swift-一行代码搞定textView占位符和字数限制
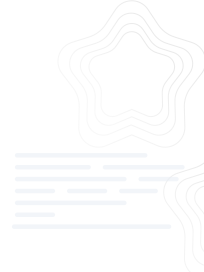

在Swift编程中,处理用户输入的文本视图(如`UITextView`)是常见的任务,尤其是在需要设置占位符和字数限制时。本篇将深入探讨如何利用Swift优雅地解决这两个需求,使你的代码更加简洁高效。 `UITextView`在默认情况下并不支持像`UITextField`那样的占位符功能,但我们可以通过自定义扩展来实现这一特性。占位符是在用户输入前显示的提示性文字,当用户开始输入时,它会自动消失。这里我们可以创建一个扩展,为`UITextView`添加一个`placeholder`属性,并处理其显示逻辑: ```swift extension UITextView { var placeholder: String? { get { return attributedText?.string } set { let attributedPlaceholder = NSAttributedString(string: newValue ?? "", attributes: [NSAttributedString.Key.font: UIFont.systemFont(ofSize: 15, weight: .light)]) addAttributedTextAttachment(attributedPlaceholder, forAttribute: NSAttributedString.Key.paragraphStyle) text = "" } } private func addAttributedTextAttachment(_ attributedString: NSAttributedString, forAttribute attributeKey: NSAttributedString.Key) { let attachment = NSTextAttachment() attachment.attributedString = attributedString let paragraphStyle = NSMutableParagraphStyle() paragraphStyle.alignment = textAlignment attributedString.addAttribute(attributeKey, value: paragraphStyle, range: NSRange(location: 0, length: attributedString.length)) let replacementRange = NSRange(location: 0, length: 0) replaceCharacters(in: replacementRange, with: NSAttributedString(attachment: attachment)) } } ``` 这段代码中,我们创建了一个可读写的`placeholder`属性,当设置新的占位符时,它会将占位符作为`NSAttributedString`添加到`UITextView`的起始位置,并在用户开始输入时自动清除。注意,我们还调整了字体和对齐方式以匹配原生的`UITextField`效果。 接下来,我们要处理字数限制。在许多应用场景中,我们可能需要限制用户输入的最大字符数,例如评论、留言等。为此,我们可以监听`UITextView`的`textDidChange`代理方法,并在用户输入超出限制时进行相应处理: ```swift class ViewController: UIViewController, UITextViewDelegate { @IBOutlet weak var textView: UITextView! override func viewDidLoad() { super.viewDidLoad() textView.delegate = self textView.placeholder = "请输入不超过140个字符的文本" } func textView(_ textView: UITextView, shouldChangeTextIn range: NSRange, replacementText text: String) -> Bool { let currentTextLength = (textView.text as NSString).length let maxLength = 140 if currentTextLength + text.count > maxLength { let alert = UIAlertController(title: "字数超限", message: "您已达到最大输入字数 \(maxLength)", preferredStyle: .alert) alert.addAction(UIAlertAction(title: "确定", style: .default, handler: nil)) present(alert, animated: true, completion: nil) return false } return true } } ``` 在这个例子中,我们在`ViewController`中实现了`UITextViewDelegate`协议,并在`textView(_:shouldChangeTextIn:replacementText:)`方法中检查当前文本长度加上新输入的字符数是否超过设定的`maxLength`。如果超过,就弹出警告对话框阻止输入,并提示用户已达到最大字数。 通过以上代码,你可以在Swift应用中轻松实现`UITextView`的占位符功能和字数限制,使用户体验更佳,同时保持代码的简洁性。这个解决方案适用于各种需要文本输入的场景,无论是简单的评论输入还是复杂的文本编辑界面。 对于压缩包中的`YLTextView-master`文件,这可能是一个第三方库,用于增强`UITextView`的功能,包括但不限于占位符和字数限制。如果你打算使用这样的第三方库,记得先了解其API、兼容性和许可证要求,确保它能很好地融入你的项目并遵循开源许可条款。同时,使用自定义库可以减少代码维护工作,但也要考虑性能和代码管理的影响。
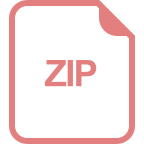
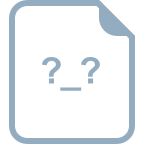
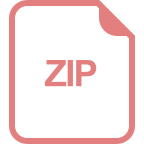
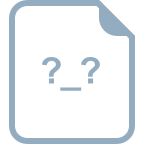
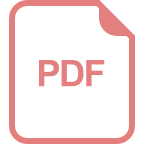
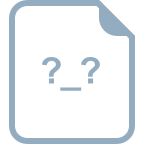
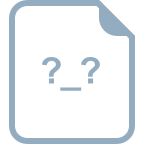
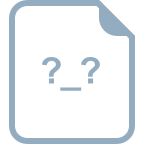
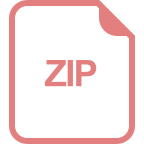
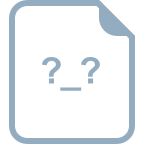
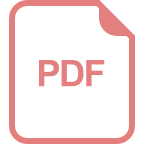
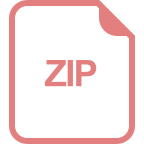
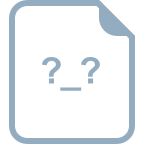



