package com.daowen.controller;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.UnsupportedEncodingException;
import java.text.MessageFormat;
import java.util.Collection;
import java.util.List;
import java.util.HashMap;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.alibaba.fastjson.JSONObject;
import com.daowen.dto.Shopcart;
import com.daowen.dto.ShopcartItem;
import com.daowen.util.JsonResult;
import com.daowen.util.SequenceUtil;
import com.daowen.util.StringUtil;
import com.daowen.vo.CouponVo;
import com.daowen.vo.CreateOrderDTO;
import com.daowen.vo.OrderDTO;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import com.daowen.entity.*;
import com.daowen.service.*;
import com.daowen.ssm.simplecrud.SimpleController;
import com.daowen.webcontrol.PagerMetal;
import org.springframework.web.bind.annotation.ResponseBody;
/**************************
*
* 订单控制
*
*/
@Controller
public class ShorderController extends SimpleController {
@Autowired
private ShorderService shorderSrv = null;
@Autowired
private HuiyuanService huiyuanSrv=null;
@Autowired
private OrderitemService orderitemSrv=null;
@Autowired
private CouponService couponSrv=null;
@Autowired
private ShangpinService shangpinSrv=null;
private String cartName = "shopcart";
@Autowired
private SpcommentService spcommentSrv=null;
@Autowired
private ShopcartService shopcartSrv = null;
@Override
@RequestMapping("/admin/shordermanager.do")
public void mapping(HttpServletRequest request, HttpServletResponse response) {
mappingMethod(request, response);
}
/********************************************************
