/*
* Copyright (C) 2009 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.lody.virtual.server.content;
import android.accounts.Account;
import android.content.ComponentName;
import android.content.ContentResolver;
import android.content.Context;
import android.content.ISyncStatusObserver;
import android.content.PeriodicSync;
import android.content.SyncStatusInfo;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteException;
import android.database.sqlite.SQLiteQueryBuilder;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.os.Parcel;
import android.os.RemoteCallbackList;
import android.os.RemoteException;
import android.util.Log;
import android.util.Pair;
import android.util.SparseArray;
import android.util.Xml;
import com.lody.virtual.helper.compat.ContentResolverCompat;
import com.lody.virtual.helper.utils.ArrayUtils;
import com.lody.virtual.helper.utils.AtomicFile;
import com.lody.virtual.helper.utils.FastXmlSerializer;
import com.lody.virtual.os.VEnvironment;
import com.lody.virtual.server.accounts.AccountAndUser;
import org.xmlpull.v1.XmlPullParser;
import org.xmlpull.v1.XmlPullParserException;
import org.xmlpull.v1.XmlSerializer;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Random;
import java.util.TimeZone;
/**
* Singleton that tracks the sync data and overall sync
* history on the device.
*
* @hide
*/
public class SyncStorageEngine extends Handler {
private static final String TAG = "SyncManager";
private static final boolean DEBUG = false;
private static final String TAG_FILE = "SyncManagerFile";
private static final String XML_ATTR_NEXT_AUTHORITY_ID = "nextAuthorityId";
private static final String XML_ATTR_LISTEN_FOR_TICKLES = "listen-for-tickles";
private static final String XML_ATTR_SYNC_RANDOM_OFFSET = "offsetInSeconds";
private static final String XML_ATTR_ENABLED = "enabled";
private static final String XML_ATTR_USER = "user";
private static final String XML_TAG_LISTEN_FOR_TICKLES = "listenForTickles";
/**
* Default time for a periodic sync.
*/
private static final long DEFAULT_POLL_FREQUENCY_SECONDS = 60 * 60 * 24; // One day
/**
* Percentage of period that is flex by default, if no flex is set.
*/
private static final double DEFAULT_FLEX_PERCENT_SYNC = 0.04;
/**
* Lower bound on sync time from which we assign a default flex time.
*/
private static final long DEFAULT_MIN_FLEX_ALLOWED_SECS = 5;
/**
* Enum value for a sync start event.
*/
public static final int EVENT_START = 0;
/**
* Enum value for a sync stop event.
*/
public static final int EVENT_STOP = 1;
// TODO: i18n -- grab these out of resources.
/**
* String names for the sync event types.
*/
public static final String[] EVENTS = {"START", "STOP"};
/**
* Enum value for a server-initiated sync.
*/
public static final int SOURCE_SERVER = 0;
/**
* Enum value for a local-initiated sync.
*/
public static final int SOURCE_LOCAL = 1;
/**
* Enum value for a poll-based sync (e.g., upon connection to
* network)
*/
public static final int SOURCE_POLL = 2;
/**
* Enum value for a user-initiated sync.
*/
public static final int SOURCE_USER = 3;
/**
* Enum value for a periodic sync.
*/
public static final int SOURCE_PERIODIC = 4;
public static final long NOT_IN_BACKOFF_MODE = -1;
// TODO: i18n -- grab these out of resources.
/**
* String names for the sync source types.
*/
public static final String[] SOURCES = {"SERVER",
"LOCAL",
"POLL",
"USER",
"PERIODIC"};
// The MESG column will contain one of these or one of the Error types.
public static final String MESG_SUCCESS = "success";
public static final String MESG_CANCELED = "canceled";
public static final int MAX_HISTORY = 100;
private static final int MSG_WRITE_STATUS = 1;
private static final long WRITE_STATUS_DELAY = 1000 * 60 * 10; // 10 minutes
private static final int MSG_WRITE_STATISTICS = 2;
private static final long WRITE_STATISTICS_DELAY = 1000 * 60 * 30; // 1/2 hour
private static final boolean SYNC_ENABLED_DEFAULT = false;
// the version of the accounts xml file format
private static final int ACCOUNTS_VERSION = 2;
private static HashMap<String, String> sAuthorityRenames;
static {
sAuthorityRenames = new HashMap<String, String>();
sAuthorityRenames.put("contacts", "com.android.contacts");
sAuthorityRenames.put("calendar", "com.android.calendar");
}
public static class PendingOperation {
final Account account;
final int userId;
final int reason;
final int syncSource;
final String authority;
final Bundle extras; // note: read-only.
