# core-js
[](#raising-funds) [](#raising-funds) [](https://gitter.im/zloirock/core-js?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge) [](https://www.npmjs.com/package/core-js) [](http://npm-stat.com/charts.html?package=core-js&author=&from=2014-11-18) [](https://travis-ci.org/zloirock/core-js) [](https://david-dm.org/zloirock/core-js?type=dev)
## As advertising: the author is looking for a good job :)
## [core-js@3, babel and a look into the future](https://github.com/zloirock/core-js/tree/master/docs/2019-03-19-core-js-3-babel-and-a-look-into-the-future.md)
## Raising funds
`core-js` isn't backed by a company, so the future of this project depends on you. Become a sponsor or a backer [**on Open Collective**](https://opencollective.com/core-js) or [**on Patreon**](https://www.patreon.com/zloirock) if you are interested in `core-js`.
---
<a href="https://opencollective.com/core-js/sponsor/0/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/0/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/1/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/1/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/2/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/2/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/3/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/3/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/4/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/4/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/5/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/5/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/6/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/6/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/7/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/7/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/8/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/8/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/9/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/9/avatar.svg"></a>
---
<a href="https://opencollective.com/core-js#backers" target="_blank"><img src="https://opencollective.com/core-js/backers.svg?width=890"></a>
---
**It's documentation for obsolete `core-js@2`. If you looking documentation for actual `core-js` version, please, check [this branch](https://github.com/zloirock/core-js/tree/master).**
Modular standard library for JavaScript. Includes polyfills for [ECMAScript 5](#ecmascript-5), [ECMAScript 6](#ecmascript-6): [promises](#ecmascript-6-promise), [symbols](#ecmascript-6-symbol), [collections](#ecmascript-6-collections), iterators, [typed arrays](#ecmascript-6-typed-arrays), [ECMAScript 7+ proposals](#ecmascript-7-proposals), [setImmediate](#setimmediate), etc. Some additional features such as [dictionaries](#dict) or [extended partial application](#partial-application). You can require only needed features or use it without global namespace pollution.
[*Example*](http://goo.gl/a2xexl):
```js
Array.from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3]
'*'.repeat(10); // => '**********'
Promise.resolve(32).then(x => console.log(x)); // => 32
setImmediate(x => console.log(x), 42); // => 42
```
[*Without global namespace pollution*](http://goo.gl/paOHb0):
```js
var core = require('core-js/library'); // With a modular system, otherwise use global `core`
core.Array.from(new core.Set([1, 2, 3, 2, 1])); // => [1, 2, 3]
core.String.repeat('*', 10); // => '**********'
core.Promise.resolve(32).then(x => console.log(x)); // => 32
core.setImmediate(x => console.log(x), 42); // => 42
```
### Index
- [Usage](#usage)
- [Basic](#basic)
- [CommonJS](#commonjs)
- [Custom build](#custom-build-from-the-command-line)
- [Supported engines](#supported-engines)
- [Features](#features)
- [ECMAScript 5](#ecmascript-5)
- [ECMAScript 6](#ecmascript-6)
- [ECMAScript 6: Object](#ecmascript-6-object)
- [ECMAScript 6: Function](#ecmascript-6-function)
- [ECMAScript 6: Array](#ecmascript-6-array)
- [ECMAScript 6: String](#ecmascript-6-string)
- [ECMAScript 6: RegExp](#ecmascript-6-regexp)
- [ECMAScript 6: Number](#ecmascript-6-number)
- [ECMAScript 6: Math](#ecmascript-6-math)
- [ECMAScript 6: Date](#ecmascript-6-date)
- [ECMAScript 6: Promise](#ecmascript-6-promise)
- [ECMAScript 6: Symbol](#ecmascript-6-symbol)
- [ECMAScript 6: Collections](#ecmascript-6-collections)
- [ECMAScript 6: Typed Arrays](#ecmascript-6-typed-arrays)
- [ECMAScript 6: Reflect](#ecmascript-6-reflect)
- [ECMAScript 7+ proposals](#ecmascript-7-proposals)
- [stage 4 proposals](#stage-4-proposals)
- [stage 3 proposals](#stage-3-proposals)
- [stage 2 proposals](#stage-2-proposals)
- [stage 1 proposals](#stage-1-proposals)
- [stage 0 proposals](#stage-0-proposals)
- [pre-stage 0 proposals](#pre-stage-0-proposals)
- [Web standards](#web-standards)
- [setTimeout / setInterval](#settimeout--setinterval)
- [setImmediate](#setimmediate)
- [iterable DOM collections](#iterable-dom-collections)
- [Non-standard](#non-standard)
- [Object](#object)
- [Dict](#dict)
- [partial application](#partial-application)
- [Number Iterator](#number-iterator)
- [escaping strings](#escaping-strings)
- [delay](#delay)
- [helpers for iterators](#helpers-for-iterators)
- [Missing polyfills](#missing-polyfills)
- [Changelog](./CHANGELOG.md)
## Usage
### Basic
```
npm i core-js
bower install core.js
```
```js
// Default
require('core-js');
// Without global namespace pollution
var core = require('core-js/library');
// Shim only
require('core-js/shim');
```
If you need complete build for browser, use builds from `core-js/client` path:
* [default](https://raw.githack.com/zloirock/core-js/v2.6.12/client/core.min.js): Includes all features, standard and non-standard.
