; generated by Component: ARM Compiler 5.06 update 7 (build 960) Tool: ArmCC [4d365d]
; commandline ArmCC [--list --debug -c --asm --interleave -o.\stm32f10x_can.o --asm_dir=.\ --list_dir=.\ --depend=.\stm32f10x_can.d --cpu=Cortex-M3 --apcs=interwork -O0 --diag_suppress=9931 -I.\lib\inc -IC:\Keil_v5\ARM\RV31\INC -IC:\Keil_v5\ARM\CMSIS\Include -IC:\Keil_v5\ARM\INC\ST\STM32F10x -D__UVISION_VERSION=533 -DVECT_TAB_RAM --omf_browse=.\stm32f10x_can.crf lib\src\stm32f10x_can.c]
THUMB
AREA ||.text||, CODE, READONLY, ALIGN=2
CAN_DeInit PROC
;;;89 *******************************************************************************/
;;;90 void CAN_DeInit(void)
000000 b510 PUSH {r4,lr}
;;;91 {
;;;92 /* Enable CAN reset state */
;;;93 RCC_APB1PeriphResetCmd(RCC_APB1Periph_CAN, ENABLE);
000002 2101 MOVS r1,#1
000004 0648 LSLS r0,r1,#25
000006 f7fffffe BL RCC_APB1PeriphResetCmd
;;;94 /* Release CAN from reset state */
;;;95 RCC_APB1PeriphResetCmd(RCC_APB1Periph_CAN, DISABLE);
00000a 2100 MOVS r1,#0
00000c f04f7000 MOV r0,#0x2000000
000010 f7fffffe BL RCC_APB1PeriphResetCmd
;;;96 }
000014 bd10 POP {r4,pc}
;;;97
ENDP
CAN_Init PROC
;;;107 *******************************************************************************/
;;;108 u8 CAN_Init(CAN_InitTypeDef* CAN_InitStruct)
000016 b510 PUSH {r4,lr}
;;;109 {
000018 4601 MOV r1,r0
;;;110 u8 InitStatus = 0;
00001a 2000 MOVS r0,#0
;;;111 u16 WaitAck;
;;;112
