#include "pcm_resample.h"
int main2()
{
//初始化重采样参数
//音频重采样 上下文初始化
SwrContext *asc = NULL; //重采样上下文
AVFrame *pcm = NULL; //重采样输出空间
uint8_t *outs_new[2];
int inSamplerate = 48000, outSamplerate = 32000,ret = 0;
AVSampleFormat inSamplefmt = AV_SAMPLE_FMT_S16;
AVSampleFormat outSamplefmt = AV_SAMPLE_FMT_S16;
int channels = 2;
outs_new[0] = (uint8_t *)malloc(4096);//len 为4096
outs_new[1] = (uint8_t *)malloc(4096);
asc = swr_alloc_set_opts(asc, av_get_default_channel_layout(channels), outSamplefmt, inSamplerate,
av_get_default_channel_layout(channels), inSamplefmt, outSamplerate, 0, 0);
if (!asc)
{
printf("swr_alloc_set_opts failed\n");
return -1;
}
ret = swr_init(asc);
if (ret != 0)
{
printf("swr_init failed\n");
return -1;
}
printf("重采样初始化成功\n");
//重采样输出分配空间
pcm = av_frame_alloc();
pcm->format = outSamplefmt;
pcm->channels = channels;
pcm->channel_layout = av_get_default_channel_layout(channels);
pcm->nb_samples = 1024; //一帧音频一通道的采样数量
ret = av_frame_get_buffer(pcm, 0);//给pcm分配存储空间
if (ret != 0)
{
printf("重采样分配输出空间失败\n");
return -1;
}
FILE *fb_in = fopen("in_data48Khz.pcm", "rb");
FILE *fb_out = fopen("out_data32Khz.pcm", "wb");
int nRead = 0, len_swr = 0;
char indata1[4096] = {0};
//进行重采样数据
const uint8_t *indata[AV_NUM_DATA_POINTERS] = { 0 };
indata[0] = (const uint8_t*)indata1;
int len = swr_convert(asc, outs_new, pcm->nb_samples, indata, pcm->nb_samples);
pcm->data[0] = outs_new[0];
pcm->data[1] = outs_new[1];
while (1)
{
nRead = fread((void*)indata[0], 1, 4096, fb_in);