* [as a library](https://raw.githack.com/zloirock/core-js/v2.6.12/client/library.min.js): Like "default", but does not pollute the global namespace (see [2nd example at the top](#core-js)).
* [shim only](https://raw.githack.com/zloirock/core-js/v2.6.12/client/shim.min.js): Only includes the standard methods.
Warning: if you use `core-js` with the extension of native objects, require all needed `core-js` modules at the beginning of entry point of your application, otherwise, conflicts may occur.
### CommonJS
You can require only needed modules.
```js
require('core-js/fn/set');
require('core-js/fn/array/from');
require('core-js/fn/array/find-index');
Array.from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3]
[1, 2, NaN, 3, 4].findIndex(isNaN); // => 2
// or, w/o global namespace pollution:
var Set = require('core-js/library/fn/set');
var from = require('core-js/library/fn/array/from');
var findIndex = require('core-js/library/fn/array/find-index');
from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3]
findIndex([1, 2, NaN, 3, 4], isNaN); // => 2
```
Available entry points for methods / construc
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
springboot+vue+mysql,该项目主要功能包括宠物信息管理和用户管理,具体涵盖公告信息、宠物信息、房间信息、领养和寄养记录、领养统计、宠物用品管理、订单信息以及发现宠物等,旨在为用户提供全面的宠物管理服务。
资源推荐
资源详情
资源评论
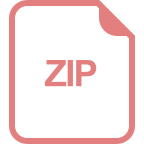
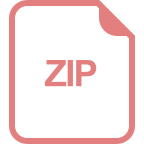
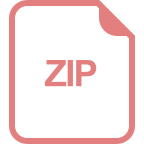
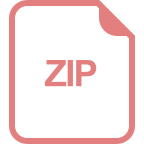
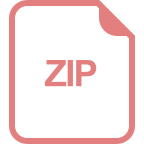
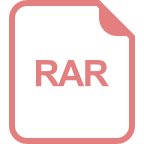
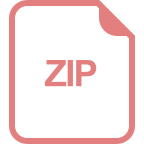
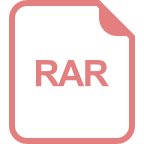
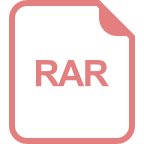
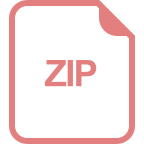
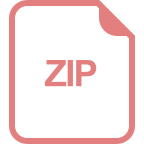
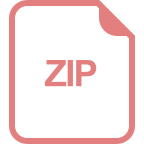
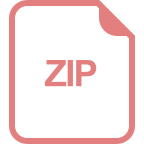
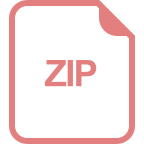
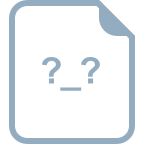
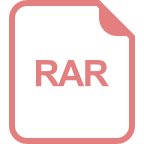
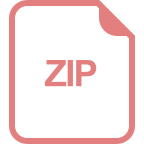
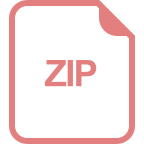
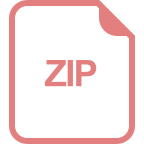
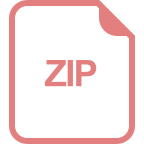
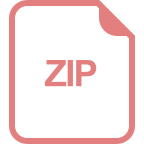
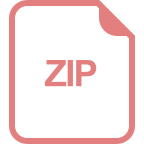
收起资源包目录

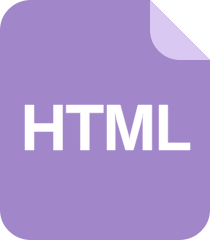
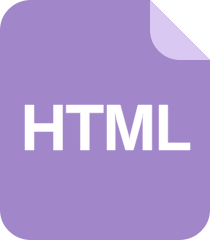
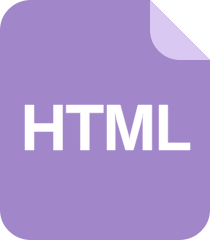
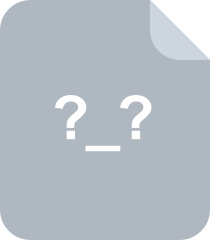