;;;113 /* Check the parameters */
;;;114 assert_param(IS_FUNCTIONAL_STATE(CAN_InitStruct->CAN_TTCM));
;;;115 assert_param(IS_FUNCTIONAL_STATE(CAN_InitStruct->CAN_ABOM));
;;;116 assert_param(IS_FUNCTIONAL_STATE(CAN_InitStruct->CAN_AWUM));
;;;117 assert_param(IS_FUNCTIONAL_STATE(CAN_InitStruct->CAN_NART));
;;;118 assert_param(IS_FUNCTIONAL_STATE(CAN_InitStruct->CAN_RFLM));
;;;119 assert_param(IS_FUNCTIONAL_STATE(CAN_InitStruct->CAN_TXFP));
;;;120 assert_param(IS_CAN_MODE(CAN_InitStruct->CAN_Mode));
;;;121 assert_param(IS_CAN_SJW(CAN_InitStruct->CAN_SJW));
;;;122 assert_param(IS_CAN_BS1(CAN_InitStruct->CAN_BS1));
;;;123 assert_param(IS_CAN_BS2(CAN_InitStruct->CAN_BS2));
;;;124 assert_param(IS_CAN_PRESCALER(CAN_InitStruct->CAN_Prescaler));
;;;125
;;;126 /* Request initialisation */
;;;127 CAN->MCR = CAN_MCR_INRQ;
00001c 2301 MOVS r3,#1
00001e 4cfa LDR r4,|L1.1032|
000020 6023 STR r3,[r4,#0]
;;;128
;;;129 /* ...and check acknowledged */
;;;130 if ((CAN->MSR & CAN_MSR_INAK) == 0)
000022 1d23 ADDS r3,r4,#4
000024 681b LDR r3,[r3,#0]
000026 f0030301 AND r3,r3,#1
00002a b903 CBNZ r3,|L1.46|
;;;131 {
;;;132 InitStatus = CANINITFAILED;
00002c e084 B |L1.312|
|L1.46|
;;;133 }
;;;134 else
;;;135 {
;;;136 /* Set the time triggered communication mode */
;;;137 if (CAN_InitStruct->CAN_TTCM == ENABLE)
00002e 780b LDRB r3,[r1,#0]
000030 2b01 CMP r3,#1
000032 d106 BNE |L1.66|
;;;138 {