final ComponentName serviceName;
final boolean expedited;
int authorityId;
byte[] flatExtras;
PendingOperation(Account account, int userId, int reason, int source,
String authority, Bundle extras, boolean expedited) {
this.account = account;
this.userId = userId;
this.syncSource = source;
this.reason = reason;
this.authority = authority;
this.extras = extras != null ? new Bundle(extras) : extras;
this.expedited = expedited;
this.authorityId = -1;
this.serviceName = null;
}
PendingOperation(PendingOperation other) {
this.account = other.account;
this.userId = other.userId;
this.reason = other.reason;
this.syncSource = other.syncSource;
this.authority = other.authority;
this.extras = other.extras;
this.authorityId = other.authorityId;
this.expedited = other.expedited;
this.serviceName = other.serviceName;
}
}
static class AccountInfo {
final AccountAndUser accountAndUser;
final HashMap<String, AuthorityInfo> authorities =
new HashMap<String, AuthorityInfo>();
AccountInfo(AccountAndUser accountAndUser) {
this.accountAndUser = accountAndUser;
}
}
public static class AuthorityInfo {
final ComponentName service;
final Account account;
final int userId;
final String authority;
final int ident;
boolean enabled;
int syncable;
long backoffTime;
long backoffDelay;
long delayUntil;
final ArrayList<PeriodicSync> periodicSyncs;
/**
* Copy constructor for making deep-ish copies. Only the bundles stored
* in periodic syncs can make unexpected changes.
*
* @param toCopy AuthorityInfo to be copied.
*/
AuthorityInfo(AuthorityInfo toCopy) {
account = toCopy.account;
userId = toCopy.userId;
authority = toCopy.auth
没有合适的资源?快使用搜索试试~ 我知道了~
具有Android 12/11/11兼容性的VirtualApp-Android开发
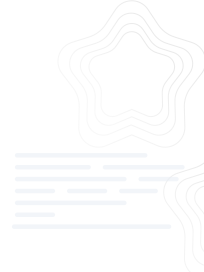
共1246个文件
java:842个
xml:93个
aidl:86个


温馨提示
VirtualApp With Compatibility Of Android 10/11/12 VirtualApp 工程 全新体验,多种优化 特性 高性能、高稳定性、修复构建错误等等 本内核仅供开发人员参考,请勿构建成品并发表到任何地方 为了避免SB骚扰,请不要在社区提及本工程,万分感谢 仅供自行测试使用 如有修改建议欢迎提交PR 注意,源代码需要遵循GPLv3协议进行开源 请使用本工程的项目注明出处 联系方式:[email protected] QQ 2737996094 网站:http://www.die.lu/ 构建方式: gradle build
资源详情
资源评论
资源推荐
收起资源包目录

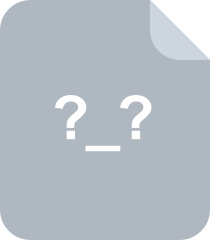
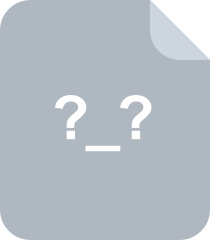
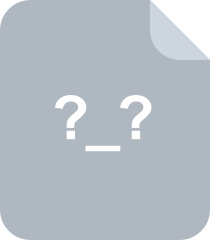
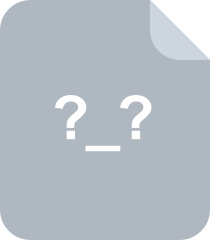
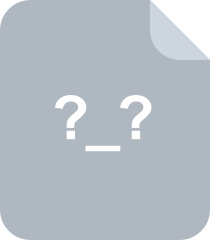
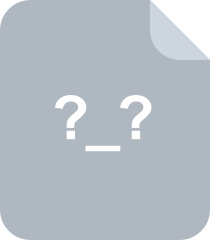
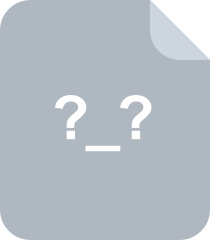
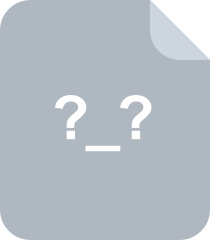
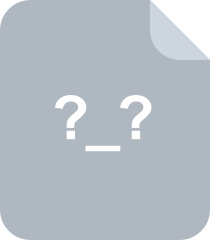
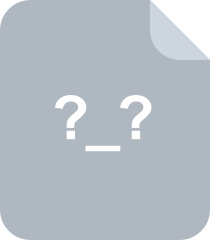
