<?php
class TP_yyToken implements ArrayAccess
{
public $string = '';
public $metadata = array();
public function __construct($s, $m = array())
{
if ($s instanceof TP_yyToken) {
$this->string = $s->string;
$this->metadata = $s->metadata;
} else {
$this->string = (string) $s;
if ($m instanceof TP_yyToken) {
$this->metadata = $m->metadata;
} elseif (is_array($m)) {
$this->metadata = $m;
}
}
}
public function __toString()
{
return $this->string;
}
public function offsetExists($offset)
{
return isset($this->metadata[$offset]);
}
public function offsetGet($offset)
{
return $this->metadata[$offset];
}
public function offsetSet($offset, $value)
{
if ($offset === null) {
if (isset($value[0])) {
$x = ($value instanceof TP_yyToken) ? $value->metadata : $value;
$this->metadata = array_merge($this->metadata, $x);
return;
}
$offset = count($this->metadata);
}
if ($value === null) {
return;
}
if ($value instanceof TP_yyToken) {
if ($value->metadata) {
$this->metadata[$offset] = $value->metadata;
}
} elseif ($value) {
$this->metadata[$offset] = $value;
}
}
public function offsetUnset($offset)
{
unset($this->metadata[$offset]);
}
}
class TP_yyStackEntry
{
public $stateno; /* The state-number */
public $major; /* The major token value. This is the code
** number for the token at this stack level */
public $minor; /* The user-supplied minor token value. This
** is the value of the token */
}
;
#line 13 "../smarty/lexer/smarty_internal_templateparser.y"
/**
* Smarty Internal Plugin Templateparser
*
* This is the template parser.
