在本课程设计中,我们探讨的是一个基于SQL和Java实现的学生信息管理系统。这个系统的核心功能是管理和处理学生的各种信息,包括但不限于学号、姓名、性别、出生日期、专业等。这个项目不仅提供了对数据的增删改查操作,还可能包含对学生信息的统计和查询功能,比如按年级、专业进行分类统计。 让我们关注SQL部分。SQL(Structured Query Language)是用于管理和处理关系型数据库的标准编程语言。在这个项目中,SQL主要负责创建、更新和查询数据表。可能的数据表结构包括“学生表”(可能有字段如id, name, gender, birthdate, major等)、“班级表”(class_id, class_name等)以及可能的“成绩表”(student_id, course_id, score等)。SQL语句如`CREATE TABLE`用于建表,`INSERT INTO`用于插入数据,`SELECT`用于查询,`UPDATE`用于修改,`DELETE`用于删除。 接着,我们来看Java部分。Java是一种广泛使用的面向对象的编程语言,尤其适用于开发跨平台的桌面应用和Web应用。在这个系统中,Java主要用于编写前端界面和后端逻辑。前端可能使用Swing或JavaFX来构建用户交互界面,用户可以通过这些界面进行数据操作。后端则通过JDBC(Java Database Connectivity)来连接和操作数据库,执行SQL语句。JDBC接口提供了一系列的方法,如`Connection`用于建立数据库连接,`Statement`或`PreparedStatement`用于执行SQL,`ResultSet`用于获取查询结果。 此外,数据库的设计和优化也是关键。为了保证高效的数据访问,可能需要考虑索引的创建,例如在经常用于查询的字段上建立索引,以提高查询速度。同时,合理的数据范式化能减少数据冗余和提高数据一致性。 在系统开发过程中,还需考虑异常处理和安全性。Java的try-catch语句可以捕获并处理运行时可能出现的错误。对于SQL注入等安全问题,应使用预编译的SQL语句,并对用户输入进行验证和清理。 为了便于团队协作和版本控制,项目通常会使用版本控制系统如Git,同时结合Maven或Gradle等构建工具进行依赖管理,确保所有开发者使用的库版本一致。 这个课程设计涵盖了数据库管理、面向对象编程、用户界面设计、数据操作和安全等多个IT领域的核心知识点,对于提升开发者综合技能非常有帮助。通过实际操作,学生不仅能深入理解SQL和Java的使用,还能了解软件开发的整体流程。
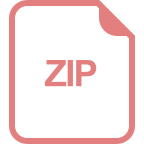
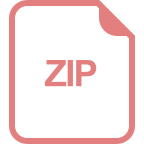
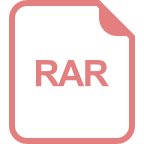
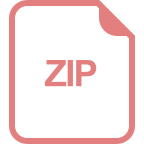
