/*
Library: STM32F40x Internal FLASH read/write
Written by: Mohamed Yaqoob (MYaqoobEmbedded YouTube Channel)
Last modified: 15/03/2019
Description:
MY_FLASH library implements the following basic functionalities
- Set sectos address
- Flash Sector Erase
- Flash Write
- Flash Read
* Copyright (C) 2019 - M. Yaqoob
This is a free software under the GNU license, you can redistribute it and/or modify it under the terms
of the GNU General Public Licenseversion 3 as published by the Free Software Foundation.
This software library is shared with puplic for educational purposes, without WARRANTY and Author is not liable for any damages caused directly
or indirectly by this software, read more about this on the GNU General Public License.
*/
#include "MY_FLASH.h"
//Private variables
//1. sector start address
static uint32_t MY_SectorAddrs;
static uint8_t MY_SectorNum;
//functions definitions
//1. Erase Sector
static void MY_FLASH_EraseSector(void)
{
HAL_FLASH_Unlock();
//Erase the required Flash sector
FLASH_Erase_Sector(MY_SectorNum, FLASH_VOLTAGE_RANGE_3);
HAL_FLASH_Lock();
}
//2. Set Sector Adress
void MY_FLASH_SetSectorAddrs(uint8_t sector, uint32_t addrs)
{
MY_SectorNum = sector;
MY_SectorAddrs = addrs;
}
//3. Write Flash
void MY_FLASH_WriteN(uint32_t idx, void *wrBuf, uint32_t Nsize, DataTypeDef dataType)
{
uint32_t flashAddress = MY_SectorAddrs + idx;
//Erase sector before write
MY_FLASH_EraseSector();
//Unlock Flash
HAL_FLASH_Unlock();
//Write to Flash
switch(dataType)
{
case DATA_TYPE_8:
for(uint32_t i=0; i<Nsize; i++)
{
HAL_FLASH_Program(FLASH_TYPEPROGRAM_BYTE, flashAddress , ((uint8_t *)wrBuf)[i]);
flashAddress++;
}
break;
case DATA_TYPE_16:
for(uint32_t i=0; i<Nsize; i++)
{
HAL_FLASH_Program(FLASH_TYPEPROGRAM_HALFWORD, flashAddress , ((uint16_t *)wrBuf)[i]);
flashAddress+=2;
}
break;
case DATA_TYPE_32:
for(uint32_t i=0; i<Nsize; i++)
{
HAL_FLASH_Program(FLASH_TYPEPROGRAM_WORD, flashAddress , ((uint32_t *)wrBuf)[i]);
flashAddress+=4;
}
break;
}
//Lock the Flash space
HAL_FLASH_Lock();
}
//4. Read Flash
void MY_FLASH_ReadN(uint32_t idx, void *rdBuf, uint32_t Nsize, DataTypeDef dataType)
{
uint32_t flashAddress = MY_SectorAddrs + idx;
switch(dataType)
{
case DATA_TYPE_8:
for(uint32_t i=0; i<Nsize; i++)
{
*((uint8_t *)rdBuf + i) = *(uint8_t *)flashAddress;
flashAddress++;
}
break;
case DATA_TYPE_16:
for(uint32_t i=0; i<Nsize; i++)
{
*((uint16_t *)rdBuf + i) = *(uint16_t *)flashAddress;
flashAddress+=2;
}
break;
case DATA_TYPE_32:
for(uint32_t i=0; i<Nsize; i++)
{
*((uint32_t *)rdBuf + i) = *(uint32_t *)flashAddress;
flashAddress+=4;
}
break;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
STM32F40x微控制器上进行内部FLASH的读写操作: 1.设置扇区地址 (MY_FLASH_SetSectorAddrs): 功能:设置特定扇区的起始地址。 参数: sector:要设置的扇区号。 addrs:扇区的起始地址。 2. 扇区擦除 (MY_FLASH_EraseSector): 功能:擦除之前设置的扇区。 操作: 解锁FLASH。 使用FLASH_Erase_Sector函数擦除扇区。 锁定FLASH。 3. 写入FLASH (MY_FLASH_WriteN): 功能:向FLASH中写入数据。 参数: idx:起始偏移地址。 wrBuf:指向要写入数据的缓冲区。 Nsize:要写入的数据数量。 dataType:数据类型(DATA_TYPE_8、DATA_TYPE_16、DATA_TYPE_32)。 操作: 4. 擦除目标扇区。 解锁FLASH。 根据数据类型,循环写入数据。 锁定FLASH。 5.读取FLASH (MY_FLASH_ReadN): 功能:从FLASH中读取数据。 参数: idx:起始偏移地址。 rdBuf:指向存放读取数据的缓冲区。
资源推荐
资源详情
资源评论
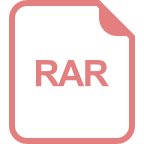
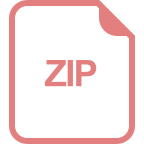
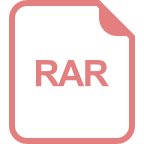
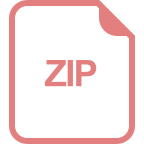
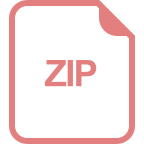
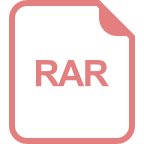
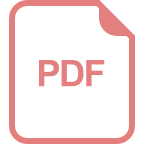
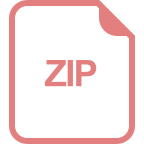
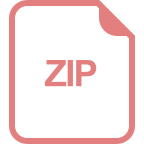
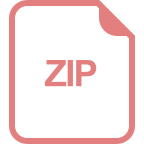
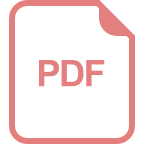
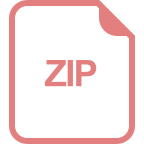
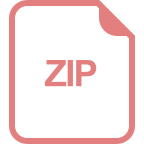
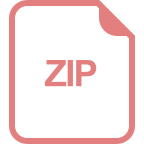
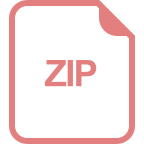
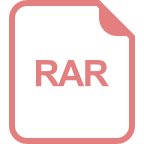
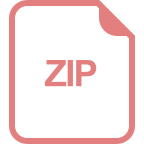
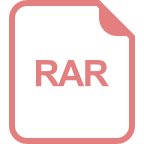
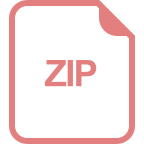
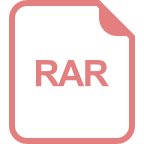
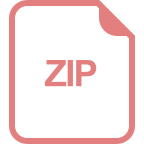
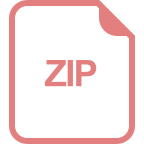
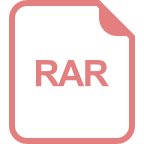
收起资源包目录