* It is generated from the smarty_internal_templateparser.y file
*
* @package Smarty
* @subpackage Compiler
* @author Uwe Tews
*/
class Smarty_Internal_Templateparser
{
#line 26 "../smarty/lexer/smarty_internal_templateparser.y"
const Err1 = "Security error: Call to private object member not allowed";
const Err2 = "Security error: Call to dynamic object member not allowed";
const Err3 = "PHP in template not allowed. Use SmartyBC to enable it";
/**
* result status
*
* @var bool
*/
public $successful = true;
/**
* return value
*
* @var mixed
*/
public $retvalue = 0;
/**
* counter for prefix code
*
* @var int
*/
public static $prefix_number = 0;
/**
* @var
*/
public $yymajor;
/**
* last index of array variable
*
* @var mixed
*/
public $last_index;
/**
* last variable name
*
* @var string
*/
public $last_variable;
/**
* root parse tree buffer
*
* @var Smarty_Internal_ParseTree
*/
public $root_buffer;
/**
* current parse tree object
*
* @var Smarty_Internal_ParseTree
*/
public $current_buffer;
/**
* lexer object
*
* @var Smarty_Internal_Templatelexer
*/
private $lex;
/**
* internal error flag
*
* @var bool
*/
private $internalError = false;
/**
* {strip} status
*
* @var bool
*/
public $strip = false;
/**
* compiler object
*
* @var Smarty_Internal_TemplateCompilerBase
*/
public $compiler = null;
/**
* smarty object
*
* @var Smarty
*/
public $smarty = null;
/**
* template object
*
* @var Smarty_Internal_Template
*/
public $template = null;
/**
* block nesting level
*
* @var int
*/
public $block_nesting_level = 0;
/**
