# ![NFC logo][logo] Plugin.NFC
A Cross-Platform NFC (_Near Field Communication_) plugin to easily read and write NFC tags in your application.
This plugin uses **NDEF** (_NFC Data Exchange Format_) for maximum compatibilty between NFC devices, tag types, and operating systems.
## Status
|Package|Build|NuGet|MyGet
|:---|:---|:---|:---
|Plugin.NFC | [](https://dev.azure.com/franckbour/franckbour/_build/latest?definitionId=1) |  | 
CI Feed : https://www.myget.org/F/plugin-nfc/api/v3/index.json
## Supported Platforms
Platform|Version|Tested on
:---|:---|:---
Android|4.4+|Google Nexus 5, Huawei Mate 10 Pro
iOS|11+|iPhone 7
> Windows is currently not supported. Pull Requests are welcomed!
## Setup
### Android Specific
* Add NFC Permission `android.permission.NFC` and NFC feature `android.hardware.nfc` in your `AndroidManifest.xml`
```xml
<uses-permission android:name="android.permission.NFC" />
<uses-feature android:name="android.hardware.nfc" android:required="false" />
```
* Add the line `CrossNFC.Init(this)` in your `OnCreate()`
```csharp
protected override void OnCreate(Bundle savedInstanceState)
{
TabLayoutResource = Resource.Layout.Tabbar;
ToolbarResource = Resource.Layout.Toolbar;
base.OnCreate(savedInstanceState);
// Plugin NFC: Initialization
CrossNFC.Init(this);
global::Xamarin.Forms.Forms.Init(this, savedInstanceState);
LoadApplication(new App());
}
```
* Add the line `CrossNFC.OnResume()` in your `OnResume()`
```csharp
protected override void OnResume()
{
base.OnResume();
// Plugin NFC: Restart NFC listening on resume (needed for Android 10+)
CrossNFC.OnResume();
}
```
* Add the line `CrossNFC.OnNewIntent(intent)` in your `OnNewIntent()`
```csharp
protected override void OnNewIntent(Intent intent)
{
base.OnNewIntent(intent);
// Plugin NFC: Tag Discovery Interception
CrossNFC.OnNewIntent(intent);
}
```
### iOS Specific
> iOS 13+ is required for writing tags.
An iPhone 7+ and iOS 11+ are required in order to use NFC with iOS devices.
* Add `Near Field Communication Tag Reading` capabilty in your `Entitlements.plist`
```xml
<key>com.apple.developer.nfc.readersession.formats</key>
<array>
<string>NDEF</string>
<string>TAG</string>
</array>
```
* Add a NFC feature description in your Info.plist
```xml
<key>NFCReaderUsageDescription</key>
<string>NFC tag to read NDEF messages into the application</string>
```
* Add these lines in your Info.plist if you want to interact with ISO 7816 compatible tags
```xml
<key>com.apple.developer.nfc.readersession.iso7816.select-identifiers</key>
<string>com.apple.developer.nfc.readersession.iso7816.select-identifiers</string>
```
## API Usage
Before to use the plugin, please check if NFC feature is supported by the platform using `CrossNFC.IsSupported`.
To get the current platform implementation of the plugin, please call `CrossNFC.Current`:
* Check `CrossNFC.Current.IsAvailable` to verify if NFC is available.
* Check `CrossNFC.Current.IsEnabled` to verify if NFC is enabled.
* Register events:
```csharp
// Event raised when a ndef message is received.
CrossNFC.Current.OnMessageReceived += Current_OnMessageReceived;
// Event raised when a ndef message has been published.
CrossNFC.Current.OnMessagePublished += Current_OnMessagePublished;
// Event raised when a tag is discovered. Used for publishing.
CrossNFC.Current.OnTagDiscovered += Current_OnTagDiscovered;
// Event raised when NFC listener status changed
CrossNFC.Current.OnTagListeningStatusChanged += Current_OnTagListeningStatusChanged;
// Android Only:
// Event raised when NFC state has changed.