;;;139 CAN->MCR |= CAN_MCR_TTCM;
000034 4bf4 LDR r3,|L1.1032|
000036 681b LDR r3,[r3,#0]
000038 f0430380 ORR r3,r3,#0x80
00003c 4cf2 LDR r4,|L1.1032|
00003e 6023 STR r3,[r4,#0]
000040 e005 B |L1.78|
|L1.66|
;;;140 }
;;;141 else
;;;142 {
;;;143 CAN->MCR &= ~CAN_MCR_TTCM;
000042 4bf1 LDR r3,|L1.1032|
000044 681b LDR r3,[r3,#0]
000046 f0230380 BIC r3,r3,#0x80
00004a 4cef LDR r4,|L1.1032|
00004c 6023 STR r3,[r4,#0]
|L1.78|
;;;144 }
;;;145
;;;146 /* Set the automatic bus-off management */
;;;147 if (CAN_InitStruct->CAN_ABOM == ENABLE)
00004e 784b LDRB r3,[r1,#1]
000050 2b01 CMP r3,#1
000052 d106 BNE |L1.98|
;;;148 {
;;;149 CAN->MCR |= CAN_MCR_ABOM;
000054 4bec LDR r3,|L1.1032|
000056 681b LDR r3,[r3,#0]
000058 f0430340 ORR r3,r3,#0x40
00005c 4cea LDR r4,|L1.1032|
00005e 6023 STR r3,[r4,#0]
000060 e005 B |L1.110|
|L1.98|
;;;150 }
;;;151 else
;;;152 {
;;;153 CAN->MCR &= ~CAN_MCR_ABOM;
000062 4be9 LDR r3,|L1.1032|
000064 681b LDR r3,[r3,#0]
000066 f0230340 BIC r3,r3,#0x40
00006a 4ce7 LDR r4,|L1.1032|
00006c 6023 STR r3,[r4,#0]
|L1.110|
;;;154 }
;;;155
;;;156 /* Set the automatic wake-up mode */
;;;157 if (CAN_InitStruct->CAN_AWUM == ENABLE)
00006e 788b LDRB r3,[r1,#2]
000070 2b01 CMP r3,#1
000072 d106 BNE |L1.130|
;;;158 {
;;;159 CAN->MCR |= CAN_MCR_AWUM;
000074 4be4 LDR r3,|L1.1032|
000076 681b LDR r3,[r3,#0]
000078 f0430320 ORR r3,r3,#0x20
00007c 4ce2 LDR r4,|L1.1032|
00007e 6023 STR r3,[r4,#0]
