RMSwitch
======
<img src="switch-sample.gif" title="sample" />
A simple View that works like a switch, but with more customizations. <br />
With the option to choose between two or three states. (from v1.1.0) <br /><br />
##### ** If you're upgrading from a version < 1.2.0, check the changelog of the 1.2.0 version, there are breaking changes! <br />
[Changelog] (CHANGELOG.md)
##### From version 1.2.0 you can choose between three switch design: <br />
-"Slim" <br />
-"Large" <br />
-"Android" <br />
<img src="switch_design_samples.png" title="design_sample" />
Download
------
#### Gradle:
```groovy
compile 'com.rm:rmswitch:1.2.2'
```
<br />
<b>Min API level: 16 (Android 4.1) </b>
<br />
## Usage
To use them, just add this to your layout file
```xml
<!-- Two states switch -->
<com.rm.rmswitch.RMSwitch
android:id="@+id/your_id"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:checked="true"
app:forceAspectRatio="false"
app:enabled="true"
app:switchDesign="android"
app:switchBkgCheckedColor="@color/green"
app:switchBkgNotCheckedColor="@color/red"
app:switchToggleCheckedColor="@color/green"
app:switchToggleCheckedImage="@drawable/happy"
app:switchToggleNotCheckedColor="@color/red"
app:switchToggleNotCheckedImage="@drawable/sad"/>
<!-- Three states switch -->
<com.rm.rmswitch.RMTristateSwitch
android:id="@+id/your_id2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:forceAspectRatio="false"
app:state="left"
app:enabled="true"
app:switchDesign="large"
app:switchBkgLeftColor="@color/red"
app:switchBkgMiddleColor="@color/grey"
app:switchBkgRightColor="@color/green"
app:switchToggleLeftColor="@color/red"
app:switchToggleLeftImage="@drawable/sad"
app:switchToggleMiddleColor="@color/grey"
app:switchToggleMiddleImage="@drawable/neutral"
app:switchToggleRightColor="@color/green"
app:switchToggleRightImage="@drawable/happy"/>
```
... if you need to use this View's custom xml attributes (shown in a table below or in the example above) do not forget to add this to your root layout
```
xmlns:app="http://schemas.android.com/apk/res-auto"
```
To see how it looks in the preview screen of Android Studio, build your project first
And this in your Activity
```java
public class MainActivity extends AppCompatActivity {
RMSwitch mSwitch;
RMTristateSwitch mTristateSwitch;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mSwitch = (RMSwitch) findViewById(R.id.your_id);
mTristateSwitch = (RMTristateSwitch) findViewById(R.id.your_id2);
// Setup the switch
mSwitch.setChecked(true);
mSwitch.setEnabled(true);
mSwitch.setForceAspectRatio(false);
mSwitch.setSwitchBkgCheckedColor(Color.GREEN);
mSwitch.setSwitchBkgNotCheckedColor(Color.RED);
mSwitch.setSwitchToggleCheckedColor(Color.BLACK);
mSwitch.setSwitchToggleNotCheckedColor(Color.BLACK);
mSwitch.setSwitchDesign(RMTristateSwitch.DESIGN_ANDROID);
// You can choose if use drawable or drawable resource
//mSwitch.setSwitchToggleCheckedDrawableRes(android.R.drawable.ic_media_next);
mSwitch.setSwitchToggleCheckedDrawable(ContextCompat.getDrawable(this, android.R.drawable.ic_media_next));
//mSwitch.setSwitchToggleNotCheckedDrawableRes(android.R.drawable.ic_media_previous);
mSwitch.setSwitchToggleNotCheckedDrawable(ContextCompat.getDrawable(this, android.R.drawable.ic_media_previous));
// Setup the tristate switch
mTristateSwitch.setState(RMTristateSwitch.STATE_LEFT);
mTristateSwitch.setForceAspectRatio(true);
mTristateSwitch.setEnabled(true);
mTristateSwitch.setRightToLeft(false);
mTristateSwitch.setSwitchDesign(RMTristateSwitch.DESIGN_LARGE);
mTristateSwitch.setSwitchToggleLeftColor(Color.DKGRAY);
mTristateSwitch.setSwitchToggleMiddleColor(Color.DKGRAY);
mTristateSwitch.setSwitchToggleRightColor(Color.DKGRAY);
mTristateSwitch.setSwitchBkgLeftColor(Color.LTGRAY);
mTristateSwitch.setSwitchBkgMiddleColor(Color.LTGRAY);
mTristateSwitch.setSwitchBkgRightColor(Color.LTGRAY);
// You can choose if use drawable or drawable resource
//mTristateSwitch.setSwitchToggleLeftDrawableRes(android.R.drawable.ic_media_previous);
mTristateSwitch.setSwitchToggleLeftDrawable(ContextCompat.getDrawable(this, android.R.drawable.ic_media_previous));
//mTristateSwitch.setSwitchToggleMiddleDrawableRes(android.R.drawable.ic_media_play);
mTristateSwitch.setSwitchToggleMiddleDrawable(ContextCompat.getDrawable(this, android.R.drawable.ic_media_play));
//mTristateSwitch.setSwitchToggleRightDrawableRes(android.R.drawable.ic_media_next);
mTristateSwitch.setSwitchToggleRightDrawable(ContextCompat.getDrawable(this, android.R.drawable.ic_media_next));
// Add a Switch state observer
mSwitch.addSwitchObserver(new RMSwitch.RMSwitchObserver() {
@Override
public void onCheckStateChange(RMSwitch switchView, boolean isChecked) {
Toast.makeText(MainActivity.this,
"Switch state: " +
(isChecked ? "checked" : "not checked"), Toast.LENGTH_LONG)
.show();
}
});
mTristateSwitch.addSwitchObserver(new RMTristateSwitch.RMTristateSwitchObserver() {
@Override
public void onCheckStateChange(RMTristateSwitch switchView, @RMTristateSwitch.State int state) {
Toast
.makeText(MainActivity.this,
state == RMTristateSwitch.STATE_LEFT ?
"Left" :
state == RMTristateSwitch.STATE_MIDDLE ?
"Middle" :
"Right",
Toast.LENGTH_SHORT).show();
}
});
}
}
```
#### Supported Attributes
RMSwitch
------
| XML Attribute | Java method | Description | Default value |
|------------------------- |----------------------------------------------------------------- |----------------------------------------------------------------------------------------------------------------- |--------------------------------------------------------------------------------------------- |
| checked | setChecked(boolean checked) | The initial state of the Switch, if checked or not | false |
| enabled | setEnabled(boolean enabled) | If not enabled, the Switch
没有合适的资源?快使用搜索试试~ 我知道了~
Android代码-Android 两状态 或者 三状态 Switch 按钮,三状态切换超实用。
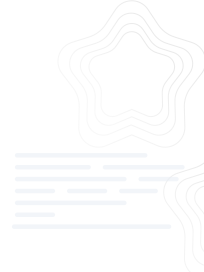
共57个文件
png:19个
xml:14个
java:7个


