/*
* qrencode - QR Code encoder
*
* Input data chunk class
* Copyright (C) 2006-2011 Kentaro Fukuchi <kentaro@fukuchi.org>
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
*/
#if HAVE_CONFIG_H
# include "config.h"
#endif
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include "qrencode.h"
#include "qrspec.h"
#include "mqrspec.h"
#include "bitstream.h"
#include "qrinput.h"
/******************************************************************************
* Utilities
*****************************************************************************/
int QRinput_isSplittableMode(QRencodeMode mode)
{
return (mode >= QR_MODE_NUM && mode <= QR_MODE_KANJI);
}
/******************************************************************************
* Entry of input data
*****************************************************************************/
static QRinput_List *QRinput_List_newEntry(QRencodeMode mode, int size, const unsigned char *data)
{
QRinput_List *entry;
if(QRinput_check(mode, size, data)) {
errno = EINVAL;
return NULL;
}
entry = (QRinput_List *)malloc(sizeof(QRinput_List));
if(entry == NULL) return NULL;
entry->mode = mode;
entry->size = size;
if(size > 0) {
entry->data = (unsigned char *)malloc(size);
if(entry->data == NULL) {
free(entry);
return NULL;
}
memcpy(entry->data, data, size);
}
entry->bstream = NULL;
entry->next = NULL;
return entry;
}
static void QRinput_List_freeEntry(QRinput_List *entry)
{
if(entry != NULL) {
free(entry->data);
BitStream_free(entry->bstream);
free(entry);
}
}
static QRinput_List *QRinput_List_dup(QRinput_List *entry)
{
QRinput_List *n;
n = (QRinput_List *)malloc(sizeof(QRinput_List));
if(n == NULL) return NULL;
n->mode = entry->mode;
n->size = entry->size;
n->data = (unsigned char *)malloc(n->size);
if(n->data == NULL) {
free(n);
return NULL;
}
memcpy(n->data, entry->data, entry->size);
n->bstream = NULL;
n->next = NULL;
return n;
}
/******************************************************************************
* Input Data
*****************************************************************************/
QRinput *QRinput_new(void)
{
return QRinput_new2(0, QR_ECLEVEL_L);
}
QRinput *QRinput_new2(int version, QRecLevel level)
{
QRinput *input;
if(version < 0 || version > QRSPEC_VERSION_MAX || level > QR_ECLEVEL_H) {
errno = EINVAL;
return NULL;
}
input = (QRinput *)malloc(sizeof(QRinput));
if(input == NULL) return NULL;
input->head = NULL;
input->tail = NULL;
input->version = version;
input->level = level;
input->mqr = 0;
input->fnc1 = 0;
return input;
}
QRinput *QRinput_newMQR(int version, QRecLevel level)
{
QRinput *input;
if(version <= 0 || version > MQRSPEC_VERSION_MAX) goto INVALID;
if((MQRspec_getECCLength(version, level) == 0)) goto INVALID;
input = QRinput_new2(version, level);
if(input == NULL) return NULL;
input->mqr = 1;
return input;
INVALID:
errno = EINVAL;
return NULL;
}
int QRinput_getVersion(QRinput *input)
{
return input->version;
}
int QRinput_setVersion(QRinput *input, int version)
{
if(input->mqr || version < 0 || version > QRSPEC_VERSION_MAX) {
errno = EINVAL;
return -1;
}
input->version = version;
return 0;
}
QRecLevel QRinput_getErrorCorrectionLevel(QRinput *input)
{
return input->level;
}
int QRinput_setErrorCorrectionLevel(QRinput *input, QRecLevel level)
{
if(input->mqr || level > QR_ECLEVEL_H) {
errno = EINVAL;
return -1;
}
input->level = level;
return 0;
}
int QRinput_setVersionAndErrorCorrectionLevel(QRinput *input, int version, QRecLevel level)
{
if(input->mqr) {
if(version <= 0 || version > MQRSPEC_VERSION_MAX) goto INVALID;
if((MQRspec_getECCLength(version, level) == 0)) goto INVALID;
} else {
if(version < 0 || version > QRSPEC_VERSION_MAX) goto INVALID;
if(level > QR_ECLEVEL_H) goto INVALID;
}
input->version = version;
input->level = level;
return 0;
INVALID:
errno = EINVAL;
return -1;
}
static void QRinput_appendEntry(QRinput *input, QRinput_List *entry)
{
if(input->tail == NULL) {
input->head = entry;
input->tail = entry;
} else {
input->tail->next = entry;
input->tail = entry;
}
entry->next = NULL;
}
int QRinput_append(QRinput *input, QRencodeMode mode, int size, const unsigned char *data)
{
QRinput_List *entry;
entry = QRinput_List_newEntry(mode, size, data);
if(entry == NULL) {
return -1;
}
QRinput_appendEntry(input, entry);
return 0;
}
/**
* Insert a structured-append header to the head of the input data.
