[![npm][npm]][npm-url]
[![node][node]][node-url]
[![deps][deps]][deps-url]
[![test][test]][test-url]
[![coverage][cover]][cover-url]
[![code style][style]][style-url]
[![chat][chat]][chat-url]
<div align="center">
<img width="100" height="100" title="Load Options" src="http://michael-ciniawsky.github.io/postcss-load-options/logo.svg">
<a href="https://github.com/postcss/postcss">
<img width="110" height="110" title="PostCSS" src="http://postcss.github.io/postcss/logo.svg" hspace="10">
</a>
<img width="100" height="100" title="Load Plugins" src="http://michael-ciniawsky.github.io/postcss-load-plugins/logo.svg">
<h1>Load Config</h1>
</div>
<h2 align="center">Install</h2>
```bash
npm i -D postcss-load-config
```
<h2 align="center">Usage</h2>
```bash
npm i -S|-D postcss-plugin
```
Install all required postcss plugins and save them to your **package.json** `dependencies`/`devDependencies`
Then create a postcss config file by choosing one of the following formats
### `package.json`
Create a **`postcss`** section in your project's **`package.json`**
```
Project (Root)
|– client
|– public
|
|- package.json
```
```json
{
"postcss": {
"parser": "sugarss",
"map": false,
"plugins": {
"postcss-plugin": {}
}
}
}
```
### `.postcssrc`
Create a **`.postcssrc`** file in JSON or YAML format
> ℹ️ It's recommended to use an extension (e.g **`.postcssrc.json`** or **`.postcssrc.yml`**) instead of `.postcssrc`
```
Project (Root)
|– client
|– public
|
|- (.postcssrc|.postcssrc.json|.postcssrc.yml)
|- package.json
```
**`.postcssrc.json`**
```json
{
"parser": "sugarss",
"map": false,
"plugins": {
"postcss-plugin": {}
}
}
```
**`.postcssrc.yml`**
```yaml
parser: sugarss
map: false
plugins:
postcss-plugin: {}
```
### `.postcssrc.js` or `postcss.config.js`
You may need some logic within your config. In this case create JS file named **`.postcssrc.js`** or **`postcss.config.js`**
```
Project (Root)
|– client
|– public
|
|- (.postcssrc.js|postcss.config.js)
|- package.json
```
You can export the config as an `{Object}`
**.postcssrc.js**
```js
module.exports = {
parser: 'sugarss',
map: false,
