# ScrollMagic <a href='https://github.com/janpaepke/ScrollMagic/blob/master/CHANGELOG.md' class='version' title='Whats New?'>v2.0.8</a> [](https://travis-ci.org/janpaepke/ScrollMagic)
### The javascript library for magical scroll interactions.
[](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=8BJC8B58XHKLL 'Shut up and take my money!') <a href="https://nerdpool.io/support/scrollmagic?utm_source=github&utm_medium=btn" title="Get personal live support"><img src="https://cdn.nerdpool.io/np-btn-support-blue.png" style="height: 40px" height="40" alt="Request personal live support"></a>
**Quicklinks:** [About](#about-the-library) | [Download](#availability) | [Installation](#installation) | [Usage](#usage) | [Help](#help) | [Compatibility](#browser-support) | [Author](#about-the-author) | [License](#license) | [Thanks](#thanks)
---
ScrollMagic helps you to easily react to the user's current scroll position.
It's the perfect library for you, if you want to ...
- animate based on scroll position – either trigger an animation or synchronize it to the scrollbar movement (like a playback scrub control).
- pin an element starting at a specific scroll position – either indefinitely or for a limited amount of scroll progress (sticky elements).
- toggle CSS classes of elements on and off based on scroll position.
- effortlessly add parallax effects to your website.
- create an infinitely scrolling page (ajax load of additional content).
- add callbacks at specific scroll positions or while scrolling past a specific section, passing a progress parameter.
Check out [the demo page](http://scrollmagic.io), browse [the examples](http://scrollmagic.io/examples/index.html) or read [the documentation](http://scrollmagic.io/docs/index.html) to get started.
If you want to contribute please [get in touch](mailto:e-mail@janpaepke.de) and let me know about your specialty and experience.
## About the Library
ScrollMagic is a scroll interaction library.
It's a complete rewrite of its predecessor [Superscrollorama](https://github.com/johnpolacek/superscrollorama) by [John Polacek](http://johnpolacek.com).
A plugin-based architecture offers easy customizability and extendability.
To implement animations, ScrollMagic can work with multiple frameworks.
The recommended solution is the [Greensock Animation Platform (GSAP)](http://www.greensock.com/gsap-js/) due to its stability and feature richness. For a more lightweight approach the [VelocityJS](http://VelocityJS.org) framework is also supported. Alternatively custom extensions can be implemented or the necessity of a framework can be completely avoided by animating simply using CSS and class toggles.
ScrollMagic was developed with these principles in mind:
* optimized performance
* lightweight (6KB gzipped)
* flexibility and extendibility
* mobile compatibility
* event management
* support for responsive web design
* object oriented programming and object chaining
* readable, centralized code and intuitive development
* support for both x and y direction scrolling (even both on one page)
* support for scrolling inside div containers (even multiple on one page)
* extensive debugging and logging capabilities
* detailed documentation
* many application examples
**Is ScrollMagic the right library for you?**
ScrollMagic takes an object oriented approach using a controller for each scroll container and attaching multiple scenes defining what should happen at what part of the page. While this offers a great deal of control, it might be a little confusing, especially if you're just starting out with javascript.
If the above points are not crucial for you and you are just looking for a simple solution to implement css animations I would strongly recommend taking a look at the awesome [skrollr](http://prinzhorn.github.io/skrollr/) project. It almost solely relies on element attributes and thus requires minimal to no javascript knowledge.
## Availability
To get your copy of ScrollMagic you have the choice between four options:
**Option 1: GitHub**
Download a zip file containing the source code, demo page, all examples and documentation from the [GitHub releases page](https://github.com/janpaepke/ScrollMagic/releases) or clone the package to your machine using the git command line interface:
```bash
git clone https://github.com/janpaepke/ScrollMagic.git
```
**Option 2: Bower**
ScrollMagic is also [available on bower](http://bower.io/search/?q=scrollmagic) and will only install the necessary source code, ignoring all example and documentation files.
Please mind that since they are not core dependencies, you will have to add frameworks like GSAP, jQuery or Velocity manually, should you choose to use them.
```bash
bower install scrollmagic
```
**Option 3: npm**
If you prefer the [node package manager](https://www.npmjs.com/package/scrollmagic), feel free to use it.
Keep in mind that like with bower non-crucial files will be ignored (see above).