温馨提示
RMSwitch A simple View that works like a switch, but with more customizations. With the option to choose between two or three states. (from v1.1.0) ** If you're upgrading from a version < 1.2.0, check the changelog of the 1.2.0 version, there are breaking changes! [Changelog] (CHANGELOG.md) From version 1.2.0 you can choose between three switch design: -"Slim" -"Large" -"Android" Download Gradle: compile 'com.rm:rmswitch:1.2.2' Min API level: 16 (Android 4.1) Usage To use them, just
资源推荐
资源详情
资源评论
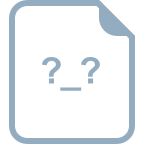
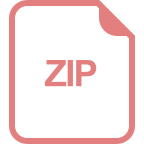
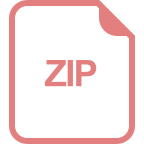
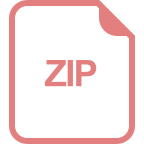
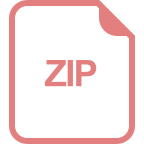
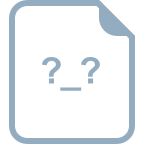
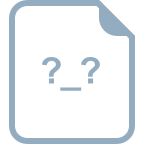
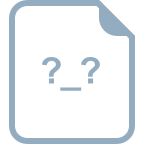
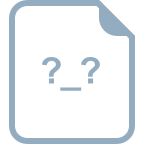
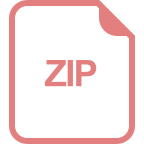
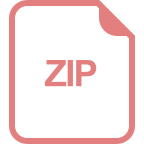
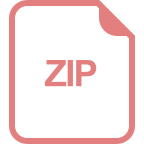
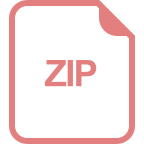
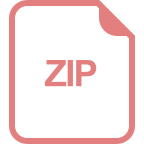
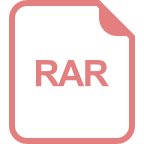
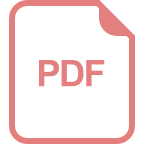
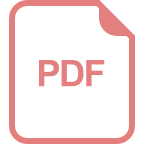
收起资源包目录