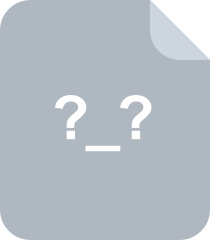
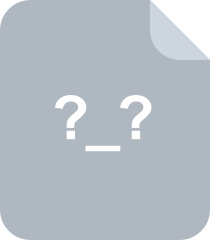
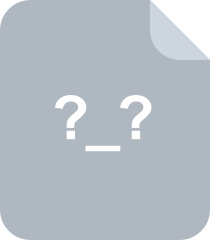
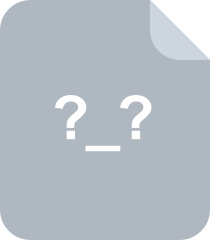
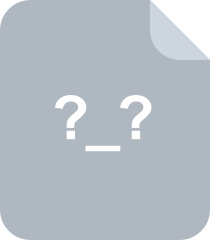
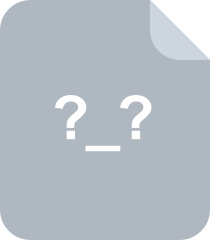
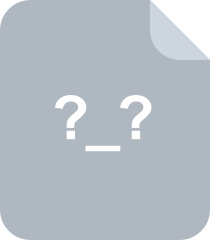
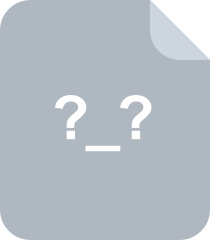
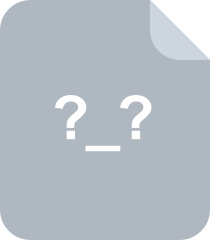
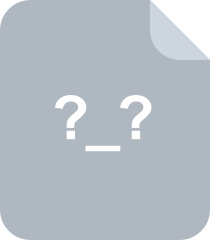
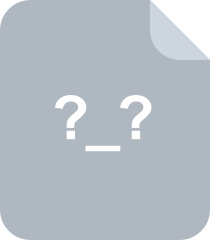
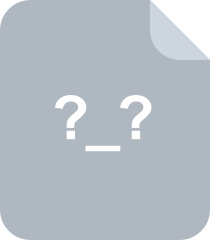
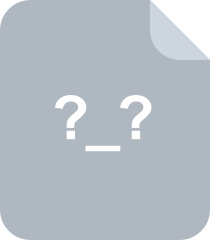
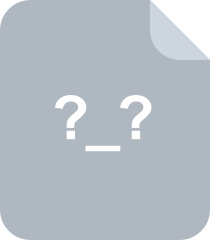
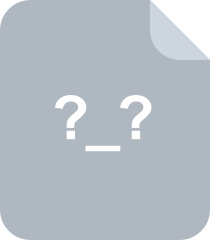
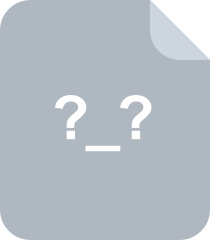
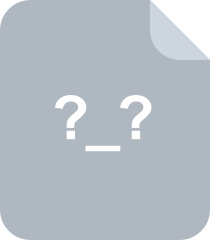
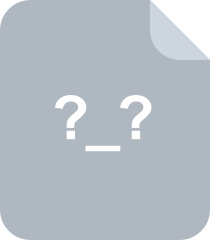
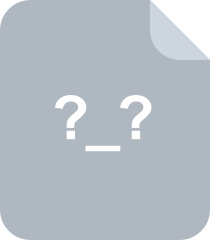
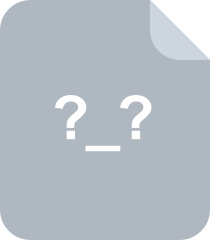
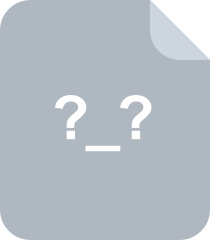
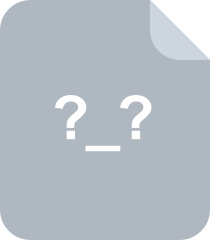
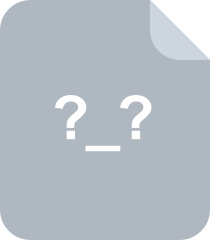
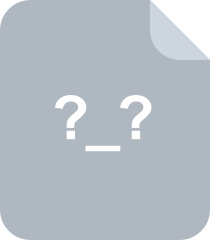
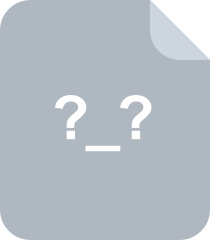
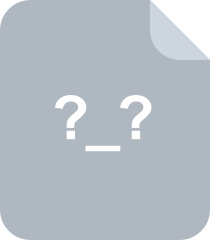
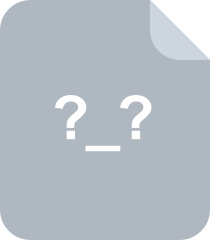
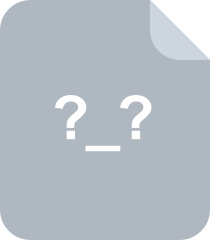
