# String & slice utility library for Solidity
## Overview
Functionality in this library is largely implemented using an abstraction called a 'slice'. A slice represents a part of a string - anything from the entire string to a single character, or even no characters at all (a 0-length slice). Since a slice only has to specify an offset and a length, copying and manipulating slices is a lot less expensive than copying and manipulating the strings they reference.
To further reduce gas costs, most functions on slice that need to return a slice modify the original one instead of allocating a new one; for instance, `s.split(".")` will return the text up to the first '.', modifying s to only contain the remainder of the string after the '.'. In situations where you do not want to modify the original slice, you can make a copy first with `.copy()`, for example: `s.copy().split(".")`. Try and avoid using this idiom in loops; since Solidity has no memory management, it will result in allocating many short-lived slices that are later discarded.
Functions that return two slices come in two versions: a non-allocating version that takes the second slice as an argument, modifying it in place, and an allocating version that allocates and returns the second slice; see `nextRune` for example.
Functions that have to copy string data will return strings rather than slices; these can be cast back to slices for further processing if required.
## Examples
### Basic usage
import "github.com/Arachnid/solidity-stringutils/strings.sol";
contract Contract {
using strings for *;
// ...
}
### Getting the character length of a string
var len = "Unicode snowman ☃".toSlice().len(); // 17
### Splitting a string around a delimiter
var s = "foo bar baz".toSlice();
var foo = s.split(" ".toSlice());
After the above code executes, `s` is now "bar baz", and `foo` is now "foo".
### Splitting a string into an array
var s = "www.google.com".toSlice();
var delim = ".".toSlice();
var parts = new string[](s.count(delim) + 1);
for(uint i = 0; i < parts.length; i++) {
parts[i] = s.split(delim).toString();
}
### Extracting the middle part of a string
var s = "www.google.com".toSlice();
strings.slice memory part;
s.split(".".toSlice(), part); // part and return value is "www"
s.split(".".toSlice(), part); // part and return value is "google"
This approach uses less memory than the above, by reusing the slice `part` for each section of string extracted.
### Converting a slice back to a string
var myString = mySlice.toString();
### Finding and returning the first occurrence of a substring
var s = "A B C B D".toSlice();
s.find("B".toSlice()); // "B C B D"
`find` modifies `s` to contain the part of the string from the first match onwards.
### Finding and returning the last occurrence of a substring
var s = "A B C B D".toSlice();
s.rfind("B".toSlice()); // "A B C B"
`rfind` modifies `s` to contain the part of the string from the last match back to the start.
### Finding without modifying the original slice.
var s = "A B C B D".toSlice();
var substring = s.copy().rfind("B".toSlice()); // "A B C B"
`copy` lets you cheaply duplicate a slice so you don't modify the original.
### Prefix and suffix matching
var s = "A B C B D".toSlice();
s.startsWith("A".toSlice()); // True
s.endsWith("D".toSlice()); // True
s.startsWith("B".toSlice()); // False
### Removing a prefix or suffix
var s = "A B C B D".toSlice();
s.beyond("A ".toSlice()).until(" D".toSlice()); // "B C B"
`beyond` modifies `s` to contain the text after its argument; `until` modifies `s` to contain the text up to its argument. If the argument isn't found, `s` is unmodified.
### Finding and returning the string up to the first match
var s = "A B C B D".toSlice();
var needle = "B".toSlice();
var substring = s.until(s.copy().find(needle).beyond(needle));
Calling `find` on a copy of `s` returns the part of the string from `needle` onwards; calling `.beyond(needle)` removes `needle` as a prefix, and finally calling `s.until()` removes the entire end of the string, leaving everything up to and including the first match.
### Concatenating strings
var s = "abc".toSlice().concat("def".toSlice()); // "abcdef"
## Reference
### toSlice(string self) internal returns (slice)
Returns a slice containing the entire string.
Arguments:
- self The string to make a slice from.
Returns A newly allocated slice containing the entire string.
### copy(slice self) internal returns (slice)
Returns a new slice containing the same data as the current slice.
Arguments:
- self The slice to copy.
Returns A new slice containing the same data as `self`.
### toString(slice self) internal returns (string)
Copies a slice to a new string.
Arguments:
- self The slice to copy.
Returns A newly allocated string containing the slice's text.
### len(slice self) internal returns (uint)
Returns the length in runes of the slice. Note that this operation takes time proportional to the length of the slice; avoid using it in loops, and call `slice.empty()` if you only need to know whether the slice is empty or not.
Arguments:
- self The slice to operate on.
Returns The length of the slice in runes.
### empty(slice self) internal returns (bool)
Returns true if the slice is empty (has a length of 0).
Arguments:
- self The slice to operate on.
Returns True if the slice is empty, False otherwise.
### compare(slice self, slice other) internal returns (int)
Returns a positive number if `other` comes lexicographically after `self`, a negative number if it comes before, or zero if the contents of the two slices are equal. Comparison is done per-rune, on unicode codepoints.
Arguments:
- self The first slice to compare.
- other The second slice to compare.
Returns The result of the comparison.
### equals(slice self, slice other) internal returns (bool)
Returns true if the two slices contain the same text.
Arguments:
- self The first slice to compare.
- self The second slice to compare.
Returns True if the slices are equal, false otherwise.
### nextRune(slice self, slice rune) internal returns (slice)
Extracts the first rune in the slice into `rune`, advancing the slice to point to the next rune and returning `self`.
Arguments:
- self The slice to operate on.
- rune The slice that will contain the first rune.
Returns `rune`.
### nextRune(slice self) internal returns (slice ret)
Returns the first rune in the slice, advancing the slice to point to the next rune.
Arguments:
- self The slice to operate on.
Returns A slice containing only the first rune from `self`.
### ord(slice self) internal returns (uint ret)
Returns the number of the first codepoint in the slice.
Arguments:
- self The slice to operate on.
Returns The number of the first codepoint in the slice.
### keccak(slice self) internal returns (bytes32 ret)
Returns the keccak-256 hash of the slice.
Arguments:
- self The slice to hash.
Returns The hash of the slice.
### startsWith(slice self, slice needle) internal returns (bool)
Returns true if `self` starts with `needle`.
Arguments:
- self The slice to operate on.
- needle The slice to search for.
Returns True if the slice starts with the provided text, false otherwise.
### beyond(slice self, slice needle) internal returns (slice)
If `self` starts with `needle`, `needle` is removed from the beginning of `self`. Otherwise, `self` is unmodified.
Arguments:
- self The slice to operate on.
- needle The slice to search for.
Returns `self`
### endsWith(slice self, slice needle) internal returns (bool)
Returns true if the slice ends with `needle`.
Arguments:
- self The slice to operate on.
- needle The slice to search for.
Returns True if the
没有合适的资源?快使用搜索试试~ 我知道了~
solidity-stringutils:用于Solidity的基本字符串实用程序
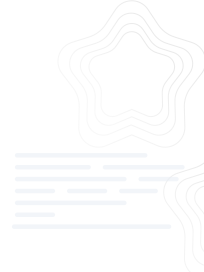
共11个文件
sol:3个
gitignore:1个
md:1个


