
A PHP string manipulation library with multibyte support. Offers both OO method
chaining and a procedural-style static wrapper. Tested and compatible with
PHP 5.3+ and HHVM. Inspired by underscore.string.js.
[](https://travis-ci.org/danielstjules/Stringy)
* [Requiring/Loading](#requiringloading)
* [OO and Procedural](#oo-and-procedural)
* [Implemented Interfaces](#implemented-interfaces)
* [PHP 5.6 Creation](#php-56-creation)
* [Methods](#methods)
* [at](#at)
* [camelize](#camelize)
* [chars](#chars)
* [collapseWhitespace](#collapsewhitespace)
* [contains](#contains)
* [containsAll](#containsall)
* [containsAny](#containsany)
* [countSubstr](#countsubstr)
* [create](#create)
* [dasherize](#dasherize)
* [delimit](#delimit)
* [endsWith](#endswith)
* [ensureLeft](#ensureleft)
* [ensureRight](#ensureright)
* [first](#first)
* [getEncoding](#getencoding)
* [hasLowerCase](#haslowercase)
* [hasUpperCase](#hasuppercase)
* [htmlDecode](#htmldecode)
* [htmlEncode](#htmlencode)
* [humanize](#humanize)
* [indexOf](#indexof)
* [indexOfLast](#indexoflast)
* [insert](#insert)
* [isAlpha](#isalpha)
* [isAlphanumeric](#isalphanumeric)
* [isBlank](#isblank)
* [isHexadecimal](#ishexadecimal)
* [isJson](#isjson)
* [isLowerCase](#islowercase)
* [isSerialized](#isserialized)
* [isUpperCase](#isuppercase)
* [last](#last)
* [length](#length)
* [longestCommonPrefix](#longestcommonprefix)
* [longestCommonSuffix](#longestcommonsuffix)
* [longestCommonSubstring](#longestcommonsubstring)
* [lowerCaseFirst](#lowercasefirst)
* [pad](#pad)
* [padBoth](#padboth)
* [padLeft](#padleft)
* [padRight](#padright)
* [regexReplace](#regexreplace)
* [removeLeft](#removeleft)
* [removeRight](#removeright)
* [replace](#replace)
* [reverse](#reverse)
* [safeTruncate](#safetruncate)
* [shuffle](#shuffle)
* [slugify](#slugify)
* [startsWith](#startswith)
* [substr](#substr)
* [surround](#surround)
* [swapCase](#swapcase)
* [tidy](#tidy)
* [titleize](#titleize)
* [toAscii](#toascii)
* [toLowerCase](#tolowercase)
* [toSpaces](#tospaces)
* [toTabs](#totabs)
* [toTitleCase](#totitlecase)
* [toUpperCase](#touppercase)
* [trim](#trim)
* [trimLeft](#trimLeft)
* [trimRight](#trimRight)
* [truncate](#truncate)
* [underscored](#underscored)
* [upperCamelize](#uppercamelize)
* [upperCaseFirst](#uppercasefirst)
* [Links](#links)
* [Tests](#tests)
* [License](#license)
## Requiring/Loading
If you're using Composer to manage dependencies, you can include the following
in your composer.json file:
```json
{
"require": {
"danielstjules/stringy": "~1.10"
}
}
```
Then, after running `composer update` or `php composer.phar update`, you can
load the class using Composer's autoloading:
```php
require 'vendor/autoload.php';
```
Otherwise, you can simply require the file directly:
```php
require_once 'path/to/Stringy/src/Stringy.php';
// or
require_once 'path/to/Stringy/src/StaticStringy.php';
```
And in either case, I'd suggest using an alias.