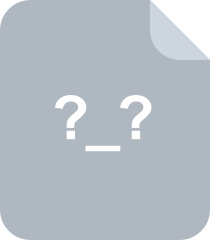
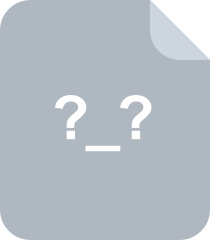
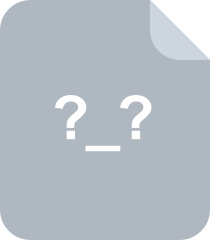
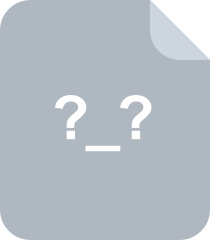
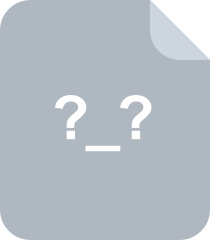
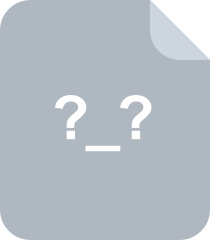
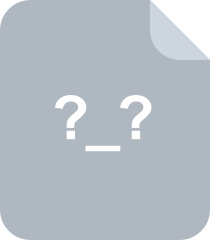
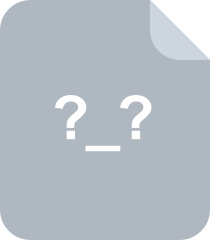
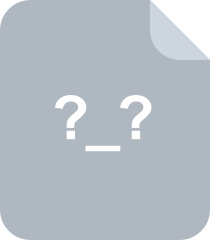
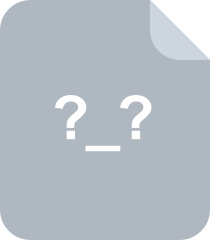
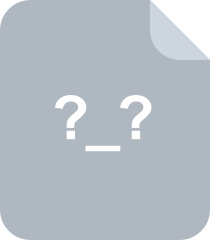
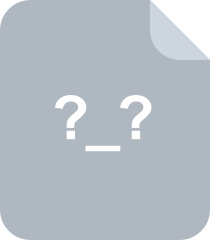
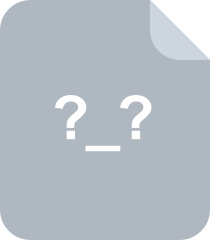
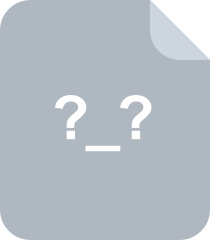
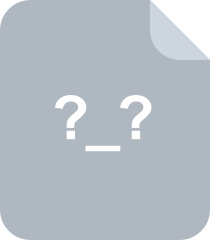
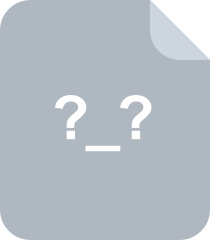
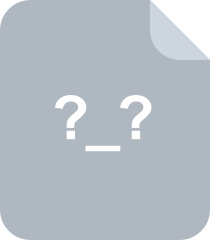
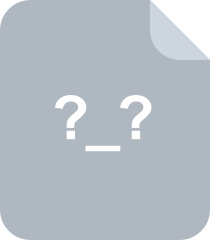
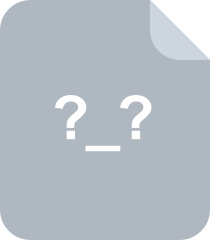
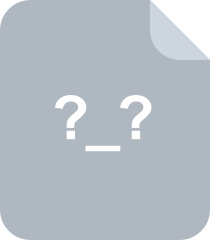
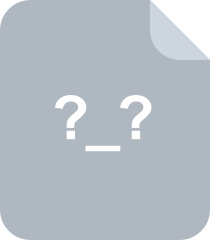
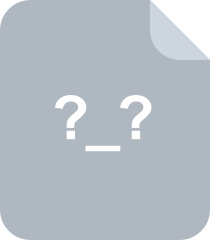
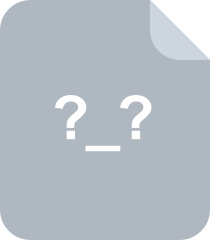
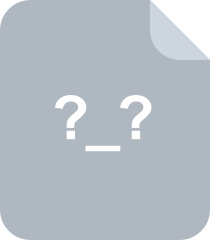
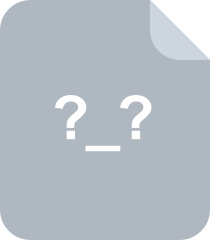
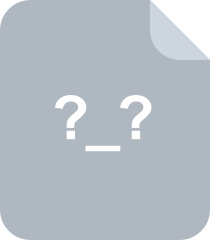
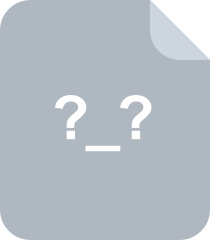
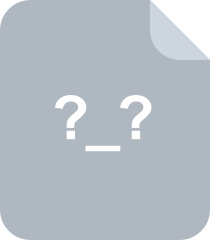
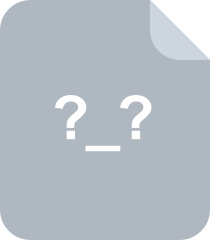
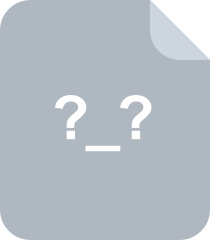