CrossNFC.Current.OnNfcStatusChanged += Current_OnNfcStatusChanged;
// iOS Only:
// Event raised when a user cancelled NFC session.
CrossNFC.Current.OniOSReadingSessionCancelled += Current_OniOSReadingSessionCancelled;
```
### Launch app when a compatible tag is detected on Android
In Android, you can use `IntentFilter` attribute on your `MainActivity` to initialize tag listening.
```csharp
[IntentFilter(new[] { NfcAdapter.ActionNdefDiscovered }, Categories = new[] { Intent.CategoryDefault }, DataMimeType = "application/com.companyname.yourapp")]
public class MainActivity : global::Xamarin.Forms.Platform.Android.FormsAppCompatActivity
{
...
}
```
To launch/open an app with a tag, `TypeFormat` of the record must be set to `NFCNdefTypeFormat.Mime` and `MimeType` should be setted to the same value of `IntentFilter.DataMimeType` (e.g. application/com.companyname.yourapp):
```csharp
var record = new NFCNdefRecord {
TypeFormat = NFCNdefTypeFormat.Mime,
MimeType = "application/com.companyname.yourapp",
Payload = NFCUtils.EncodeToByteArray(_writePayload)
};
```
### Read a tag
* Start listening with `CrossNFC.Current.StartListening()`.
* When a NDEF message is received, the event `OnMessageReceived` is raised.
### Write a tag
* To write a tag, call `CrossNFC.Current.StartPublishing()`
* Then `CrossNFC.Current.PublishMessage(ITagInfo)` when `OnTagDiscovered` event is raised.
* Do not forget to call `CrossNFC.Current.StopPublishing()` once the tag has been written.
### Clear a tag
* To clear a tag, call `CrossNFC.Current.StartPublishing(clearMessage: true)`
* Then `CrossNFC.Current.PublishMessage(ITagInfo)` when `OnTagDiscovered` event is raised.
* Do not forget to call `CrossNFC.Current.StopPublishing()` once the tag has been cleared.
For more examples, see sample application in the repository.
### Customizing UI messages
* Set a new `NfcConfiguration` object to `CrossNFC.Current` with `SetConfiguration(NfcConfiguration cfg)` method like below
```Csharp
// Custom NFC configuration (ex. UI messages in French)
CrossNFC.Current.SetConfiguration(new NfcConfiguration
{
Messages = new UserDefinedMessages
{
NFCWritingNotSupported = "L'écriture des TAGs NFC n'est pas supporté sur cet appareil",
NFCDialogAlertMessage = "Approchez votre appareil du tag NFC",
NFCErrorRead = "Erreur de lecture. Veuillez rééssayer",
NFCErrorEmptyTag = "Ce tag est vide",
NFCErrorReadOnlyTag = "Ce tag n'est pas accessible en écriture",
NFCErrorCapacityTag = "La capacité de ce TAG est trop basse",
NFCErrorMissingTag = "Aucun tag trouvé",
NFCErrorMissingTagInfo = "Aucune information à écrire sur le tag",
NFCErrorNotSupportedTag = "Ce tag n'est pas supporté",
NFCErrorNotCompliantTag = "Ce tag n'est pas compatible NDEF",
NFCErrorWrite = "Aucune information à écrire sur le tag",
NFCSuccessRead = "Lecture réussie",
NFCSuccessWrite = "Ecriture réussie",
NFCSuccessClear = "Effaçage réussi"
}
});
```
## Tutorials
Thanks to Saamer Mansoor ([@saamerm](https://github.com/saamerm)) who wrote this excellent article on [Medium](https://medium.com/@prototypemakers/start-building-with-nfc-rfid-tags-on-ios-android-using-xamarin-today-2268cf86d3b4) about Plugin.NFC and how to use it, check it out!
