<?php
namespace app\common\services;
use app\common\repositories\system\config\ConfigValueRepository;
use Exception;
use GuzzleHttp\Client as HttpClient;
use kornrunner\Secp256k1;
use think\exception\ValidateException;
use think\facade\Cache;
use think\facade\Log;
use Web3\Contract;
use Web3\Providers\HttpProvider;
use Web3\RequestManagers\HttpRequestManager;
use Web3\Utils;
use Web3\Web3;
use Web3p\EthereumTx\Transaction;
use Web3p\EthereumUtil\Util;
class JsonRpc
{
protected $web3;
protected $callback;
protected $chainId;
public function __construct($callback = null)
{
// Set the custom chain RPC URL and Chain ID
$rpcUrl = env('moralis.rpc_url');
$this->chainId = env('moralis.bscchain');
// Create a new HttpProvider instance
$provider = new HttpProvider(new HttpRequestManager($rpcUrl, 30));
$this->web3 = new Web3($provider);
// Set the custom chain ID
// $this->web3->provider->requestManager->ethereum->net->version(function ($err) use ($chainId) {
// if ($err !== null) {
// throw new \Exception('Error setting custom chain ID: ' . $err->getMessage());
// }
// $this->web3->provider->requestManager->ethereum->_chainId = $chainId;
// });
// Set the callback function if provided
if ($callback !== null) {
$this->callback = $callback;
}
}
public function getAddress($privateKey)
{
$util = new Util();
return $util->publicKeyToAddress($util->privateKeyToPublicKey($privateKey));
}
public function getBalance(string $address, callable $callback = null)
{
$callback = $callback ?? $this->callback;
$this->web3->eth->getBalance($address, function ($err, $balance) use ($callback) {
if ($err !== null) {
throw new \Exception('Error getting balance: ' . $err->getMessage());
}
if ($callback !== null) {
// $balanceInEther = $this->web3->utils->fromWei($balance, 'ether');
$balanceInEther = $balance->toString();
call_user_func($callback, $balanceInEther);
}
});
}
/**基于给定的选项创建一个过滤器对象,接收状态变化时的通知
* @param $contractAddress
* @param callable|null $callback
* @return void
* @throws \Exception
* @author Admin
* @date 2023-09-09 12:00
*/
public function newFilter($contractAddress, callable $callback = null)
{
$callback = $callback ?? $this->callback;
$this->web3->eth->newFilter([
"fromBlock" => '0x' . dechex(33128536),
"toBlock" => "latest",
'address' => $contractAddress, // 合约地址
[
"topics" => ["0xddf252ad1be2c89b69c2b068fc378daa952ba7f163c4a11628f55a4df523b3ef"]
]
],function ($err, $fid) use ($callback) {
if ($err !== null) {
throw new \Exception('Error getting newFilter: ' . $err->getMessage());
}
if ($callback !== null) {
call_user_func($callback, $fid);
}
});
}
/** 返回指定编号过滤器中的全部日志
* @param $fid
* @param callable|null $callback
* @return void
* @throws \Exception
* @author Admin
* @date 2023-09-09 12:00
*/
public function getFilterLogs($fid, callable $callback = null)
{
$callback = $callback ?? $this->callback;
$this->web3->eth->getFilterLogs($fid,function ($err, $log) use ($callback) {
if ($err !== null) {
throw new \Exception('Error getting getFilterLogs: ' . $err->getMessage());
}
if ($callback !== null) {
call_user_func($callback, $log);
}
});
}
public function getGasPrice(callable $callback = null)
{
$callback = $callback ?? $this->callback;
$this->web3->eth->gasPrice(function ($err, $gasPrice) use ($callback) {
if ($err !== null) {
throw new \Exception('Error getting gas price: ' . $err->getMessage());
}
if ($callback !== null) {
$gasPrice = $gasPrice->toString();
call_user_func($callback, $gasPrice);
}
});
}
public function sendTransaction(
string $from,
string $to,
float $value,
string $privateKey,
callable $callback = null
) {
$callback = $callback ?? $this->callback;
$eth = $this->web3->eth;
if (substr($privateKey, 0, 2) !== '0x') {
$privateKey = '0x' . $privateKey;
}
$customGas = 21000; // Custom gas limit (in decimal)
$customGasPrice = 5 * 1000000000; // Custom gas price (15 Gwei, in decimal)
$amount = bcmul($value, bcpow(10, 18), 0);
$transaction = [
'from' => $from,
'to' => $to,
'value' => '0x' . dechex($amount),
'gas' => '0x' . dechex($customGas), // Custom gas limit (in hexadecimal)
'gasPrice' => '0x' . dechex($customGasPrice), // Custom gas price (in hexadecimal)
'chainId' => $this->chainId,
];
// Check the sender's balance
$eth->getBalance($from, 'latest', function ($err, $balance) use ($transaction, $privateKey,$from, $callback, $amount) {
if ($err !== null) {
throw new \Exception('Error getting balance: ' . $err->getMessage());
}
if(bccomp($balance->toString(), $amount, 0) < 0){
throw new \Exception(lang("没有足够的",["BRT"]));
}
// Calculate the total cost of the transaction (value + gas * gasPrice)
$totalCost = bcadd($transaction['value'], bcmul(hexdec($transaction['gas']), hexdec($transaction['gasPrice']), 0), 0);
// Compare the sender's balance to the total cost of the transaction
if (bccomp($balance->toString(), $totalCost, 0) < 0) {
throw new \Exception('Insufficient funds for gas * price + value');
}
// If there are sufficient funds, proceed with sending the transaction
// Get the transaction count for the sender address
$this->web3->eth->getTransactionCount($from, 'latest', function ($err, $transactionCount) use ($transaction, $privateKey, $callback) {
if ($err !== null) {
throw new \Exception('Error getting transaction count: ' . $err->getMessage());
}
// Create and sign the transaction
$transaction['nonce'] = '0x' . dechex((int)$transactionCount->toString());
$ethTx = new Transaction($transaction);
$signedTransaction = $ethTx->sign($privateKey);
// Send the signed transaction
$this->web3->eth->sendRawTransaction('0x' . $signedTransaction, function ($err, $transactionHash) use ($callback) {
if ($err !== null) {
throw new \Exception('Error sending transaction: ' . $err->getMessage());
}
if ($callback !== null) {
call_user_func($callback, $transactionHash);
}
});
});
});
}
/** 通过hash 获取交易信息
* @param string $transactionHash
* @param callable|null $callback
* @return void
* @throws \Exception
* @author Admin
* @date 2023-09-04 10:15
*/
public function getTransaction(string $transactionHash, c
没有合适的资源?快使用搜索试试~ 我知道了~
eth系列json_rpc调用类 1:支持任意以太坊系列json_rpc调用 2:支持事务监听,监听合约,事件,账户等 3:支持EIP712调用,包含js,php等方法调用
资源推荐
资源详情
资源评论
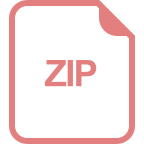
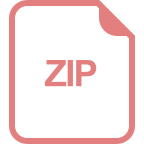
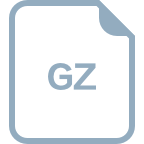
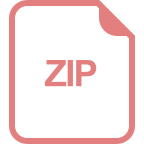
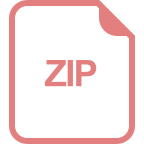
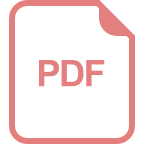
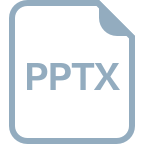
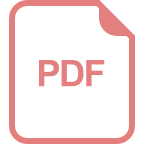
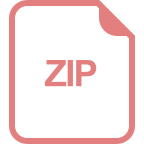
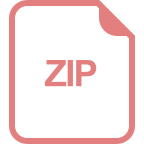
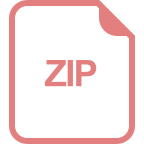
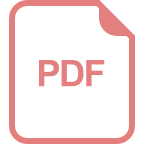
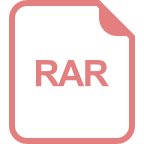
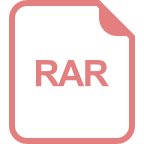
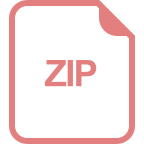
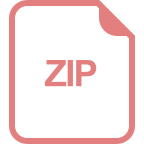
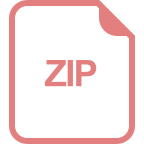
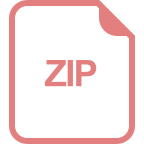
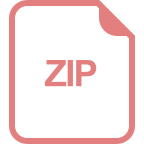
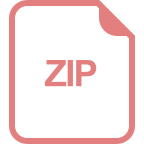
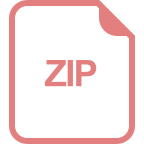
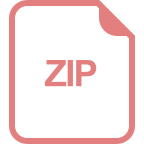
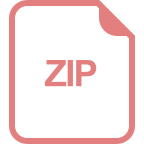
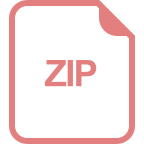
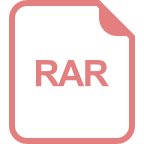
收起资源包目录

