# Shizuku
## Background
When developing apps that requires root, the most common method is to run some commands in the su shell. For example, there is an app that uses the `pm enable/disable` command to enable/disable components.
This method has very big disadvantages:
1. **Extremely slow** (Multiple process creation)
2. Needs to process texts (**Super unreliable**)
3. The possibility is limited to available commands
4. Even if ADB has sufficient permissions, the app requires root privileges to run
Shizuku uses a completely different way. See detailed description below.
## User guide & Download
<https://shizuku.rikka.app/>
## How does Shizuku work?
First, we need to talk about how app use system APIs. For example, if the app wants to get installed apps, we all know we should use `PackageManager#getInstalledPackages()`. This is actually an interprocess communication (IPC) process of the app process and system server process, just the Android framework did the inner works for us.
Android uses `binder` to do this type of IPC. `Binder` allows the server-side to learn the uid and pid of the client-side, so that the system server can check if the app has the permission to do the operation.
Usually, if there is a "manager" (e.g., `PackageManager`) for apps to use, there should be a "service" (e.g., `PackageManagerService`) in the system server process. We can simply think if the app holds the `binder` of the "service", it can communicate with the "service". The app process will receive binders of system services on start.
Shizuku guides users to run a process, Shizuku server, with root or ADB first. When the app starts, the `binder` to Shizuku server will also be sent to the app.
The most important feature Shizuku provides is something like be a middle man to receive requests from the app, sent them to the system server, and send back the results. You can see the `transactRemote` method in `moe.shizuku.server.ShizukuService` class, and `moe.shizuku.api.ShizukuBinderWrapper` class for the detail.
So, we reached our goal, to use system APIs with higher permission. And to the app, it is almost identical to the use of system APIs directly.
## Developer guide
### API & sample
https://github.com/RikkaApps/Shizuku-API
### Migrating from pre-v11
> Existing applications still works, of course.
https://github.com/RikkaApps/Shizuku-API#migration-guide-for-existing-applications-use-shizuku-pre-v11
### Attention
1. ADB permissions are limited
ADB has limited permissions and different on various system versions. You can see permissions granted to ADB [here](https://github.com/aosp-mirror/platform_frameworks_base/blob/master/packages/Shell/AndroidManifest.xml).
Before calling the API, you can use `ShizukuService#getUid` to check if Shizuku is running user ADB, or use `ShizukuService#checkPermission` to check if the server has sufficient permissions.
2. Hidden API limitation from Android 9
As of Android 9, the usage of the hidden APIs is limited for normal apps. Please use other methods (such as <https://github.com/tiann/FreeReflection>).
3. Android 8.0 & ADB
At present, the way Shizuku service gets the app process is to combine `IActivityManager#registerProcessObserver` and `IActivityManager#registerUidObserver` (26+) to ensure that the app process will be sent when the app starts. However, on API 26, ADB lacks permissions to use `registerUidObserver`, so if you need to use Shizuku in a process that might not be started by an Activity, it is recommended to trigger the send binder by starting a transparent activity.
4. Direct use of `transactRemote` requires attention
* The API may be different under different Android versions, please be sure to check it carefully. Also, the `android.app.IActivityManager` has the aidl form in API 26 and later, and `android.app.IActivityManager$Stub` exists only on API 26.
* `SystemServiceHelper.getTransactionCode` may not get the correct transaction code, such as `android.content.pm.IPackageManager$Stub.TRANSACTION_getInstalledPackages` does not exist on API 25 and there is `android.content.pm.IPackageManager$Stub.TRANSACTION_getInstalledPackages_47` (this situation has been dealt with, but it is not excluded that there may be other circumstances). This problem is not encountered with the `ShizukuBinderWrapper` method.
## Developing Shizuku itself
### Build
- Clone with `git clone --recurse-submodules`
- Run gradle task `:manager:assembleDebug` or `:manager:assembleRelease`
The `:manager:assembleDebug` task generates a debuggable server. You can attach a debugger to `shizuku_server` to debug the server.
