[](https://github.com/hank-cp/sbp/releases)




sbp introduce plugin oriented programming to Spring Boot. It is inspired and builds
on top of [Pf4j](https://pf4j.org/) project.
### Why we need plugin for Spring Boot?
* Spring boot is good, but it is monolithic. It means you have to delivery the whole application
for every single code change every time.
* We need a modern framework with flexibility and extensibility to rapidly deliver solution
for complex business scenario.
* Not all projects need to consider scaling at the beginning stage, like Spring Cloud does.
* With **sbp**, we could think in micro service architecture only with Spring Boot,
but no need to worried too much about "cloud native" things, like service discovery, traffic control, etc.
* It's a medium level preference between monolithic Spring Boot application and distributed
Spring Cloud application.
### Feature / Benefits
* Turn monolithic Spring Boot application to be **MODULAR**.
* Much more **LIGHTWEIGHT** development and deployment for spiral extensible architecture.
* Install/update/start/stop plugins **ON THE FLY**.
* **FULL STACK** Web/Rest server-side features powered by Spring Boot, including:
* controller for request handling
* persistence (Spring Data/JPA/Jooq/Plain SQL)
* security
* AOP
* resource loading
* Code and test plugin project as **STANDALONE** Spring Boot project.
* **NO** extra knowledge **NEED TO LEARN** as long as you are familiar with Spring Boot.
* **NO XML**
##### vs OSGi Based Server ([Eclipse Virgo](https://www.eclipse.org/virgo/) \ [Apache Karaf](http://karaf.apache.org/))
* OSGi based server need a server container to deploy, which is not _cloud friendly_.
* OSGi configuration is complex and strict, the learning curve is steep.
* Bundle cannot be run standalone, which is hard for debug and test.
* ~~Spring dm server has been dead, and its successor Virgo is now still struggling to live.~~
##### vs Spring Cloud
* Spring Cloud does not aim to solve similar problem as `sbp`, but so far it maybe the
best choice to solve application extension problem.
* With **sbp**, you will no need to consider too much about computer resource arrangement,
everything stick tight within one process.
* Again, **sbp** is good for medium level applications, which have problem to handle business
change rapidly. For large scale application, Spring Cloud is still your best choice.
* **sbp** should be able to live with Spring Cloud. After all, it is still a Spring Boot
application, just like any single service provider node in Spring Cloud network.
### Getting Start
##### Create app project
1. Create a Spring Boot project with multi sub project structure.
1. For Gradle, it means [multiple projects](https://docs.gradle.org/current/userguide/intro_multi_project_builds.html)
2. For Maven, it means [multiple modules](https://maven.apache.org/guides/mini/guide-multiple-modules.html)
3. Take the demo projects for reference.
2. Introduce `sbp-spring-boot-starter` to dependencies.
* Maven
```
<dependency>
<groupId>org.laxture</groupId>
<artifactId>sbp-spring-boot-starter</artifactId>
<version>0.1.11</version>
</dependency>
```
* Gradle
```
dependencies {
implementation 'org.laxture:sbp-spring-boot-starter:0.1.11'
}
```
* Latest master code is always available with version `-SNAPSHOT`
3. Add belows to `application.properties`.
```
spring.sbp.runtimeMode = development
spring.sbp.enabled = true
# remember to add this line in case you are using IDEA
spring.sbp.classes-directories = "out/production/classes, out/production/resources"
```
4. Add anything you want in this project like `Controller`, `Service`, `Repository`, `Model`, etc.
5. Create an empty folder named `plugins`.
##### Create plugin project
1. Create a plain Spring Boot project in the `plugins` folder.
2. Add `plugin.properties` file to the plugin project.
```properties
plugin.id=<>
plugin.class=demo.sbp.admin.DemoPlugin
plugin.version=0.0.1
plugin.provider=Your Name
plugin.dependencies=
```
3. Introduce `sbp-core` to dependencies.
* Maven
```
<dependency>
<groupId>org.laxture</groupId>
<artifactId>sbp-core</artifactId>
<version>0.1.11</version>
</dependency>
```
* Gradle
```
dependencies {
implementation 'org.laxture:sbp-core:0.1.11'
}
```
4. Add Plugin class
```java
public class DemoPlugin extends SpringBootPlugin {
public DemoPlugin(PluginWrapper wrapper) {
super(wrapper);
}
@Override
protected SpringBootstrap createSpringBootstrap() {
return new SpringBootstrap(this, AdminPluginStarter.class);
}
}
```
4. Add anything you want in the plugin project like `Controller`, `Service`, `Repository`, `Model`, etc.
Everything is done and now you could start the app project to test the plugin.