******************信息注销监听支持*****************************
*********************************************************/
public void delete() {
String[] ids = request.getParameterValues("ids");
if (ids == null)
return;
for(String id : ids){
shorderSrv.delete("where id="+id);
orderitemSrv.delete("where orderid="+id);
}
}
private void saleTongji() {
String begdate = request.getParameter("begindate");
String enddate = request.getParameter("enddate");
HashMap<String,Object> map=new HashMap<>();
if(begdate!=null)
map.put("begdate",begdate);
if(enddate!=null)
map.put("enddate",enddate);
List<HashMap<String,Object>> listMap=shorderSrv.saleStat(map);
if(listMap!=null)
request.setAttribute("listMap",listMap);
dispatchParams(request, response);
String forwardurl = request.getParameter("forwardurl");
if (forwardurl == null) {
forwardurl = "/admin/xiaoshoutongjitext.jsp";
}
forward(forwardurl);
}
@ResponseBody
@PostMapping("/admin/shorder/shouhuo")
public JsonResult shouhuo(){
String oiid=request.getParameter("oiid");
String star=request.getParameter("star");
String des=request.getParameter("des");
String appraiserid=request.getParameter("appraiserid");
if(oiid==null||oiid.equals(""))
return JsonResult.error(-1,"参数异常");
Orderitem orderitem=orderitemSrv.load("where id="+oiid);
Spcomment spcomment=new Spcomment();
spcomment.setCreatetime(new Date());
spcomment.setSpid(orderitem.getSpid());
spcomment.setOrderid(orderitem.getId());
spcomment.setCresult(star==null?1:Integer.parseInt(star));
spcomment.setAppraiserid(appraiserid==null?0:Integer.parseInt(appraiserid));
spcomment.setDes(des);
spcommentSrv.save(spcomment);
int count= orderitemSrv.executeUpdate(MessageFormat.format("update orderitem set state=4 where id={0} ",oiid));
