# Training, Classifying (Haar Cascades), and Tracking
Training our own Haar Cascade in OpenCV with Python. A cascade classifier has multiple stages of filtration. Using a sliding window approach, the image region within the window goes through the cascade. Can easily test accuracy of cascade with `classifier.py` script, which takes single images, directory of images, videos, and camera inputs. However, we want to also track our ROI (region of interest). This is because detection (through cascades, etc) is in general, more consuming and computationally taxing. Tracking algorithms are generally considered less taxing, because you know a lot about the apperance of the object in the ROI already. Thus, in the next frame, you use the information from the previous frame to predict and localize where the object is in the frames after.
There are many different types of tracking algorithms that are available through `opencv_contrib/tracking`, such as KCF, MOSSE, TLD, CSRT, etc. Here's a [good video](https://www.youtube.com/watch?v=61QjSz-oLr8) that demonstrates some of these tracking algorithms. Depending on your use case, the one you choose will differ.
## Jump to Section
* [Environment Setup](#environment-setup)
* [Image Scraping](#image-scraping)
* [Postive & Negative Image Sets](#positive-&-negative-image-sets)
* [Positive Samples Image Augmentation](#positive-samples-image-augmentation)
* [Training](#training)
* [Testing Cascade](#testing-cascade)
* [Video Conversions](#video-conversions)
* [Acknowledgements](#acknowledgements)
* [References](#references)
## Environment Setup
* Ubuntu 18.04, 20.04
* OpenCV 4.4.0
* OpenCV Contrib (branch parallel with OpenCV 4.4.0)
I used a Python virtual environment `venv` for package management. You can build and install OpenCV from source in the virtual environment (especially if you want a specific development branch or full control of compile options), or you can use `pip` locally in the `venv`. Packages included are shown in the `requirements.txt` file for reproducing the specific environment.
The project directory tree will look similar to the following below, and might change depending on the arguments passed to the scripts.
```
.
├── classifier.py
├── bin
│ └── createsamples.pl
├── negative_images
│ └── *.jpg / *.png
├── positive_images
│ └── *.jpg / *.png
├── negatives.txt
├── positives.txt
├── requirements.txt
├── samples
│ └── *.vec
├── stage_outputs
│ ├── cascade.xml
│ ├── params.xml
│ └── stage*.xml
├── tools
└── venv
```
## Image Scraping
Go ahead and web scrape relevant negative images for training. Once you have a good amount, filter extensions that aren't `*.jpg` or `*.png` such as `*.gif`. Afterwards, we'll convert all the `*.png` images to `*jpg` using the following command:
```
mogrify -format jpg *.png
```
Then we can delete these `*.png` images. Let's also rename all the images within the directoy to be `img.jpg`
```
ls | cat -n | while read n f; do mv "$f" "img$n.jpg"; done
```
To check if all files within our directory are valid `*.jpg` files:
```
find -name '*.jpg' -exec identify -format "%f" {} \; 1>pass.txt 2>errors.txt
```
## Positive & Negative Image Sets
Positive images correspond to images with detected objects. Images were cropped to 150 x 150 px training set. Negative images are images that are visually close to positive images, but *must not have* any positive image sets within.
<blockquote>
```
/images
img1.png
img2.png
positives.txt
```
</blockquote>
To generate your `*.txt` file, run the following command, make sure to change image extension to whatever file type you're using.
```
find ./positive_images -iname "*.png" > positives.txt
```
As a quote from OpenCV docs:
<blockquote>
Negative samples are taken from arbitrary images. These images must not contain detected objects. [...] Described images may be of different sizes. But each image should be (but not nessesarily) larger then a training window size, because these images are used to subsample negative image to the training size.
