# fastify-cors

[](https://www.npmjs.com/package/fastify-cors)
[](https://snyk.io/test/github/fastify/fastify-cors)
[](https://coveralls.io/github/fastify/fastify-cors?branch=master)
[](https://standardjs.com/)
`fastify-cors` enables the use of [CORS](https://en.wikipedia.org/wiki/Cross-origin_resource_sharing) in a Fastify application.
Supports Fastify versions `3.x`.
Please refer to [this branch](https://github.com/fastify/fastify-cors/tree/3.x) and related versions for Fastify `^2.x` compatibility.
Please refer to [this branch](https://github.com/fastify/fastify-cors/tree/1.x) and related versions for Fastify `^1.x` compatibility.
## Install
```
npm i fastify-cors
```
## Usage
Require `fastify-cors` and register it as any other plugin, it will add a `preHandler` hook and a [wildcard options route](https://github.com/fastify/fastify/issues/326#issuecomment-411360862).
```js
const fastify = require('fastify')()
fastify.register(require('fastify-cors'), {
// put your options here
})
fastify.get('/', (req, reply) => {
reply.send({ hello: 'world' })
})
fastify.listen(3000)
```
You can use it as is without passing any option or you can configure it as explained below.
### Options
* `origin`: Configures the **Access-Control-Allow-Origin** CORS header. The value of origin could be of different types:
- `Boolean` - set `origin` to `true` to reflect the [request origin](http://tools.ietf.org/html/draft-abarth-origin-09), or set it to `false` to disable CORS.
- `String` - set `origin` to a specific origin. For example if you set it to `"http://example.com"` only requests from "http://example.com" will be allowed. The special `*` value (default) allows any origin.
- `RegExp` - set `origin` to a regular expression pattern that will be used to test the request origin. If it is a match, the request origin will be reflected. For example, the pattern `/example\.com$/` will reflect any request that is coming from an origin ending with "example.com".
- `Array` - set `origin` to an array of valid origins. Each origin can be a `String` or a `RegExp`. For example `["http://example1.com", /\.example2\.com$/]` will accept any request from "http://example1.com" or from a subdomain of "example2.com".
- `Function` - set `origin` to a function implementing some custom logic. The function takes the request origin as the first parameter and a callback as a second (which expects the signature `err [Error | null], origin`), where `origin` is a non-function value of the origin option. *Async-await* and promises are supported as well. The Fastify instance is bound to function call and you may access via `this`. For example:
```js
origin: (origin, cb) => {
if(/localhost/.test(origin)){
// Request from localhost will pass
cb(null, true)
return
}
// Generate an error on other origins, disabling access
cb(new Error("Not allowed"))
}
```
* `methods`: Configures the **Access-Control-Allow-Methods** CORS header. Expects a comma-delimited string (ex: 'GET,PUT,POST') or an array (ex: `['GET', 'PUT', 'POST']`).
* `allowedHeaders`: Configures the **Access-Control-Allow-Headers** CORS header. Expects a comma-delimited string (ex: `'Content-Type,Authorization'`) or an array (ex: `['Content-Type', 'Authorization']`). If not specified, defaults to reflecting the headers specified in the request's **Access-Control-Request-Headers** header.
* `exposedHeaders`: Configures the **Access-Control-Expose-Headers** CORS header. Expects a comma-delimited string (ex: `'Content-Range,X-Content-Range'`) or an array (ex: `['Content-Range', 'X-Content-Range']`). If not specified, no custom headers are exposed.
* `credentials`: Configures the **Access-Control-Allow-Credentials** CORS header. Set to `true` to pass the header, otherwise it is omitted.
* `maxAge`: Configures the **Access-Control-Max-Age** CORS header. In seconds. Set to an integer to pass the header, otherwise it is omitted.
* `preflightContinue`: Pass the CORS preflight response to the route handler (default: `false`).
* `optionsSuccessStatus`: Provides a status code to use for successful `OPTIONS` requests, since some legacy browsers (IE11, various SmartTVs) choke on `204`.
* `preflight`: if needed you can entirely disable preflight by passing `false` here (default: `true`).
* `strictPreflight`: Enforces strict requirement of the CORS preflight request headers (**Access-Control-Request-Method** and **Origin**) as defined by the [W3C CORS specification](https://www.w3.org/TR/2020/SPSD-cors-20200602/#resource-preflight-requests) (the current [fetch living specification](https://fetch.spec.whatwg.org/) does not define server behavior for missing headers). Preflight requests without the required headers will result in 400 errors when set to `true` (default: `true`).
* `hideOptionsRoute`: hide options route from the documentation built using [fastify-swagger](https://github.com/fastify/fastify-swagger) (default: `true`).
### Configuring CORS Asynchronously
```js
const fastify = require('fastify')()
fastify.register(require('fastify-cors'), (instance) => (req, callback) => {
let corsOptions;
// do not include CORS headers for requests from localhost
if (/localhost/.test(origin)) {
corsOptions = { origin: false }
} else {
corsOptions = { origin: true }
}
callback(null, corsOptions) // callback expects two parameters: error and options
})
fastify.get('/', (req, reply) => {
reply.send({ hello: 'world' })
})
fastify.listen(3000)
```
## Acknowledgements
The code is a port for Fastify of [`expressjs/cors`](https://github.com/expressjs/cors).
## License
Licensed under [MIT](./LICENSE).<br/>
[`expressjs/cors` license](https://github.com/expressjs/cors/blob/master/LICENSE)
没有合适的资源?快使用搜索试试~ 我知道了~
fastify-cors:加速 CORS
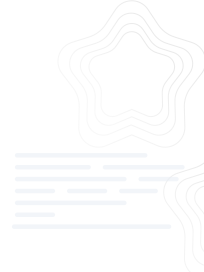
共18个文件
js:6个
yml:3个
ts:2个