* @param input input data.
* @param size number of structured symbols.
* @param number index number of the symbol. (1 <= number <= size)
* @param parity parity among input data. (NOTE: each symbol of a set of structured symbols has the same parity data)
* @retval 0 success.
* @retval -1 error occurred and errno is set to indeicate the error. See Execptions for the details.
* @throw EINVAL invalid parameter.
* @throw ENOMEM unable to allocate memory.
*/
__STATIC int QRinput_insertStructuredAppendHeader(QRinput *input, int size, int number, unsigned char parity)
{
QRinput_List *entry;
unsigned char buf[3];
if(size > MAX_STRUCTURED_SYMBOLS) {
errno = EINVAL;
return -1;
}
if(number <= 0 || number > size) {
errno = EINVAL;
return -1;
}
buf[0] = (unsigned char)size;
buf[1] = (unsigned char)number;
buf[2] = parity;
entry = QRinput_List_newEntry(QR_MODE_STRUCTURE, 3, buf);
if(entry == NULL) {
return -1;
}
entry->next = input->head;
input->head = entry;
return 0;
}
int QRinput_appendECIheader(QRinput *input, unsigned int ecinum)
{
unsigned char data[4];
if(ecinum > 999999) {
errno = EINVAL;
return -1;
}
/* We manually create byte array of ecinum because
(unsigned char *)&ecinum may cause bus error on some architectures, */
data[0] = ecinum & 0xff;
data[1] = (ecinum >> 8) & 0xff;
data[2] = (ecinum >> 16) & 0xff;
data[3] = (ecinum >> 24) & 0xff;
return QRinput_append(input, QR_MODE_ECI, 4, data);
}
void QRinput_free(QRinput *input)
{
QRinput_List *list, *next;
if(input != NULL) {
list = input->head;
while(list != NULL) {
next = list->next;
QRinput_List_freeEntry(list);
list = next;
}
free(input);
}
}
static unsigned char QRinput_calcParity(QRinput *input)
{
unsigned char parity = 0;
QRinput_List *list;
int i;
list = input->head;
while(list != NULL) {
if(list->mode != QR_MODE_STRUCTURE) {
for(i=list->size-1; i>=0; i--) {
parity ^= list->data[i];
}
}
list = list->next;
}
return parity;
}
QRinput *QRinput_dup(QRinput *input)
{
QRinput *n;
QRinput_List *list, *e;
if(input->mqr) {
n = QRinput_newMQR(input->version, input->level);
} else {
n = QRinput_new2(input->version, input->level);
}
if(n == NULL) return NULL;
list = input->head;
while(list != NULL) {
e = QRinput_List_dup(list);
if(e == NULL) {
QRinput_free(n);
return NULL;
}
QRinput_appendEntry(n, e);
list = list->next;
}
return n;
}
/******************************************************************************
* Numeric data
*****************************************************************************/
/**
* Check the input data.
