# aeron-go/archive
Implementation of [Aeron Archive](https://github.com/real-logic/Aeron/tree/master/aeron-archive) client in Go.
The [Aeron Archive
protocol](http://github.com/real-logic/aeron/blob/master/aeron-archive/src/main/resources/archive/aeron-archive-codecs.xml)
is specified in xml using the [Simple Binary Encoding (SBE)](https://github.com/real-logic/simple-binary-encoding)
## Current State
The implementation is an alpha release. The API is not yet considered 100% stable.
# Design
## Guidelines
The structure of the archive client library is heavily based on the
Java archive library. It's hoped this will aid comprehension, bug fixing,
feature additions etc.
Many design choices are also based upon the golang client library as
the archive library is a layering on top of that.
Finally golang idioms are used where reasonable.
The archive library does not lock and concurrent calls to archive
library calls that invoke the aeron-archive protocol calls should be
externally locked to ensure only one concuurrent access.
### Naming and other choices
Function names used in archive.go which contains the main API are
based on the Java names so that developers can more easily switch
between langugages and so that any API documentation is more useful
across implementations. Some differences exist due to capitalization
requirements, lack of polymorphism, etc.
Function names used in encoders.go and proxy.go are based on the
protocol specification. Where the protocol specifies a type that cab
ne naturally repreented in golang, the golang type is used used where
possible until encoding. Examples include the use of `bool` rather than
`BooleanType` and `string` over `[]uint8`
## Structure
The archive protocol is largely an RPC mechanism built on top of
Aeron. Each Archive instance has it's own aeron instance running a
[proxy](proxy.go) (publication/request) and [control](control.go) (subscription/response)
pair. This mirrors the Java implementation. The proxy invokes the
encoders to marshal packets using SBE.
Additionally there are some asynchronous events that can arrive on a
[recordingevents](recordingevents.go) subscription. These
are not enabled by default to avoid using resources when not required.
## Synchronous unlocked API optionally using polling
The implementation provides a synchronous API as the underlying
mechanism is largely an RPC mechanism and archive operations are not
considered high frequency.
Associated with this, the library does not lock and assumes management
of reentrancy is handled by the caller.
If needed it is simple in golang to wrap a synchronous API with a
channel (see for example aeron.AddSubscription(). If overlapping
asynchronous calls are needed then this is where you can add locking.
Some asynchronous events do exist (e.g, recording events) and to be
delivered a polling mechanism is provided. Again this can be easily
wrapped in a goroutine if it's desired but ensure there are no other
operations in progress when polling.
## Examples
Examples are provided for a [basic_recording_publisher](examples/basic_recording_publisher/basic_recording_publisher.go) and [basic_replayed_subscriber](examples/basic_replayed_subscriber/basic_replayed_subscriber.go) that interoperate with the Java examples
# Backlog
## Working Set
* [S] [Bug] RecordingSignalEvents currently throw off the count of
fragments/records we want. Need a mechanism to adjust for them.
* [L] Expand testing
* [M] So many tests to write
* [?] archive-media-driver mocking/execution
* test cleanup in the media driver can be problematic
* [S} The archive state is largely unused.
* IsOpen()?
* 10 FIXMEs
* [?] Implement AuthConnect, Challenge/Response
* [?] Add remaining archive protocol packets to proxy, control, archive API, and tests.
## Recently Done
* Logging at level normal should be mostly quiet if nothing goes wrong
* Improve the Error handling / Error listeners (mostly)a
* Ephemeral port usage is dependent upon accessing the counters which is out of scope here and doesn't buy much
* Error listener
* Logging tidying
* Removed the archive context, it was offering little value. Instead,
the proxy, control, and recrodingevents all have a reference
* Made tests a little reliable but cleanup is still a problem
# Bigger picture issues
* Decided not to do locking in sync api, could subsequently add locks, or just async with locks if desired.
It may be that the marshaller should be parameterized for this.
* Java and C++ poll the counters to determine when a recording has actually started but the counters are not
availabe in go. As a result we use delays and hope which isn't ideal.