需积分: 11 2 下载量 162 浏览量
2021-07-23
19:23:56
上传
评论
收藏 16KB ZIP 举报
温馨提示
fastify-cors fastify-cors允许在 Fastify 应用程序中使用 。 支持 Fastify 3.x版本。 Fastify ^2.x兼容性请参考及相关版本。 Fastify ^1.x兼容性请参考及相关版本。 安装 npm i fastify-cors 用法 需要fastify-cors并将其注册为任何其他插件,它将添加一个preHandler钩子和一个 。 const fastify = require ( 'fastify' ) ( ) fastify . register ( require ( 'fastify-cors' ) , { // put your options here } ) fastify . get ( '/' , ( req , reply ) => { reply . send ( { hello : 'world'
资源推荐
资源详情
资源评论
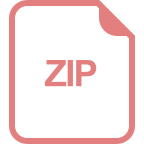
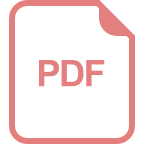
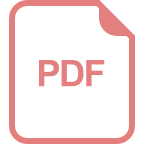
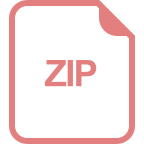
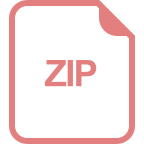
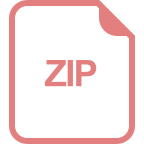
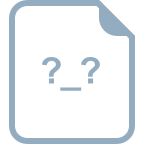
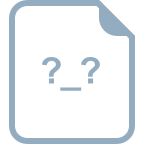
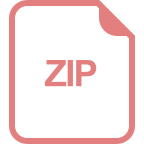
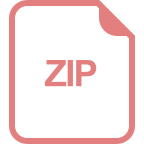
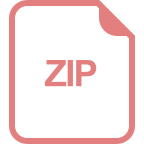
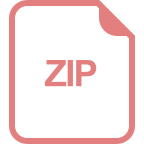
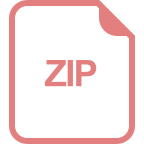
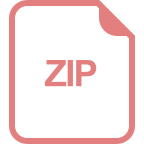
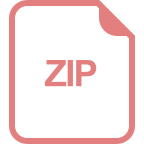
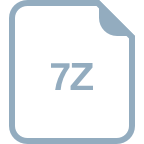
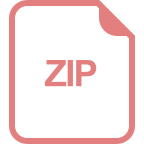
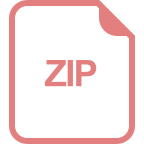
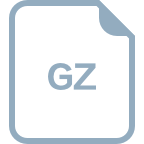
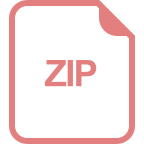
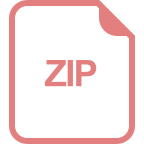
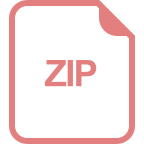
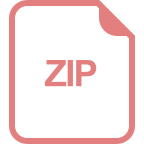
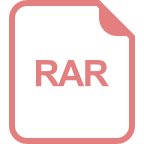
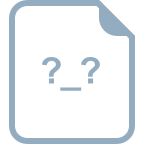
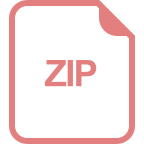
收起资源包目录