if (nRead == 0)
break;
len_swr = swr_convert(asc, outs_new, pcm->nb_samples, (const uint8_t**)(&indata), pcm->nb_samples);
printf("len_swr:%d\n", len_swr);
fwrite(outs_new[0], 1, len_swr*4, fb_out);
}
if (pcm != NULL)
{
av_frame_free(&pcm);
pcm = NULL;
}
if (asc != NULL)
{
swr_free(&asc);
asc = NULL;
}
if (outs_new[1] != NULL)
{
free(outs_new[1]);
outs_new[1] = NULL;
}
if (outs_new[0] != NULL)
{
free(outs_new[0]);
outs_new[0] = NULL;
}
return 0;
}
/*函数名称:pcm_resample_init
*函数功能:初始化pcm重采样参数
*传入参数:int insample:输入数据采样频率, int outsample:输出采样频率
*函数返回值:成功返回0,失败返回-1
*函数作者:吴豪乐
*日期:2021年1月5日
*/
int pcm_resample::pcm_resample_init(int insample, int outsample)
{
//音频重采样 上下文初始化
inSamplerate = insample;
outSamplerate = outsample;
int ret = 0;
inSamplefmt = AV_SAMPLE_FMT_S16;
outSamplefmt = AV_SAMPLE_FMT_S16;
channels = 2;
dst_nb_channels = av_get_channel_layout_nb_channels(AV_CH_LAYOUT_STEREO);
printf("dst_nb_channels:%d\n", dst_nb_channels);
//outs_new[0] = (uint8_t *)malloc(4096);//len 为4096
//outs_new[1] = (uint8_t *)malloc(4096);
asc = swr_alloc_set_opts(asc, av_get_default_channel_layout(channels), outSamplefmt, outSamplerate,
av_get_default_channel_layout(channels), inSamplefmt, inSamplerate, 0, 0);
if (!asc)
{
printf("swr_alloc_set_opts failed\n");
return -1;
}
#if 0
/* set options */
int64_t src_ch_layout = AV_CH_LAYOUT_STEREO, dst_ch_layout = AV_CH_LAYOUT_STEREO;//AV_CH_LAYOUT_SURROUND;
av_opt_set_int(asc, "in_channel_layout", src_ch_layout, 0);