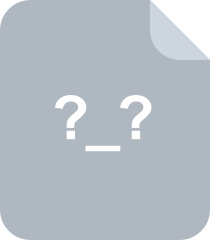
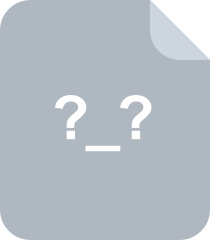
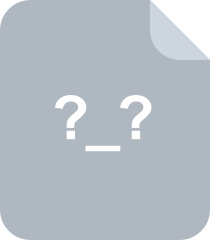
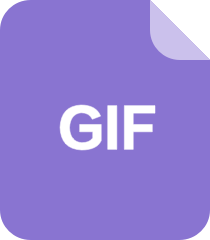

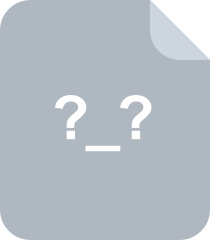




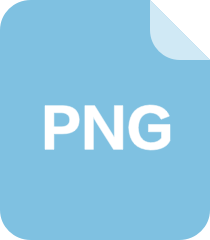

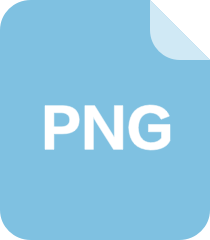
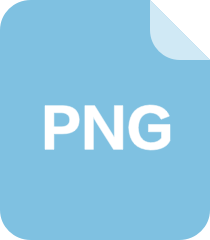
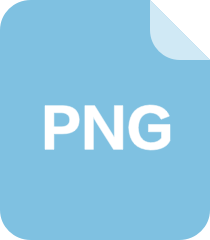
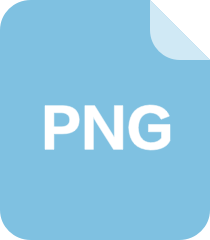
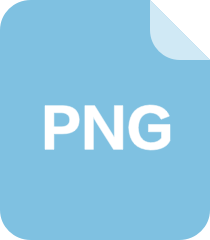
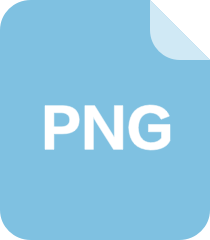
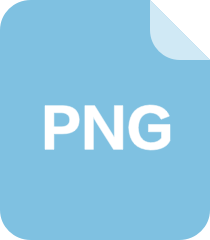
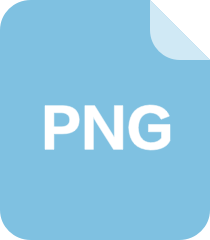
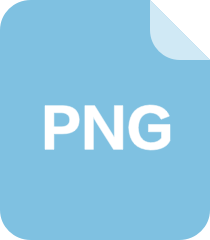
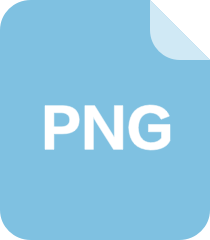
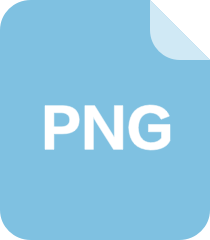
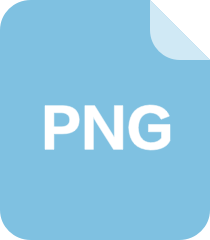

