### Java SpringWeb 接收安卓Android传来的图片集合及其他信息入库存储 #### 背景与需求 在现代移动应用开发中,Android客户端经常需要与后端服务器进行交互,尤其是上传图片等多媒体数据以及相关的元数据(如描述、位置等)。Spring Web是Java后端开发中的一个流行框架,它提供了强大的功能来处理HTTP请求和响应,非常适合搭建RESTful服务。本文将详细介绍如何使用Java Spring Web框架接收来自Android客户端的图片集合及其他相关信息,并将其存储到数据库中。 #### 技术栈 - **前端**:Android客户端(Java/Kotlin) - **后端**:Java + Spring Boot/Spring Web - **数据库**:MySQL/PostgreSQL #### 实现步骤 ### 一、Spring Web后端实现 #### 1. 创建Spring Boot项目 首先使用Spring Initializr创建一个新的Spring Boot项目,并添加`Spring Web`和`Thymeleaf`(用于简单的前端展示)依赖。 #### 2. 配置文件 编辑`application.properties`或`application.yml`文件,配置数据库连接和其他相关属性: ```properties spring.datasource.url=jdbc:mysql://localhost:3306/mydb?useUnicode=true&characterEncoding=utf8 spring.datasource.username=root spring.datasource.password=123456 spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver ``` #### 3. 设计实体类 定义一个实体类来表示需要存储的信息,例如`ImageInfo`: ```java import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; @Entity public class ImageInfo { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String imageUrl; private String description; // 其他字段... // Getter & Setter } ``` #### 4. 创建Repository接口 利用Spring Data JPA提供的功能简化数据库操作: ```java import org.springframework.data.jpa.repository.JpaRepository; public interface ImageInfoRepository extends JpaRepository<ImageInfo, Long> { } ``` #### 5. 控制器实现 创建一个控制器类来处理HTTP请求: ```java import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.RestController; import org.springframework.web.multipart.MultipartFile; import java.io.File; import java.io.IOException; import java.util.List; @RestController @RequestMapping("/api/images") public class ImageController { @Autowired private ImageInfoRepository repository; @PostMapping public void uploadImages(@RequestParam("files") List<MultipartFile> files, @RequestParam("description") String description) throws IOException { for (MultipartFile file : files) { String fileName = file.getOriginalFilename(); if (fileName != null && !fileName.isEmpty()) { File outputFile = new File("/path/to/save/" + fileName); file.transferTo(outputFile); ImageInfo imageInfo = new ImageInfo(); imageInfo.setImageUrl(fileName); imageInfo.setDescription(description); repository.save(imageInfo); } } } } ``` ### 二、Android客户端实现 #### 1. 添加依赖 在Android项目的`build.gradle`文件中添加必要的依赖,如OkHttp库: ```groovy dependencies { implementation 'com.squareup.okhttp3:okhttp:4.9.0' } ``` #### 2. 