plugins: {
'postcss-plugin': {}
}
}
```
Or export a `{Function}` that returns the config (more about the `ctx` param below)
**.postcssrc.js**
```js
module.exports = (ctx) => ({
parser: ctx.parser ? 'sugarss' : false,
map: ctx.env === 'development' ? ctx.map : false,
plugins: {
'postcss-plugin': ctx.options.plugin
}
})
```
Plugins can be loaded either using an `{Object}` or an `{Array}`
#### `{Object}`
**.postcssrc.js**
```js
module.exports = ({ env }) => ({
...options,
plugins: {
'postcss-plugin': env === 'production' ? {} : false
}
})
```
#### `{Array}`
**.postcssrc.js**
```js
module.exports = ({ env }) => ({
...options,
plugins: [
env === 'production' ? require('postcss-plugin')() : false
]
})
```
> :warning: When using an `{Array}`, make sure to `require()` each plugin
<h2 align="center">Options</h2>
|Name|Type|Default|Description|
|:--:|:--:|:-----:|:----------|
|[**`to`**](#to)|`{String}`|`undefined`|Destination File Path|
|[**`map`**](#map)|`{String\|Object}`|`false`|Enable/Disable Source Maps|
|[**`from`**](#from)|`{String}`|`undefined`|Source File Path|
|[**`parser`**](#parser)|`{String\|Function}`|`false`|Custom PostCSS Parser|
|[**`syntax`**](#syntax)|`{String\|Function}`|`false`|Custom PostCSS Syntax|
|[**`stringifier`**](#stringifier)|`{String\|Function}`|`false`|Custom PostCSS Stringifier|
### `parser`
**.postcssrc.js**
```js
module.exports = {
parser: 'sugarss'
}
```
### `syntax`
**.postcssrc.js**
```js
module.exports = {
syntax: 'postcss-scss'
}
```
### `stringifier`
**.postcssrc.js**
```js
module.exports = {
stringifier: 'midas'
}
```
### [**`map`**](https://github.com/postcss/postcss/blob/master/docs/source-maps.md)
**.postcssrc.js**
```js
module.exports = {
map: 'inline'
}
```
> :warning: In most cases `options.from` && `options.to` are set by the third-party which integrates this package (CLI, gulp, webpack). It's unlikely one needs to set/use `options.from` && `options.to` within a config file. Unless you're a third-party plugin author using this module and its Node API directly **dont't set `options.from` && `options.to` yourself**