##### Checkout the demo projects for more details
* demo-shared: Shared code for app and plugin projects.
* demo-security: Security configuration demonstrate how to introduce Spring Security and secure your
API via AOP.
* demo-apis: Class shared between app\<-\>plugins and plugins\<-\>plugins need to be declared as API.
* demo-app: The entry point and master project. It provides two `SpringApplication`
* `DemoApp` does not have Spring Security configured.
* `DomeSecureApp` include Spring Security, so you need to provide authentication information
when access its rest API.
* plugins
* demo-plugin-admin
* Demonstrate Spring Security integration to plugin projects
* Demonstrate [Spring Boot profile](https://docs.spring.io/spring-boot/docs/current/reference/html/boot-features-profiles.html)
feature support.
* demo-plugin-author: Demonstrate share resource (like DataSource, TransactionManager) between
api/plugins.
* demo-plugin-library: Demonstrate using Spring Data/JPA.
* demo-plugin-shelf: Demonstrate api expose and invocation between plugins.
* Every single projects with `SpringApplication` could be run standalone.
* It is basically a skeleton project that you could starts your own project. It almost contains
everything we need in the real project.
### Documentation
* [How it works](docs/how_it_works.md)
* [Configuration](docs/configuration.md)
* [Serve Static Content](docs/resource_handling.md)
* [Persistence](docs/persistence.md)
* [Security / AOP](docs/security_aop.md)
* [Deployment](docs/deployment.md)
* [Trouble Shoot & Misc](docs/trouble_shoot.md)
* [About demo project](docs/demo_project.md)
* [Road map](docs/roadmap.md)
<!--
### Credit & Contribution
-->
### License
```
/*
* Copyright (C) 2019-present the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
```
没有合适的资源?快使用搜索试试~ 我知道了~
sbp:基于pf4j的Spring Boot插件框架
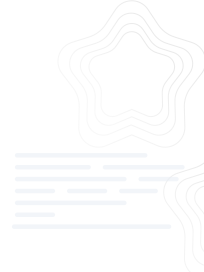
共162个文件
java:80个
gradle:13个
yml:12个

需积分: 39 17 下载量 31 浏览量
2021-02-05
20:35:53
上传
评论 2
收藏 594KB ZIP 举报
温馨提示
sbp向Spring Boot引入了面向插件的编程。 它的灵感来自于项目。 为什么我们需要Spring Boot插件? Spring Boot很好,但是是整体的。 这意味着您每次都必须为每个代码更改交付整个应用程序。 我们需要一个具有灵活性和可扩展性的现代框架,以快速为复杂的业务场景提供解决方案。 并非所有项目都需要像Spring Cloud一样在开始阶段就考虑扩展。 使用sbp ,我们可以仅在Spring Boot中考虑微服务架构,而无需过多担心“云原生”问题,例如服务发现,流量控制等。 在整体式Spring Boot应用程序和分布式Spring Cloud应用程序之间,它是中等优
资源详情
资源评论
资源推荐
收起资源包目录

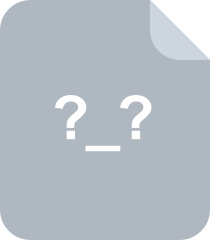
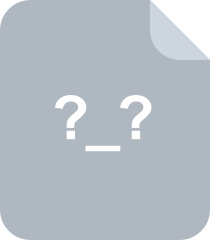
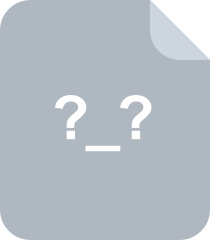
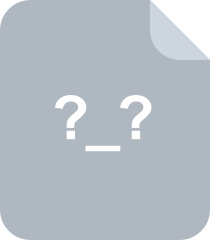
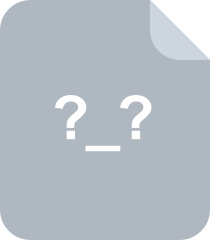
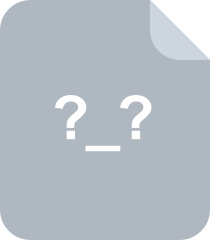