He also made this video:
[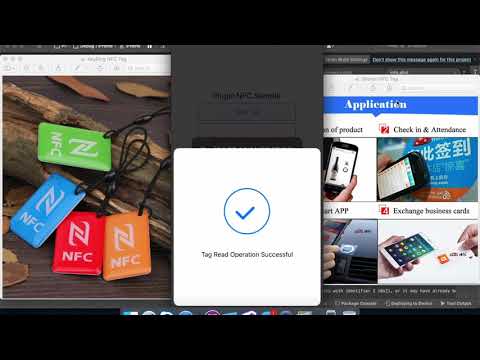](https://www.youtube.com/watch?v=STfzU18v7gE "Click to play on YouTube.com")
## Contributing
Feel free to contribute. PRs are accepted and welcomed.
## Credits
Inspired by the great work of many developers. Many thanks to:
- James Montemagno ([@jamesmontemagno](https://github.com/jamesmontemagno)).
- Matthew Leibowitz ([@mattleibow](https://github.com/mattleibow)) for [Xamarin.Essentials PR #131](https://github.com/xamarin/Essentials/pull/131).
- Alessandro Pozone ([@poz1](https://github.com/poz1)) for [NFCForms](https://github.com/poz1/NFCForms).
- Ultz ([@ultz](https://github.com/Ultz)) for [XNFC](https://github.com
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
插件NFC 一个跨平台NFC(近场通信)插件,可轻松在您的应用程序中读写NFC标签。 该插件使用NDEF ( NFC数据交换格式)来最大程度地在NFC设备,标签类型和操作系统之间实现兼容性。 状态 包 建立 NuGet 我的Get 插件NFC CI订阅源: : 支持平台 平台 版 经过测试 安卓 4.4+ Google Nexus 5,华为Mate 10 Pro 的iOS 11+ iPhone 7 当前不支持Windows。 拉请求是受欢迎的! 建立 特定于Android 在AndroidManifest.xml添加NFC权限android.permission.NF
资源详情
资源评论
资源推荐
收起资源包目录

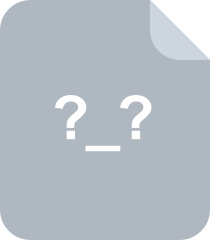
