# matlab_imresize
Python implementation of MatLab imresize() function.
## Table of contents
1. [Background](#background)
2. [System requirements](#req)
3. [Usage](#usage)
4. [Additional info](#addinfo)
5. [Note](#note)
## Background <a name="background"></a>
In the latest Super Resolution challenges (e.g. see [NTIRE 2017](http://www.vision.ee.ethz.ch/ntire17/)) the downscaling - *bicubic interpolation* - is performed via MatLab imresize() function.
[Track info](http://www.vision.ee.ethz.ch/ntire17/#challenge):
> Track 1: bicubic uses the **bicubic downscaling (Matlab imresize)**, one of the most common settings from the recent single-image super-resolution literature.
[More info](https://competitions.codalab.org/competitions/16303):
> For obtaining the low res images we use the **Matlab function "imresize" with default settings (bicubic interpolation)** and the desired downscaling factors: 2, 3, and 4.
Moreover, the quality (PSNR) of a tested solution is compared with the reference solution - upsampling with bicubic interpolation - which is done again with MatLab imresize() function with the default settings.
All this leads to:
1. Preparing train database (downscaling High-Resolution images) using MatLab
2. Reference solution (upscaling with bicubic interpolation) is also should be done using MatLab
As the most of the Deep Learning code is written under the python, we need to do some additional preprocessing/postprocessing using completely different environment (MatLab), and can't do upscaling/downscaling in-place using simple python functions. As a result, the implemented python imresize() function is done to overcome these difficulties.
## System requirements <a name="req"></a>
* python 2.7
* numpy
## Usage <a name="usage"></a>
imresize of *uint8* image using scale (e.g. 0.5 or 2):
```python
Img_out = imresize(Img_in, scalar_scale=0.333) # Img_out of type uint8
```
imresize of *uint8* image using shape (e.g. (100, 200)):
```python
Img_out = imresize(Img_in, output_shape=(123, 324)) # Img_out of type uint8
```
Above examples are working when input image `Img_in` is of the type **uint8**. But often the image processing is done in **float64**, and converted to uint8 only before saving on disk. The following code is for obtaining the same result as doing *imresize+imwrite* in MatLab for input image of doubles:
```python
import numpy as np
from skimage.io import imsave, imread
from skimage import img_as_float
img_uint8 = imread('test.png')
img_double = img_as_float(img_uint8)
new_img_double = imresize(img_double, output_shape=(123, 324))
imsave('test_double.png', convertDouble2Byte(new_img_double))
```
## Additional information <a name="addinfo"></a>
Actually, the implemented python code was made by re-writing MatLab code `toolbox/images/images/imresize.m`, and it can't be done without brilliant insight made by [S. Sheen](https://stackoverflow.com/users/6073407/s-sheen) about how `imresizemex` can be implemented (originally, it is binary provided with MatLab distribution): [stackoverflow](https://stackoverflow.com/questions/36047357/what-does-imresizemex-do-in-matlab-imresize-function).
In fact, if you have **OpenCV** and have the ability to re-compile it, probably the best solution is to change parameter `A` inside function `interpolateCubic`, which is located (at least for release 3.2.0) on line `3129` inside file `opencv-3.2.0/modules/imgproc/src/imgwarp.cpp`, from *-0.75f* to *-0.5f* (the value used in MatLab). Then simply use function `cv::resize` from OpenCV. For more information please refer to [stackoverflow](https://stackoverflow.com/questions/26823140/imresize-trying-to-understand-the-bicubic-interpolation). Also, see [another stackoverflow answer](https://stackoverflow.com/questions/29958670/how-to-use-matlabs-imresize-in-python) about different ways of resizing the image inside python.
## Note <a name="note"></a>
Please note that no optimization (aside from preliminary numpy-based vectorizing) was made, so the code can be (and it is) times slower than the original MatLab code.
没有合适的资源?快使用搜索试试~ 我知道了~
matlab_imresize:MatLab imresize函数的Python实现
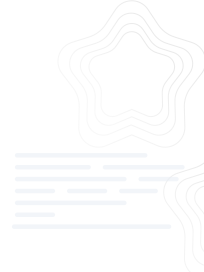
共9个文件
py:3个
png:3个
md:2个