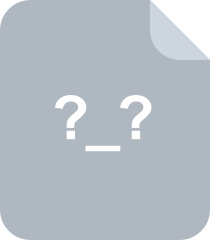
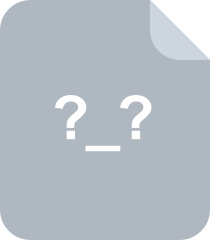

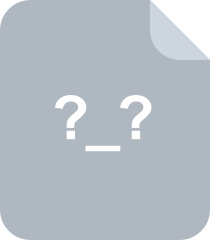
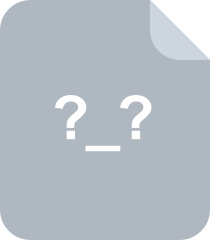

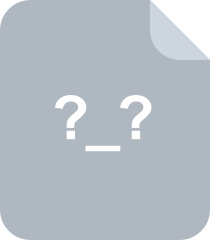
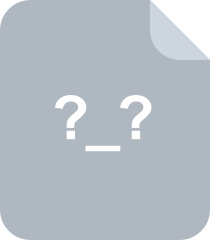
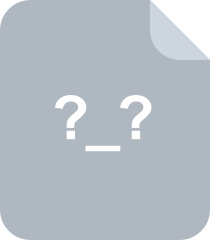
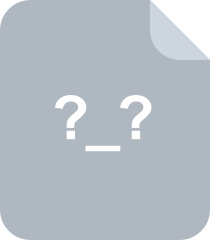

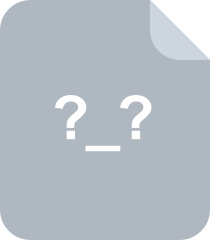
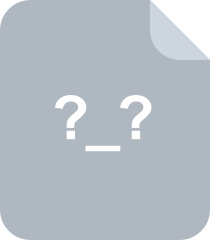
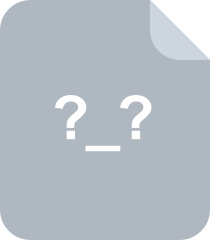
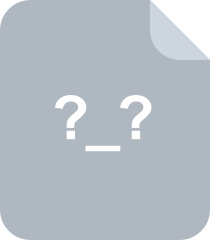
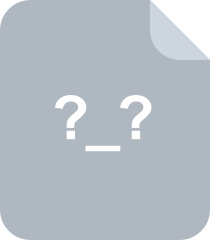
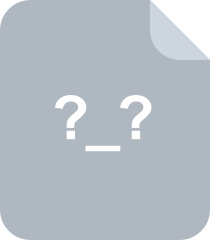
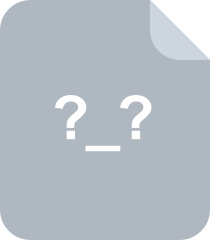
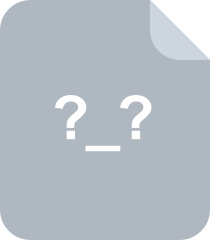
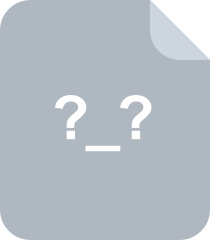
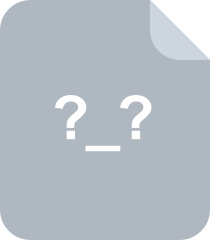
共 18 条
- 1
资源评论
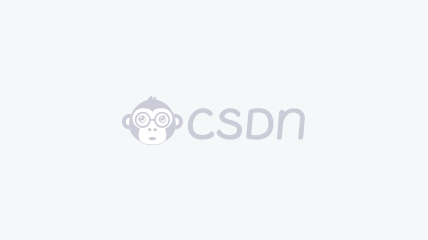

好摩
- 粉丝: 31
- 资源: 4634
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

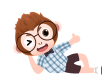
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


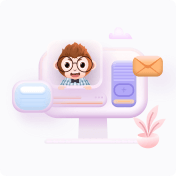
安全验证
文档复制为VIP权益,开通VIP直接复制