av_opt_set_int(asc, "in_sample_rate", inSamplerate, 0);
av_opt_set_sample_fmt(asc, "in_sample_fmt", inSamplefmt, 0);
av_opt_set_int(asc, "out_channel_layout", dst_ch_layout, 0);
av_opt_set_int(asc, "out_sample_rate", outSamplerate, 0);
av_opt_set_sample_fmt(asc, "out_sample_fmt", outSamplefmt, 0);
#endif
ret = swr_init(asc);
if (ret != 0)
{
printf("swr_init failed\n");
return -1;
}
indata[0] = (const uint8_t*)indata1;
//重采样输出分配空间
pcm = av_frame_alloc();
pcm->format = outSamplefmt;
pcm->channels = channels;
pcm->channel_layout = av_get_default_channel_layout(channels);
pcm->nb_samples = 1024; //一帧音频一通道的采样数量
ret = av_frame_get_buffer(pcm, 0);//给pcm分配存储空间
if (ret != 0)
{
printf("重采样分配输出空间失败\n");
return -1;
}
printf("pcm_resample_init 重采样初始化成功, inSamplerate:%d, outSamplerate:%d\n", inSamplerate, outSamplerate);
src_nb_samples = 1024;
//计算理论重采样后的数据量
/* compute the number of converted samples: buffering is avoided
* ensuring that the output buffer will contain at least all the
* converted input samples */
//e=av_rescale_rnd(a,b,c,d) => e=a*b/c
max_dst_nb_samples = dst_nb_samples =
av_rescale_rnd(src_nb_samples, outSamplerate, inSamplerate, AV_ROUND_UP);//这是按比例来求取的目标通道的采样数据:src_num/src_rate = dst_num/dst_rate => dst_num = src_num * dst_rate/src_rate
printf("111max_dst_nb_samples:%d, dst_nb_samples:%d\n", max_dst_nb_samples, dst_nb_samples);
//为重采样后的接收数据申请缓存
ret = av_samples_alloc_array_and_samples(&dst_data, &dst_linesize, 2,
dst_nb_samples, outSamplefmt, 0);
if (ret < 0) {
