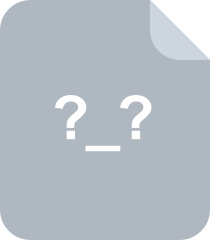

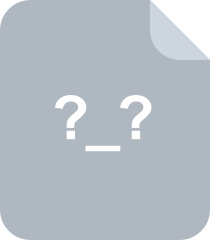

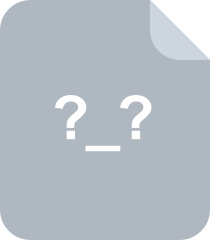
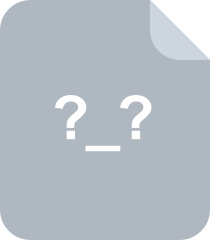
- 1
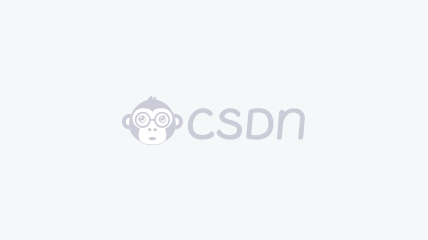
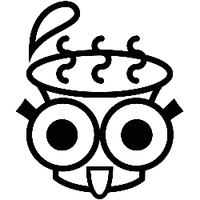
- 粉丝: 33
- 资源: 4624
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

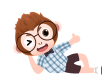
最新资源
- 青藏高原冻土空间分布-2023年最新绘制
- order system(1).c
- 基于微博数据的舆情分析项目(包括微博爬虫、LDA主题分析和情感分析)高分项目
- 测试电路板用的双针床设备(含工程图sw17可编辑+cad)全套技术开发资料100%好用.zip
- 基于Python控制台的网络入侵检测
- 基于微博数据的舆情分析项目-包括数据分析、LDA主题分析和情感分析(高分项目源码)
- 制作生成自己专属的安卓app应用 制作apk
- 基于python开发的贪食蛇(源码)
- frmcurvechart.ui
- NSFetchedResultsControllerError如何解决.md
- 基于java银行客户信息管理系统论文.doc
- EmptyStackException(解决方案).md
- RuntimeError.md
- wqwerwerwere
- 基于java+ssm+mysql的4S店预约保养系统任务书.docx
- 基于java在线考试系统2毕业论文.doc

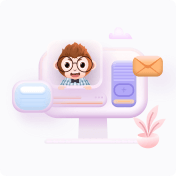