### `to`
```js
module.exports = {
to: 'path/to/dest.css'
}
```
### `from`
```js
module.exports = {
from: 'path/to/src.css'
}
```
<h2 align="center">Plugins</h2>
### `{} || null`
The plugin will be loaded with defaults
```js
'postcss-plugin': {} || null
```
**.postcssrc.js**
```js
module.exports = {
plugins: {
'postcss-plugin': {} || null
}
}
```
> :warning: `{}` must be an **empty** `{Object}` literal
### `{Object}`
The plugin will be loaded with given options
```js
'postcss-plugin': { option: '', option: '' }
```
**.postcssrc.js**
```js
module.exports = {
plugins: {
'postcss-plugin': { option: '', option: '' }
}
}
```
### `false`
The plugin will not be loaded
```js
'postcss-plugin': false
```
**.postcssrc.js**
```js
module.exports = {
plugins: {
'postcss-plugin': false
}
}
```
### `Ordering`
Plugin **execution order** is determined by declaration in the plugins section (**top-down**)
```js
{
plugins: {
'postcss-plugin': {}, // [0]
'postcss-plugin': {}, // [1]
'postcss-plugin': {} // [2]
}
}
```
<h2 align="center">Context</h2>
When using a `{Function}` (`postcss.config.js` or `.postcssrc.js`), it's possible to pass context to `postcss-load-config`, which will be evaluated while loading your config. By default `ctx.env (process.env.NODE_ENV)` and `ctx.cwd (process.cwd())` are available on the `ctx` `{Object}`
> ℹ️ Most third-party integrations add additional properties to the `ctx` (e.g `postcss-loader`). Check the specific module's README for more information about what is available on the respective `ctx`
<h2 align="center">Examples</h2>
**postcss.config.js**
```js
module.exports = (ctx) => ({
parser: ctx.parser ? 'sugarss' : false,
map: ctx.env === 'development' ? ctx.map : false,
plugins: {
'postcss-import': {},
'postcss-nested': {},
cssnano: ctx.env === 'production' ? {} : false
}
})
```
<div align="center">
<img width="80" height="80" src="https://worldvectorlogo.com/logos/nodejs-icon.svg">
</div>
```json
"scripts": {
"build": "NODE_ENV=production node postcss",
"start": "NODE_ENV=development node postcss"
}
```
### `Async`
```js
const { readFileSync } = require('fs')