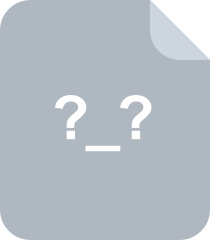
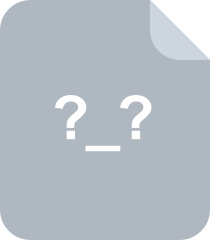
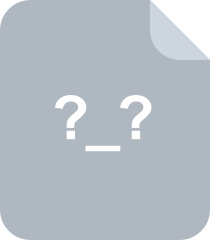
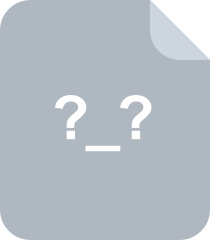
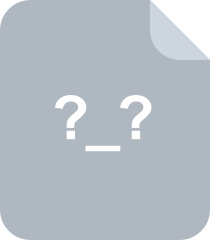
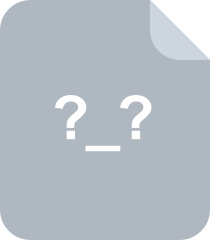
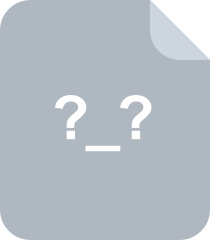
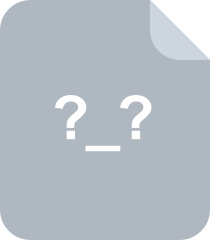
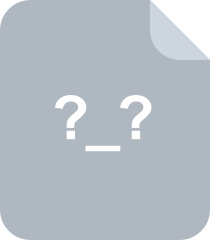
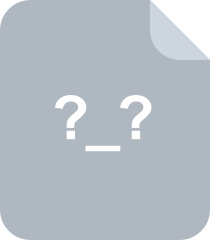
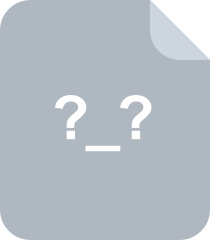
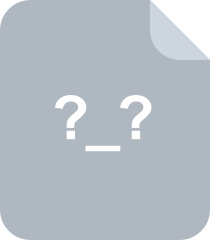
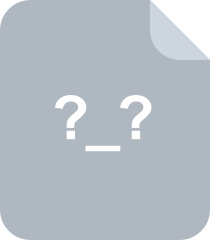
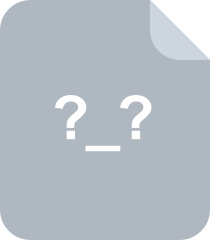
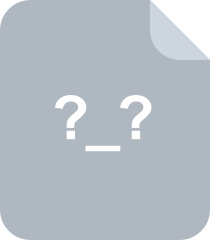
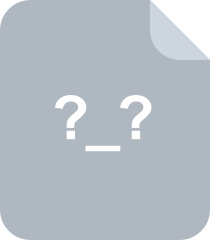
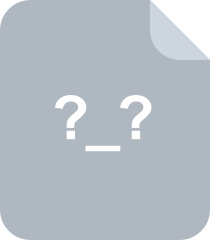
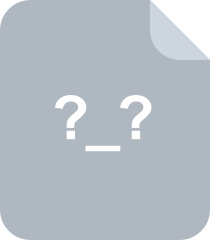
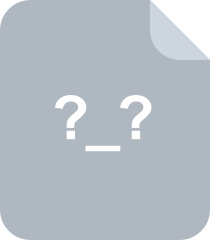
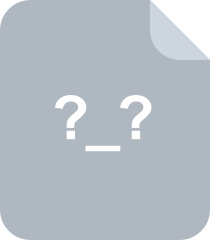
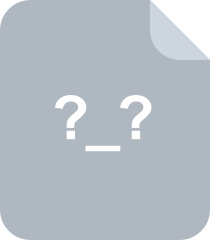
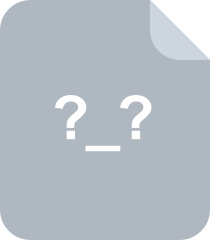
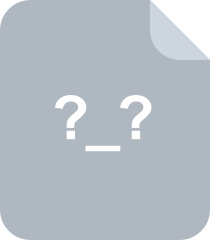
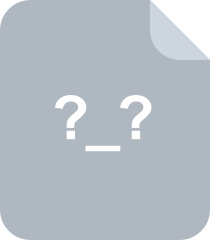
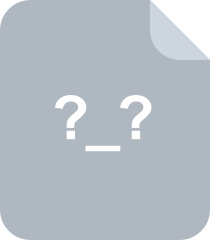
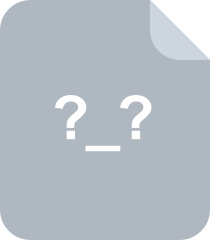
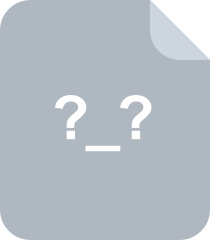
