# BinanceDotNet <img src="https://i.imgur.com/x2YPVe6.png" width="56" />
## C# Wrapper for the official Binance exchange API
<img src="https://img.shields.io/nuget/dt/BinanceDotNet.svg" />
<img src="https://img.shields.io/nuget/v/BinanceDotNet.svg" />
Compatible with **.NET 4.5.1, .NET 4.5.2, .NET 4.6.1, .NETSTANDARD2.0**
This repository provides a C# wrapper for the official Binance API, and provides rate limiting features _(set to 10 by 10 out the box)_, a `IAPICacheManager` interface to allow users to provide their own cache implementations, all `REST` endpoints covered, and a best practice solution coupled with strongly typed responses and requests. It is built on the latest .NET Framework and in .NET Core
Feel free to raise issues and Pull Request to help improve the library. If you found this API useful, and you wanted to give back feel free to sign up to Binance via my referral link [**here**](https://www.binance.com/?ref=10886925).
## Documentation
- [Binance Clients](/docs/BINANCE-CLIENTS.md)
- [REST API Calls](/docs/REST-API.md)
- [WebSocket API Calls](/docs/WEBSOCKET-API.md)
## Installation
The package is available in NuGet, or feel free to download:
https://www.nuget.org/packages/BinanceDotNet/
**Nuget PM**
```
Install-Package BinanceDotNet
```
**dotnet cli**
```
dotnet add package BinanceDotNet
```
## Donations and Contribution
Upkeep of this API takes a lot of time from answering issues and PR's on the Repository, as well as tweets and direct emails.
You can donate cryptocurrency of any amount to the following wallets:
```
ETH: 0xd5775e2dee4b9fa9a3be5222970c04ac574e8412
```
_Want to send something else? Just tweet me! @Glitch100_
Or you can help maintain the repository! Donations of time are welcome, just hit me up and we can work on it. From answering issues, to contributing code anyone can assist.
```git
git clone git@github.com:glitch100/BinanceDotNet.git
```
- Navigate to `ExampleProgram.cs`
- Add your own Private and Secret keys
- Play around with the API
## Features
- Simple, Configurable, Extendable
- Rate limiting, with 10 requests in 10 seconds _(disabled by default)_
- `log4net` Interfaces supported
- dotnet standard, dotnet core, 4.5.1, 4.6.1 support
- Binance WebSockets
- Unit test coverage (_in progress_)
- `IAPICacheManager` abstraction for providing your own cache or using the build in concrete implementation. _(Currently only one endpoint has caching)_
- Console app with examples ready to launch _(provide API keys)_
## Examples
More examples are available to play around with within the repositorys Console application which can be found [here](/BinanceExchange.Console/ExampleProgram.cs). Otherwise there are some examples around utilising both `WebSockets` and `REST` API in the `Usage` section below.
## Roadmap
Work will continue on this API wrapper over the coming months adding and extending out the number of features that the `BinanceDotNet` library has. Please raise issues for new features desired
- Start building out Unit Test support - >~2.1.0
- Provide Builder support for queries - 2.5.0~
- Abstract out the HttpClient - 3.0.0~
## Usage
Code examples below, or clone the repository and run the `BinanceExchange.Console` project.
**This repository is built off dotnet core, and runs against C# 7.1**
### Creating a Client
General usage just requires setting up the client with your credentials, and then calling the Client as necessary.
```c#
// Build out a client, provide a logger, and more configuration options, or even your own APIProcessor implementation
var client = new BinanceClient(new ClientConfiguration()
{
ApiKey = "YOUR_API_KEY",
SecretKey = "YOUR_SECRET_KEY",
});
//You an also specify symbols like this.
var desiredSymbol = TradingPairSymbols.BNBPairs.BNB_BTC,
IReponse response = await client.GetCompressedAggregateTrades(new GetCompressedAggregateTradesRequest(){
Symbol = "BNBBTC",
StartTime = DateTime.UtcNow().AddDays(-1),
EndTime = Date.UtcNow(),
});
```
### Creating a WebSocket Client
For WebSocket endpoints, just instantiate the `BinanceClient`, and provide it into the `BinanceWebSocketClient`
You can use a `using` block or manual management.