* security object
*
* @var Smarty_Security
*/
private $security = null;
/**
* constructor
*
* @param Smarty_Internal_Templatelexer $lex
* @param Smarty_Internal_TemplateCompilerBase $compiler
*/
function __construct(Smarty_Internal_Templatelexer $lex, Smarty_Internal_TemplateCompilerBase $compiler)
{
$this->lex = $lex;
$this->compiler = $compiler;
$this->template = $this->compiler->template;
$this->smarty = $this->template->smarty;
$this->security = isset($this->smarty->security_policy) ? $this->smarty->security_policy : false;
$this->current_buffer = $this->root_buffer = new Smarty_Internal_ParseTree_Template($this);
}
/**
* insert PHP code in current buffer
*
* @param string $code
*/
public function insertPhpCode($code)
{
$this->current_buffer->append_subtree(new Smarty_Internal_ParseTree_Tag($this, $code));
}
/**
* merge PHP code with prefix code and return parse tree tag object
*
* @param string $code
*
* @return Smarty_Internal_ParseTree_Tag
*/
public function mergePrefixCode($code)
{
$tmp = '';
foreach ($this->compiler->prefix_code as $preCode) {
$tmp = empty($tmp) ? $preCode : $this->compiler->appendCode($tmp, $preCode);
}
$this->compiler->prefix_code = array();
$tmp = empty($tmp) ? $code : $this->compiler->appendCode($tmp, $code);
return new Smarty_Internal_ParseTree_Tag($this, $this->compiler->processNocacheCode($tmp, true));
}
const TP_VERT = 1;
const TP_COLON = 2;
const TP_PHP = 3;
const TP_NOCACHE = 4;
const TP_TEXT = 5;
const TP_STRIPON = 6;
const TP_STRIPOFF = 7;
const TP_BLOCKSOURCE = 8;
const TP_LITERALSTART = 9;