</blockquote>
## Positive Samples Image Augmentation
We need to create a whole bunch of image samples, and we'll be using OpenCV to augment these images. To create a training set as a collection of PNG images:
```
opencv_createsamples -img ~/opencv-cascade-tracker/positive_images/img1.png -bg ~/opencv-cascade-tracker/negatives.txt -info ~/opencv-cascade-tracker/annotations/annotations.lst -pngoutput -maxxangle 0.1 -maxyangle 0.1 -maxzangle 0.1
```
But we need a whole bunch of these. To augment a set of positive samples with negative samples, let's run the perl script that Naotoshi Seo wrote:
```
perl bin/createsamples.pl positives.txt negatives.txt samples 1500\
"opencv_createsamples -bgcolor 0 -bgthresh 0 -maxxangle 1.1\
-maxyangle 1.1 maxzangle 0.5 -maxidev 40 -w 50 -h 50"
```
Merge all `*.vec` files into a single `samples.vec` file:
```
python ./tools/mergevec.py -v samples/ -o samples.vec
```
Note: others have said that using artifical data vectors is not the best way to train a classifier. Personally, I have used this method and it worked fine for my use cases. However, you may approach this idea with a grain of salt and skip this step.
## Training
There are two ways in OpenCV to train cascade classifier.
* `opencv_haartraining`
* `opencv_traincascade` - Newer version. Supports both Haar and LBP (Local Binary Patterns)
To begin training using `opencv_traincascade`:
```
opencv_traincascade -data stage_outputs -vec samples.vec -bg negatives.txt\
-numStages 20 -minHitRate 0.999 -maxFalseAlarmRate 0.5 -numPos 390\
-numNeg 600 -w 50 -h 50 -mode ALL -precalcValBufSize 8192\
-precalcIdxBufSize 8192
```
Parameters for tuning `opencv_traincascade` are available in the [documentation](https://docs.opencv.org/4.4.0/dc/d88/tutorial_traincascade.html). `precalcValBufSize` and `precalcIdxBufSize` are buffer sizes. Currently set to 8192 Mb. If you have available memory, tune this parameter as training will be faster.
Something important to note is that
> vec-file has to contain `>= [numPos + (numStages - 1) * (1 - minHitRate) * numPos] + S`, where `S` is a count of samples from vec-file that can be recognized as background right away
`numPos` and `numNeg` are the number of positive and negative samples we use in training for every classifier stage. Therefore, `numPos` should be relatively less than our total number of positive samples, taking into consideration the number of stages we'll be running.
Each row of the training output for each stage represents a feature that's being trained. HR stands for Hit Ratio and FA stands for False Alarm. Note that if a training stage only has a few features (e.g. N = 1 ~ 3), that can suggest that the training data you used was not optimized.
## Testing Cascade
To test how well our cascade performs, run the `classifier.py` script.
```
usage: classifier.py [-h] [-s] [-c] [-i] [-d] [-v] [-w] [-f] [-o] [-z] [-t]
Cascade Classifier
optional arguments:
-h, --help show this help message and exit
-s, --save specify output name
-c, --cas specify specific trained cascade
-i, --img specify image to be classified
-d, --dir specify directory of images to be classified
-v, --vid specify video to be classified
-w, --cam enable camera access for classification
-f, --fps enable frames text (TODO)
-o, --circle enable circle detection
-z, --scale decrease video scale by scale factor
-t, --track select tracking algorithm [KCF, CSRT, MEDIANFLOW]
```
When testing a tracking algorithm, **pass the scale parameter**. For example, to run a video through the classifier and save the output:
```
./classifier.py -v ~/video_input.MOV -s ~/video_output -z 2 -t KCF
```
## Video Conversions
The `classifier.py` automatically saves output videos
没有合适的资源?快使用搜索试试~ 我知道了~
opencv-cascade-tracker:使用Python在OpenCV中训练,检测和跟踪Haar级联
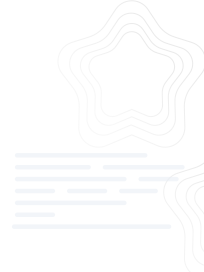
共7个文件
py:2个
license:1个
txt:1个