const postcss = require('postcss')
const postcssrc = require('postcss-load-config')
const css = readFileSync('index.sss', 'utf8')
const ctx = { parser: true, map: 'inline' }
postcssrc(ctx).then(({ plugins, options }) => {
postcss(plugins)
.process(css, options)
.then((result) => console.log(result.css))
})
```
### `Sync`
```js
const { readFileSync } = require('fs')
const postcss = require('postcss')
const postcssrc = require('postcss-load-config')
const css = readFileSync('index.sss', 'utf8')
const ctx = { parser: true, map: 'inline' }
const { plugins, options } = postcssrc.sync(ctx)
```
<div align="center">
<img width="80" height="80" halign="10" src="https://worldvectorlogo.com/logos/gulp.svg">
</div>
```json
"scripts": {
"build": "NODE_ENV=production gulp",
"start": "NODE_ENV=development gulp"
}
```
```js
const { task, src, dest, series, watch } = require('gulp')
const postcss = require('gulp-postcssrc')
const css = () => {
src('src/*.css')
.pipe(postcss())
.pipe(dest('dest'))
})
task('watch', () => {
watch(['src/*.css', 'postcss.config.js'], css)
})
task('default', series(css, 'watch'))
```
<div align="center">
<img width="80" height="80" src="https://cdn.rawgit.com/webpack/media/e7485eb2/logo/icon.svg">
</div>
```json
"scripts": {
"build": "NODE_ENV=production webpack",
"start": "NODE_ENV=development webpack-dev-serve
没有合适的资源?快使用搜索试试~ 我知道了~
postcss-load-config:PostCSS的自动加载配置
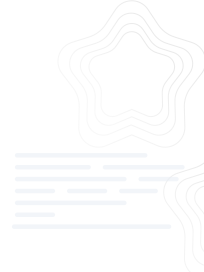
共62个文件
js:19个
css:12个
sss:12个


温馨提示
加载配置 安装 npm i -D postcss-load-config 用法 npm i -S | -D postcss-plugin 安装所有必需的postcss插件并将其保存到package.json dependencies / devDependencies 然后通过选择以下格式之一创建一个postcss配置文件 package.json 在项目的package.json创建一个postcss部分 Project (Root) |– client |– public | |- package.json { " postcss " : { " parser " : " sugarss " , " map " : false , " plugins " : { " postcss-plugin " : {} }
资源推荐
资源详情
资源评论
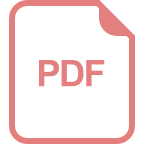
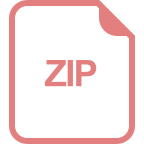
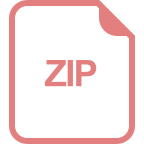
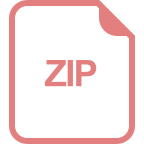
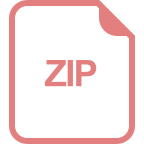