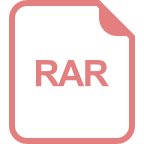
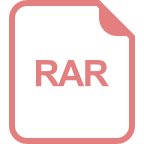
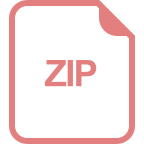
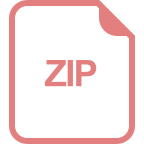
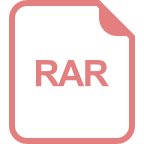
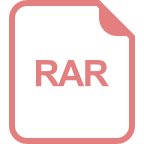
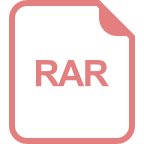
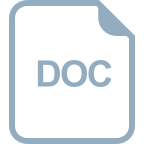
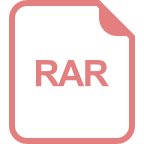
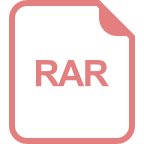
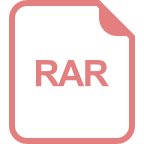
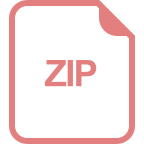
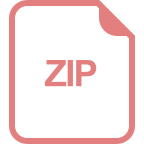
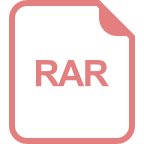
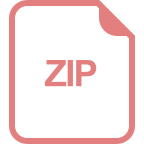
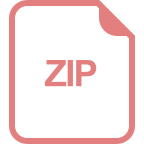
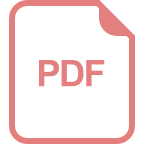
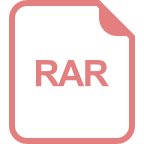
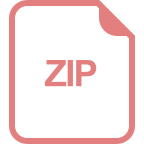
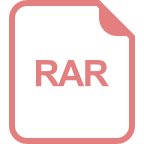
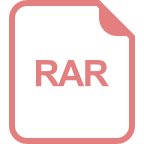
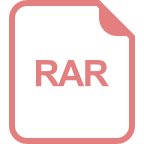
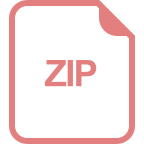



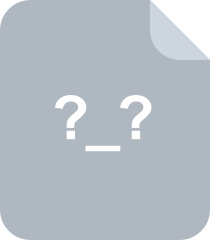
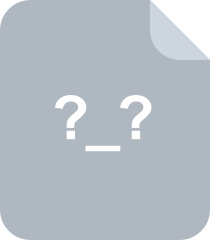
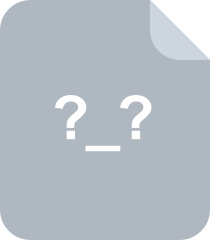
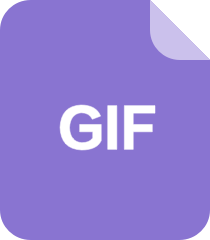
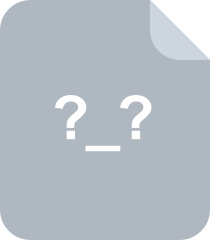
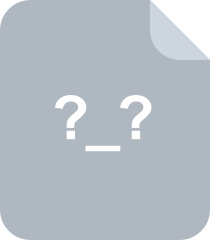
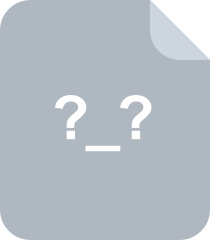
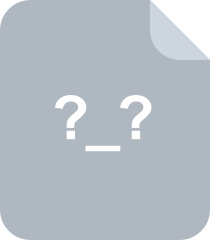

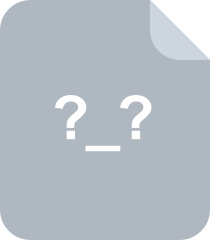