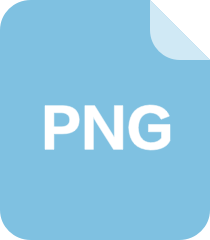

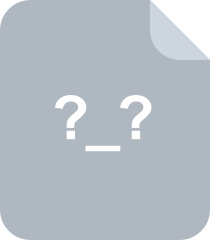
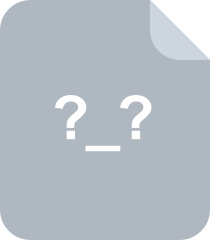
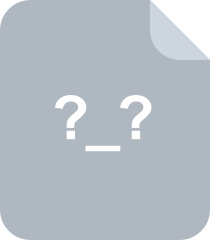
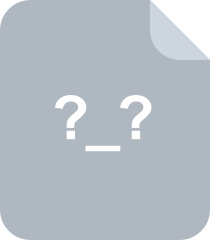

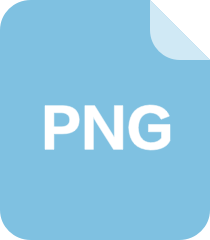

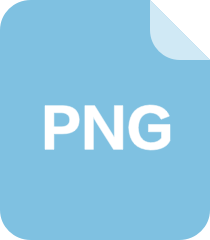

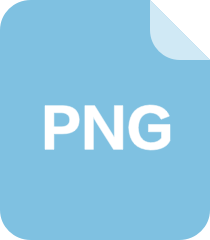

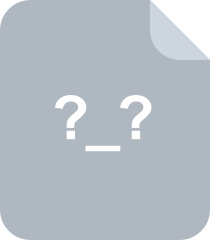

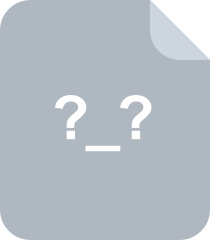




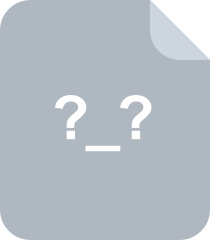
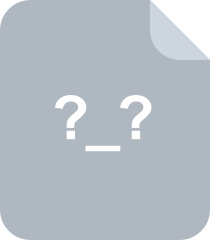
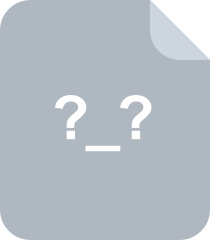
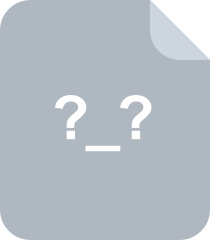
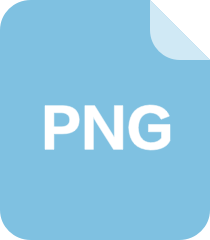


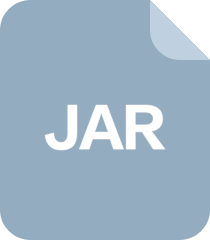
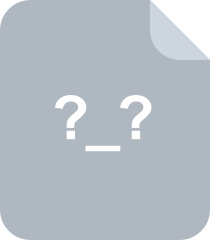

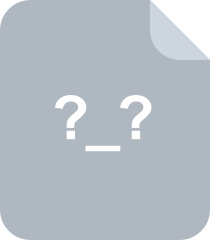




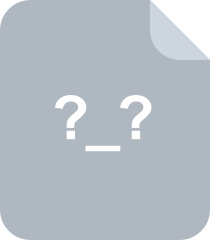

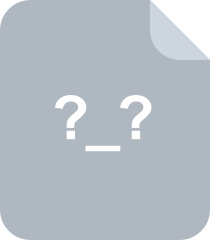
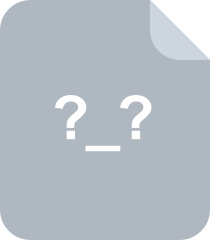
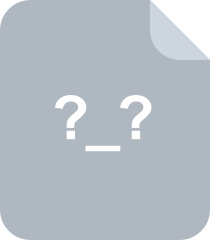

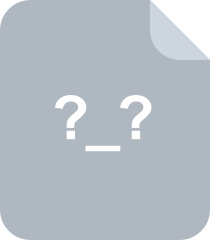
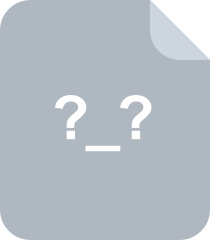