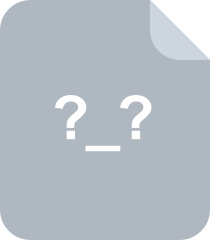
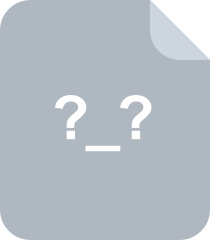
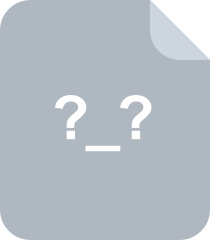
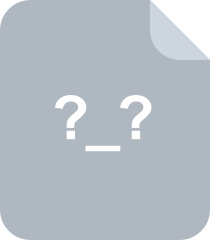
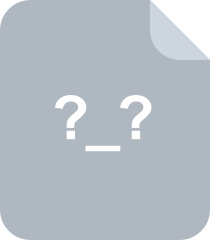
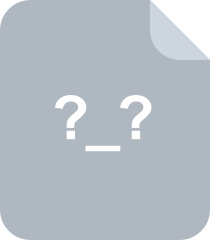
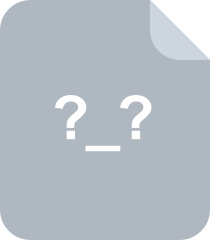
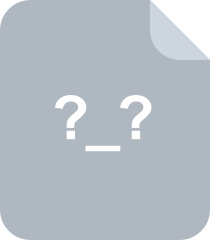
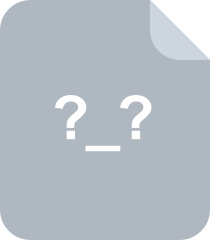
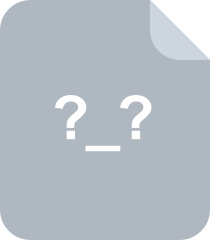
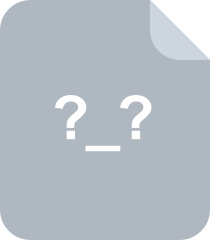
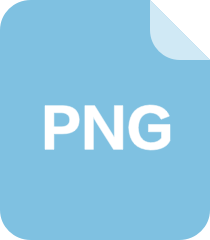
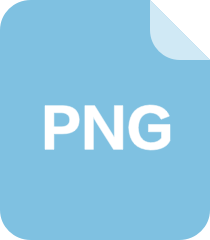
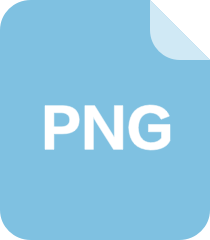
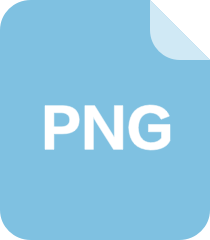
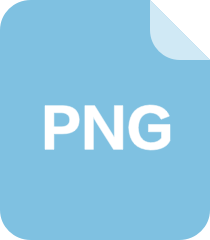
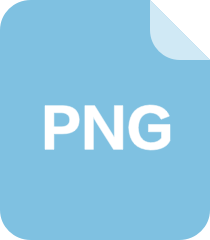
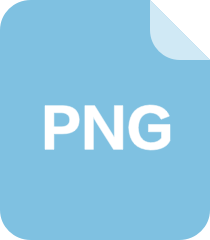
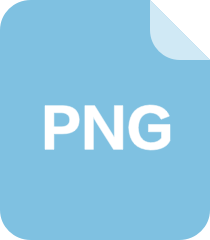
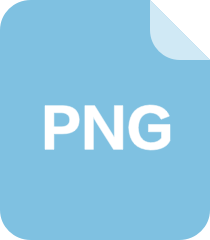
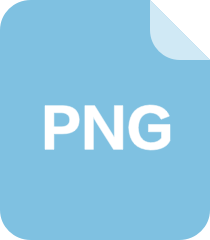
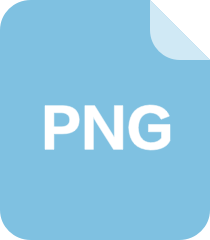
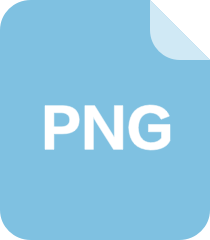
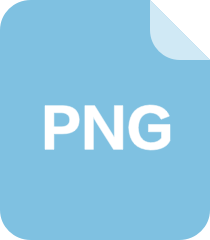
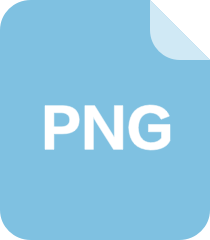
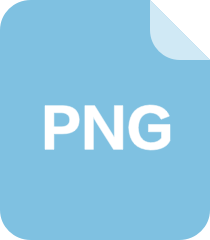
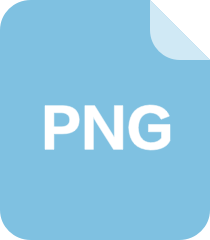