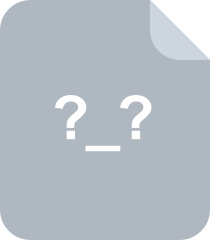
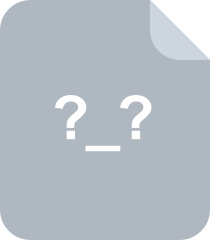
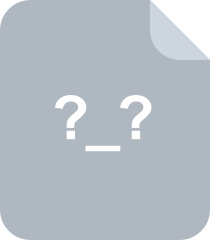
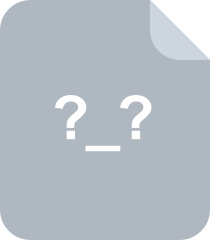
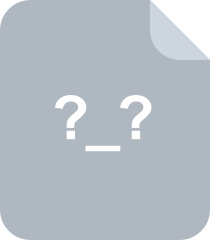
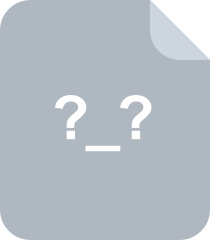
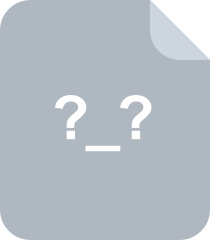
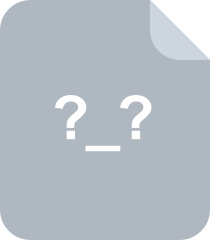
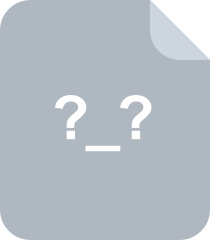
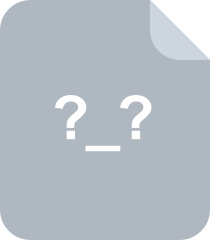
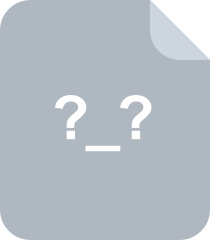
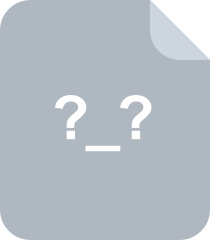
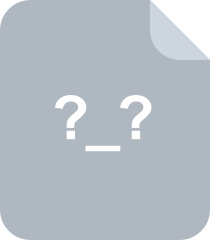
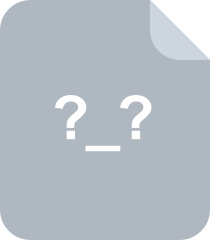
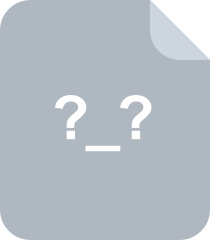
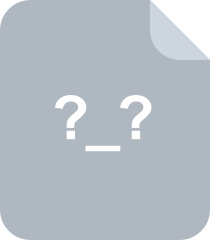
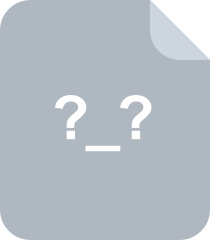
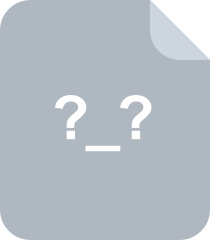
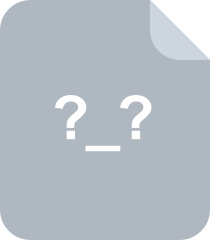
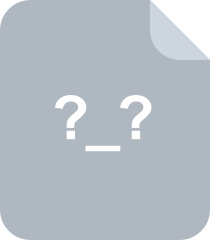
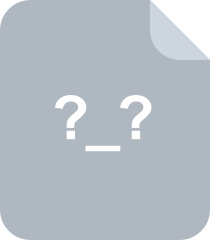
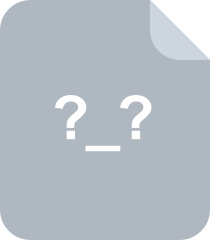
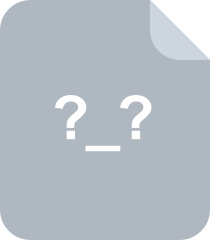
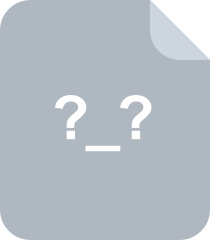
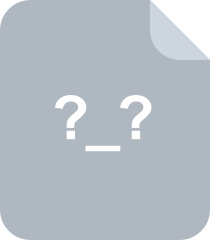
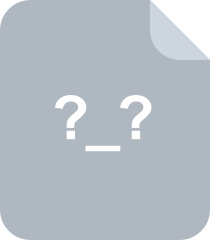
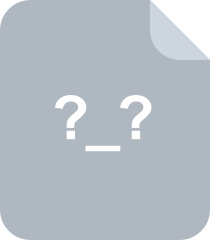
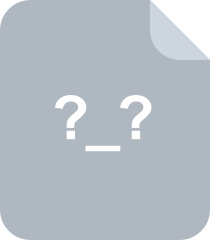
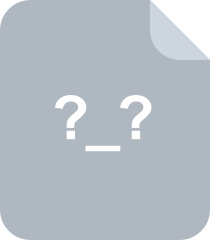
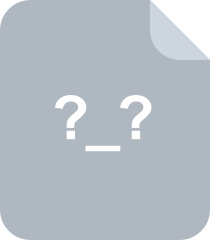
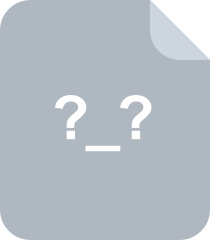