000080 e005 B |L1.142|
|L1.130|
;;;160 }
;;;161 else
;;;162 {
;;;163 CAN->MCR &= ~CAN_MCR_AWUM;
000082 4be1 LDR r3,|L1.1032|
000084 681b LDR r3,[r3,#0]
000086 f0230320 BIC r3,r3,#0x20
00008a 4cdf LDR r4,|L1.1032|
00008c 6023 STR r3,[r4,#0]
|L1.142|
;;;164 }
;;;165
;;;166 /* Set the no automatic retransmission */
;;;167 if (CAN_InitStruct->CAN_NART == ENABLE)
00008e 78cb LDRB r3,[r1,#3]
000090 2b01 CMP r3,#1
000092 d106 BNE |L1.162|
;;;168 {
;;;169 CAN->MCR |= CAN_MCR_NART;
000094 4bdc LDR r3,|L1.1032|
000096 681b LDR r3,[r3,#0]
000098 f0430310 ORR r3,r3,#0x10
00009c 4cda LDR r4,|L1.1032|
00009e 6023 STR r3,[r4,#0]
0000a0 e005 B |L1.174|
|L1.162|
;;;170 }
;;;171 else
;;;172 {
;;;173 CAN->MCR &= ~CAN_MCR_NART;
0000a2 4bd9 LDR r3,|L1.1032|
0000a4 681b LDR r3,[r3,#0]
0000a6 f0230310 BIC r3,r3,#0x10
0000aa 4cd7 LDR r4,|L1.1032|
0000ac 6023 STR r3,[r4,#0]
|L1.174|
;;;174 }
;;;175
;;;176 /* Set the receive FIFO locked mode */
;;;177 if (CAN_InitStruct->CAN_RFLM == ENABLE)
0000ae 790b LDRB r3,[r1,#4]
0000b0 2b01 CMP r3,#1
0000b2 d106 BNE |L1.194|
;;;178 {
;;;179 CAN->MCR |= CAN_MCR_RFLM;
0000b4 4bd4 LDR r3,|L1.1032|
0000b6 681b LDR r3,[r3,#0]
0000b8 f0430308 ORR r3,r3,#8
0000bc 4cd2 LDR r4,|L1.1032|
0000be 6023 STR r3,[r4,#0]
0000c0 e005 B |L1.206|
|L1.194|
;;;180
没有合适的资源?快使用搜索试试~ 我知道了~
嵌入式物联网项目实战-STM32F103实现CAN通讯polling方式
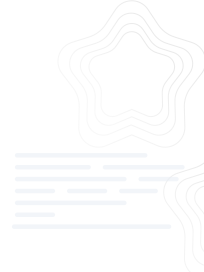
共140个文件
h:35个
c:29个
