# Python Module for 2Captcha API
The easiest way to quickly integrate [2Captcha] captcha solving service into your code to automate solving of any types of captcha.
- [Installation](#installation)
- [Configuration](#configuration)
- [Solve captcha](#solve-captcha)
- [Normal Captcha](#normal-captcha)
- [Text](#text-captcha)
- [ReCaptcha v2](#recaptcha-v2)
- [ReCaptcha v3](#recaptcha-v3)
- [FunCaptcha](#funcaptcha)
- [GeeTest](#geetest)
- [hCaptcha](#hcaptcha)
- [KeyCaptcha](#keycaptcha)
- [Capy](#capy)
- [Grid (ReCaptcha V2 Old Method)](#grid)
- [Canvas](#canvas)
- [ClickCaptcha](#clickcaptcha)
- [Rotate](#rotate)
- [Other methods](#other-methods)
- [send / getResult](#send--getresult)
- [balance](#balance)
- [report](#report)
- [Error handling](#error-handling)
## Installation
This package can be installed with Pip:
```pip3 install 2captcha-python```
## Configuration
TwoCaptcha instance can be created like this:
```python
from twocaptcha import TwoCaptcha
solver = TwoCaptcha('YOUR_API_KEY')
```
Also there are few options that can be configured:
```python
config = {
'server': '2captcha.com',
'apiKey': 'YOUR_API_KEY',
'softId': 123,
'callback': 'https://your.site/result-receiver',
'defaultTimeout': 120,
'recaptchaTimeout': 600,
'pollingInterval': 10,
}
solver = TwoCaptcha(**config)
```
### TwoCaptcha instance options
|Option|Default value|Description|
|---|---|---|
|server|`2captcha.com`|API server. You can set it to `rucaptcha.com` if your account is registered there|
|softId|-|your software ID obtained after publishing in [2captcha sofware catalog]|
|callback|-|URL of your web-sever that receives the captcha recognition result. The URl should be first registered in [pingback settings] of your account|
|defaultTimeout|120|Polling timeout in seconds for all captcha types except ReCaptcha. Defines how long the module tries to get the answer from `res.php` API endpoint|
|recaptchaTimeout|600|Polling timeout for ReCaptcha in seconds. Defines how long the module tries to get the answer from `res.php` API endpoint|
|pollingInterval|10|Interval in seconds between requests to `res.php` API endpoint, setting values less than 5 seconds is not recommended|
> **IMPORTANT:** once `callback` is defined for `TwoCaptcha` instance, all methods return only the captcha ID and DO NOT poll the API to get the result. The result will be sent to the callback URL.
To get the answer manually use [getResult method](#send--getresult)
## Solve captcha
When you submit any image-based captcha use can provide additional options to help 2captcha workers to solve it properly.
### Captcha options
|Option|Default Value|Description|
|---|---|---|
|numeric|0|Defines if captcha contains numeric or other symbols [see more info in the API docs][post options]|
|minLength|0|minimal answer lenght|
|maxLength|0|maximum answer length|
|phrase|0|defines if the answer contains multiple words or not|
|caseSensitive|0|defines if the answer is case sensitive|
|calc|0|defines captcha requires calculation|
|lang|-|defines the captcha language, see the [list of supported languages] |
|hintImg|-|an image with hint shown to workers with the captcha|
|hintText|-|hint or task text shown to workers with the captcha|
Below you can find basic examples for every captcha type. Check out [examples directory] to find more examples with all available options.
### Normal Captcha
To bypass a normal captcha (distorted text on image) use the following method. This method also can be used to recognize any text on the image.
```python
result = solver.normal('path/to/captcha.jpg', param1=..., ...)
```
### Text Captcha
This method can be used to bypass a captcha that requires to answer a question provided in clear text.
```python
result = solver.text('If tomorrow is Saturday, what day is today?', param1=..., ...)
```
### ReCaptcha v2
Use this method to solve ReCaptcha V2 and obtain a token to bypass the protection.