printf("Could not allocate destination samples\n");
return -1;
}
return 0;
}
/*函数名称:pcm_resample_run
*函数功能:开始进行重采样,每次重采样数据大小为4096字节
*传入参数:char *in_data:输入数据, int len_in:输入数据的大小(字节), char *out_data:输出数据, int len_out:输出数据的大小(字节)
*函数返回值:成功返回0,失败返回-1
*函数作者:吴豪乐
*日期:2021年1月5日
*/
int pcm_resample::pcm_resample_run(char *in_data, int len_in, char *out_data, int *len_out)
{
if (in_data == NULL || out_data == NULL || len_out == NULL)
{
printf("pcm_resample_run in_data or out_data error\n");
getchar();
return -1;
}
if (len_in < 4096)
{
printf("pcm_resample_run len_in or len_out error\n");
getchar();
return -1;
}
int ret = 0;
//拷贝输入数据
memcpy(indata1, in_data, 4096);
//计算实际重采样后得到的数据大小以及重新分配缓存
/* compute destination number of samples */
dst_nb_samples = av_rescale_rnd(swr_get_delay(asc, inSamplerate) +
src_nb_samples, outSamplerate, inSamplerate, AV_ROUND_UP);//这里将重采样时间也计算进去的意思
if (dst_nb_samples > max_dst_nb_samples) {
av_freep(&dst_data[0]);
ret = av_samples_alloc(dst_data, &dst_linesize, 2,
dst_nb_samples, outSamplefmt, 1);
if (ret < 0)
{
printf("av_samples_alloc error\n");
return -1;
}
max_dst_nb_samples = dst_nb_samples;
}
printf("222max_dst_nb_samples:%d, dst_nb_samples:%d\n", max_dst_nb_samples, dst_nb_samples);
int len_swr = 0;
len_swr = swr_convert(asc, dst_data, dst_nb_samples, (const uint8_t**)(&indata), pcm->nb_samples);
if (len_swr < 0)
{
printf("swr_convert error\n");
return -1;
}
dst_bufsize = av_samples_get_buffer_size(&dst_linesize, dst_nb_channels,
len_swr, outSamplefmt, 1);
