// TI File $Revision: /main/2 $
// Checkin $Date: April 4, 2007 14:25:27 $
//###########################################################################
//
// FILE: DSP2833x_SWPrioritizedDefaultIsr.c
//
// TITLE: DSP2833x Device Default Software Prioritized Interrupt Service Routines.
//
//###########################################################################
//
// $TI Release: DSP2833x Header Files V1.01 $
// $Release Date: September 26, 2007 $
//###########################################################################
#include "DSP2833x_Device.h" // DSP2833x Headerfile Include File
#include "DSP2833x_Examples.h" // DSP2833x Examples Include File
#include "DSP2833x_SWPrioritizedIsrLevels.h"
// Connected to INT13 of CPU (use MINT13 mask):
// Note CPU-Timer1 is reserved for TI use, however XINT13
// ISR can be used by the user.
#if (INT13PL != 0)
interrupt void INT13_ISR(void) // INT13 or CPU-Timer1
{
IER |= MINT13; // Set "global" priority
EINT;
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
#endif
// Connected to INT14 of CPU (use MINT14 mask):
#if (INT14PL != 0)
interrupt void INT14_ISR(void) // CPU-Timer2
{
IER |= MINT14; // Set "global" priority
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
#endif
// Connected to INT15 of CPU (use MINT15 mask):
#if (INT15PL != 0)
interrupt void DATALOG_ISR(void) // Datalogging interrupt
{
IER |= MINT15; // Set "global" priority
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
#endif
// Connected to INT16 of CPU (use MINT16 mask):
#if (INT16PL != 0)
interrupt void RTOSINT_ISR(void) // RTOS interrupt
{
IER |= MINT16; // Set "global" priority
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
#endif
// Connected to EMUINT of CPU (non-maskable):
interrupt void EMUINT_ISR(void) // Emulation interrupt
{
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// Connected to NMI of CPU (non-maskable):
interrupt void NMI_ISR(void) // Non-maskable interrupt
{
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
interrupt void ILLEGAL_ISR(void) // Illegal operation TRAP
{
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
interrupt void USER1_ISR(void) // User Defined trap 1
{
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
interrupt void USER2_ISR(void) // User Defined trap 2
{
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
interrupt void USER3_ISR(void) // User Defined trap 3
{
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
interrupt void USER4_ISR(void) // User Defined trap 4
{
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
interrupt void USER5_ISR(void) // User Defined trap 5
{
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
interrupt void USER6_ISR(void) // User Defined trap 6
{
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
interrupt void USER7_ISR(void) // User Defined trap 7
{
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
interrupt void USER8_ISR(void) // User Defined trap 8
{
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
interrupt void USER9_ISR(void) // User Defined trap 9
{
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
interrupt void USER10_ISR(void) // User Defined trap 10
{
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
interrupt void USER11_ISR(void) // User Defined trap 11
{
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
interrupt void USER12_ISR(void) // User Defined trap 12
{
EINT;
// Insert ISR Code here.......
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// -----------------------------------------------------------
// PIE Group 1 - MUXed into CPU INT1
// -----------------------------------------------------------
// Connected to PIEIER1_1 (use MINT1 and MG11 masks):
#if (G11PL != 0)
interrupt void SEQ1INT_ISR( void ) // ADC
{
// Set interrupt priority:
volatile Uint16 TempPIEIER = PieCtrlRegs.PIEIER1.all;
IER |= M_INT1;
IER &= MINT1; // Set "global" priority
PieCtrlRegs.PIEIER1.all &= MG11; // Set "group" priority
PieCtrlRegs.PIEACK.all = 0xFFFF; // Enable PIE interrupts
EINT;
// Insert ISR Code here.......
// Restore registers saved:
DINT;
PieCtrlRegs.PIEIER1.all = TempPIEIER;
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
#endif
// Connected to PIEIER1_2 (use MINT1 and MG12 masks):
#if (G12PL != 0)
interrupt void SEQ2INT_ISR( void ) // ADC
{
// Set interrupt priority:
volatile Uint16 TempPIEIER = PieCtrlRegs.PIEIER1.all;
IER |= M_INT1;
IER &= MINT1; // Set "global" priority
PieCtrlRegs.PIEIER1.all &= MG12; // Set "group" priority
PieCtrlRegs.PIEACK.all = 0xFFFF; // Enable PIE interrupts
EINT;
// Insert ISR Code here.......
// Restore registers saved:
DINT;
PieCtrlRegs.PIEIER1.all = TempPIEIER;
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
没有合适的资源?快使用搜索试试~ 我知道了~
基于TMS320F28335处理器的开关电源模块并联供电系统设计资料 包含原理图及源代码
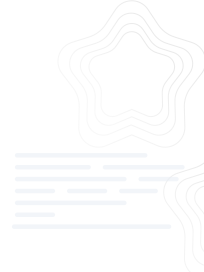
共37个文件
c:22个
asm:6个
pcbdoc:4个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
采用TI公司的DSP TMS320C28335作为主控,实现PWM输出,并控制A/D对输入输出的电压电流信号进行采样,从而进行可靠的闭环控制。与模拟控制方法相比,数字控制方法灵活性高、可靠性好、抗干扰能力强。
资源推荐
资源详情
资源评论
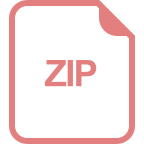
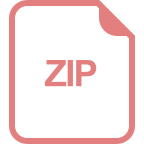
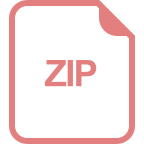
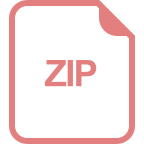
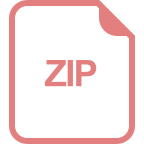
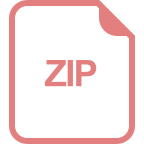
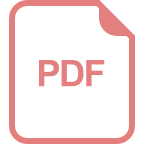
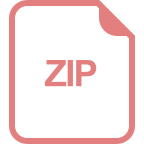
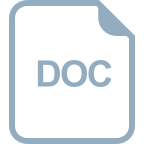
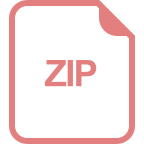
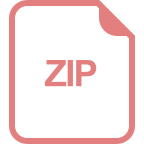
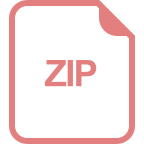
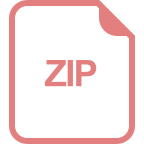
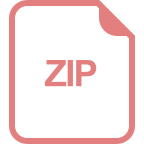
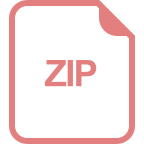
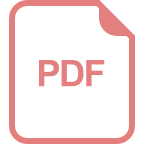
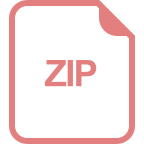
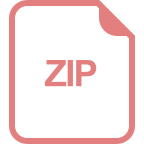
收起资源包目录