```python
result = solver.recaptcha(sitekey='6Le-wvkSVVABCPBMRTvw0Q4Muexq1bi0DJwx_mJ-',
url='https://mysite.com/page/with/recaptcha’,
param1=..., ...)
```
### ReCaptcha v3
This method provides ReCaptcha V3 solver and returns a token.
```python
result = solver.recaptcha(sitekey=’6Le-wvkSVVABCPBMRTvw0Q4Muexq1bi0DJwx_mJ-',
url='https://mysite.com/page/with/recaptcha',
version='v3',
param1=..., ...)
```
### FunCaptcha
FunCaptcha (Arkoselabs) solving method. Returns a token.
```python
result = solver.funcaptcha(sitekey='6Le-wvkSVVABCPBMRTvw0Q4Muexq1bi0DJwx_mJ-',
url='https://mysite.com/page/with/funcaptcha',
param1=..., ...)
```
### GeeTest
Method to solve GeeTest puzzle captcha. Returns a set of tokens as JSON.
```python
result = solver.geetest(gt='f1ab2cdefa3456789012345b6c78d90e',
challenge='12345678abc90123d45678ef90123a456b',
url='https://www.site.com/page/',
param1=..., ...)
```
### hCaptcha
Use this method to solve hCaptcha challenge. Returns a token to bypass captcha.
```python
result = solver.hcaptcha(sitekey='10000000-ffff-ffff-ffff-000000000001',
url='https://www.site.com/page/',
param1=..., ...)
```
### KeyCaptcha
Token-based method to solve KeyCaptcha.
```python
result = solver.keycaptcha(s_s_c_user_id=10,
s_s_c_session_id='493e52c37c10c2bcdf4a00cbc9ccd1e8',
s_s_c_web_server_sign='9006dc725760858e4c0715b835472f22-pz-',
s_s_c_web_server_sign2='2ca3abe86d90c6142d5571db98af6714',
url='https://www.keycaptcha.ru/demo-magnetic/',
param1=..., ...)
```
### Capy
Token-based method to bypass Capy puzzle captcha.
```python
result = solver.capy(sitekey='PUZZLE_Abc1dEFghIJKLM2no34P56q7rStu8v',
url='http://mysite.com/',
api_server='https://jp.api.capy.me/',
param1=..., ...)
```
### Grid
Grid method is originally called Old ReCaptcha V2 method. The method can be used to bypass any type of captcha where you can apply a grid on image and need to click specific grid boxes. Returns numbers of boxes.
```python
result = solver.grid('path/to/captcha.jpg', param1=..., ...)
```
### Canvas
Canvas method can be used when you need to draw a line around an object on image. Returns a set of points' coordinates to draw a polygon.
```python
result = solver.canvas('path/to/captcha.jpg', param1=..., ...)
```
### ClickCaptcha
ClickCaptcha method returns coordinates of points on captcha image. Can be used if you need to click on particular points on the image.
```python
result = solver.coordinates('path/to/captcha.jpg', param1=..., ...)
```
### Rotate
This method can be used to solve a captcha that asks to rotate an object. Mostly used to bypass FunCaptcha. Returns the rotation angle.
```python
result = solver.rotate('path/to/captcha.jpg', param1=..., ...)
```
## Other methods
### send / getResult
These methods can be used for manual captcha submission and answer polling.
```python
import time
. . . . .
id = solver.send(file='path/to/captcha.jpg')
time.sleep(20)
code = solver.get_result(id)
```
### balance
Use this method to get your account's balance
```python
balance = solver.balance()
```
### report
Use this method to report good or bad captcha answer.
```python
solver.report(id, True) # captcha solved correctly
solver.report(id, False) # captcha solved incorrectly
```
### Error handling
If case of an error captcha solver throws an exception. It's important to properly handle these cases. We recommend to use `try except` to handle exceptions.
```python
Try:
result = solver.text('If t
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
2Captcha API的Python模块 快速将码解决方案服务集成到您的代码中以自动解决任何类型的验证码的最简单方法。 ClickCaptcha 旋转 其他方法 发送/获取结果 平衡 报告 错误处理 安装 该软件包可以与Pip一起安装: pip3 install 2captcha-python 配置 可以这样创建TwoCaptcha实例: from twocaptcha import TwoCaptcha solver = TwoCaptcha ( 'YOUR_API_KEY' ) 另外,可以配置的选项很少: config = { 'server' : '2captcha.com' , 'apiKey' : 'YOUR_API_KEY' , 'softId' :
资源详情
资源评论
资源推荐
收起资源包目录