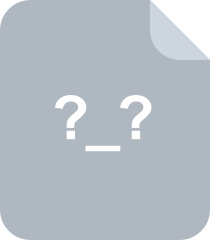
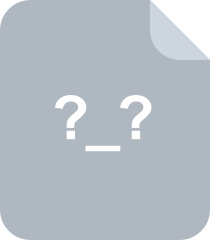
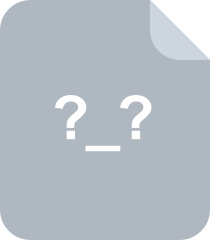
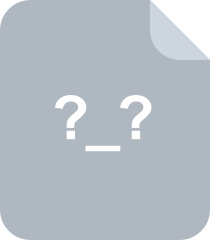
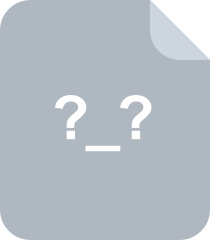
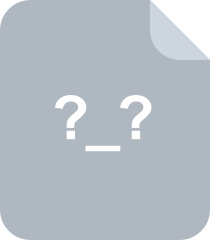
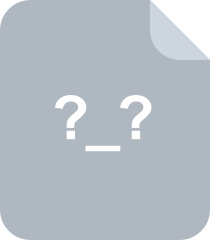
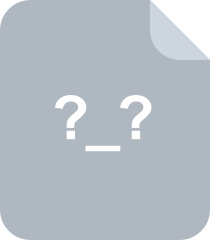
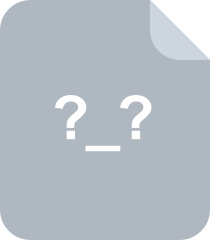
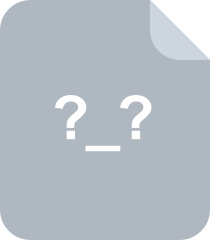
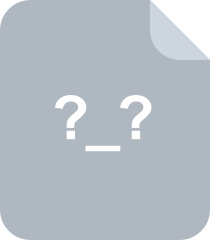
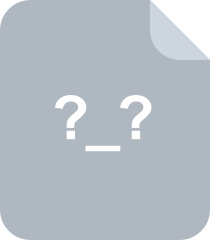
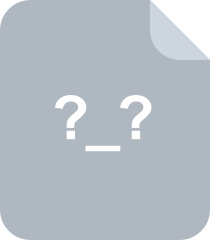
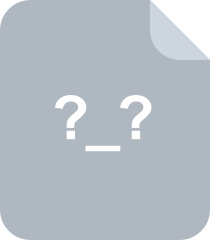
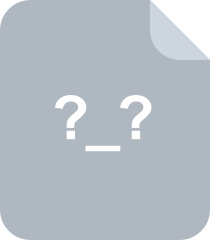
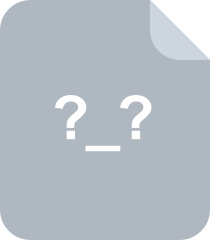
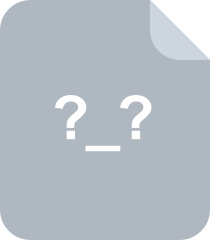
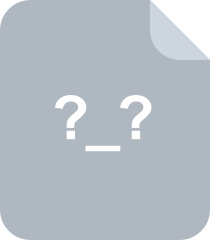
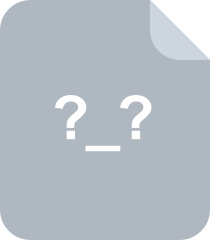
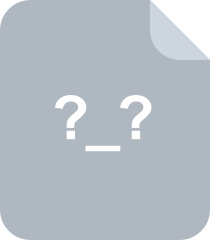
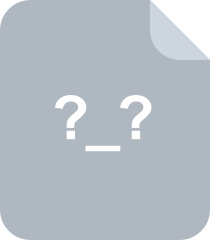
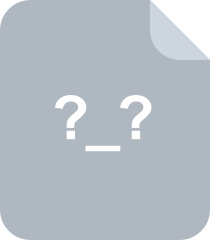
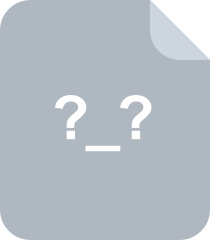
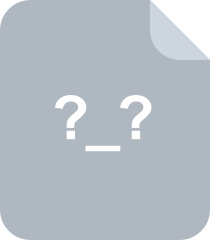
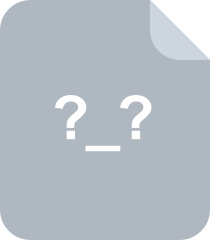
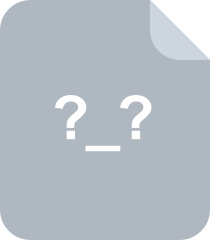
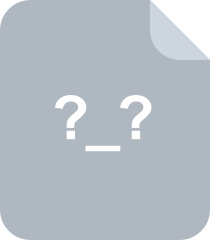
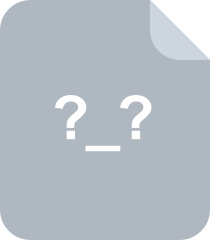
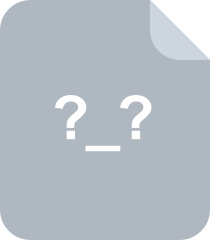