* OnAvailableCounter noise
* Within aeron-go there are cases of Log.Fatalf(), see for example trying to add a publication on a "bogus" channel.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
aeron-go在Go中实现Aeron消息传递客户端。 可以在此处找到Aeron的体系结构,设计和协议。在此处可以找到用法示例订户。 示例出版物可在此处找到。 aeron-go在Go中实现Aeron消息传递客户端。 可以在此处找到Aeron的体系结构,设计和协议,在此处可以找到用法示例订户。 示例出版物可在此处找到。 通用实例化带有上下文的Aeron:ctx:= aeron.NewContext()。MediaDriverTimeout(time.Second * 10)a:= aeron.Connect(ctx)订阅者创建订阅:subscription:=
资源推荐
资源详情
资源评论
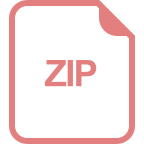
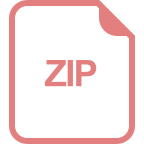
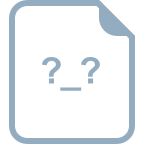
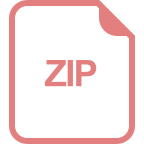
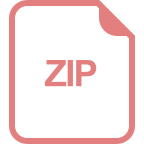
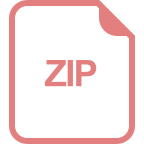
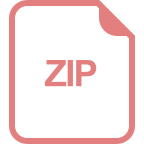
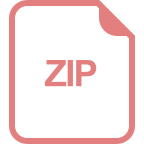
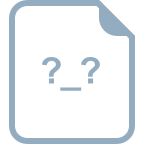
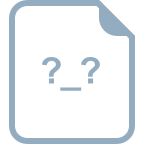
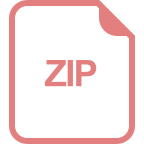
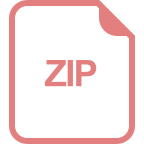
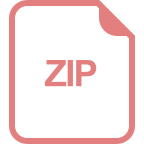
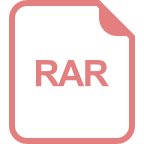
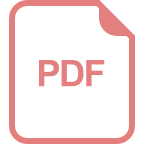
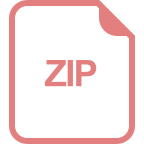
收起资源包目录

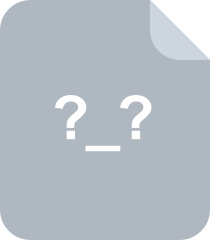