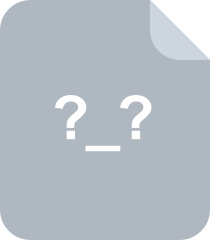
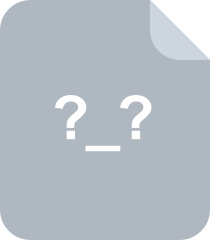
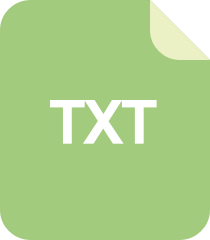

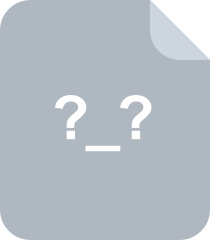

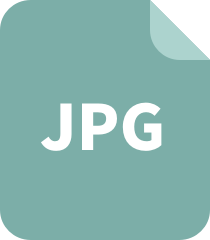
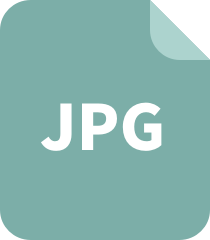
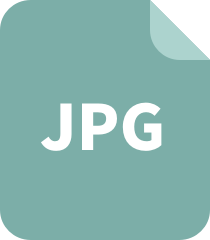
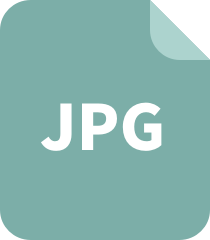
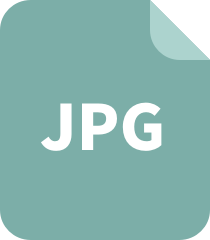
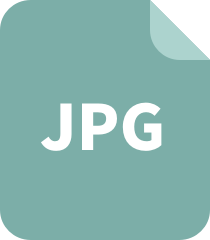
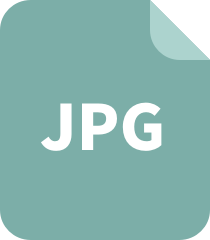
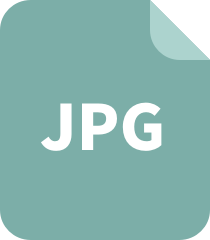
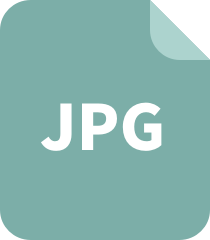
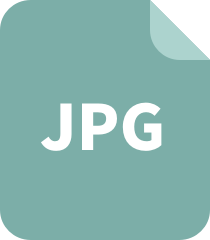
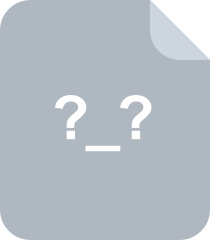
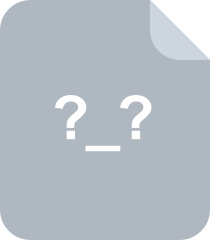
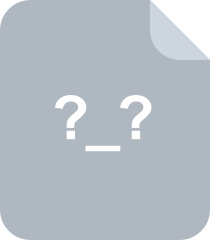
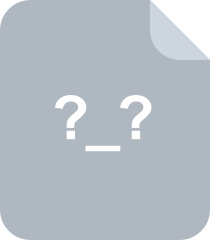
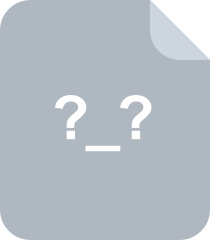
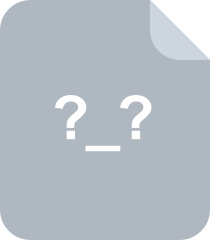
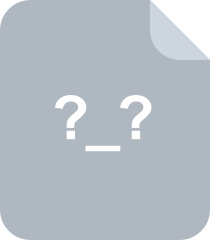
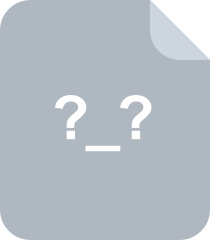
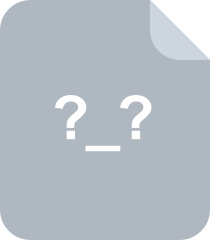
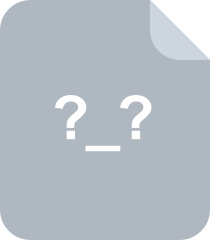
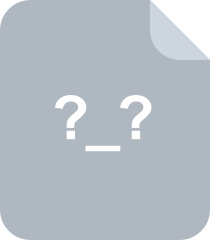
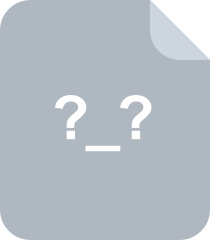
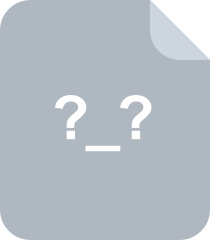
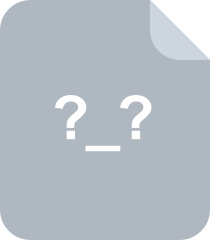
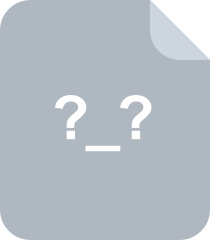
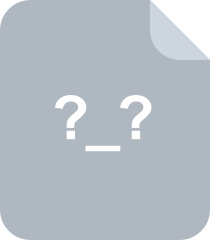
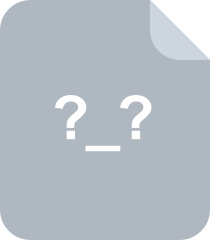
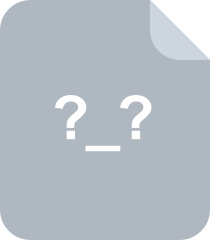
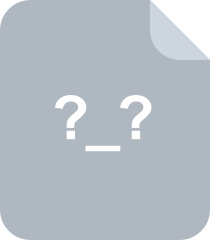
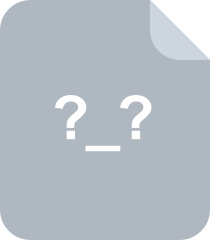
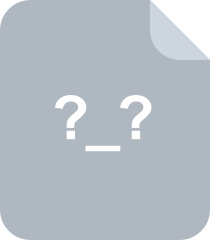
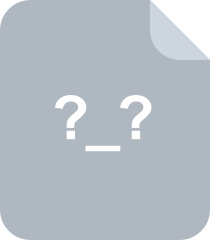
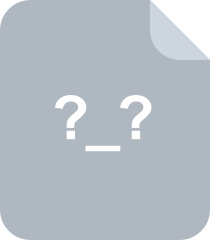
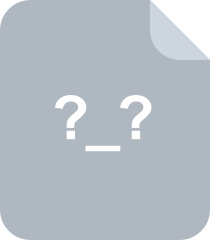
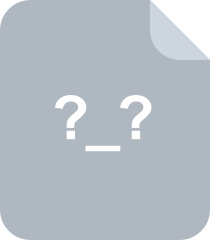
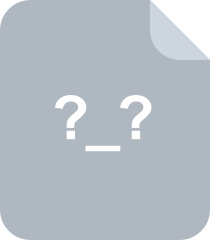
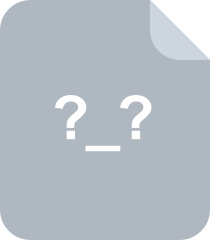
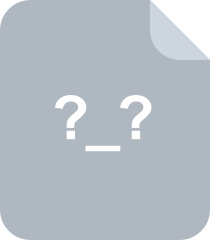
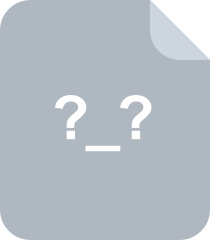
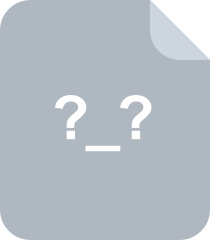