if (dst_bufsize < 0) {
printf("av_samples_get_buffer_size error\n");
return -1;
}
printf("dst_bufsize:%d, dst_nb_samples:%d, pcm->nb_samples:%d\n", dst_bufsize, dst_nb_samples, pcm->nb_samples);
printf("outSamplerate:%d, inSamplerate:%d\n", outSamplerate, inSamplerate);
//getchar();
*len_out = dst_bufsize;
memcpy(out_data, dst_data[0], dst_bufsize);
return 0;
}
/*函数名称:pcm_resample_release
*函数功能:释放资源
*传入参数:无
*函数返回值:无
*函数作者:吴豪乐
*日期:2021年1月5日
*/
void pcm_resample::pcm_resample_release(void)
{
if (pcm != NULL)
{
av_frame_free(&pcm);
pcm = NULL;
}
if (asc != NULL)
{
swr_free(&asc);
asc = NULL;
}
if (dst_data != NULL)
{
av_freep(&dst_data[0]);
dst_data = NULL;
}
return;
}
pcm_resample::~pcm_resample()
{
pcm_resample_release();
}
int main()
{
FILE *fb_in = fopen("in_data48Khz.pcm", "rb");
FILE *fb_out = fopen("out_data32Khz.pcm", "wb");
int nRead = 0, len_swr = 0;
//初始化重采样
pcm_resample obj_pcm_resample;
obj_pcm_resample.pcm_resample_init(48000, 32000);
//进行重采样数据
char data_read[8192]
没有合适的资源?快使用搜索试试~ 我知道了~
资源详情
资源评论
资源推荐
收起资源包目录

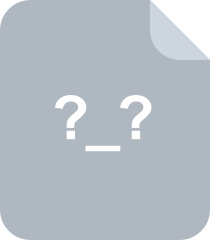
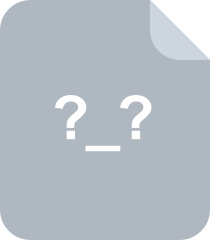
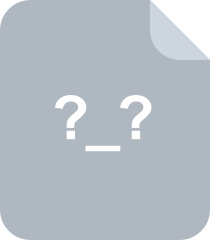
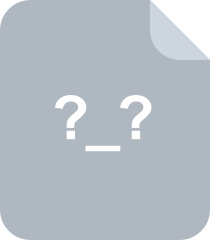
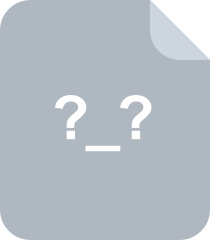
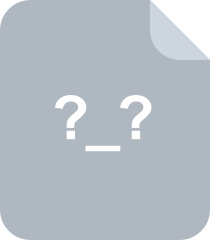
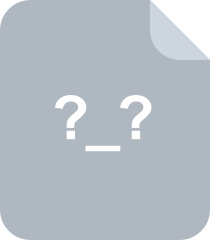
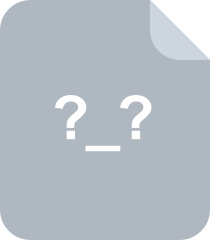
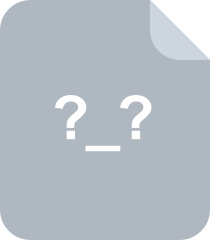
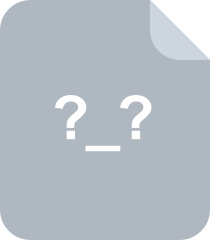
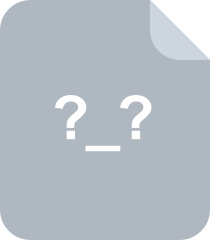