```c#
var client = new BinanceClient(new ClientConfiguration()
{
ApiKey = "YOUR_API_KEY",
SecretKey = "YOUR_SECRET_KEY",
});
// Manual management
var manualWebSocketClient = new InstanceBinanceWebSocketClient(client);
var socketId = binanceWebSocketClient.ConnectToDepthWebSocket("ETHBTC", data =>
{
System.Console.WriteLine($"DepthCall: {JsonConvert.SerializeObject(data)}");
});
manualWebSocketClient.CloseWebSocketInstance(socketId);
// Disposable and managed
using (var binanceWebSocketClient = new DisposableBinanceWebSocketClient(client))
{
binanceWebSocketClient.ConnectToDepthWebSocket("ETHBTC", data =>
{
System.Console.WriteLine($"DepthCall: {JsonConvert.SerializeObject(data)}");
});
Thread.Sleep(180000);
}
```
### Error Handling
The Binance API provides rich exceptions based on different error types. You can decorate calls like this if you would like to handle the various exceptions.
```c#
// Firing off a request and catching all the different exception types.
try
{
accountTrades = await client.GetAccountTrades(new AllTradesRequest()
{
FromId = 352262,
Symbol = "ETHBTC",
});
}
catch (BinanceBadRequestException badRequestException)
{
}
catch (BinanceServerException serverException)
{
}
catch (BinanceTimeoutException timeoutException)
{
}
catch (BinanceException unknownException)
{
}
```