const TP_LITERALEND = 10;
const TP_LITERAL = 11;
const TP_RDEL = 12;
const TP_SIMPLEOUTPUT = 13;
const TP_LDEL = 14;
const TP_DOLLARID = 15;
const TP_EQUAL = 16;
const TP_SIMPLETAG = 17;
const TP_ID = 18;
const TP_PTR = 19;
const TP_LDELIF = 20;
const TP_LDELFOR = 21;
const TP_SEMICOLON = 22;
const TP_INCDEC = 23;
const TP_TO = 24;
const TP_STEP = 25;
const TP_LDELFOREACH = 26;
const TP_SPACE = 27;
const TP_AS = 28;
const TP_APTR = 29;
const TP_LDELSETFILTER = 30;
const TP_SMARTYBLOCKCHILDPARENT = 31;
const TP_CLOSETAG = 32;
const TP_LDELSLASH = 33;
const TP_ATTR = 34;
const TP_INTEGER = 35;
const TP_COMMA = 36;
const TP_OPENP = 37;
const TP_CLOSEP = 38;
const TP_MATH = 39;
const TP_UNIMATH = 40;
const TP_ISIN = 41;
const TP_INSTANCEOF = 42;
const TP_QMARK = 43;
const TP_NOT = 44;
const TP_TYPECAST = 45;
const TP_HEX = 46;
const TP_DOT = 47;
const TP_SINGLEQUOTESTRING = 48;
const TP_DOUBLECOLON = 49;
const TP_NAMESPACE = 50;
const TP_AT = 51;
const TP_HATCH = 52;
const TP_OPENB = 53;
const TP_CLOSEB = 54;
const TP_DOLLAR = 55;
const TP_LOGOP = 56;
const TP_TLOGOP = 57;
const TP_SINGLECOND = 58;
const TP_QUOTE = 59;
const TP_BACKTICK = 60;
const YY_NO_ACTION = 525;
const YY_ACCEPT_ACTION = 524;
const YY_ERROR_ACTION = 523;
const YY_SZ_ACTTAB = 1908;
static public $yy_action = array(287, 9, 129, 251, 273, 194, 441, 2, 82, 280, 281, 282, 216, 110, 353, 223, 212,
229, 441, 258, 217, 12, 199, 240, 32, 257, 257, 39, 17, 12, 25, 43, 42, 263, 224, 233, 17, 206, 441, 80, 1, 244,