需积分: 14 15 下载量 133 浏览量
2021-05-07
19:55:53
上传
评论
收藏 572KB ZIP 举报
温馨提示
matlab_imresize MatLab imresize()函数的Python实现。 目录 背景 在最新的“超分辨率”挑战中(例如,请参见 ),通过MatLab imresize()函数执行了降尺度(三次三次插值)。 : 轨迹1:bicubic使用bicubic缩小比例(Matlab imresize) ,这是最近的单图像超分辨率文献中最常见的设置之一。 : 为了获得低分辨率图像,我们使用Matlab函数“ imresize”(默认设置)(双三次插值)以及所需的缩小比例:2、3和4。 此外,将经过测试的解决方案的质量(PSNR)与参考解决方案进行了比较-使用三次三次插值进行上采样-使用默认设置的MatLab imresize()函数再次进行此操作。 所有这些导致: 使用MatLab准备火车数据库(缩小高分辨率图像) 参考解决方案(使用三次三次插值法进行放大)也应使用
资源推荐
资源详情
资源评论
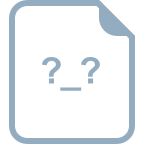
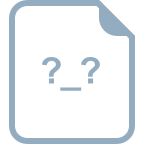
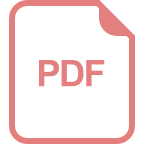
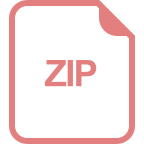
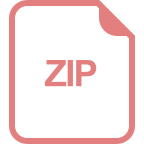
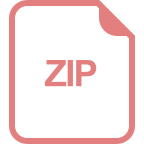
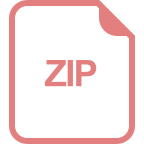
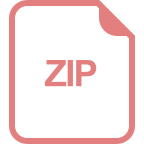
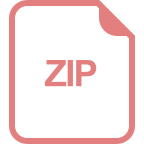
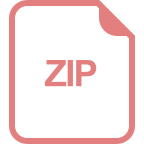
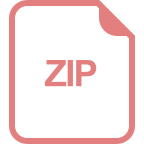
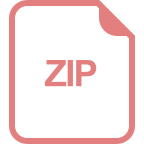
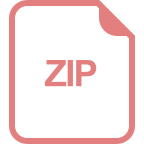
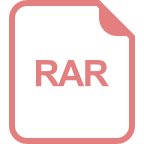
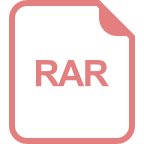
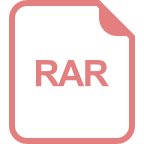
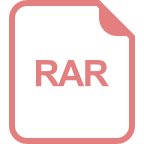
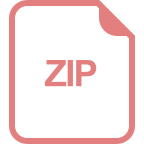
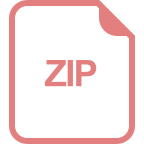
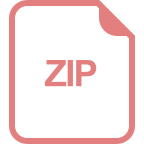
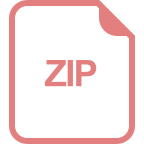
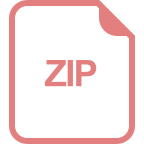
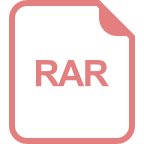
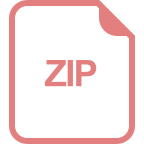
收起资源包目录


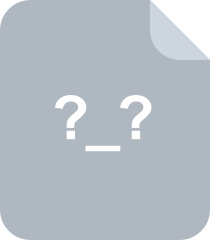
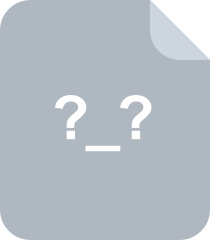
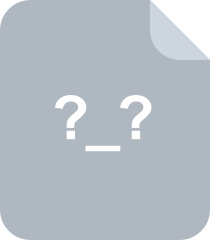
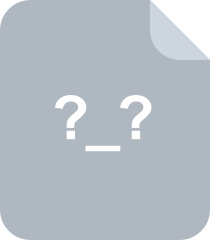

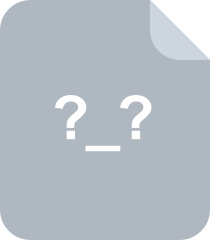
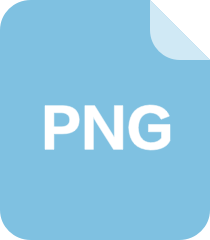
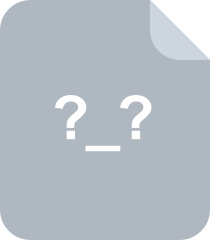
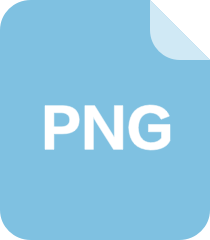
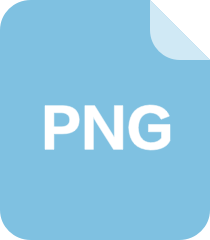
共 9 条
- 1
资源评论
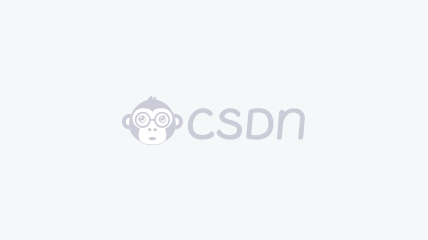

缪之初
- 粉丝: 32
- 资源: 4720
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

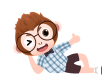
最新资源
- 常用正则表达式.docx
- 【java毕业设计】点餐系统网站源码(ssm+mysql+说明文档).zip
- 网络安全中的系统信息收集与防护机制探讨
- Vue搭建AudioPlaySation(三)
- 【java毕业设计】班级同学录管理系统源码(ssm+mysql+说明文档).zip
- (2024年最新更新!!!)经管类期刊-投稿指南
- 2001-2022三个版本企业数字化转型合集【重磅,更新!】
- 网络安全领域中关于资产泄漏、CMS识别与代码版本管理工具安全性的技术探讨
- 【java毕业设计】东风锻造有限公司点检管理系统源码(ssm+mysql+说明文档).zip
- Web架构与信息打点技术综合解析及其应用场景
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


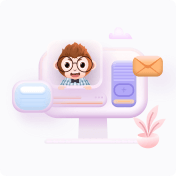
安全验证
文档复制为VIP权益,开通VIP直接复制
