//----------------------------------------------------------------------------------
// FILE: SolarMicroInv_F2803x-Main.C
//
// Description: Solar MicroInverter Project
//
// Version: 1.0
//
// Target: TMS320F2803x(PiccoloB),
//
//----------------------------------------------------------------------------------
// Copyright Texas Instruments � 2004-2013
//----------------------------------------------------------------------------------
// Revision History:
//----------------------------------------------------------------------------------
// Date | Description / Status
//----------------------------------------------------------------------------------
// June 26 2013 : (Manish Bhardwaj, Shamim Choudhury)
//----------------------------------------------------------------------------------
//
// PLEASE READ - Useful notes about this Project
// Although this project is made up of several files, the most important ones are:
// "{ProjectName}-Main.C" - this file
// - Application Initialization, Peripheral config,
// - Application management
// - Slower background code loops and Task scheduling
// "{ProjectName}-DevInit_F28xxx.C
// - Device Initialization, e.g. Clock, PLL, WD, GPIO mapping
// - Peripheral clock enables
// - DevInit file will differ per each F28xxx device series, e.g. F280x, F2833x,
// "{ProjectName}-Settings.h"
// - Global defines (settings) project selections are found here
// - This file is referenced by both C and ASM files.
// "{ProjectName}-Includes.h"
// - Include file used by project are defined in this file
//
// Code is made up of sections, e.g. "FUNCTION PROTOTYPES", "VARIABLE DECLARATIONS" ,..etc
// each section has FRAMEWORK and USER areas.
// FRAMEWORK areas provide useful ready made "infrastructure" code which for the most part
// does not need modification, e.g. Task scheduling, ISR call, GUI interface support,...etc
// USER areas have functional example code which can be modified by USER to fit their appl.
//
// Code can be compiled with various build options (Incremental Builds IBx), these
// options are selected in file "{ProjectName}-Settings.h". Note: "Rebuild All" compile
// tool bar button must be used if this file is modified.
//----------------------------------------------------------------------------------
#include "SolarMicroInv-Includes.h"
//%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
// FUNCTION PROTOTYPES
//%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
// Add prototypes of functions being used in the project here
void DeviceInit(void);
#ifdef FLASH
void InitFlash();
#endif
void MemCopy();
#ifdef FLASH
#pragma CODE_SECTION(Inv_ISR,"ramfuncs");
#pragma CODE_SECTION(OneKhzISR,"ramfuncs");
#endif
//This is the control loop ISR run at 50Khz
interrupt void Inv_ISR(void);
//This is 1 Khz ISR slaved off CPU timer 2,
interrupt void OneKhzISR();
void InitECapture();
void ADC_SOC_CNF(int ChSel[], int Trigsel[], int ACQPS[], int IntChSel, int mode);
void PWM_1ch_UpDwnCnt_CNF(int16 n, Uint16 period, int16 mode, int16 phase);