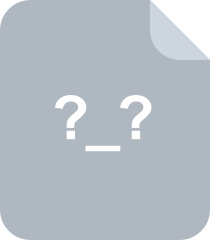
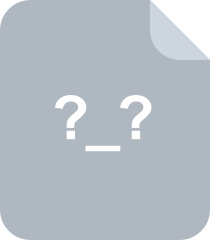
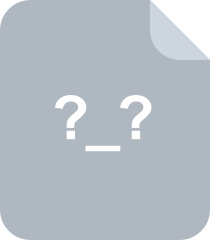
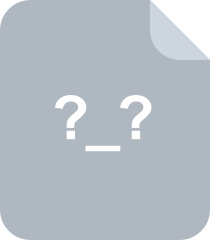
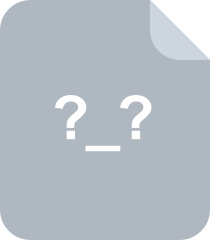
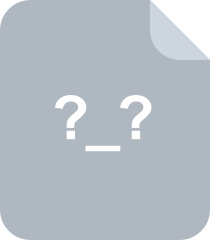
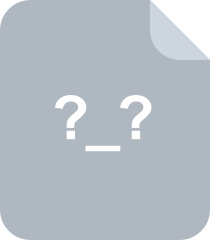
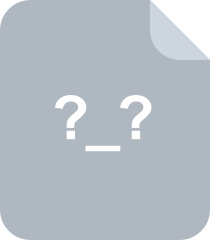
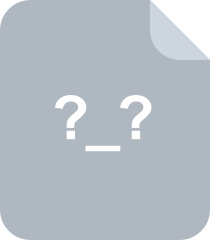
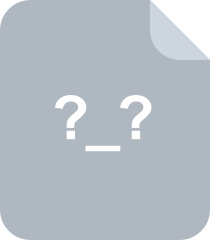
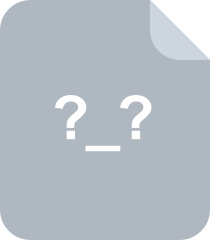
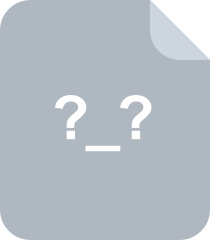
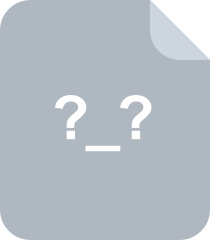
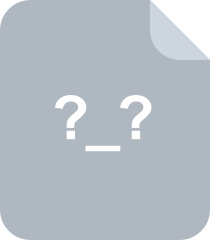
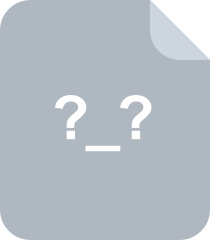
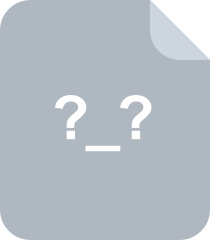
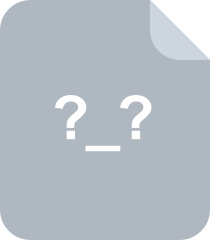
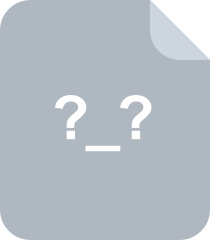
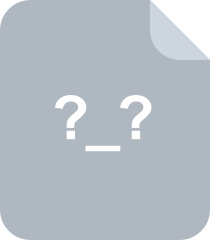
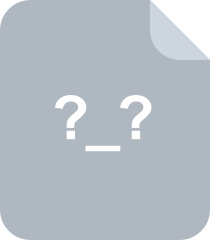
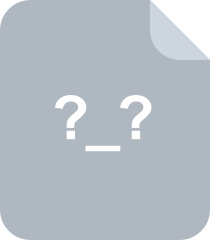
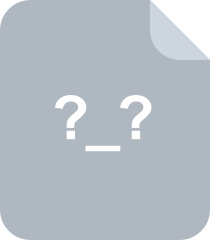
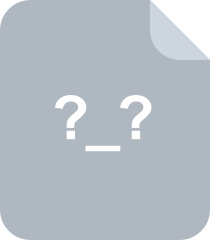
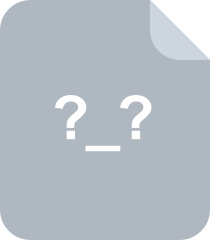
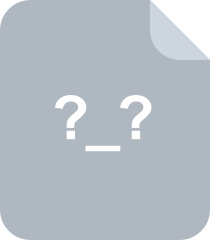
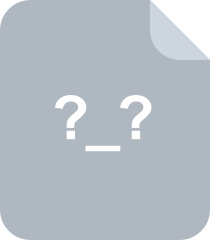
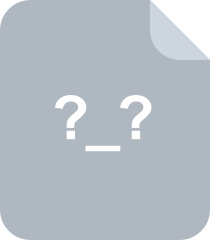
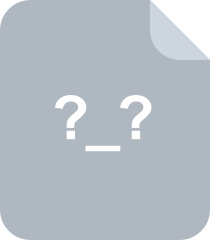