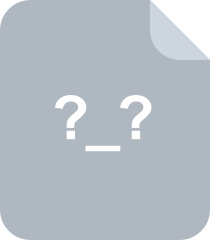
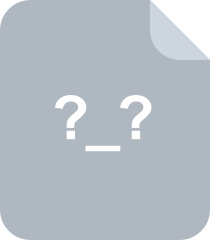
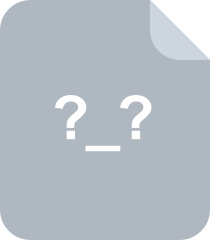
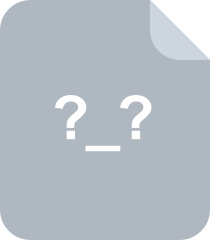
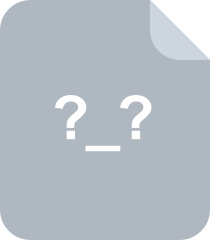
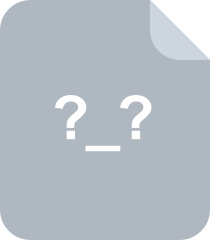
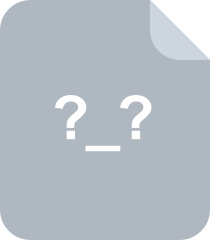
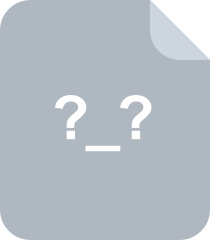
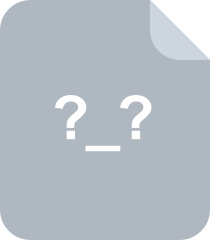
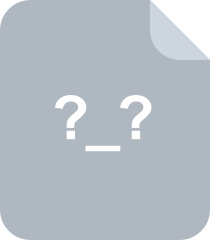
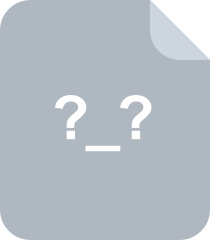
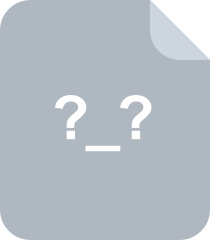
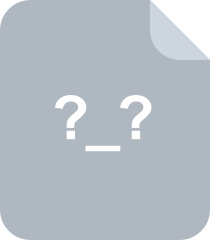
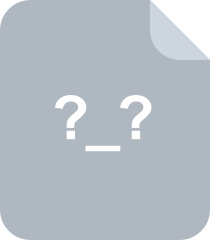
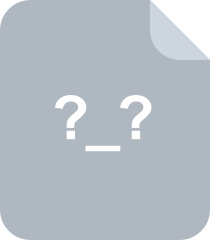
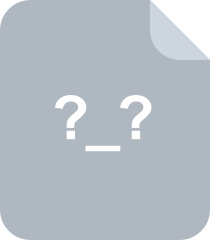
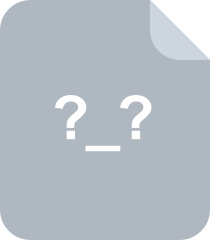
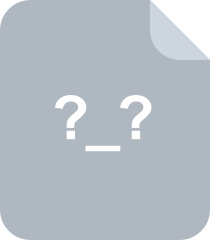
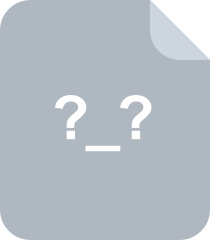
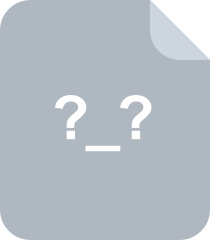
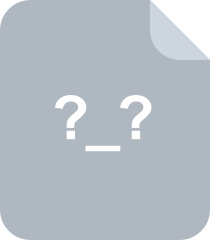
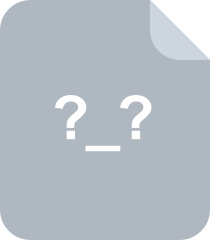
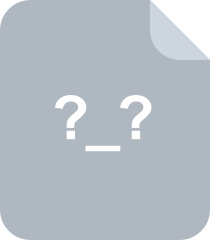
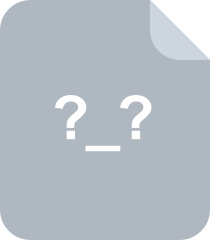
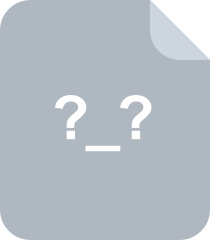
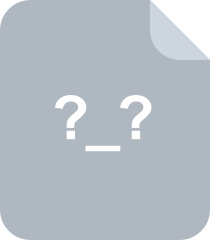
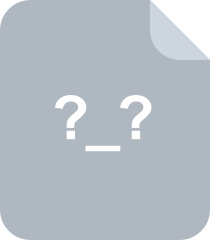
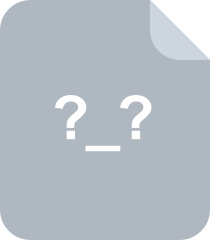
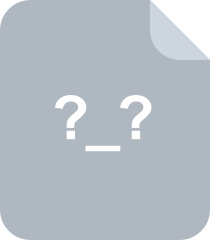
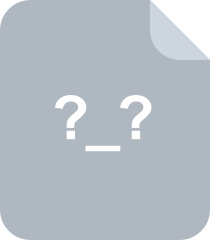
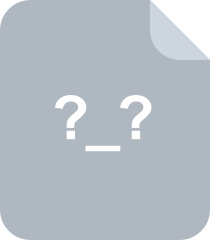
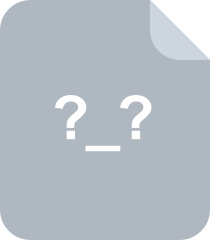
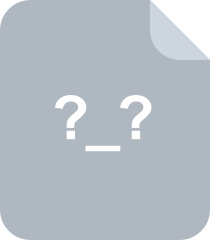
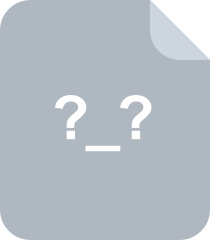
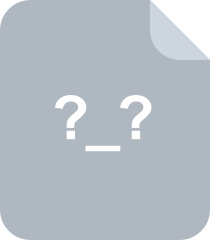
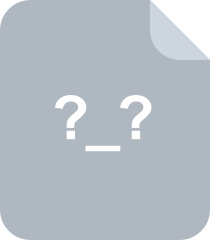