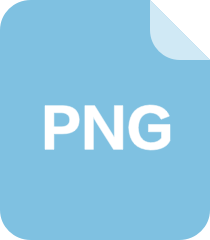
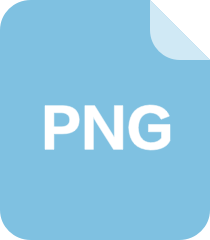
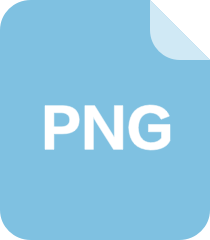
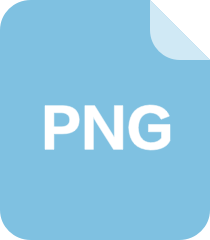
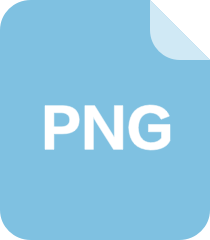
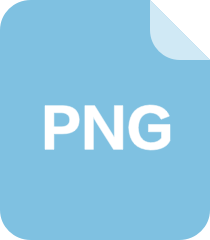
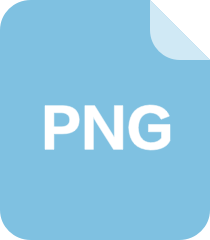
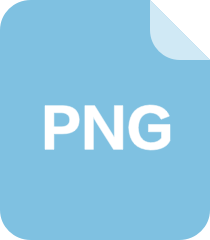
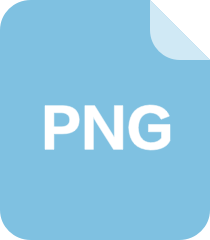
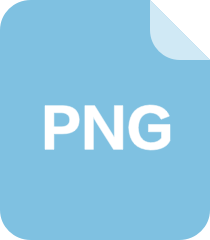
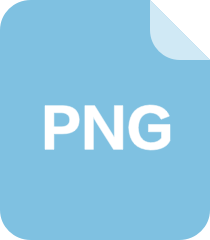
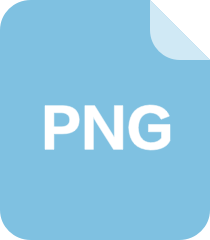
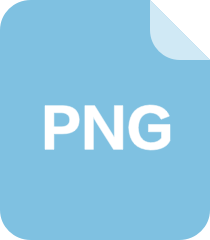
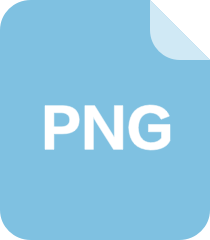
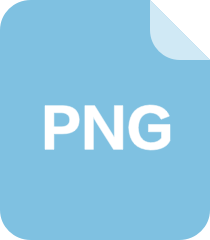
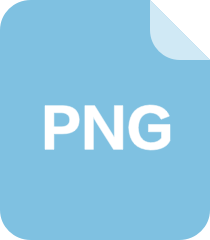
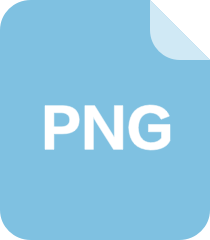
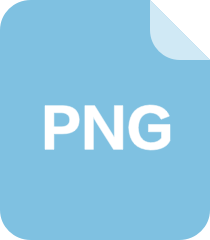
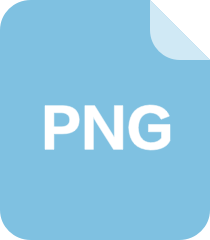
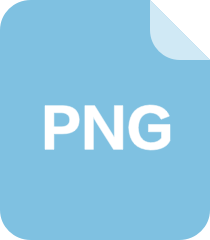
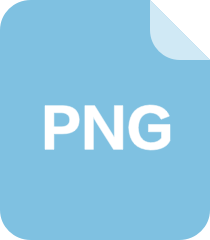
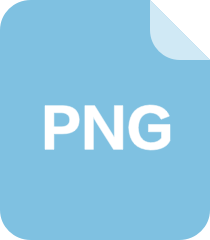
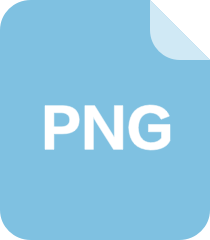
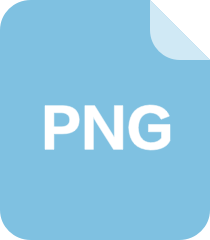
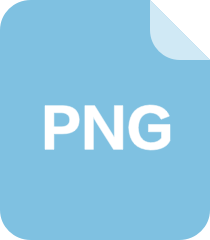
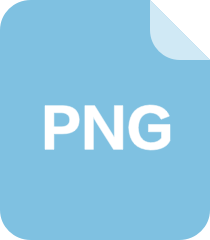
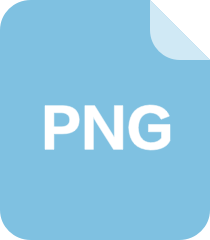