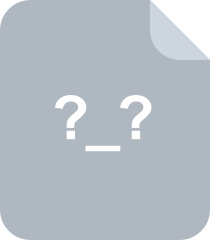
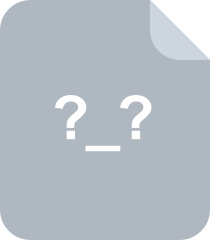
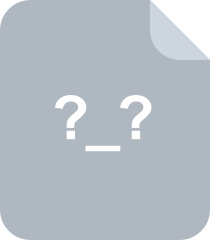
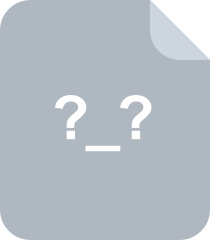
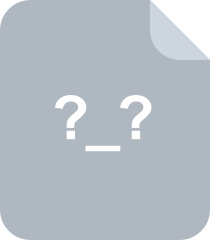
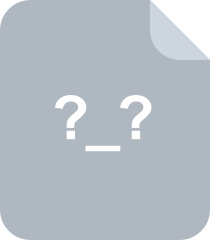
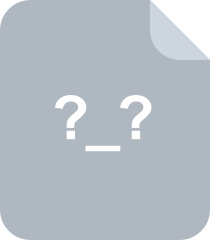
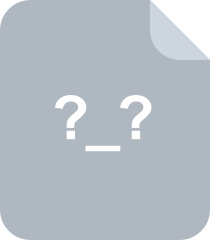
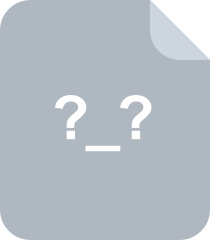
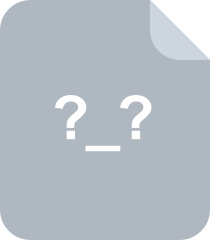
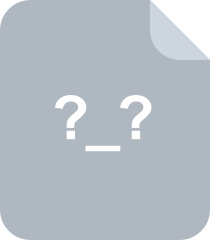
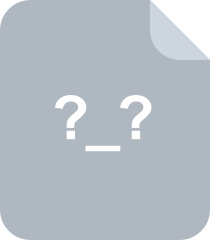
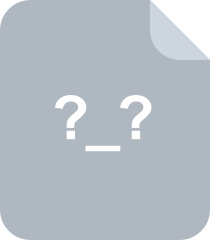
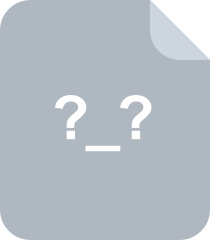
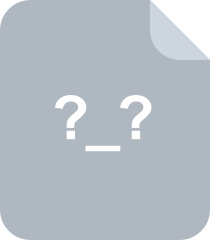
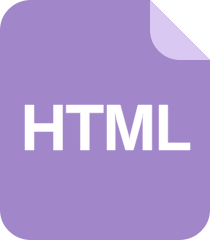
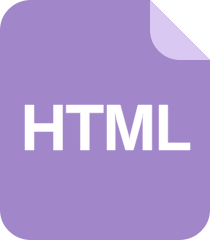
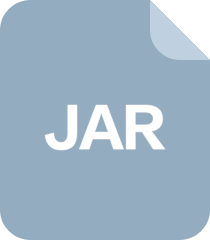
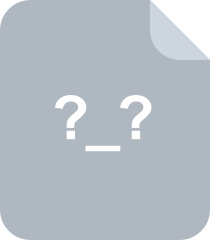
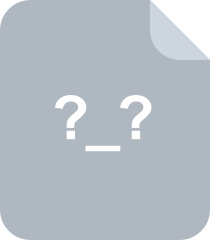
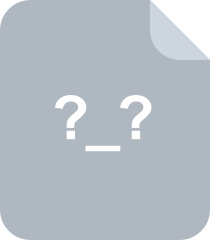
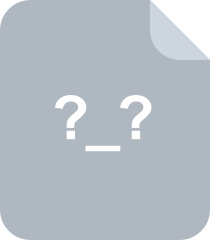
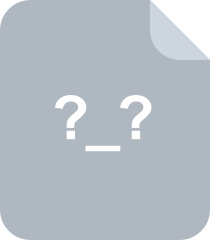
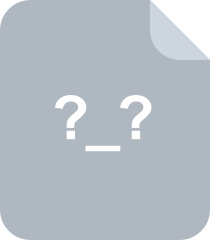
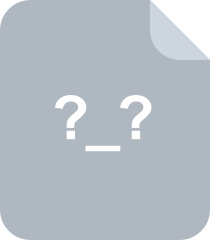
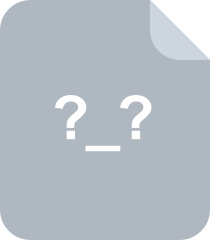
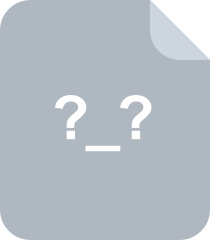
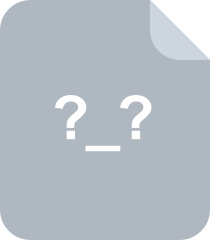
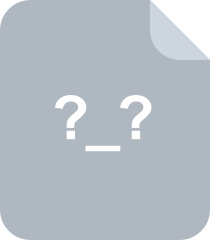
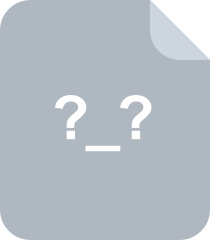
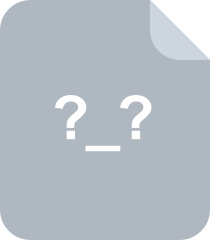