### Building out a local cache per symbol from the depth WebSocket
The example is mainly 'all in one' so you can see a full runthrough of how it works. In your own implementations you may want to have a cache of only the most recent bids/asks, or perhaps will want the empty quanity/price trades.
You can also calculate volume and more from this cache. The following code is _partial_ from the `ExampleProgram.cs`.
```c#
private static async Task<Dictionary<string, DepthCacheObject>> BuildLocalDepthCache(IBinanceClient client)
{
// Code example of building out a Dictionary local cache for a symbol using deltas from the WebSocket
var localDepthCache = new Dictionary<string, DepthCacheObject> {{ "BNBBTC", new DepthCacheObject()
{
Asks = new Dictionary<decimal, decimal>(),
Bids = new Dictionary<decimal, decimal>(),
}}};
var bnbBtcDepthCache = localDepthCache["BNBBTC"];
// Get Order Book, and use Cache
var depthResults = await client.GetOrderBook("BNBBTC", true, 100);
//Populate our depth cache
depthResults.Asks.ForEach(a =>
{
if (a.Quantity != 0.00000000M)
{
bnbBtcDepthCache.Asks.Add(a.Price, a.Quantity);
}
});
depthResults.Bids.ForEach(a =>
{
if (a.Quantity != 0.00000000M)
{
bnbBtcDepthCache.Bids.Add(a.Price, a.Quantity);
}
});
// Store the last update from our result set;
long lastUpdateId = depthResults.LastUpdateId;
using (var binanceWebSocketClient = new DisposableBinanceWebSocketClient(client))
{
binanceWebSocketClient.ConnectToDepthWebSocket("BNBBTC", data =>
{
if (lastUpdateId < data.UpdateId)
{