txt:14个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 173 浏览量
2024-03-04
22:14:40
上传
评论
收藏 1.06MB RAR 举报
温馨提示
1、嵌入式物联网单片机项目开发实战,每个例程都经过实战检验,简单好用。 2、代码使用KEIL 标准库开发,当前在STM32F407V运行,如果是STM32F407其他型号芯片,依然适用,请自行更改KEIL芯片型号以及FLASH容量即可。 3、软件下载时,请注意keil选择项是jlink还是stlink。 4、技术v:wulianjishu666; 5、如果接入其他传感器,请查看发布的其他资料。 6、单片机与模块的接线,在代码当中均有定义,请自行对照。
资源推荐
资源详情
资源评论
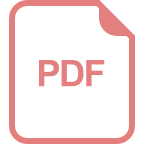
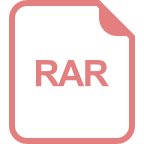
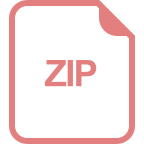
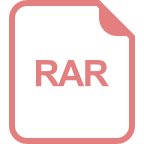
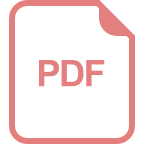
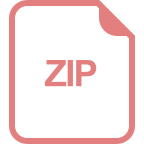
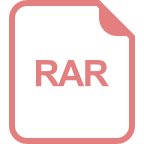
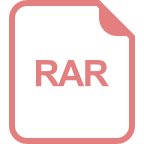
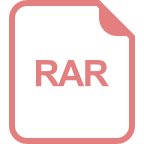
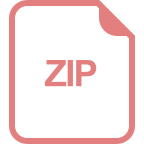
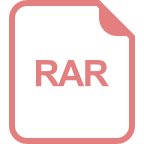
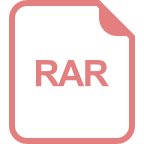
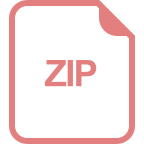
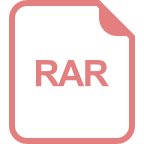
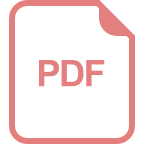
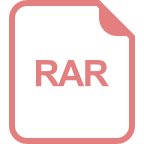
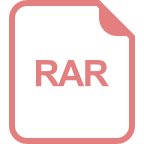
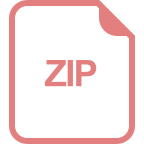
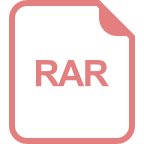
收起资源包目录

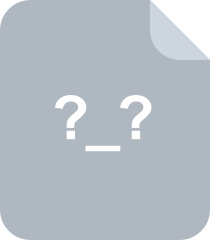
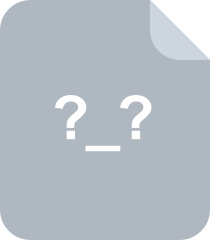
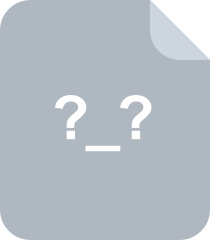
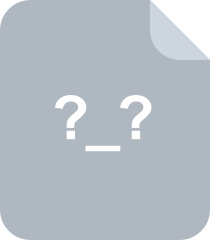
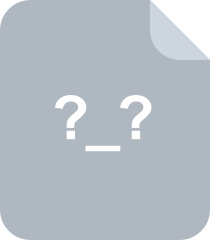
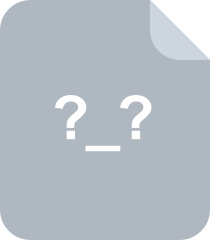