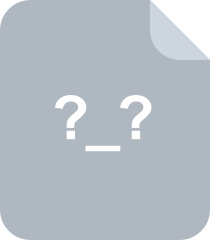
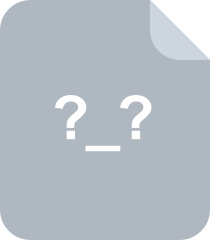
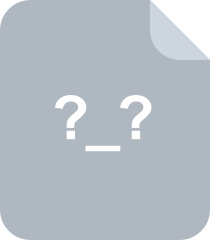
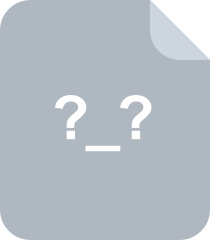
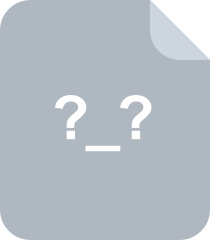
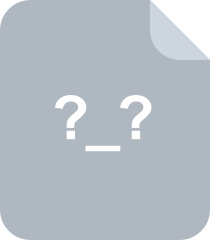
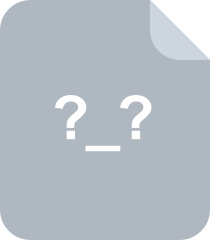
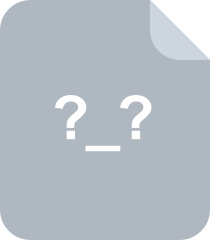
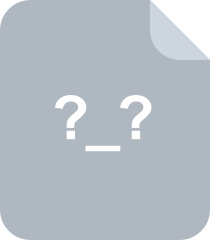
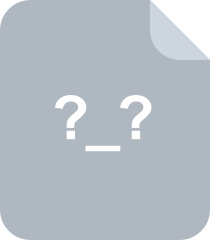
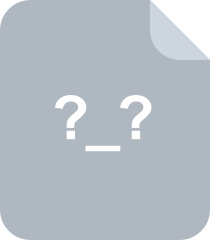
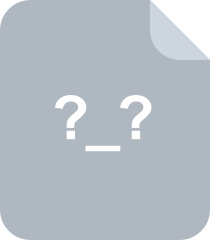
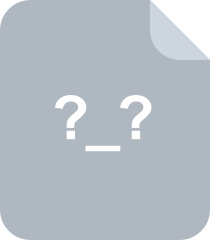
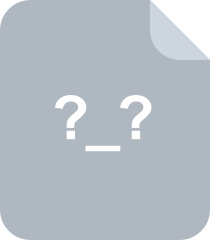
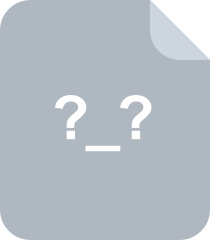
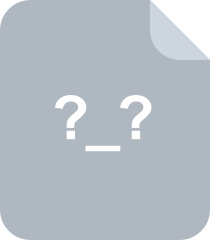
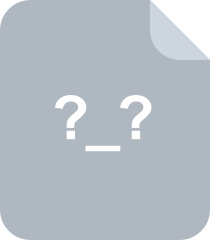
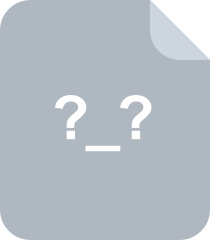
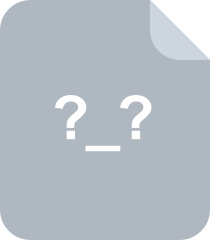
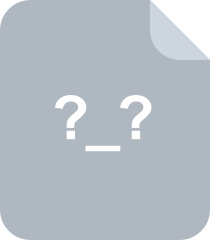
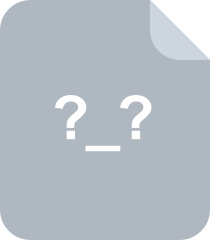
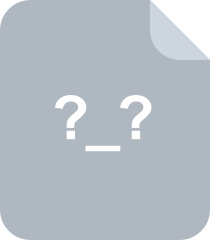
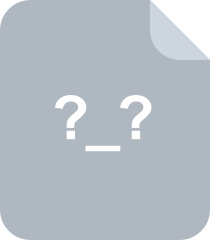
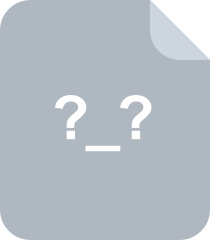
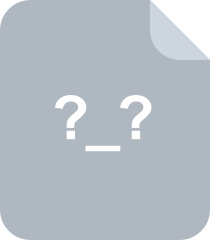
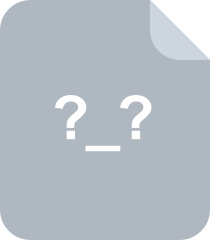
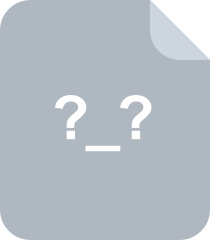
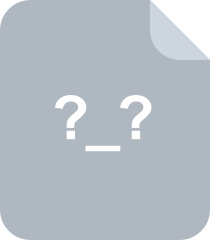
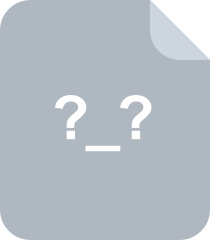