* @param size
* @param data
* @return result
*/
static int QRinput_checkModeNum(int size, const char *dat
没有合适的资源?快使用搜索试试~ 我知道了~
基于QT的小型OA-ERP系统(源码1)
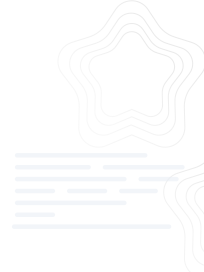
共523个文件
png:328个
h:62个
cpp:52个


温馨提示
展示链接:https://blog.csdn.net/weixin_39852922/article/details/88723037 Windows环境:Qt5.10(MinGW)、Mysql5.6. 该软件基于C/S架构以及MySql数据库, 采用Qt(C++)开发,设计多线程以及网络通信。实现自动登录、开机自启动、一键自动更新、考勤电子流(加班、请假、忘打卡、外出、出差)、考勤汇总、硬件PCB导出的BOM单处理(导出电装明细表Word,导出采购清单Excel,一键生成采购电子流申请表),库存管理、员工信息管理、图/文编号、上班打卡记录、个人周报提交、邮件、留言板等功能。不同功能操作间设置权限限制
资源推荐
资源详情
资源评论
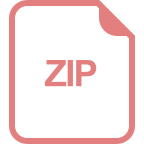
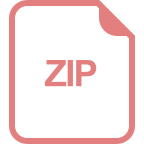
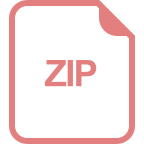
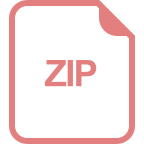
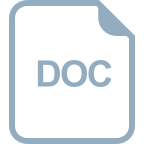
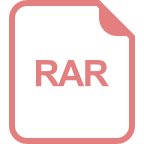
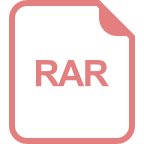
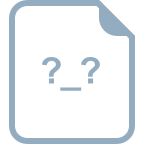
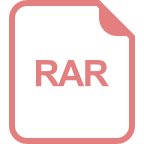
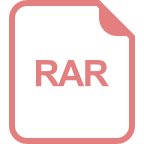
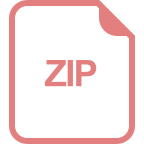
收起资源包目录

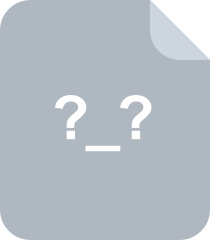
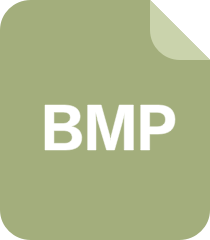
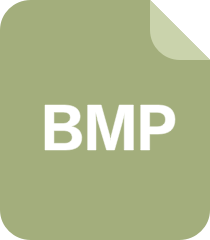
