# Steam Market
[![Build status][build-image]][build]
[![Updates][dependency-image]][pyup]
[![Python 3][python3-image]][pyup]
[![Code coverage][codecov-image]][codecov]
[![Code Quality][codacy-image]][codacy]
This repository contains Python code to find arbitrages on the Steam Market.
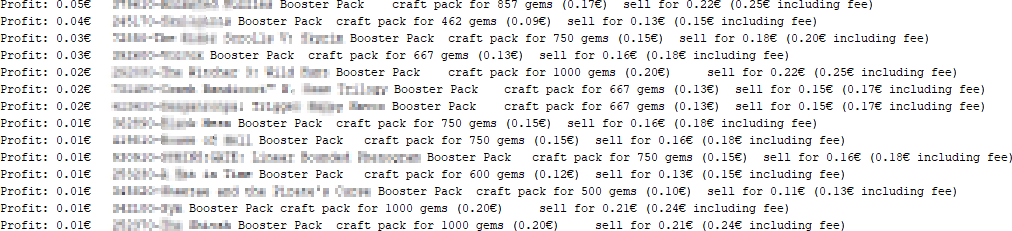
Arbitrages could consist in:
- purchasing gems to craft booster packs, which are then immediately sold for more than their crafting cost,
- purchasing items, typically foil cards, which are then immediately turned into more gems than they are worth,
- purchasing games in order to produce the corresponding booster packs, with total profit greater than the game price,
- turning normal cards into badges, if the expected value is positive, to create items worth more than the badge cost.
## Requirements
- Install the latest version of [Python 3.X](https://www.python.org/downloads/).
- Install the required packages:
```bash
pip install -r requirements.txt
```
## Data acquisition
### Cookie
To relax the rate limits enforced by Steam API, fill-in your cookie information in a file called `personal_info.json`:
1. To do so, make sure you are connected to your Steam account on a Steam Community page, e.g. [Steam Market](https://steamcommunity.com/market/).<br/>

2. Press `<Shift-F9>` in your web browser to access the storage section of the developer tools.<br/>

3. Use the filtering option (in the top right of the storage section) to find the cookie value for `steamLoginSecure`.<br/>

4. Copy-paste this cookie value into a new file called `personal_info.json`, which will be read by [`personal_info.py`](personal_info.py).<br/>
```json
{
"steamLoginSecure": "PASTE_YOUR_COOKIE_VALUE_HERE"
}
```
> **NB**: In the future, if you notice that the program bugs out due to seemingly very strict rate limits, then it may
be a sign that the cookie value tied to your session has changed.
In this case, try to fill-in your cookie information with its new value.
> **NB²**: If you want to automate the creation and sale of booster packs, you may need:
> 1. to have a [mobile authenticator app](https://github.com/Jessecar96/SteamDesktopAuthenticator) running in the background and auto-confirming market transactions,
> 2. to fill-in more cookie information.
I have been using the following entries, but you might not need to use all of them. Except for `steamLoginSecure` and `sessionid`, the values of the other entries are set in stone and do not need to be updated afterwards.
> ```json
> {
> "browserid": "PASTE_YOUR_COOKIE_VALUE_HERE",
> "steamMachineAuth_PASTE_YOUR_STEAM_ID_HERE": "PASTE_YOUR_COOKIE_VALUE_HERE",
> "steamRememberLogin": "PASTE_YOUR_COOKIE_VALUE_HERE",
> "sessionid": "PASTE_YOUR_COOKIE_VALUE_HERE",
> "steamLoginSecure": "PASTE_YOUR_COOKIE_VALUE_HERE"
> }
> ```
### Gem cost for crafting Booster Packs
To have access to the gem cost for crafting Booster Packs, you will need to manually copy information available [here](https://steamcommunity.com/tradingcards/boostercreator/).
There are two solutions:
- solution A is my original solution, but it requires a browser extension called *Augmented Steam*,
- solution B is a more recent solution, and does not require any third-party browser extension.
#### Solution A
1. Install the browser extension called [*Augmented Steam*](https://es.isthereanydeal.com/), so that the number of gems required to craft a Booster Pack appears in the drop-down menu:<br/>
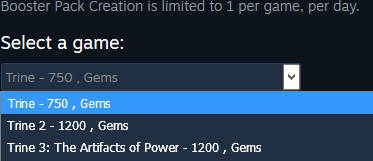
2. Then, right-click the drop-down menu and "inspect" the corresponding HTML code in your browser:<br/>
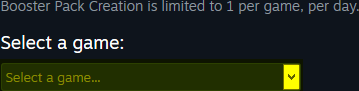
3. Copy the following line and paste it to `data/booster_game_creator.txt`:<br/>
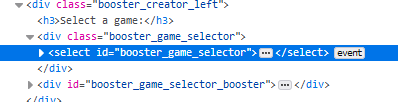
4. Add line-breaks, so that the file is formatted in the following way:<br/>

For instance, with [Visual Studio Code](https://code.visualstudio.com/), press `<Ctrl-H>` and run:<br/>

5. Strip the following unnecessary lines:
- three lines (including an empty line) at the beginning:
```html
<select id="booster_game_selector">
<option value="">Select a game...</option>
```
- one line at the end:
```html
</select>
```
#### Solution B
Alternatively, if you wish not to install any browser extension:
1. Press `<Ctrl-U>` to display the HTML code of [the Booser Creation webpage](https://steamcommunity.com/tradingcards/boostercreator/).