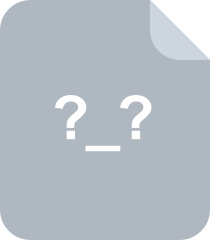
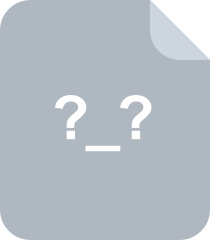
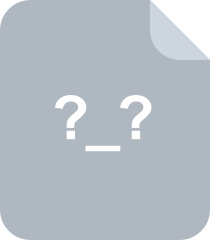
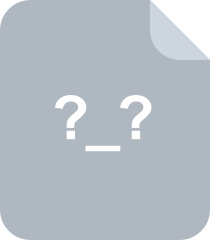
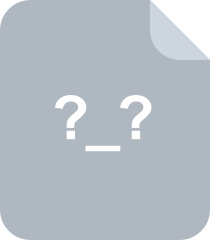
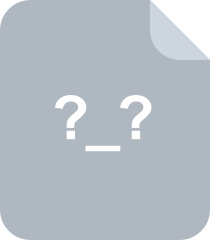
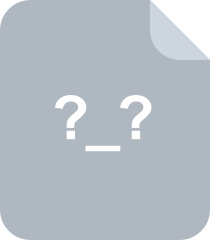
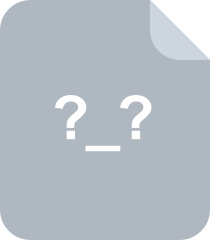
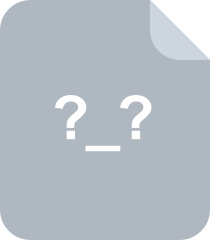
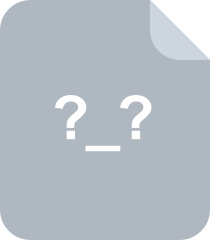
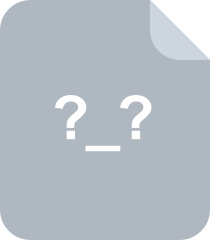
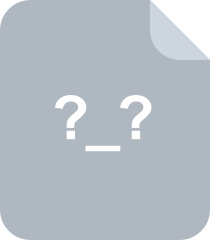
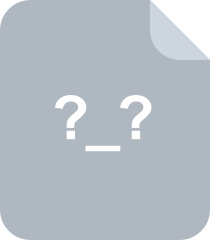
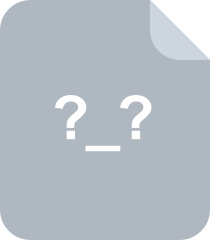
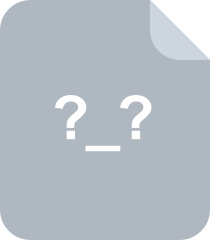
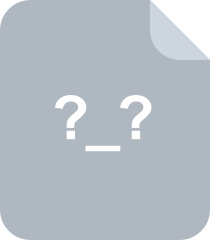
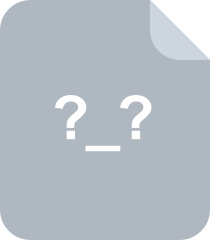
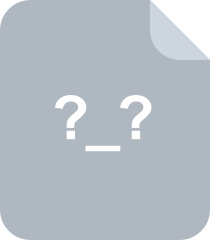
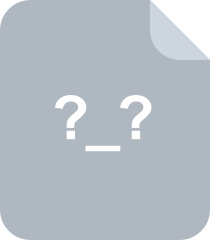
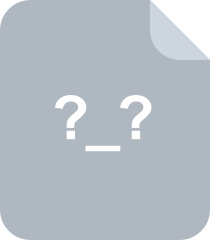
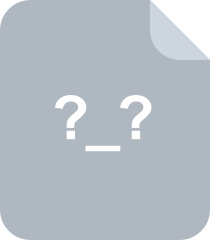
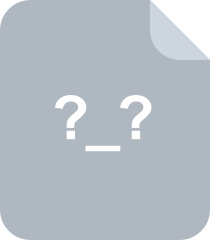
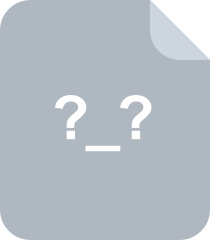
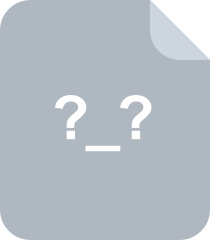
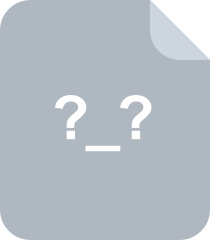
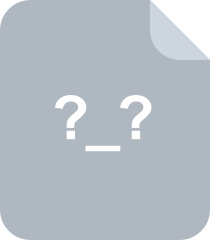
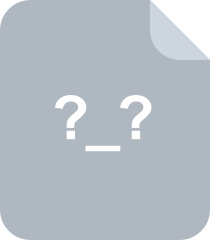
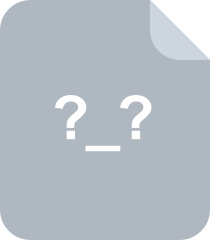
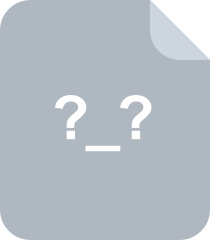