int ndCount=orderitemSrv.getRecordCount(MessageFormat.format("where orderid={0,number,#} and state=3 ",orderitem.getOrderid()));
//商品全部发货则更新订单为已发货状态
if(ndCount==0)
shorderSrv.executeUpdate(MessageFormat.format("update shorder set state=4 where id={0,number,#}",orderitem.getOrderid()));
if(count>0)
return JsonResult.success(1,"收货成功");
return JsonResult.error(-2,"收货失败");
}
@ResponseBody
@PostMapping("/admin/shorder/payment")
public JsonResult payment(){
String id=request.getParameter("id");
String paystyle=request.getParameter("paystyle");
String paypwd=request.getParameter("paypwd");
if(paypwd==null||paypwd.equals("")){
return JsonResult.error(-1,"请输入支付密码");
}
if(id==null||paypwd.equals(""))
return JsonResult.error(-2,"订单编号异常");
if(!StringUtil.isNumeric(id))
return JsonResult.error(-3,"订单编号异常");
String couponid=request.getParameter("couponid");
Shorder order=shorderSrv.load("where id="+id);
if(order==null)
return JsonResult.error(-4,"订单号不存在");
Huiyuan huiyuan= huiyuanSrv.load("where id="+order.getPurchaser());
if(huiyuan==null)
return JsonResult.error(-5,"账户信息异常");
if(!paypwd.equals(huiyuan.getPaypwd()))
return JsonResult.error(-6,"支付密码不正确");
CouponVo couponVo =null;
if(couponid!=null){
HashMap<String,Object> map=new HashMap<>();
map.put("id",couponid);
couponVo = couponSrv.loadPlus(map);
if(couponVo.getHyid()!=huiyuan.getId()){
return JsonResult.error(-1,"这尼玛盗用?");
}
}
double fee=shorderSrv.getTotalPrice(order.getId());
if(couponVo!=null) {
fee = fee - couponVo.getFee();
couponSrv.executeUpdate(" update courecord set state =2 where id="+couponVo.getCrid());
}
int count= huiyuanSrv.deduct(order.getPurchaser(),fee);