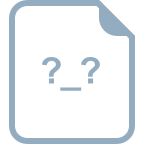
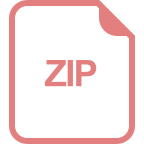
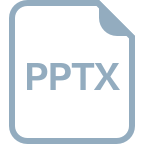
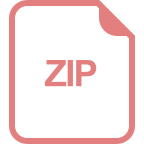
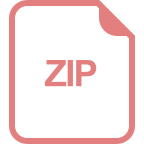
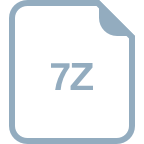
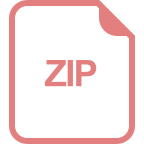
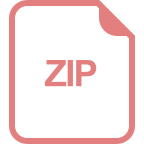
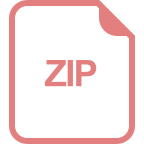
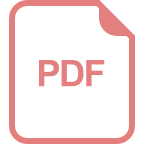
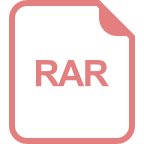
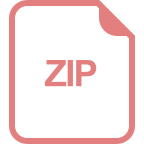
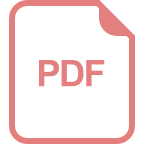
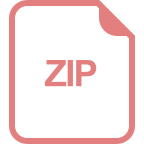
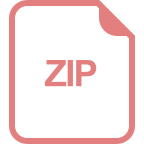
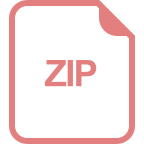
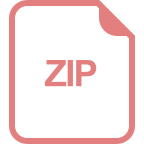
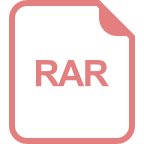
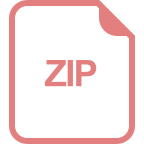
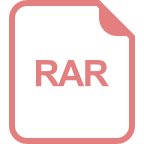
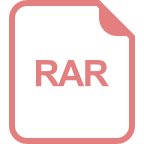
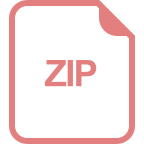
收起资源包目录



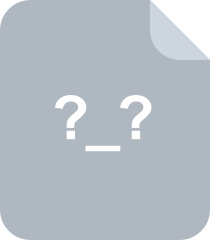
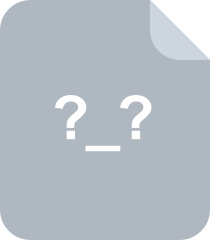

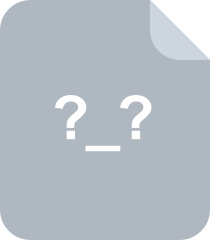
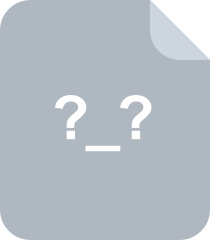
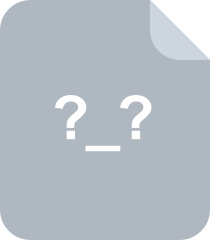

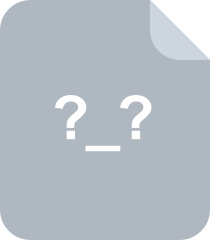
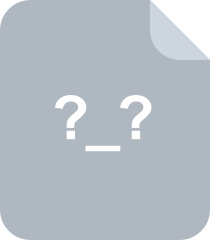
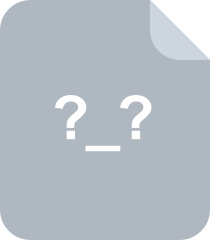
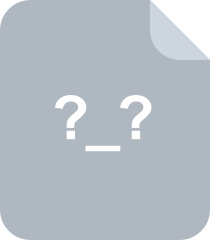
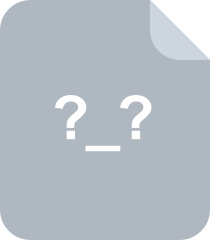
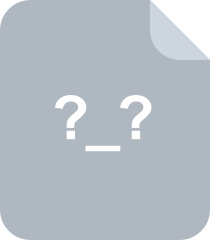
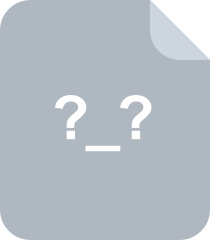
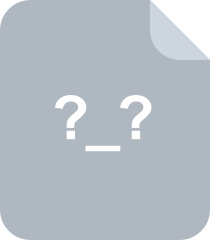
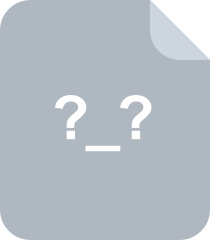



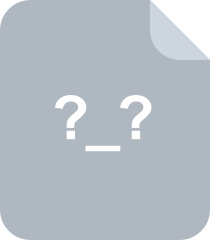
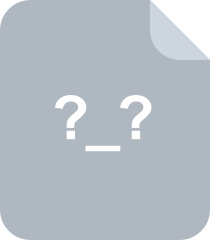

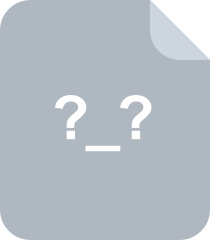
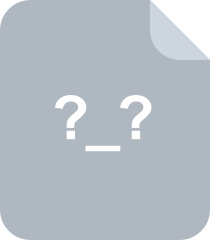

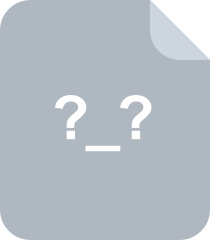
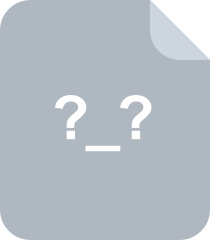
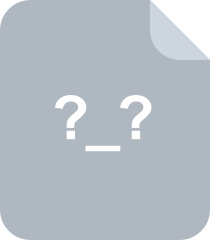
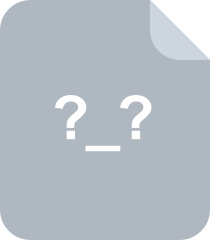
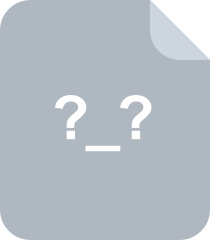



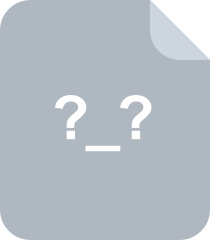
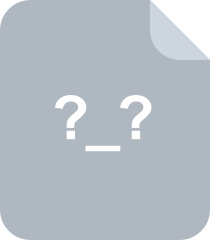
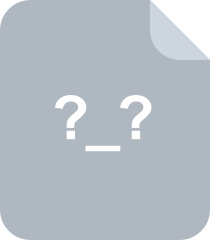

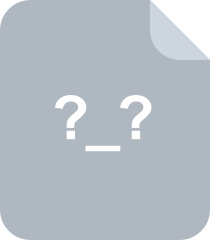
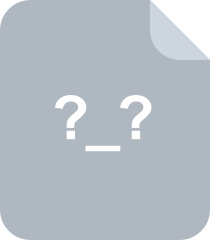

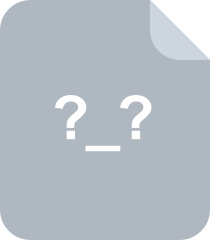