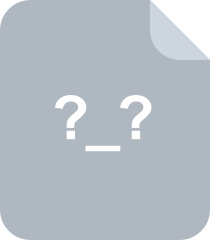
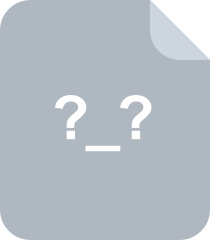
共 2 条
- 1
资源评论
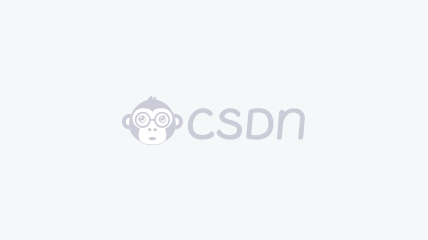

weixin_41997115
- 粉丝: 127
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

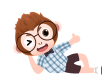
最新资源
- 5G模组升级刷模块救砖以及5G模组资料路由器固件
- C183579-123578-c1235789.jpg
- Qt5.14 绘画板 Qt Creator C++项目
- python实现Excel表格合并
- Java实现读取Excel批量发送邮件.zip
- 【java毕业设计】商城后台管理系统源码(springboot+vue+mysql+说明文档).zip
- 【java毕业设计】开发停车位管理系统(调用百度地图API)源码(springboot+vue+mysql+说明文档).zip
- 星耀软件库(升级版).apk.1
- 基于Django后端和Vue前端的多语言购物车项目设计源码
- 基于Python与Vue的浮光在线教育平台源码设计
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


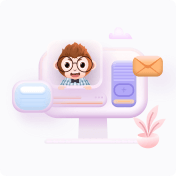
安全验证
文档复制为VIP权益,开通VIP直接复制