311, 311, 172, 172, 52, 287, 9, 128, 441, 273, 65, 178, 2, 82, 268, 14, 184, 298, 110, 262, 13, 319, 229, 297,
258, 217, 31, 225, 12, 32, 170, 257, 39, 239, 189, 17, 43, 42, 263, 224, 292, 214, 206, 249, 80, 1, 113, 311,
164, 442, 172, 52, 287, 9, 132, 201
没有合适的资源?快使用搜索试试~ 我知道了~
基于PHP的711cms应用市场系统.zip
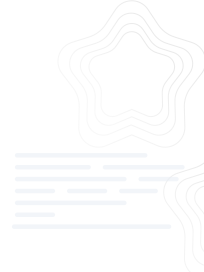
共1822个文件
php:761个
png:468个
js:282个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 70 浏览量
2023-07-17
23:52:19
上传
评论
收藏 11.01MB ZIP 举报
温馨提示
基于PHP的711cms应用市场系统.zip
资源推荐
资源详情
资源评论
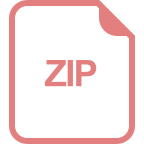
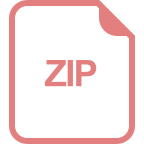
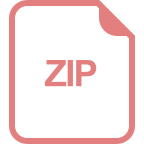
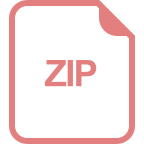
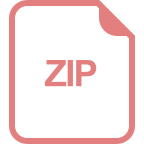
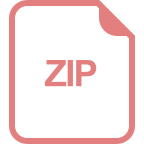
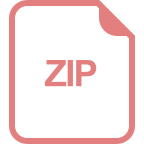
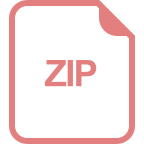
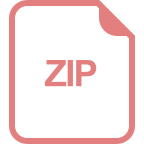
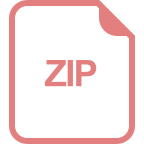
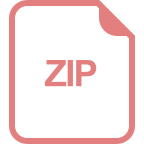
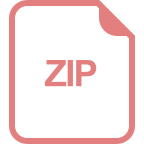
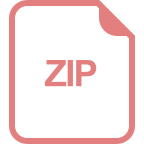
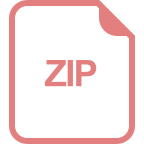
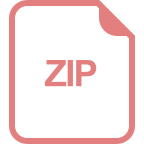
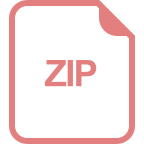
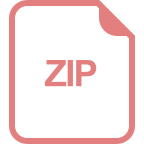
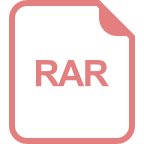
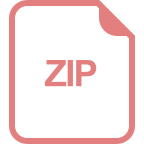
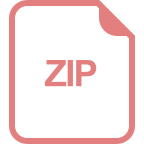
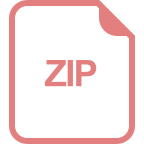
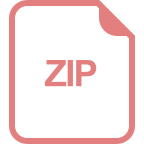
收起资源包目录

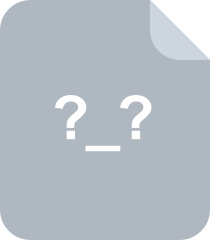
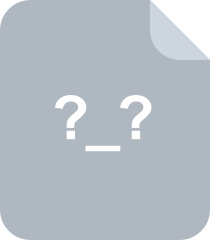
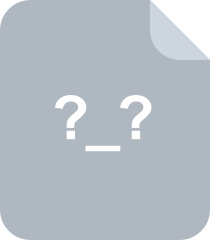