```php
use Stringy\Stringy as S;
// or
use Stringy\StaticStringy as S;
```
## OO and Procedural
The library offers both OO method chaining with `Stringy\Stringy`, as well as
procedural-style static method calls with `Stringy\StaticStringy`. An example
of the former is the following:
```php
use Stringy\Stringy as S;
echo S::create('Fòô Bàř', 'UTF-8')->collapseWhitespace()->swapCase(); // 'fÒÔ bÀŘ'
```
`Stringy\Stringy` has a __toString() method, which returns the current string
when the object is used in a string context, ie:
`(string) S::create('foo') // 'foo'`
Using the static wrapper, an alternative is the following:
```php
use Stringy\StaticStringy as S;
$string = S::collapseWhitespace('Fòô Bàř', 'UTF-8');
echo S::swapCase($string, 'UTF-8'); // 'fÒÔ bÀŘ'
```
## Implemented Interfaces
`Stringy\Stringy` implements the `IteratorAggregate` interface, meaning that
`foreach` can be used with an instance of the class:
``` php
$stringy = S::create('Fòô Bàř', 'UTF-8');
foreach ($stringy as $char) {
echo $char;
}
// 'Fòô Bàř'
```
It implements the `Countable` interface, enabling the use of `count()` to
retrieve the number of characters in the string:
``` php
$stringy = S::create('Fòô', 'UTF-8');
count($stringy); // 3
```
Furthermore, the `ArrayAccess` interface has been implemented. As a result,
`isset()` can be used to check if a character at a specific index exists. And
since `Stringy\Stringy` is immutable, any call to `offsetSet` or `offsetUnset`
will throw an exception. `offsetGet` has been implemented, however, and accepts
both positive and negative indexes. Invalid indexes result in an
`OutOfBoundsException`.
``` php
$stringy = S::create('Bàř', 'UTF-8');
echo $stringy[2]; // 'ř'
echo $stringy[-2]; // 'à'
isset($stringy[-4]); // false
$stringy[3]; // OutOfBoundsException
$stringy[2] = 'a'; // Exception
```
## PHP 5.6 Creation
As of PHP 5.6, [`use function`](https://wiki.php.net/rfc/use_function) is
available for importing functions. Stringy exposes a namespaced function,
`Stringy\create`, which emits the same behaviour as `Stringy\Stringy::create()`.
If running PHP 5.6, or another runtime that supports the `use function` syntax,
you can take advantage of an even simpler API as seen below:
``` php
use function Stringy\create as s;
// Instead of: S::create('Fòô Bàř', 'UTF-8')
s('Fòô Bàř', 'UTF-8')->collapseWhitespace()->swapCase();
```
## Methods
In the list below, any static method other than S::create refers to a method in
`Stringy\StaticStringy`. For all others, they're found in `Stringy\Stringy`.
Furthermore, all methods that return a Stringy object or string do not modify
the original. Stringy objects are immutable.
*Note: If `$encoding` is not given, it defaults to `mb_internal_encoding()`.*
#### at
$stringy->at(int $index)
S::at(int $index [, string $encoding ])
Returns the character at $index, with indexes starting at 0.
```php
S::create('fòô bàř', 'UTF-8')->at(6);
S::at('fòô bàř', 6, 'UTF-8'); // 'ř'
```
#### camelize
$stringy->camelize();
S::camelize(string $str [, string $encoding ])
Returns a camelCase version of the string. Trims surrounding spaces,
capitalizes letters following digits, spaces, dashes and underscores,
and removes spaces, dashes, as well as underscores.
```php
S::create('Camel-Case')->camelize();
S::camelize('Camel-Case'); // 'camelCase'
```
#### chars
$stringy->chars();
S::chars(string $str [, string $encoding ])
Returns an array consisting of the characters in the string.
```php
S::create('Fòô Bàř', 'UTF-8')->chars();
S::chars('Fòô Bàř', 'UTF-8'); // array(F', 'ò', 'ô', ' ', 'B', 'à', 'ř')
```
#### collapseWhitespace
$stringy->collapseWhitespace()
S::collapseWhitespace(string $str [, string $encoding ])
Trims the string and replaces consecutive whitespace characters with a
single space. This includes tabs and newline characters, as well as
multibyte whitespace such as the thin space and ideographic space.