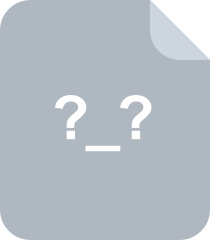
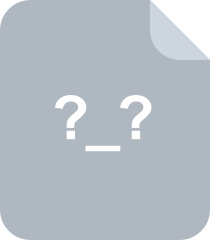
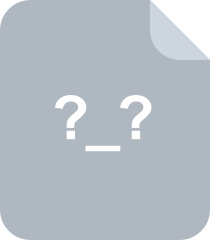
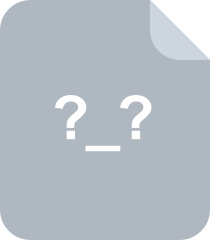
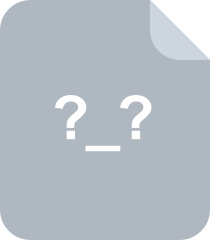
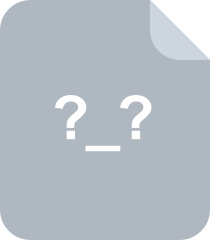
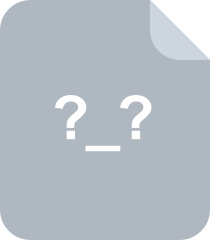
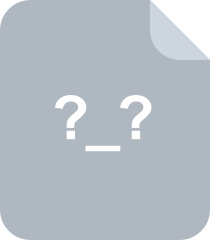