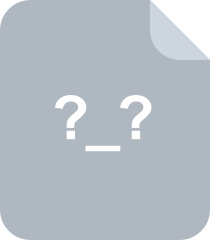
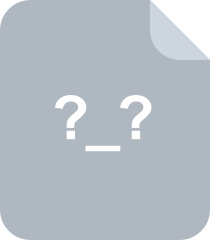
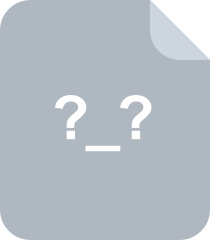
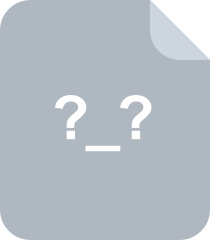


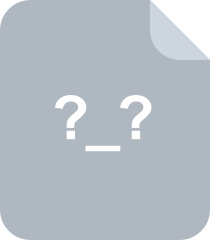
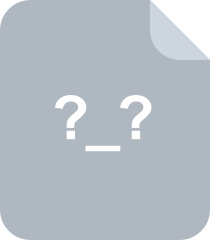


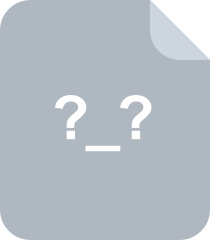


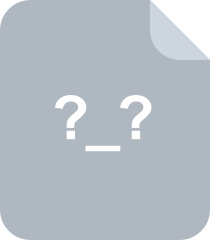

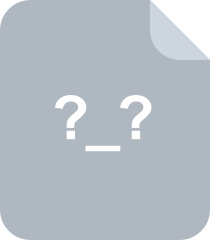

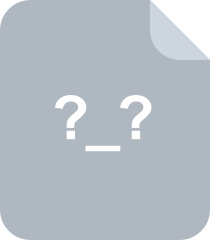
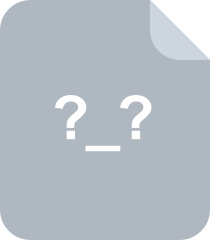
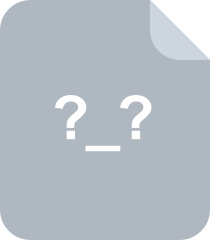
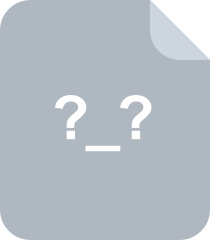


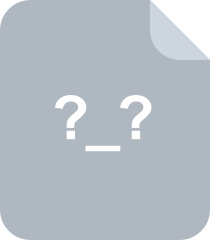
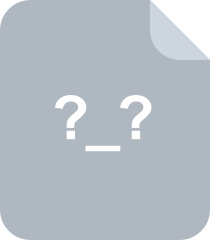



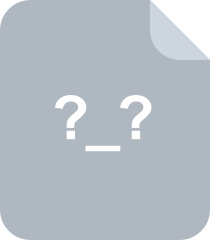
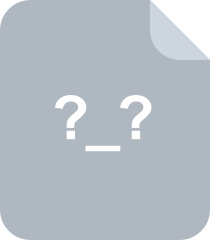