```php
S::create(' Ο συγγραφέας ')->collapseWhitespace();
S::collapseWhitespace(' Ο συγγραφέας '); // 'Ο συγγραφέας'
```
#### contains
$stringy->contains(string $needle [, boolean $caseSensitive = true ])
S::contains(string $haystack, string $needle [, boolean $caseSensitive = true [, string $encoding ]])
Returns true if the string contains $needle, false otherwise. By default,
the comparison is case-sensitive, but can be made insensitive
by setting $caseSensitive to false.
```php
S::create('Ο συγγραφέας �
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
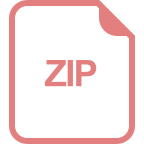
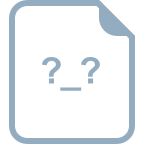
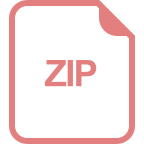
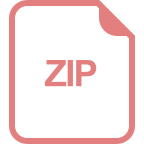
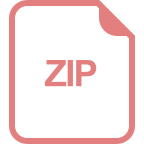
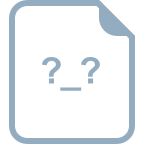
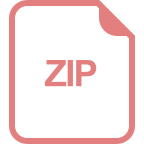
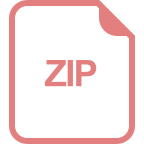
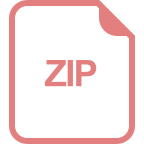
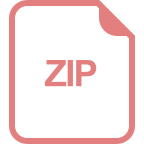
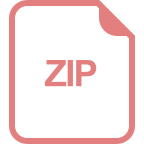
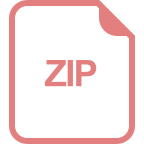