## License
This project is available under the Apache-2.0 license.
### Exceptions
* You are **FORBIDDEN** to use image files listed below in any way (unless for displaying Shizuku itself).
```
manager/src/main/res/mipmap-hdpi/ic_launcher.png
manager/src/main/res/mipmap-hdpi/ic_launcher_background.png
manager/src/main/res/mipmap-hdpi/ic_launcher_foreground.png
manager/src/main/res/mipmap-xhdpi/ic_launcher.png
manager/src/main/res/mipmap-xhdpi/ic_launcher_background.png
manager/src/main/res/mipmap-xhdpi/ic_launcher_foreground.png
manager/src/main/res/mipmap-xxhdpi/ic_launcher.png
manager/src/main/res/mipmap-xxhdpi/ic_launcher_background.png
manager/src/main/res/mipmap-xxhdpi/ic_launcher_foreground.png
manager/src/main/res/mipmap-xxxhdpi/ic_launcher.png
manager/src/main/res/mipmap-xxxhdpi/ic_launcher_background.png
manager/src/main/res/mipmap-xxxhdpi/ic_launcher_foreground.png
```
* You are **FORBIDDEN** to distribute the apk compiled by you (including modified, e.g., rename "Shizuku" to something else) to any store (IBNLT Google Play Store, etc.).
没有合适的资源?快使用搜索试试~ 我知道了~
Shizuku:通过以app_process开头的Java进程直接从普通应用程序使用具有adbroot特权的系统API