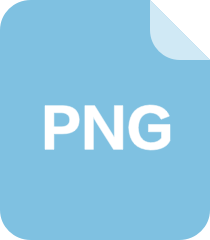
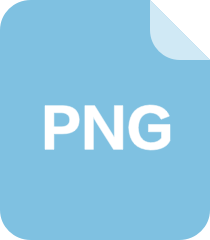
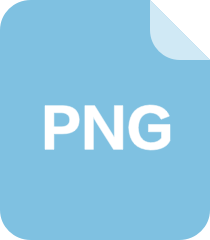
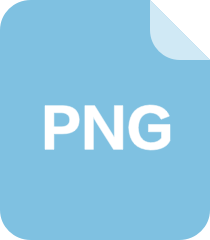
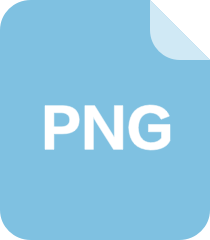
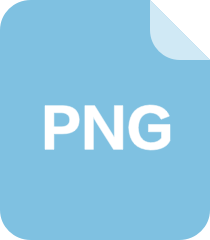
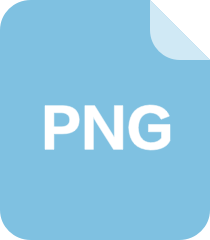
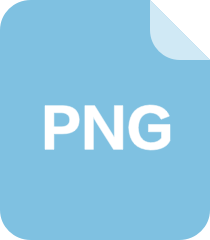
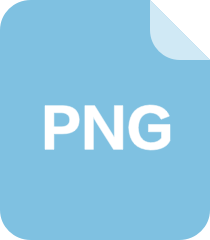
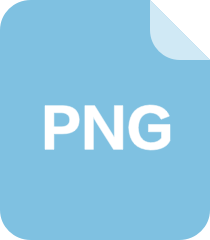
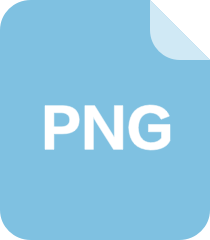
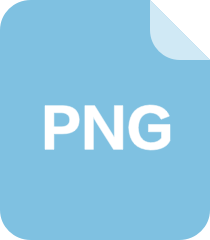
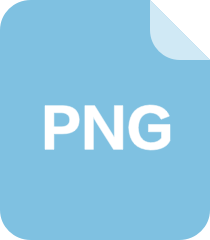
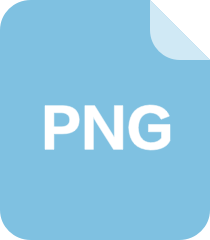
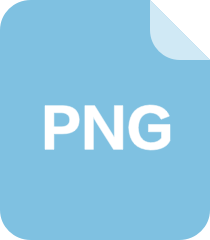
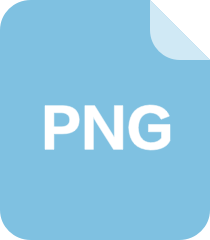
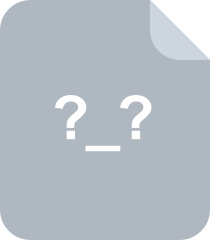
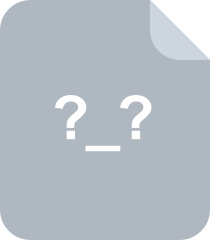
共 113 条
- 1
- 2
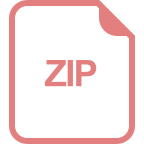
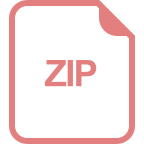
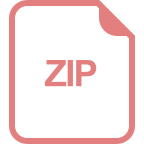
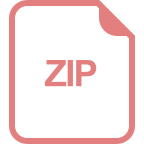
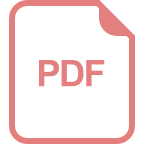
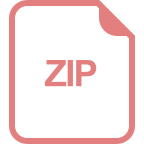
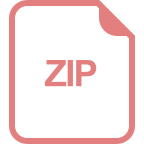
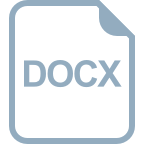