data.BidDepthDeltas.ForEach((bd) =>
{
CorrectlyUpdateDepthCache(bd, bnbBtcDepthCache.Bids);
});
data.AskDepthDeltas.ForEach((ad) =>
{
CorrectlyUpdateDepthCache(ad, bnbBtcDepthCache.Asks);
});
}
lastUpdateId = data.UpdateId;
System.Console.Clear();
System.Console.WriteLine($"{JsonConvert.SerializeObject(bnbBtcDepthCache, Formatting.Indented)}");
System.Console.SetWindowPosition(0, 0);
});
Thread.Sleep(8000);
}
return localDepthCache;
}
```
### Result Transformations
You
没有合适的资源?快使用搜索试试~ 我知道了~
BinanceDotNet:带有REST和WebSocket端点的Binance交换API的官方C#包装器
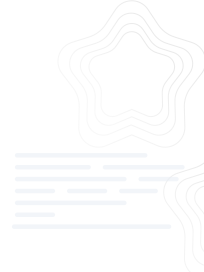
共154个文件
cs:139个
md:8个
csproj:3个


温馨提示
BinanceDotNet 官方Binance交换API的C#包装器 与.NET 4.5.1,.NET 4.5.2,.NET 4.6.1,.NETSTANDARD2.0兼容 该存储库为官方Binance API提供了C#包装,并提供了速率限制功能(开箱即用10设置为10) ,允许用户提供自己的缓存实现的IAPICacheManager接口,涵盖了所有REST端点以及最佳实践解决方案以及强类型的响应和请求。 它基于最新的.NET Framework和.NET Core构建 随时提出问题,并请求请求来改进图书馆。 如果你发现这个API有用的,你希望自己能回馈随时通过我的推荐链接注册到Binanc
资源推荐
资源详情
资源评论
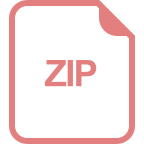
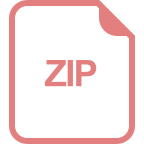
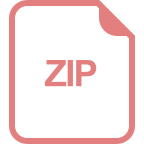
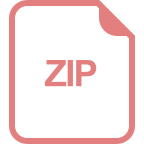
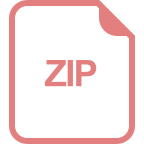
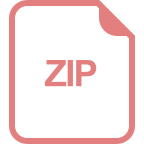
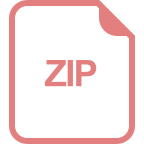
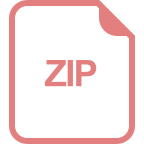
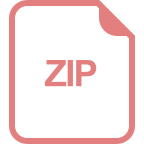
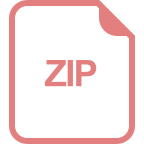
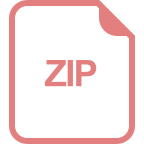
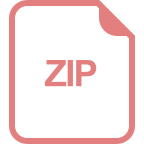