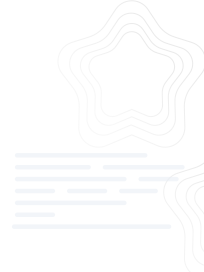
共339个文件
xml:108个
java:105个
kt:57个


温馨提示
uku宿 背景 在开发需要root的应用程序时,最常见的方法是在su shell中运行一些命令。 例如,有一个应用程序使用pm enable/disable命令启用/禁用组件。 这种方法有很大的缺点: 极慢(创建多个进程) 需要处理文本(超级不可靠) 可能性仅限于可用命令 即使ADB具有足够的权限,该应用也需要具有root权限才能运行 Shizuku使用完全不同的方式。 请参阅下面的详细说明。 用户指南和下载 Shizuku如何工作? 首先,我们需要讨论应用程序如何使用系统API。 例如,如果应用程序想要安装应用程序,我们都知道我们应该使用PackageManager#getInstalledPackages() 。 这实际上是应用程序进程和系统服务器进程的进程间通信(IPC)进程,只是Android框架为我们完成了内部工作。 Android使用binder来执行这种类型的IP
资源详情
资源评论
资源推荐
收起资源包目录

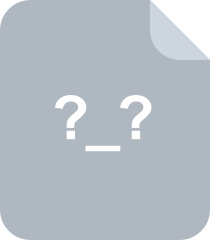
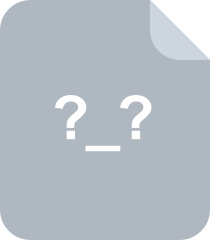
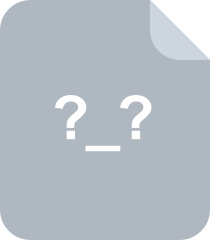
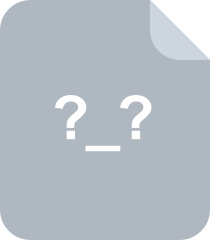
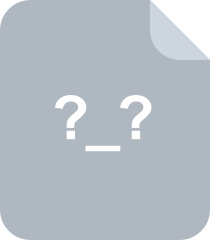
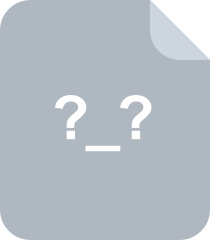
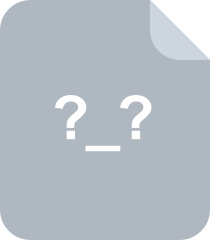
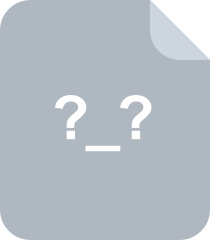
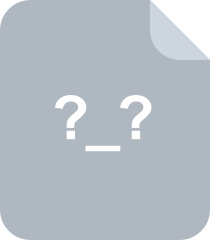
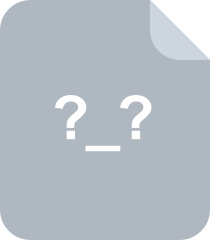
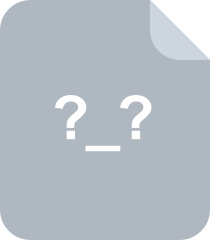
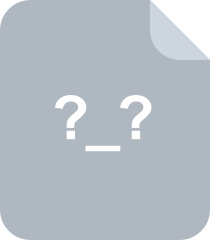
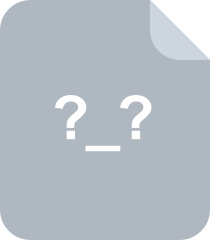
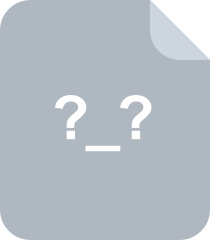
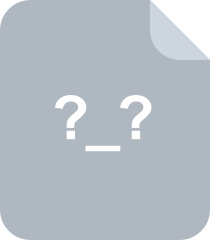