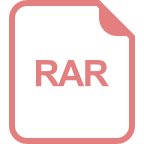
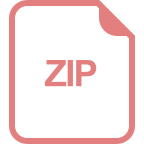
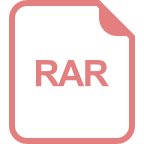
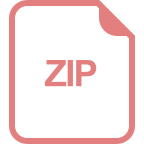
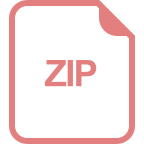
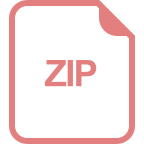
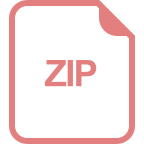
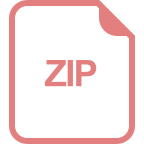
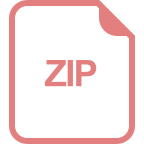
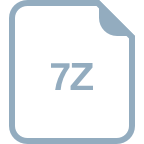
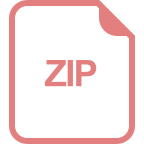
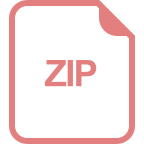

小马甲不小
- 粉丝: 30
- 资源: 4714
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

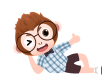
最新资源
- 用QT写的usb摄像头播放器,可以用于linux平台和Windows平台 因为QT配置不同在不同平台下都可以编译,希望对你有所帮助
- Matlab语言教程:覆盖基础知识至高级应用
- 计算机科学中汇编语言的基础教程与应用
- 【重磅,更新!】国内外期刊最全信息库(6万多本期刊)(2024版)
- ECAM ODB++资料解析C++调用和C#调用的例程
- 安装office2010时提示MSXML问题的一键修复工具
- R语言中机器学习基础与实战:监督学习和无监督学习的应用
- 价值50元的茅子单页商城 PHP单页下单商城源码
- 【重磅,更新!】国自然管理学部标书80+份(内附清单)(2005-2021年)
- windows 自动关机小程序
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


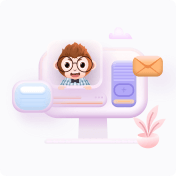
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0