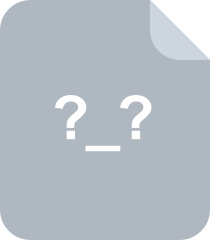
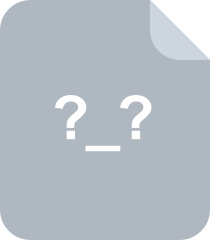
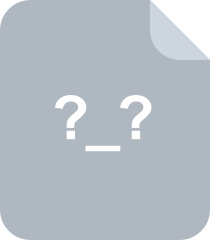
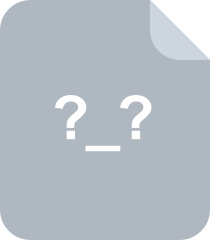
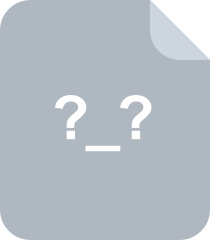
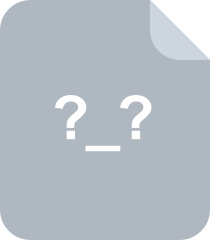
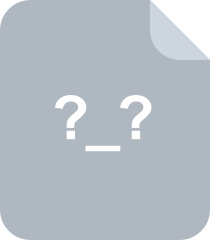
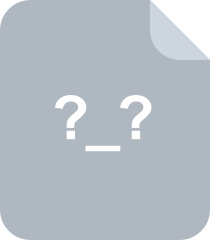
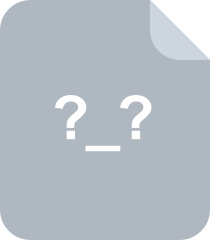
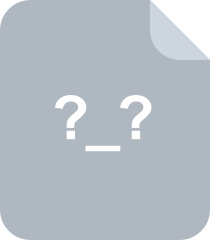
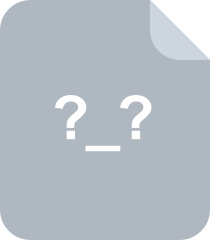
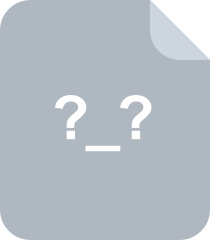
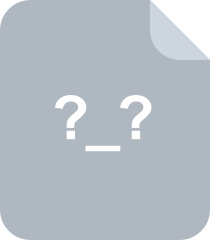
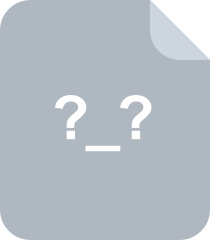
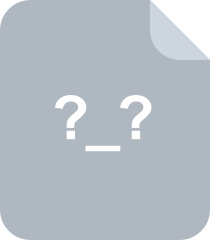
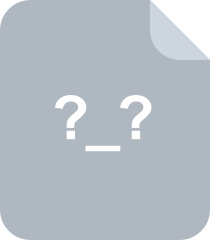
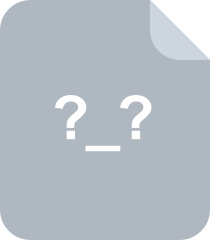
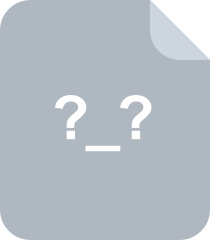
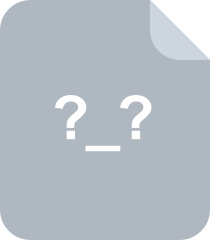
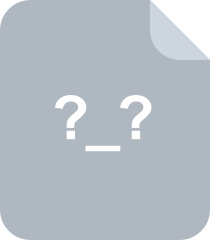
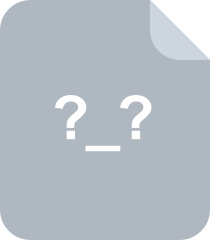
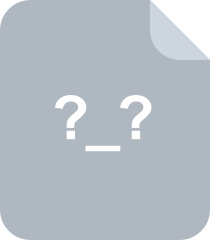
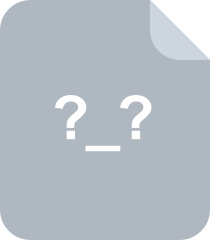
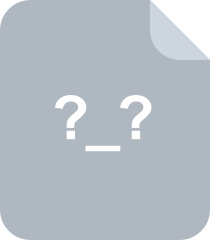
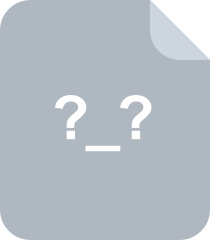
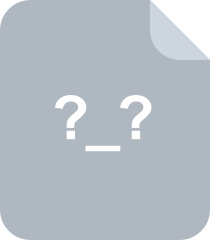
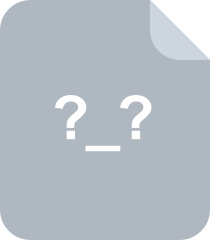
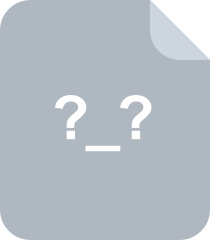
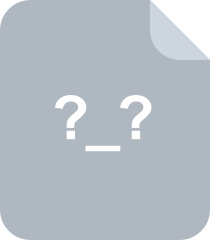
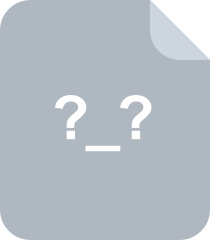
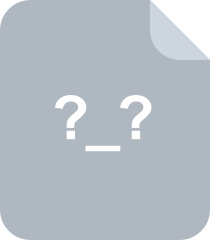