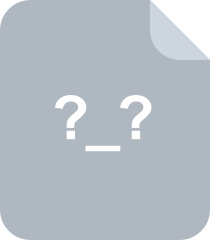
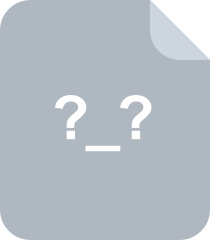
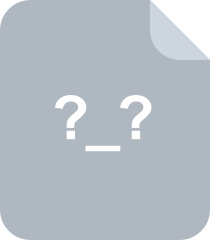
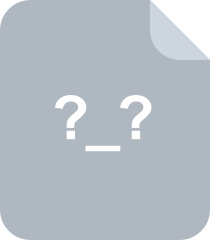
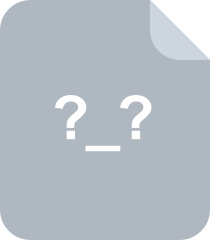
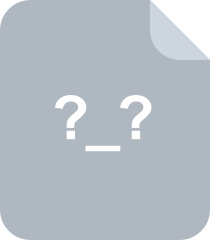
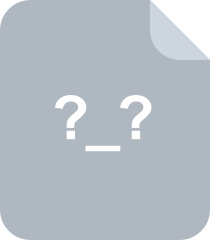
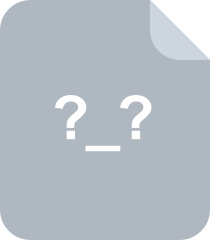
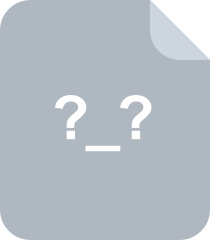
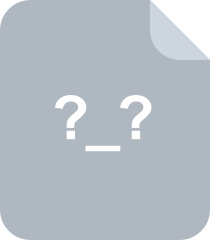
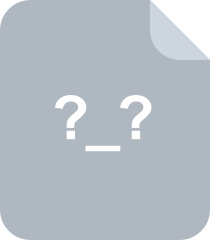
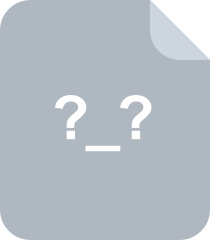
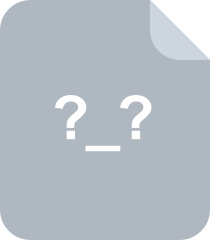
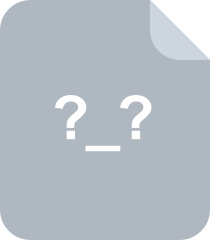
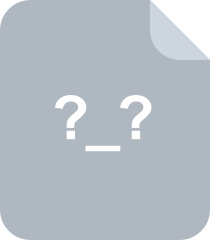
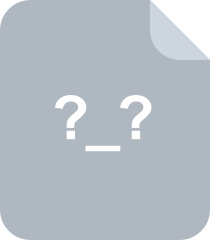
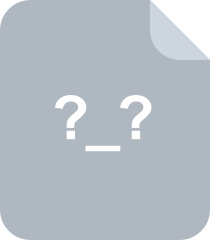
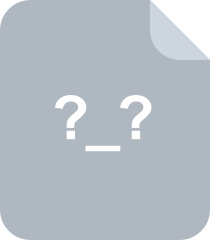
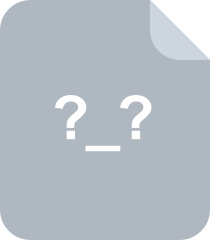
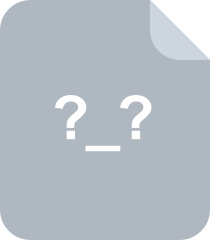
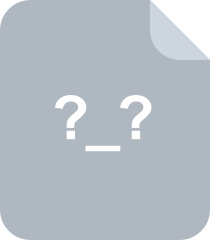
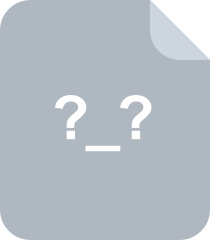
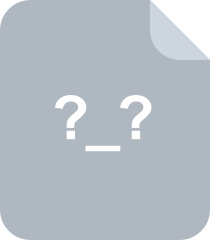
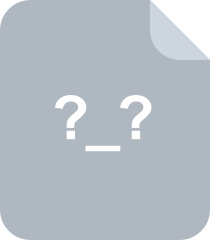
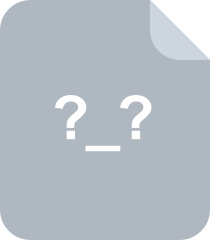
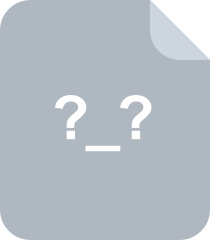
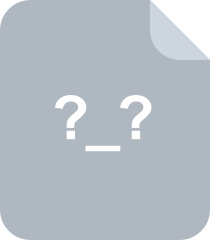
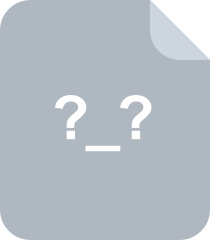