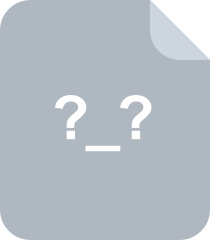
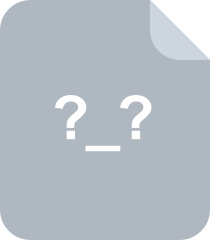
共 2 条
- 1
资源评论
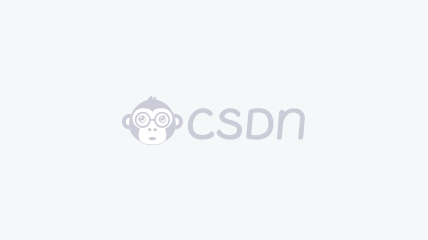

香菜配汤
- 粉丝: 508
- 资源: 30
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

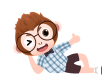
最新资源
- 基于Spring Boot + Vue 3.2 + Vite 4.3开发的前后端分离博客项目源代码.zip
- Go语言基础知识到高级应用全面解析
- 毕业设计前后端分离博客项目源代码.zip
- E008 库洛米(3页).zip
- 前端拿到的列表数据里id都一样的处理办法.txt
- 批量导出多项目核心目录工具
- 课程设计前后端分离博客项目源代码.zip
- C#语言教程:面向对象与高级编程技术全面指南
- PHP语言详细教程:从基础到实战
- 电影管理系统,数据库系统概论大作业
- Web开发中JavaScript编程语言的全面解析
- ajax发请求示例.txt
- 企业数据管理系统项目源代码.zip
- 计算机技术-JAVA语言介绍-基本语法(上)
- 基于西门子 PLC 的晶圆研磨机自动控制系统设计与实现-论文
- 家庭理财系统源代码+答辩PPT+论文.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


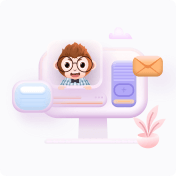
安全验证
文档复制为VIP权益,开通VIP直接复制