if(count<=0)
return JsonResult.error(-7,"账户余额不足");
Boolean res=shorderSrv.changeToPayed(order.getId());
if(!res)
return JsonResult.error(-8,"系统异常");
return JsonResult.success(1,"付款成功");
}
@ResponseBody
@PostMapping("/admin/shorder/cancel")
public JsonResult cancel(){
String id=request.getParameter("id");
if(!StringUtil.isNumeric(id))
return JsonResult.error(-1,"订单编号异常");
shorderSrv.executeUpdate("update shorder set state=5 where id="+id);
orderitemSrv.executeUpdate("update orderitem set state=5 where orderid="+id);
return JsonResult.success(1,"付款成功");
}
@ResponseBody
@PostMapping("/admin/shorder/info")
public JsonResult info(){
String id=request.getParameter("id");
String state=request.getParameter("state");
if(id==null||id=="")
return JsonResult.error(-1,"需要id参数");
if(!StringUtil.isNumeric(id)){
return JsonResult.error(-2,"id参数应该为数字");
}
HashMap map=new HashMap<String,Object>();
if(id!=null)
map.put("id",id);
if(state!=null)
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
技术栈:springboot+jdk1.8+mybatis+mysql 实现功能:图书管理、商品管理、商品标签、优惠券管理、限时秒杀、订单管理、销售统计、商品统计、账户管理、 用户管理、会员管理、新闻资讯、用户留言、系统公告、网站导航设置
资源推荐
资源详情
资源评论
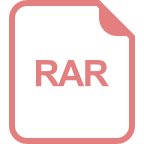
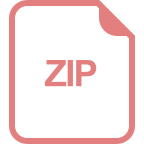
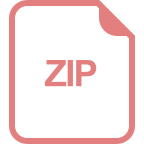
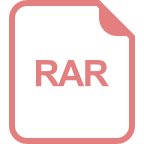
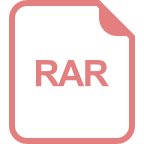
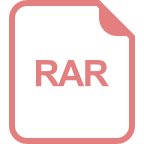
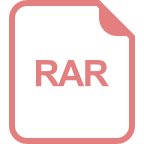
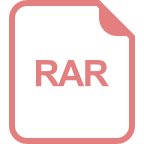
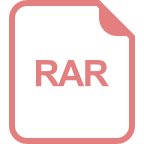
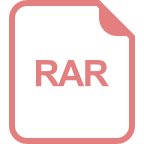
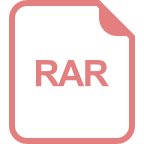
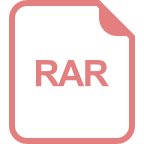