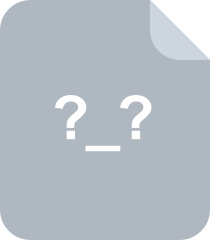
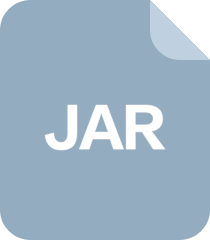
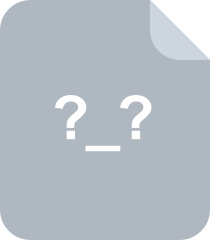
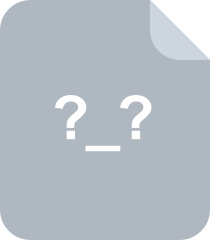
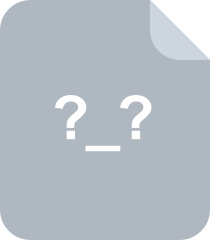
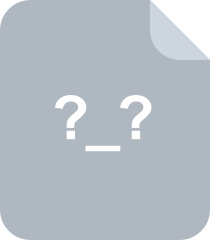
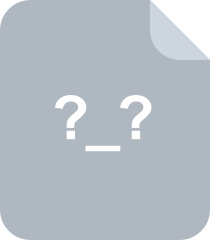
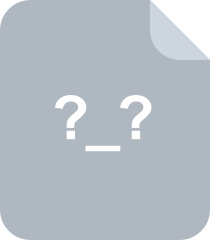
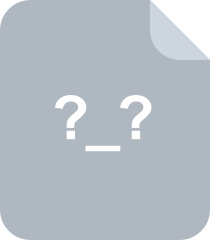
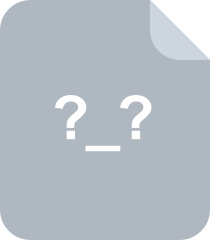
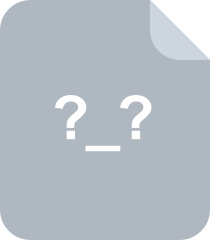
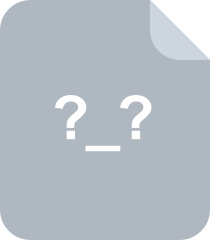
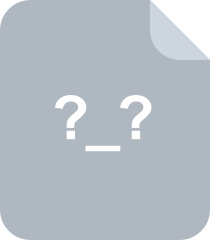
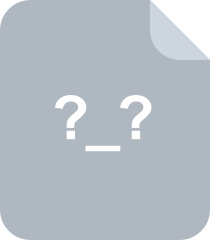
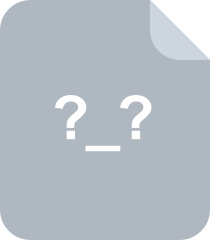
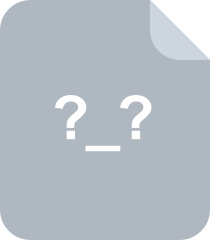
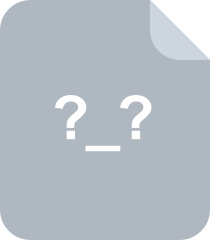
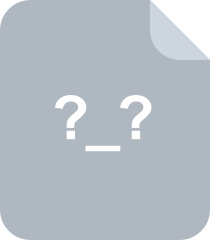
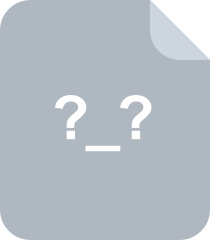
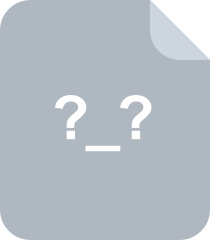
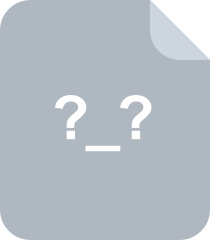
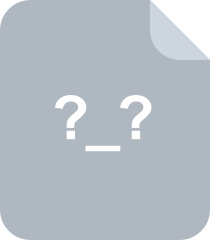
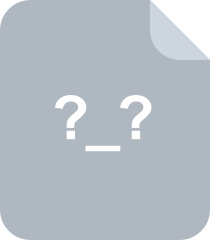
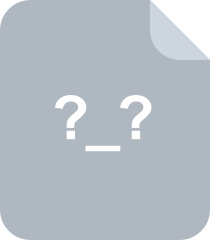
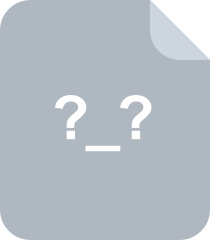
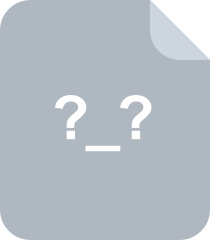
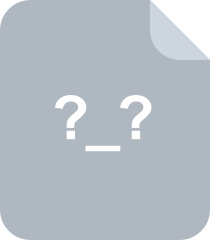
