package com.legend.word.docx;
import com.legend.word.entity.CustomElementMeta;
import com.legend.word.utils.MathMlUtils;
import com.microsoft.schemas.vml.CTGroup;
import com.microsoft.schemas.vml.CTShape;
import org.apache.poi.openxml4j.opc.PackagePart;
import org.apache.poi.xwpf.converter.core.*;
import org.apache.poi.xwpf.converter.core.styles.XWPFStylesDocument;
import org.apache.poi.xwpf.converter.core.utils.DxaUtil;
import org.apache.poi.xwpf.converter.core.utils.StringUtils;
import org.apache.poi.xwpf.converter.core.utils.XWPFRunHelper;
import org.apache.poi.xwpf.converter.core.utils.XWPFTableUtil;
import org.apache.poi.xwpf.usermodel.*;
import org.apache.xmlbeans.XmlCursor;
import org.apache.xmlbeans.XmlException;
import org.apache.xmlbeans.XmlObject;
import org.apache.xmlbeans.XmlTokenSource;
import org.openxmlformats.schemas.drawingml.x2006.main.CTGraphicalObject;
import org.openxmlformats.schemas.drawingml.x2006.main.CTGraphicalObjectData;
import org.openxmlformats.schemas.drawingml.x2006.picture.CTPicture;
import org.openxmlformats.schemas.drawingml.x2006.wordprocessingDrawing.*;
import org.openxmlformats.schemas.officeDocument.x2006.math.CTOMath;
import org.openxmlformats.schemas.officeDocument.x2006.math.CTOMathPara;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.*;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.HdrDocument.Factory;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.STMerge.Enum;
import org.xml.sax.ContentHandler;
import java.io.IOException;
import java.math.BigInteger;
import java.util.*;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* @author legend
* @date 2021年5月25日
*/
public abstract class CustomXWPFDocumentVisitor<T, O extends Options, E extends IXWPFMasterPage> implements IMasterPageHandler<E> {
private static final Logger LOGGER = Logger.getLogger(XWPFDocumentVisitor.class.getName());
protected static final String WORD_MEDIA = "word/media/";
protected final XWPFDocument document;
public final MasterPageManager masterPageManager;
private XWPFHeader currentHeader;
private XWPFFooter currentFooter;
protected final XWPFStylesDocument stylesDocument;
protected final O options;
private boolean pageBreakOnNextParagraph;
protected boolean processingTotalPageCountField = false;
protected boolean totalPageFieldUsed = false;
private Map<Integer, ListContext> listContextMap;
//额外新增
private ContentHandler contentHandler;
//额外新增
private boolean isScanHeading;
public CustomXWPFDocumentVisitor(XWPFDocument document, O options, ContentHandler contentHandler) throws Exception {
this.document = document;
this.options = options;
this.stylesDocument = this.createStylesDocument(document);
this.masterPageManager = new MasterPageManager(document.getDocument(), this);
//额外新增
this.contentHandler = contentHandler;
}
protected XWPFStylesDocument createStylesDocument(XWPFDocument document) throws XmlException, IOException {
return new XWPFStylesDocument(document);
}
public XWPFStylesDocument getStylesDocument() {
return this.stylesDocument;
}
public O getOptions() {
return this.options;
}
public MasterPageManager getMasterPageManager() {
return this.masterPageManager;
}
public Map start() throws Exception {
//T container = this.startVisitDocument();
List<IBodyElement> bodyElements = this.document.getBodyElements();
// this.visitBodyElements(bodyElements, container);
return this.visitBodyElements(bodyElements, null);
//this.endVisitDocument();
}
protected abstract T startVisitDocument() throws Exception;
protected abstract void endVisitDocument() throws Exception;