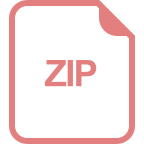
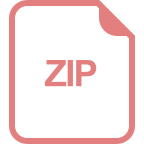
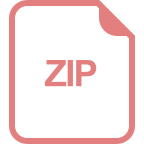
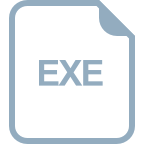
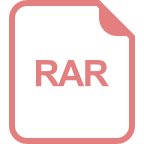
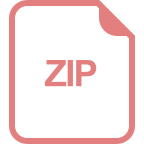
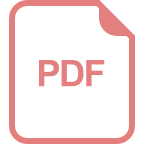
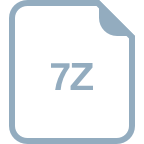
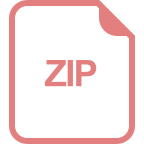
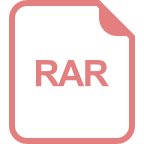
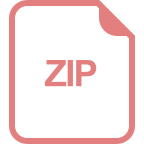
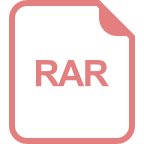
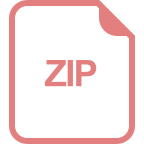
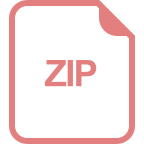
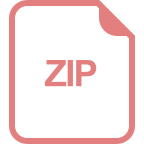
收起资源包目录

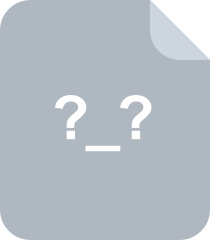
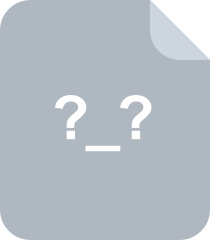
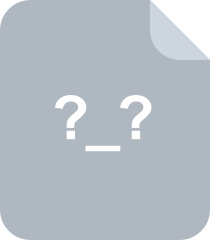
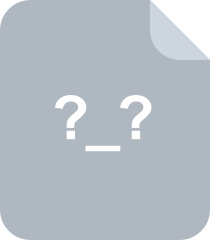
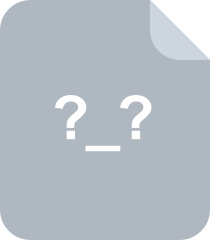
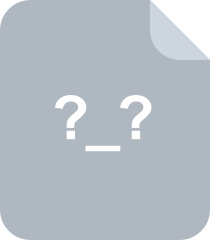
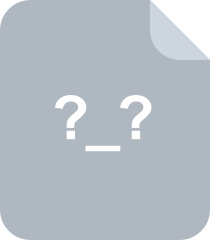
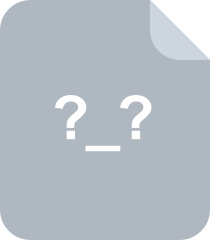
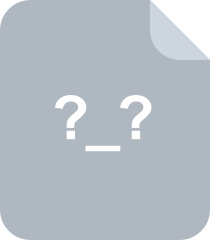
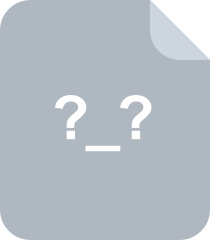
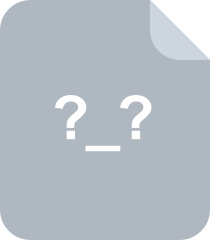
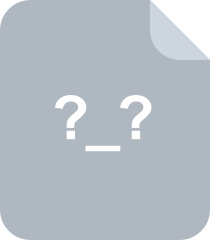
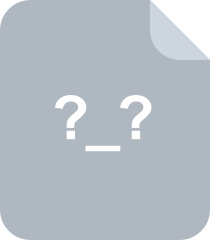
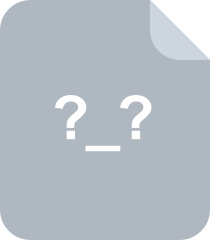
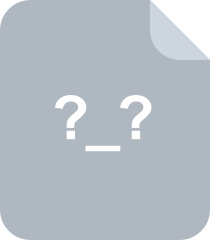
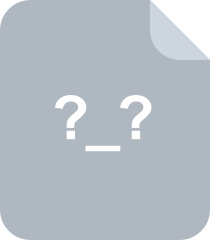
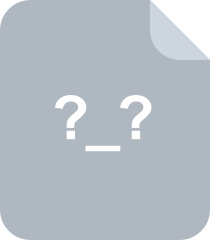
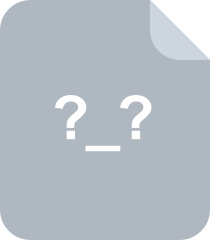
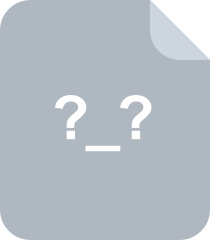
