在信号处理领域,滤波器的设计是至关重要的环节,它用于去除噪声、改善信号质量或提取特定频带的信息。IIR(无限 impulse response)和FIR(有限 impulse response)是两种常见的数字滤波器类型,它们各有优缺点,广泛应用于通信、音频处理、图像处理等多个领域。本项目“基于IIR滤波器和FIR滤波器的matlab仿真”提供了完整的MATLAB代码,可直接运行,对于理解和应用这两种滤波器有着极大的帮助。 IIR滤波器以其相对简单的结构和较少的计算量而受到青睐。它的特点是利用反馈机制,使得系统对输入信号的响应不仅取决于当前输入,还与过去输入有关,因此能够实现非常陡峭的过渡带。然而,由于存在反馈,可能会导致稳定性问题,设计时需特别注意避免自激振荡。 FIR滤波器则以其线性相位特性、稳定性和设计灵活性而著名。FIR滤波器的输出只与当前及之前输入信号有关,没有反馈,因此在稳定性和实现上更加简单。通过窗函数法、频率采样法或最优化方法等设计方法,可以实现各种类型的滤波特性。但相比IIR滤波器,FIR滤波器通常需要更多的计算资源,特别是在要求高精度和宽通带时。 MATLAB是进行滤波器设计和仿真的强大工具,其Signal Processing Toolbox提供了丰富的滤波器设计函数,如`designfilt`可以用于创建IIR和FIR滤波器,`filter`和`filtfilt`用于滤波操作,以及`freqz`用于可视化频率响应。项目中的"Voice-Filter-Design-master"可能包含了这些函数的示例应用,以及针对语音信号处理的滤波器设计。 在语音处理中,IIR和FIR滤波器常用于语音增强,去除背景噪声,或者实现语音编码和解码。例如,低通滤波器可用于平滑信号,高通滤波器则可以提取语音的高频成分,而带通滤波器则有助于分离不同频率范围的语音特征。 通过这个MATLAB仿真项目,学习者可以深入理解滤波器的工作原理,比较IIR和FIR滤波器的性能差异,并掌握如何在实际应用中选择合适的滤波器。同时,项目提供的代码可以帮助验证理论知识,提升实践能力,是进行数字信号处理学习和研究的宝贵资源。
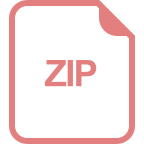
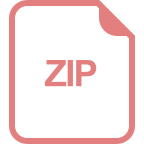
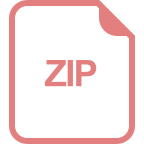
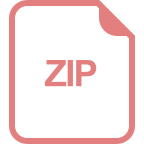
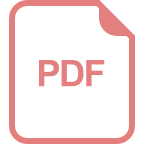
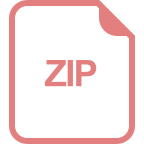
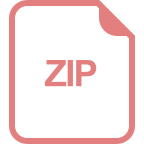
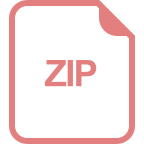
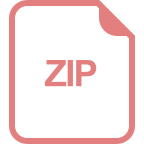
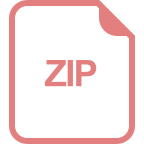
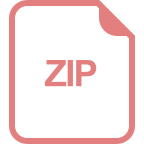
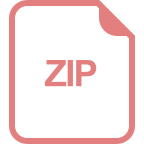
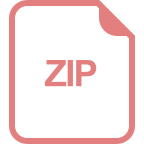
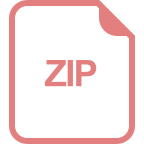
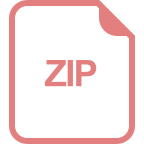
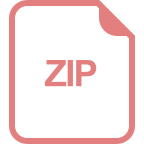


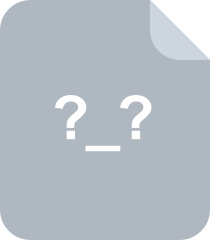
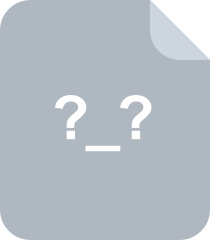
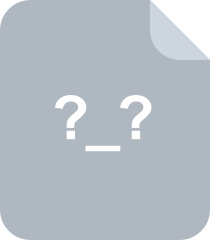
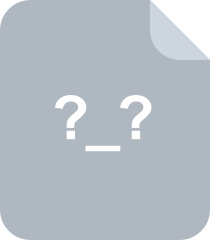
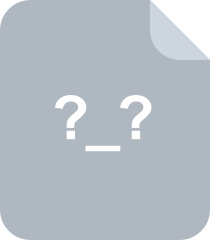
- 1
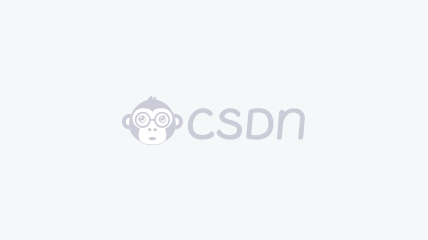

- 粉丝: 16
- 资源: 51
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

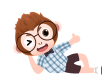
最新资源
- 电影购票系统-Java Web项目
- SPD-Conv-main.zip
- 使用Python和Pygame库创建新年烟花动画效果
- chapter9.zip
- 安居客Python爬虫代码.zip
- 企业可持续发展性数据集,ESG数据集,公司可持续发展性数据(可用于多种企业可持续性研究场景)
- 车辆轨迹自适应预瞄跟踪控制和自适应p反馈联合控制,自适应预苗模型和基于模糊p控制均在simulink中搭建 个人觉得跟踪效果相比模糊pid效果好很多,轨迹跟踪过程,转角控制平滑自然,车速在36到72
- 数据分析-49-客户细分-K-Means聚类分析
- TIA PORTAL V18 UPD5更新包(2024.10最新)-链接地址.txt
- 使用Python和Pygame实现圣诞节动画效果
- 自动驾驶不同工况避障模型(perscan、simulink、carsim联仿),能够避开预设的(静态)障碍物
- 100个情侣头像,唯美手绘情侣头像
- 国际象棋检测10-YOLO(v5至v9)、COCO、CreateML、Paligemma数据集合集.rar
- 2024~2025(1)Oracle数据库技术A卷-22软单、软嵌.doc
- 睡眠健康与生活方式数据集,睡眠和生活习惯关联分析(睡眠影响因素)
- 浪漫节日代码 - 爱心代码、圣诞树代码

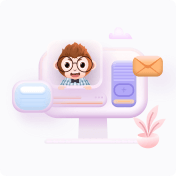