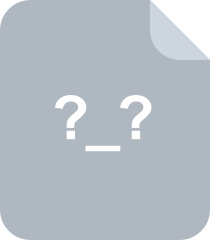
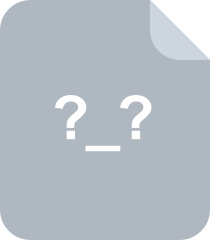
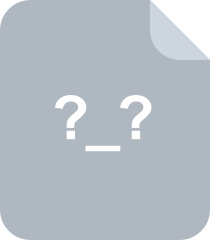
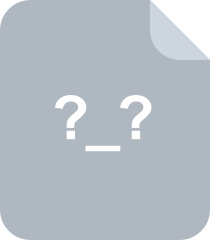
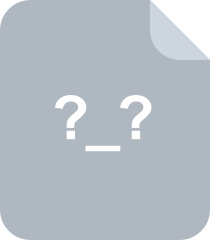
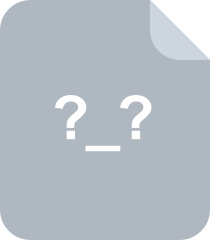
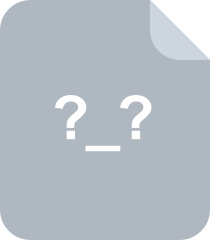
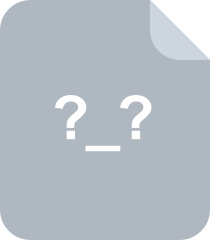
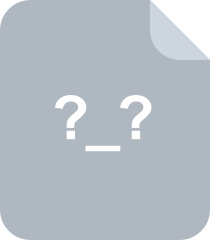
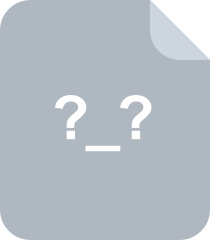
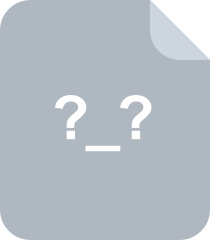
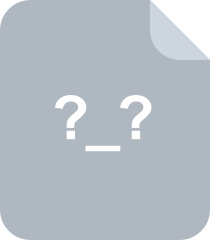
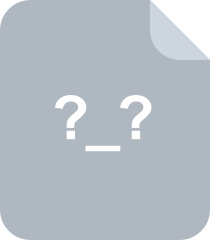
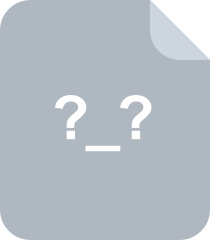
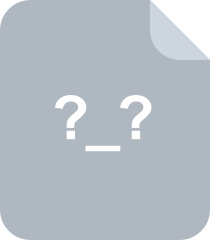
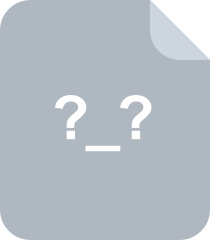
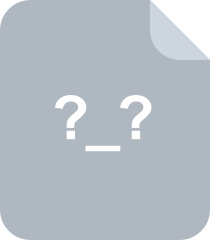
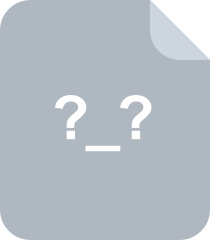
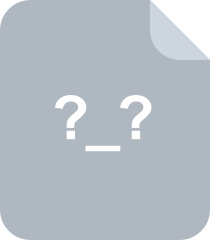
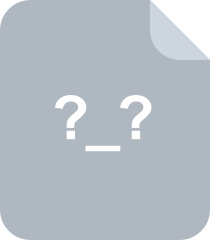
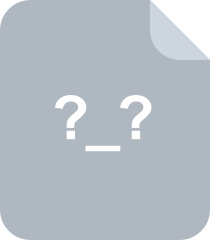
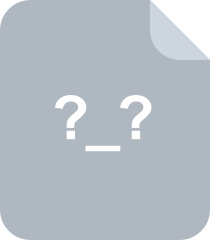
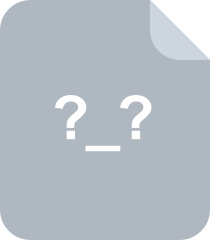
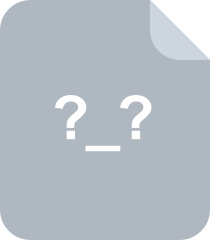
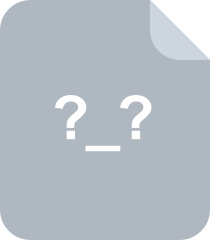
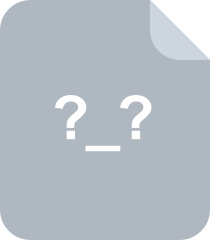
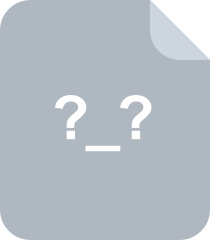
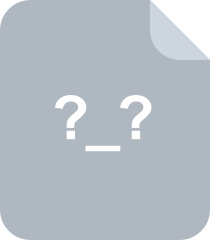