2. At the end of the HTML code, find and copy the line below `CBoosterCreatorPage.Init`:<br/>
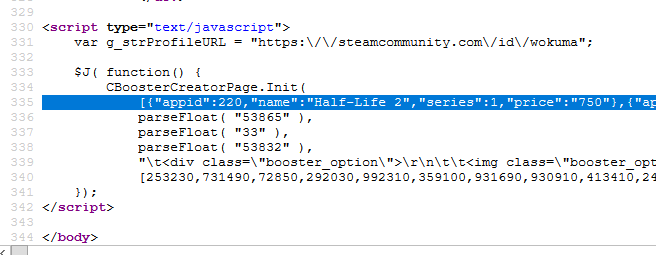
3. Paste the line to `data/booster_game_creator_from_javascript.txt`.
4. Strip mentions of packs unavailable because they were crafted less than 24 hours ago. For instance:
```json
{"appid":996580,"name":"Spyro\u2122 Reignited Trilogy","series":1,"price":"400",
"unavailable":true,"available_at_time":"4 Sep @ 7:06pm"}
```
should be replaced with:
```json
{"appid":996580,"name":"Spyro\u2122 Reignited Trilogy","series":1,"price":"400"}
```
To do so, with [Visual Studio Code](https://code.visualstudio.com/), press `<Ctrl-H>` and remove occurences of :<br/>

```regexp
,"unavailable":true,"available_at_time":"[\w ]*@[\w :]*"
```
## Usage
- To parse all the options to craft 'Booster Packs', for the games you own, run:
```bash
python parsing_utils.py
```
- To retrieve all the listings of 'Booster Packs' on the Steam Market, along with the sell price and volume, run:
```bash
python market_search.py
```
- To retrieve the price which sellers ask for a ['Sack of Gems'](https://steamcommunity.com/market/listings/753/753-Sack%20of%20Gems), run:
```bash
python sack_of_gems.py
```
- To retrieve i) the "item name id" of a listing, and ii) whether a *crafted* item would really be marketable, run:
```bash
python market_listing.py
```
- To match listing hashes with badge creation details, run:
```bash
python market_utils.py
```
- To retrieve the ask and bid for 'Booster Packs', run:
```bash
python market_order.py
```
- To look for **free** games which i) feature trading cards (and thus crafting of booster packs), and ii) which I do not own, run:
```bash
python free_games_with_trading_cards.py
```