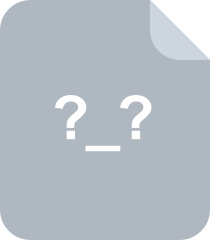
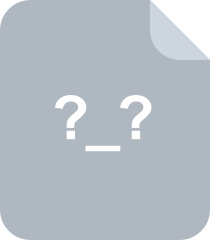
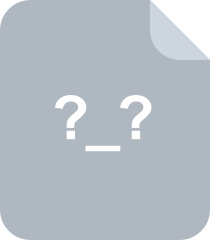
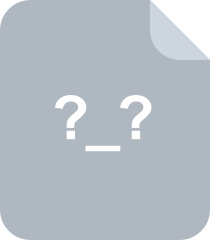

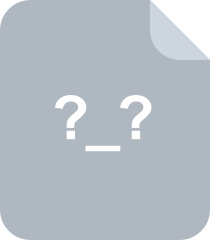
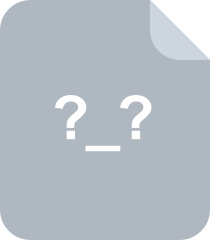
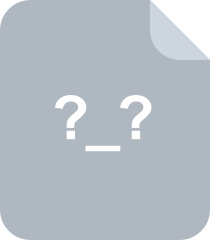
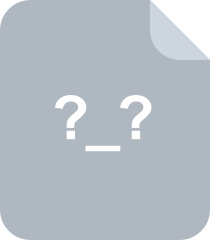
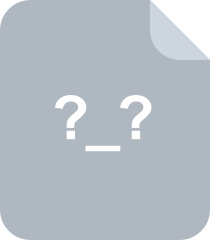


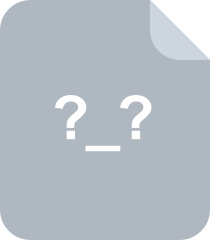


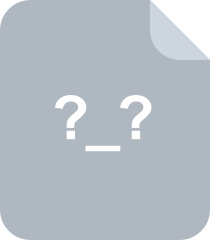
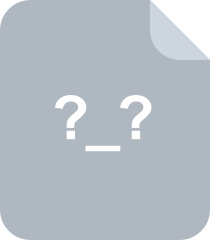



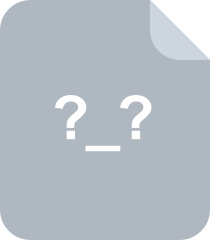
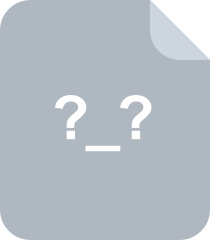

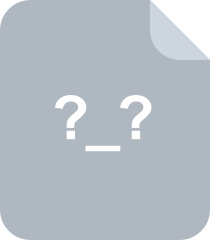
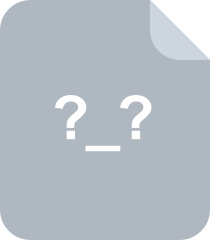
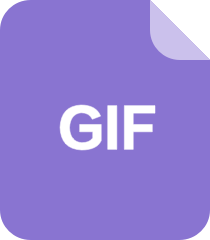
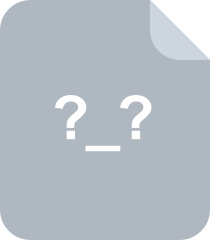
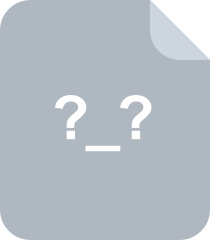
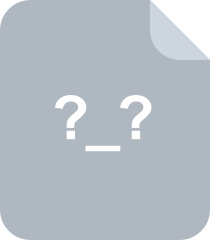
- 1
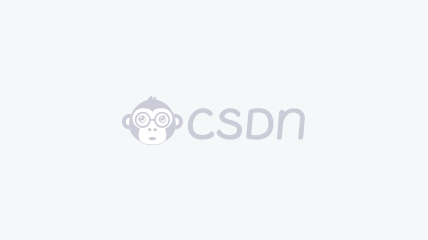

- 粉丝: 495
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

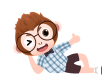
最新资源

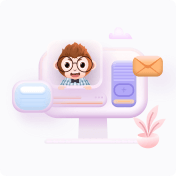