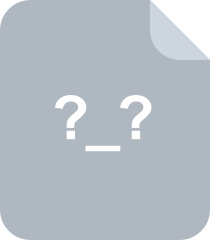
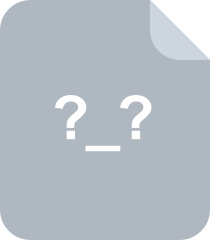
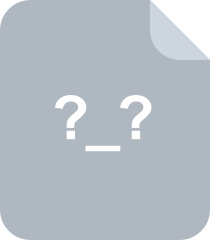
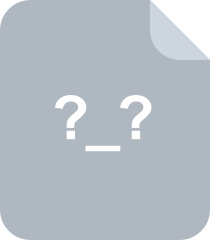
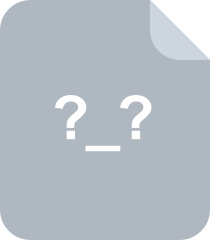
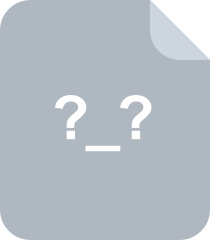
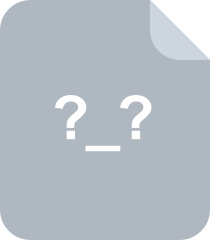
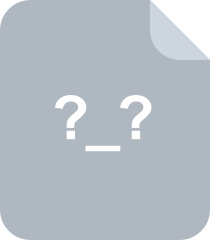
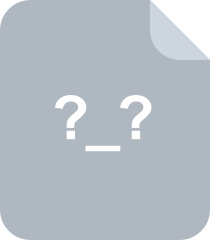
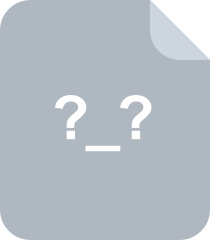
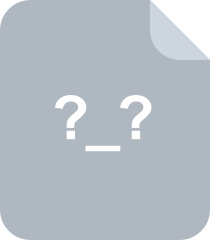
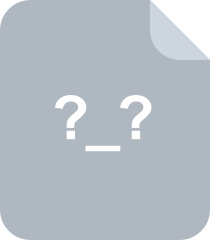
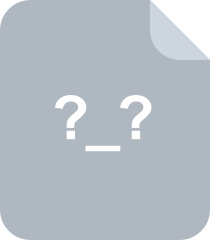
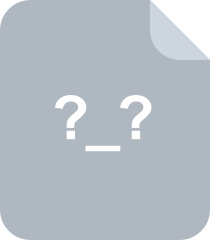
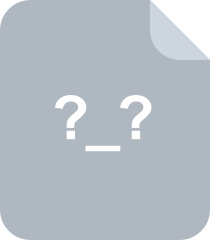
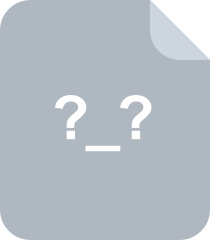
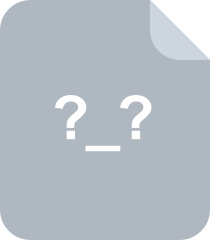
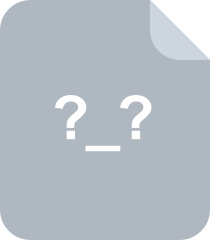
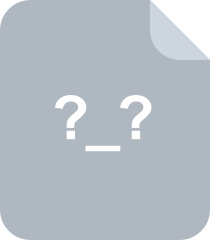
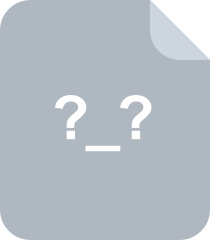
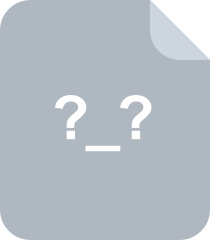
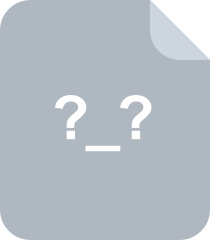
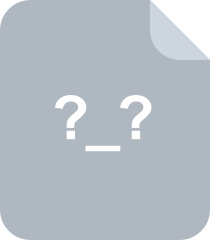
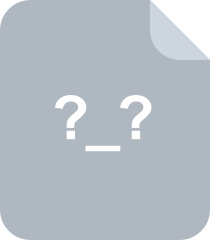
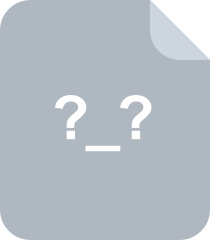
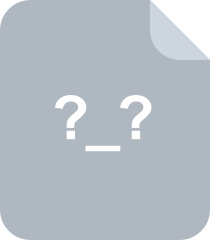
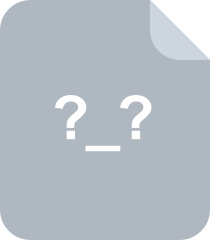