温馨提示
用于Solidity的String&Slice实用程序库 概述 该库中的功能主要使用称为“切片”的抽象来实现。 切片代表字符串的一部分-从整个字符串到单个字符,甚至根本没有任何字符(长度为0的切片)。 由于切片只需指定偏移量和长度,因此复制和处理切片比复制和处理它们引用的字符串要便宜得多。 为了进一步降低天然气成本,切片上需要返回切片的大多数功能都修改了原来的功能,而不是分配新的功能。 例如, s.split(".")将返回直到第一个'。'的文本,将s修改为仅包含'。'之后的字符串的其余部分。 在您不想修改原始切片的情况下,可以首先使用.copy()进行复制,例如: s.copy().split(".") 。 尝试避免在循环中使用此惯用法; 由于Solidity没有内存管理,因此将导致分配许多短命的切片,这些切片随后将被丢弃。 返回两个切片的函数有两种版本:非分配版本,它以第二个切片为
资源推荐
资源详情
资源评论
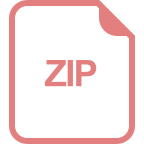
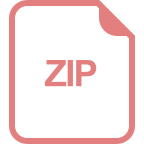
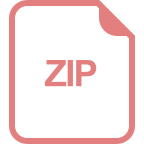
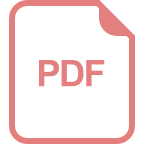
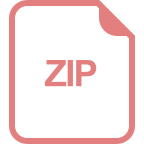
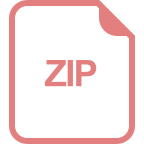
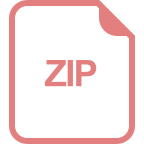
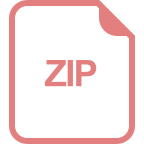
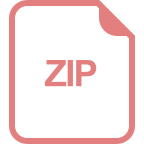
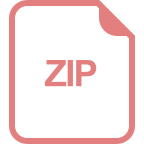
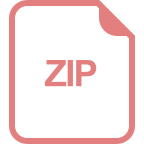
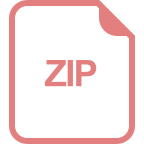
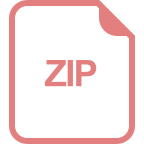
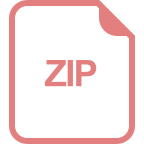
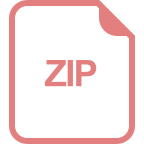
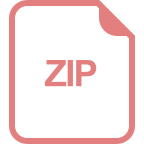
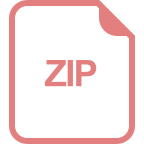
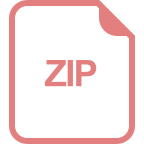
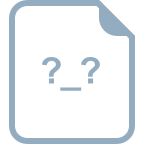
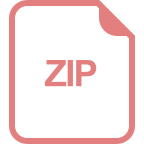
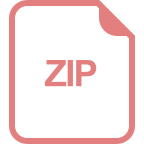
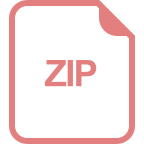
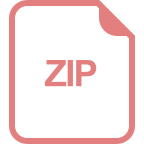
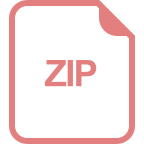
收起资源包目录