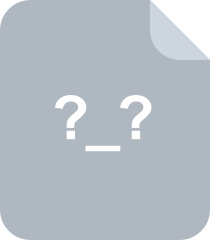
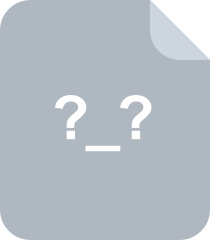
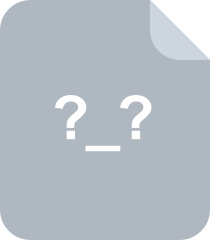
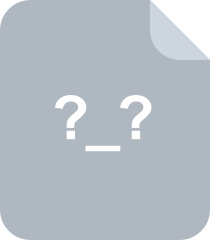
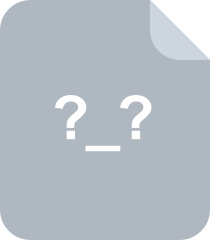
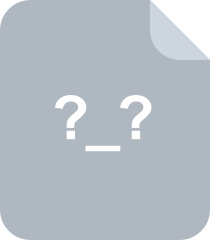
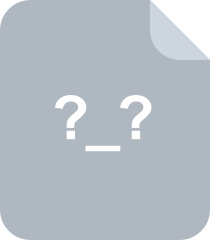
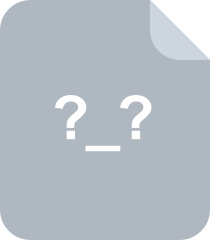
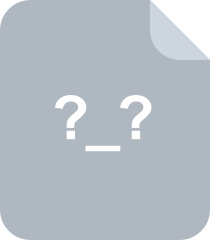
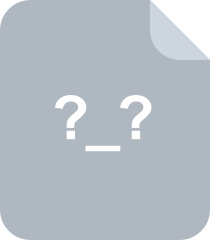
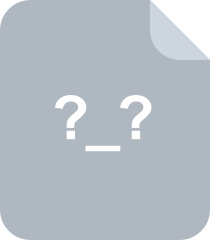
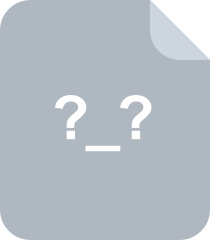
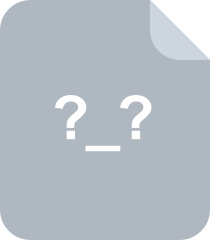
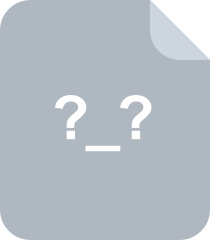
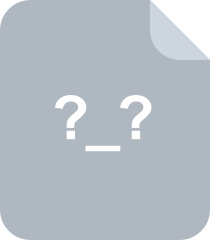
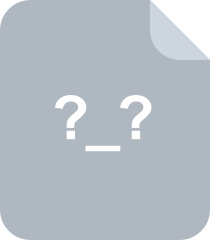
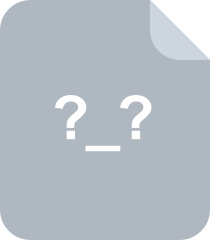
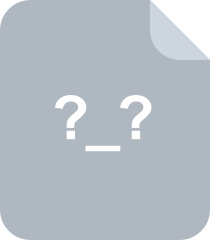
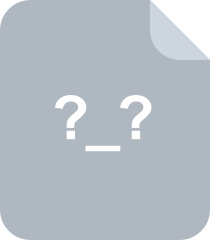
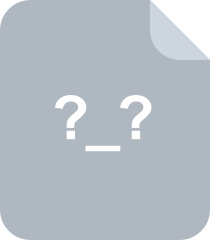
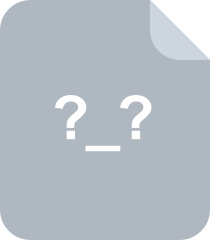
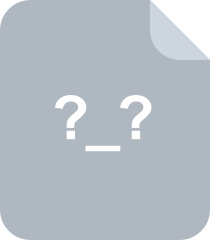
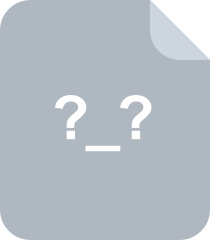
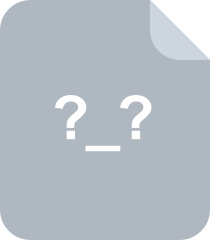
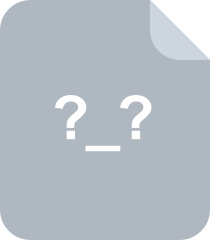
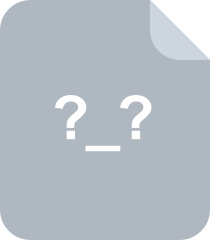
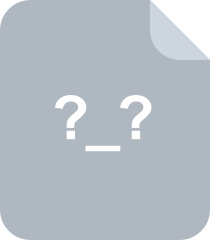
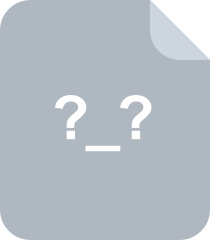
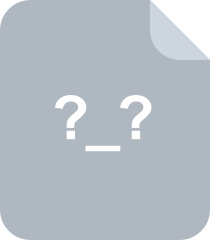
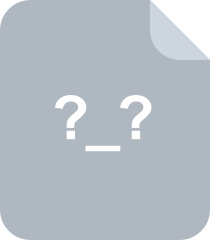
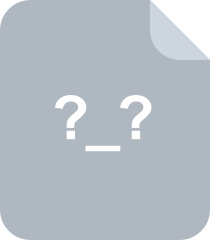