```bash
npm install scrollmagic
```
**Option 4: CDN**
If you don't want to host ScrollMagic yourself, you can include it from [cdnjs](https://cdnjs.com/libraries/ScrollMagic):
```
https://cdnjs.cloudflare.com/ajax/libs/ScrollMagic/2.0.8/ScrollMagic.min.js
```
All plugins and uncompressed files are also available on cdnjs.
For example:
```
https://cdnjs.cloudflare.com/ajax/libs/ScrollMagic/2.0.8/plugins/debug.addIndicators.js
https://cdnjs.cloudflare.com/ajax/libs/ScrollMagic/2.0.8/plugins/debug.addIndicators.min.js
```
## Installation
Include the **core** library in your HTML file:
```html
<script src="js/scrollmagic/uncompressed/ScrollMagic.js"></script>
```
And you're ready to go!
For deployment use the minified version **instead**:
```html
<script src="js/scrollmagic/minified/ScrollMagic.min.js"></script>
```
_**NOTE:** The logging feature is removed in the minified version due to file size considerations._
To use **plugins** like the indicators visualization, simply include them additionally to the main library:
```html
<script src="js/scrollmagic/uncompressed/plugins/debug.addIndicators.js"></script>
```
To learn how to configure **RequireJS**, when using AMD, please [read here](https://github.com/janpaepke/ScrollMagic/wiki/Getting-Started-:-Using-AMD).
## Usage
The basic ScrollMagic design pattern is one controller, which has one or more scenes attached to it.
Each scene is used to define what happens when the container is scrolled to a specific offset.