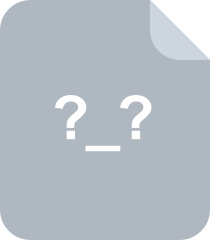
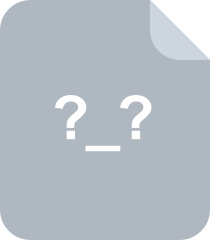
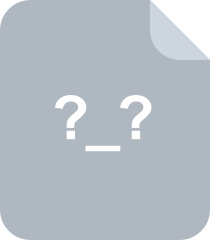
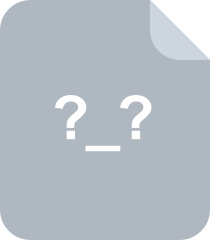
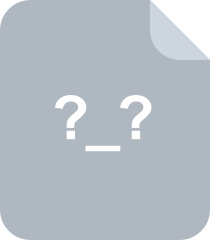
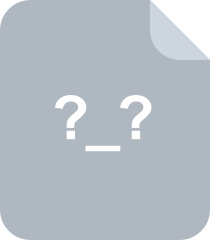
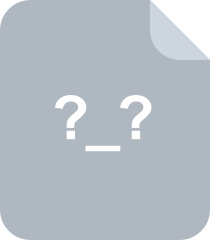
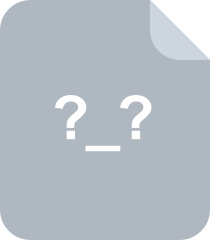
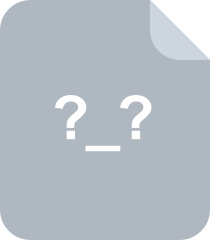
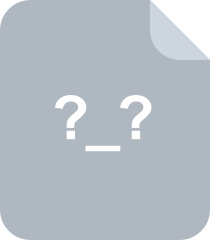
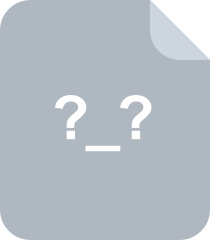
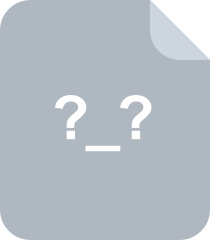
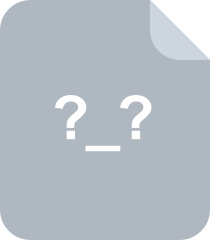
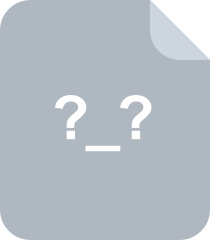
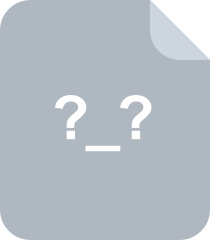
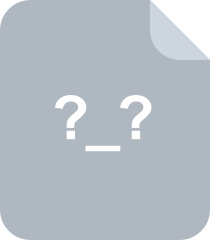
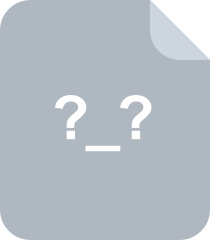
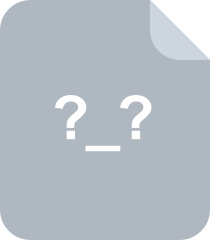
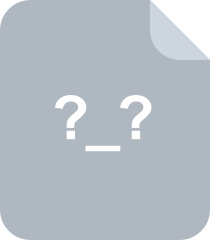
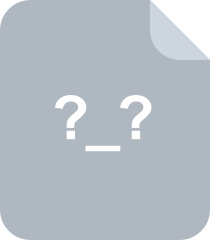
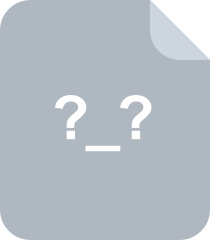
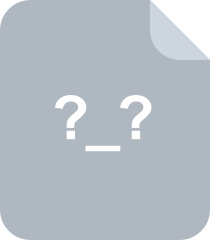
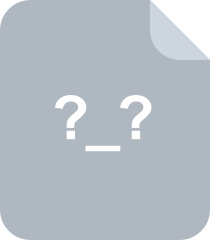
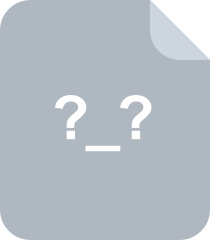
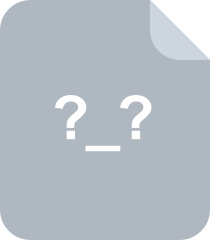
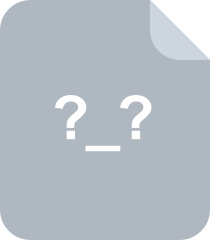
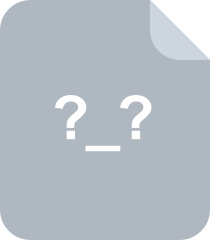
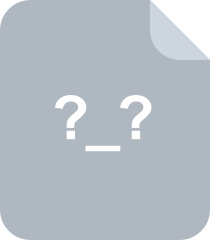
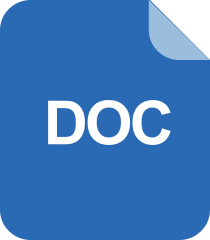
共 37 条
- 1
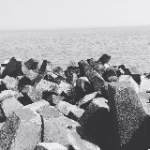
木头1233
- 粉丝: 281
- 资源: 631

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

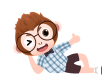
最新资源
- W3CSchool全套Web开发手册中文CHM版15MB最新版本
- Light Table 的 Python 语言插件.zip
- UIkit中文帮助文档pdf格式最新版本
- kubernetes 的官方 Python 客户端库.zip
- 公开整理-2024年全国产业园区数据集.csv
- Justin Seitz 所著《Black Hat Python》一书的源代码 代码已完全转换为 Python 3,重新格式化以符合 PEP8 标准,并重构以消除涉及弃用库实现的依赖性问题 .zip
- java炸弹人游戏.zip学习资料程序资源
- Jay 分享的一些 Python 代码.zip
- 彩色形状的爱心代码.zip学习资料程序资源
- SQLAlchemy库:Python数据库操作的全方位指南
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


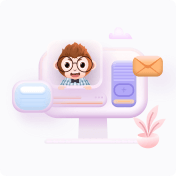
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
前往页