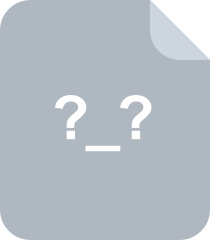
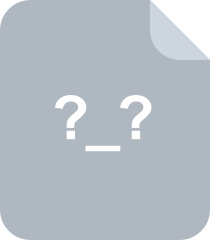
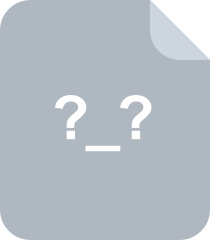
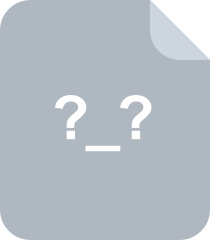
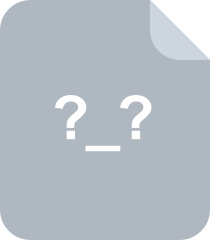
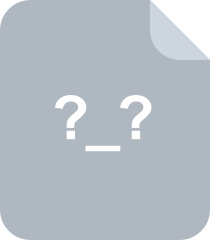
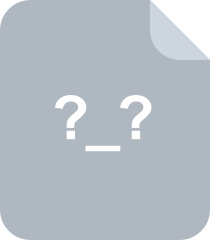
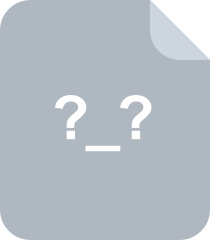
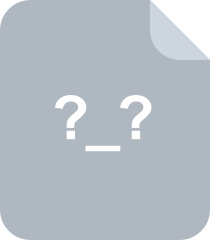
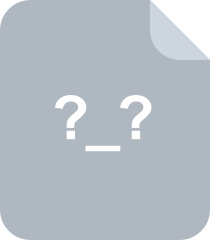
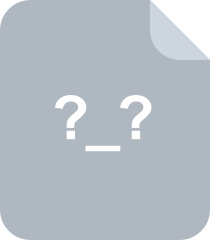
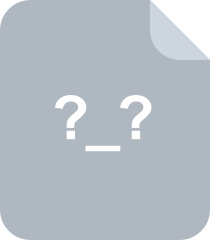
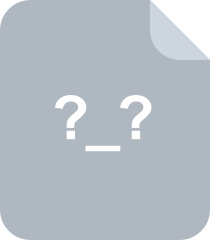
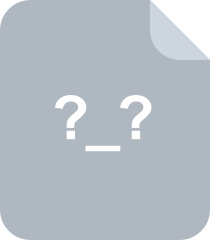
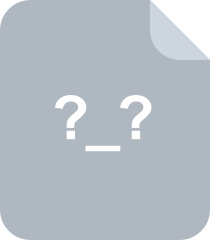
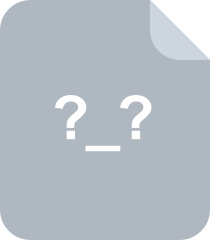
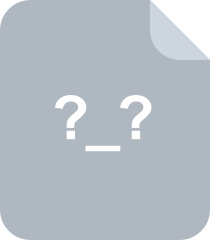
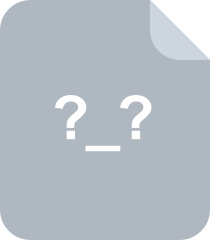
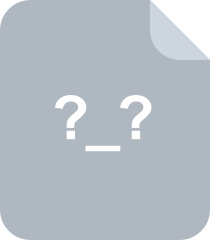
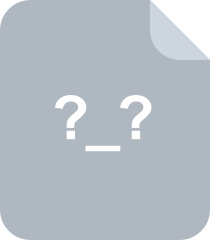
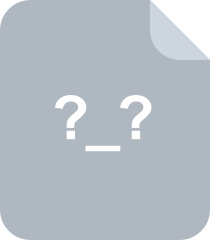
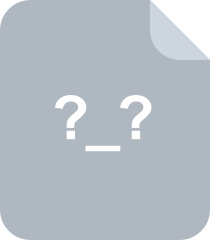
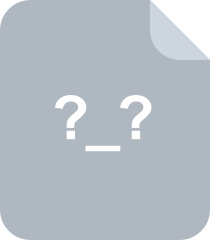
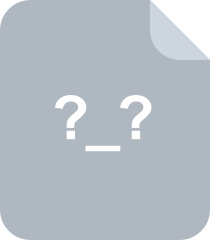
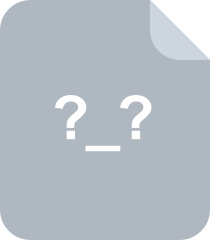
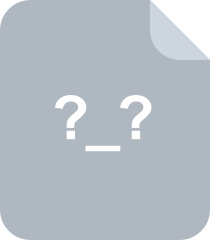
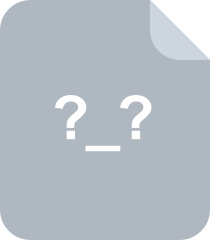
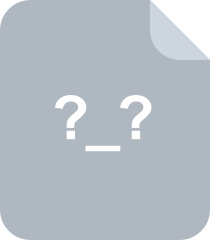
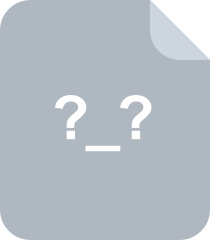
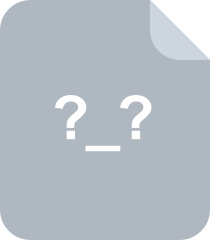
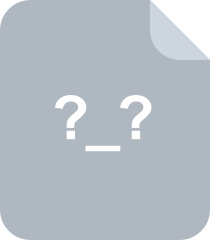
