在Android开发中,自定义视图是提升用户体验和界面独特性的重要手段。本文将深入探讨如何在Android中实现一个动态的、自定义的圆形进度条。这个圆形进度条不仅可以显示进度,还可以根据需要进行样式调整,如颜色、宽度、字体大小等。 我们来看如何在代码中绘制这个圆形进度条。主要涉及以下几个步骤: 1. **绘制圆环**:通过`Canvas`的`drawCircle`方法来绘制圆环。确定圆心坐标(circleX, circleY)和半径(radius),然后设置画笔(paint)的颜色、线宽和样式(STROKE)。例如: ```java float circleX = width / 2; float circleY = width / 2; float radius = width / 2 - roundWidth / 2; paint.setColor(roundColor); paint.setStrokeWidth(roundWidth); paint.setStyle(Paint.Style.STROKE); canvas.drawCircle(circleX, circleY, radius, paint); ``` 2. **绘制圆弧**:利用`drawArc`方法来绘制代表进度的圆弧。创建一个`RectF`对象表示圆环的边界,并设定圆弧的起始角度(0)和结束角度(progress * 360 / max)。注意这里的`false`参数表示不填充圆弧内部。 ```java RectF oval = new RectF(roundWidth/2,roundWidth/2,width-roundWidth/2,width - roundWidth/2); paint.setColor(roundProgressColor); canvas.drawArc(oval, 0, progress * 360 / max, false, paint); ``` 3. **绘制文本**:显示进度百分比。计算文本大小、颜色,使用`drawText`方法在适当的位置绘制文本。 ```java paint.setTextSize(textSize); paint.setColor(textColor); paint.setStrokeWidth(0); String text = progress * 100 / max + "%"; Rect bounds = new Rect(); paint.getTextBounds(text, 0, text.length(), bounds); canvas.drawText(text, width / 2 - bounds.width() / 2, width / 2 + bounds.height() / 2, paint); ``` 接下来,我们讨论如何自定义属性以实现更多样化的外观: 1. **定义属性**:在`res/values`目录下创建`attrs.xml`文件,声明需要的自定义属性。比如: ```xml <declare-styleable name="RoundProgress"> <attr name="roundColor" format="color"/> <attr name="roundProgressColor" format="color"/> <attr name="textColor" format="color"/> <attr name="roundWidth" format="dimension"/> <attr name="textSize" format="dimension"/> </declare-styleable> ``` 2. **引用命名空间**:在布局文件中添加自定义视图的命名空间,以便能够使用自定义属性。 ```xml xmlns:atguigu="http://schemas.android.com/apk/res-auto" ``` 3. **使用自定义属性**:在自定义视图标签中设置属性值。 ```xml <com.atguigu.p2p.util.RoundProgress android:id="@+id/rp_home_progress" android:layout_width="120dp" android:layout_height="120dp" android:layout_gravity="center_horizontal" android:layout_marginTop="20dp" atguigu:roundColor="@android:color/darker_gray" atguigu:roundProgressColor="@android:color/holo_red_dark" atguigu:textColor="@color/text_progress" atguigu:roundWidth="10dp" atguigu:textSize="20sp" /> ``` 4. **获取属性值**:在自定义视图的构造函数中,使用`Context`的`obtainStyledAttributes`方法获取属性值,并用`TypedArray`来读取这些值。 ```java TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.RoundProgress); roundColor = typedArray.getColor(R.styleable.RoundProgress_roundColor, Color.RED); roundProgressColor = typedArray.getColor(R.styleable.RoundProgress_roundProgressColor, Color.BLUE); textColor = typedArray.getColor(R.styleable.RoundProgress_textColor, Color.BLACK); roundWidth = typedArray.getDimensionPixelSize(R.styleable.RoundProgress_roundWidth, 10); textSize = typedArray.getDimensionPixelSize(R.styleable.RoundProgress_textSize, 20); typedArray.recycle(); ``` 通过以上步骤,我们可以创建一个具备动态更新和自定义外观的圆形进度条。用户可以根据需要更改颜色、宽度、字体大小等属性,以适应不同场景的需求。同时,这种自定义视图的方法也适用于其他类型的图形组件,为Android应用提供了无限的界面设计可能性。









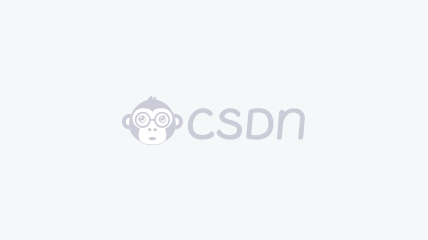

- 粉丝: 7
- 资源: 909
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

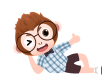
最新资源
- python进入文件夹的操作.txt
- python切片的复杂操作.txt
- python识别图片操作鼠标.txt
- python实现文件搜索工具.txt
- python数字图像常用操作.txt
- python使用pillow操作图片.txt
- python搜索sql文件.txt
- python搜索docx文件内容.txt
- python搜索fbd文件的内容.txt
- python搜索大文件文本.txt
- python搜索文件夹内容.txt
- python搜索文件是否存在.txt
- python搜索文件中的内容.txt
- python图片的平移操作.txt
- python图像基础操作.txt
- python图像卷积等操作.txt

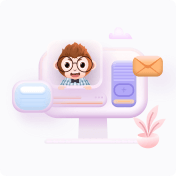