void PWM_MicroInvLineClamp_CNF(int n,int period,int deadband_rising,int deadband_falling);
// -------------------------------- FRAMEWORK --------------------------------------
// State Machine function prototypes
//----------------------------------------------------------------------------------
// Alpha states
void A0(void); //state A0
void B0(void); //state B0
// A branch states
void A1(void); //state A1
void A2(void); //state A2
void A3(void); //state A3
void A4(void); //state A4
// B branch states
void B1(void); //state B1
void B2(void); //state B2
void B3(void); //state B3
// Variable declarations
void (*Alpha_State_Ptr)(void); // Base States pointer
void (*A_Task_Ptr)(void); // State pointer A branch
void (*B_Task_Ptr)(void); // State pointer B branch
//----------------------------------------------------------------------------------
//%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
// VARIABLE DECLARATIONS - GENERAL
//%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
// -------------------------------- FRAMEWORK --------------------------------------
int16 VTimer0[4]; // Virtual Timers slaved off CPU Timer 0
int16 VTimer1[4]; // Virtual Timers slaved off CPU Timer 1
// Used for running BackGround in flash, and ISR in RAM
extern Uint32 *RamfuncsLoadStart, *RamfuncsLoadEnd, *RamfuncsRunStart;
extern Uint32 *IQfuncsLoadStart, *IQfuncsLoadEnd, *IQfuncsRunStart;
// Used for ADC Configuration
int ChSel[16] = { 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 };
int TrigSel[16] = { 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 };
int ACQPS[16] = { 8, 8, 8, 8, 8, 8, 8, 8, 8, 8, 8, 8, 8, 8, 8, 8 };
//---------------------------------------------------------------------------
// Used to indirectly access all EPWM modules
volatile struct EPWM_REGS *ePWM[] = {
&EPwm1Regs, //intentional: (ePWM[0] not used)
&EPwm1Regs, &EPwm2Regs, &EPwm3Regs, &EPwm4Regs, &EPwm5Regs, &EPwm6Regs,
&EPwm7Regs, };
// Used to indirectly access all Comparator modules
volatile struct COMP_REGS *Comp[] = { &Comp1Regs,//intentional: (Comp[0] not used)
&Comp1Regs, &Comp2Regs, &Comp3Regs };
// ---------------------------------- USER -----------------------------------------
//-----------------------------Inverter & DC-DC Control Loop Variables -------------
// String to describe the state of the PV Inverter
char cPV_State[40];
// Solar Library Objects
// RAMP to generate forced angle when grid is not present
RAMPGEN_IQ rgen1;
// CNTL 3p3z for inverter current control
#pragma DATA_SECTION(cntl3p3z_InvI_vars,"cntl_var_RAM")
#pragma DATA_SECTION(cntl3p3z_InvI_coeff,"cntl_coeff_RAM")
CNTL_3P3Z_IQ_VARS cntl3p3z_InvI_vars;
CNTL_3P3Z_IQ_COEFFS cntl3p3z_InvI_coeff;
// CNTL 2p2z for flyback current control
#pragma DATA_SECTION(cntl2p2z_DCDCI_vars,"cntl_var_RAM")
#pragma DATA_SECTION(cntl2p2z_DCDCI_coeff,"cntl_coeff_RAM")
CNTL_2P2Z_IQ_VARS cntl2p2z_DCDCI_vars;
CNTL_2P2Z_IQ_COEFFS cntl2p2z_DCDCI_coeff;
// CNTL PI for bus control
CNTL_PI_IQ cntlPI_BusInv;
int16 UpdateCoef;
long Pgain_BusInv, Dgain_BusInv, Igain_BusInv, Dmax_BusInv;