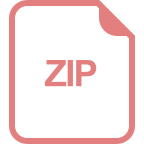
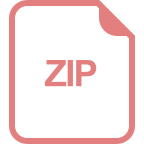
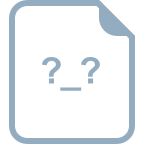
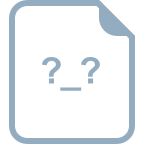
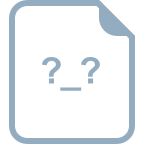
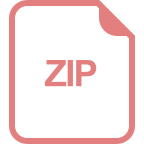
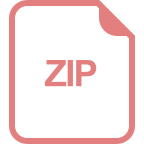
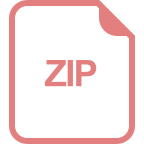
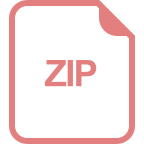
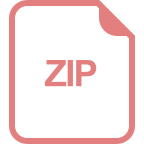
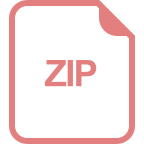
收起资源包目录

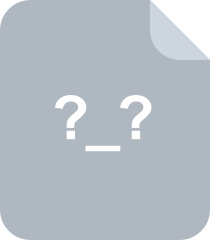
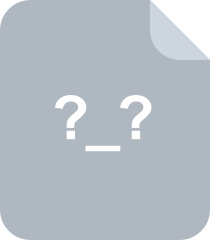
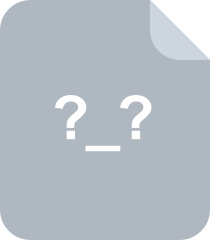
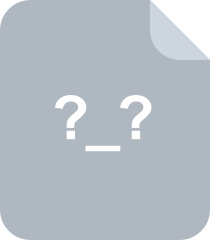
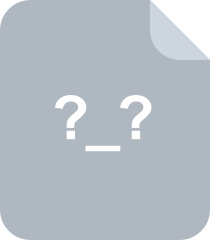
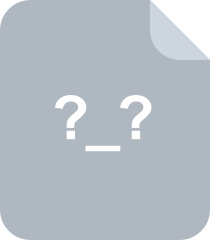
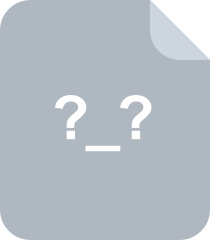
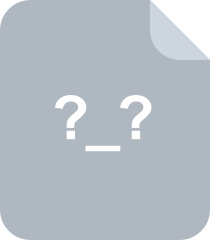
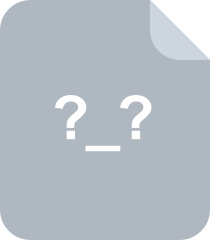
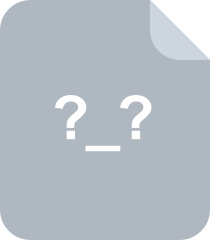
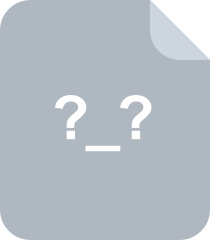
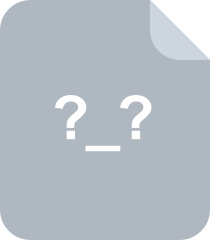
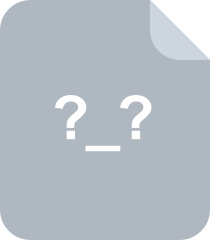
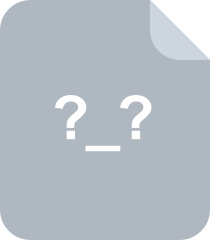