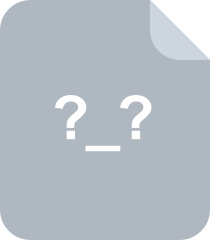
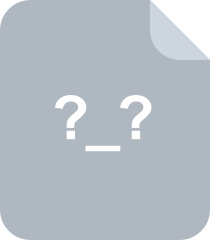
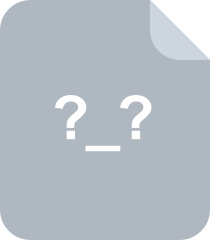
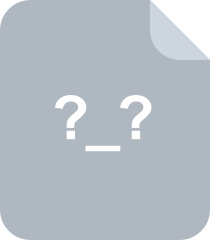
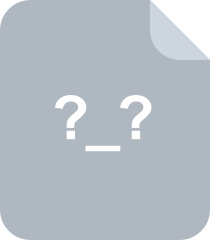
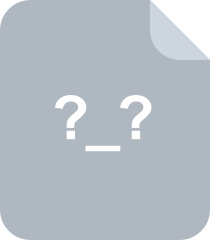
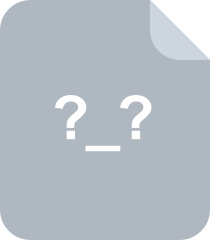
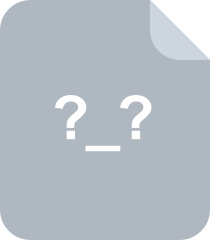
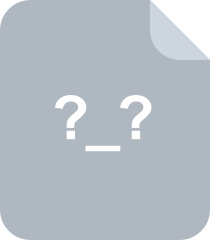
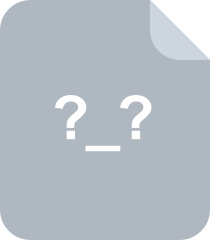
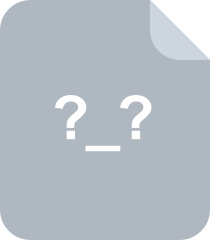
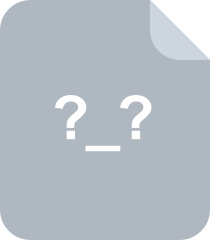
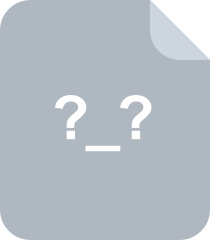
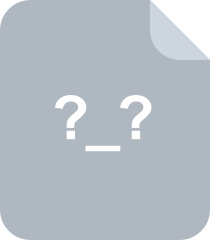
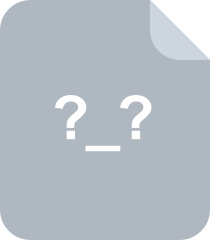
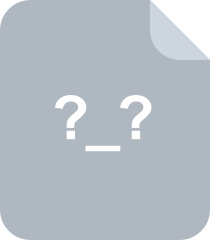
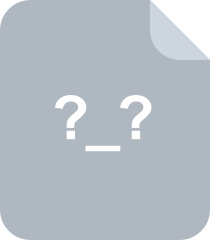
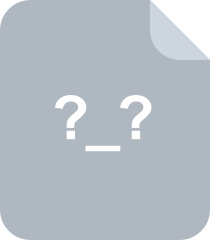
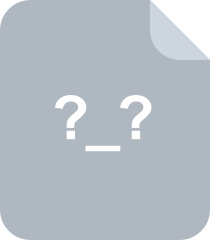
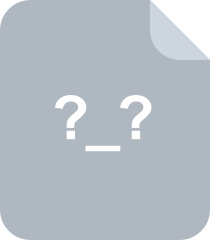
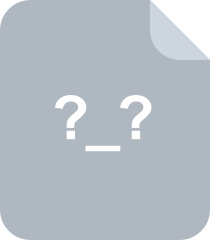
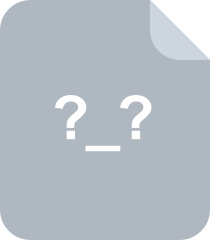
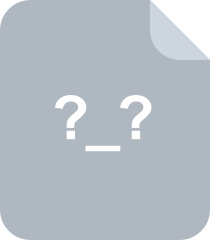
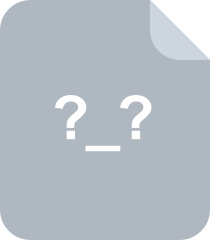
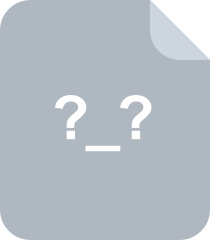
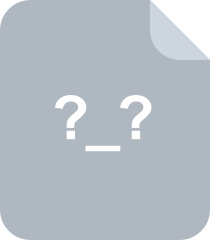
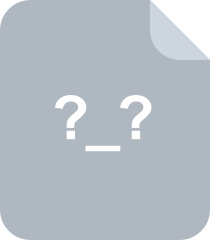
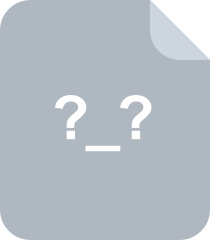
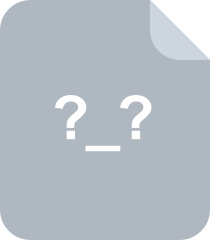