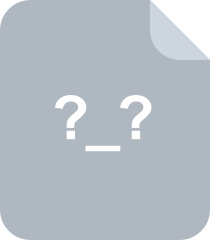
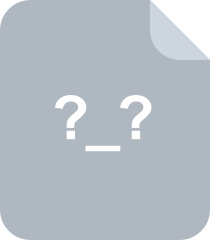
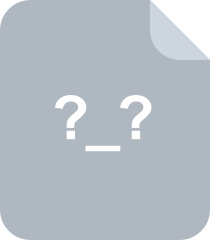
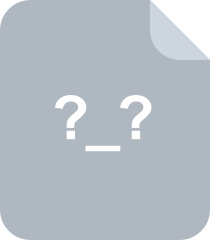
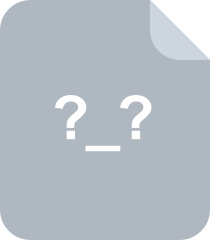
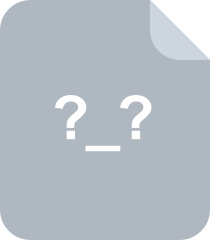
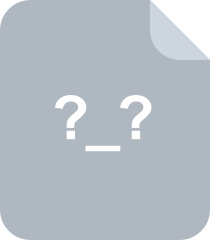
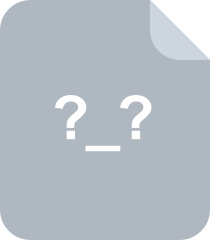
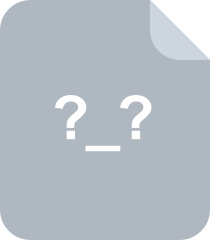
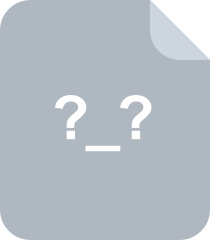
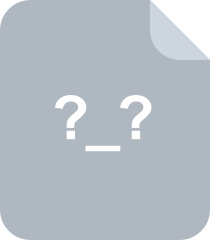
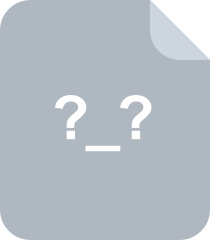
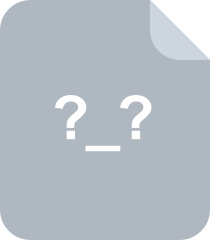
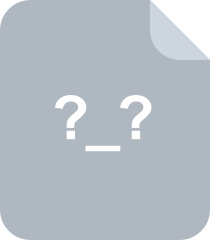
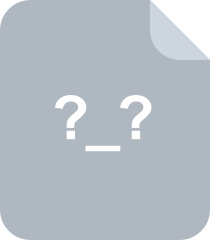
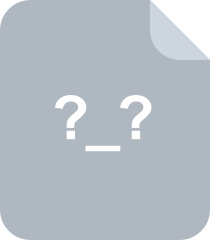
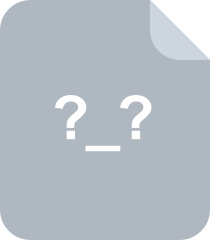
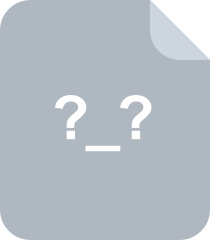
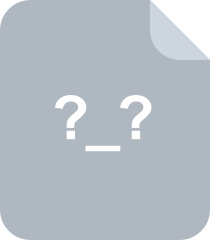
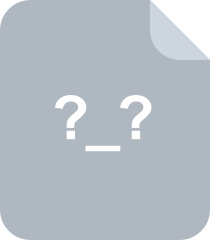
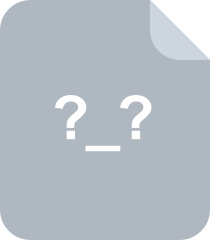
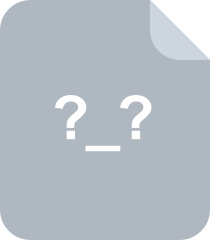
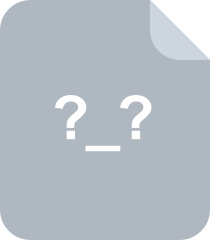
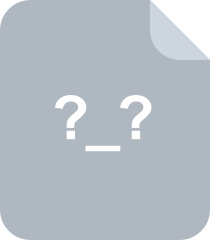
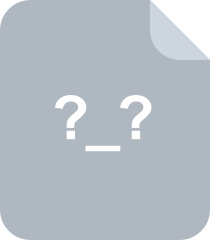
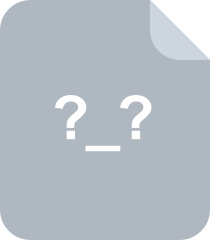
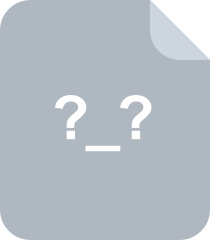
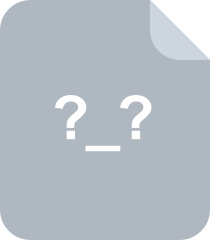
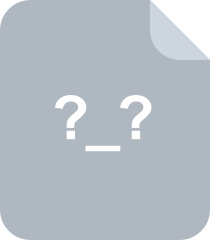
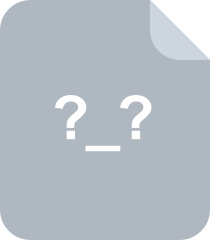
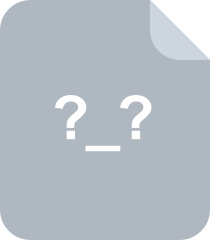
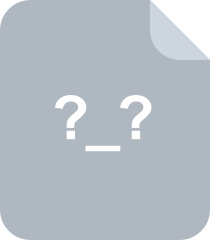
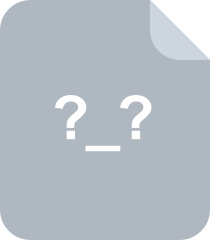
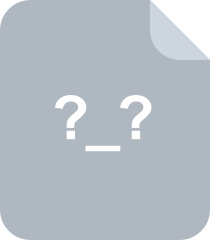