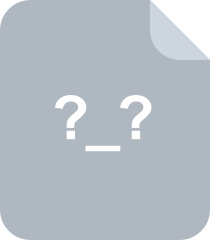
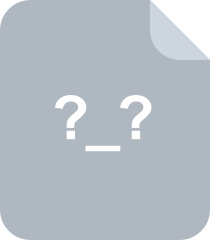
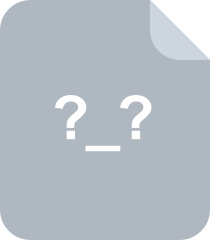
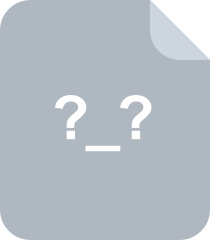
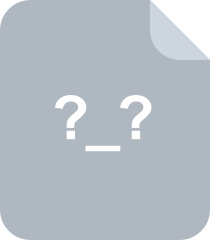
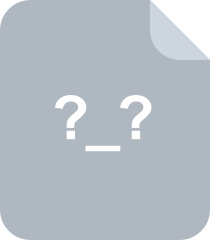
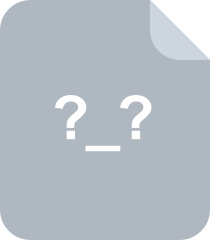
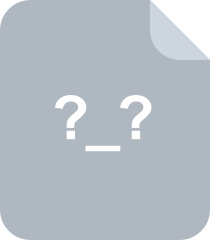
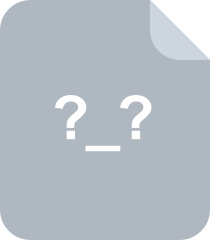
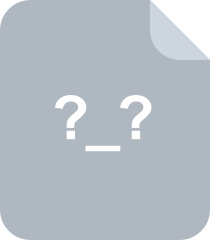
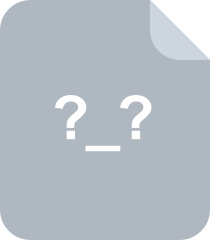
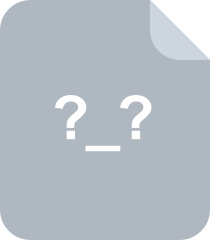
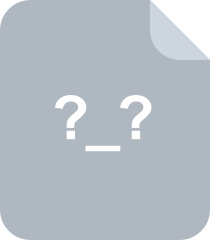
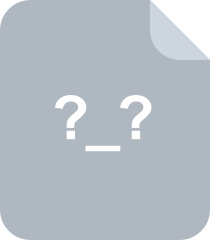
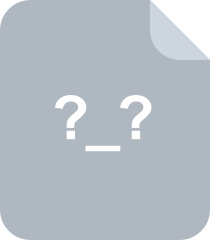
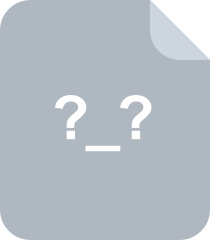
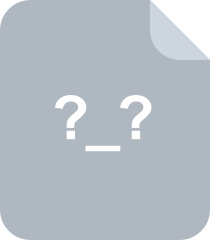
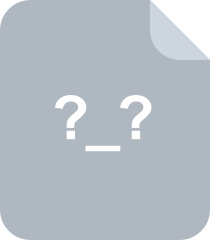
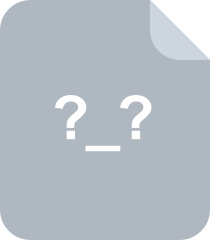
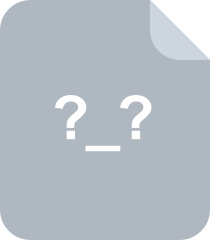
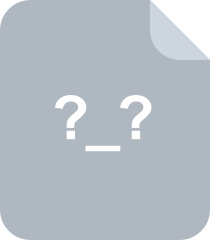
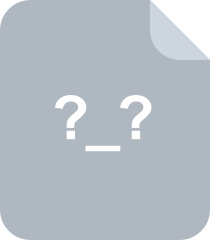
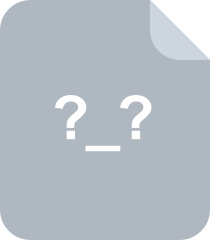
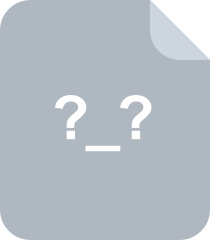
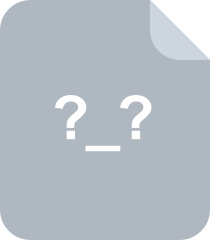
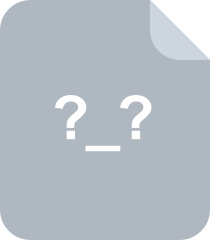
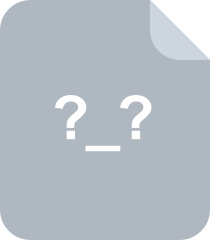