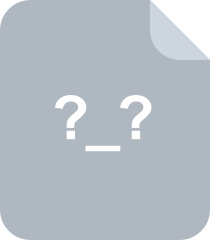
共 162 条
- 1
- 2
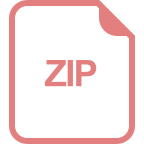
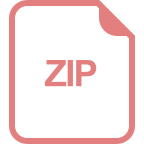
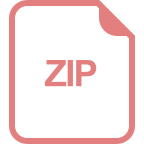
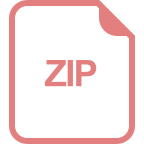
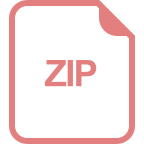
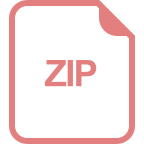
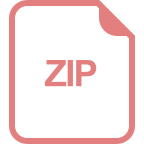
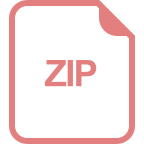
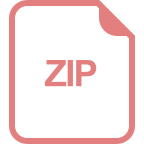
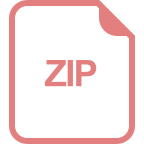
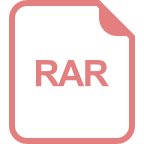
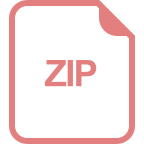
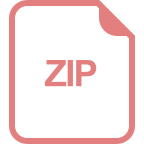
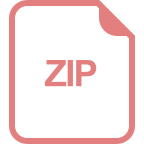
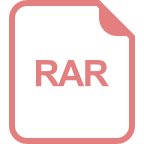
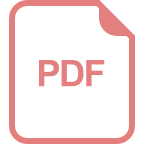
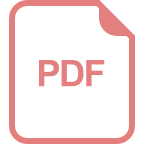
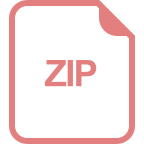
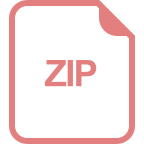
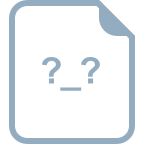
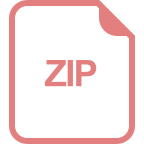
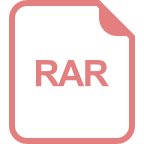
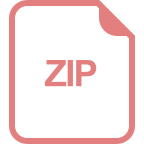

Jeckaijew
- 粉丝: 37
- 资源: 4532
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

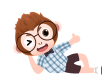
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


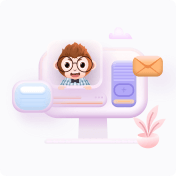
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0