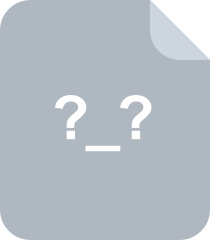
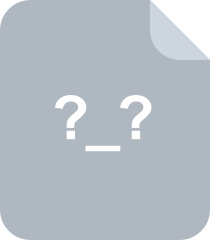
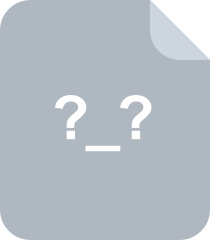
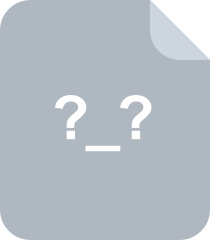
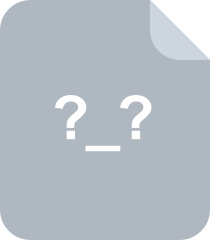
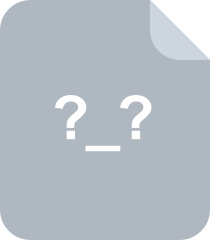
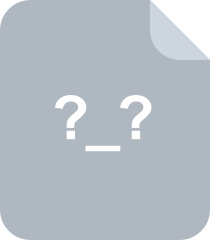
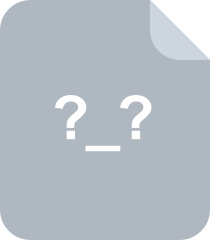
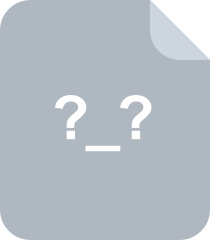
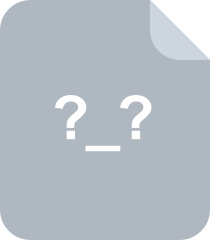
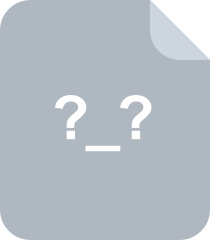
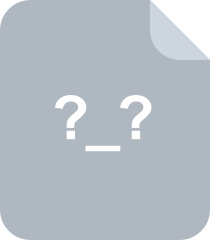
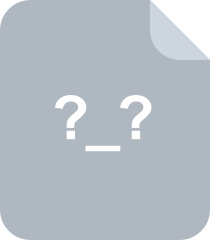
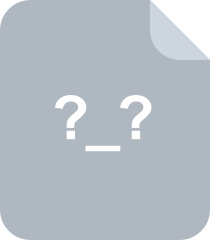
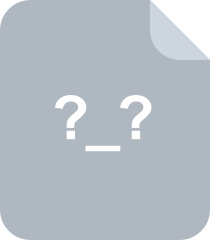
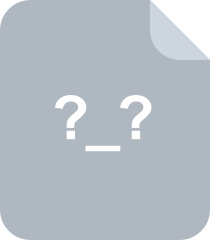
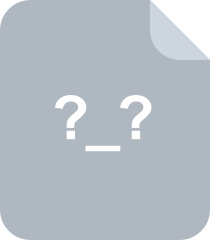
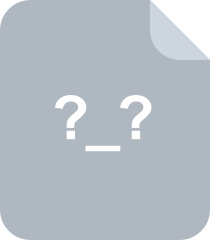
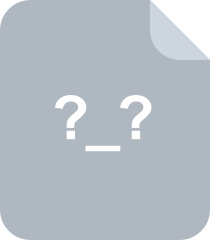
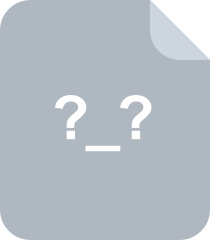
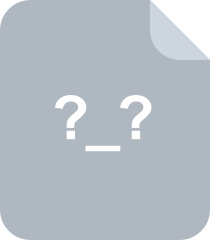
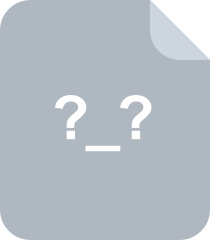
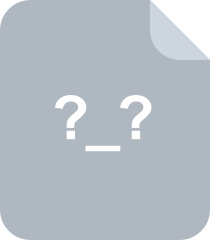
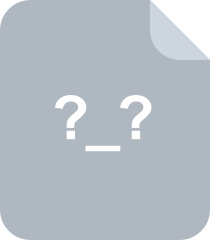
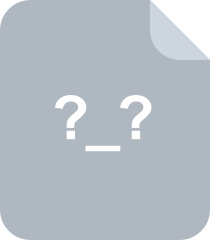
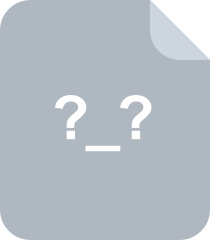