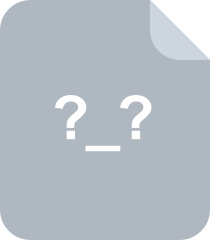
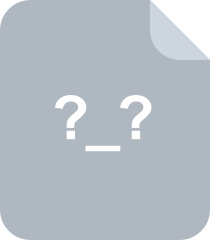
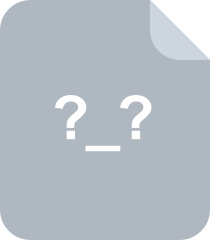
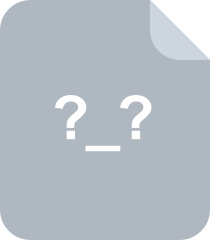
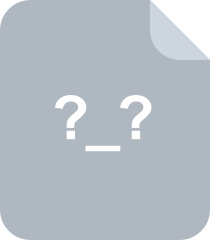
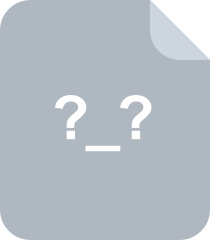
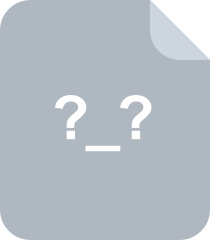
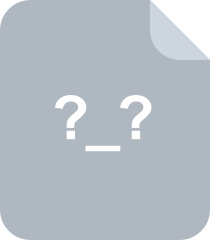
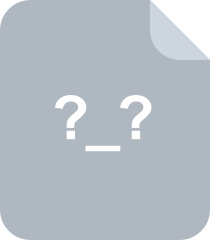
- 1
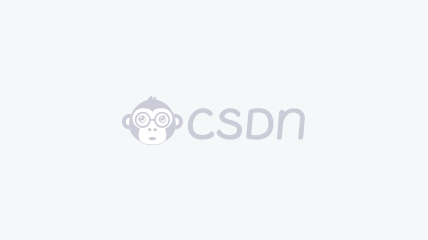

- 粉丝: 2104
- 资源: 2873
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

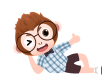
最新资源
- HTML5+Canvas漂亮的3D烟花2025跨年特效
- 一段跨年烟花代码,红红火火,再接再厉
- 微信支付接口 V3 版 python 库
- 基于python的二手房数据分析完整源码+说明文档+报告PPT(高分必过项目)
- HCIE-openEuler欧拉认证笔试题库
- HCIE-openEuler欧拉认证实验实战
- dc-dc电源芯片电路 TPS63000是一款高效升 降压转器,它采用3mmX3mm的QFN-10封装工艺 主要性能:输入电压:3.6V~5.5V(降压模式).1.8V~5.5V(升压模式);输出
- 腾讯云开发者工具套件3.0,SDK3.0是云API3.0平台的配套工具 目前已经支持cvm、vpc、cbs等产品,后续所有的云服务产品都会接入进来 新版SDK实现了统一化,具有各个语言版本的SDK使用
- 基于Spring Boot + Vue +MySQL的学生社团管理系统源代码+数据库
- DAB暂态直流偏置抑制Matlab/Simulink仿真【2018b版本】 本仿真对DAB变换器的状态切换过程的暂态直流偏置进行抑制,可以实现状态切换过程的暂态直流偏置
- AT89C52 amtel单片机芯片微控制器(MCU)cadence orcad pcb
- 视频编码标准VVC中增强电影胶片颗粒处理方法:提升视觉质量和效率
- MATLAB神经网络原理与实例视频精解
- 资料:灯珠记录笔记灯珠记录笔记
- Qt人脸识别,基于opencv,dlib
- 高效仿射运动估计技术优化视频编码器复杂度并加速Versatile Video Coding (VVC)

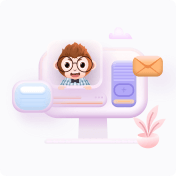