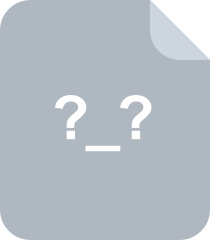
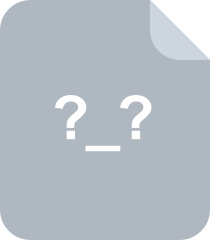

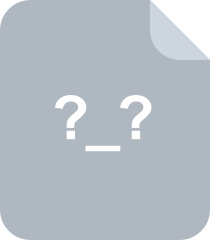
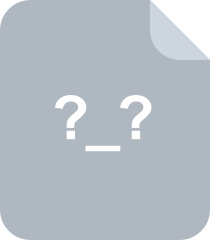


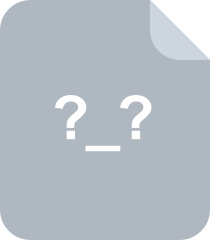
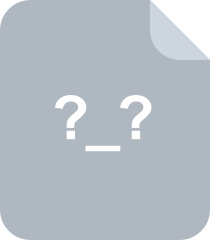
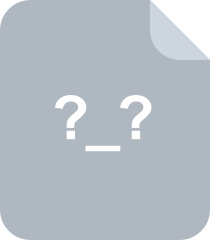
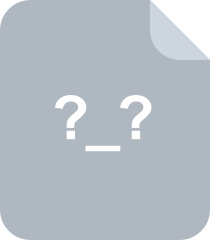
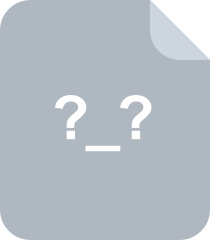
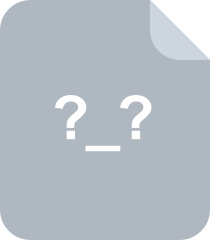
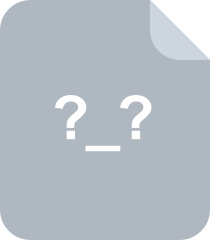
共 11 条
- 1
资源评论
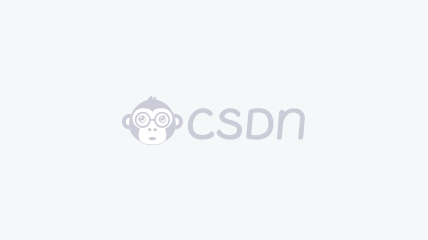
- SeaNico2023-07-24这个文件充分考虑了Solidity语言的特点,提供了一些实用的函数,可以很好地解决字符串相关的问题。
- 村上树树8252023-07-24虽然这个文件只是提供了一些基本的字符串工具,但它确实在处理Solidity中的字符串操作方面非常有帮助。
- 独角兽邹教授2023-07-24它提供了一些基本的字符串工具,可以帮助我们处理字符串的拼接和切割。
- ai2023-07-24使用这个文件可以使我们在Solidity开发中更加高效地处理字符串,节省了不少时间和精力。
- 华亿2023-07-24这个文件提供了一些实用的函数,可以方便地处理Solidity中的字符串操作。

600Dreams
- 粉丝: 19
- 资源: 4629
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

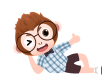
最新资源
- 全球干旱数据集【自校准帕尔默干旱程度指数scPDSI】-190101-202312-0.5x0.5
- 基于Python实现的VAE(变分自编码器)训练算法源代码+使用说明
- 全球干旱数据集【标准化降水蒸发指数SPEI-12】-190101-202312-0.5x0.5
- C语言小游戏-五子棋-详细代码可运行
- 全球干旱数据集【标准化降水蒸发指数SPEI-03】-190101-202312-0.5x0.5
- spring boot aop记录修改前后的值demo
- 全球干旱数据集【标准化降水蒸发指数SPEI-01】-190101-202312-0.5x0.5
- ActiveReports
- vgbvdsbnjkbfnb
- effsefefeffsfwfse
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


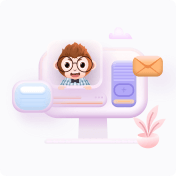
安全验证
文档复制为VIP权益,开通VIP直接复制