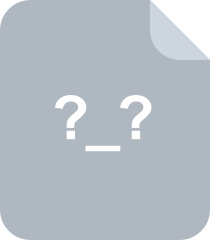
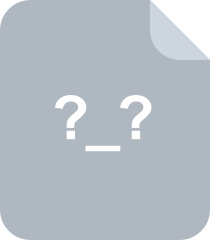
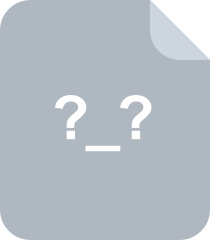
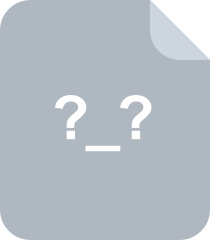
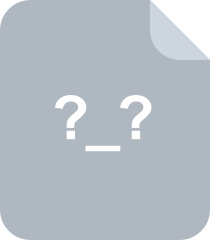
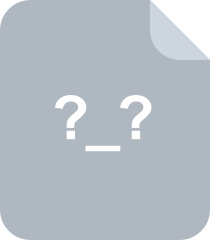
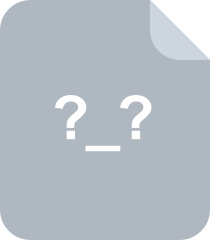
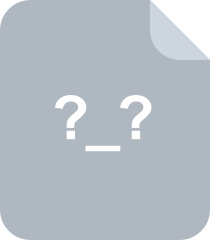
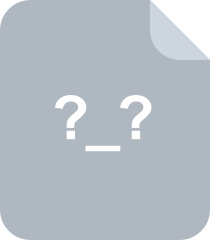
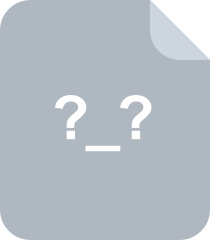
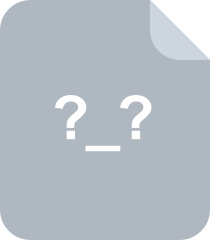
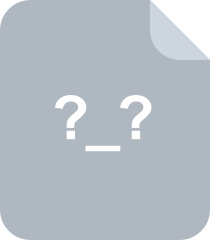
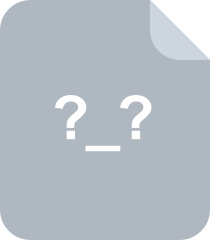
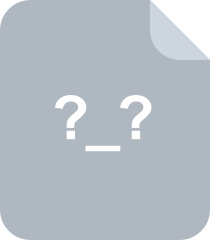
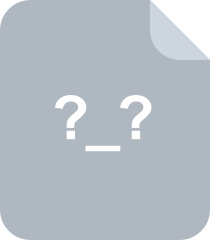
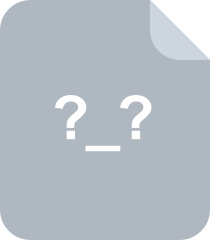
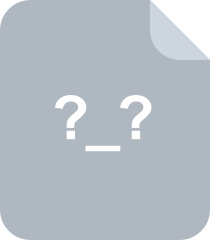
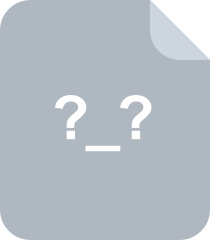
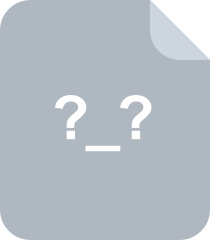
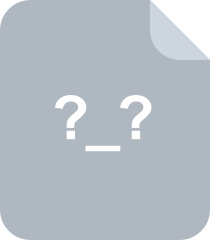
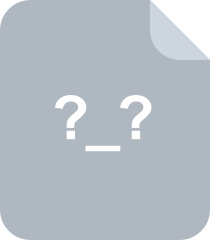
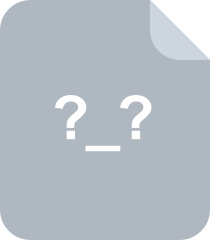
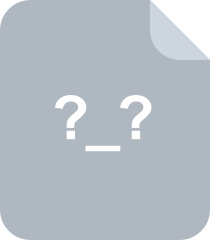
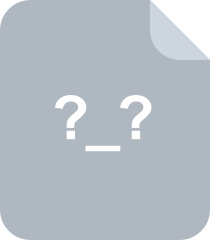
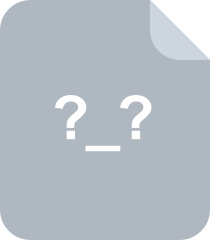
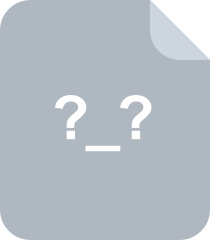