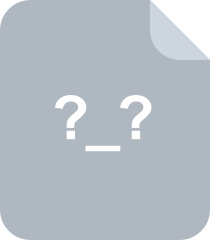


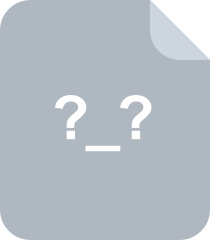
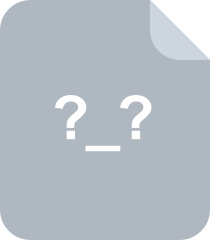
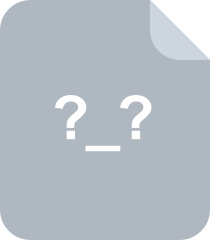

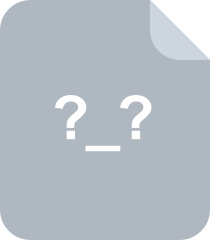
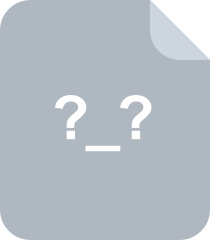

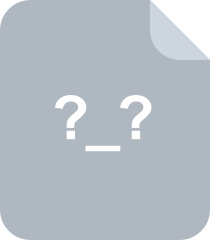
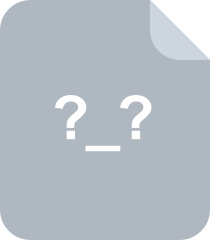
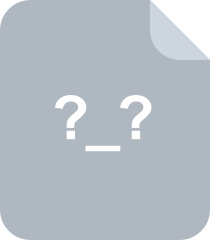



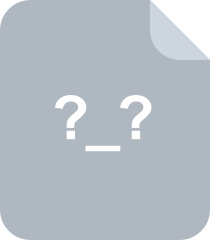

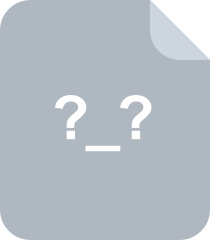
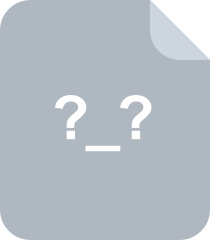
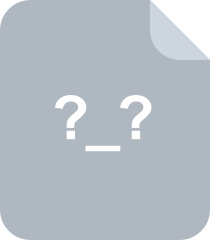

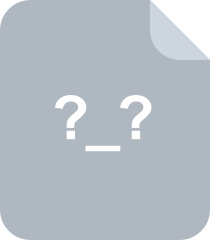

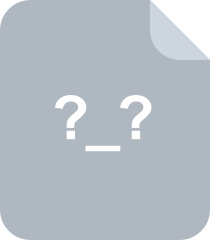


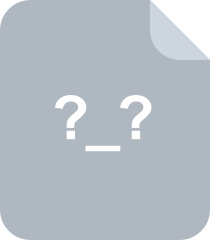

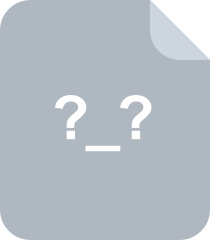

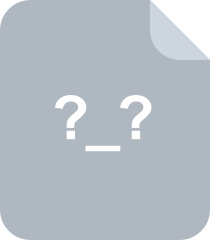


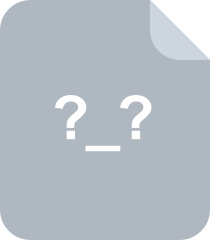
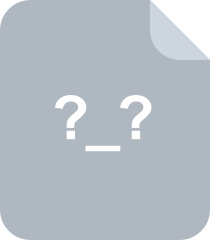

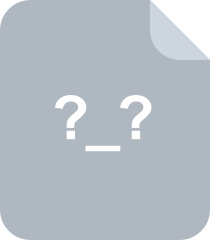
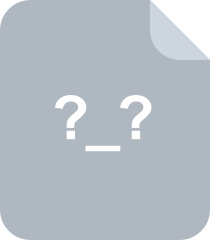

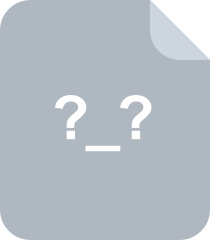
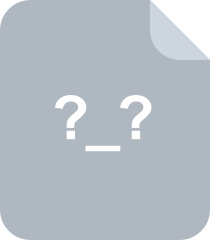
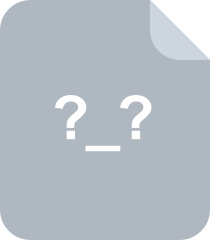
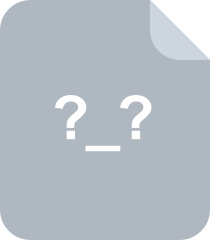
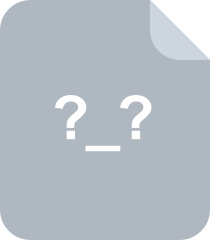
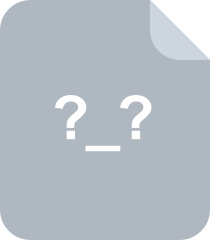
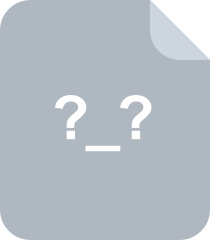
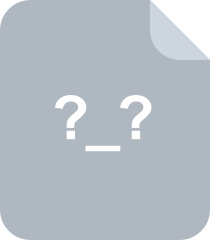
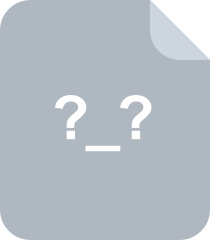
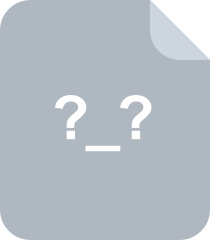
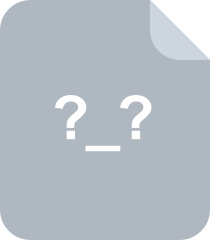
共 62 条
- 1
资源评论
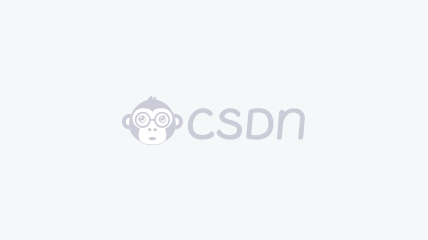
- sunsuncode2021-08-20用户下载后在一定时间内未进行评价,系统默认好评。

初見目
- 粉丝: 22
- 资源: 4594
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

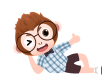
最新资源
- 学校课程软件工程常见10道题目以及答案demo
- javaweb新手开发中常见的目录结构讲解
- 新手小白的git使用的手册入门学习demo
- 基于Java观察者模式的info-express多对多广播通信框架设计源码
- 利用python爬取豆瓣电影评分简单案例demo
- 机器人开发中常见的几道问题以及答案demo
- 基于SpringBoot和layuimini的简洁美观后台权限管理系统设计源码
- 实验报告五六代码.zip
- hdw-dubbo-ui基于vue、element-ui构建开发,实现后台管理前端功能.zip
- (Grafana + Zabbix + ASP.NET Core 2.1 + ECharts + Dapper + Swagger + layuiAdmin)基于角色授权的权限体系.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


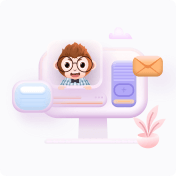
安全验证
文档复制为VIP权益,开通VIP直接复制