Here's a basic workflow example:
```javascript
// init controller
var controller = new ScrollMagic.Controller();
// create a scene
new ScrollMagic.Scene({
duration: 100, // the scene should last for a scroll distance of 100px
offset: 50 // start this scene after scrolling for 50px
})
.setPin('#my-sticky-element') // pins the element for the the scene's duration
.addTo(controller); // assign the scene to the controller
```
To learn more about the ScrollMagic code structure, please [read here](https://github.com/janpaepke/ScrollMagic/wiki/Getting-Started-:-How-to-use-ScrollMagic).
## Help
To get started, check out the available learning resources [in the wiki section](https://github.com/janpaepke/ScrollMagic/wiki).
Be sure to have a look at the [examples](http://janpaepke.github.com/ScrollMagic/examples/index.html) to get source code pointers and make use of the [documentation](http://janpaepke.github.com/ScrollMagic/docs/index.html) for a complete reference.
If you run into trouble using ScrollMagic please follow the [Troubleshooting guide](https://github.com/janpaepke/ScrollMagic/wiki/Troubleshooting-Guide).
**Please do not post support requests in the github issue section**, as it's reserverd for issue and bug reporting.
If all above options for self-help fail, please
没有合适的资源?快使用搜索试试~ 我知道了~
ScrollMagic:神奇的滚动交互的javascript库
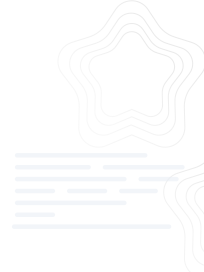
共274个文件
js:115个
html:59个
png:38个