protected Map visitBodyElements(List<IBodyElement> bodyElements, T container) throws Exception {
if (!this.masterPageManager.isInitialized()) {
this.masterPageManager.initialize();
}
CustomContentHandler customContentHandler = ((CustomContentHandler)contentHandler);
XWPFStyles styles = document.getStyles();
Map dataMap = new LinkedHashMap<String, String>();
for(int i = 0; i < bodyElements.size(); ++i) {
IBodyElement bodyElement = (IBodyElement)bodyElements.get(i);
CustomElementMeta customElementMeta;
switch(bodyElement.getElementType()) {
case PARAGRAPH:
//System.out.println("--开始---");
//String style = ((XWPFParagraph) bodyElement).getStyle();
String style = ((XWPFParagraph) bodyElement).getStyle();
if(StringUtils.isNotEmpty(style)){
style = styles.getStyle(((XWPFParagraph) bodyElement).getStyle()).getCTStyle().getName().getVal();
}
//System.out.println("标题的级别:"+style);
XWPFParagraph paragraph = (XWPFParagraph)bodyElement;
customElementMeta = this.visitParagraph(paragraph, i, container, style);
if(customContentHandler.isClearData()){
//组装数据
dataMap.put(customElementMeta,customContentHandler.getStringData());
//清理数据
customContentHandler.clearData();
}
break;
case TABLE:
style = ((XWPFTable)bodyElement).getStyleID();
//System.out.println("标题的级别:"+style);
customContentHandler.setClearData(false);
this.visitTable((XWPFTable)bodyElement, i, container);
customContentHandler.setClearData(true);
//设置类型为table
customElementMeta = new CustomElementMeta(CustomElementMeta.ELEMENT_TYPE_TABLE,i);
if(!isScanHeading){
customElementMeta.setMainTitle(true);
}
//组装数据
dataMap.put(customElementMeta,customContentHandler.getStringData());
//清理数据
((CustomContentHandler)contentHandler).clearData();
}
}
return dataMap;
}
protected CustomElementMeta visitParagraph(XWPFParagraph paragraph, int index, T container,String elementType) throws Exception {
if (this.isWordDocumentPartParsing()) {
this.masterPageManager.update(paragraph.getCTP());
}
if (this.pageBreakOnNextParagraph) {
this.pageBreak();
}
this.pageBreakOnNextParagraph = false;
ListItemContext itemContext = null;
CTNumPr originalNumPr = this.stylesDocument.getParagraphNumPr(paragraph);
CTNumPr numPr = this.getNumPr(originalNumPr);
if (numPr != null) {
XWPFNum num = this.getXWPFNum(numPr);
if (num != null) {
XWPFAbstractNum abstractNum = this.getXWPFAbstractNum(num);
CTDecimalNumber ilvl = numPr.getIlvl();
int level = ilvl != null ? ilvl.getVal().intValue() : 0;
CTLvl lvl = abstractNum.getAbstractNum().getLvlArray(level);
if (lvl != null) {
ListContext listContext = this.getListContext(originalNumPr.getNumId().getVal().intValue());
itemContext = listContext.addItem(lvl);
}
}
}
T paragraphContainer = this.startVisitParagraph(paragraph, itemContext, container);
CustomElementMeta customElementMeta = this.visitParagraphBody(paragraph, index, paragraphContainer, elementType);
this.endVisitParagraph(paragraph, container, paragraphContainer);
return customElementMeta;
}
private CTNumPr getNumPr(CTNumPr numPr) {
if (numPr != null) {
XWPFNum

@Legend
- 粉丝: 3
- 资源: 2
最新资源
- 圣诞树项目中的硬件和MATLAB实现指南
- 免费的PDF/图片转excel工具-调用百度OCR API接口
- HTML与CSS实现简单圣诞树网页
- 用Python实现带装饰效果的圣诞树打印功能
- HTML与CSS创建节日主题的圣诞树网页
- Web开发全栈学习指南与核心技术解析
- UML软件建模酒店视觉AI解决方案
- Qml地图应用示例,包括地图显示、区域线、获取鼠标位置等源程序
- Screenshot_20241224_015626_K.jpg
- MySQL安装环境配置指南:从系统准备到性能优化的全流程详解
- MySQL操作速查手册:数据库管理与性能优化
- Python代码实现带装饰的圣诞树控制台输出
- ls.mobileconfig
- HTML-Christmas Tree Code
- HCIA..............
- 微流控芯片底..克力.STEP
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


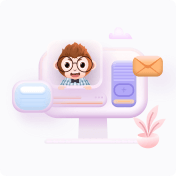
- 1
- 2
- 3
前往页