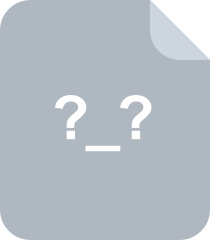
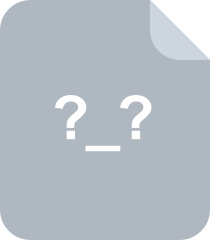
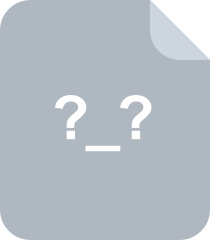
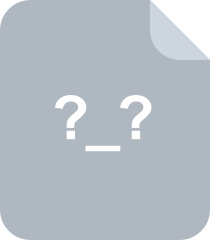
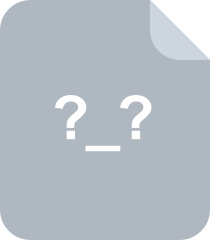
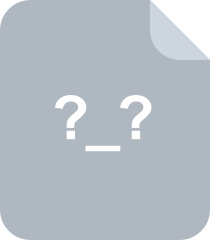
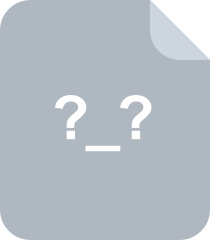
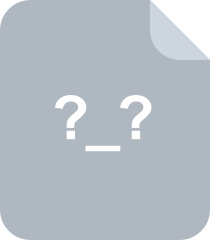
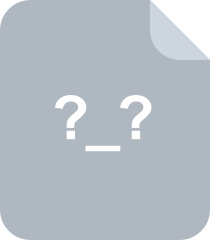
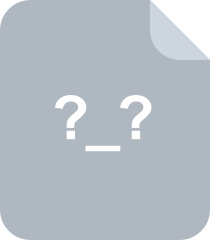
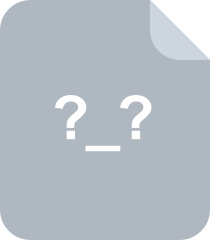
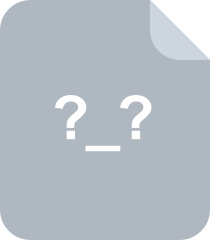
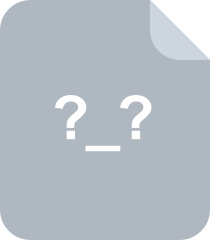
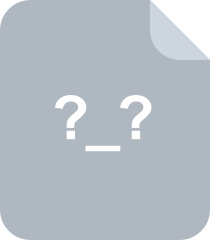
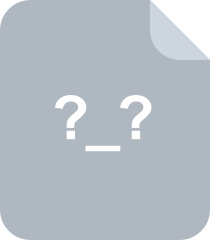
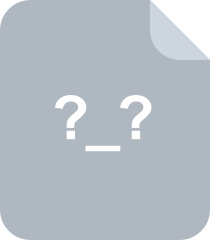
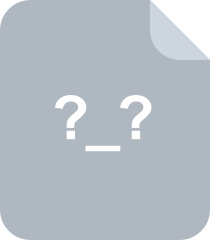
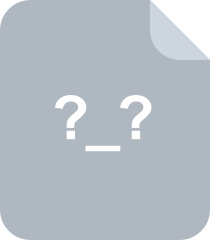
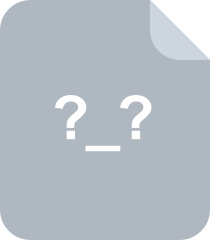
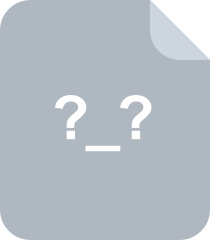
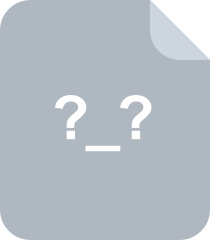
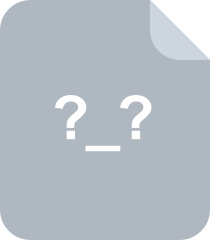
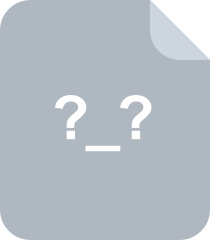
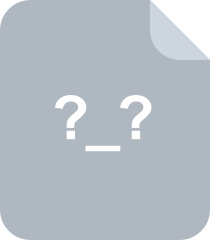
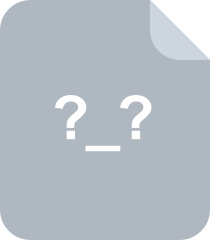
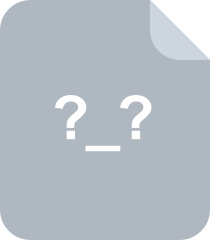
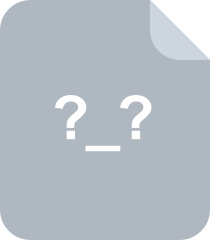
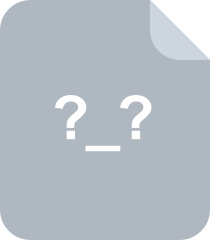
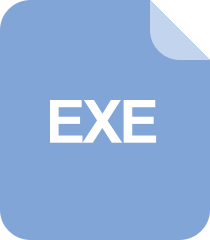
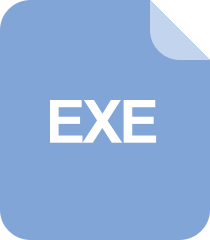
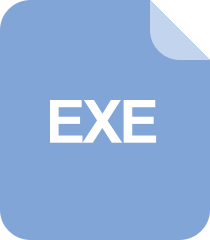
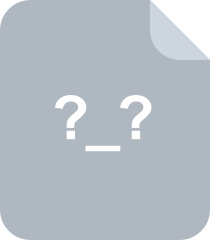
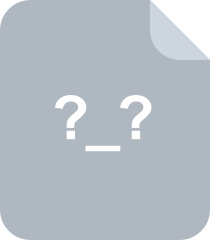
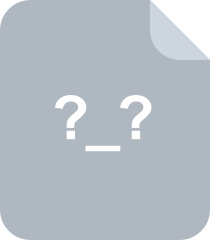
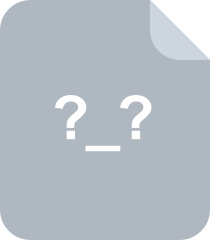
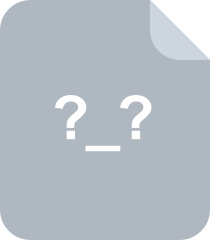