- To create packs for a **manual** selection of games, e.g. if you want to create these specific packs every day, run:
```bash
python batch_create_packs.py
```
- To list appIDs of interest for which we can under-cut the lowest sell order and still hope to make a profit:
```bash
python list_possible_lures.py
```
- To look for games which i) are likely to have high bid orders for their booster packs, and ii) which I may not own yet, run:
```bash
python market_buzz_detector.py
```
- To look for potentially profitable gambles on profile backgrounds and emoticons of "Common" rarity, run:
```bash
python market_gamble_detector.py
```
- To find market arbitrages, e.g. sell a pack for more (fee excluded) than the cost to craft it (fee included), run:
```bash
python market_arbitrage.p
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
蒸汽市场 该存储库包含用于在Steam市场上查找套利的Python代码。 套利可包括: 购买宝石来制作辅助包,然后立即以高于其制作成本的价格出售这些宝石, 购买物品,通常是铝箔卡,这些物品立即变成了价值不菲的宝石, 购买游戏以生产相应的补充包,而获得的总利润大于游戏价格, 如果期望值是正数,则将普通卡变成徽章,以制造出价值超过徽章成本的物品。 要求 安装最新版本的 。 安装所需的软件包: pip install -r requirements.txt 数据采集 曲奇饼 为了放宽Steam API规定的速率限制,请在名为personal_info.json的文件中填写cookie信息: 为此,请确保您已连接到Steam社区页面(例如上的Steam帐户。 在Web浏览器中按<Shift>以访问开发人员工具的存储部分。 使用过滤选项(在存储部分的右上角)查找stea
资源详情
资源评论
资源推荐
收起资源包目录


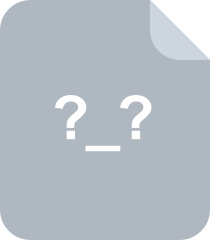
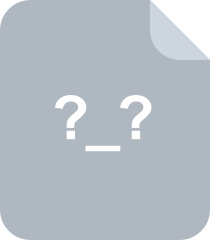
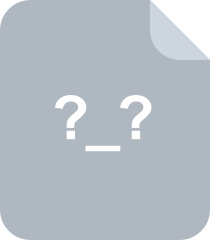
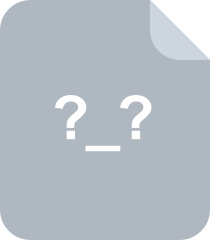
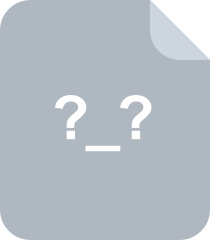
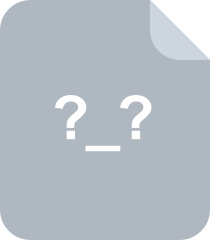
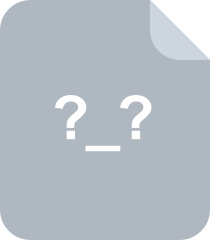
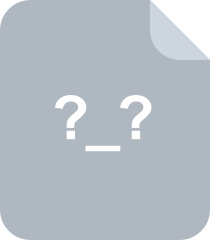
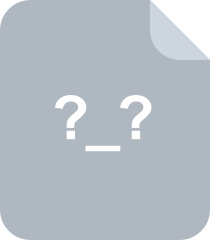
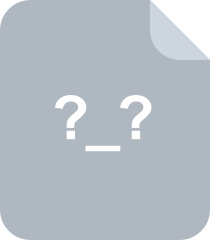
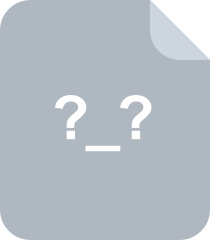
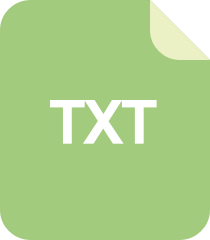
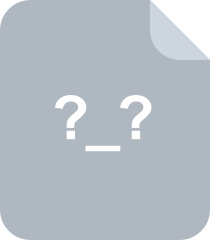