温馨提示
ScrollMagic 神奇的滚动交互的javascript库。 快速链接:| | | | | | | ScrollMagic可帮助您轻松响应用户的当前滚动位置。 如果您想...它是您的理想图书馆。 根据滚动位置设置动画–触发动画或将其同步到滚动条移动(如播放擦洗控件)。 从特定的滚动位置开始固定元素-无限期或有限的滚动进度(粘性元素)。 根据滚动位置打开和关闭元素CSS类。 轻松地将视差效果添加到您的网站。 创建一个无限滚动的页面(附加内容的ajax加载)。 通过进度参数在特定滚动位置或滚动经过特定部分时添加回调。 查看,浏览或阅读以开始使用。 如果您想做出贡献,请,让我知道您的专业和经验。 关于图书馆 ScrollMagic是滚动交互库。 这是对其前身的完全重写。 基于插件的体系结构可轻松实现自定义和扩展。 要实现动画,ScrollMagic可以与多个框架一起使用
资源详情
资源评论
资源推荐
收起资源包目录

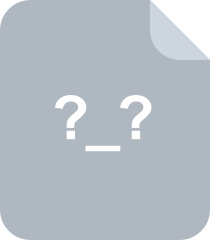
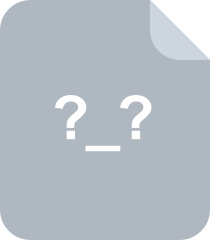
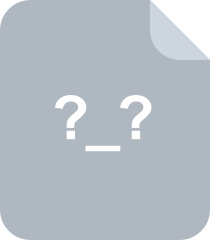
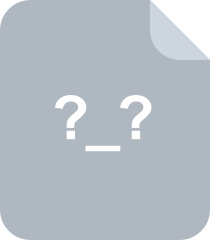
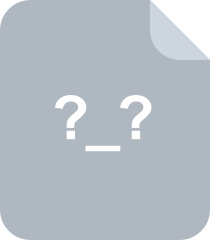
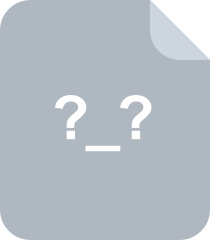
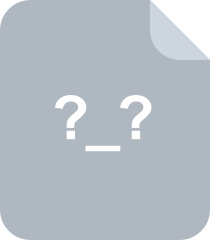
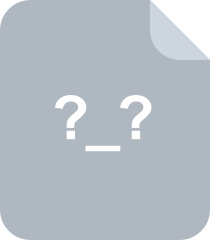
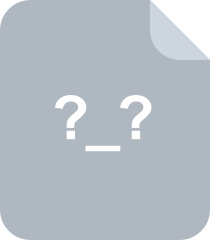
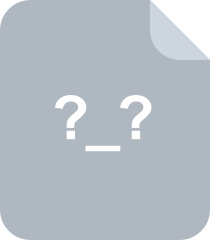
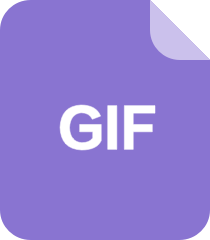
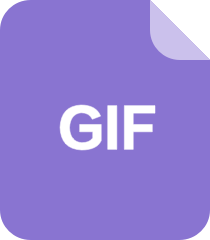
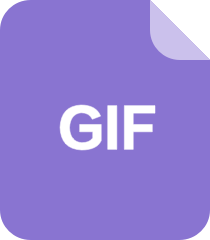
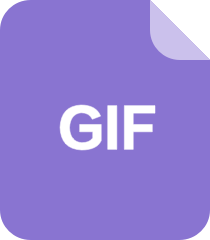
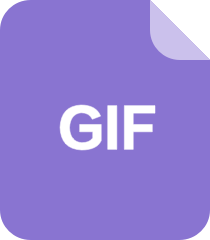
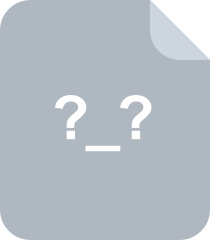
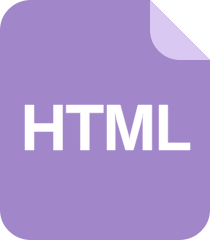
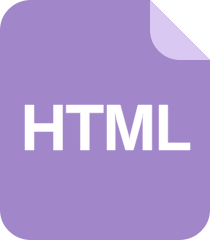
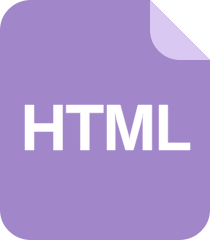
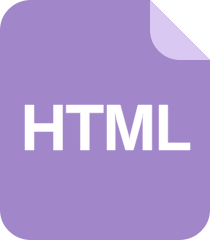
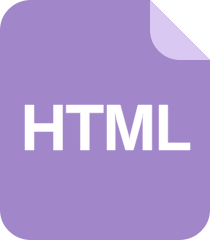
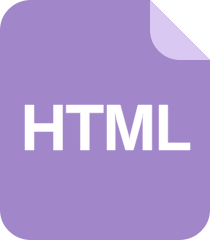
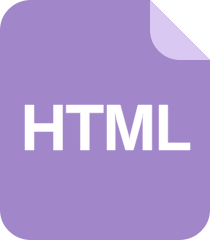
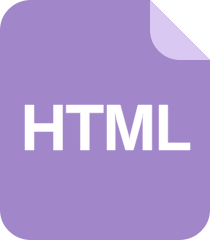
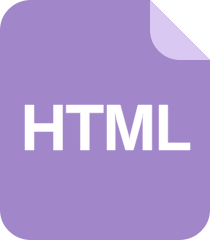
