package com.mobileclient.util;
import java.io.ByteArrayOutputStream;
import java.io.DataOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLConnection;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
import android.content.Intent;
import android.net.Uri;
import android.os.Environment;
import com.mobileclient.activity.photoListActivity;
import com.mobileclient.app.Declare;
public class HttpUtil {
// 基础URL
public static final String BASE_URL = "http://192.168.1.103:8080/JavaWebProject/";
public static final String FILE_PATH = Environment.getExternalStorageDirectory().getAbsolutePath() + "/mobileclient";
public static final String Cach_Dir = Declare.context.getCacheDir().getAbsolutePath();
// 获得Get请求对象request
public static HttpGet getHttpGet(String url) {
HttpGet request = new HttpGet(url);
return request;
}
// 获得Post请求对象request
public static HttpPost getHttpPost(String url) {
HttpPost request = new HttpPost(url);
return request;
}
// 根据请求获得响应对象response
public static HttpResponse getHttpResponse(HttpGet request)
throws ClientProtocolException, IOException {
HttpResponse response = new DefaultHttpClient().execute(request);
return response;
}
// 根据请求获得响应对象response
public static HttpResponse getHttpResponse(HttpPost request)
throws ClientProtocolException, IOException {
HttpResponse response = new DefaultHttpClient().execute(request);
return response;
}
// 发送Post请求,获得响应查询结果
public static String queryStringForPost(String url) {
// 根据url获得HttpPost对象
HttpPost request = HttpUtil.getHttpPost(url);
String result = null;
try {
// 获得响应对象
HttpResponse response = HttpUtil.getHttpResponse(request);
// 判断是否请求成功
if (response.getStatusLine().getStatusCode() == 200) {
// 获得响应
result = EntityUtils.toString(response.getEntity());
return result;
}
} catch (ClientProtocolException e) {
e.printStackTrace();
result = "网络异常!";
return result;
} catch (IOException e) {
e.printStackTrace();
result = "网络异常!";
return result;
}
return null;
}
// 获得响应查询结果
public static String queryStringForPost(HttpPost request) {
String result = null;
try {
// 获得响应对象
HttpResponse response = HttpUtil.getHttpResponse(request);
// 判断是否请求成功
if (response.getStatusLine().getStatusCode() == 200) {
// 获得响应
result = EntityUtils.toString(response.getEntity());
return result;
}
} catch (ClientProtocolException e) {
e.printStackTrace();
result = "网络异常!";
return result;
} catch (IOException e) {
e.printStackTrace();
result = "网络异常!";
return result;
}
return null;
}
// 发送Get请求,获得响应查询结果
public static String queryStringForGet(String url) {
// 获得HttpGet对象
HttpGet request = HttpUtil.getHttpGet(url);
String result = null;
try {
// 获得响应对象
HttpResponse response = HttpUtil.getHttpResponse(request);
// 判断是否请求成功
if (response.getStatusLine().getStatusCode() == 200) {
// 获得响应
result = EntityUtils.toString(response.getEntity());
return result;
}
} catch (ClientProtocolException e) {
e.printStackTrace();
result = "网络异常!";
return result;
} catch (IOException e) {
e.printStackTrace();
result = "网络异常!";
return result;
}
return null;
}
public static boolean sendXML(String path, String xml) throws Exception {
byte[] data = xml.getBytes();
URL url = new URL(path);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setConnectTimeout(5 * 1000);
conn.setDoOutput(true);// 如果通过post提交数据,必须设置允许对外输出数据
conn.setRequestProperty("Content-Type", "text/xml; charset=UTF-8");
conn.setRequestProperty("Content-Length", String.valueOf(data.length));