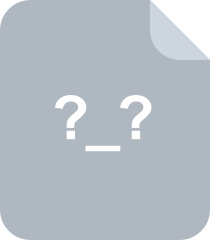
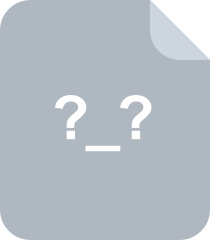
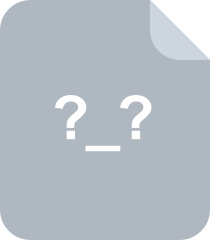
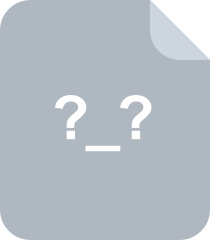
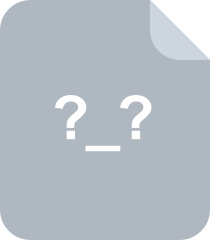
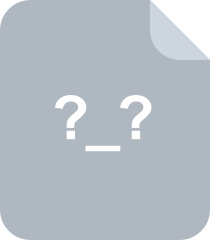
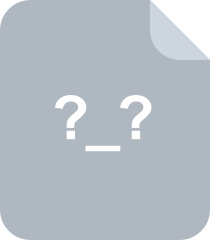
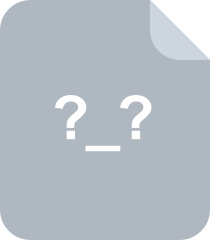
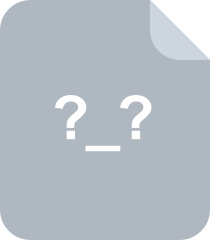
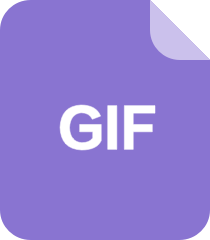
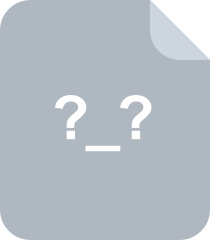
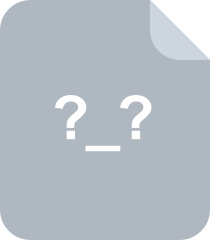
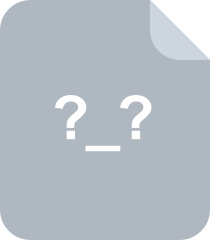
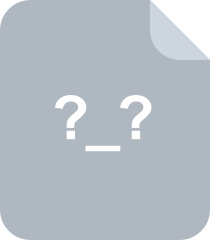
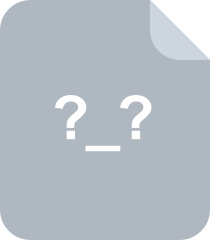
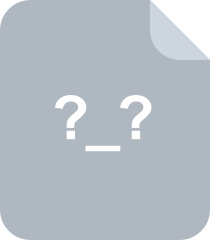
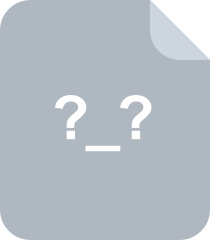
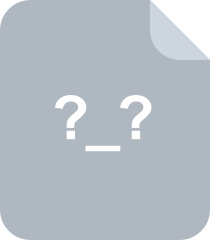
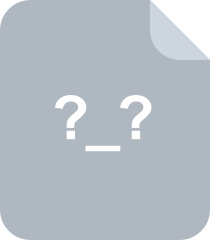
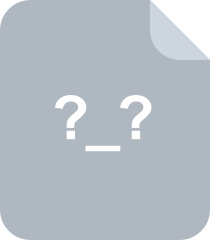
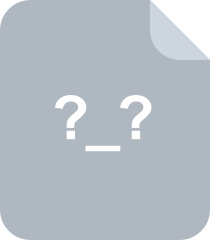
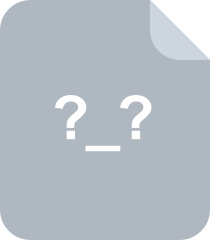
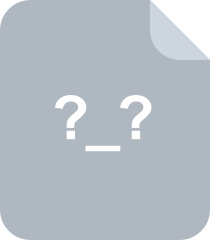
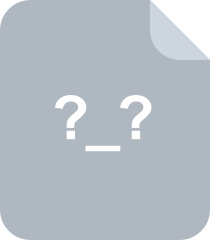
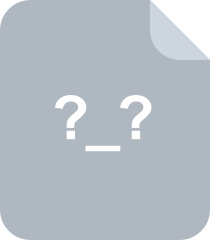
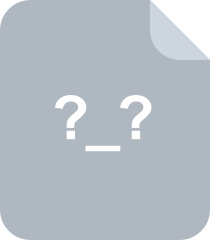
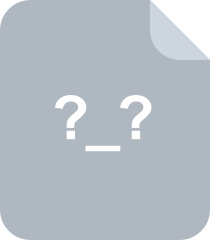