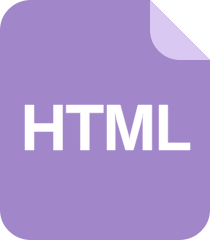
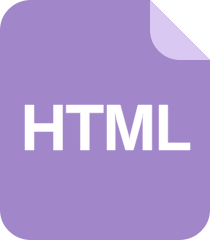
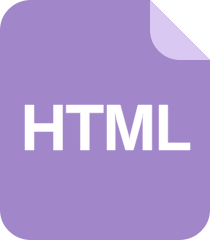
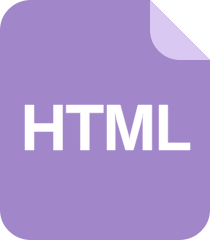
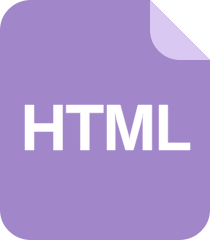
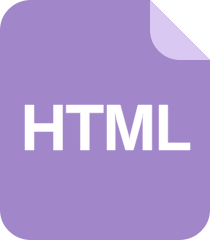
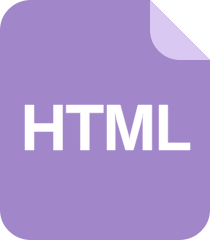
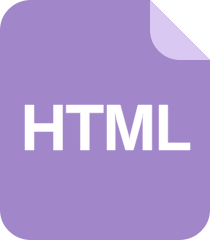
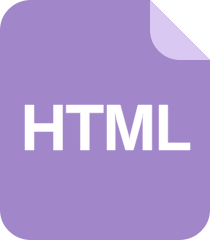
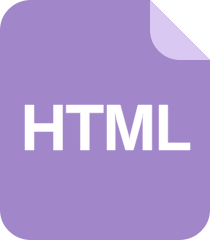
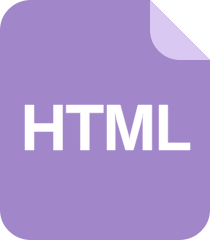
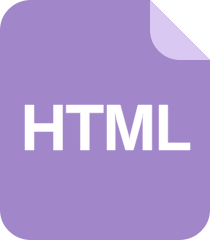
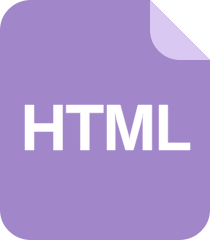
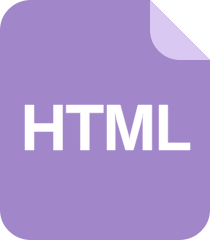
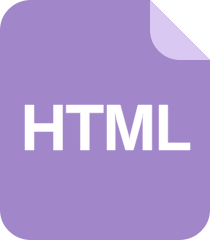
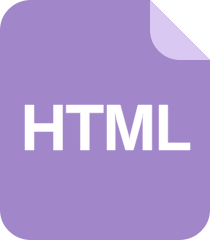
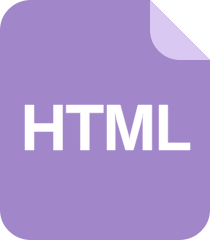
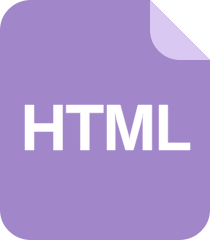
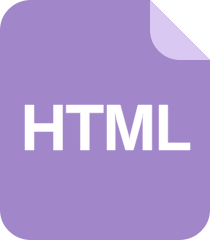
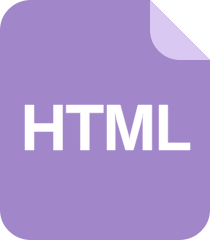
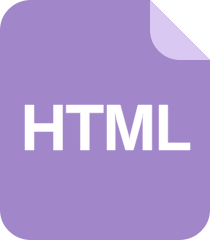
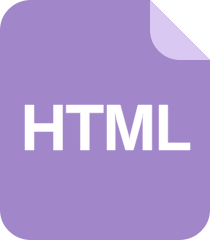
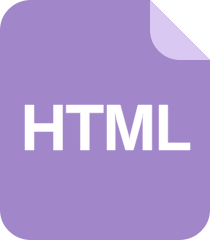
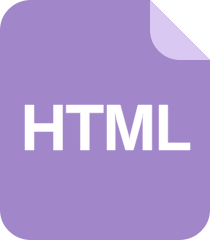
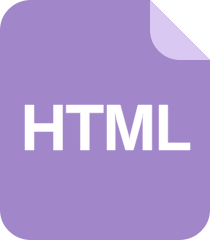
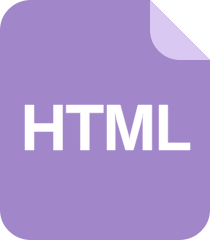
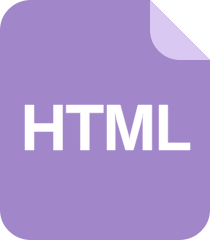
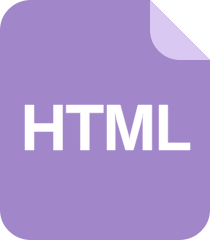
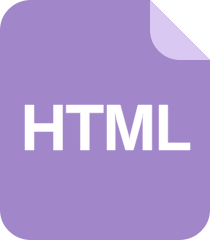
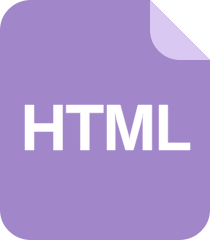
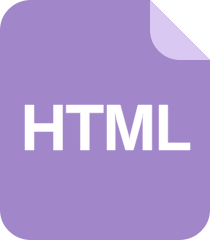
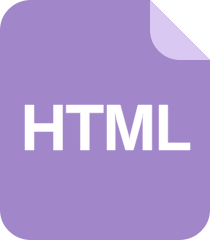
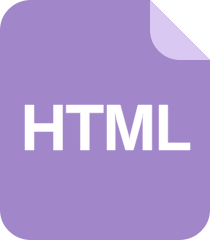
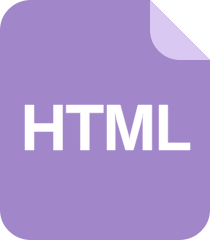
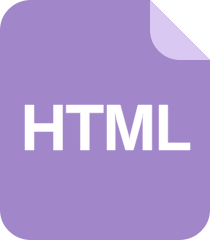
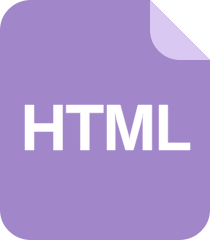