发送请求 编写代码来发送POST请求,携带图片文件和额外的信息到服务器: ```java import okhttp3.MediaType; import okhttp3.MultipartBody; import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.RequestBody; import java.io.File; import java.io.IOException; import java.util.ArrayList; import java.util.List; public class ImageUploader { private static final MediaType MEDIA_TYPE_PNG = MediaType.parse("image/png"); public static void uploadImages(List<File> images, String description) throws IOException { OkHttpClient client = new OkHttpClient(); for (File image : images) { RequestBody requestBody = new MultipartBody.Builder() .setType(MultipartBody.FORM) .addFormDataPart("files", image.getName(), RequestBody.create(MEDIA_TYPE_PNG, image)) .addFormDataPart("description", description) .build(); Request request = new Request.Builder() .url("http://yourserver.com/api/images") .post(requestBody) .build(); Response response = client.newCall(request).execute(); System.out.println(response.body().string()); } } } ``` ### 三、总结 通过上述步骤,我们成功地实现了使用Java Spring Web框架接收来自Android客户端的图片集合及其他相关信息,并将其存储到数据库中的功能。这个过程涉及到了前后端的紧密协作,包括但不限于文件上传、数据库操作以及RESTful API的设计与实现。此外,为了确保系统的健壮性和安全性,还可以进一步考虑错误处理机制、输入验证等方面的工作。
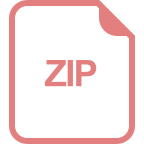
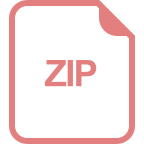
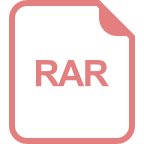
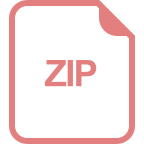
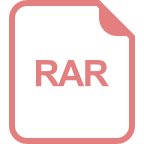
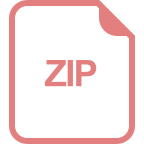
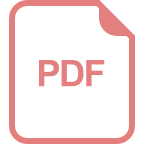
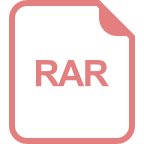
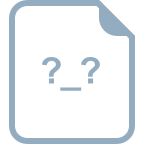
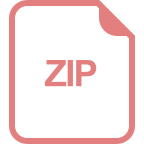
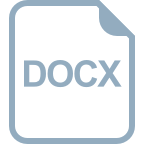
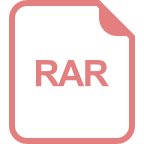
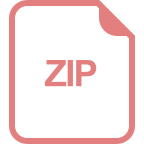
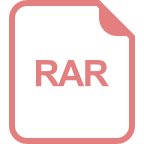
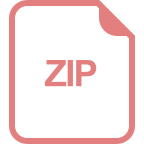
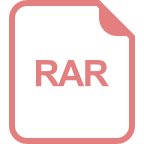
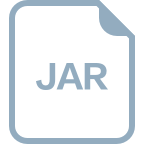
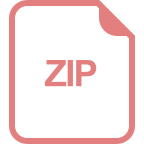
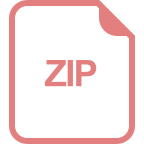
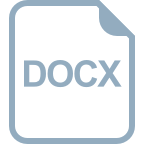
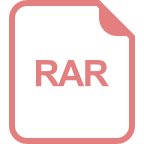
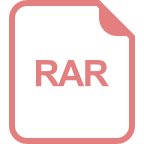
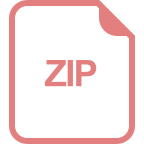
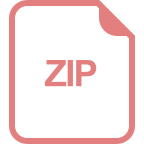
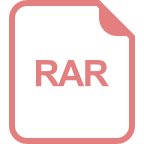
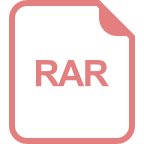
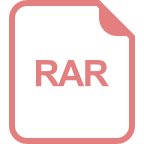
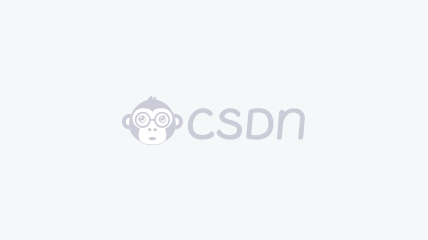

- 粉丝: 16
- 资源: 200
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

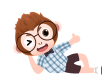
最新资源
- flinksql专用资源,各种jar包
- CLShanYanSDKDataList.sqlite
- C#ASP.NET销售管理系统源码数据库 SQL2008源码类型 WebForm
- 1111232132132132
- 基于MAPPO算法与DL优化预编码的多用户MISO通信系统双时间尺度传输方案设计源码
- 基于微信拍照功能的ohos开源CameraView控件设计源码
- 基于JavaCV的RTSP转HTTP-FLV流媒体服务设计源码
- 基于Python的西北工业大学MobilePhone软件开发项目设计源码
- 基于Java语言实现的LeetCode-hot100题库精选设计源码
- 基于ThinkPHP5.0的壹凯巴cms设计源码,适用于小型企业建站灵活组装开发

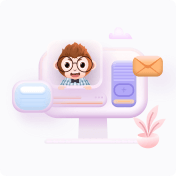