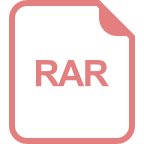
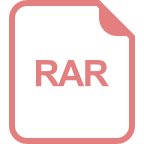
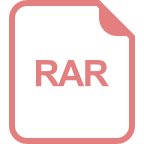
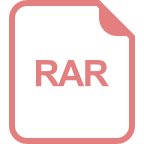
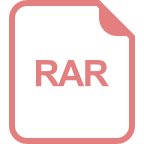
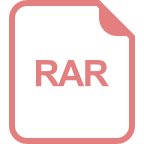
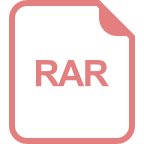
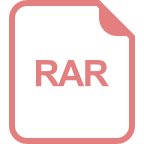
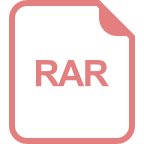
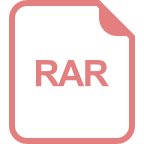
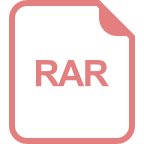
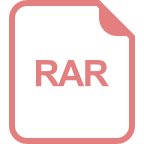
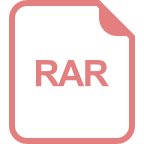
收起资源包目录

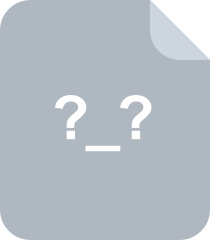
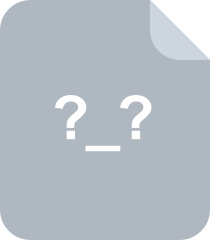
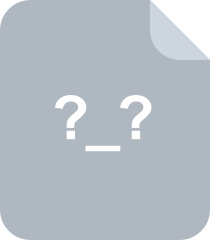
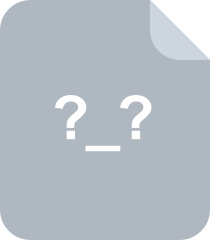
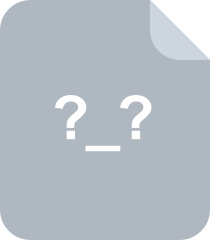
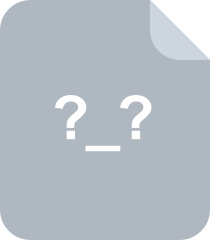
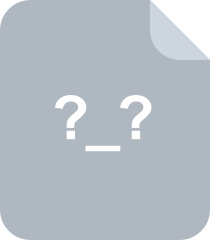
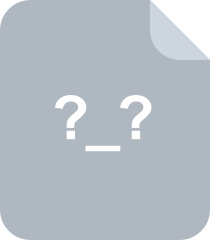
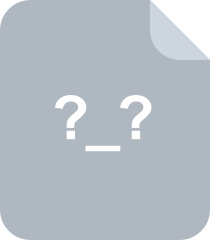
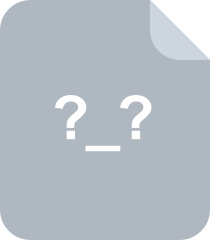
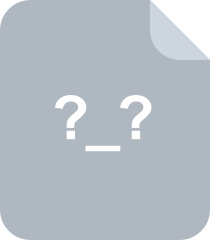
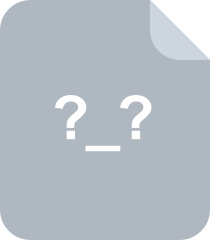
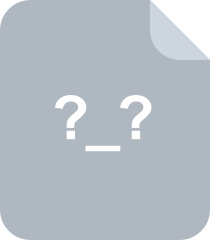
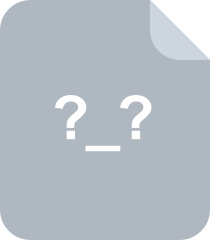
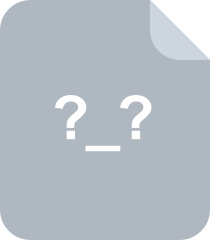
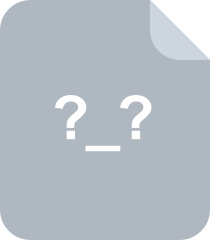
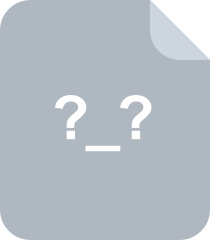
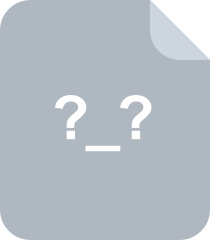
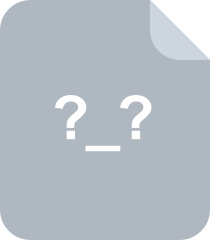
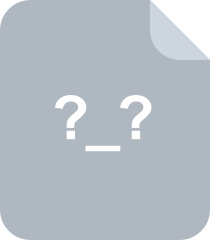
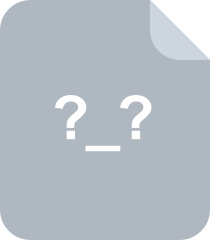
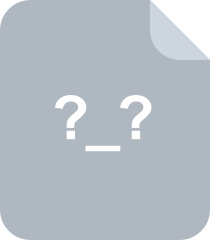
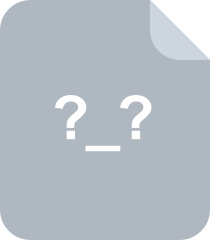
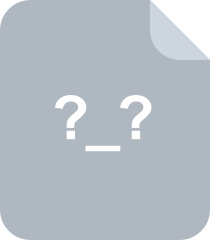
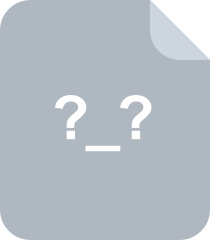
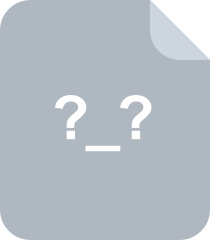
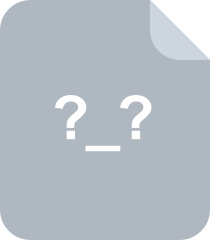