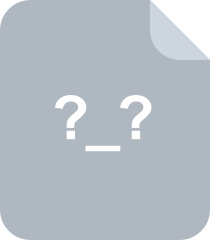
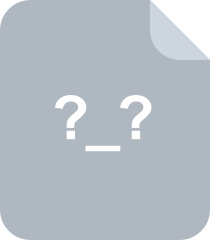
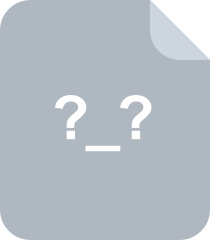
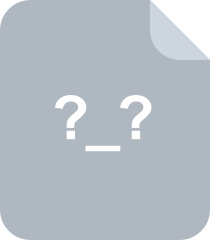
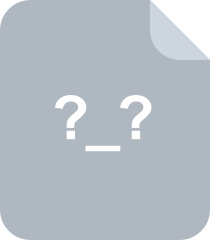
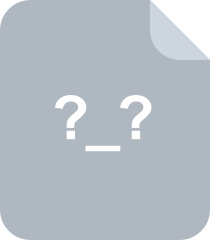
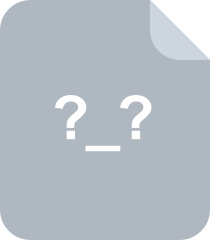
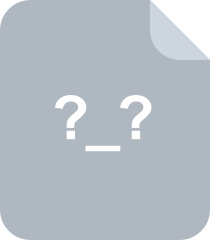
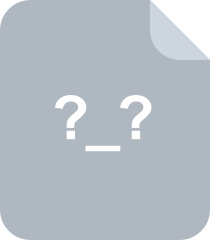
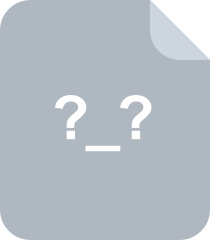
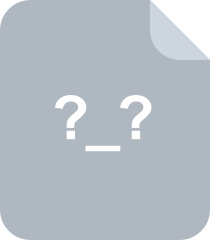
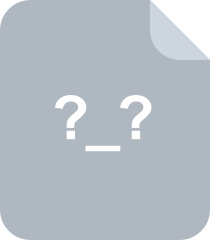
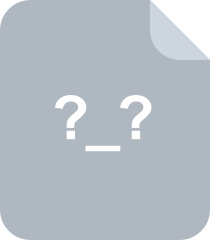
共 137 条
- 1
- 2
资源评论
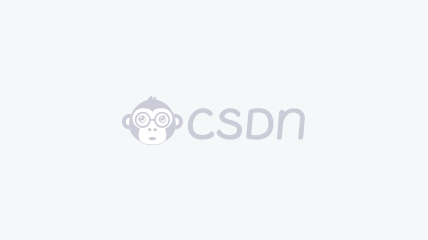

普通网友
- 粉丝: 30
- 资源: 4570
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

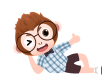
最新资源
- 美宝莲郑州国贸360店图纸增加灯片完稿.rar
- 基于C++实现的Hough Forests算法用于人体动作识别检测(提供了可视化功能).zip
- this is a GPU word
- 成都金楠天街活动包店.rar
- 【cocos creator】下拉框
- 基于pytorch实现3D ResNet网络的视频动作分类项目源码+运行说明+模型(支持得分模式和特征模式).zip
- 360国贸纽约城市&女神像.rar
- 更新城市蔓延指数数据集(1990-2023年).xlsx
- 动作识别基于PyTorch的3D ResNets模型实现的动作识别任务+运行说明(含训练、微调和测试、在UCF-101和HMDB-51等多数据集训练).zip
- datafor3dgs
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


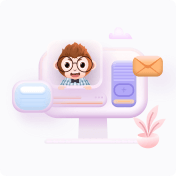
安全验证
文档复制为VIP权益,开通VIP直接复制