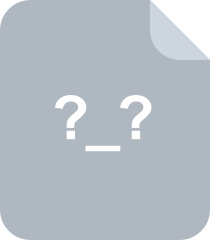
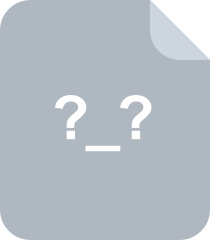
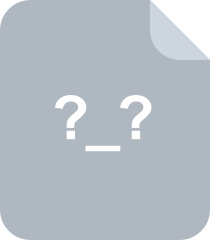
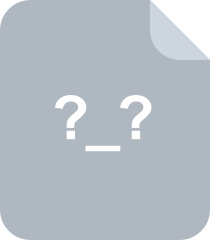
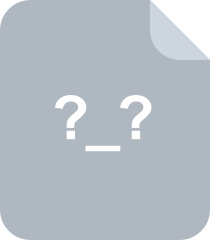
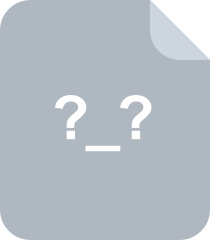
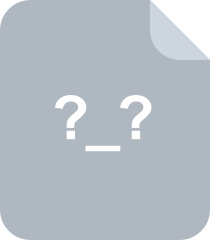
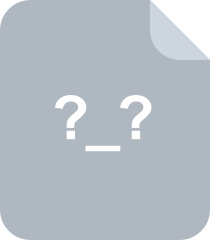
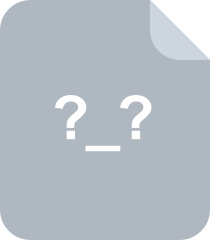
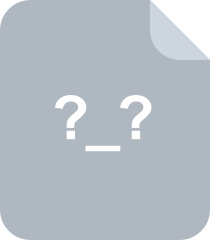
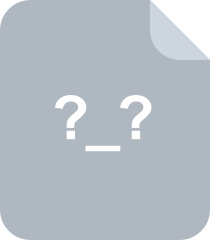
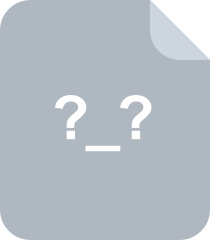
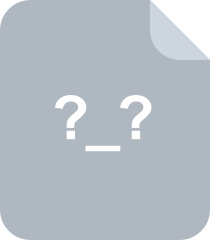
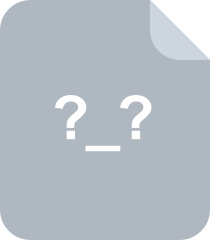
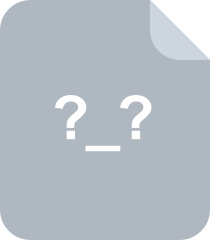
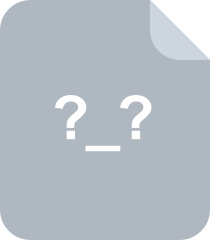
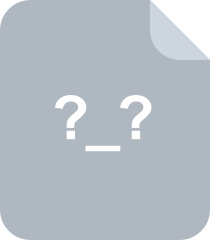
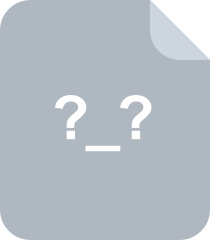
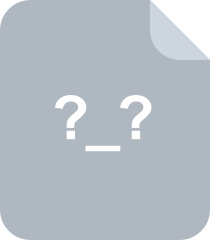
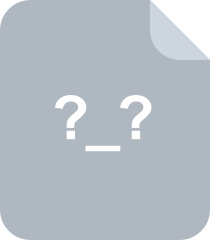
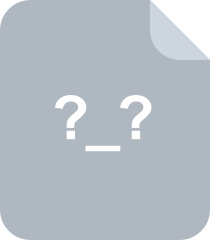
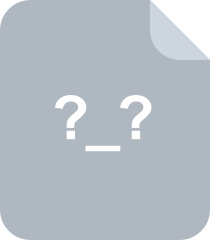
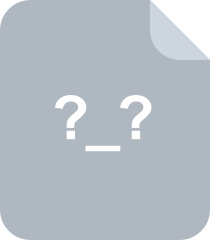
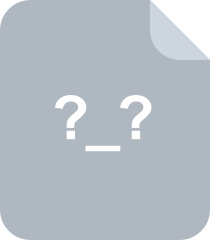
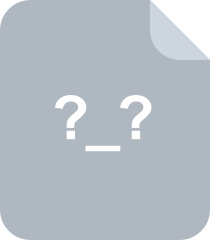
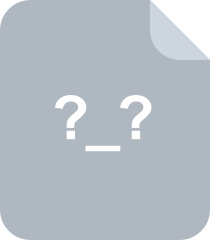
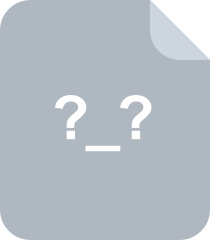
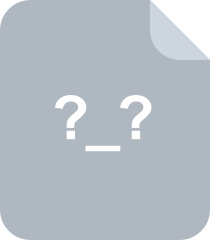
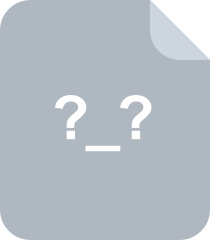
共 1246 条
- 1
- 2
- 3
- 4
- 5
- 6
- 13