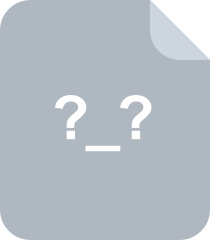
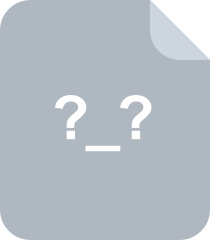
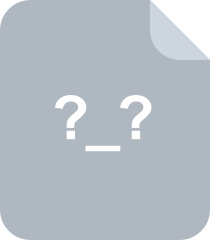
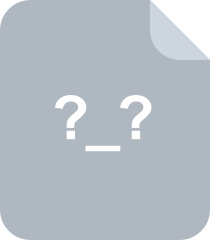
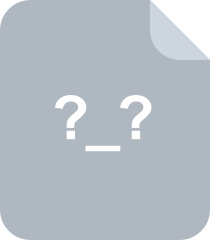
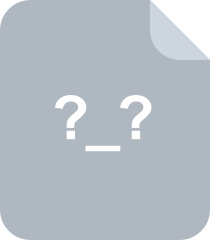
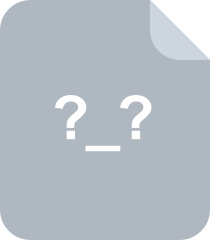
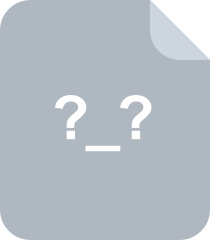
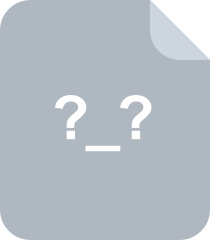
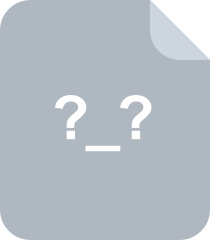
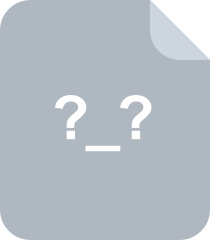
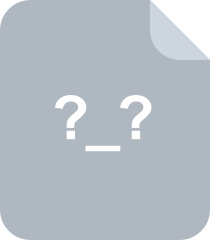
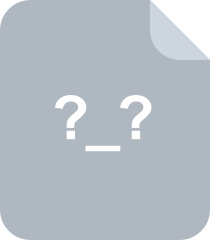
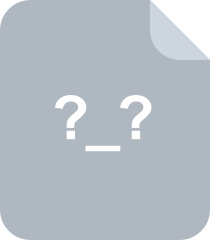
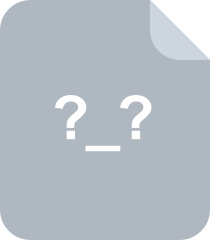
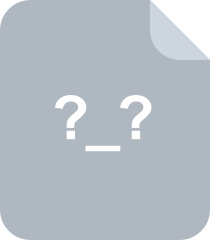
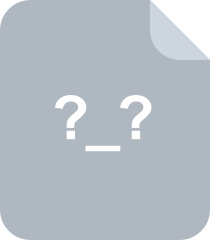
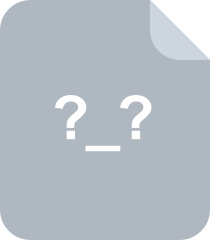
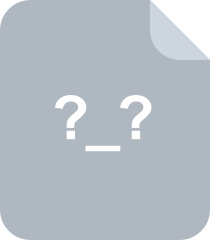
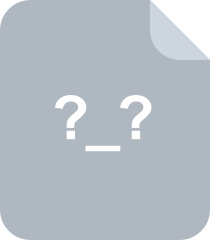
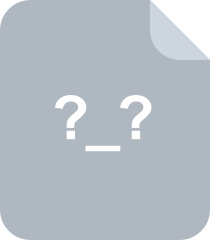
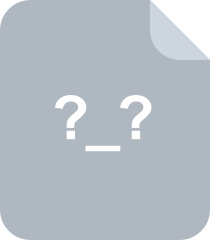
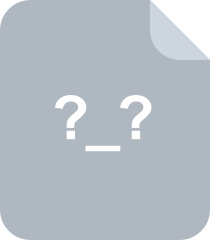
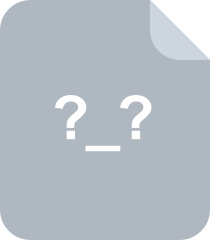
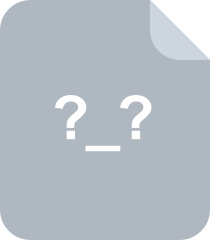
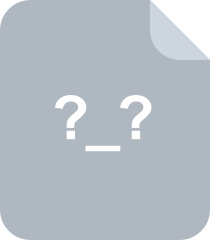
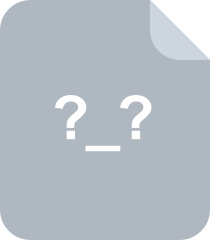
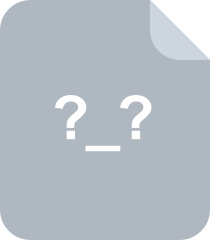
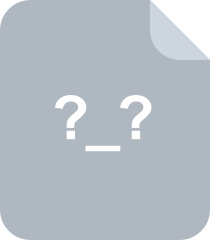
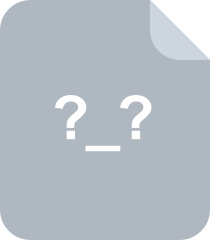
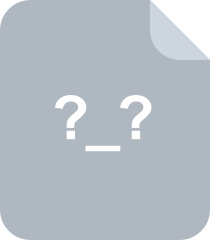
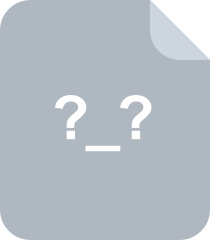
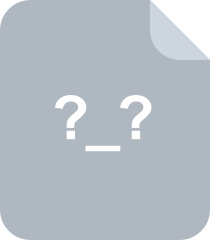
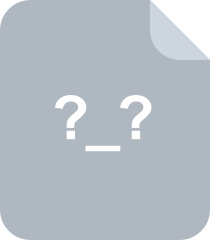
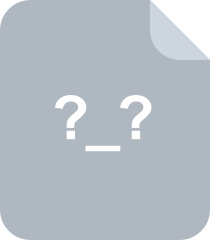