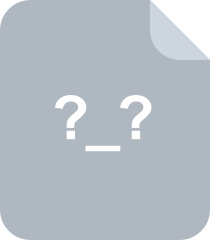
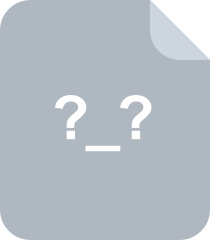
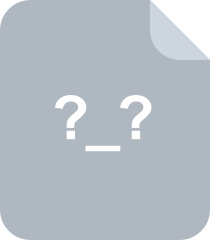
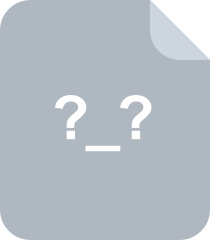
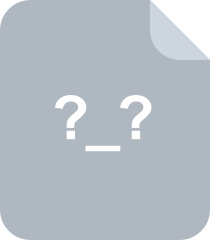
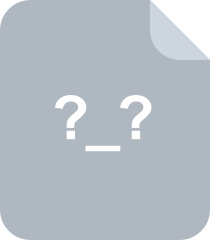
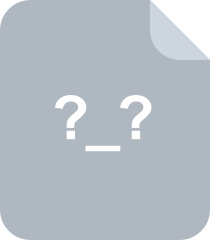
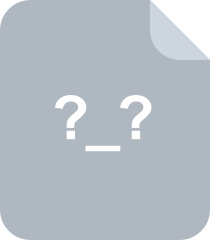
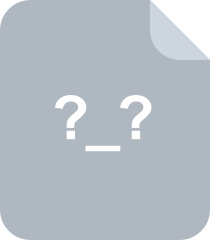
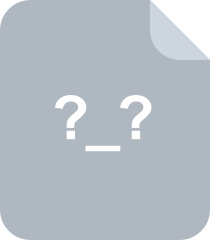
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
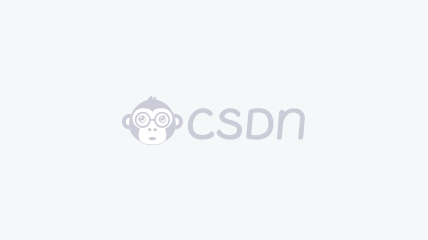

程序员小蛋
- 粉丝: 2748
- 资源: 489

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

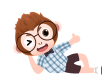
最新资源
- 将 yolov5 导出到 tflite 并在 Raspberry Pi 和 CPU 上运行推理.zip
- 将 pjreddie 的 DarkNet 带出阴影 #yolo.zip
- MPSK-AWGN性能仿真MATLAB源码
- 将 keras(tensorflow 后端)yolov3 h5 模型文件转换为 darknet yolov3 权重.zip
- 将 COCO 转换为 Pascal VOC 2012 格式的 Python 脚本.zip
- 将 COCO 注释(CVAT)转换为 YOLOv8-seg(实例分割)和 YOLOv8-obb(定向边界框检测)的注释.zip
- 章节2:编程基本概念之引用的本质-栈内存和堆内存-内存示意图.rar
- 章节2:编程基本概念之标识符-帮助系统简单使用-命名规则.rar
- fasfdsafaadfasfdasfs
- 如何使用 TensorRT 运行 yolov5 模型 .zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


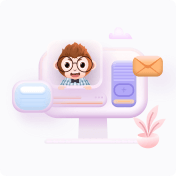
安全验证
文档复制为VIP权益,开通VIP直接复制