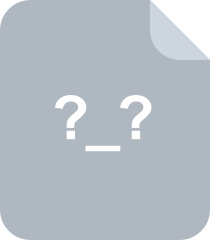
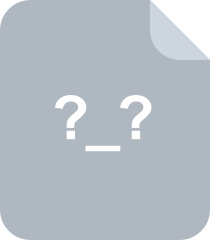
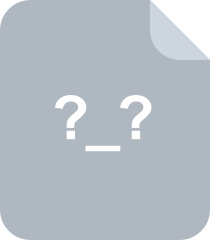
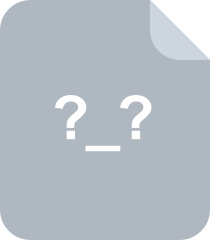
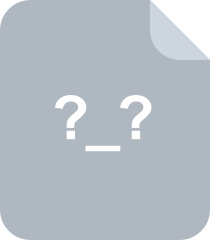
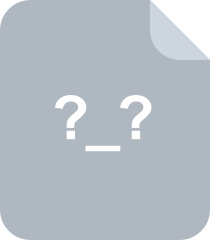
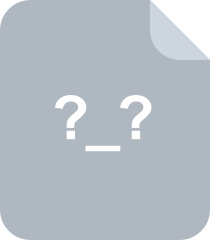
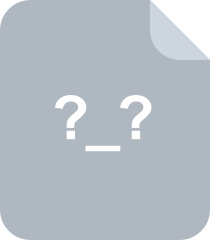
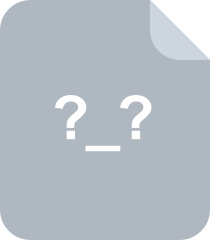
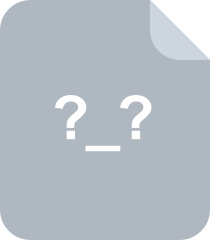
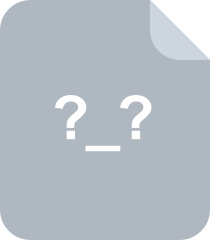
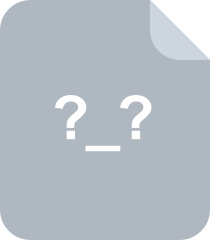
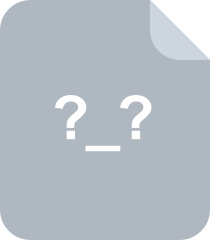
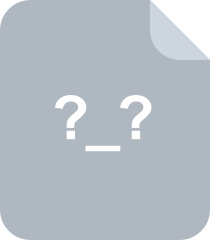
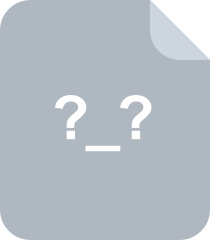
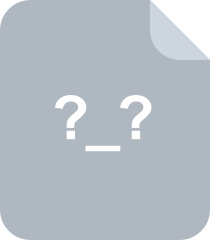
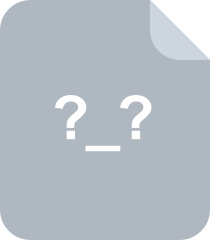
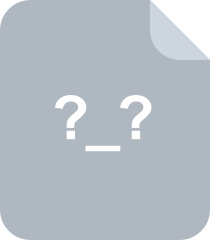
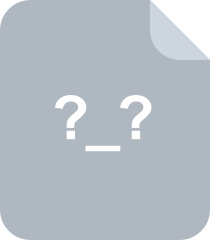
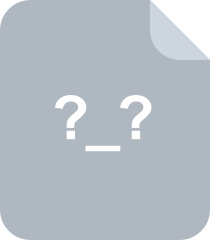
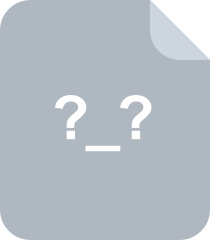
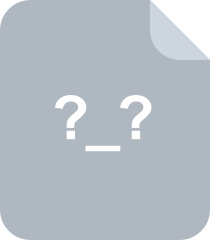
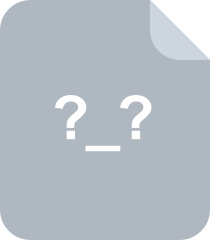
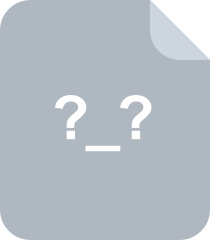
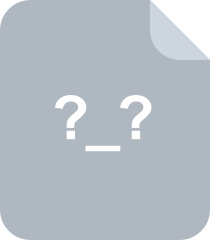
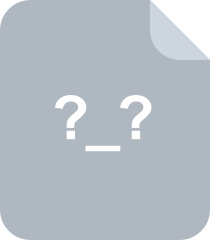
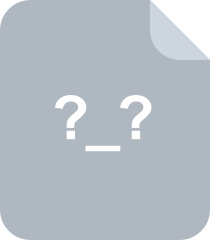
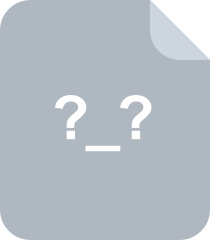
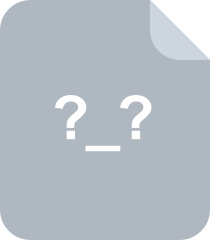
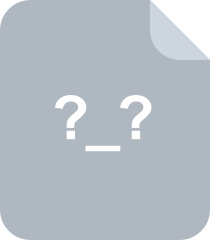
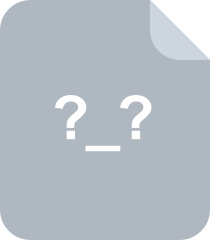
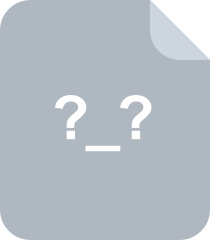
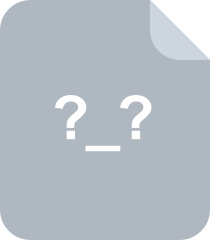
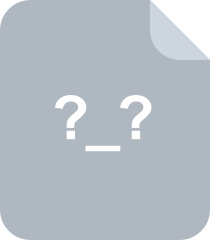