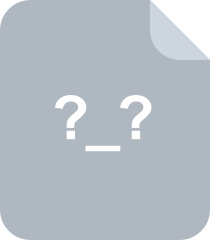
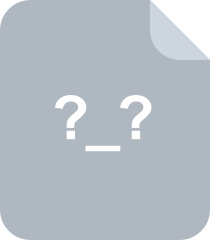
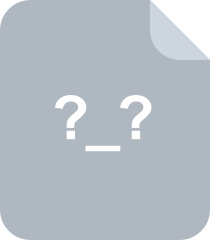
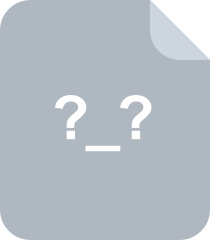
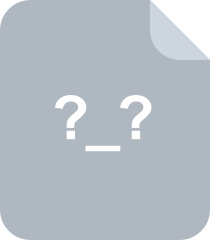

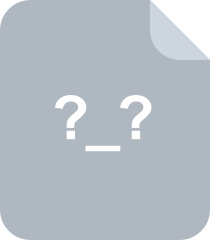
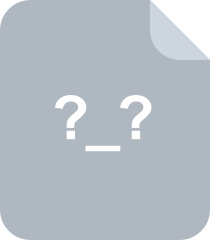
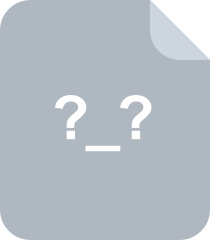
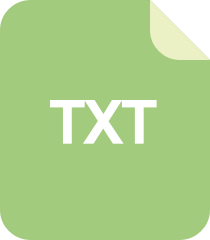
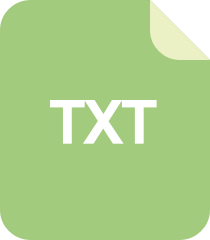
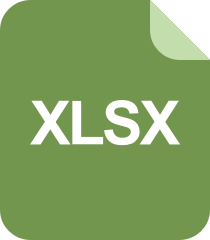
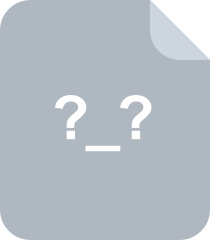
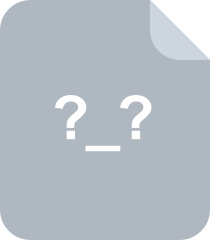
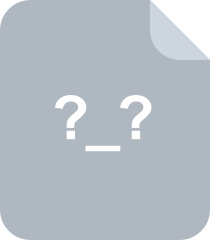
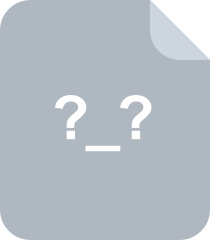
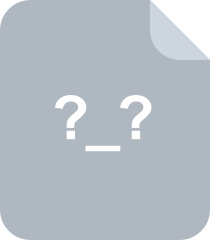
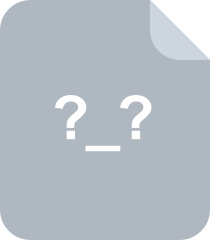
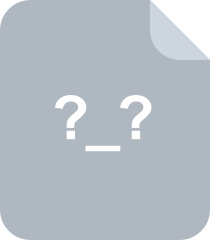
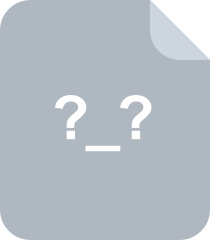
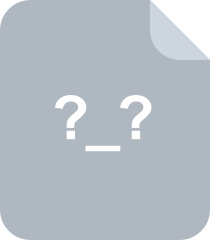
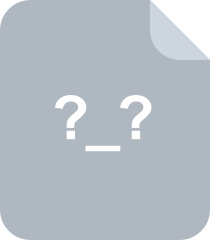
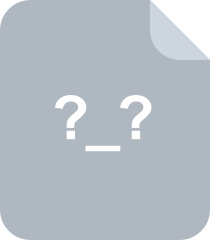
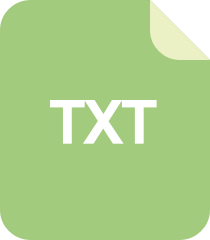
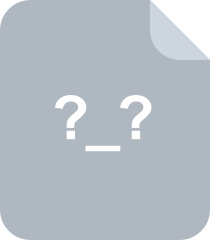
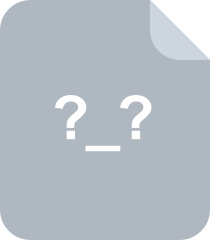
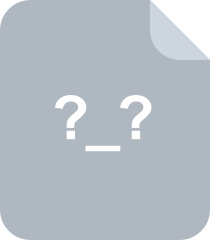
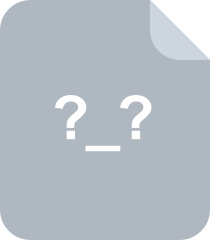
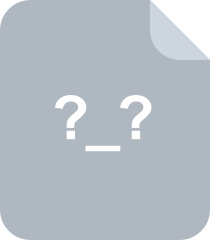
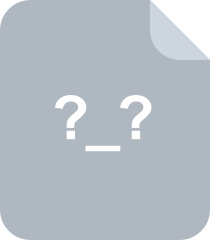
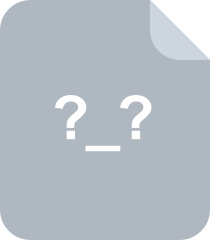
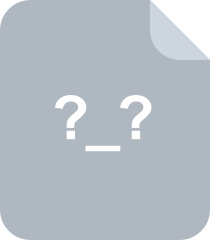
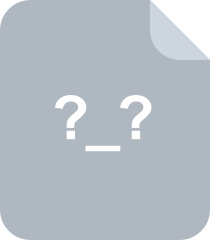
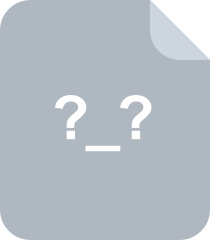
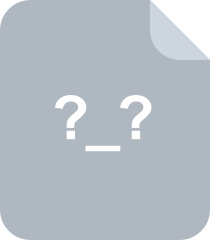
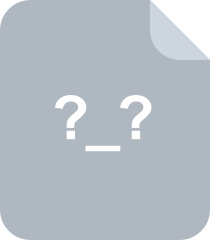
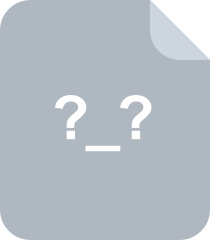
共 49 条
- 1





























FeMnO
- 粉丝: 23
- 资源: 4608
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

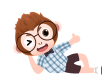
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


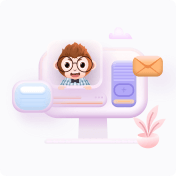
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0