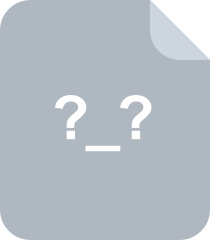
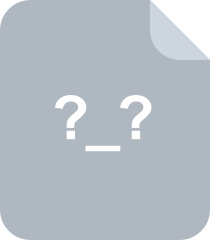
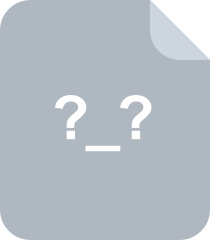
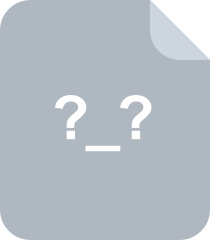
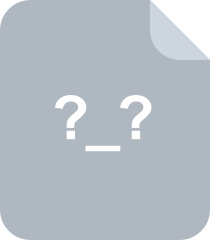
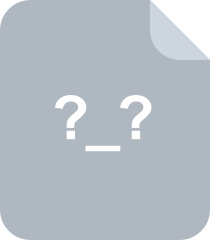
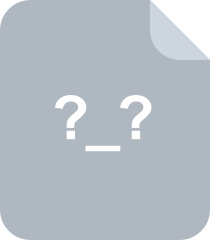
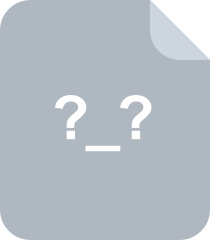
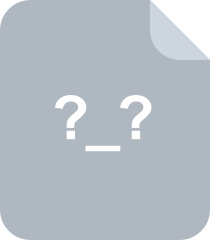
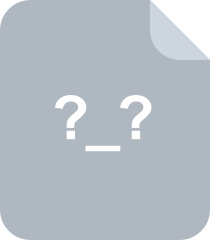
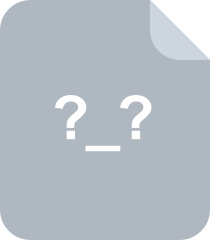
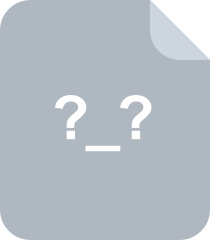
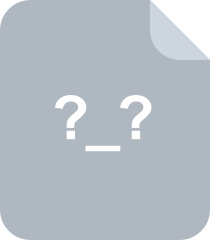
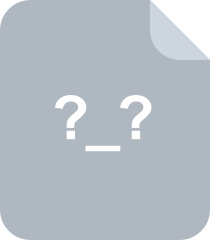
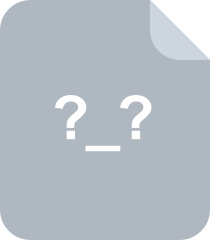
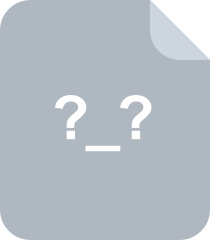
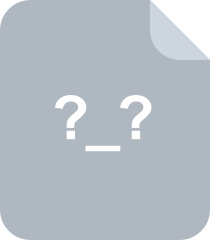
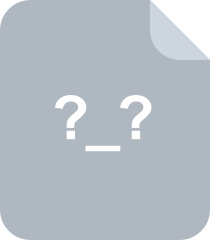
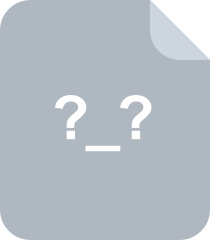
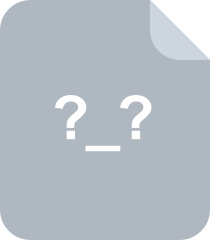
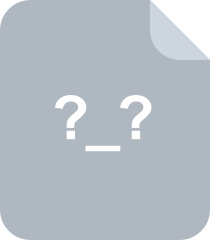
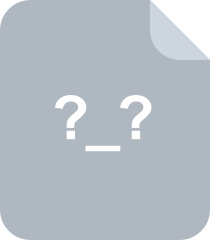
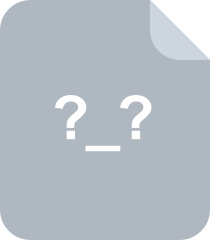
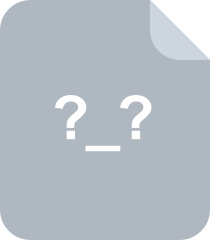
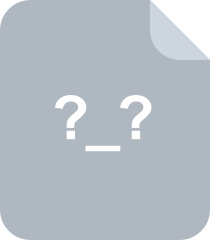
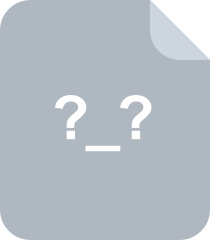
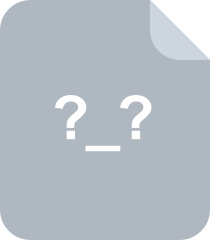
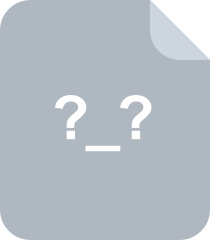
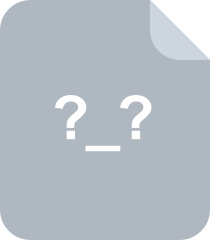
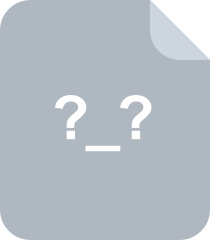
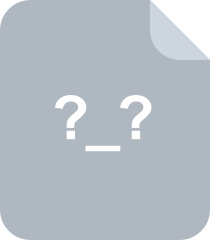
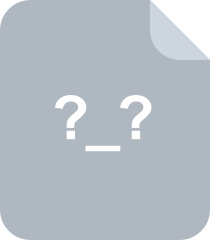
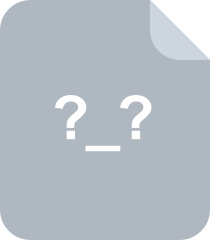
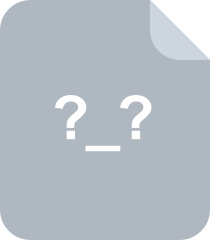
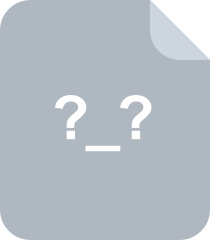
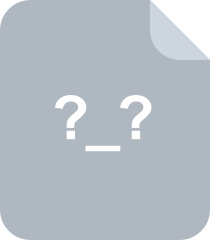
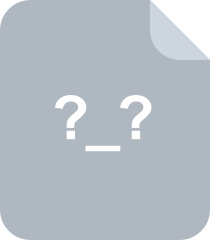
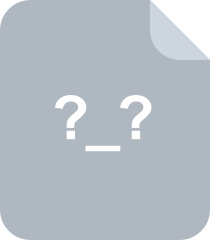