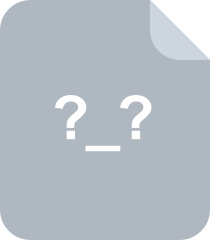
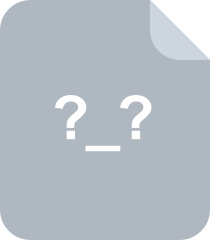
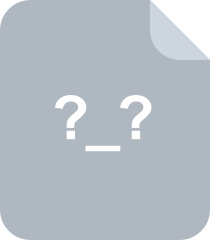
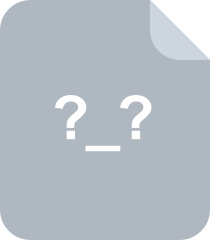
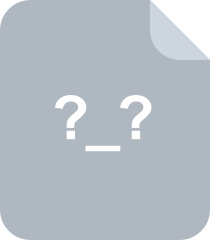
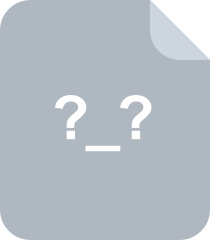
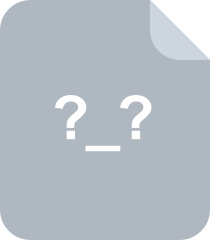
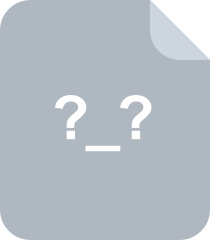
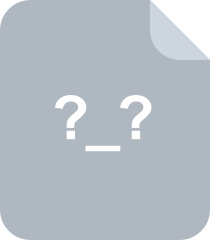
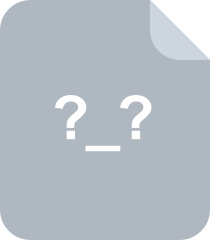
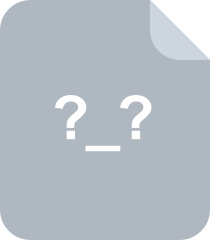
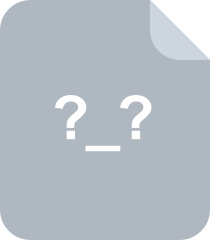
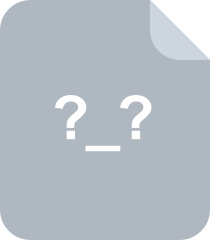
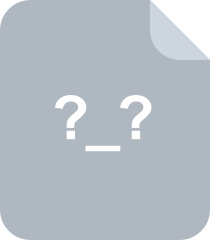
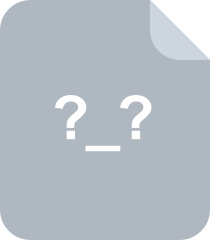
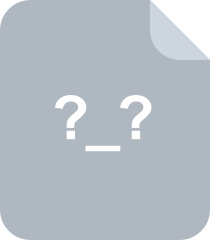
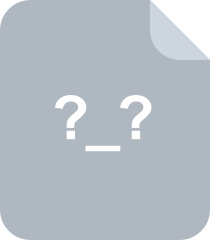
共 355 条
- 1
- 2
- 3
- 4
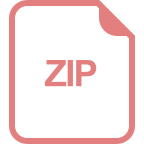
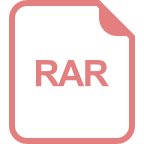
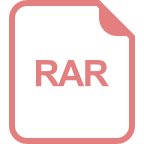
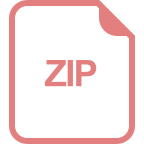
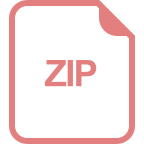
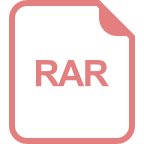
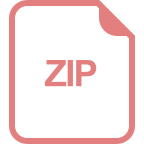
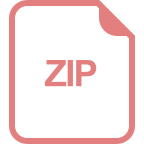
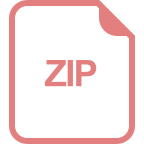
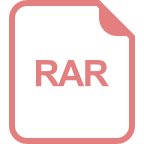
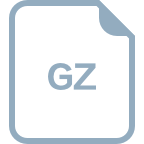
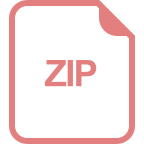
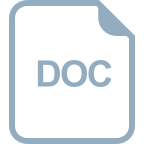
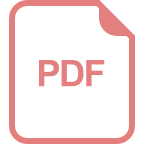
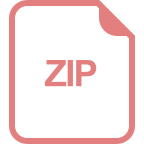

吴豪乐工作室
- 粉丝: 20
- 资源: 11
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

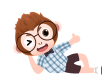
安全验证
文档复制为VIP权益,开通VIP直接复制

评论5