OutputStream outStream = conn.getOutputStream();
outStream.write(data);
outStream.flush();
outStream.close();
if (conn.getResponseCode() == 200) {
return true;
}
return false;
}
public static byte[] sendGetRequest(String path,
Map<String, String> params, String enc) throws Exception {
StringBuilder sb = new StringBuilder(path);
sb.append('?');
// ?method=save&title=435435435&timelength=89&
for (Map.Entry<String, String> entry : params.entrySet()) {
sb.append(entry.getKey()).append('=')
.append(URLEncoder.encode(entry.getValue(), enc))
.append('&');
}
sb.deleteCharAt(sb.length() - 1);
URL url = new URL(sb.toString());
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setConnectTimeout(5 * 1000);
if (conn.getResponseCode() == 200) {
return readStream(conn.getInputStream());
}
return null;
}
public static boolean sendPostRequest(String path,
Map<String, String> params, String enc) throws Exception {
// title=dsfdsf&timelength=23&method=save
StringBuilder sb = new StringBuilder();
if (params != null && !params.isEmpty()) {
for (Map.Entry<String, String> entry : params.entrySet()) {
sb.append(entry.getKey()).append('=')
.append(URLEncoder.encode(entry.getValue(), enc))
.append('&');
}
sb.deleteCharAt(sb.length() - 1);
}
byte[] entitydata = sb.toString().getBytes();// 得到实体的二进制数据
URL url = new URL(path);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setConnectTimeout(5 * 1000);
conn.setDoOutput(true);// 如果通过post提交数据,必须设置允许对外输出数据
// Content-Type: application/x-www-form-urlencoded
// Content-Length: 38
conn.setRequestProperty("Content-Type",
"application/x-www-form-urlencoded");
conn.setRequestProperty("Content-Length",
String.valueOf(entitydata.length));
OutputStream outStream = conn.getOutputStream();
outStream.write(entitydata);
outStream.flush();
outStream.close();
if (conn.getResponseCode() == 200) {
return true;
}
return false;
}
public static byte[] SendPostRequest(String path,
Map<String, String> params, String enc) throws Exception {
// title=dsfdsf&timelength=23&method=save
StringBuilder sb = new StringBuilder();
if (params != null && !params.isEmpty()) {
for (Map.Entry<String, String> entry : params.entrySet()) {
sb.append(entry.getKey()).append('=')
.append(URLEncoder.encode(entry.getValue(), enc))
.append('&');
}
sb.deleteCharAt(sb.length() - 1);
}
byte[] entitydata = sb.toString().getBytes();// 得到实体的二进制数据
URL url = new URL(path);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setConnectTimeout(5 * 1000);
conn.setDoOutput(true);// 如果通过post提交数据,必须设置允许对外输�

九转成圣
- 粉丝: 5780
- 资源: 2959
最新资源
- 电路分析基础 实验五 RLC串联谐振的multisim仿真
- 2个月涨粉8w,新玩法AI做漫画小说赛道,操作简单可批量制作,新手小白....mp4
- 高分辨率下的遥感目标分割
- 网络攻防原理与技术-实验8资料.7z
- 电机控制器,永磁同步电机调速控制软件工程PMSM,该工程主要基于DSP28335硬件控制平台,两电平IPM模块主回路,通过位置传感器,速度传感器实时检测位置和速度信号,电流传感器采集电流信号,控制器控
- 24年快手无人直播暴利变现3.0,直播间人气轻松破千上热门,普通人也能....mp4
- 2024年9月28日支付宝分成最新搬运玩法.mp4
- 西门子1200PLC模板通讯程序 modbus 包含多种通讯Modbus-RTU(485),S7通讯,Modbus-TCP,TCP IP等,博途V16及较新版本可打开,简单明了,初学者也能明白
- ICED Smart 网站部署教程文件
- 2024淘宝暴力掘金 单机500+.mp4
- 2024年最新暴力起店玩法,拼多多虚拟电商4.0,24小时实现成交,单人可以...mp4
- 2024影视解说最新玩法,AI一键生成原创影视解说, 十秒钟制作成品,解....mp4
- 2024掌握拼多多运营精髓:爆款流程、定价技巧与SKU设计实战课.mp4
- 房屋租赁推荐系统 房租租赁系统 基于协同过滤的房屋租赁推荐系统 特色功能:协同过滤推荐 对于房租租赁,结合实际场景选择用户对房子的浏览次数作为数据集,体现用户喜好度,应用余弦相似度,实现基于用户协同过
- X6程序模块 AE-10D00.GDL
- AspSweb网页服务器1.0.0.93
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


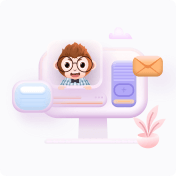