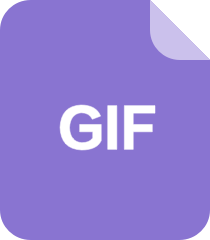
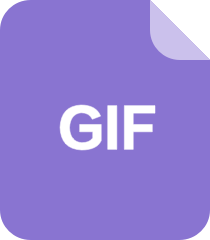
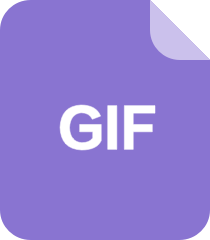
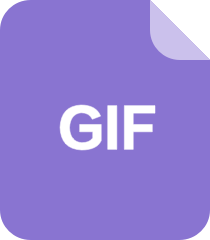
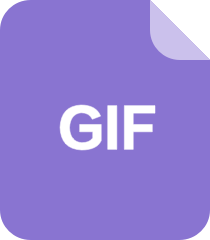
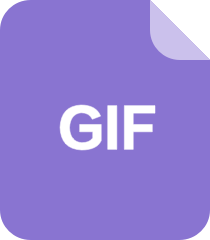
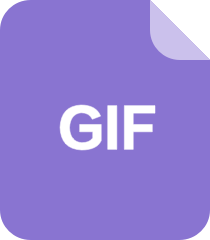
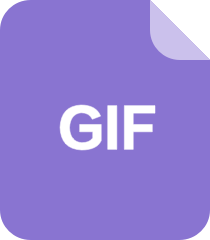
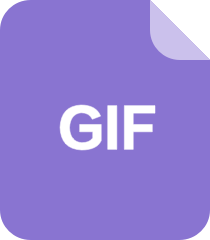
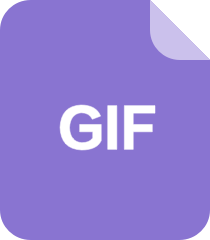
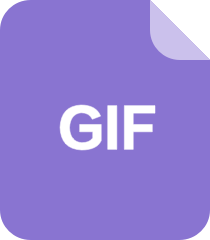
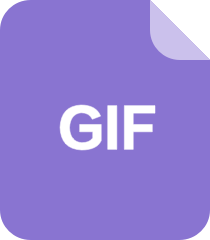
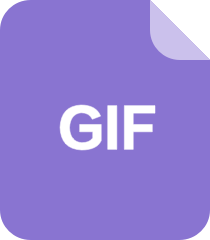
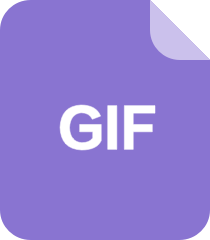
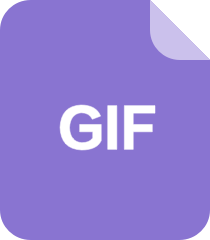
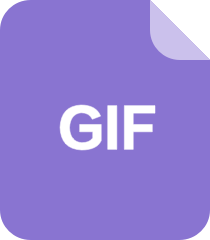
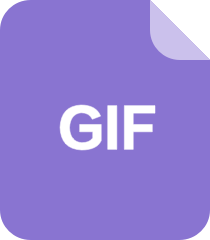
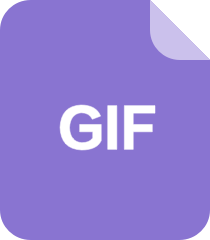
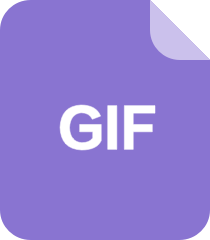
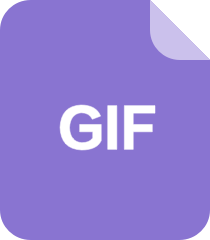
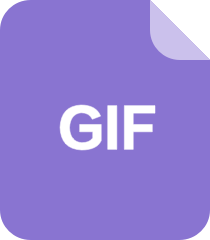
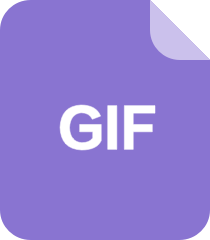
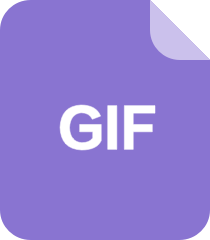
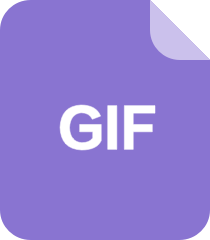
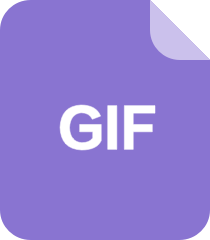
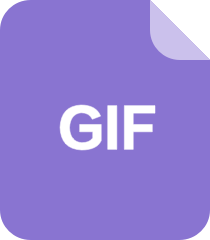
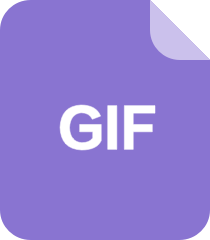
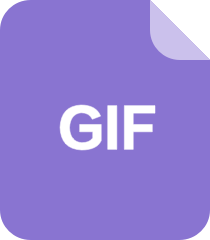
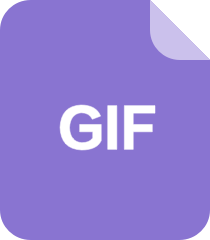
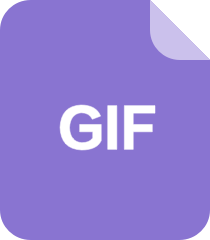
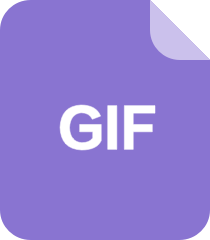
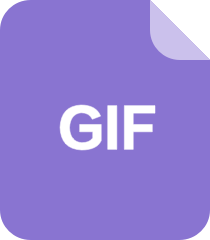
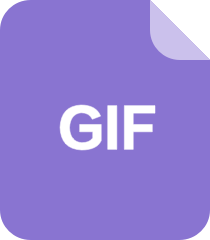
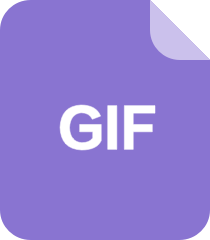
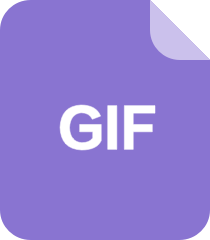
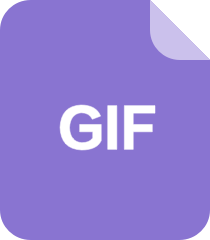
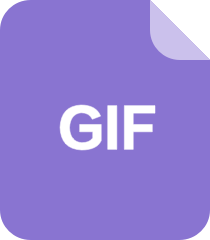