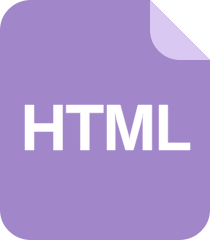
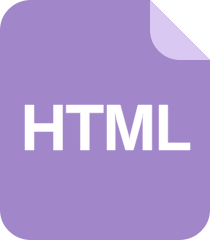
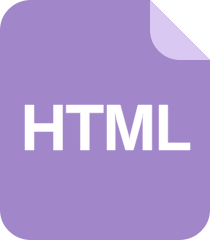
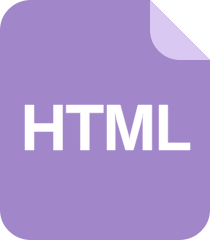
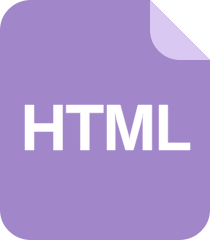
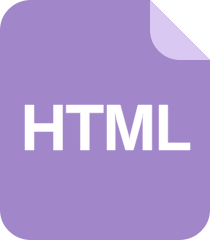
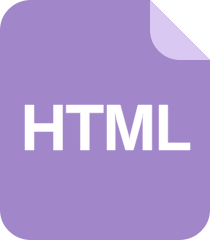
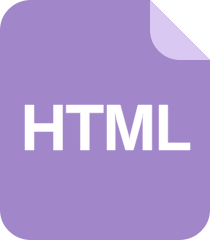
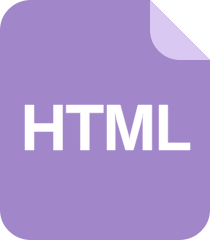
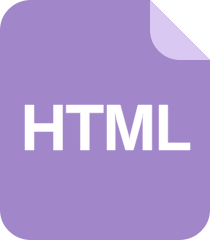
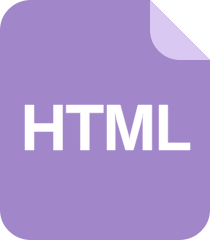
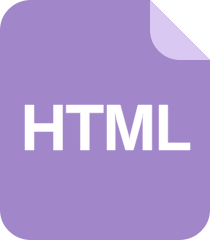
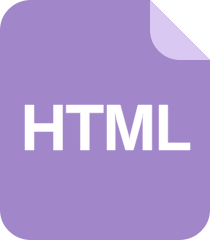
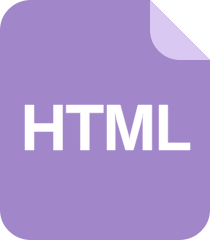
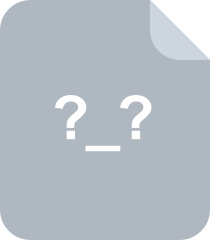
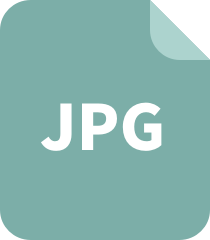
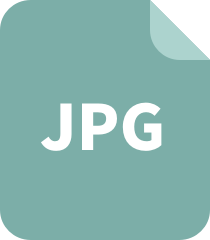
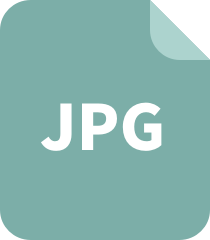
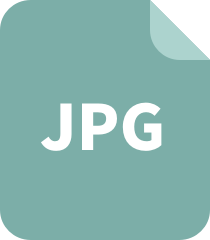
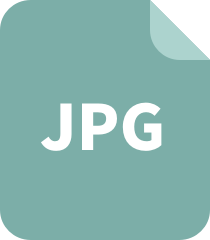
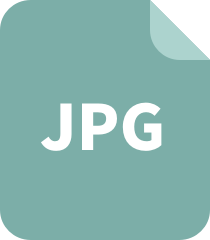
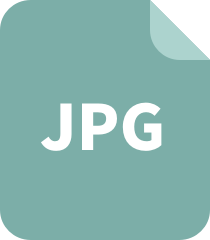
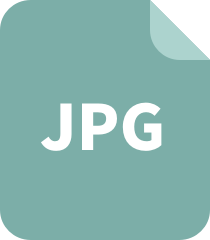
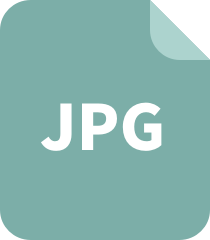
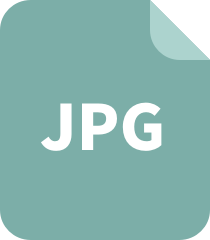
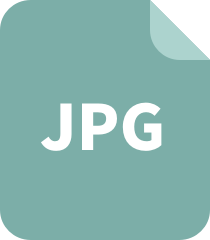
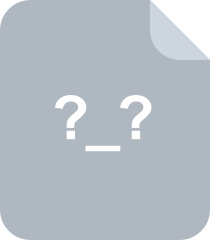
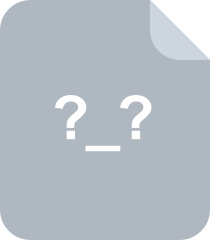
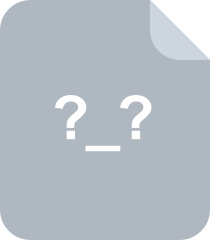
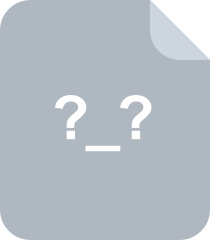
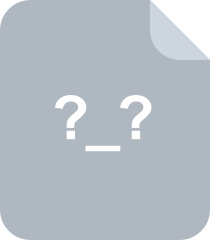
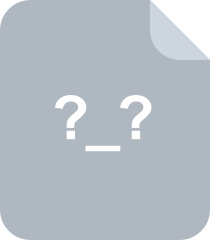
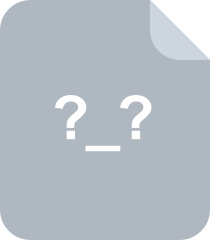
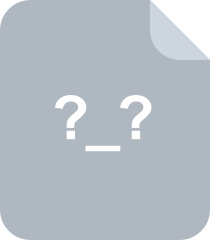
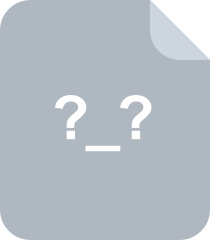