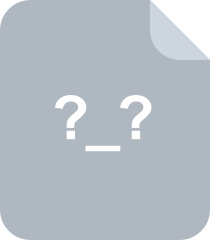
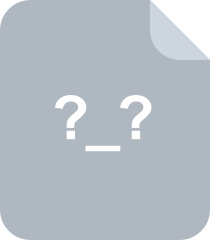
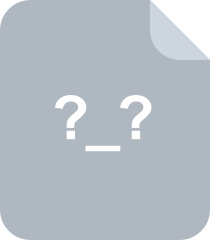
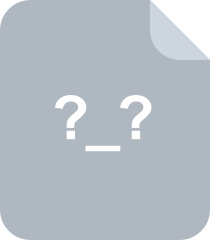
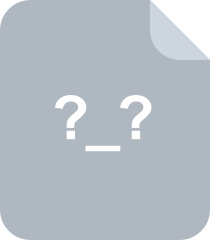
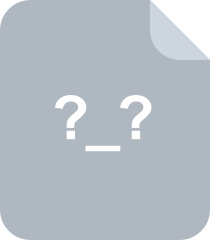
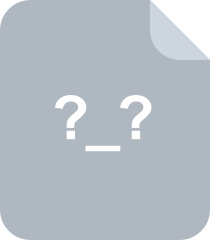
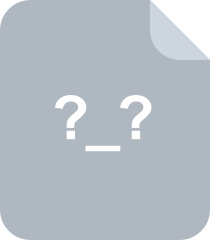
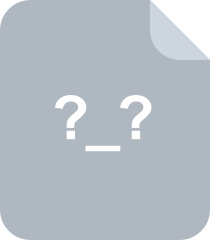
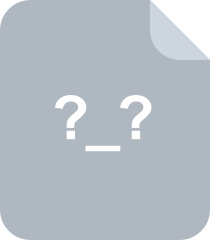
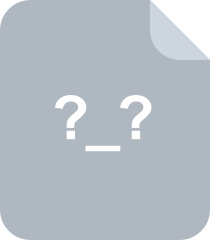
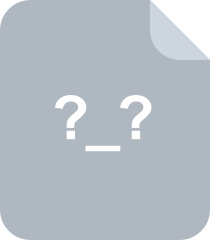
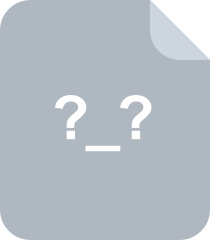
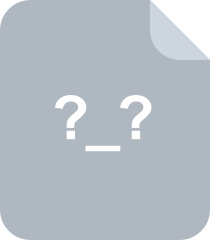
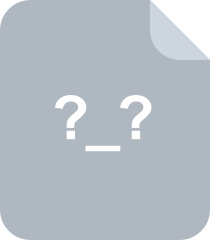
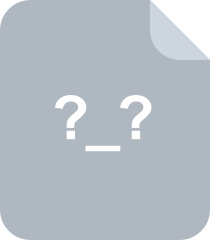
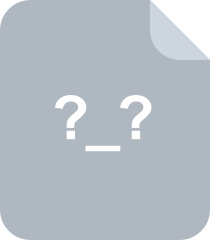
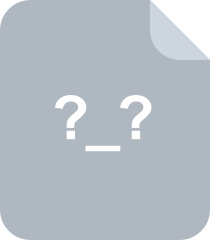
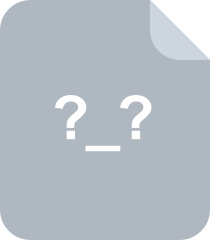
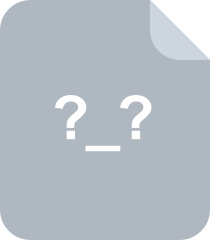
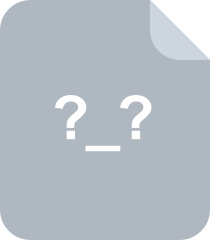
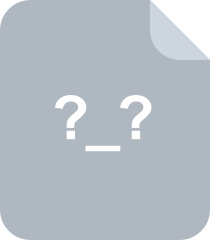
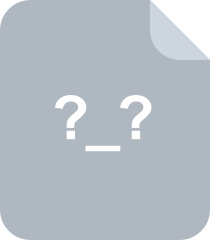
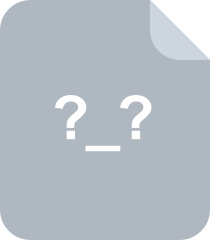
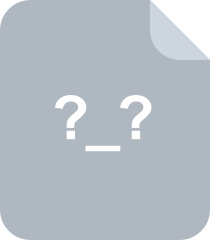
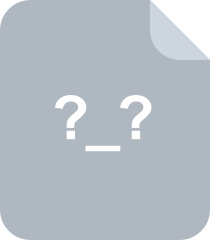
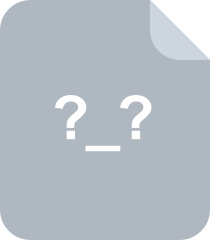
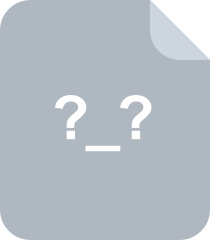
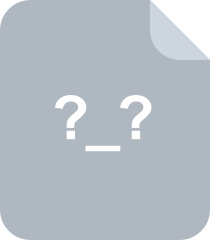
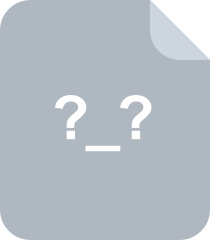
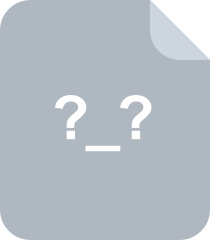
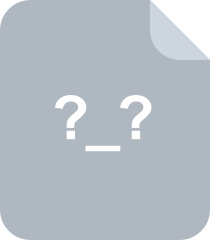
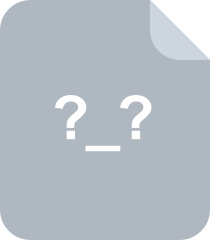
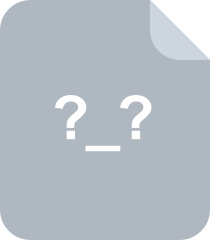
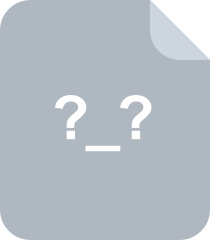
