swift-基于百度地图SDK的运动轨迹记录行进速度行进方向行走距离
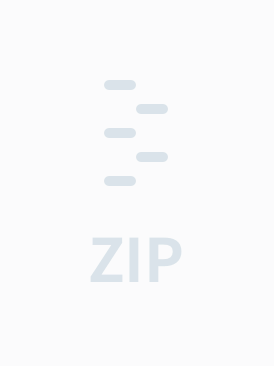

在Swift编程语言中,开发基于百度地图SDK的应用可以实现丰富的地图功能,如运动轨迹记录、行进速度计算、行进方向获取以及行走距离测量。这些功能对于健康追踪、导航、户外活动等多种应用场合非常实用。以下将详细阐述如何在Swift中利用百度地图SDK实现这些功能。 你需要在项目中集成百度地图SDK。这通常通过CocoaPods或Carthage进行管理,添加对应的依赖库。确保在Podfile或Cartfile中添加百度地图SDK的依赖,并执行相应的安装命令。 1. **集成百度地图SDK** - 使用CocoaPods:在Podfile中添加`pod 'BaiduMapAPI'`,然后运行`pod install`。 - 使用Carthage:在Cartfile中添加`github "BaiduLBS/BaiduMapAPI_iOS"`,接着运行`carthage update --platform iOS`。 2. **初始化地图** 在你的ViewController中导入`BaiduMapKit`框架,创建一个`BMKMapView`实例并设置其代理,以便接收地图相关的事件回调。 ```swift import BaiduMapKit class MyViewController: UIViewController, BMKMapViewDelegate { var mapView: BMKMapView! override func viewDidLoad() { super.viewDidLoad() // 初始化地图 mapView = BMKMapView(frame: view.bounds) mapView.delegate = self view.addSubview(mapView) } } ``` 3. **获取用户位置** 配置地图的定位服务,设置`BMKLocationService`对象并启动定位。记得在Info.plist中添加必要的隐私权限。 ```swift let locationService = BMKLocationService() locationService.delegate = self locationService.startUserLocationService() ``` 4. **轨迹记录与绘制** 实现`BMKMapViewDelegate`的`mapView(_:didUpdate:)`方法,此方法会返回用户的最新位置。在地图上添加一个新的`BMKMapPoint`,并使用`BMKPolyline`绘制轨迹。 ```swift var points: [BMKMapPoint] = [] func mapView(_ mapView: BMKMapView, didUpdate userLocation: BMKUserLocation) { let point = BMKMapPoint(x: userLocation.location.coordinate.longitude, y: userLocation.location.coordinate.latitude) points.append(point) if points.count > 2 { let polyline = BMKPolyline(points: points, count: points.count) mapView.addOverlay(polyline) } } ``` 5. **行进速度计算** 通过`userLocation.speed`属性获取用户当前的速度(单位:米/秒)。如果需要以公里/小时显示,可将其乘以3.6。 ```swift let speedKmPerHour = userLocation.speed * 3.6 ``` 6. **行进方向获取** 利用`userLocation.heading`获取设备的方向,它是一个角度,0代表正北,顺时针增加。 7. **行走距离测量** 计算两点之间的距离,可以使用`CLLocation`的`distance(from:)`方法。在每次位置更新时,累计距离。 ```swift var totalDistanceMeters = 0.0 func calculateDistance(point1: BMKMapPoint, point2: BMKMapPoint) -> CLLocationDistance { let location1 = CLLocation(latitude: point1.y, longitude: point1.x) let location2 = CLLocation(latitude: point2.y, longitude: point2.x) return location1.distance(from: location2) } func mapView(_ mapView: BMKMapView, didUpdate userLocation: BMKUserLocation) { // ... let distance = calculateDistance(point1: points.last!, point2: point) totalDistanceMeters += distance } ``` 8. **处理轨迹数据** `WZYRunningMap-master`可能是项目的源代码仓库,其中可能包含了实现这些功能的详细代码。如果你需要深入了解或定制功能,建议查看该项目的源代码,了解作者是如何处理地图数据、计算速度和距离的。 基于百度地图SDK的Swift开发可以实现运动轨迹记录、速度和方向计算以及行走距离测量等功能。通过不断迭代和优化,你可以创建出更加精准、用户友好的地图应用。
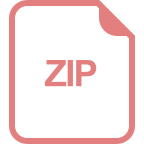
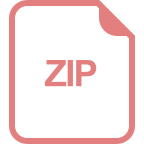
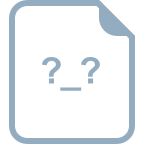
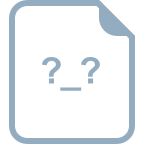
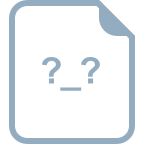
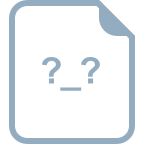
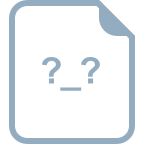
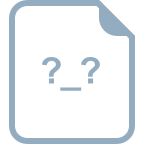
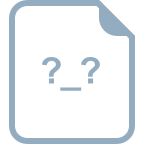
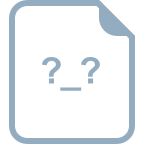
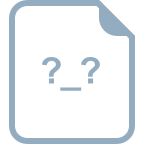
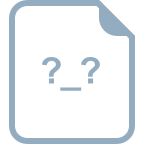
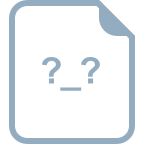
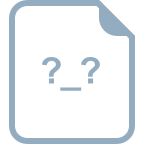
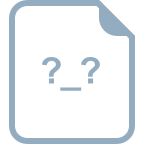
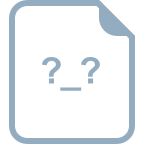
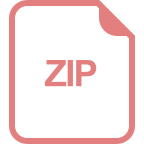
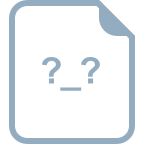
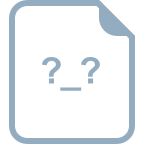
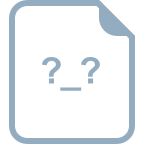
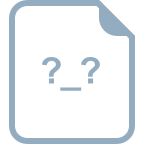
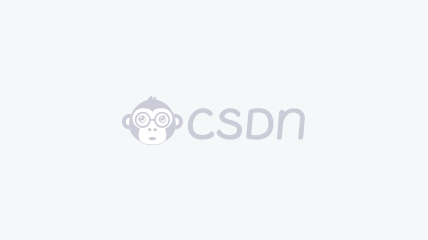

- 粉丝: 791
- 资源: 3万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

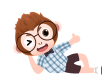
最新资源
- Windows检查电池健康度的批处理脚本实现
- 用HTML5和JavaScript实现动态过年鞭炮场景
- 快速排序在Go中的高效实现与应用
- 对象检测23-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 云原生-k8s知识学习-CKA考前培训
- Python实现HTML压缩功能
- 完结26章Java主流分布式解决方案多场景设计与实战
- ECSHOP模板堂最新2017仿E宠物模板 整合ECTouch微分销商城
- Pear Admin 是 一 款 开 箱 即 用 的 前 端 开 发 模 板,提供便捷快速的开发方式,延续 Admin 的设计规范
- 51单片机仿真摇号抽奖机源程序12864液晶显示仿真+程序

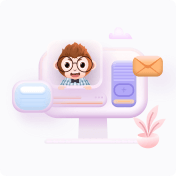