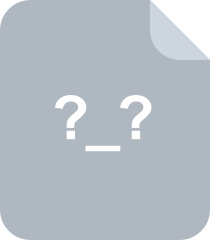
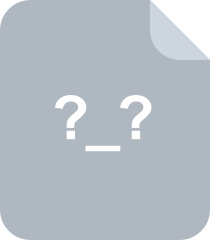
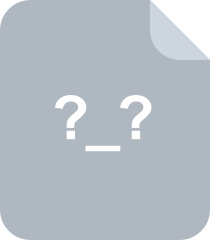
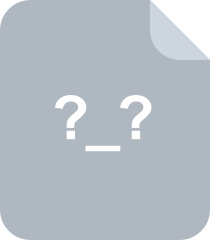
共 274 条
- 1
- 2
- 3
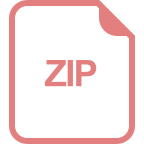
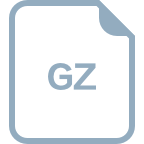
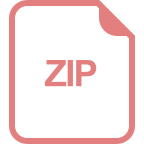
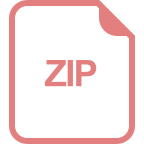
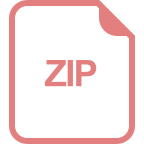
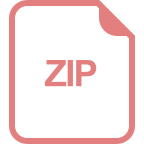
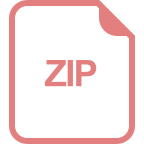
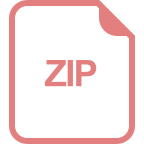
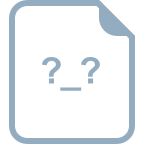
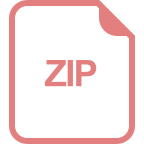
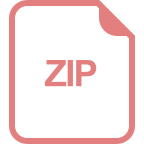
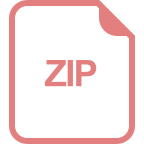
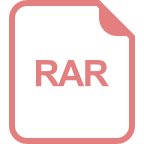
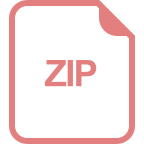
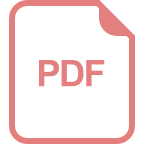
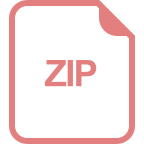
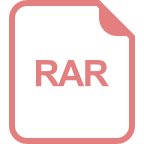
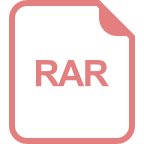
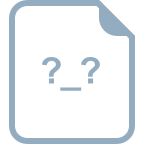
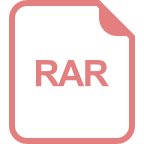
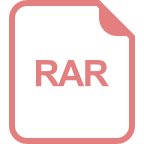
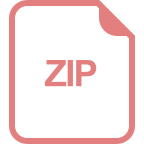
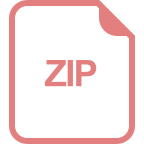
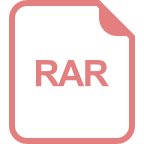
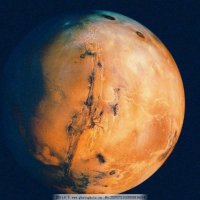
易烊千玺的小朋友
- 粉丝: 40
- 资源: 4516
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

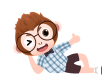
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


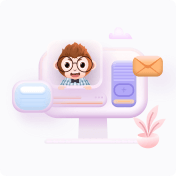
安全验证
文档复制为VIP权益,开通VIP直接复制

评论1