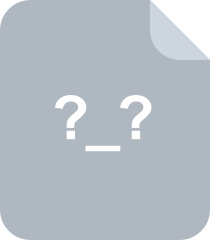
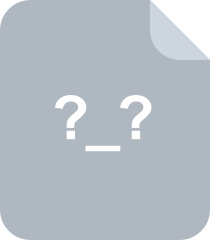
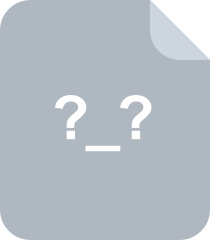
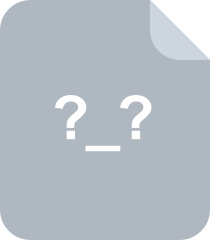
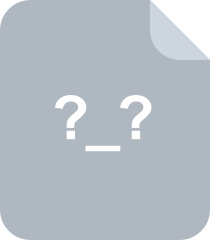
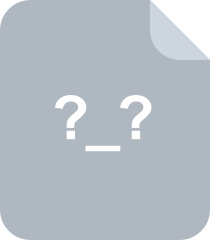
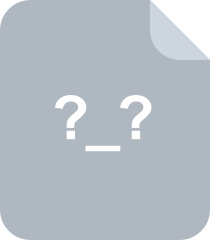
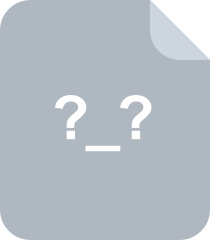
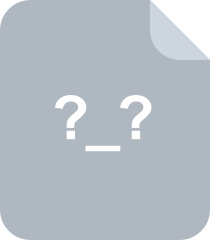
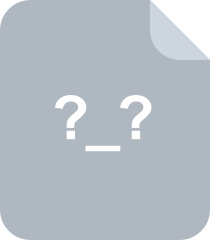
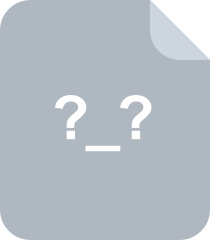
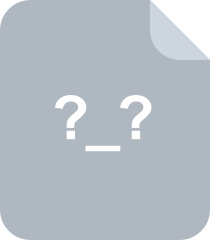
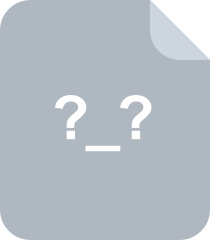
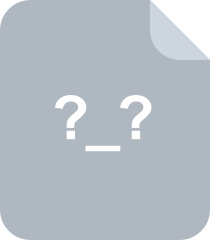