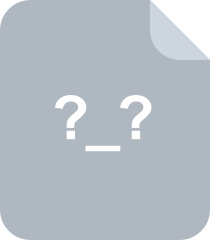
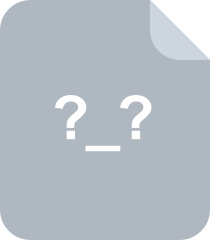
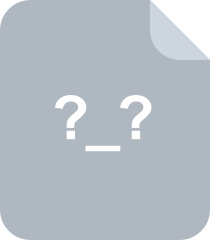
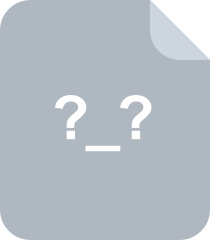
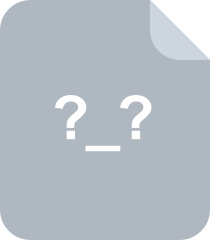
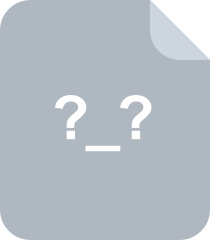
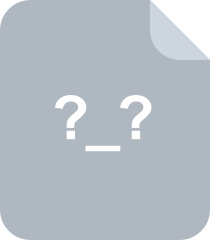
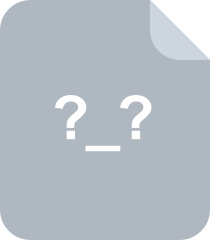
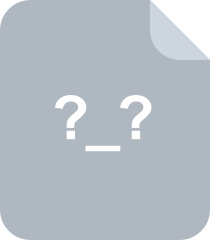
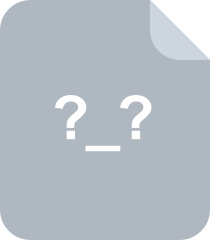
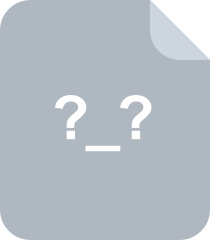
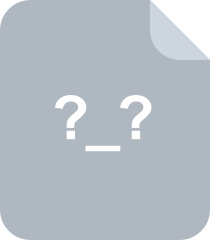
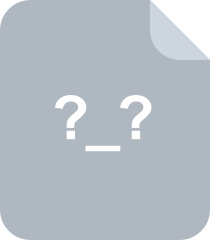
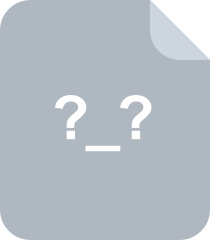
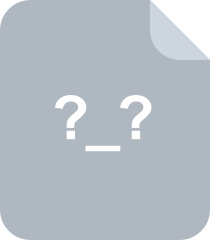
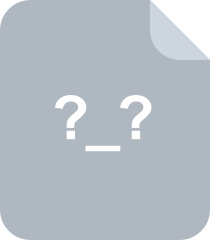
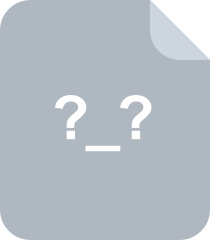
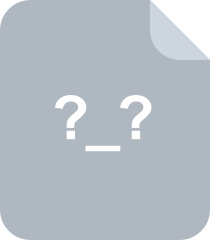
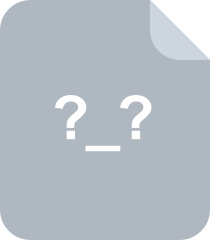
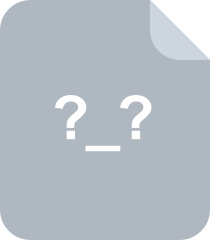
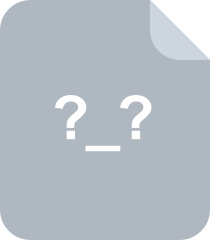
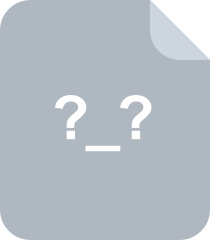
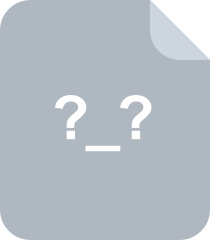
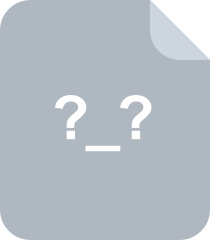
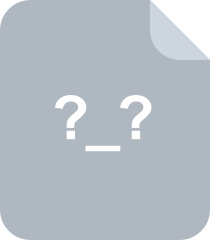