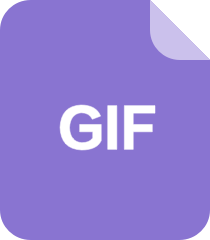
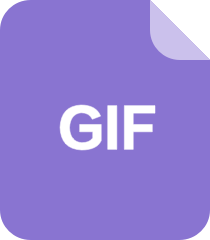
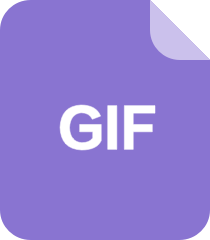
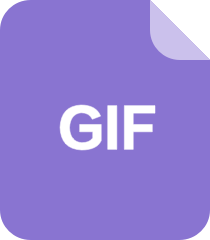
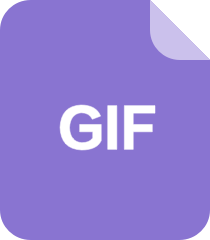
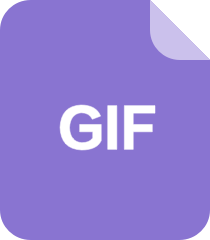
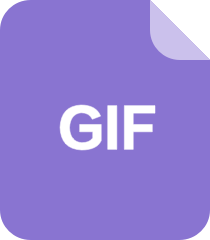
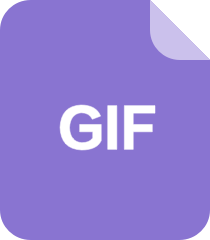
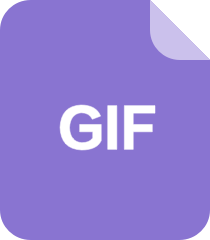
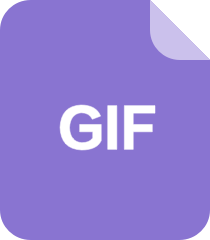
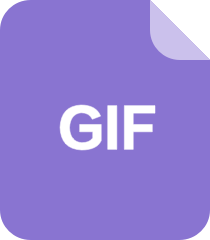
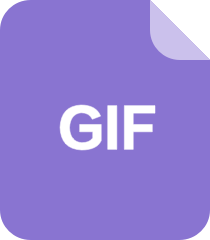
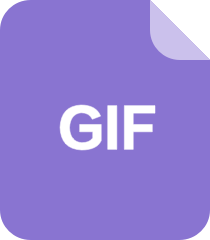
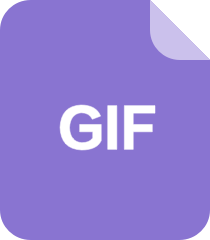
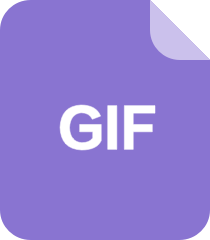
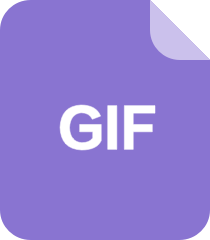
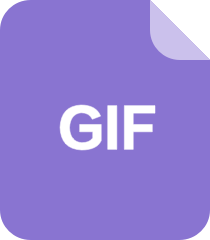
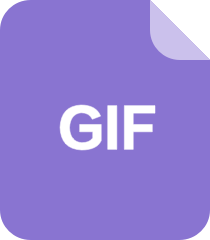
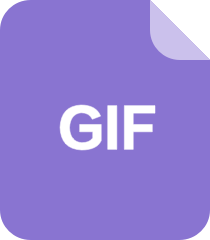
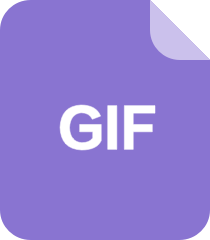
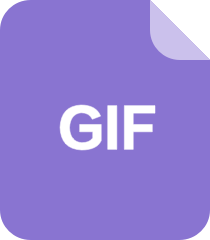
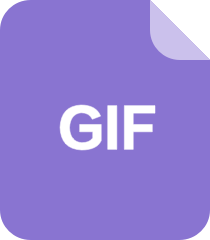
共 1822 条
- 1
- 2
- 3
- 4
- 5
- 6
- 19
资源评论
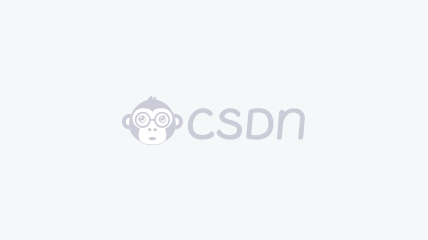

助力毕业
- 粉丝: 2182
- 资源: 5155
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

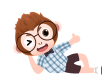
最新资源
- 在日常生活中我们常常遇到需要音视频
- Java项目-基于SSM+Vue的病人跟踪治疗信息管理系统的设计与实现(源码+数据库脚本+部署视频+代码讲解视频+全套软件)
- 5G r18最新专利研究.docx
- Java项目基于SSM实现的演出道具租赁管理系统+代码+论文+答辩PPT
- Java项目-基于SSM+Vue的驾校预约管理系统.的设计与实现(源码+数据库脚本+部署视频+代码讲解视频+全套软件)
- Java项目基于SSM实现的养老院管理系统+代码+论文+答辩PPT
- 订单管理系统的示例代码.rar
- 通信原理实验报告.zip
- python语言+PyQt5实现日历(高分课程设计)
- 计算机视觉技术(CV)详解.pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


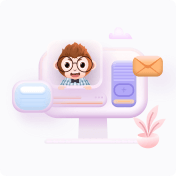
安全验证
文档复制为VIP权益,开通VIP直接复制