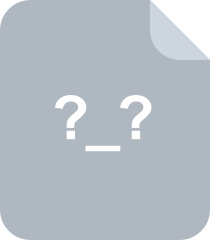
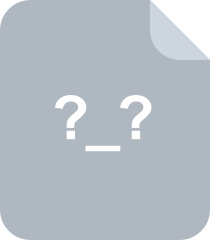
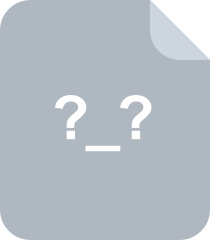
共 61 条
- 1
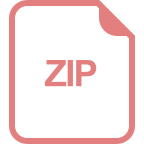
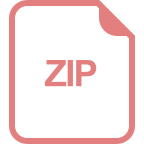
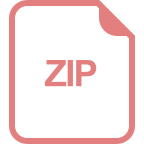
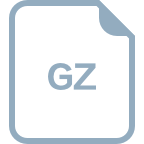
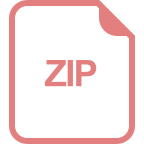
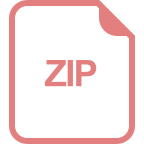
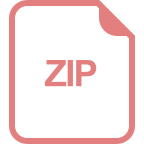
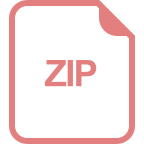
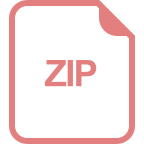
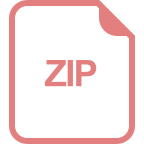
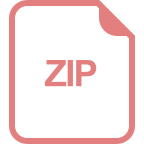
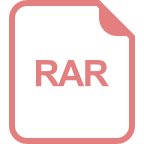
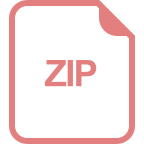
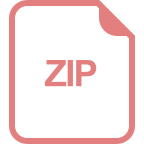
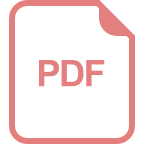
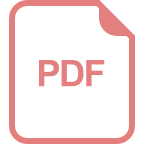
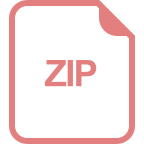
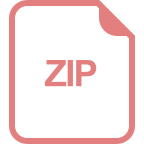
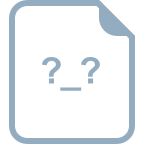
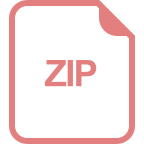
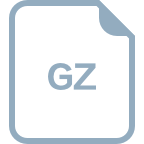
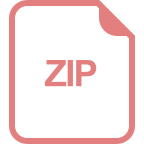
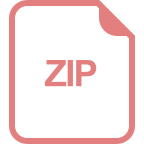
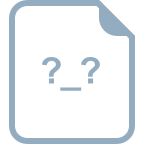
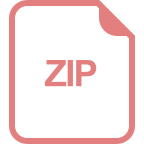
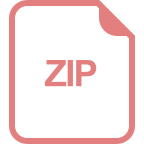

一起快走吧
- 粉丝: 33
- 资源: 4658
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

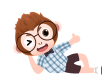
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


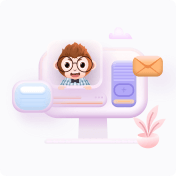
安全验证
文档复制为VIP权益,开通VIP直接复制

评论1