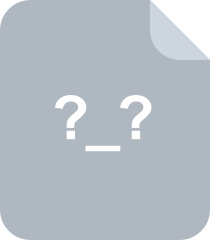
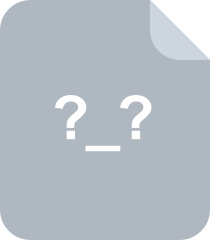
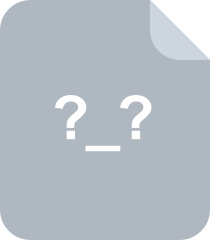
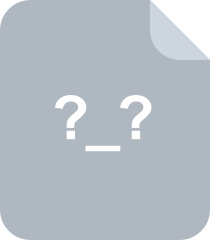
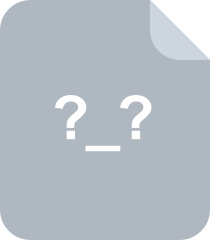
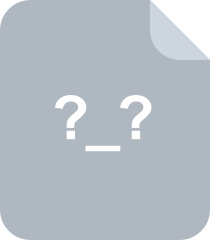
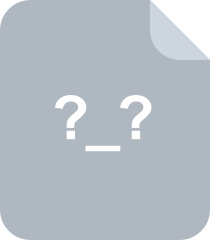
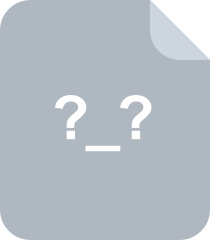
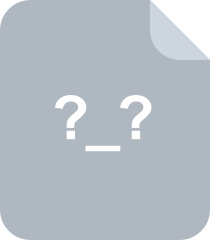
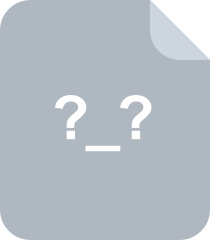
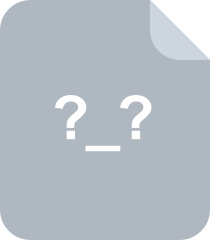
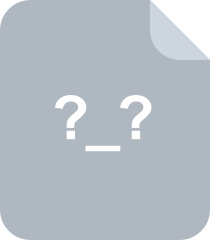
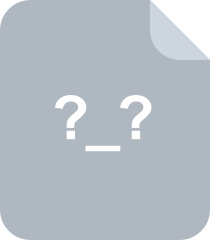
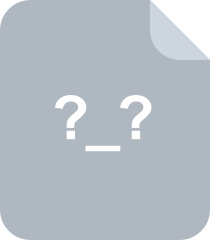
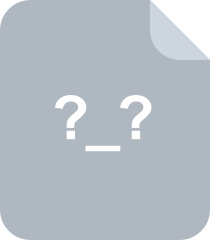
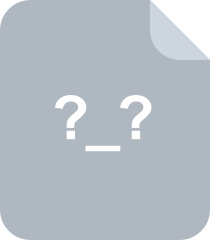
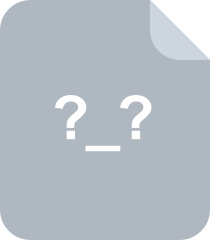
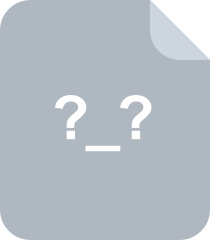
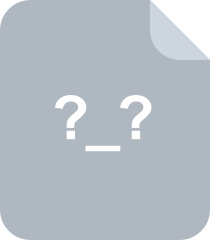
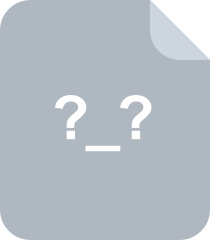
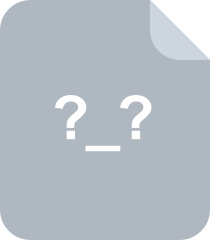
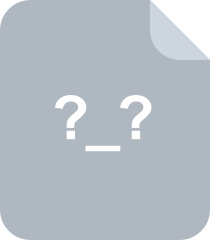
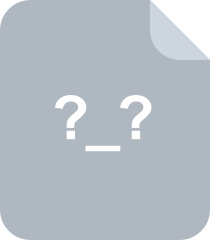
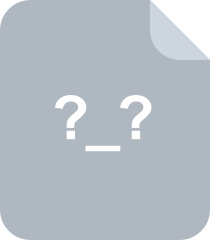
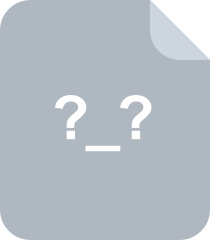
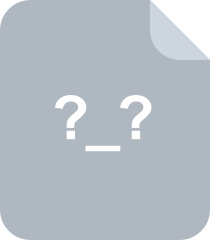
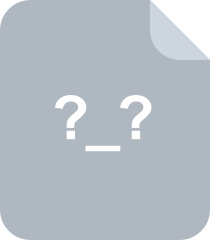
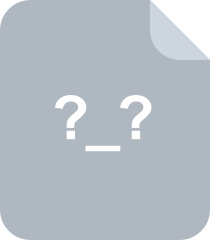
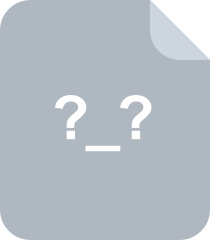
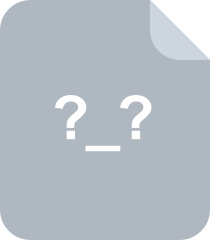
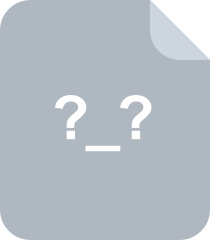
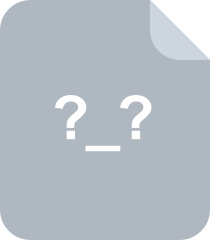
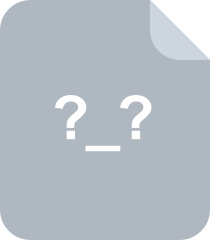
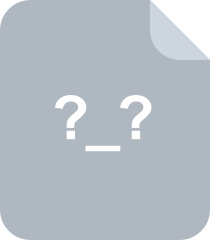
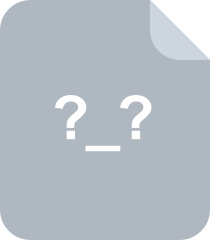
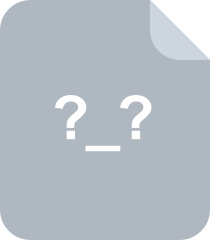
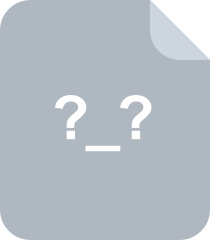
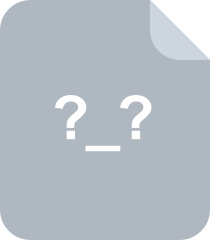
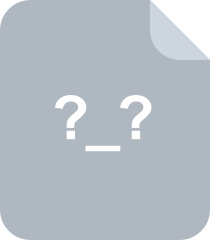
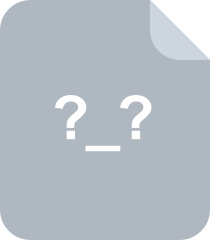
