JavaScript是一种基于原型的动态类型语言,它没有像Java或C++那样的传统类继承机制,而是通过其他方式实现了面向对象的继承。在JavaScript中,有多种实现面向对象继承的方法,包括对象冒充、`call`/`apply`方法、原型链、`Object.create()`以及ES6中的`class`语法。下面我们将详细探讨这五种方法。 1. **对象冒充(Simulated Prototypal Inheritance)** 对象冒充是通过将父类的构造函数作为子类的一个方法,并在子类构造函数中调用来实现继承。这种方法可以实现多继承,但可能会导致父类构造函数被多次调用,增加内存开销。 ```javascript function Parent(firstname) { this.fname = firstname; this.age = 40; this.sayAge = function() { console.log(this.age); }; } function Child(firstname) { this.parent = Parent; this.parent(firstname); delete this.parent; this.saySomeThing = function() { console.log(this.fname); this.sayAge(); }; } ``` 2. **`call`/`apply`方法改变函数上下文实现继承** `call`和`apply`方法可以改变函数的执行上下文,使得父类的构造函数能够以子类的实例为上下文执行。这种方式不能直接继承原型链,如果需要继承原型链,可以配合其他方式(如5的混合模式)。使用`call`的例子如下: ```javascript function Parent(firstname) { this.fname = firstname; this.age = 40; this.sayAge = function() { console.log(this.age); }; } function Child(firstname) { this.saySomeThing = function() { console.log(this.fname); this.sayAge(); }; this.getName = function() { return firstname; }; } var child = new Child("张"); Parent.call(child, child.getName()); child.saySomeThing(); ``` 3. **`apply`方法改变函数上下文实现继承** 类似于`call`,`apply`也是改变函数上下文,但允许传入一个数组或类数组作为参数,适用于参数列表不确定的情况。 ```javascript function Parent(firstname) { this.fname = firstname; this.age = 40; this.sayAge = function() { console.log(this.age); }; } function Child(firstname) { this.saySomeThing = function() { console.log(this.fname); this.sayAge(); }; this.getName = function() { return firstname; }; } var child = new Child("张"); Parent.apply(child, [child.getName()]); child.saySomeThing(); ``` 4. **原型链(Prototype Chain)方式实现继承** 原型链是最常见的继承方式,通过修改子类的`prototype`使其指向父类的实例,这样子类就可以访问父类的属性和方法。缺点是无法直接实现多继承。 ```javascript function Parent() { this.sayAge = function() { console.log(this.age); }; } function Child(firstname) { this.fname = firstname; this.age = 40; this.saySomeThing = function() { console.log(this.fname); this.sayAge(); }; } Child.prototype = new Parent(); var child = new Child(); ``` 5. **`Object.create()`方法实现继承** `Object.create()`方法创建一个新的对象,并将该对象的原型设置为传入的对象,从而实现继承。