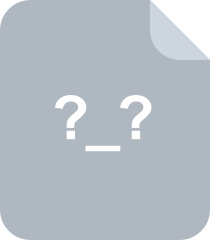
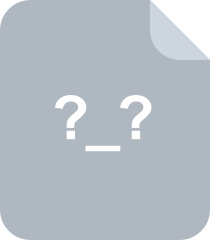
共 339 条
- 1
- 2
- 3
- 4
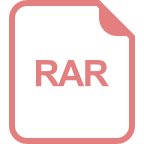
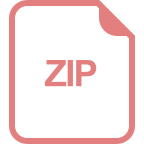
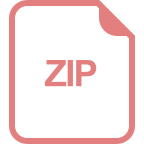
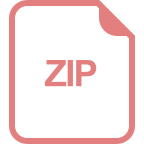
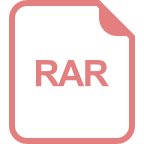
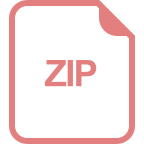
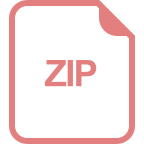
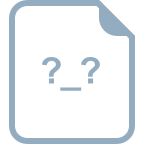
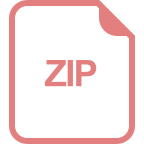
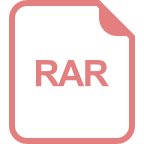
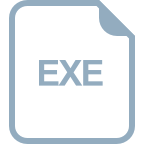
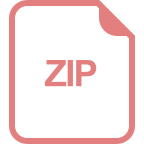
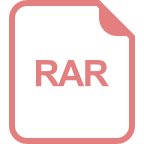
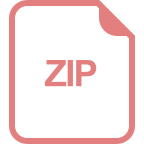
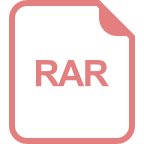
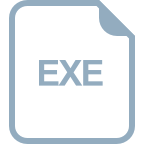
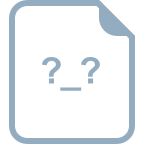
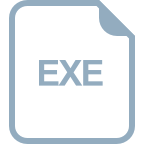
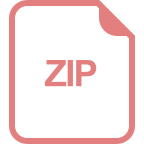

悦微评剧
- 粉丝: 19
- 资源: 4668
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

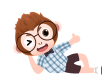
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


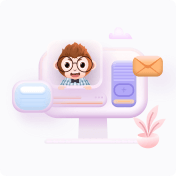
安全验证
文档复制为VIP权益,开通VIP直接复制

评论1