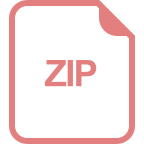
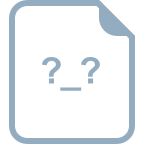
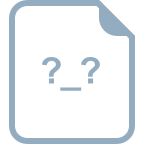
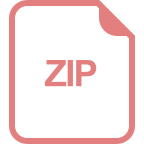
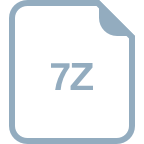
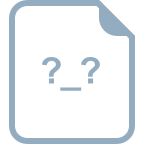
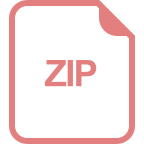
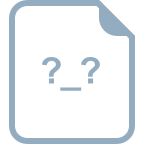
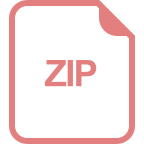
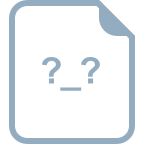
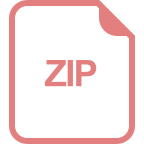
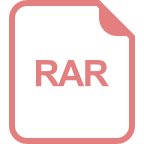
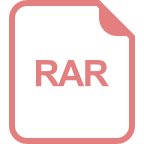
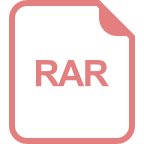
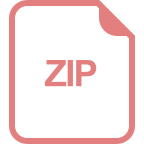
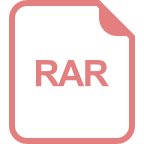
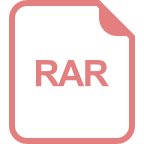
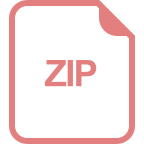
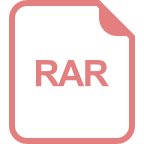
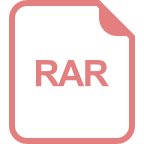
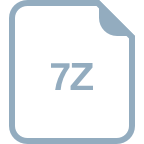
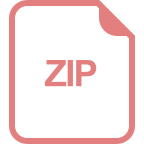
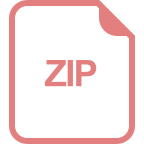
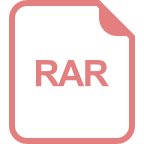

weixin_42128015
- 粉丝: 24
- 资源: 4640
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

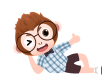
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


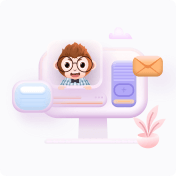
安全验证
文档复制为VIP权益,开通VIP直接复制

评论1