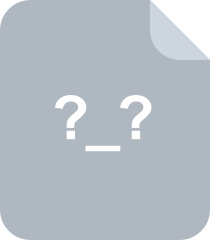
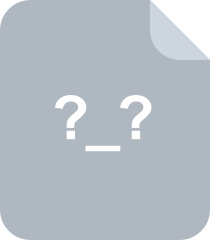
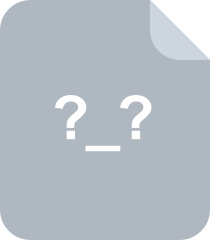
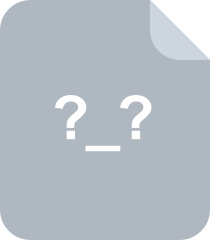
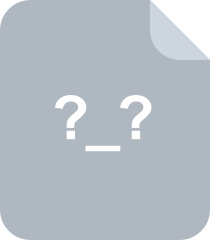
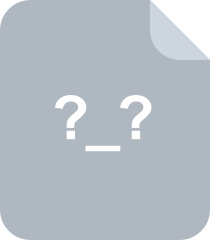
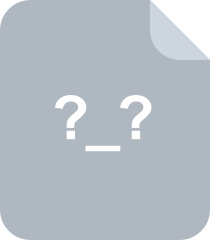
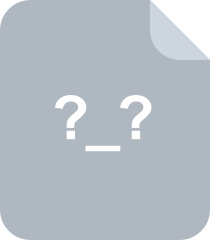
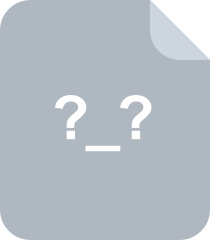
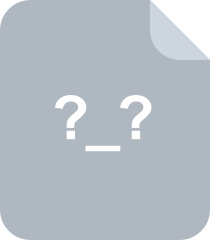
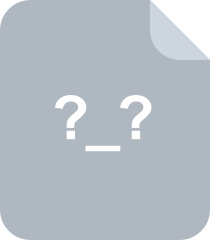
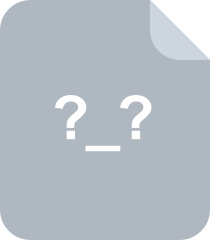
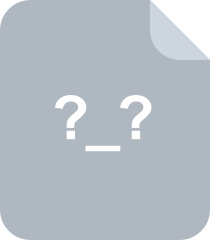
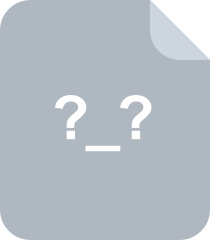
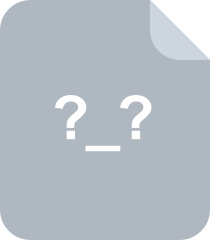
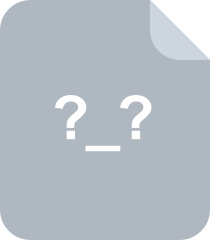
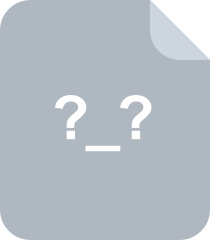
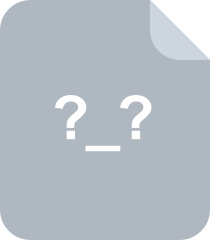
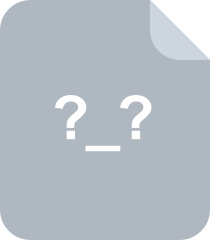
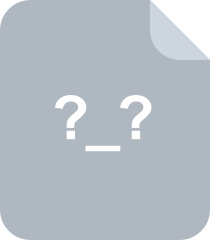
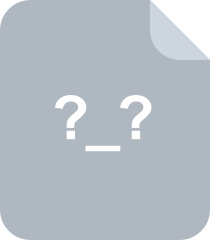
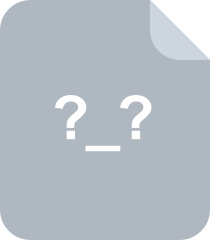
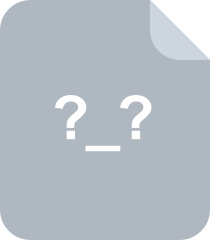
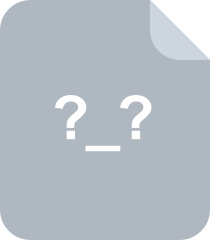
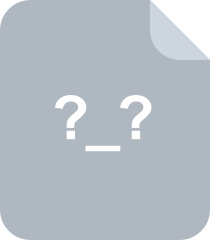
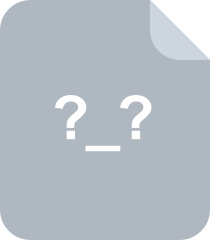
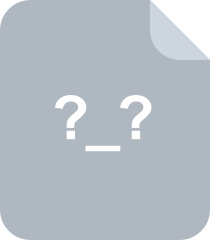
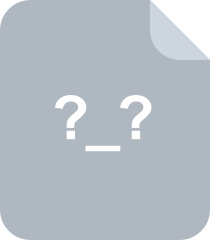
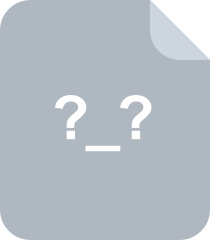
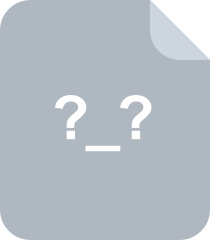
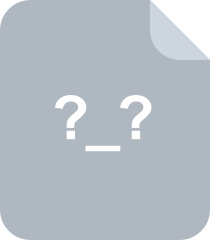
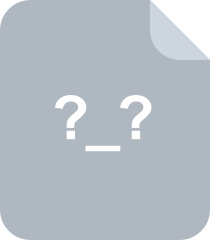
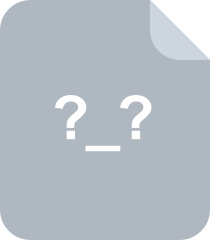
共 2729 条
- 1
- 2
- 3
- 4
- 5
- 6
- 28

程序员小蛋
- 粉丝: 2745
- 资源: 489
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

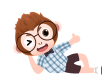
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


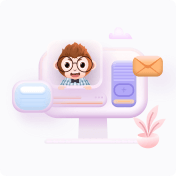
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页