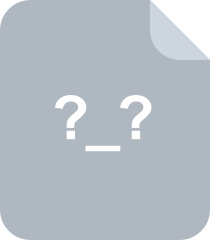
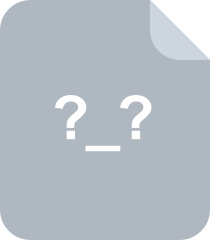
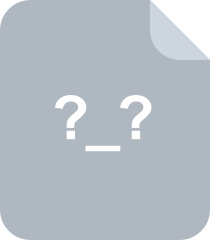
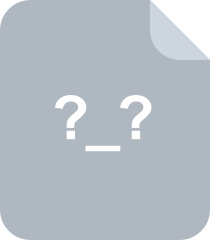
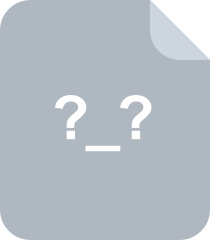
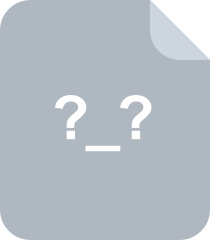
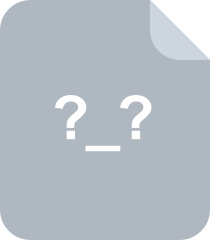
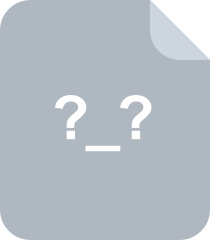
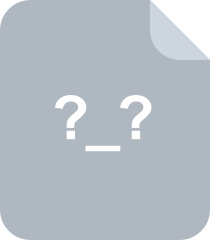
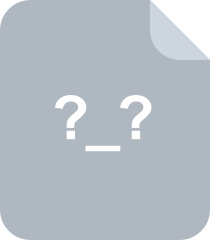
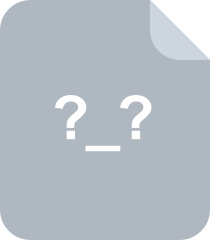
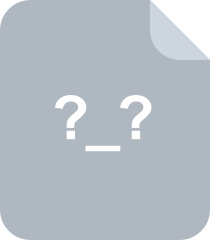
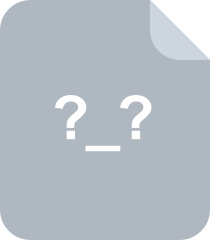
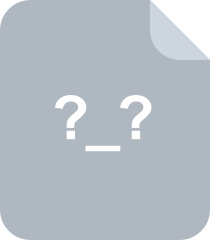
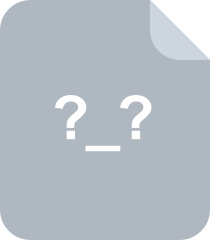
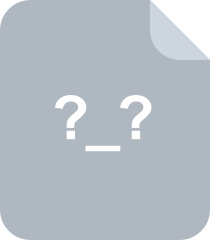
共 523 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
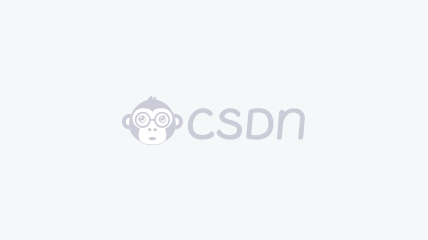
- 黄河一条鱼2022-04-01图片要全部改成英文吗
- 十启树2021-02-22适合初学者
- imsuperstarest2020-07-28:-1: error: No rule to make target '../ZYERP/images/??3.jpg', needed by 'debug/qrc_images.o'. Stop.Steve1072020-08-17图片中文名导致
- 机器视觉0012020-05-26非常好用,谢谢

Steve107
- 粉丝: 24
- 资源: 10
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

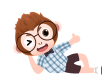
安全验证
文档复制为VIP权益,开通VIP直接复制