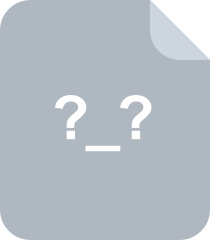
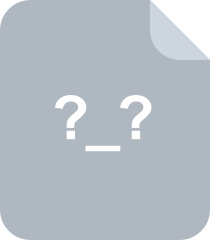
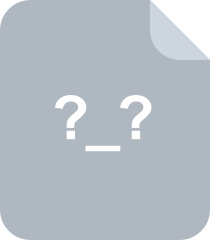
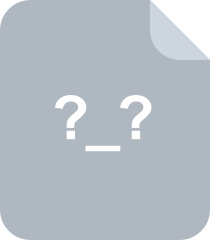
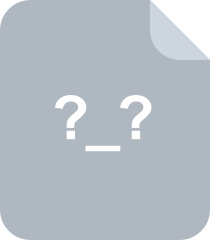
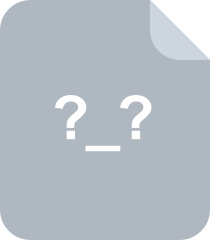
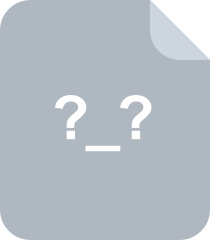
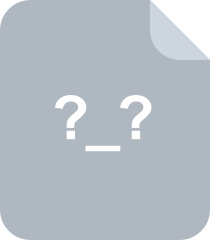
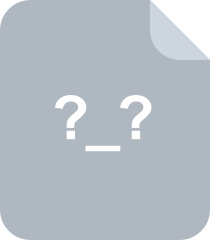
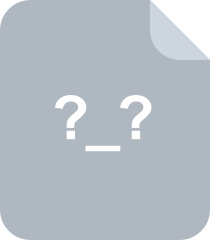
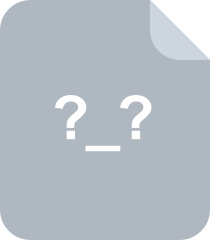
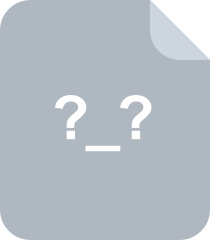
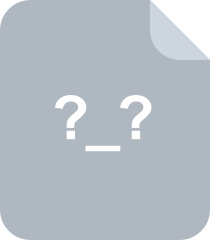
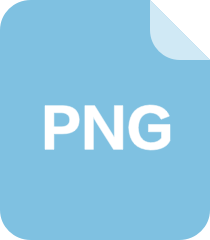
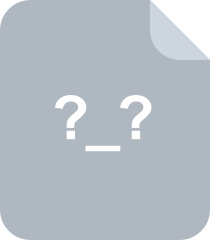
共 57 条
- 1
资源评论
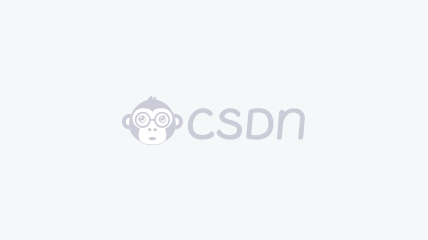
- 不放棄2020-10-22无法运行,报错

weixin_39841856
- 粉丝: 491
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

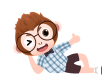
最新资源
- 国际象棋检测2-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- ssd5课件图片记录保存
- 常用算法介绍与学习资源汇总
- Python与Pygame实现带特效的圣诞节场景模拟程序
- 国际象棋检测11-YOLO(v7至v9)、COCO、Darknet、Paligemma、VOC数据集合集.rar
- 使用Python和matplotlib库绘制爱心图形的技术教程
- Java外卖项目(瑞吉外卖项目的扩展)
- 必应图片壁纸Python爬虫代码bing-img.zip
- 基于Pygame库实现新年烟花效果的Python代码
- 浪漫节日代码 - 爱心代码、圣诞树代码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


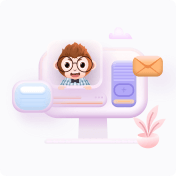
安全验证
文档复制为VIP权益,开通VIP直接复制