温馨提示
训练,分类(Haar级联)和跟踪 使用Python在OpenCV中训练我们自己的Haar级联。 级联分类器具有多个过滤阶段。 使用滑动窗口方法,窗口内的图像区域会经过级联。 可以使用classifier.py脚本轻松地测试级联的准确性,该脚本可以获取单个图像,图像目录,视频和摄像机输入。 但是,我们也想跟踪我们的ROI(感兴趣的区域)。 这是因为(通过级联等)检测通常比较耗时且计算量大。 跟踪算法通常被认为不那么费力,因为您已经非常了解对象在ROI中的外观。 因此,在下一帧中,您将使用前一帧中的信息来预测和定位对象在后一帧中的位置。 opencv_contrib/tracking提供了许多不同类型的跟踪算法,例如KCF,MOSSE,TLD,CSRT等。这是一个,演示了其中的一些跟踪算法。 根据您的用例,您选择的将有所不同。 跳到部分 环境设定 Ubuntu 18.04、20.04 Op
资源详情
资源评论
资源推荐
收起资源包目录



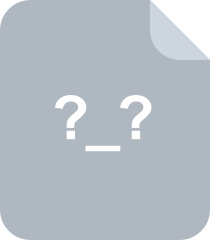

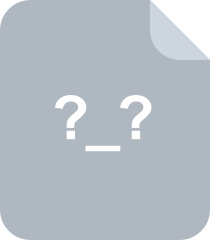
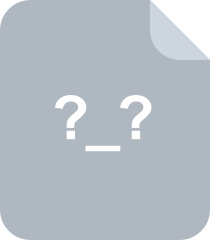
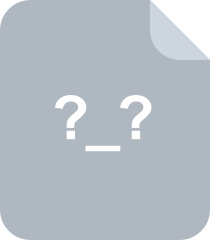
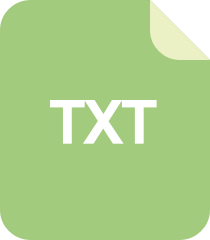
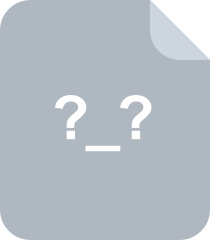
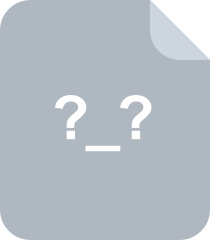
共 7 条
- 1
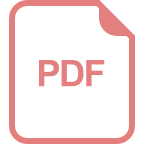
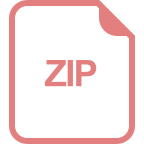
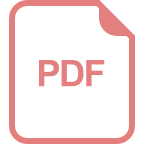
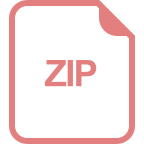
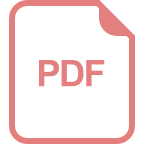
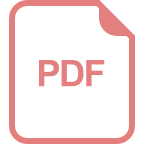
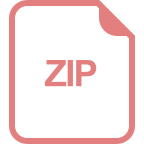
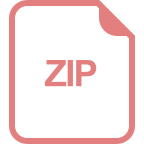
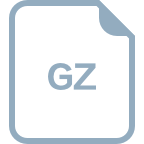
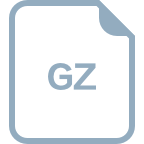
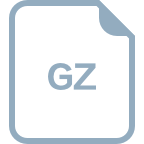
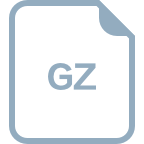
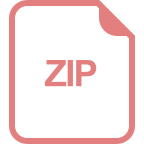
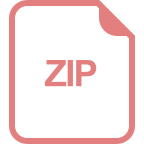
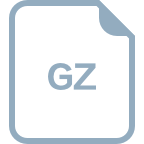
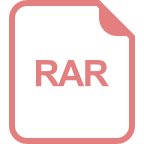
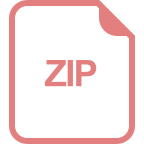
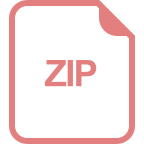
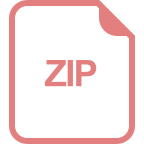
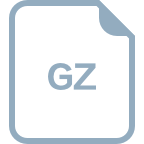
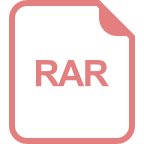
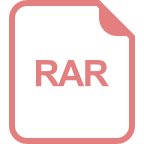
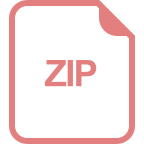
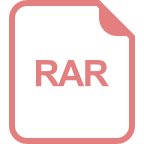

张岱珅
- 粉丝: 50
- 资源: 4689
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

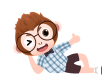
最新资源
- C语言-leetcode题解之83-remove-duplicates-from-sorted-list.c
- C语言-leetcode题解之79-word-search.c
- C语言-leetcode题解之78-subsets.c
- C语言-leetcode题解之75-sort-colors.c
- C语言-leetcode题解之74-search-a-2d-matrix.c
- C语言-leetcode题解之73-set-matrix-zeroes.c
- 树莓派物联网智能家居基础教程
- YOLOv5深度学习目标检测基础教程
- (源码)基于Arduino和Nextion的HMI人机界面系统.zip
- (源码)基于 JavaFX 和 MySQL 的影院管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


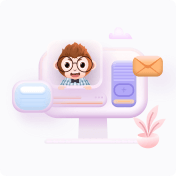
安全验证
文档复制为VIP权益,开通VIP直接复制

评论1