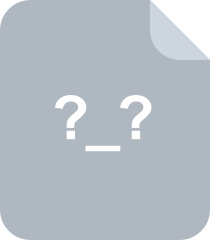
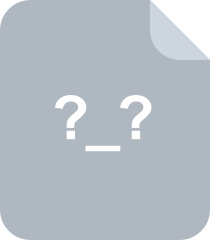
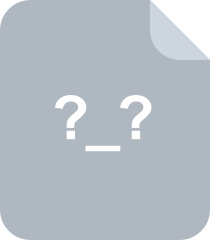
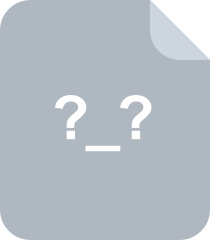
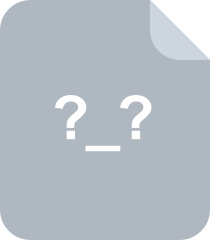
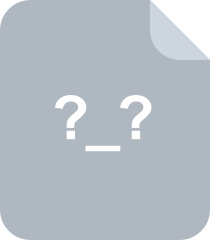
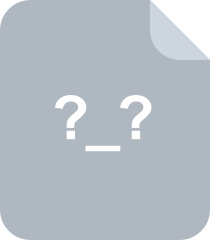
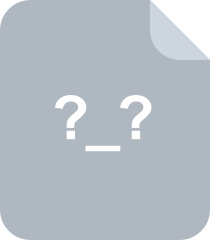
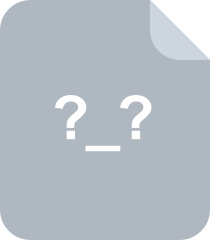
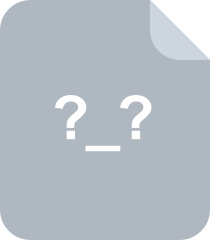
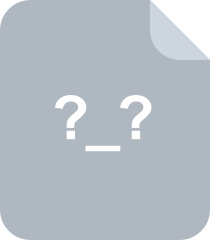
共 154 条
- 1
- 2
资源评论
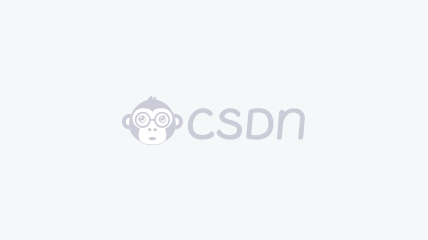
- game1382021-08-09骗子。2019年的代码重新上传一次就卖钱?看时间还以为是最新的。官方不管管?3年前的代码你们觉得还有什么用?

李念遠
- 粉丝: 19
- 资源: 4615
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

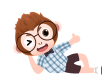
最新资源
- 【Python】基于话题相似度的夸夸机器人.zip
- 【机器人】将ChatGPT飞书机器人钉钉机器人企业微信机器人公众号部署到vercel及docker.zip
- 【java】用mirai机器人搜索音乐并以卡片的形式分享.zip
- 【爬虫】vue2聊天室,图灵机器人,node爬虫.zip
- 【Python爬虫】基于Python的淘宝千牛店铺上下架商品.zip
- 【Python】基于Python的美篇高清图片爬虫.zip
- MATLAB 风力发电系统低电压穿越-串电阻策略 低电压穿越 双馈风力发电机
- 【Python】基于Python爬虫爬取牛津三千词并导入到Anki方便背诵.zip
- 【Python】Python爬虫实战--小猪短租爬虫.zip
- 【Python学习】Python爬虫学习、总结、收集.zip
- 【PHP】一个PHP写的telegram机器人.zip
- 【Python爬虫】基于Python实现基本的网页爬虫.zip
- 【java】Java课程大项目-消息中间件比赛(实现进程内消息队列).zip
- 【C#】基于C#的消息队列服务产品中间件.zip
- 【机器人】基于code hijack和code injection极简微信机器人.zip
- 【Python】简明饭否机器人教程(使用Python).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


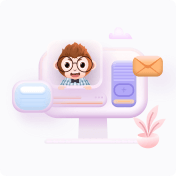
安全验证
文档复制为VIP权益,开通VIP直接复制