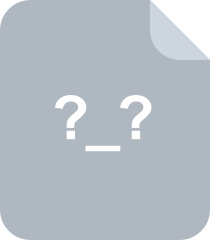
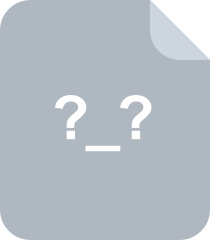
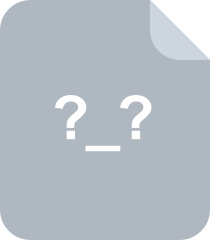
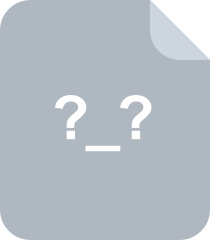
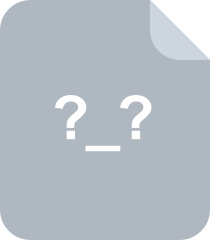
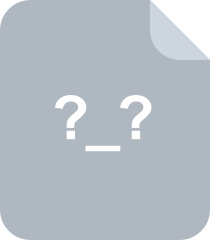
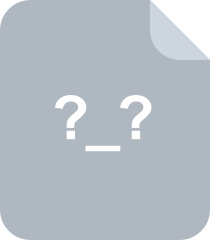
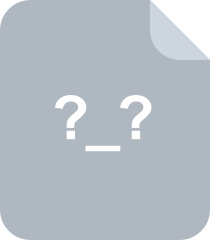
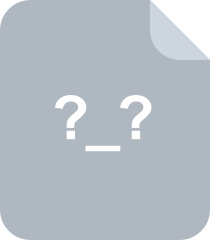
共 7897 条
- 1
- 2
- 3
- 4
- 5
- 6
- 79
资源评论
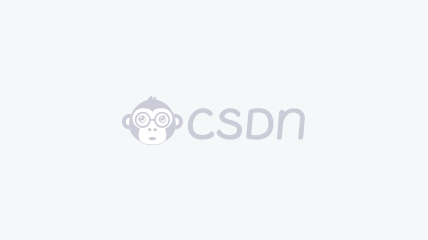

助力毕业
- 粉丝: 2192
- 资源: 5186
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

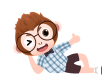
最新资源
- 基于鸿蒙Navigation系统路由表和Hvigor插件的动态路由方案(源码+说明文档).zip
- chromedriver-win64-131版本所有资源打包下载
- 百度手机输入法 v3.5.3.76 小米经典版.apk
- java项目,课程设计-#-ssm-mysql-个人健康信息管理系统.zip
- C#信息化ERP管理系统源码数据库 SQL2008源码类型 WebForm
- 【Phaser3.0】卡牌接龙
- Kettle(Pentaho Data Integration)社区版pdi-ce-10.2.0.0
- chromedriver-win64-132.zip
- C#ERP管理系统源码带文档数据库 SQL2008源码类型 WebForm
- 刘雨晨2309020147.pptx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


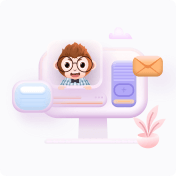
安全验证
文档复制为VIP权益,开通VIP直接复制