// SPLL Objects of two types for grid angle lock
SPLL_1ph_IQ spll1;
SPLL_1ph_SOGI_IQ spll2;
float c2, c1;
long Phase_Jump, Phase_Jump_Trig;
// Sine analyzer block for RMS Volt, Curr and Power measurements
SINEANALYZER_DIFF_wPWR_IQ sine_mains;
// notch filter objects for filtering bus voltage, pv current and voltage sense signals
#pragma DATA_SECTION(Bus_Volt_notch,"cntl_var_RAM")
#pragma DATA_SECTION(PV_volt_notch,"cntl_var_RAM")
#pragma DATA_SECTION(PV_cur_notch,"cntl_var_RAM")
#pragma DATA_SECTION( notch_TwiceGridFreq,"cntl_coeff_RAM")
NOTCH_COEFF_IQ notch_TwiceGridFreq;
NOTCH_VARS_IQ Bus_Volt_notch,PV_volt_notch,PV_cur_notch;
// MPPT object for flyback current reference selection
MPPT_INCC_I_IQ mppt_incc_I1;
int16 MPPT_slew;
int16 Run_MPPT;
int16 MPPT_ENABLE;
// Datalogger to plot variables
#if INCR_BUILD==1 || INCR_BUILD==2
DLOG_4CH_IQ dlog1;
int16 DBUFF1[100], DBUFF2[100], DBUFF3[100], DBUFF4[100];
int16 D_val1, D_val2, D_val3, D_val4;
#endif
//TODO
// Inverter and Flyback Variables
_iq24 inv_meas_cur_inst, inv_meas_vol_inst, vbus_meas_inst, vbus_meas_avg ;
// reference variable Inverter
_iq24 InvModIndex, inv_Iset, inv_ref_cur_inst, inv_bus_ref;
// Measure Values DC-DC Stage
long pv_meas_cur_inst,pv_meas_vol_inst,pv_meas_cur_avg, pv_meas_vol_avg;
// Reference Values DC-DC Stage
long pv_ref_cur_inst, pv_ref_cur_mppt, pv_ref_cur_fixed;
// Measurement Offsets
_iq24 offset_165, offset_GridCurrent, PV_SenseVolt_Offset, PV_SenseCurr_Offset,DC_Bus_Offset;
//Offset filter coefficient K1: 0.05/(T+0.05)
没有合适的资源?快使用搜索试试~ 我知道了~
TI微型光伏逆变器源代码功率转换级包括
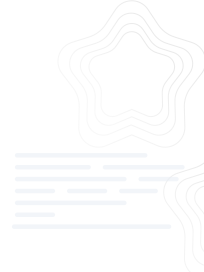
共6个文件
c:4个
h:2个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 82 浏览量
2023-10-19
11:28:18
上传
评论
收藏 26KB ZIP 举报
温馨提示
TI微型光伏逆变器源代码功率转换级包括 1) 具有次级倍压器的有源钳位反激式直流直流转换器,以及 2) 并网直流交流逆变器。此设计可实现 93% 的峰值效率和低于 4% 的总谐波失真 (THD),从而可提高单位太阳能电池板的功率输出,减少不利散热并延长系统寿命.zip
资源推荐
资源详情
资源评论
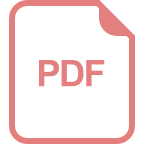
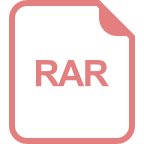
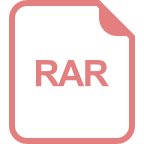
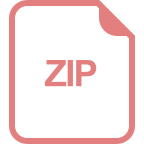
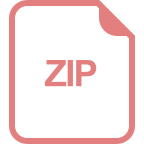
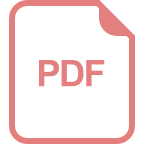
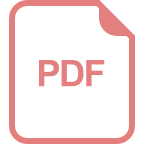
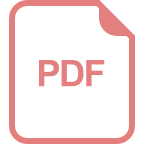
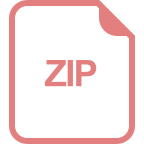
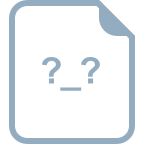
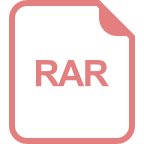
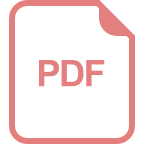
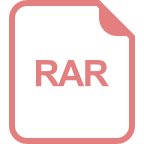
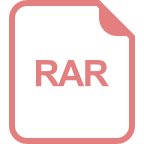
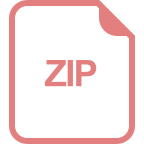
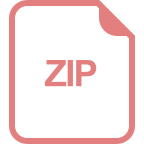
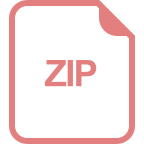
收起资源包目录


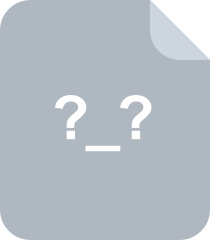
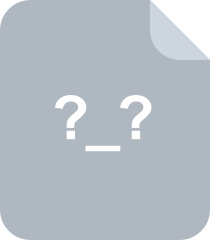
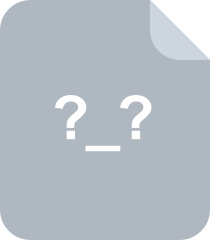
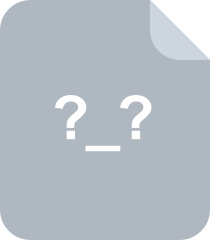
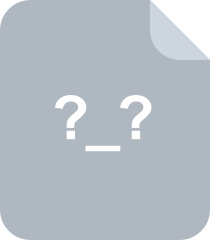
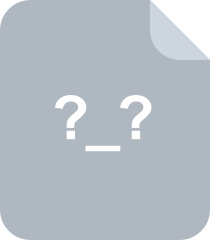

共 6 条
- 1
资源评论
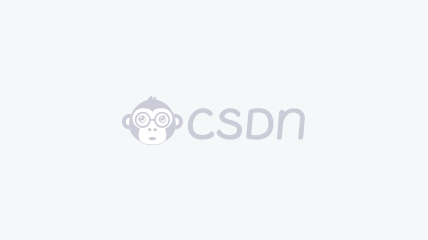

处处清欢
- 粉丝: 154
- 资源: 2504
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

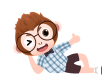
安全验证
文档复制为VIP权益,开通VIP直接复制