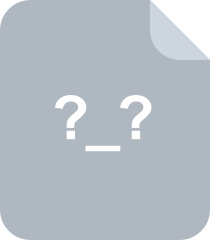
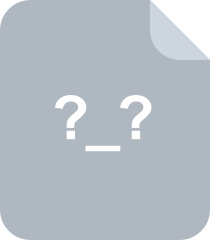
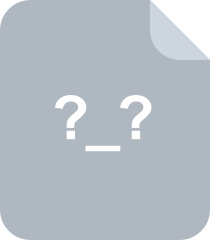
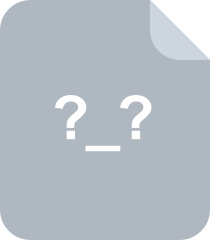
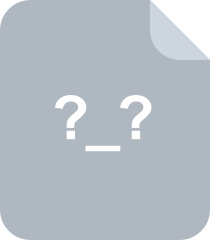
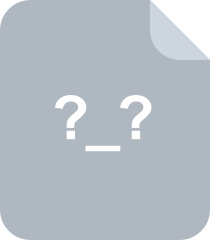
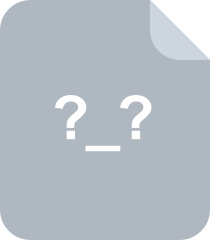
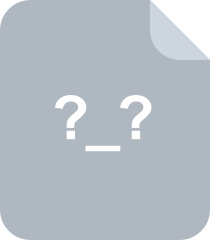
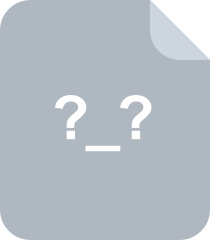
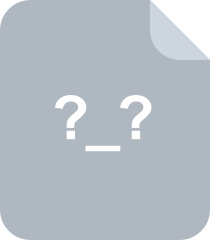
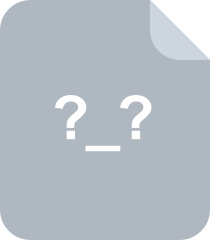
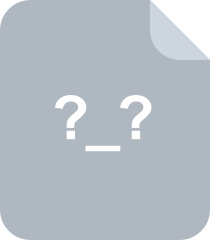
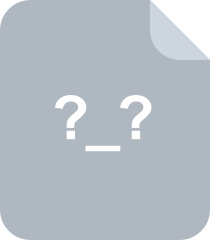
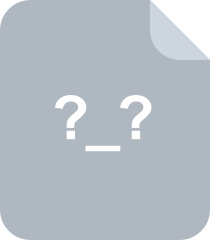
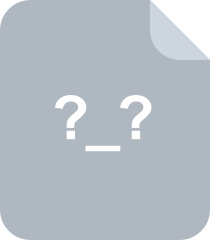
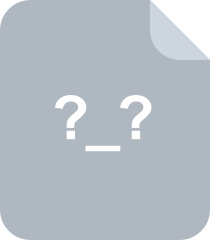
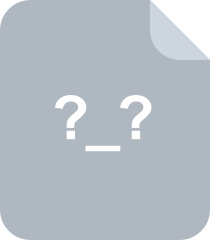
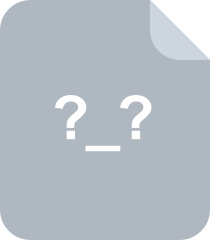
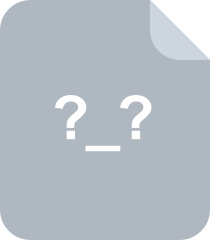
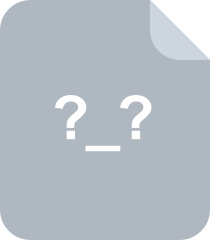
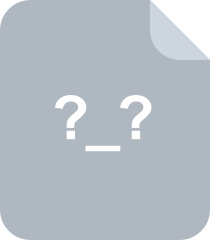
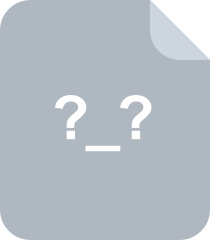
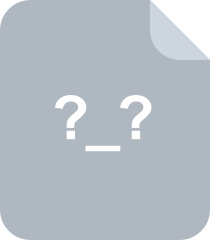
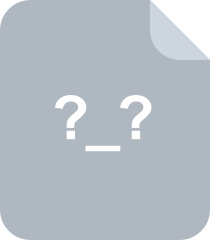
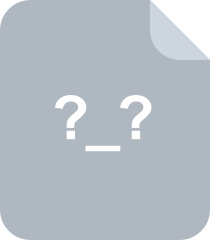
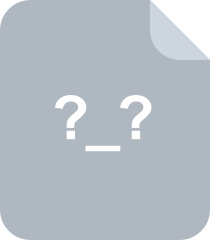
共 140 条
- 1
- 2
资源评论
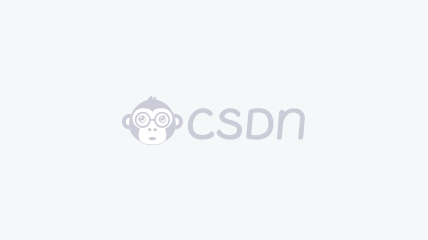
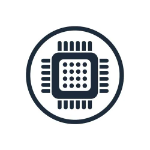
电子类产品开发
- 粉丝: 3191
- 资源: 891
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

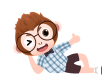
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


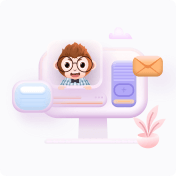
安全验证
文档复制为VIP权益,开通VIP直接复制