这种方式同样只支持单继承。 ```javascript function Parent(firstname) { this.fname = firstname; this.age = 40; this.sayAge = function() { console.log(this.age); }; } function Child(firstname) { this.saySomeThing = function() { console.log(this.fname); this.sayAge(); }; } Child.prototype = Object.create(Parent.prototype); Child.prototype.constructor = Child; var child = new Child(); ``` 6. **ES6的`class`语法实现继承(混合模式)** ES6引入了`class`语法,虽然其语法糖底层还是基于原型链,但提供了更简洁的继承方式,可以结合`super`关键字实现多继承。 ```javascript class Parent { constructor(firstname) { this.fname = firstname; this.age = 40; } sayAge() { console.log(this.age); } } class Child extends Parent { constructor(firstname) { super(firstname); this.age = 40; } saySomeThing() { console.log(this.fname); this.sayAge(); } } const child = new Child("李"); child.saySomeThing(); ``` 每种继承方式都有其适用场景,选择哪种方式取决于项目需求、兼容性考虑以及代码可读性和维护性。在实际开发中,通常会结合使用多种方式,以实现最佳的继承效果。
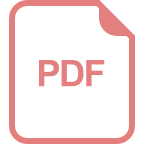
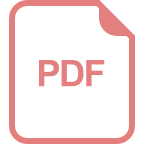
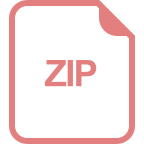
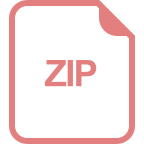
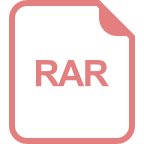
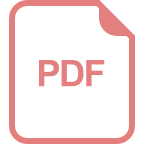
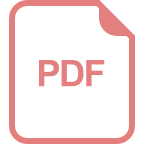
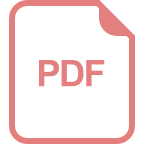
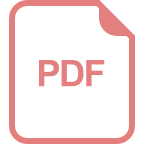
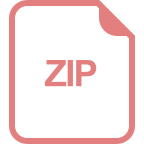
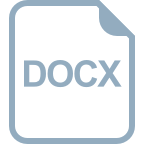
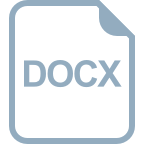
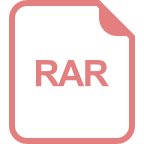
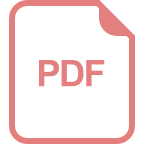
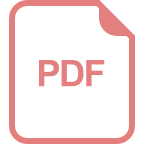
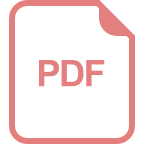
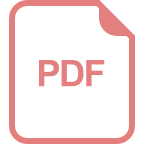
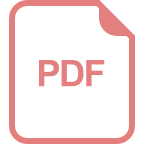
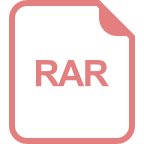
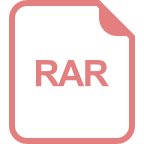
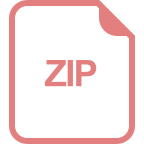
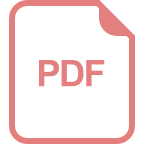
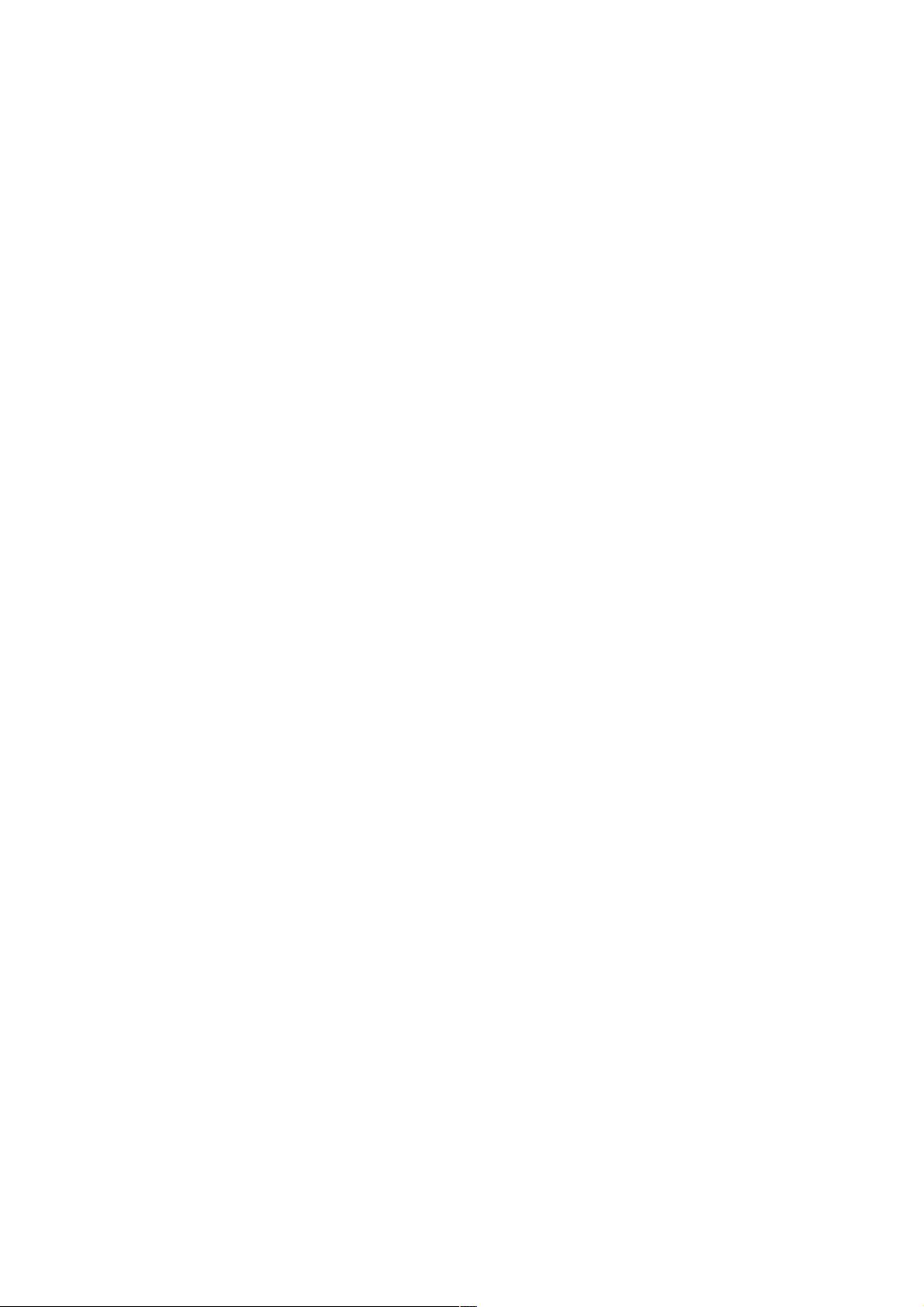
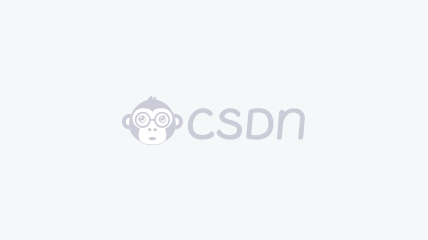

- 粉丝: 7
- 资源: 945
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

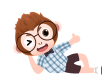
最新资源

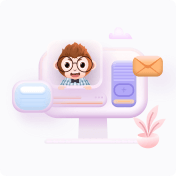
